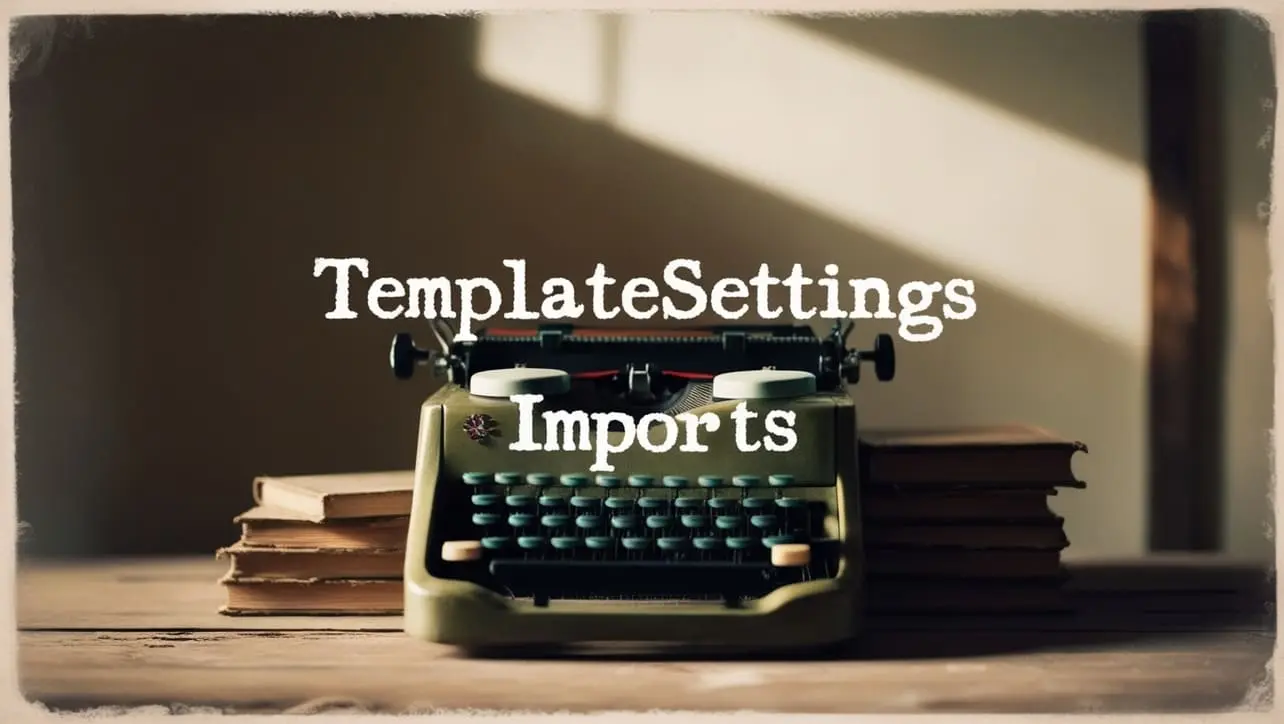
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.eq() Lang Method
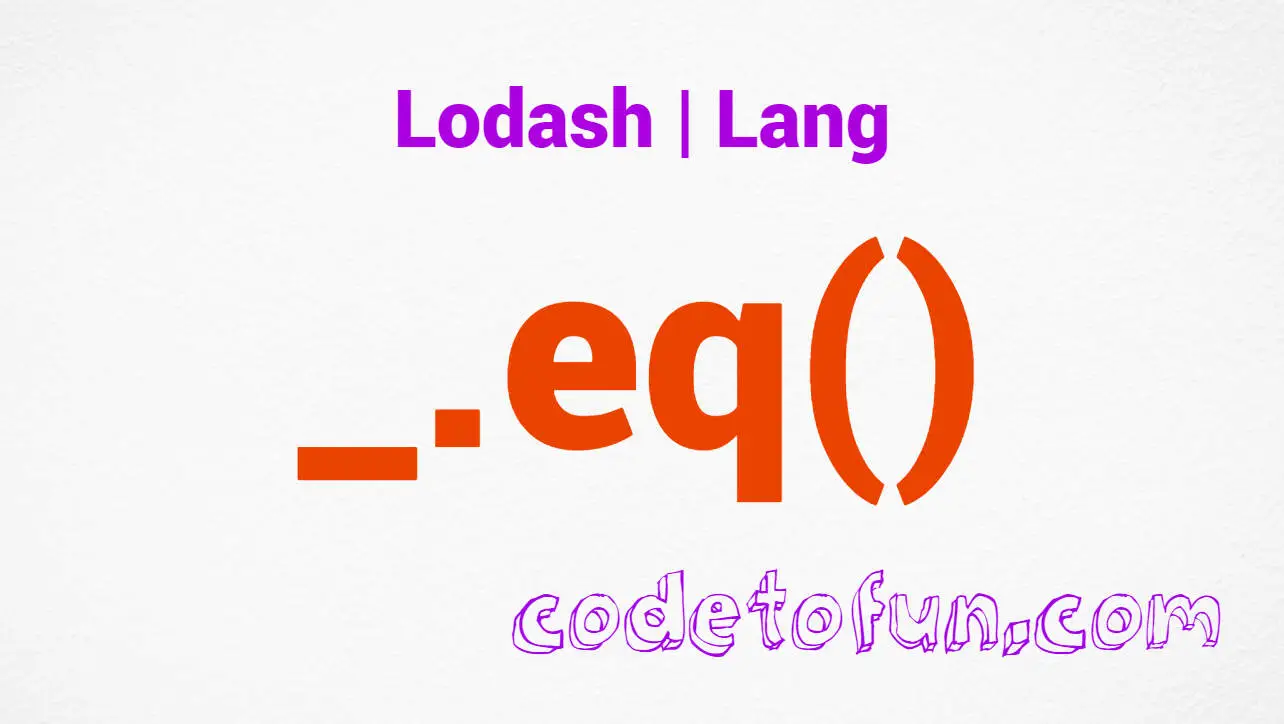
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, precise comparison of values is a fundamental aspect. Lodash, a versatile utility library, provides the _.eq()
method, a powerful tool for performing strict equality checks between two values.
This method goes beyond the capabilities of the native JavaScript === operator, offering additional features for nuanced comparison scenarios.
🧠 Understanding _.eq() Method
The _.eq()
method in Lodash is designed to perform a strict equality check between two values. While similar to the native JavaScript === operator, _.eq()
provides enhanced functionality for specific use cases, making it a valuable addition to a developer's toolkit.
💡 Syntax
The syntax for the _.eq()
method is straightforward:
_.eq(value, other)
- value: The first value to compare.
- other: The second value to compare.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.eq()
method:
const _ = require('lodash');
const value1 = 'hello';
const value2 = 'hello';
const value3 = 'world';
console.log(_.eq(value1, value2)); // Output: true
console.log(_.eq(value1, value3)); // Output: false
In this example, _.eq()
is used to compare two string values, demonstrating its ability to determine strict equality.
🏆 Best Practices
When working with the _.eq()
method, consider the following best practices:
Strict Type Comparison:
_.eq()
performs strict type comparison, similar to the === operator. Ensure that you intend to compare values of the same type, especially when dealing with variables of dynamic types.example.jsCopiedconst numberValue = 42; const stringValue = '42'; console.log(_.eq(numberValue, stringValue)); // Output: false
Handling NaN Values:
Unlike the === operator,
_.eq()
considers two NaN values as equal. This behavior can be useful when dealing with scenarios involving NaN.example.jsCopiedconst nanValue1 = NaN; const nanValue2 = NaN; console.log(_.eq(nanValue1, nanValue2)); // Output: true
Arrays and Objects:
When comparing arrays or objects,
_.eq()
performs a deep equality check, ensuring that the contents are identical.example.jsCopiedconst array1 = [1, 2, 3]; const array2 = [1, 2, 3]; console.log(_.eq(array1, array2)); // Output: true
📚 Use Cases
Handling Type-Sensitive Scenarios:
In scenarios where strict type comparison is crucial, such as when validating user input or configuration settings,
_.eq()
ensures that values are not only equal but also of the same type.example.jsCopiedconst expectedType = 'number'; const userInput = '42'; if (_.eq(typeof userInput, expectedType)) { console.log('User input is a number.'); } else { console.log('User input is not a number.'); }
Dealing with NaN Values:
When dealing with calculations that may result in NaN values,
_.eq()
can simplify checks for NaN equality.example.jsCopiedconst result = Math.sqrt(-1); if (_.eq(result, NaN)) { console.log('Result is NaN.'); } else { console.log('Result is not NaN.'); }
Deep Equality Checks:
For arrays or objects where a deep comparison is required,
_.eq()
ensures that all elements or properties are strictly equal.example.jsCopiedconst object1 = { a: 1, b: { c: 2 } }; const object2 = { a: 1, b: { c: 2 } }; const object3 = { a: 1, b: { c: 3 } }; console.log(_.eq(object1, object2)); // Output: true console.log(_.eq(object1, object3)); // Output: false
🎉 Conclusion
The _.eq()
method in Lodash provides a robust solution for performing strict equality checks in JavaScript. Whether handling type-sensitive scenarios, dealing with NaN values, or ensuring deep equality in complex data structures, _.eq()
offers a versatile and reliable tool for developers.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.eq()
method in your Lodash projects.
👨💻 Join our Community:
Author
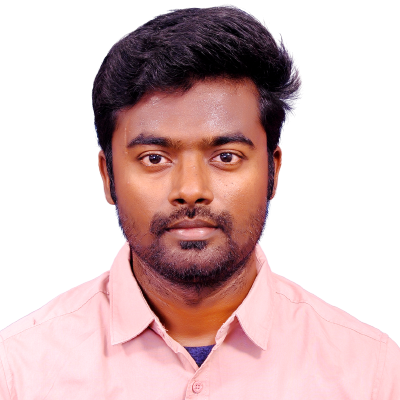
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
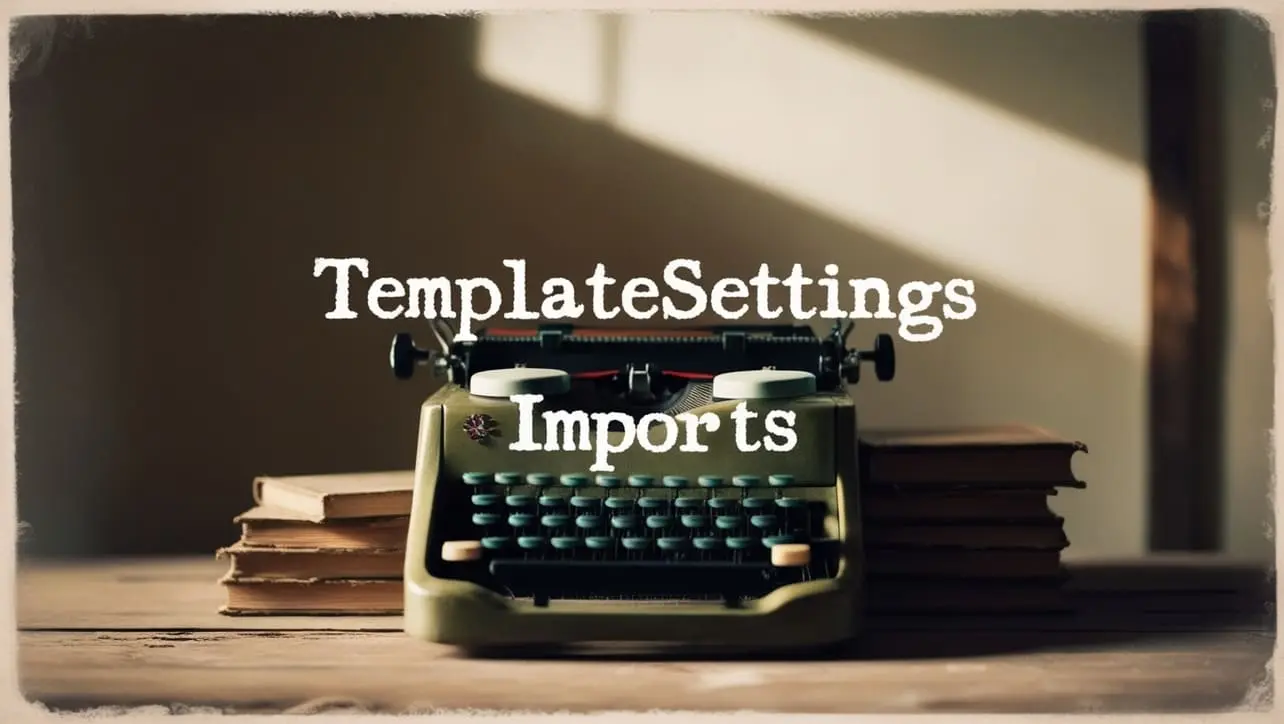
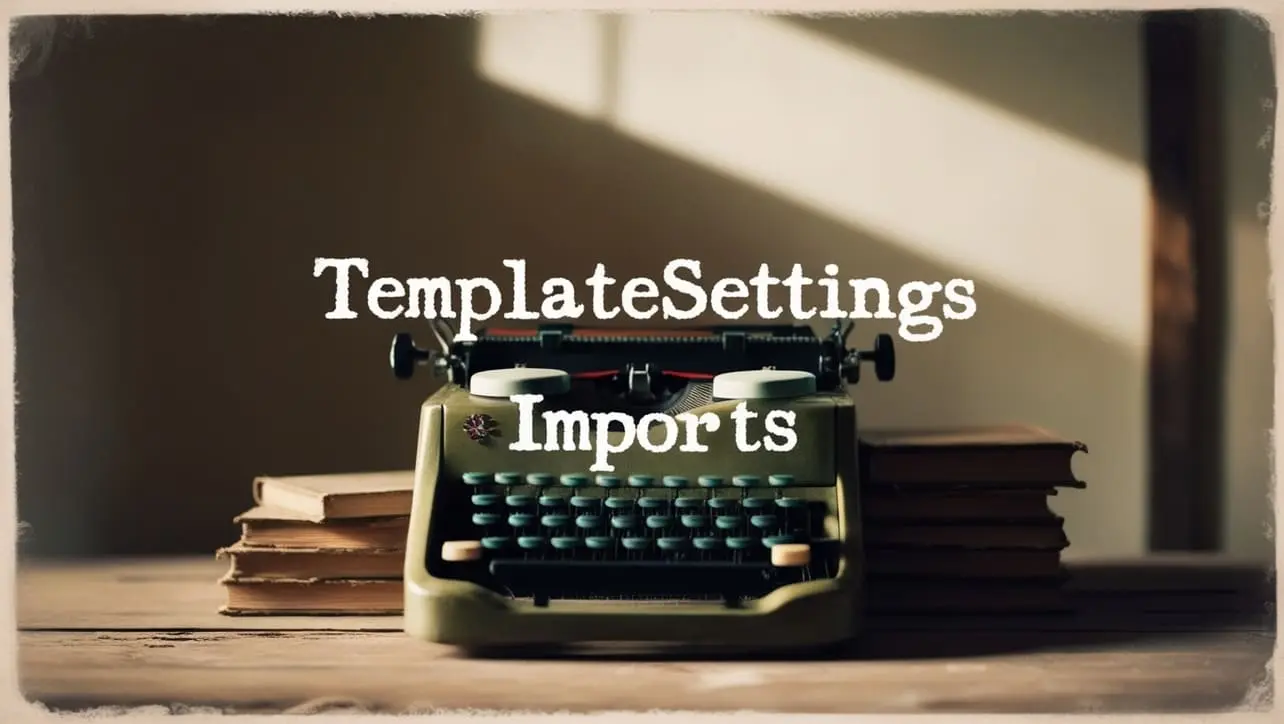
Lodash _.templateSettings.imports Property
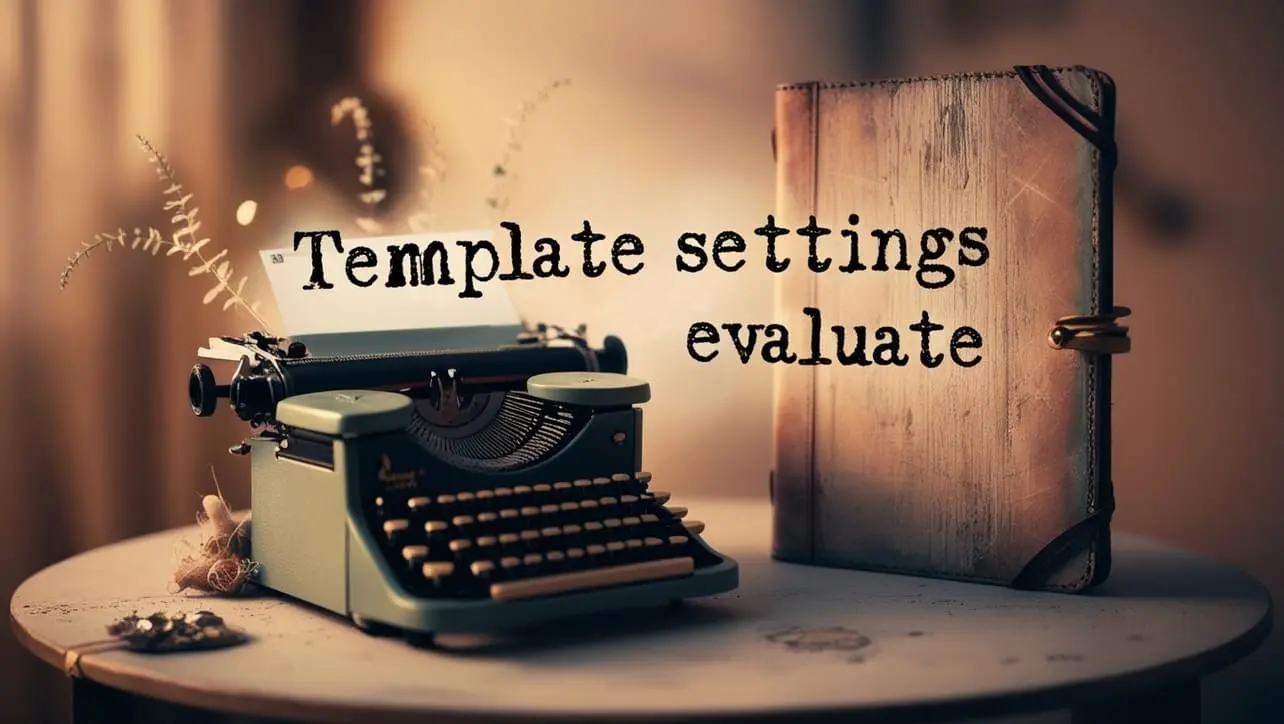
Lodash _.templateSettings.evaluate Property
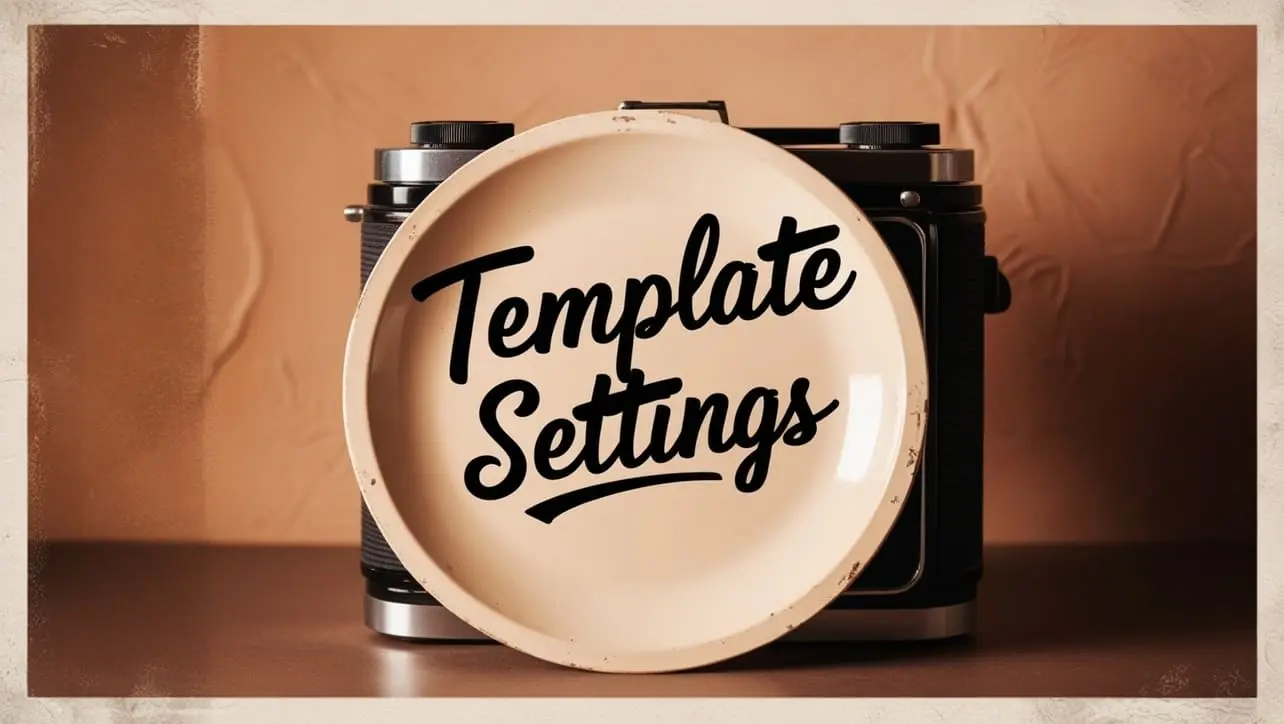
Lodash _.templateSettings Property
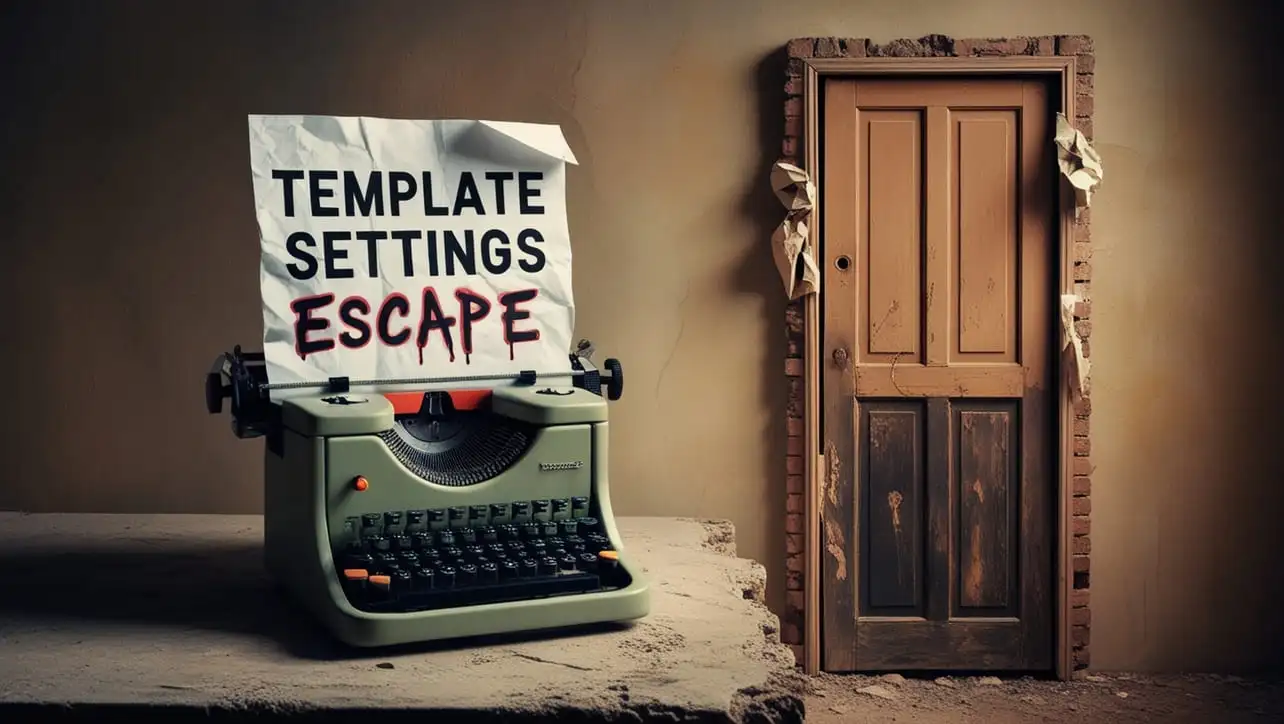
Lodash _.templateSettings.escape Property
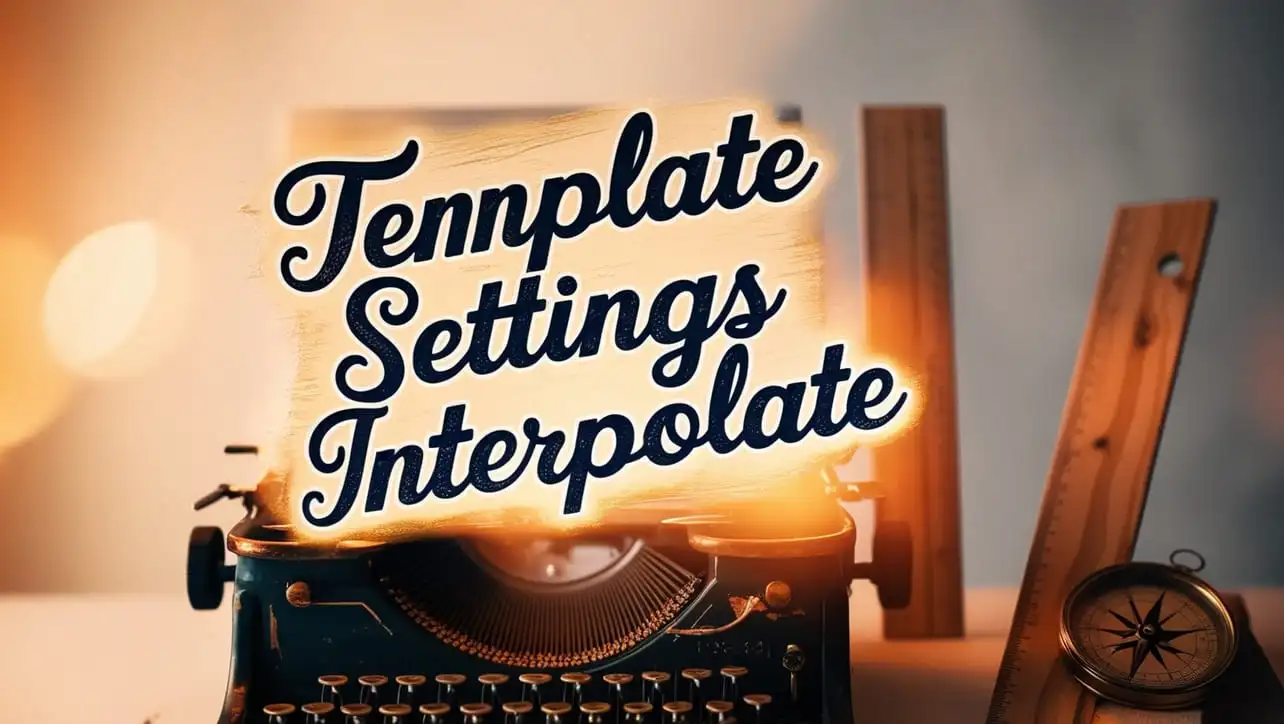
Lodash _.templateSettings.interpolate Property
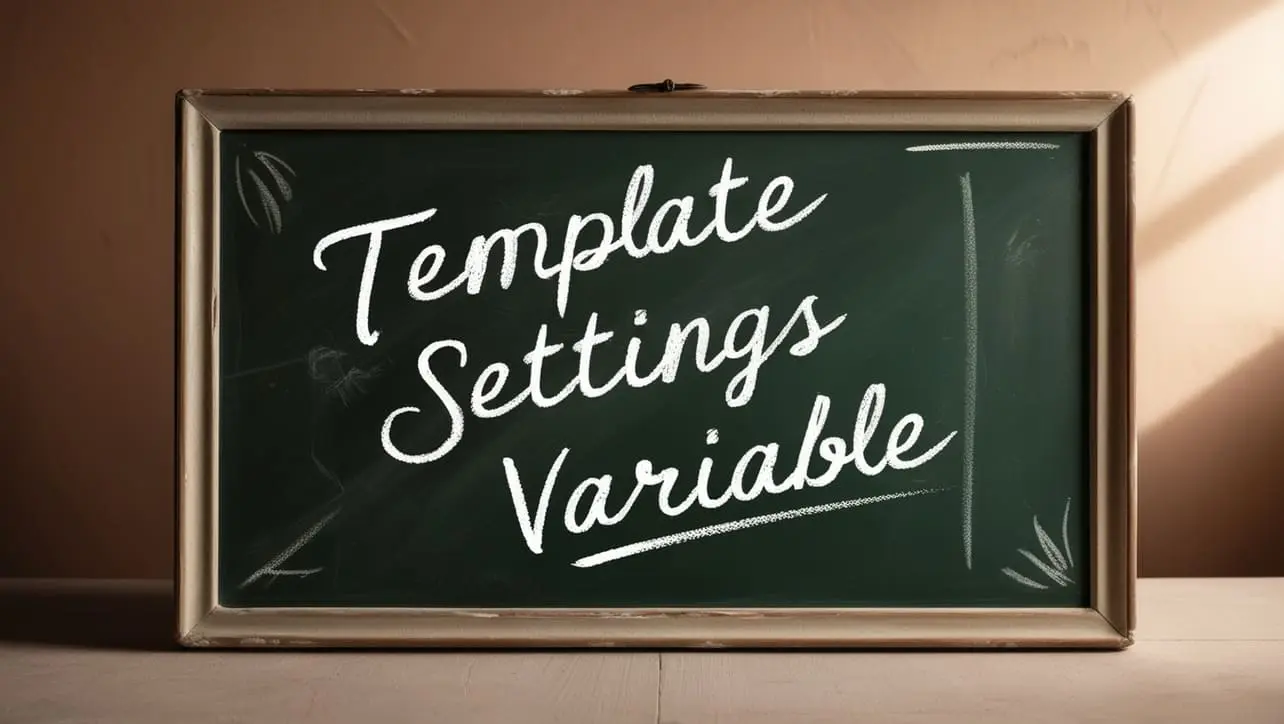
If you have any doubts regarding this article (Lodash _.eq() Lang Method), please comment here. I will help you immediately.