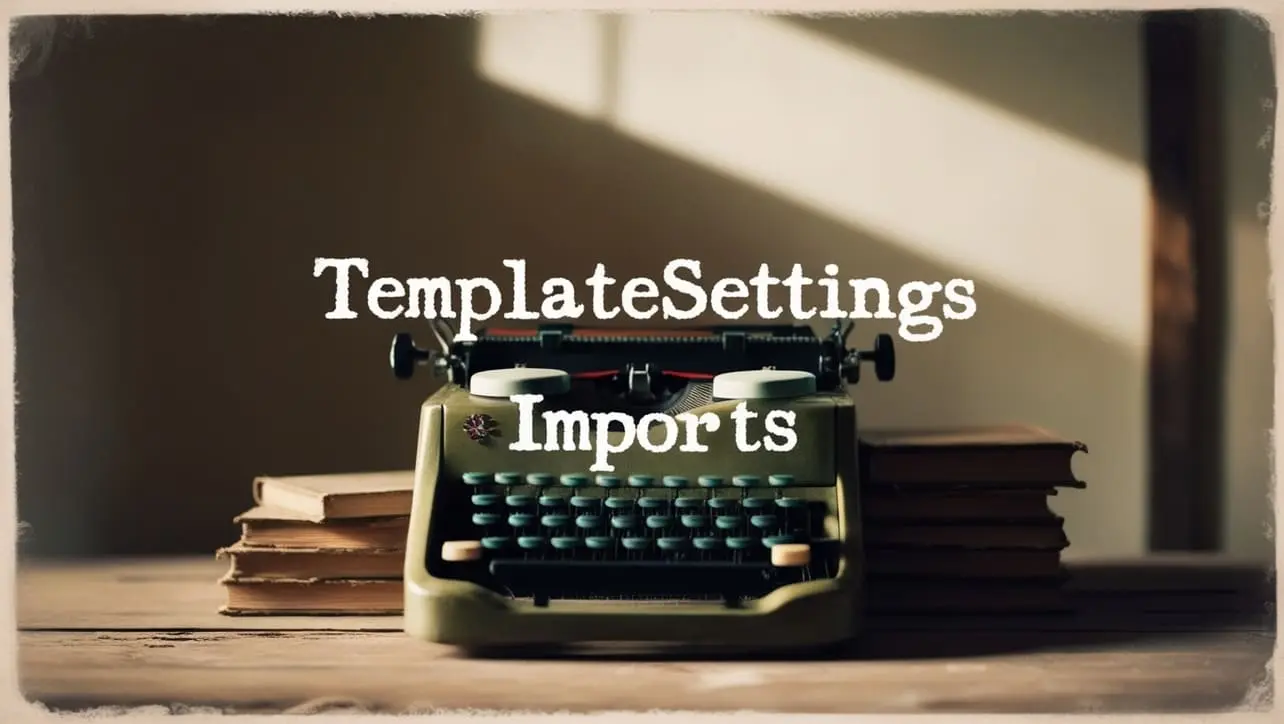
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.conformsTo() Lang Method
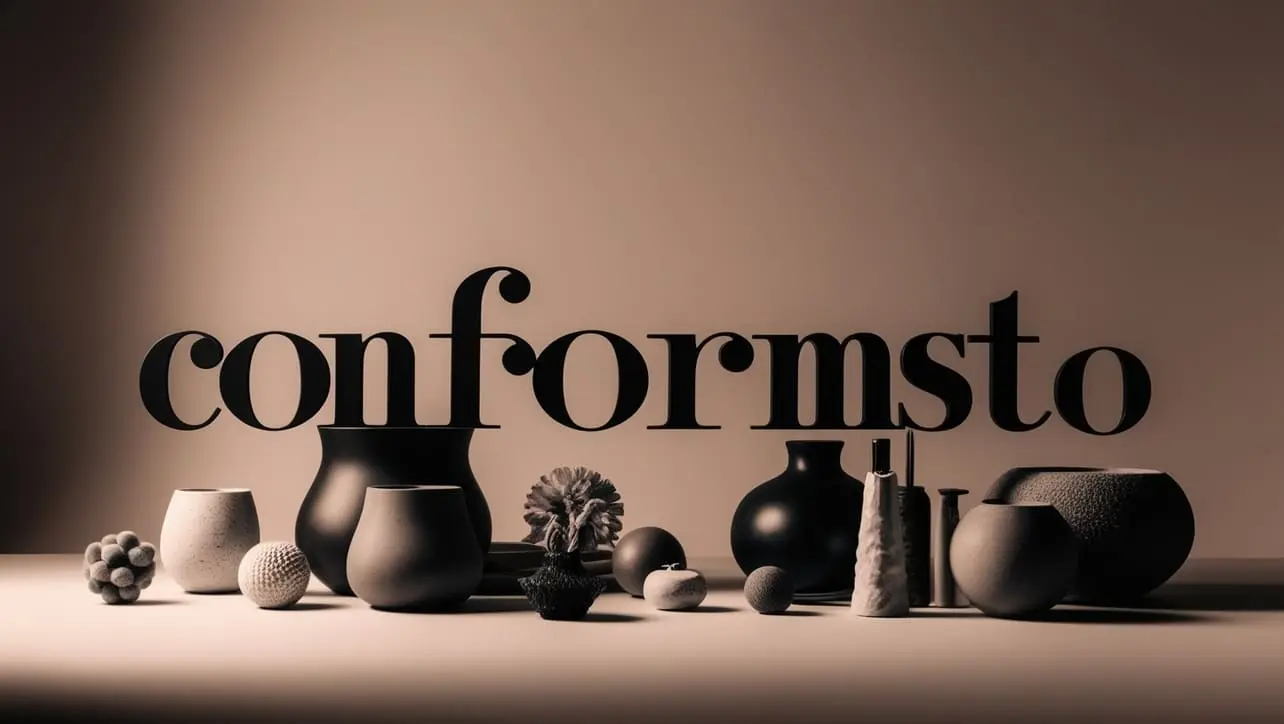
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript programming, the ability to validate objects against specific conditions is a common requirement. Lodash, a comprehensive utility library, provides the _.conformsTo()
method, offering a concise way to check if an object conforms to a specified set of conditions.
This method empowers developers to define custom validation logic, enhancing the robustness of their applications.
🧠 Understanding _.conformsTo() Method
The _.conformsTo()
method in Lodash evaluates whether an object conforms to a given set of property predicates. This allows developers to define conditions that the object must satisfy for validation. If the object meets the specified conditions, the method returns true; otherwise, it returns false.
💡 Syntax
The syntax for the _.conformsTo()
method is straightforward:
_.conformsTo(object, source)
- object: The object to check.
- source: The object of property predicates to conform to.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.conformsTo()
method:
const _ = require('lodash');
const user = {
username: 'john_doe',
age: 28,
isAdmin: false,
};
const validationConditions = {
username: val => /^[a-z_]+$/.test(val),
age: val => val >= 18 && val <= 65,
isAdmin: val => typeof val === 'boolean',
};
const isValidUser = _.conformsTo(user, validationConditions);
console.log(isValidUser);
// Output: true
In this example, the user object is validated against the specified conditions in validationConditions, and the result indicates whether the object conforms to these conditions.
🏆 Best Practices
When working with the _.conformsTo()
method, consider the following best practices:
Define Clear Validation Conditions:
Clearly define and document the validation conditions to ensure that the purpose and expectations of the validation are transparent to other developers.
example.jsCopiedconst validationConditions = { // Ensure the username contains only lowercase letters and underscores username: val => /^[a-z_]+$/.test(val), // Validate age is between 18 and 65 age: val => val >= 18 && val <= 65, // Ensure isAdmin is a boolean isAdmin: val => typeof val === 'boolean', };
Reusability of Validation Conditions:
If you have common validation conditions across different parts of your application, consider defining them in a reusable manner to avoid duplication of code.
example.jsCopiedconst commonValidationConditions = { // ... common validation conditions ... }; const userValidationConditions = { ...commonValidationConditions, // ... specific validation conditions for user ... }; const orderValidationConditions = { ...commonValidationConditions, // ... specific validation conditions for order ... };
Keep Validation Functions Simple:
Ensure that the functions used for validation are simple and focused on the specific condition they are checking. This improves readability and maintainability.
example.jsCopiedconst validationConditions = { username: val => /^[a-z_]+$/.test(val), age: val => val >= 18 && val <= 65, isAdmin: val => typeof val === 'boolean', };
📚 Use Cases
User Input Validation:
Validate user input against specific conditions to ensure it meets the required criteria before processing or storing it.
example.jsCopiedconst userInput = /* ...get user input... */ ; const userValidationConditions = { username: val => /^[a-z_]+$/.test(val), age: val => val >= 18 && val <= 65, // ... other conditions ... }; const isValidInput = _.conformsTo(userInput, userValidationConditions); if (isValidInput) { // Process or store the input } else { // Handle invalid input }
Configuration Validation:
Validate configuration objects to ensure they conform to the expected structure and contain valid values.
example.jsCopiedconst config = /* ...get configuration object... */ ; const configValidationConditions = { apiKey: val => typeof val === 'string' && val.length > 0, maxRequestsPerMinute: val => typeof val === 'number' && val > 0, // ... other conditions ... }; const isValidConfig = _.conformsTo(config, configValidationConditions); if (isValidConfig) { // Use the configuration } else { // Handle invalid configuration }
Data Integrity Checks:
Perform data integrity checks by validating objects against predefined conditions to ensure consistency and reliability.
example.jsCopiedconst dataRecord = /* ...get data record... */ ; const dataValidationConditions = { id: val => typeof val === 'number' && val > 0, timestamp: val => val instanceof Date, // ... other conditions ... }; const isDataValid = _.conformsTo(dataRecord, dataValidationConditions); if (isDataValid) { // Process the data } else { // Handle invalid data }
🎉 Conclusion
The _.conformsTo()
method in Lodash provides a versatile mechanism for validating objects against user-defined conditions. Whether you're validating user input, checking configuration settings, or ensuring data integrity, this method empowers you to implement custom validation logic with ease.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.conformsTo()
method in your Lodash projects.
👨💻 Join our Community:
Author
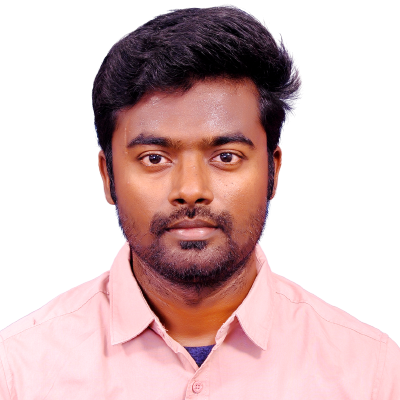
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
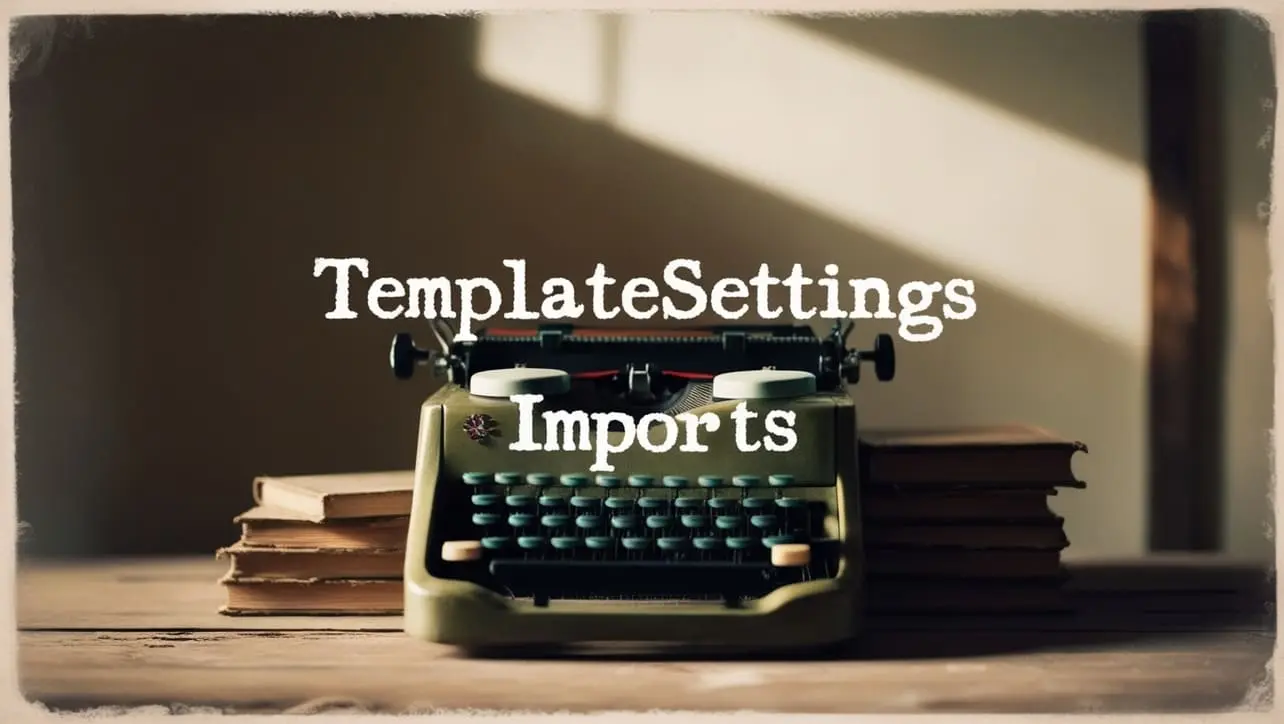
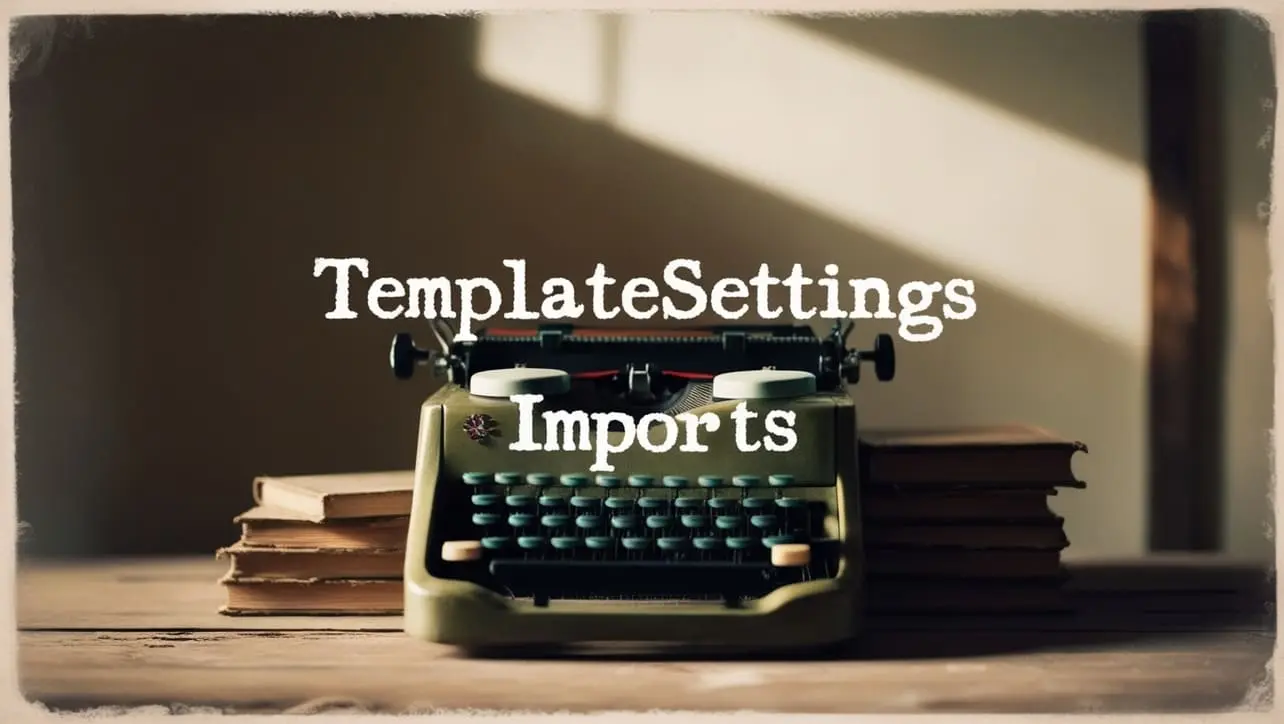
Lodash _.templateSettings.imports Property
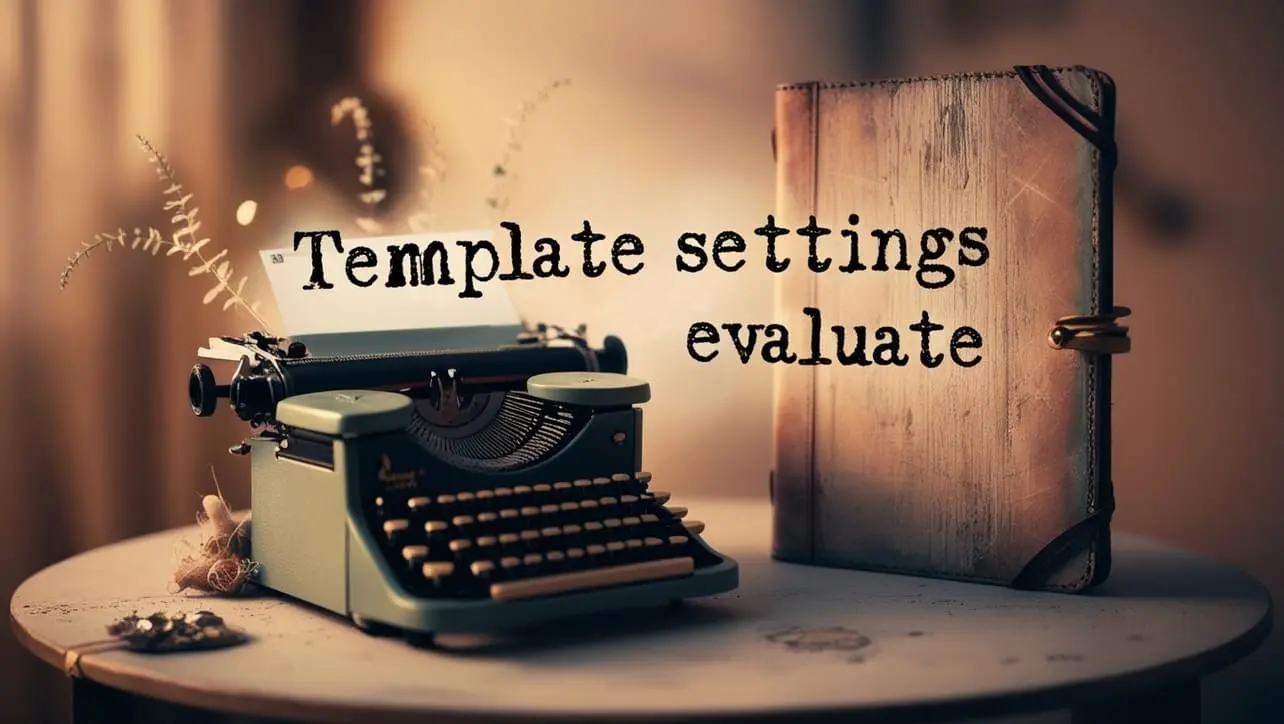
Lodash _.templateSettings.evaluate Property
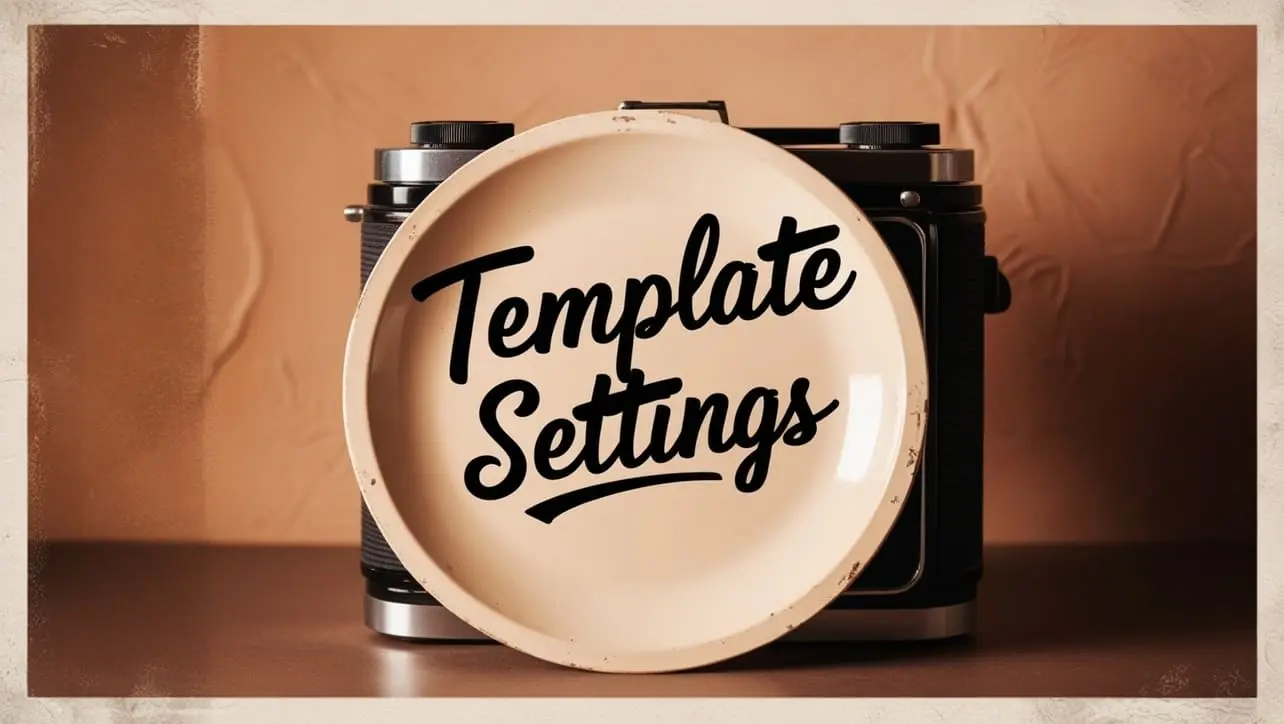
Lodash _.templateSettings Property
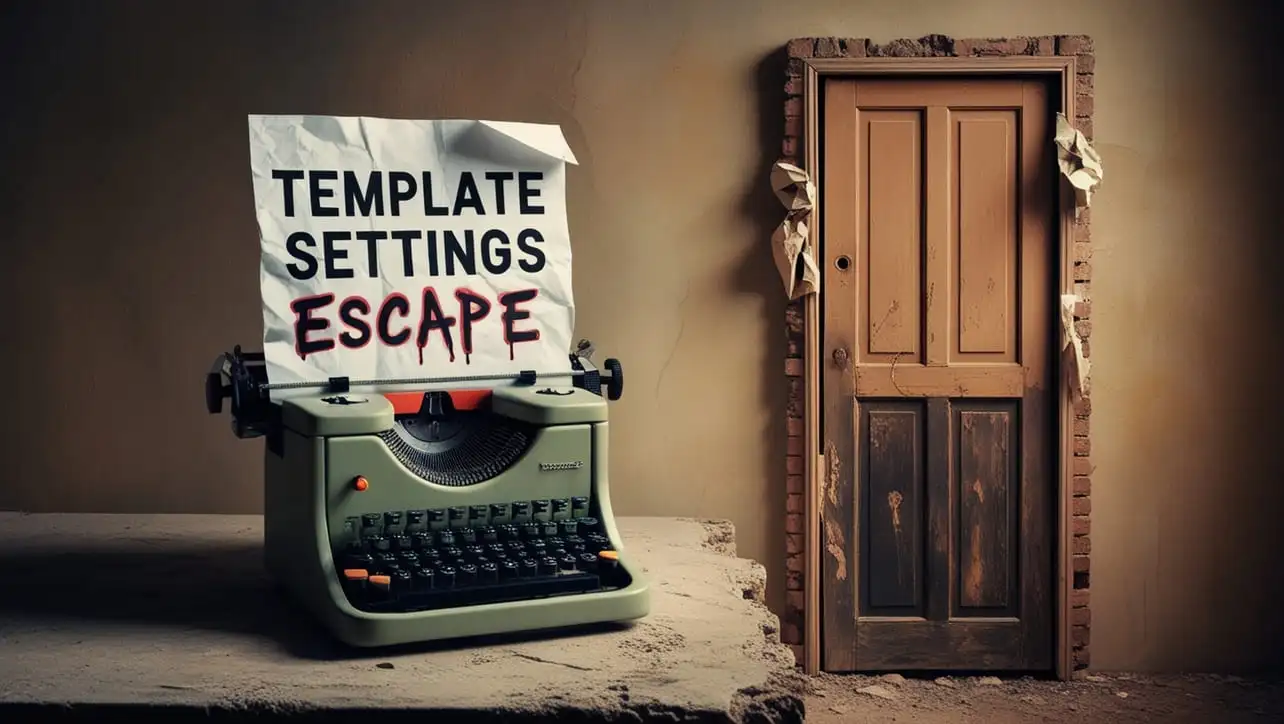
Lodash _.templateSettings.escape Property
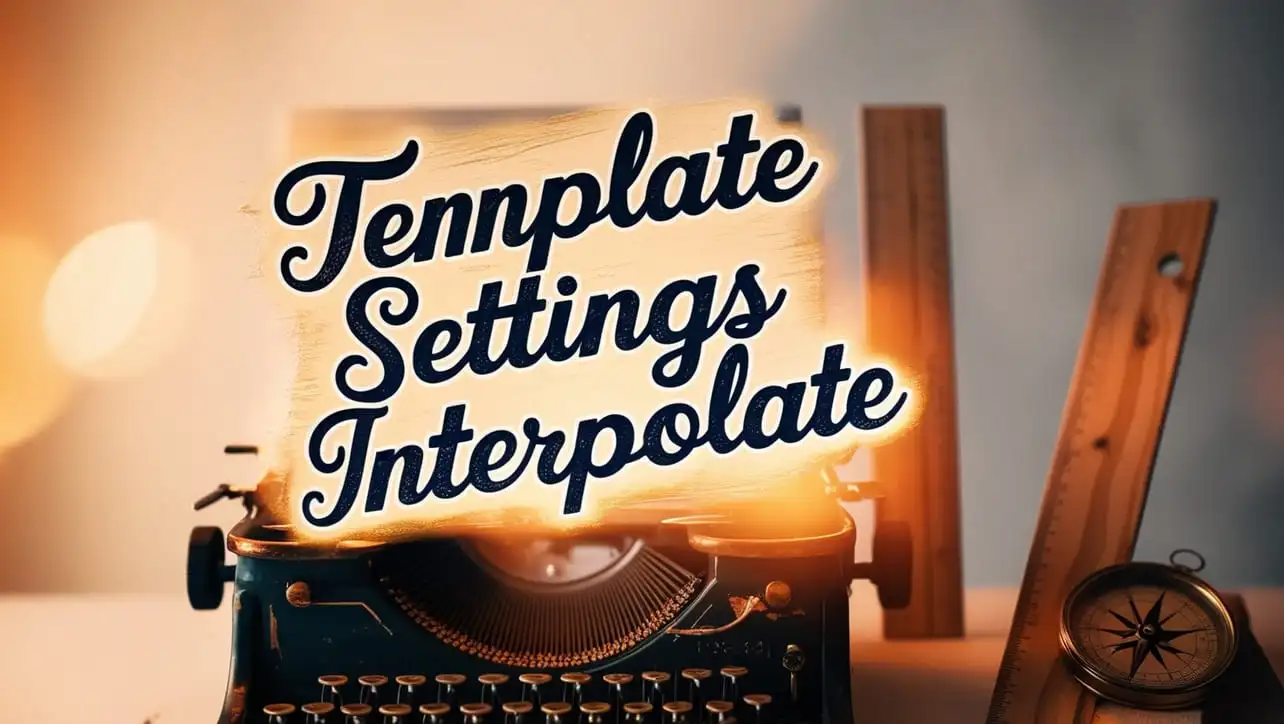
Lodash _.templateSettings.interpolate Property
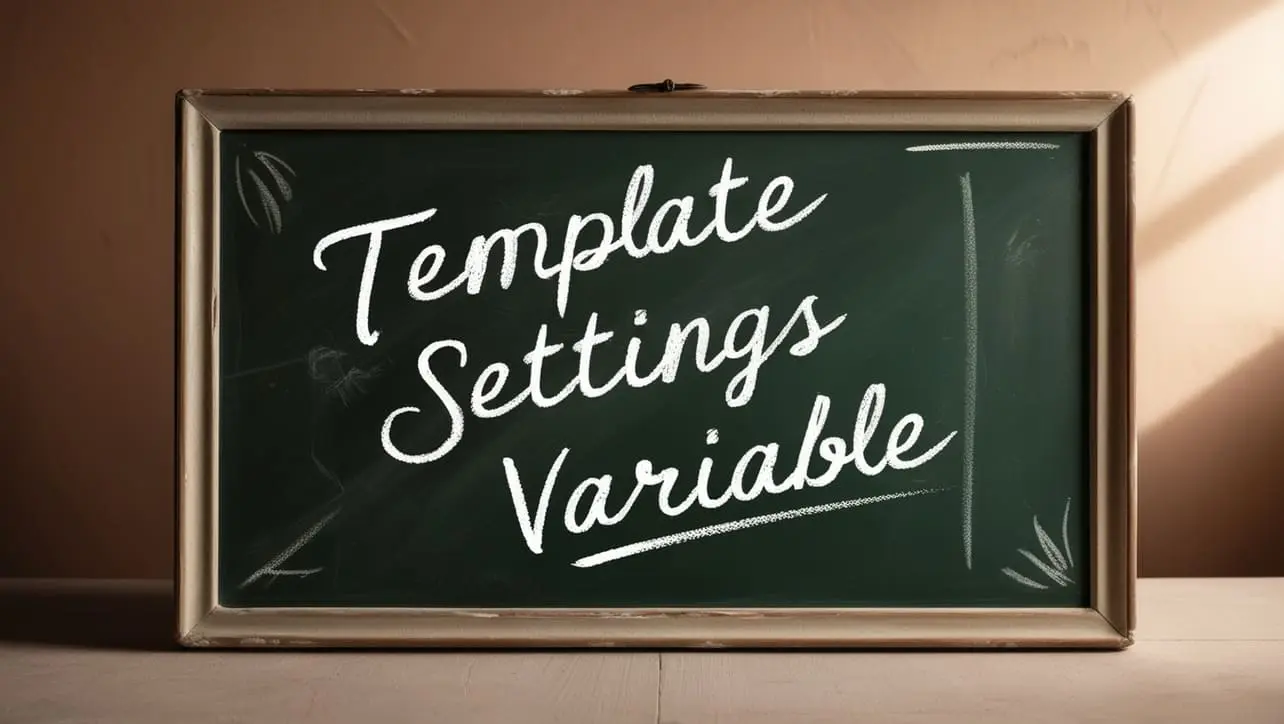
If you have any doubts regarding this article (Lodash _.conformsTo() Lang Method), please comment here. I will help you immediately.