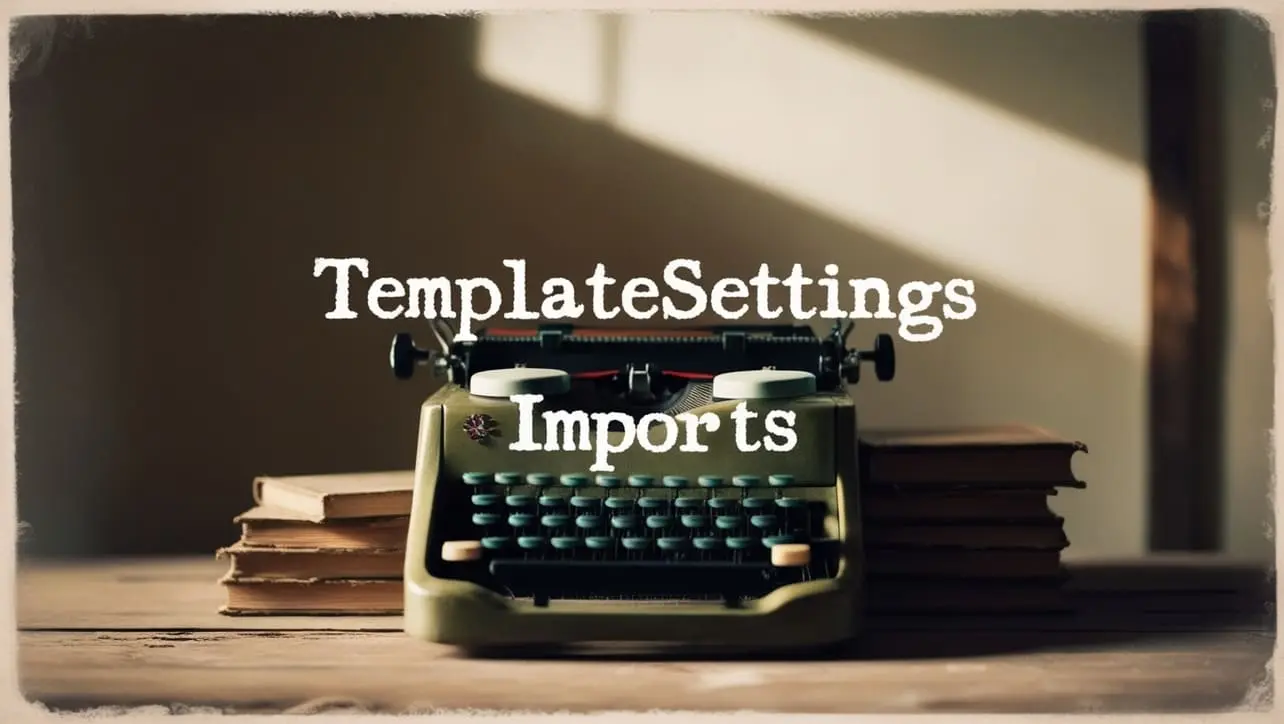
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.cloneDeep() Lang Method
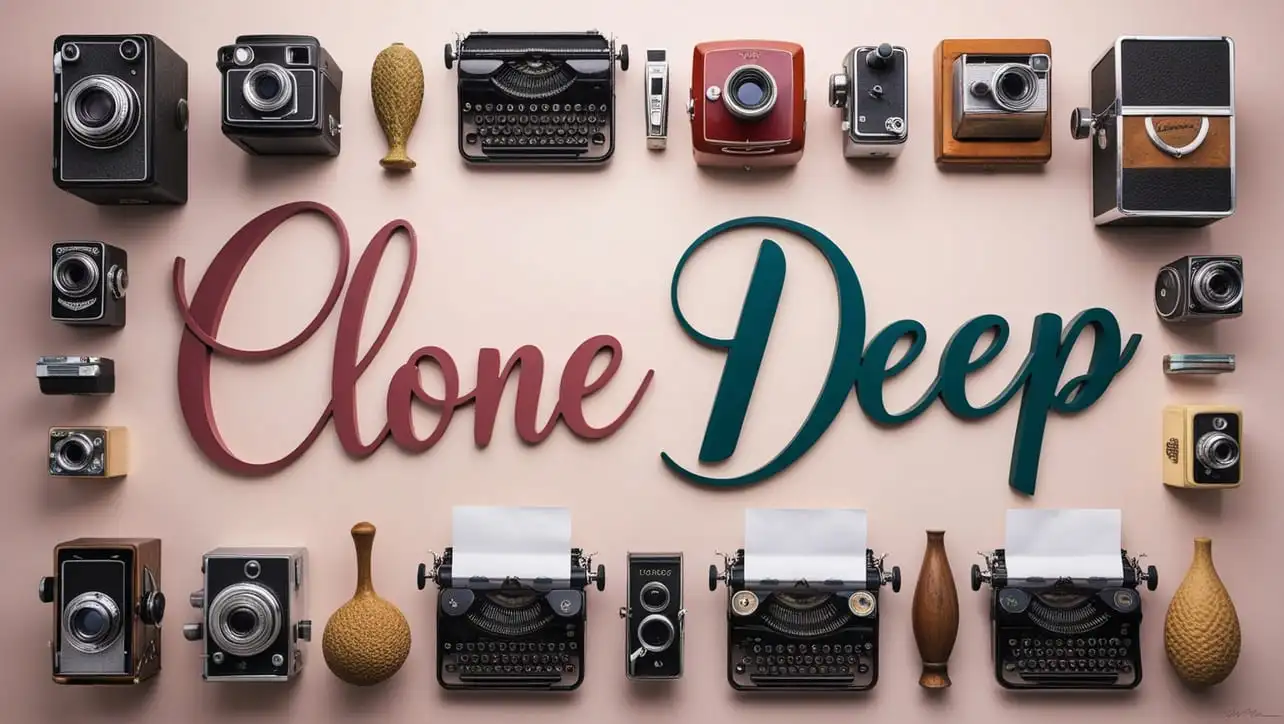
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic world of JavaScript programming, dealing with complex data structures and objects is a common challenge. Lodash, a powerful utility library, comes to the rescue with a myriad of functions, and one such lifesaver is the _.cloneDeep()
method.
This method provides a robust solution for creating deep copies of nested objects and arrays, preventing unintended side effects and ensuring data integrity.
🧠 Understanding _.cloneDeep() Method
The _.cloneDeep()
method in Lodash is designed to create a deep copy of a given object or array. Unlike a shallow copy, which only copies references to nested objects, _.cloneDeep()
recursively duplicates every level of the data structure. This is especially useful when working with complex data where changes to one object should not affect the others.
💡 Syntax
The syntax for the _.cloneDeep()
method is straightforward:
_.cloneDeep(value)
- value: The value to deep clone.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.cloneDeep()
method:
const _ = require('lodash');
const originalObject = {
key1: 'value1',
key2: {
nestedKey1: 'nestedValue1',
nestedKey2: [1, 2, 3],
},
};
const deepCopy = _.cloneDeep(originalObject);
console.log(deepCopy);
// Output: { key1: 'value1', key2: { nestedKey1: 'nestedValue1', nestedKey2: [1, 2, 3] } }
In this example, originalObject contains nested structures, and _.cloneDeep()
creates a completely independent copy.
🏆 Best Practices
When working with the _.cloneDeep()
method, consider the following best practices:
Deep Copying Nested Objects:
Ensure you use
_.cloneDeep()
when dealing with nested objects. Shallow copies might lead to unintended references, resulting in unexpected changes across objects.example.jsCopiedconst originalNestedObject = { key1: { key2: { key3: 'value' } } }; const shallowCopy = { ...originalNestedObject }; // Shallow copy shallowCopy.key1.key2.key3 = 'modifiedValue'; console.log(originalNestedObject.key1.key2.key3); // Output: 'modifiedValue' (unexpected modification with a shallow copy) const deepCopy = _.cloneDeep(originalNestedObject); // Deep copy deepCopy.key1.key2.key3 = 'newValue'; console.log(originalNestedObject.key1.key2.key3); // Output: 'modifiedValue' (no unintended modification with a deep copy)
Circular References:
_.cloneDeep()
handles circular references gracefully, making it a reliable choice when working with objects that reference themselves.example.jsCopiedconst circularObject = { key: 'value' }; circularObject.circularReference = circularObject; // Circular reference const deepCopyWithCircular = _.cloneDeep(circularObject); console.log(deepCopyWithCircular); // Output: { key: 'value', circularReference: [Circular] }
Performance Considerations:
While
_.cloneDeep()
is a powerful tool, be mindful of its performance implications, especially when working with large and deeply nested structures.example.jsCopiedconst largeNestedObject = /* ...create a large nested object... */; console.time('cloneDeep'); const deepClone = _.cloneDeep(largeNestedObject); console.timeEnd('cloneDeep'); console.log(deepClone);
📚 Use Cases
Immutable State in Redux:
When working with state management libraries like Redux, using
_.cloneDeep()
ensures that modifications to the state do not inadvertently impact previous states. This promotes a predictable and immutable state.example.jsCopiedconst currentState = /* ...get current state from Redux... */; const modifiedState = _.cloneDeep(currentState); // Make modifications to modifiedState // ... // Dispatch modifiedState to Redux store // ...
Configurations and Templates:
Deep cloning is essential when working with configuration objects or templates, where changes to one instance should not affect others.
example.jsCopiedconst defaultConfig = /* ...default configuration object... */; const userConfig = _.cloneDeep(defaultConfig); // Allow users to customize userConfig without affecting defaultConfig // ... // Use userConfig for application settings // ...
Data Transformation Pipelines:
In data transformation pipelines,
_.cloneDeep()
can be employed to create independent copies at various stages, preventing unintended interactions between processing steps.example.jsCopiedconst rawData = /* ...fetch raw data from API or elsewhere... */; const transformedData = _.cloneDeep(rawData); // Apply various transformations to transformedData // ... // Use transformedData for further processing // ...
🎉 Conclusion
The _.cloneDeep()
method in Lodash is a valuable asset for JavaScript developers, offering a reliable solution for creating deep copies of complex objects and arrays. Whether you're ensuring data immutability, handling configurations, or working with data transformation pipelines, _.cloneDeep()
provides a robust and efficient approach.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.cloneDeep()
method in your Lodash projects.
👨💻 Join our Community:
Author
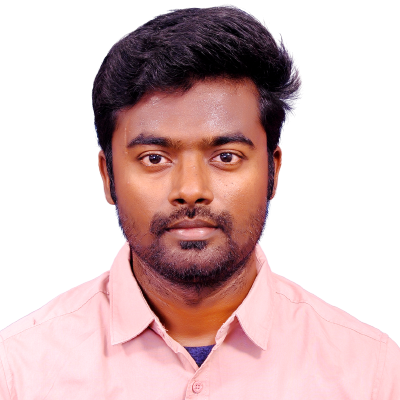
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
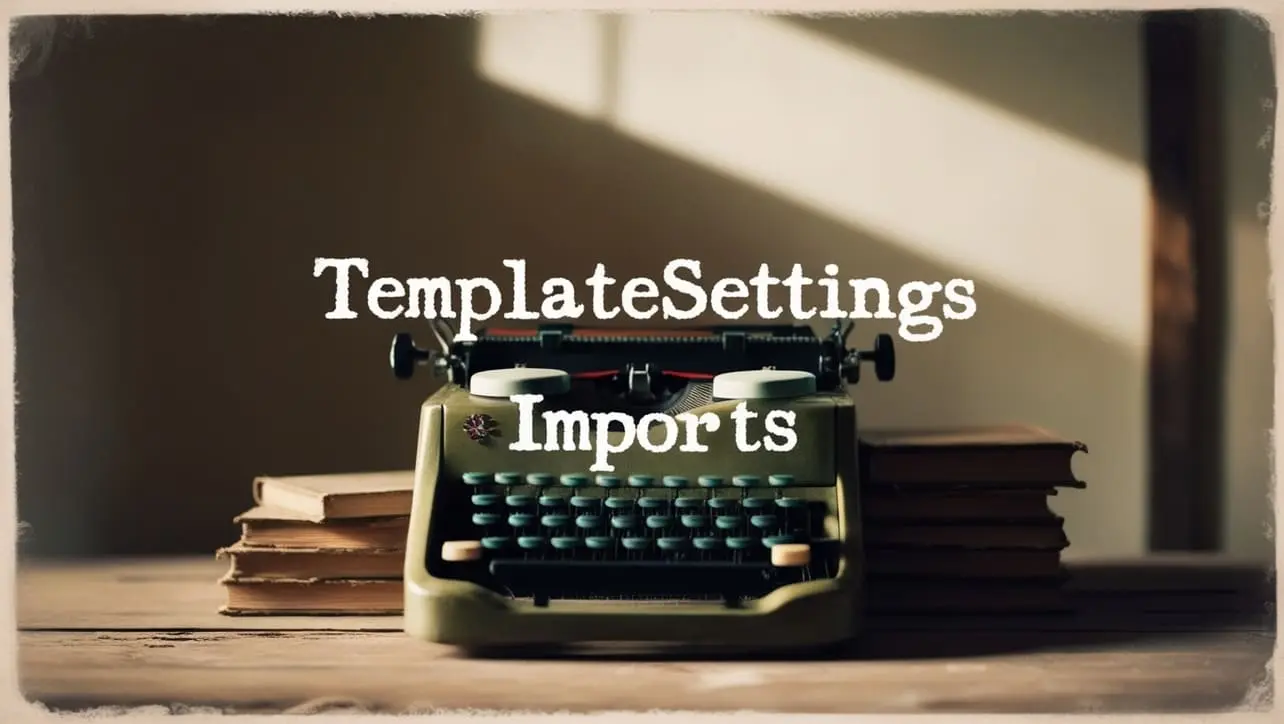
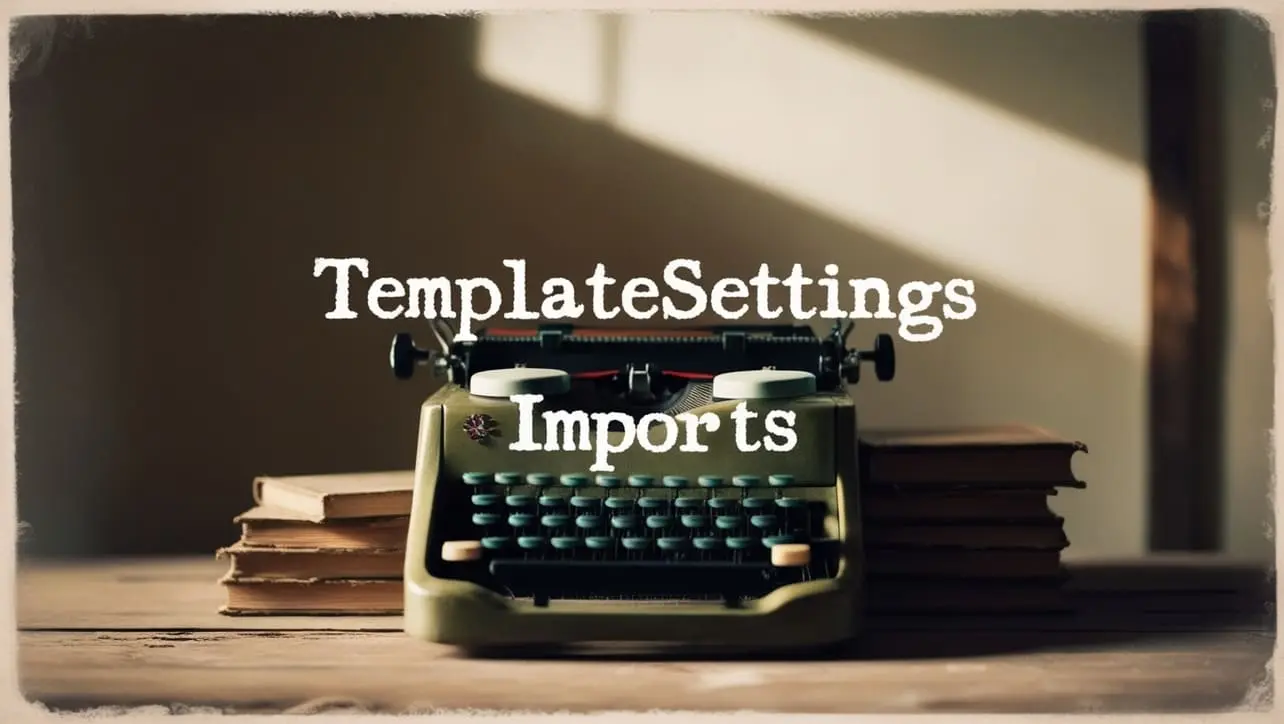
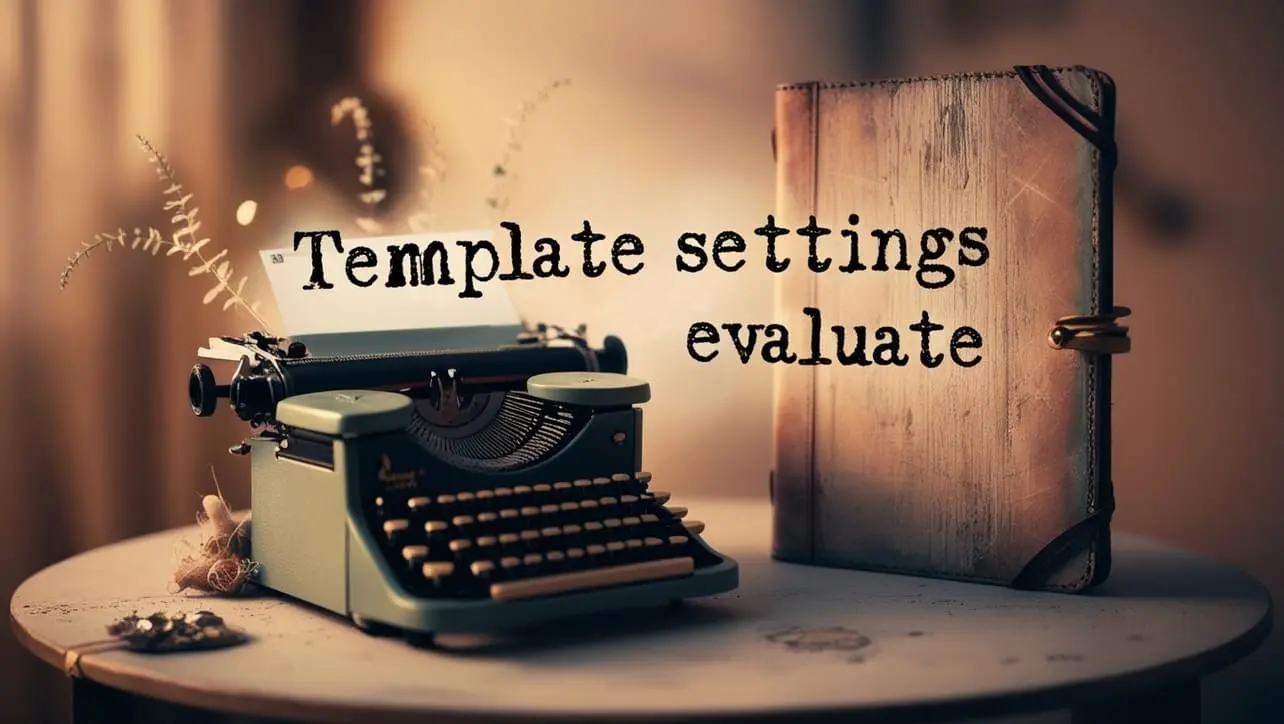
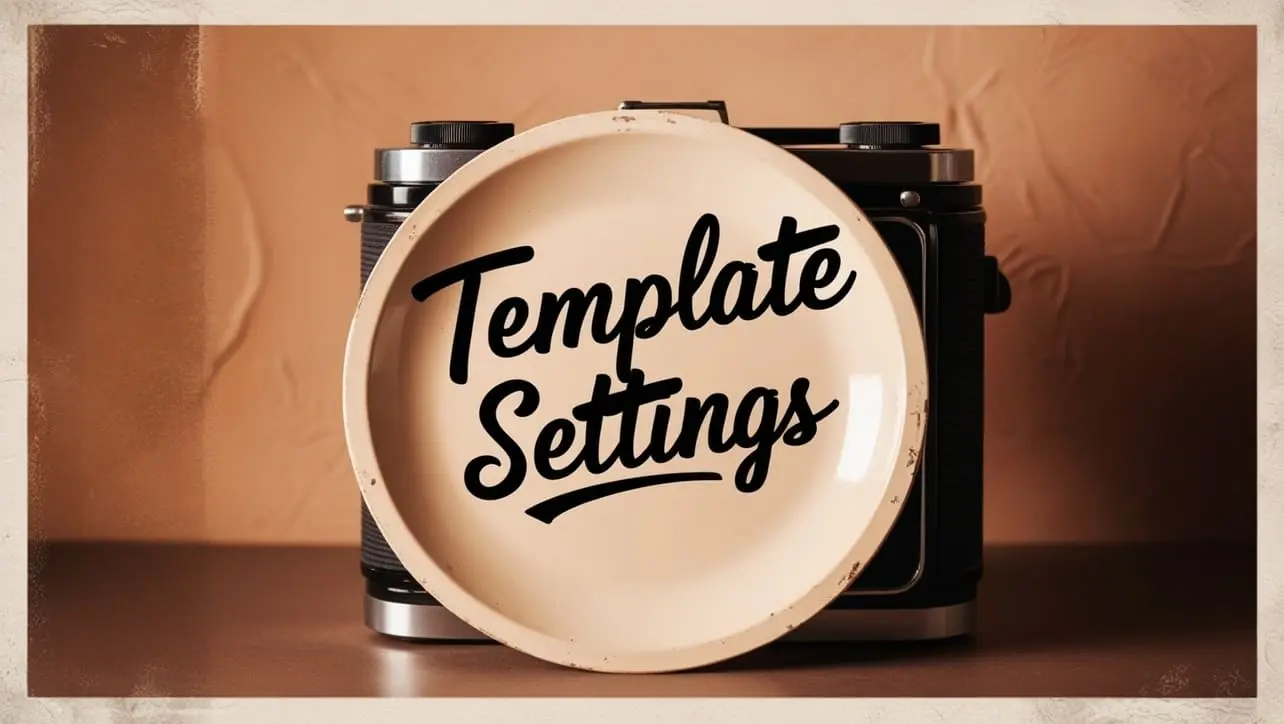
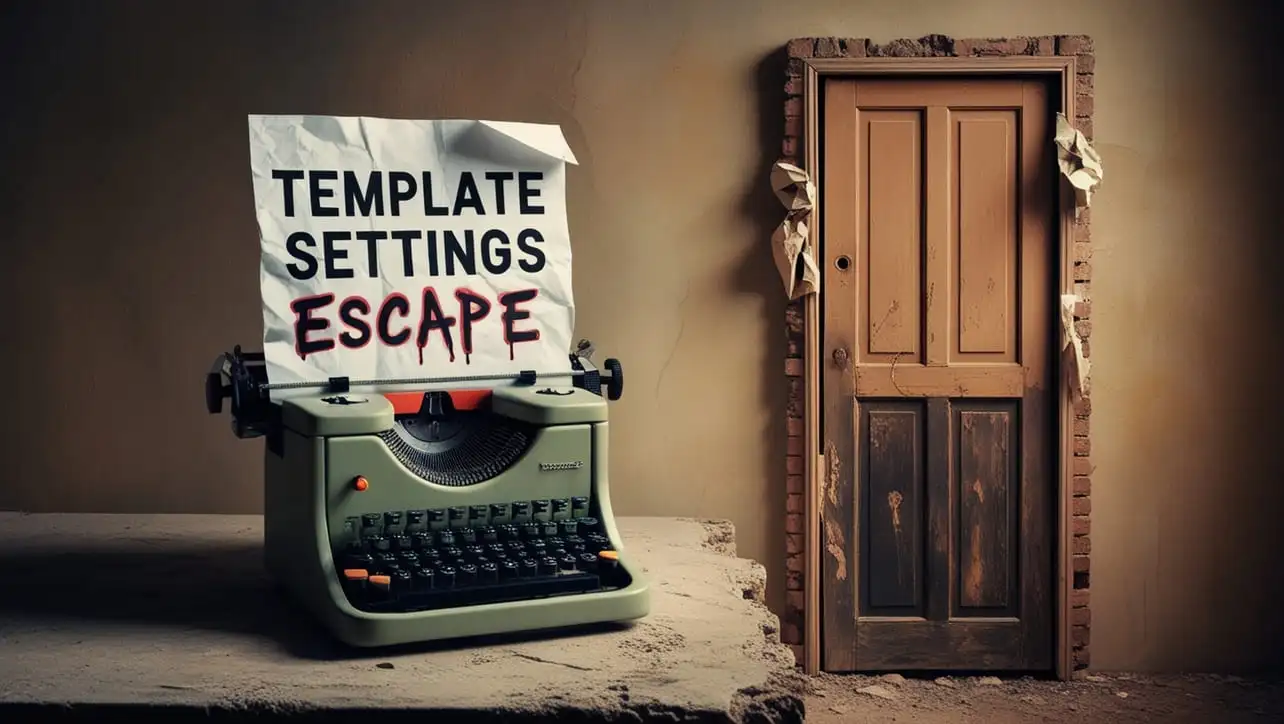
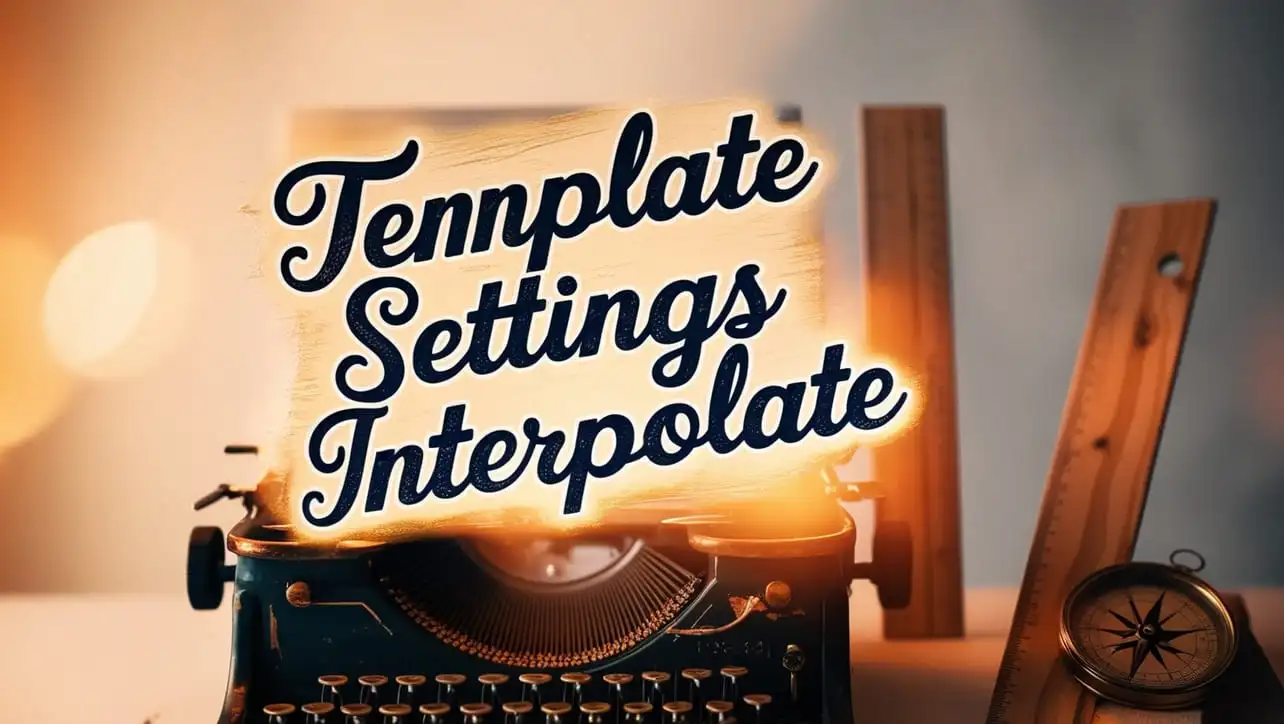
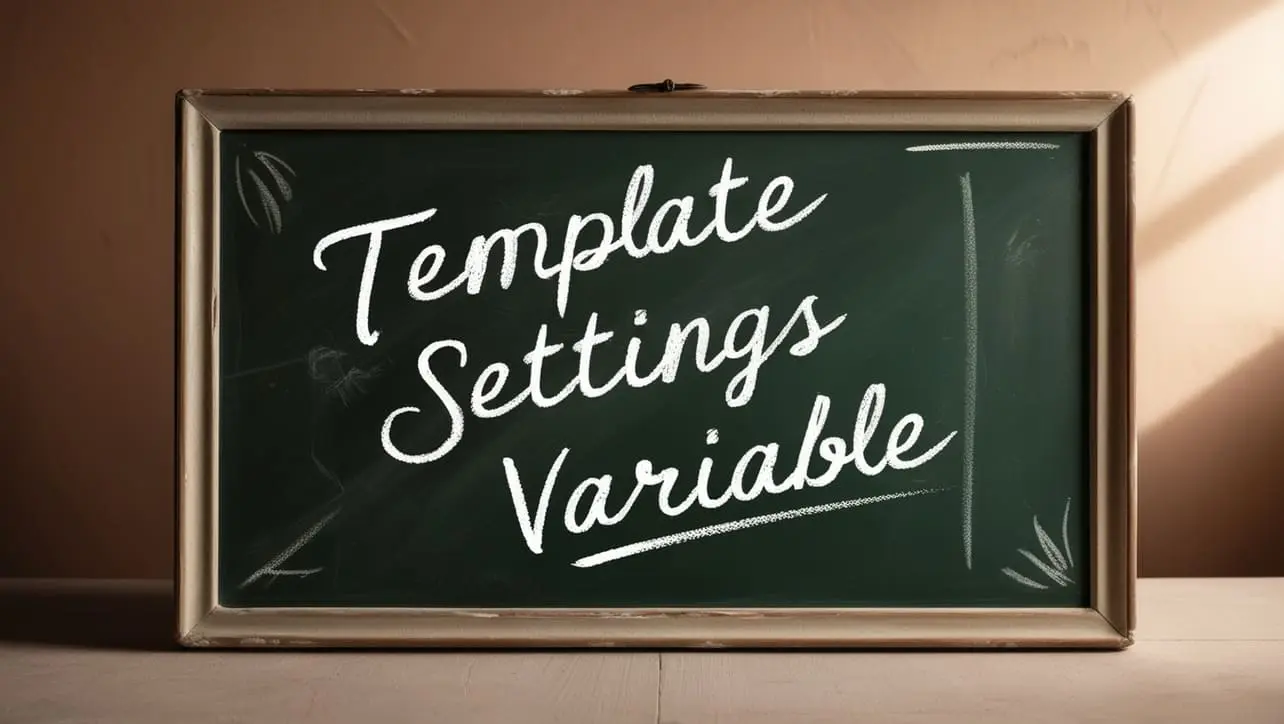
If you have any doubts regarding this article (Lodash _.cloneDeep() Lang Method), please comment here. I will help you immediately.