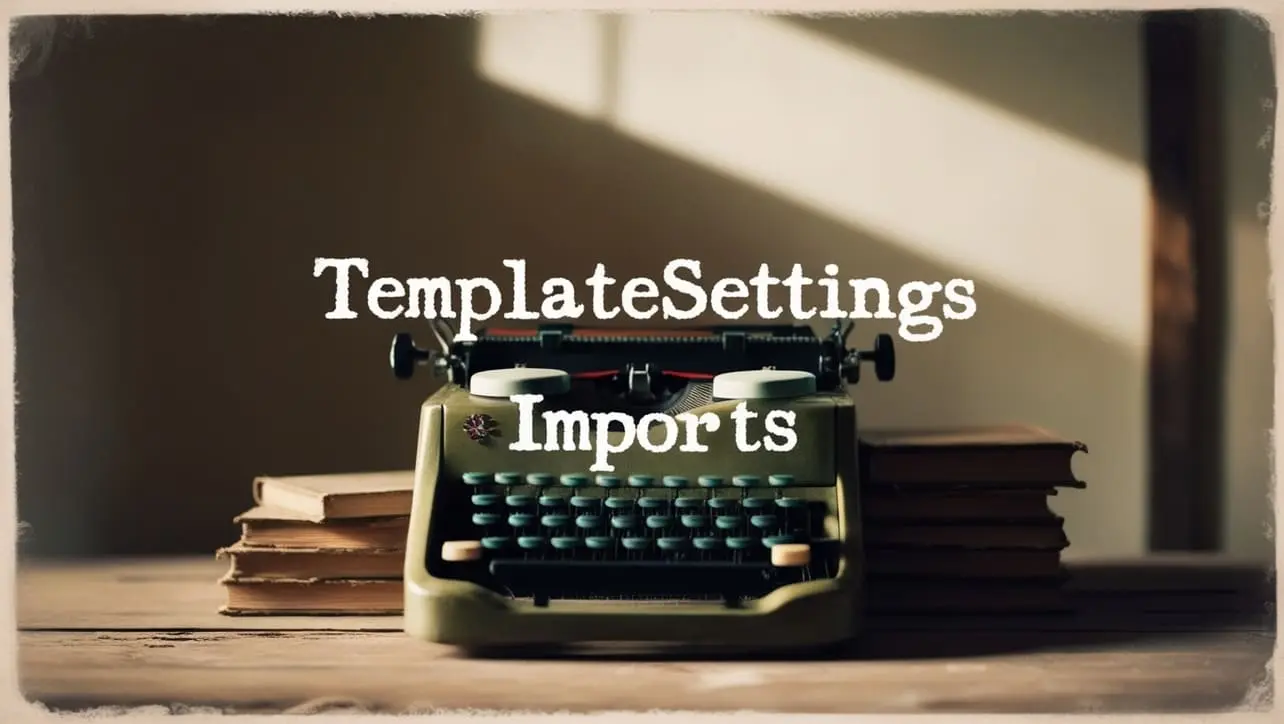
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- _.castArray
- _.clone
- _.cloneDeep
- _.cloneDeepWith
- _.cloneWith
- _.conformsTo
- _.eq
- _.gt
- _.gte
- _.isArguments
- _.isArray
- _.isArrayBuffer
- _.isArrayLike
- _.isArrayLikeObject
- _.isBoolean
- _.isBuffer
- _.isDate
- _.isElement
- _.isEmpty
- _.isEqual
- _.isEqualWith
- _.isError
- _.isFinite
- _.isFunction
- _.isInteger
- _.isLength
- _.isMap
- _.isMatch
- _.isMatchWith
- _.isNaN
- _.isNative
- _.isNil
- _.isNull
- _.isNumber
- _.isObject
- _.isObjectLike
- _.isPlainObject
- _.isRegExp
- _.isSafeInteger
- _.isSet
- _.isString
- _.isSymbol
- _.isTypedArray
- _.isUndefined
- _.isWeakMap
- _.isWeakSet
- _.lt
- _.lte
- _.toArray
- _.toFinite
- _.toInteger
- _.toLength
- _.toNumber
- _.toPlainObject
- _.toSafeInteger
- _.toString
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.castArray() Lang Method
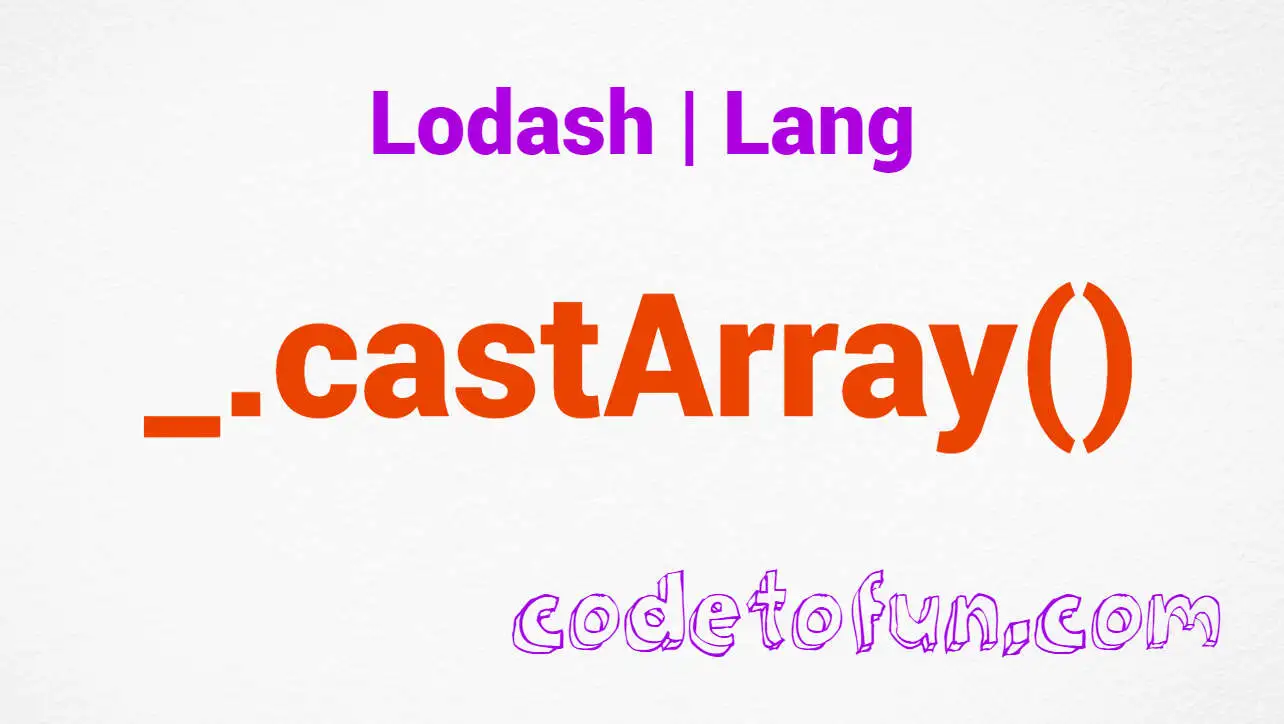
Photo Credit to CodeToFun
π Introduction
In the dynamic landscape of JavaScript programming, handling data types with flexibility is crucial. Lodash, a feature-rich utility library, provides a range of functions to simplify these challenges. Among these functions is the _.castArray()
method, a versatile tool designed to ensure that a given value is always wrapped in an array.
This method proves invaluable when dealing with uncertain or varied data types, offering consistency and ease of manipulation.
π§ Understanding _.castArray() Method
The _.castArray()
method in Lodash serves a simple yet essential purposeβit takes any value and ensures it is presented as an array. Whether the input is already an array, a single value, or even null or undefined, _.castArray()
guarantees a consistent array representation.
π‘ Syntax
The syntax for the _.castArray()
method is straightforward:
_.castArray(value)
- value: The value to cast into an array.
π Example
Let's dive into a simple example to illustrate the usage of the _.castArray()
method:
const _ = require('lodash');
const singleValue = 42;
const arrayRepresentation = _.castArray(singleValue);
console.log(arrayRepresentation);
// Output: [42]
In this example, the singleValue is cast into an array using _.castArray()
, ensuring a consistent array representation.
π Best Practices
When working with the _.castArray()
method, consider the following best practices:
Consistent Array Representation:
Use
_.castArray()
to ensure a consistent array representation, regardless of the input type. This practice simplifies code logic and promotes uniformity.example.jsCopiedconst inconsistentData = /* ...some data of various types... */; const dataArray = _.castArray(inconsistentData); console.log(dataArray); // Output: [inconsistentData] (always an array)
Handling Null and Undefined:
When dealing with values that might be null or undefined,
_.castArray()
can prevent unexpected errors by providing a default array representation.example.jsCopiedconst potentiallyNullValue = /* ...some value that might be null... */; const arrayRepresentation = _.castArray(potentiallyNullValue); console.log(arrayRepresentation); // Output: [potentiallyNullValue] or [] if potentiallyNullValue is null or undefined
Ensuring Array Consistency:
In scenarios where functions may return either a single value or an array, use
_.castArray()
to ensure consistent handling of the result.example.jsCopiedfunction fetchData() { // ...some logic to fetch data... return /* ...some data... */; } const data = _.castArray(fetchData()); console.log(data); // Output: [data] or [] if fetchData() returns null or undefined
π Use Cases
Function Parameter Standardization:
Standardize function parameters by using
_.castArray()
. This ensures that functions can seamlessly handle both single values and arrays.example.jsCopiedfunction processData(inputData) { const dataArray = _.castArray(inputData); // ...process dataArray... } processData(123); processData([456, 789]);
Configuration Options:
When dealing with configuration options that may be specified as single values or arrays,
_.castArray()
simplifies the handling of these options.example.jsCopiedconst config = { // ...other configuration options... features: _.castArray(/* ...some feature or array of features... */), }; console.log(config.features); // Output: [feature] or [] if features is null or undefined
Data Transformation:
In data transformation scenarios,
_.castArray()
ensures a consistent array structure, making subsequent processing more predictable.example.jsCopiedconst rawData = /* ...some data... */; const transformedData = _.castArray(rawData).map(/* ...some transformation logic... */); console.log(transformedData); // Output: [transformedData] or [] if rawData is null or undefined
π Conclusion
The _.castArray()
method in Lodash offers a straightforward yet powerful solution for standardizing array representations, particularly when dealing with diverse data types. Whether you're normalizing function parameters, handling configuration options, or transforming data, _.castArray()
provides a reliable tool for maintaining consistency in your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.castArray()
method in your Lodash projects.
π¨βπ» Join our Community:
Author
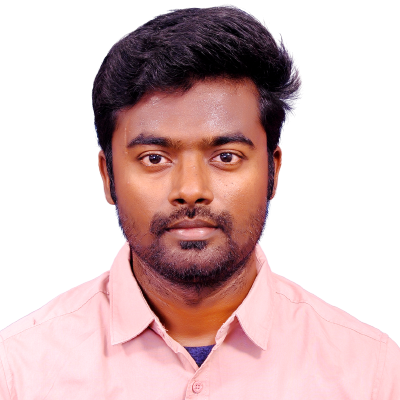
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
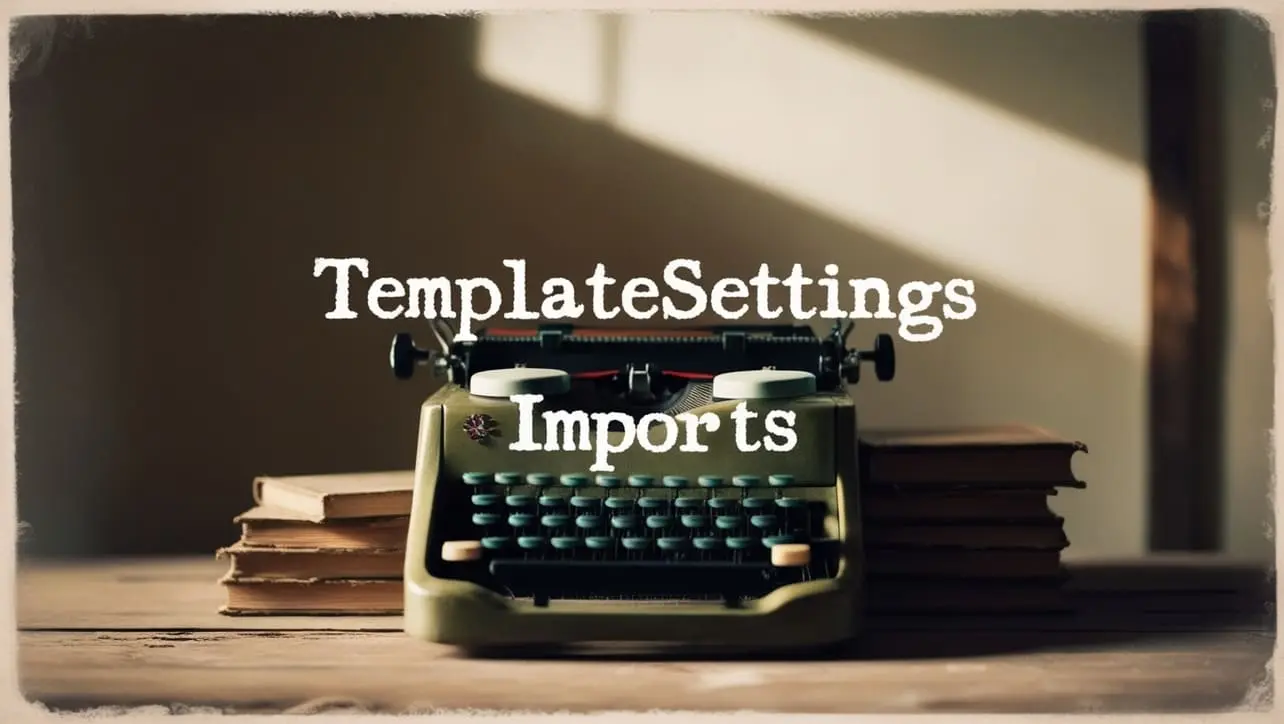
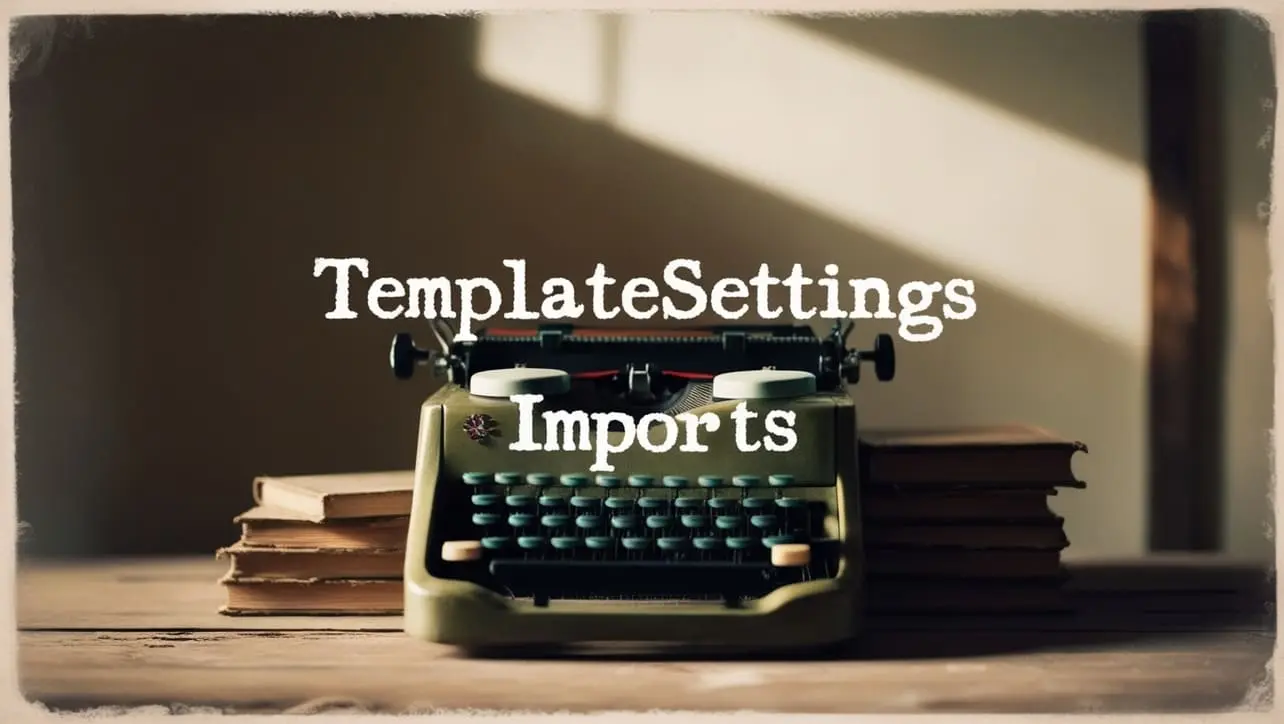
Lodash _.templateSettings.imports Property
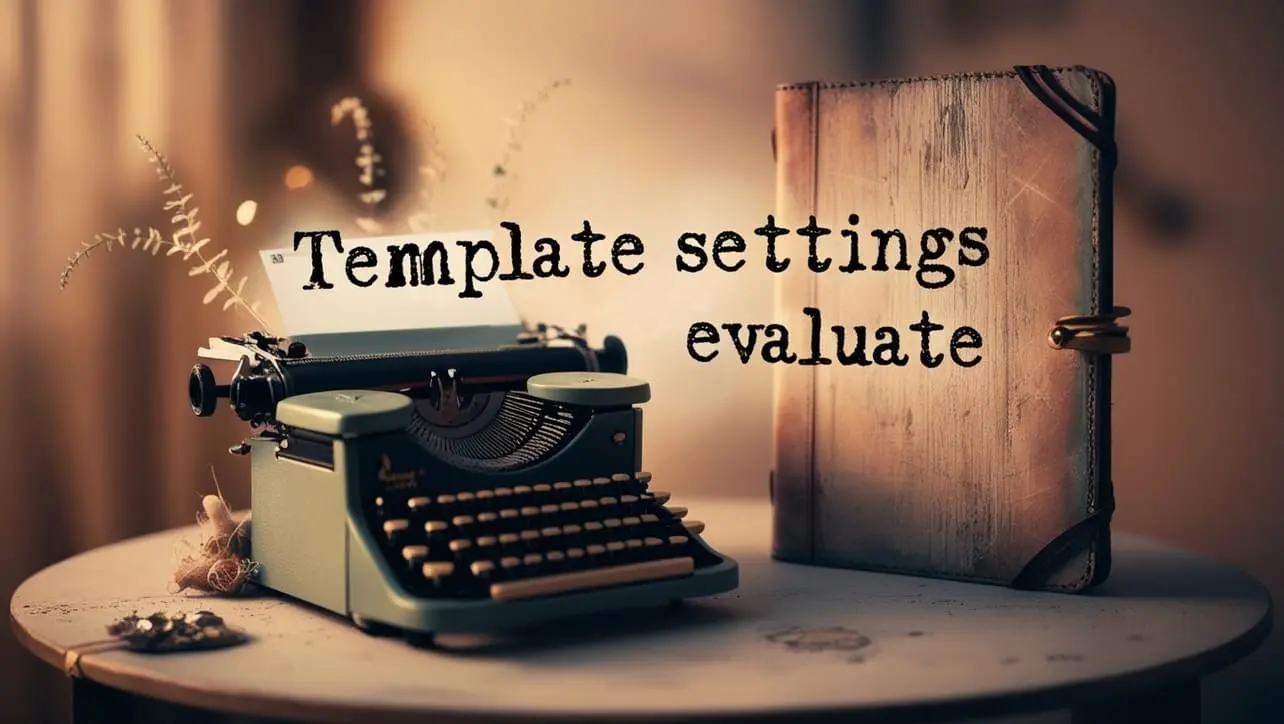
Lodash _.templateSettings.evaluate Property
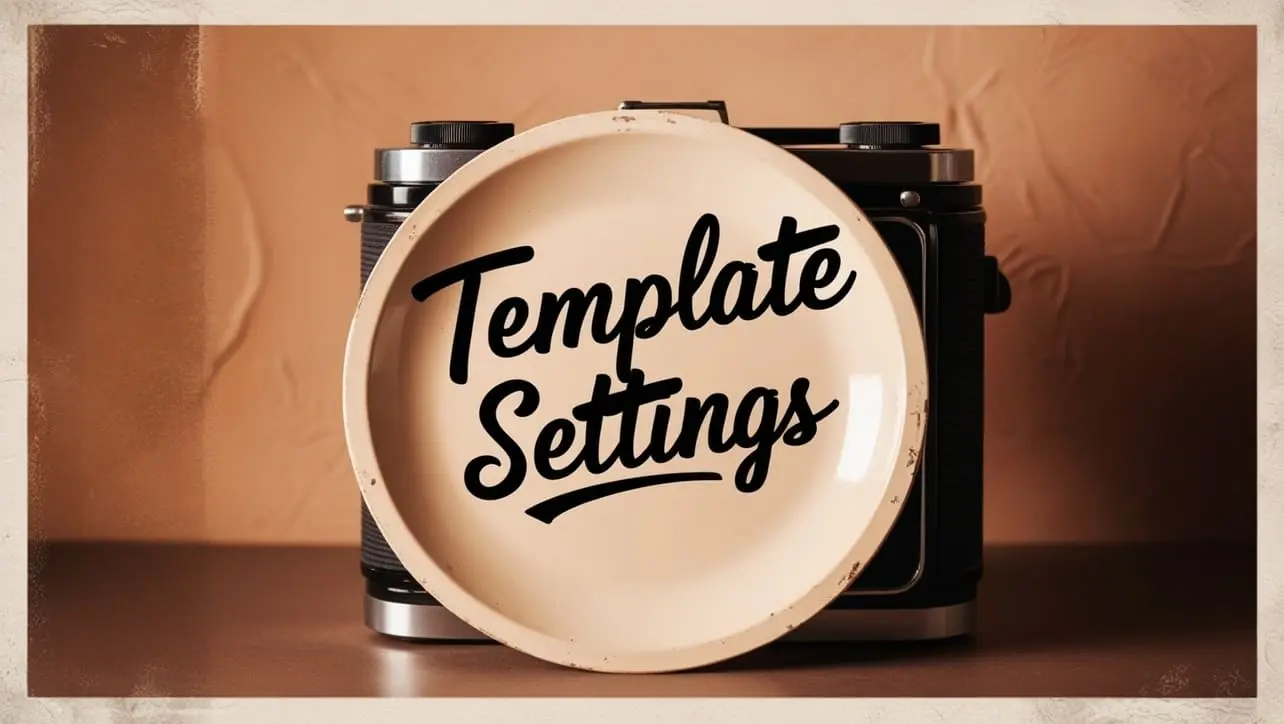
Lodash _.templateSettings Property
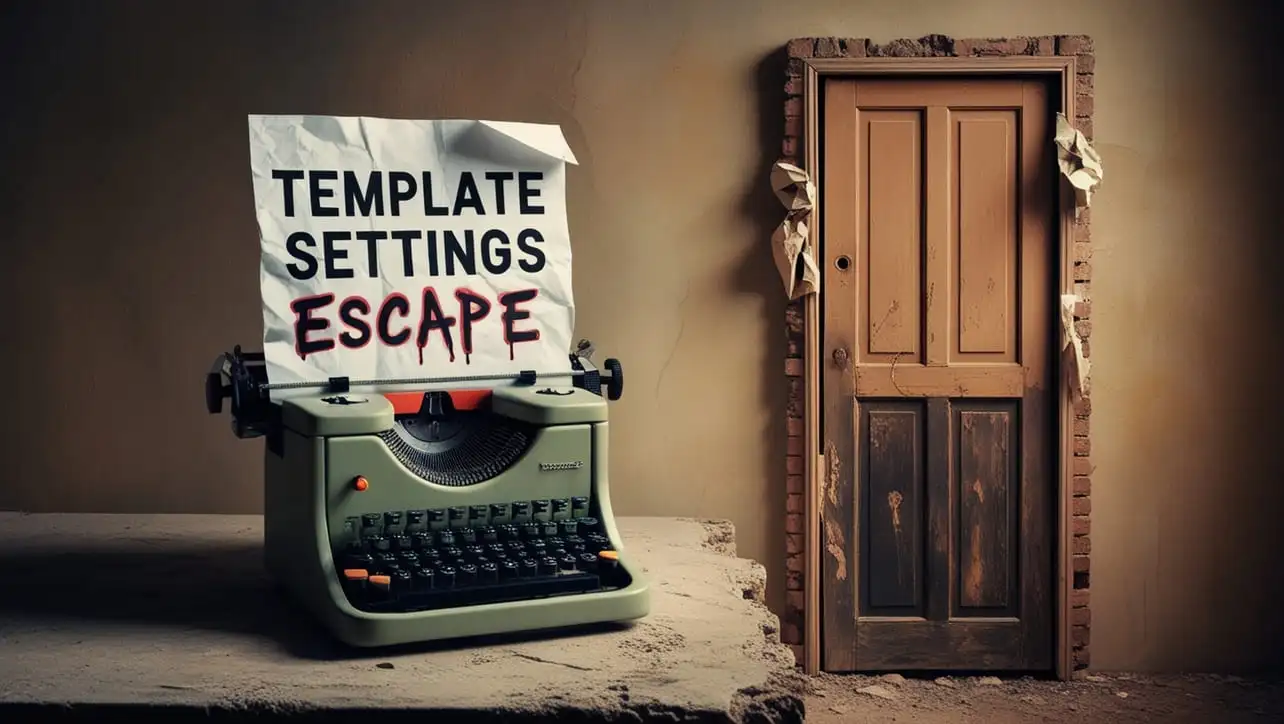
Lodash _.templateSettings.escape Property
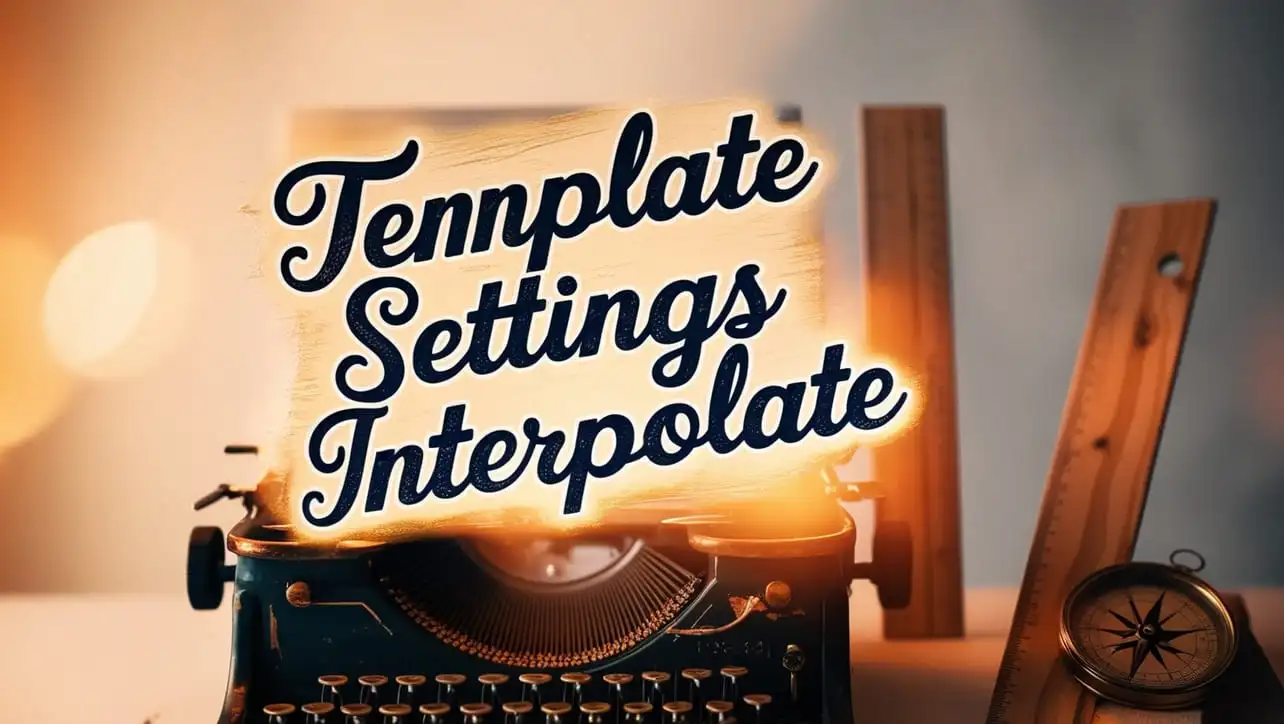
Lodash _.templateSettings.interpolate Property
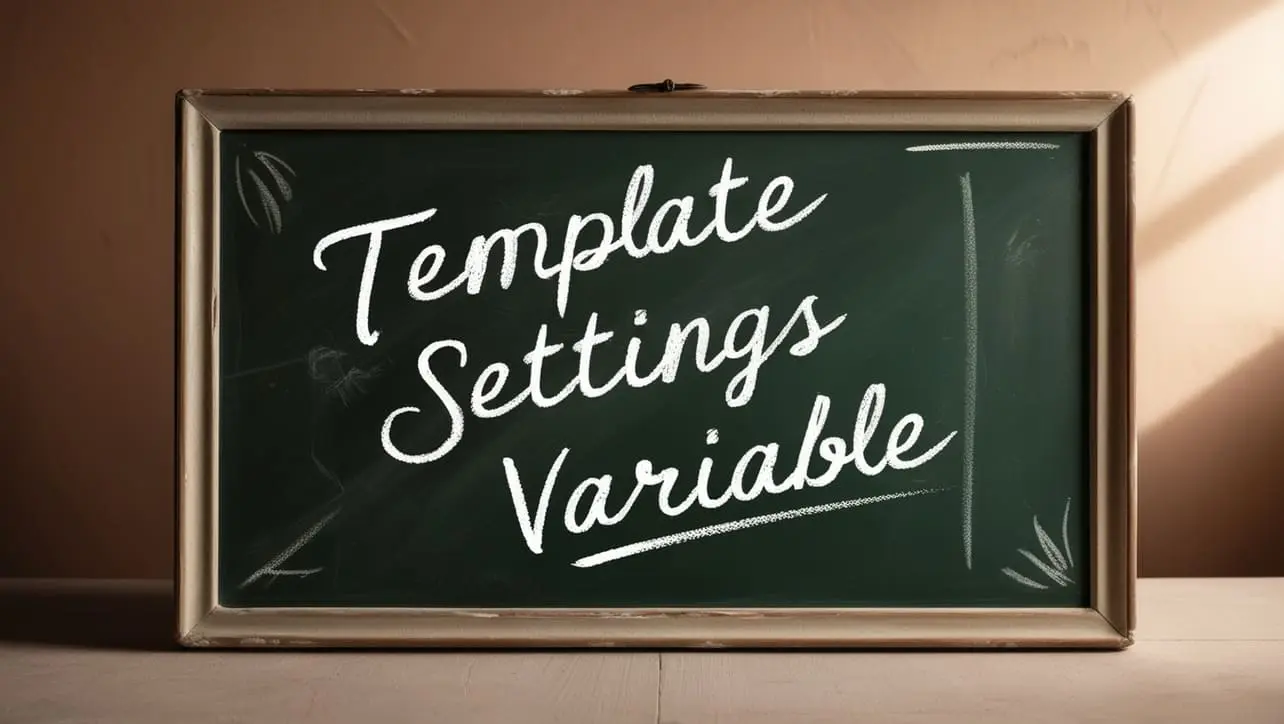
If you have any doubts regarding this article (Lodash _.castArray() Lang Method), please comment here. I will help you immediately.