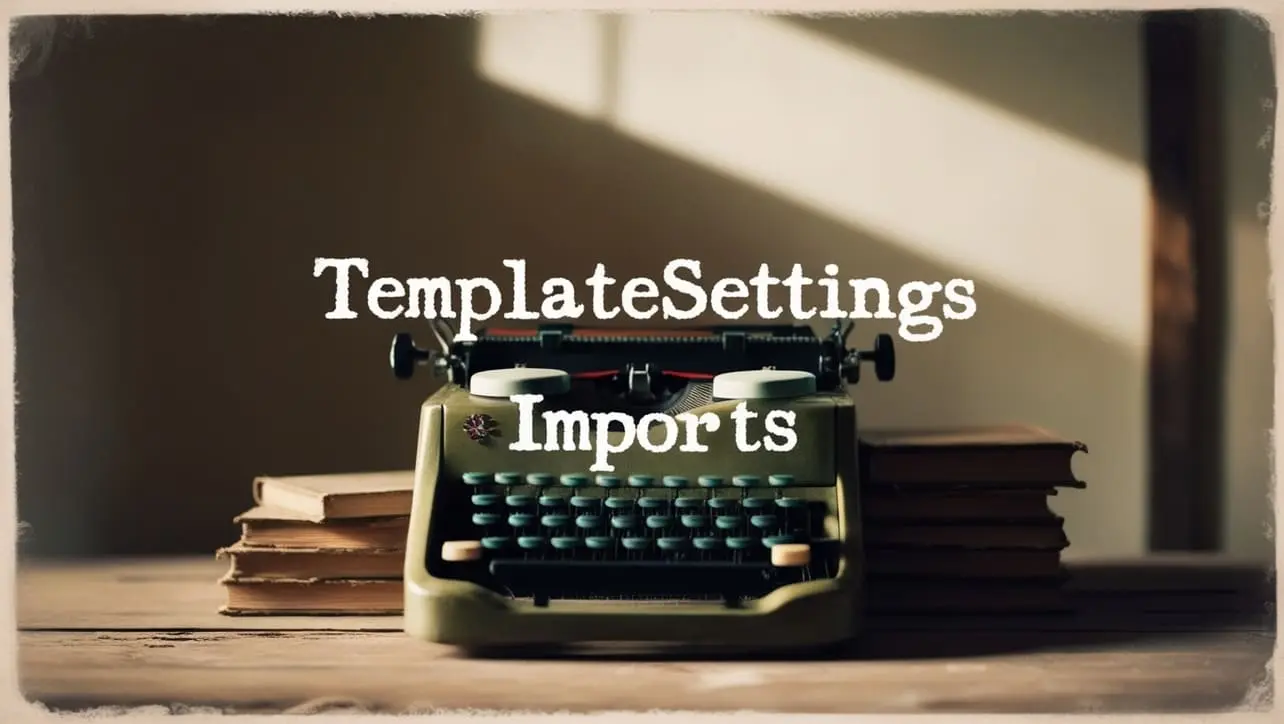
Lodash _.wrap() Function Method
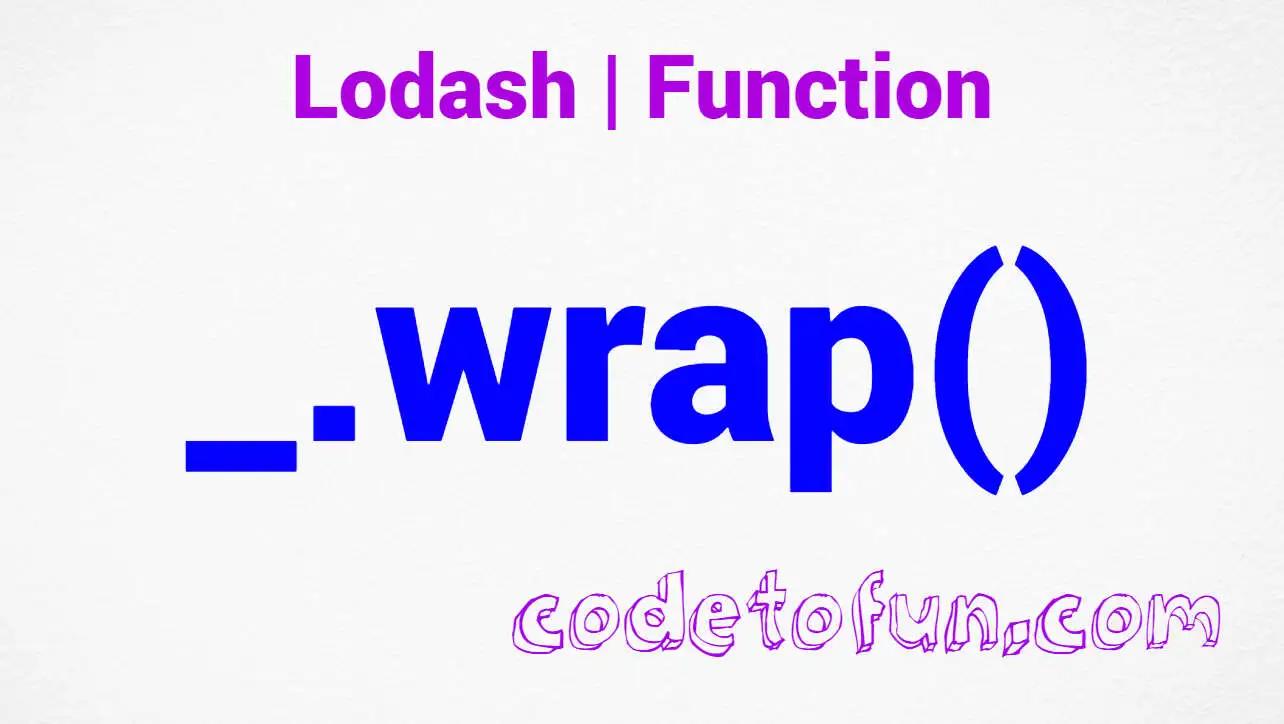
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, handling functions with care is a fundamental aspect. Lodash, a feature-rich utility library, provides developers with an arsenal of functions for streamlined code. Among these functions is the _.wrap()
method, a tool designed to create a wrapped function that invokes a given function with a provided wrapper function.
This method facilitates cleaner code organization and the application of consistent behavior around function calls.
🧠 Understanding _.wrap() Method
The _.wrap()
method in Lodash is crafted to create a new function that acts as a wrapper around a specified function. This wrapper function can be utilized to perform additional actions before or after the invocation of the original function, adding a layer of customization without modifying the original function.
💡 Syntax
The syntax for the _.wrap()
method is straightforward:
_.wrap(func, wrapper)
- func: The function to wrap.
- wrapper: The function providing the wrapper behavior.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.wrap()
method:
const _ = require('lodash');
function greet(name) {
return `Hello, ${name}!`;
}
const wrappedGreet = _.wrap(greet, function (func, name) {
return `Before ${func(name)} After`;
});
console.log(wrappedGreet('John'));
// Output: Before Hello, John! After
In this example, _.wrap()
is used to create a wrapped version of the greet function, adding custom behavior before and after the original function is called.
🏆 Best Practices
When working with the _.wrap()
method, consider the following best practices:
Preserve Context:
When using
_.wrap()
, ensure that the wrapper function maintains the context (this value) of the original function. This is crucial when working with object methods or functions that rely on the correct context for proper execution.example.jsCopiedconst obj = { value: 'Wrapped', getValue: function () { return this.value; }, }; const wrappedGetValue = _.wrap(obj.getValue, function (func) { return `Wrapper: ${func.call(obj)}`; }); console.log(wrappedGetValue()); // Output: Wrapper: Wrapped
Compose Multiple Wrappers:
Explore the ability to compose multiple wrappers for a single function. This can be achieved by chaining multiple
_.wrap()
calls, allowing for a modular and organized approach to function wrapping.example.jsCopiedconst originalFunction = (input) => `Original: ${input}`; const wrappedFunction = _.wrap( _.wrap(originalFunction, (func, input) => `Wrapper1: ${func(input)}`), (func, input) => `Wrapper2: ${func(input)}` ); console.log(wrappedFunction('Data')); // Output: Wrapper2: Wrapper1: Original: Data
Utilize Function Parameters:
Leverage the parameters of the wrapper function to make it more versatile. This allows you to pass dynamic values or behaviors to the wrapper and enhance the reusability of the wrapping logic.
example.jsCopiedfunction addPrefix(text, prefix) { return `${prefix} ${text}`; } const wrappedAddPrefix = _.wrap(addPrefix, function (func, text, prefix) { return func(text, `CustomPrefix: ${prefix}`); }); console.log(wrappedAddPrefix('Message', 'Hello')); // Output: CustomPrefix: Hello Message
📚 Use Cases
Logging and Debugging:
Use
_.wrap()
to create wrapper functions that log information or aid in debugging without modifying the core functionality of the original functions.example.jsCopiedconst originalFunction = (data) => { // ... complex logic ... return result; }; const debugWrapper = _.wrap(originalFunction, (func, data) => { console.log(`Calling function with data: ${data}`); const result = func(data); console.log(`Function result: ${result}`); return result; }); // Use the wrapped function for debugging const result = debugWrapper('InputData');
Pre and Post Processing:
Apply pre-processing or post-processing steps to functions using
_.wrap()
. This is beneficial when additional actions need to be taken before or after the execution of a function.example.jsCopiedconst originalSaveFunction = (data) => { // ... save data to database ... }; const saveWithLogging = _.wrap(originalSaveFunction, (func, data) => { console.log(`Saving data: ${data}`); func(data); console.log('Data saved successfully'); }); // Use the wrapped function for logging before and after saving saveWithLogging('NewData');
Consistent Error Handling:
Create wrappers that handle errors consistently across multiple functions. This can centralize error-handling logic and improve code maintainability.
example.jsCopiedconst originalFunction = (input) => { // ... complex logic ... if (/* error condition */) { throw new Error('Function failed'); } return result; }; const safeWrapper = _.wrap(originalFunction, (func, input) => { try { return func(input); } catch (error) { console.error(`Error in function: ${error.message}`); // Handle or log the error } }); // Use the wrapped function with consistent error handling safeWrapper('Data');
🎉 Conclusion
The _.wrap()
method in Lodash is a versatile tool for creating wrapped functions that add custom behavior before or after the execution of a given function. Whether you're aiming to enhance logging, debugging, or error handling, _.wrap()
empowers developers with a clean and organized approach to function customization.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.wrap()
method in your Lodash projects.
👨💻 Join our Community:
Author
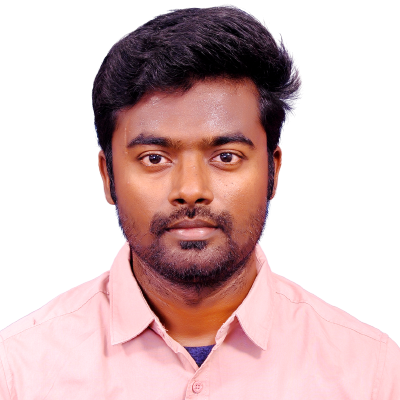
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
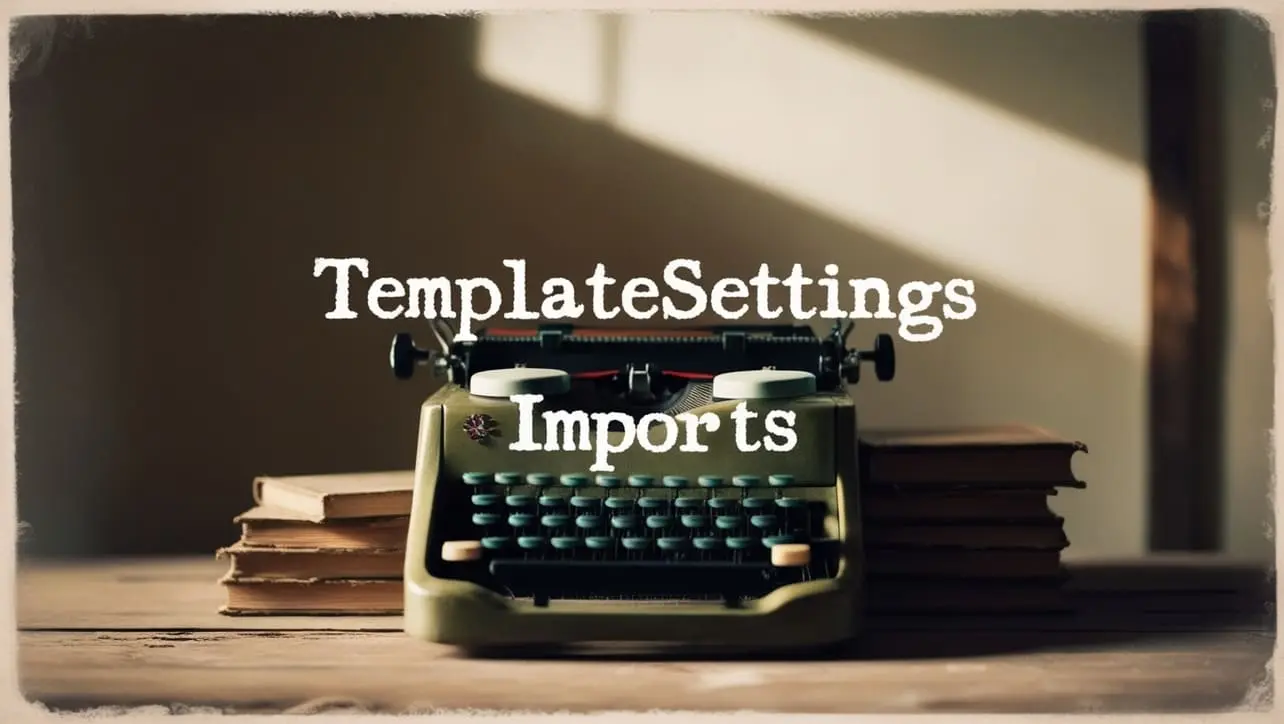
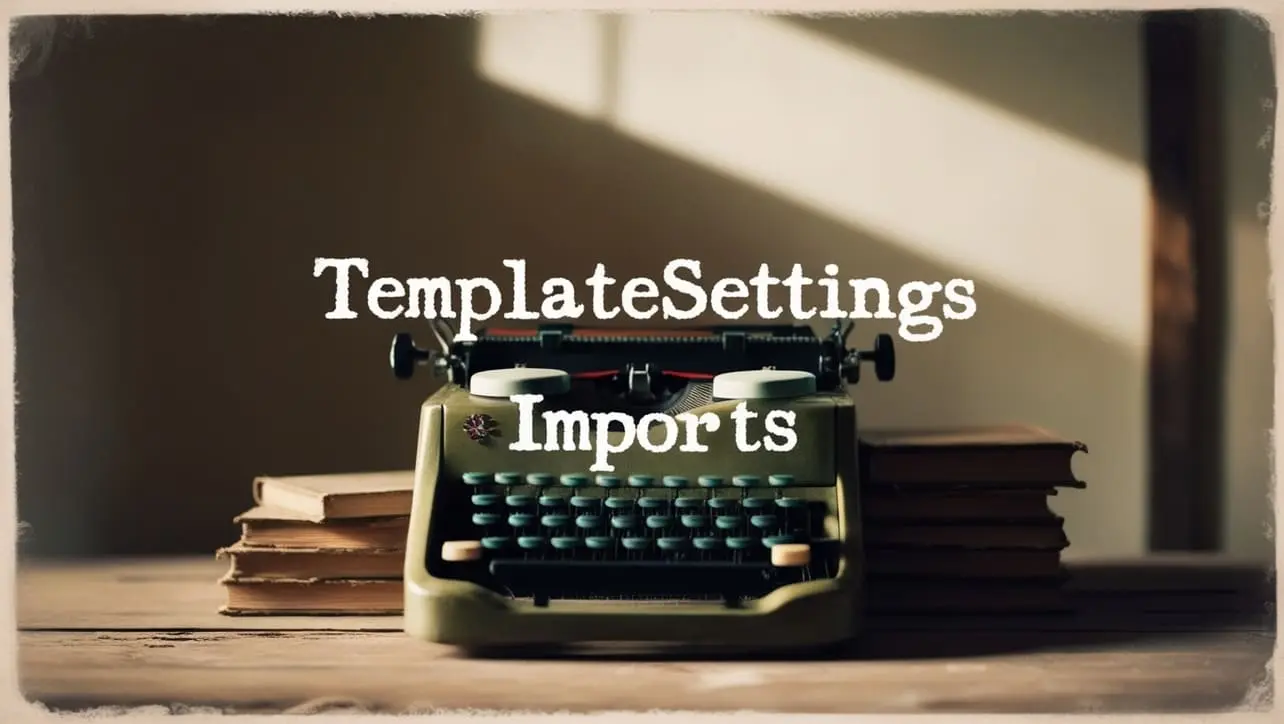
Lodash _.templateSettings.imports Property
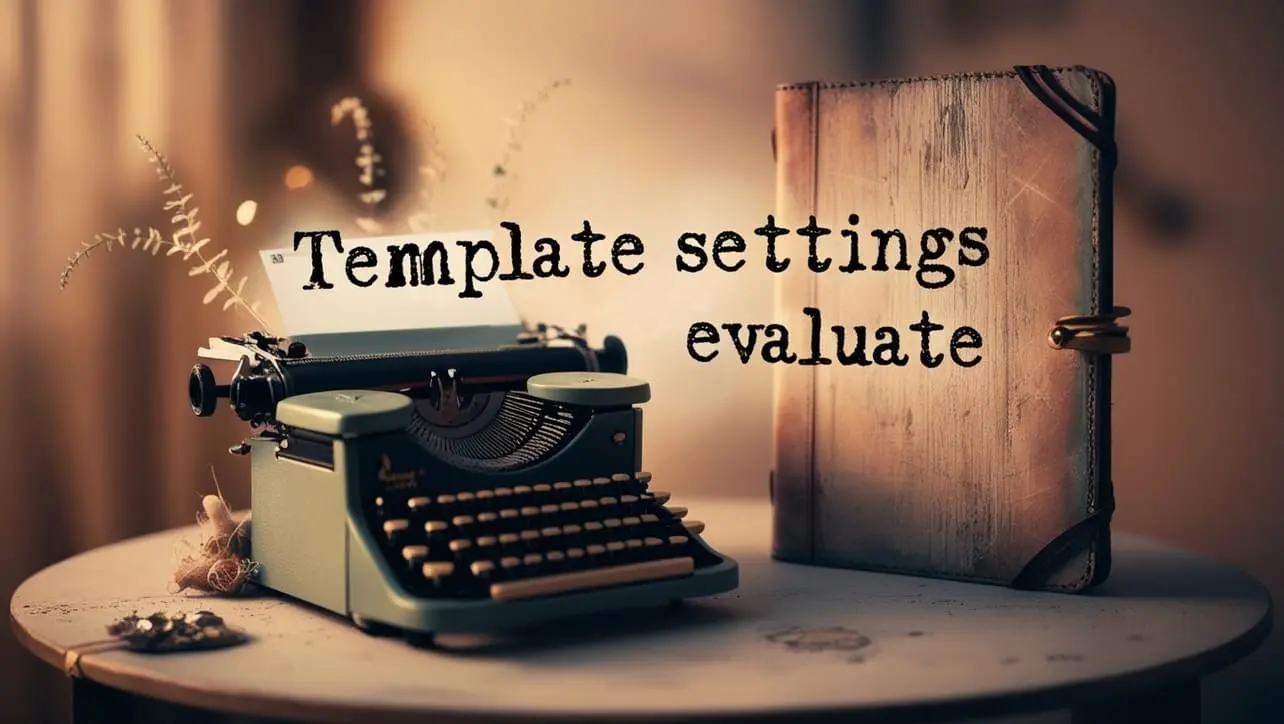
Lodash _.templateSettings.evaluate Property
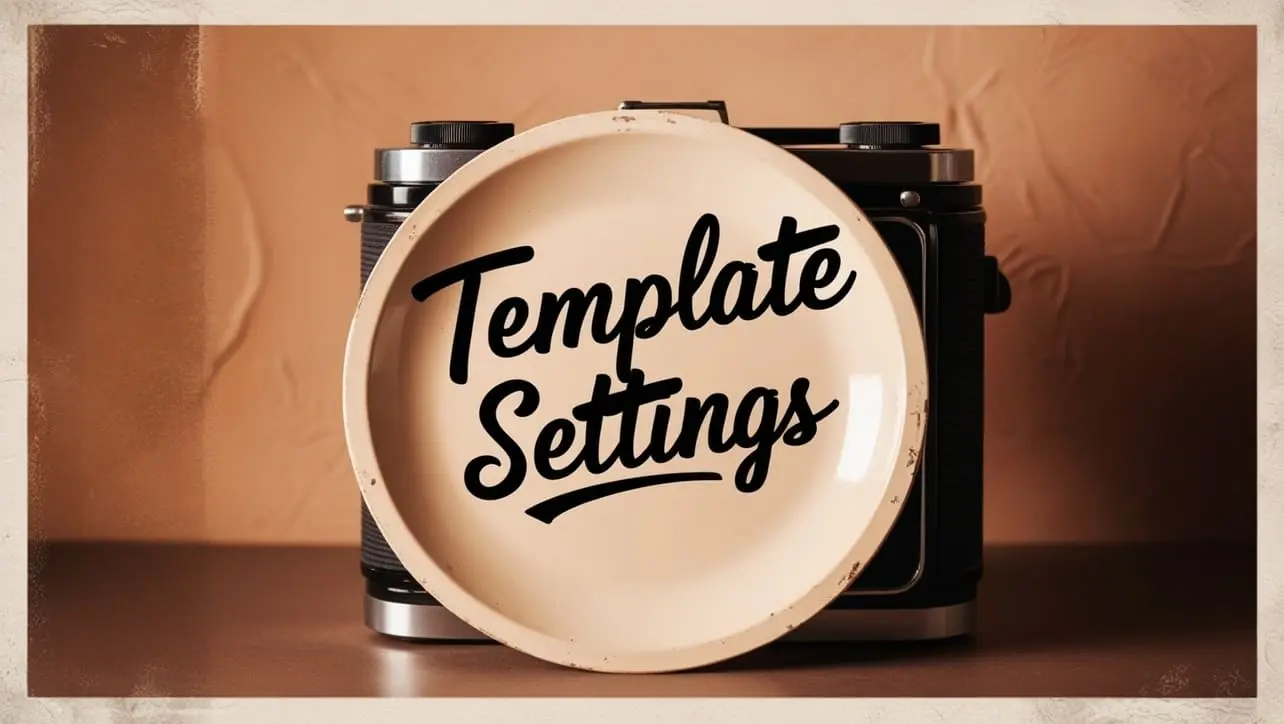
Lodash _.templateSettings Property
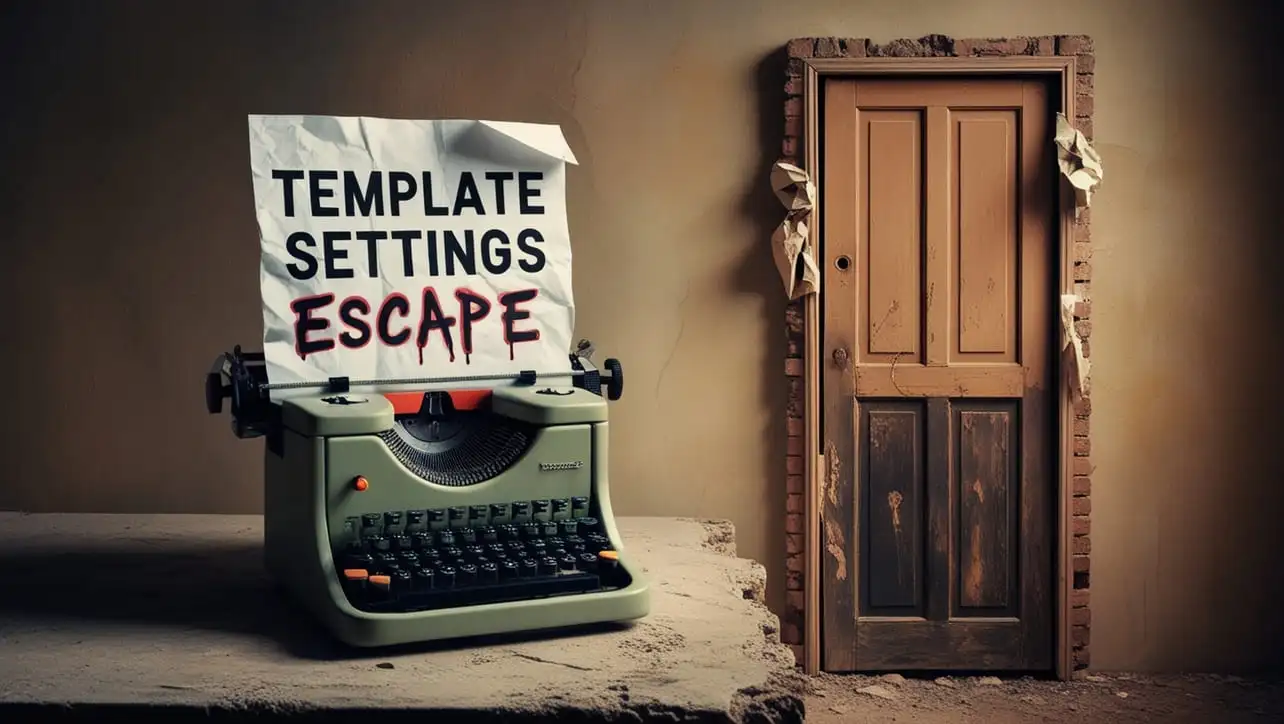
Lodash _.templateSettings.escape Property
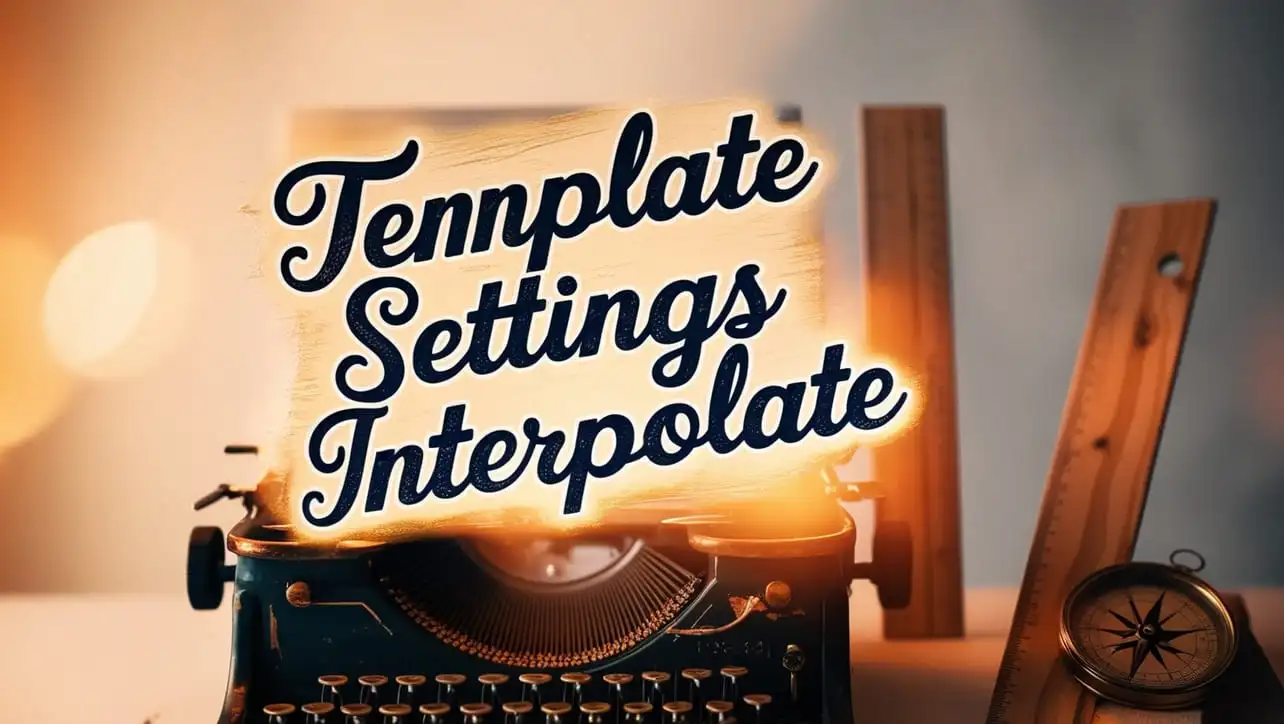
Lodash _.templateSettings.interpolate Property
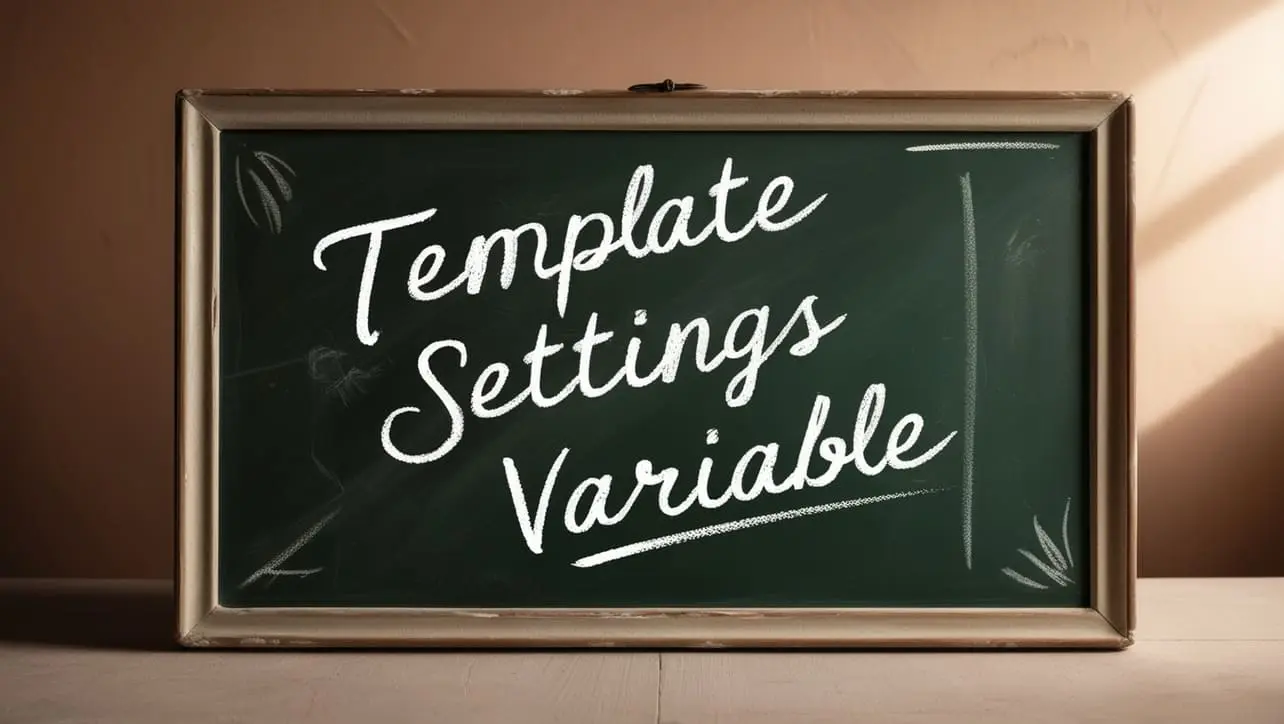
If you have any doubts regarding this article (Lodash _.wrap() Function Method), please comment here. I will help you immediately.