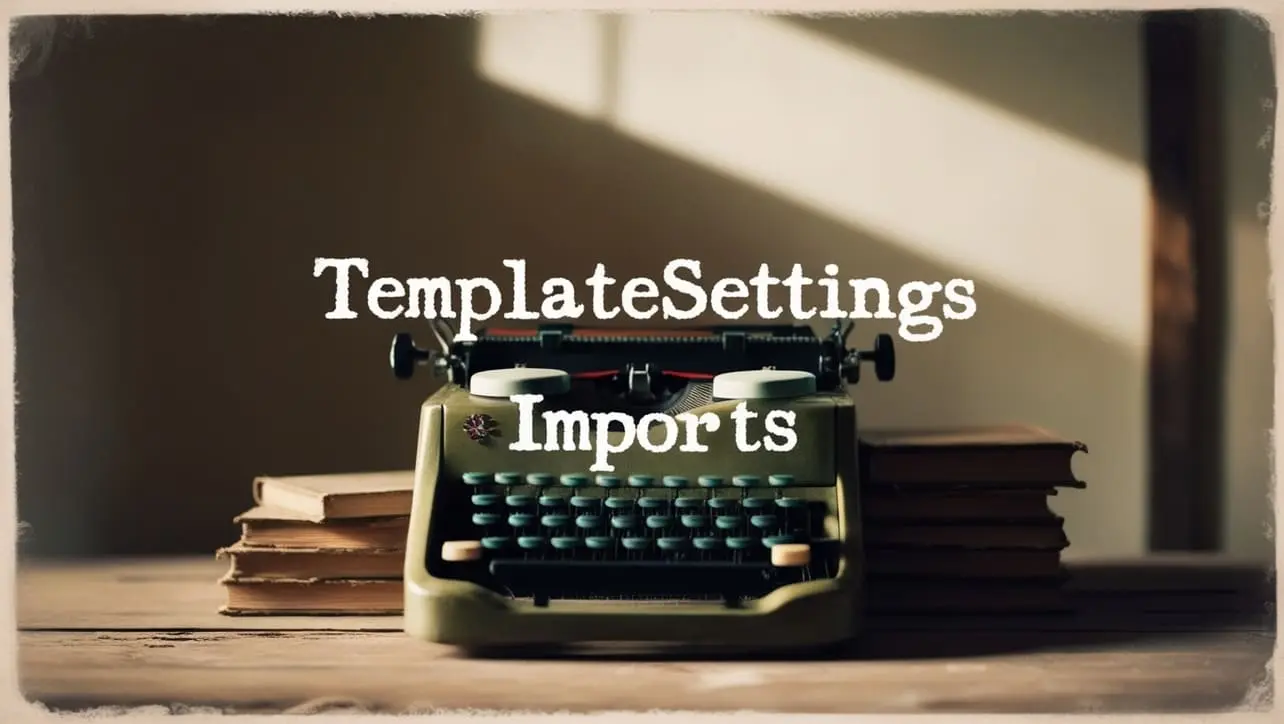
Lodash _.overArgs() Function Method
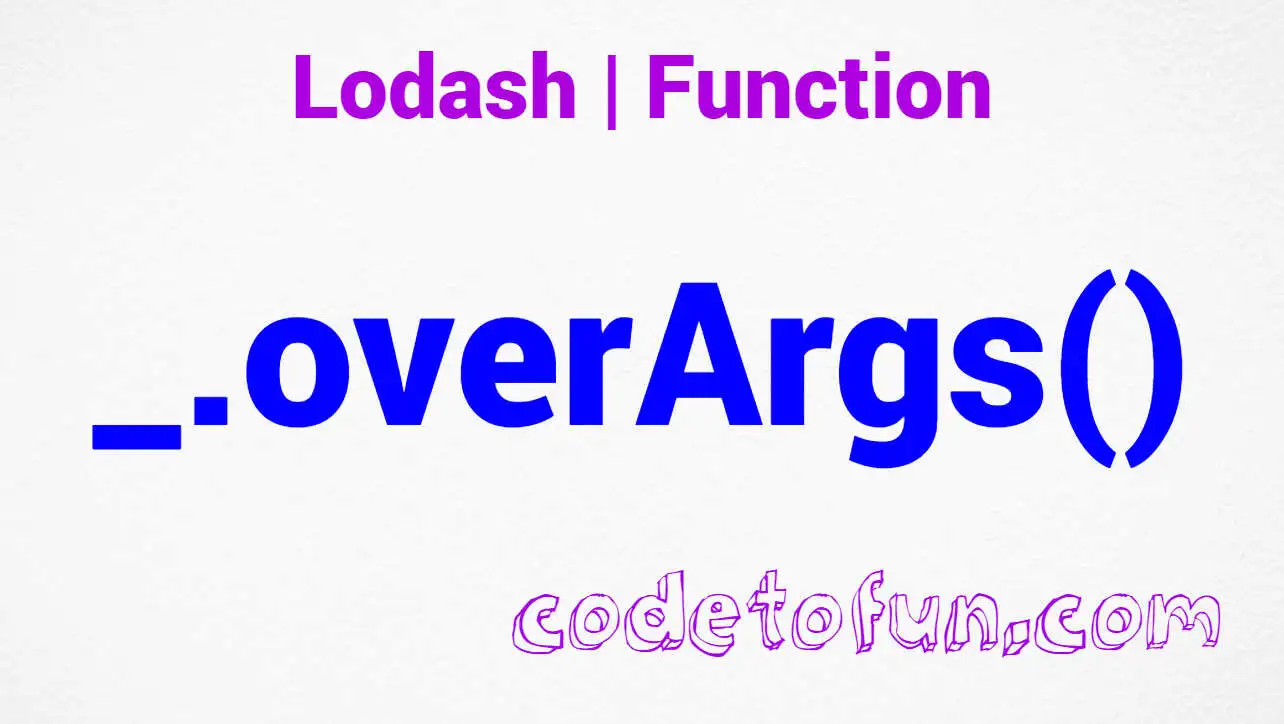
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, managing and composing functions efficiently is a key aspect of creating robust and maintainable code. Lodash, a powerful utility library, provides developers with a variety of tools to streamline these tasks. Among these tools is the _.overArgs()
method, a function-centric utility that enables the composition of functions by applying arguments transformation.
This method proves invaluable when dealing with complex function composition and enhances code readability.
🧠 Understanding _.overArgs() Method
The _.overArgs()
method in Lodash allows developers to create a new function that invokes a provided function with transformed arguments. This transformation is achieved by applying a mapper function to each argument before passing it to the original function. This function composition technique can simplify code by separating concerns and making functions more reusable.
💡 Syntax
The syntax for the _.overArgs()
method is straightforward:
_.overArgs(func, [transforms])
- func: The function to wrap.
- transforms: An array of functions that transform the arguments.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.overArgs()
method:
const _ = require('lodash');
function add(a, b) {
return a + b;
}
function square(x) {
return x * x;
}
const overArgsFunction = _.overArgs(add, [square, square]);
console.log(overArgsFunction(2, 3));
// Output: (2^2) + (3^2) = 13
In this example, the add function is wrapped with _.overArgs()
, and two square functions are applied to the arguments before invoking add. This results in the sum of the squares of the provided arguments.
🏆 Best Practices
When working with the _.overArgs()
method, consider the following best practices:
Understand Function Dependencies:
When using
_.overArgs()
, ensure a clear understanding of the dependencies and requirements of the wrapped function. The transforms applied should align with the expected input of the original function.example.jsCopiedfunction multiply(a, b, c) { return a * b * c; } const double = x => x * 2; const overArgsMultiply = _.overArgs(multiply, [double, double, double]); console.log(overArgsMultiply(2, 3, 4)); // Output: (2 * 2) * (3 * 2) * (4 * 2) = 192
Use With Complex Function Composition:
Employ
_.overArgs()
in scenarios where complex function composition is required. This method excels in situations where arguments need to be transformed before being passed to subsequent functions.example.jsCopiedfunction greet(name, greeting, punctuation) { return `${greeting}, ${name}${punctuation}`; } const upperCase = str => str.toUpperCase(); const exclamation = str => `${str}!`; const overArgsGreet = _.overArgs(greet, [upperCase, exclamation, exclamation]); console.log(overArgsGreet('John', 'hello', '!')); // Output: HELLO, JOHN!!
Keep It Readable:
While
_.overArgs()
can offer powerful capabilities, ensure that the resulting code remains readable. Use it judiciously to enhance clarity rather than obfuscate the logic.example.jsCopiedconst transformArgs = [upperCase, exclamation, exclamation]; const complexGreet = _.overArgs(greet, transformArgs); console.log(complexGreet('Alice', 'hi', '!')); // Output: HI, ALICE!!
📚 Use Cases
Argument Transformation in Mathematical Operations:
_.overArgs()
can be employed to transform arguments in mathematical operations, providing a concise and readable way to express complex formulas.example.jsCopiedfunction calculateArea(length, width) { return length * width; } const overArgsCalculateArea = _.overArgs(calculateArea, [square, square]); console.log(overArgsCalculateArea(2, 3)); // Output: (2^2) * (3^2) = 36
String Manipulation with Multiple Transforms:
When dealing with string manipulation that involves multiple transformations,
_.overArgs()
can simplify the code and enhance maintainability.example.jsCopiedfunction formatMessage(name, greeting, punctuation) { return `${greeting}, ${name}${punctuation}`; } const capitalize = str => str.charAt(0).toUpperCase() + str.slice(1); const exclamationPoint = str => `${str}!`; const overArgsFormatMessage = _.overArgs(formatMessage, [capitalize, exclamationPoint, exclamationPoint]); console.log(overArgsFormatMessage('Bob', 'hey', '!')); // Output: Hey, Bob!!
-
Reusable Transform Functions
Utilize
_.overArgs()
with reusable transform functions to create a more modular and maintainable codebase.example.jsCopiedconst triple = x => x * 3; const overArgsTriple = _.overArgs(triple, [double]); console.log(overArgsTriple(4)); // Output: (4 * 2) * 3 = 24
🎉 Conclusion
The _.overArgs()
method in Lodash empowers developers to compose functions by transforming arguments before invoking the original function. With its flexibility and utility, this method proves valuable in scenarios where complex function composition and argument transformation are required.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.overArgs()
method in your Lodash projects.
👨💻 Join our Community:
Author
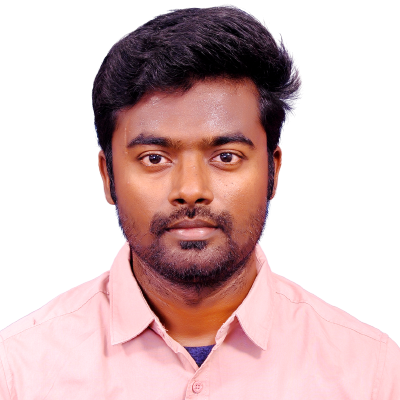
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
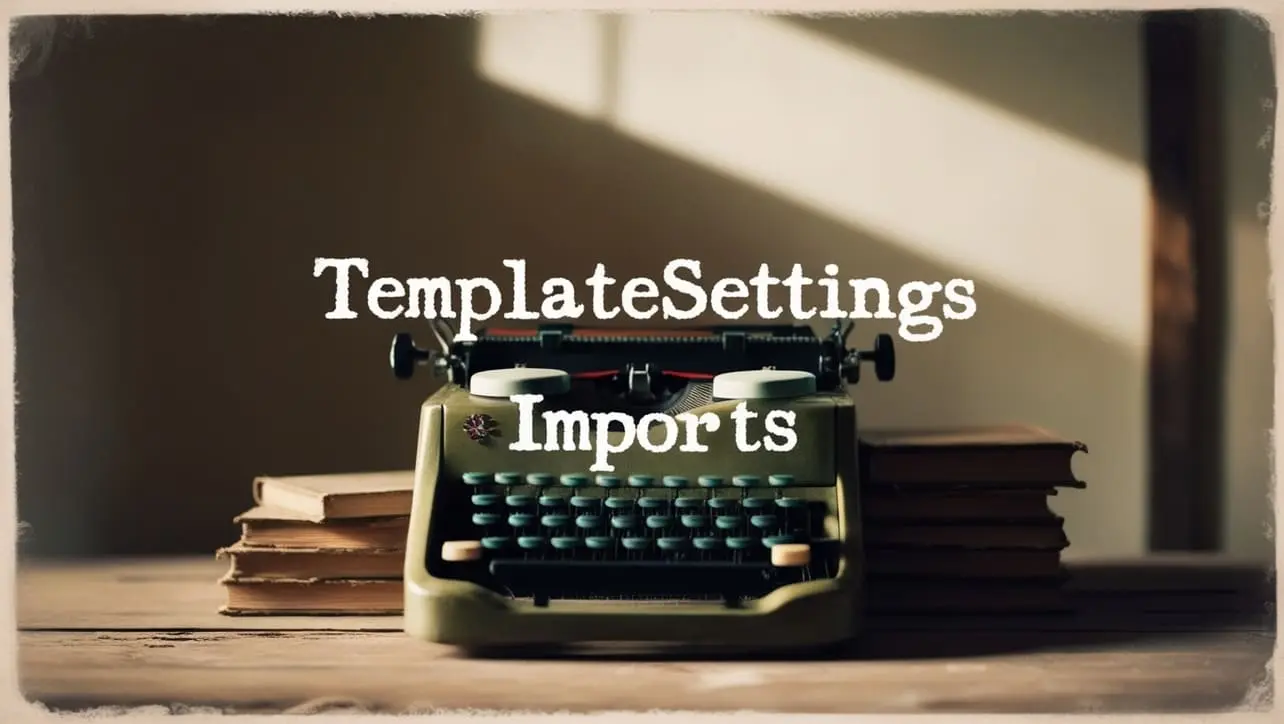
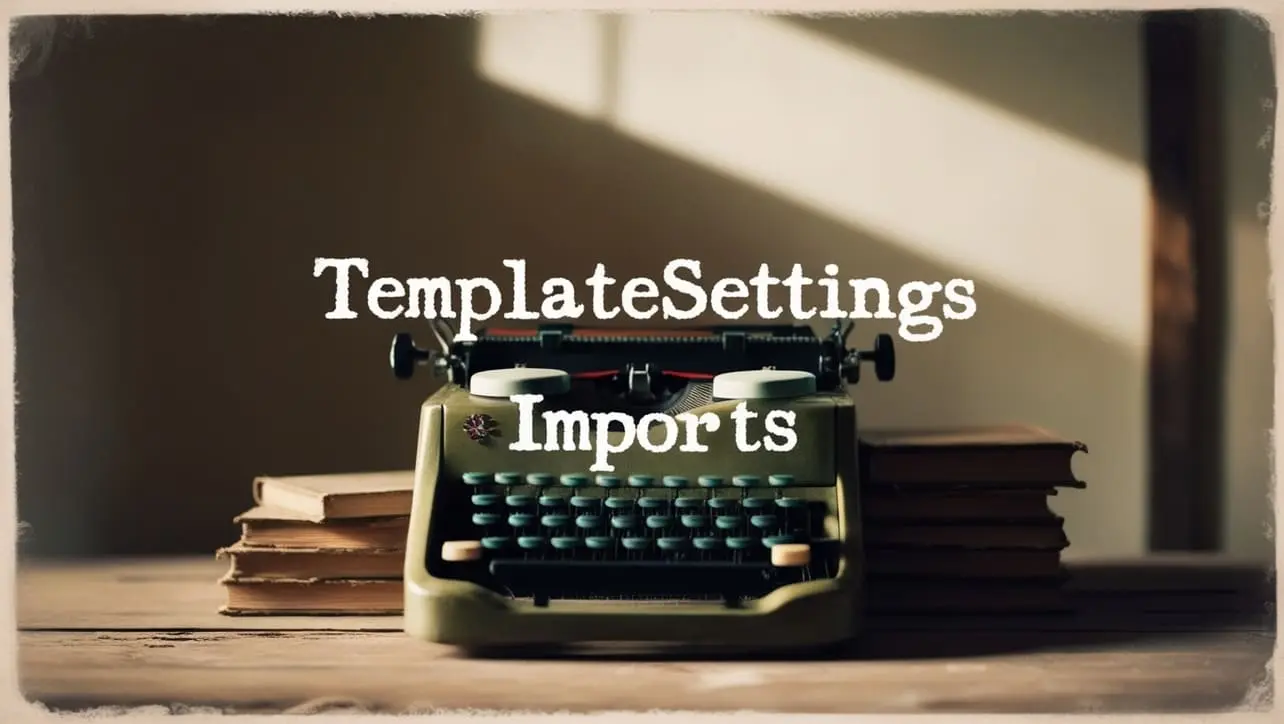
Lodash _.templateSettings.imports Property
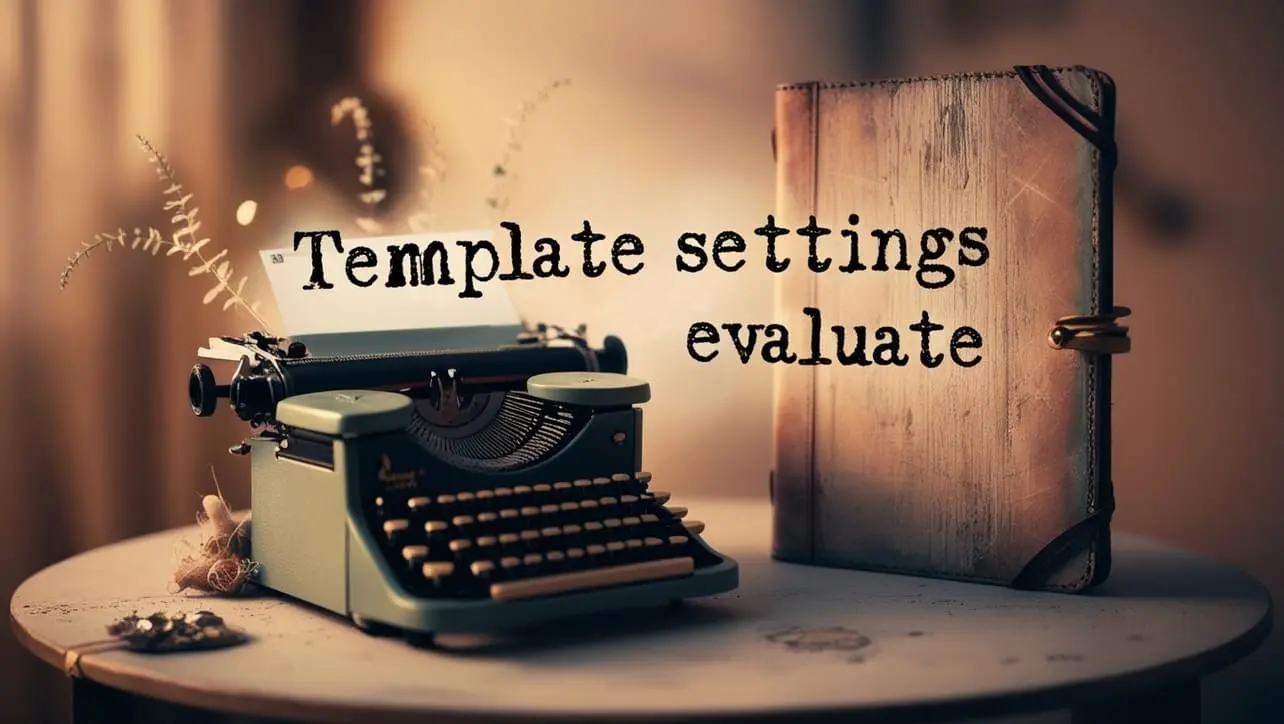
Lodash _.templateSettings.evaluate Property
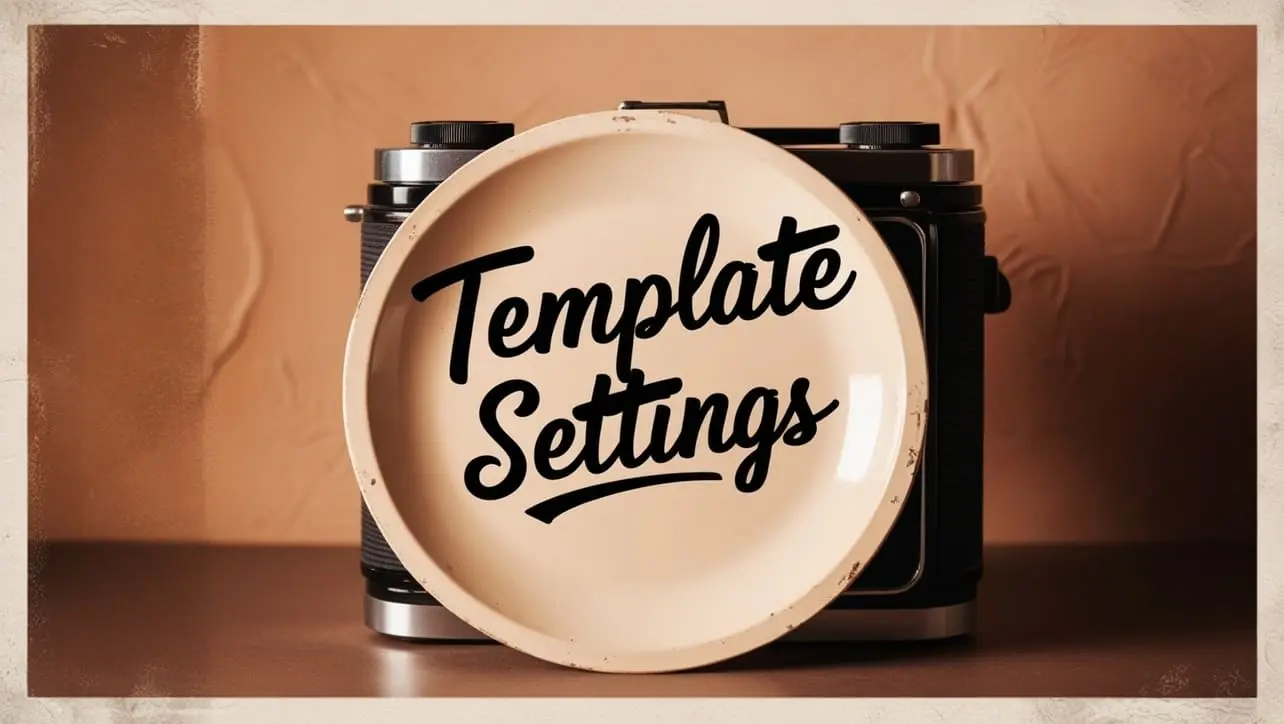
Lodash _.templateSettings Property
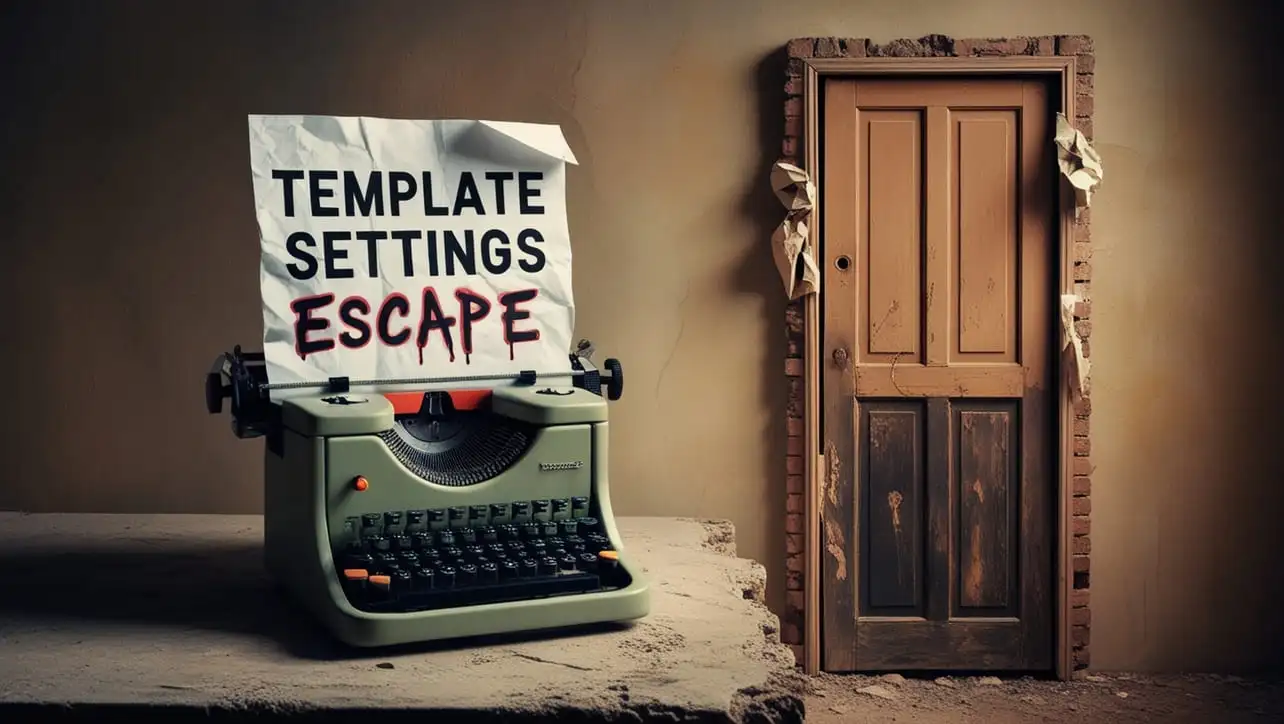
Lodash _.templateSettings.escape Property
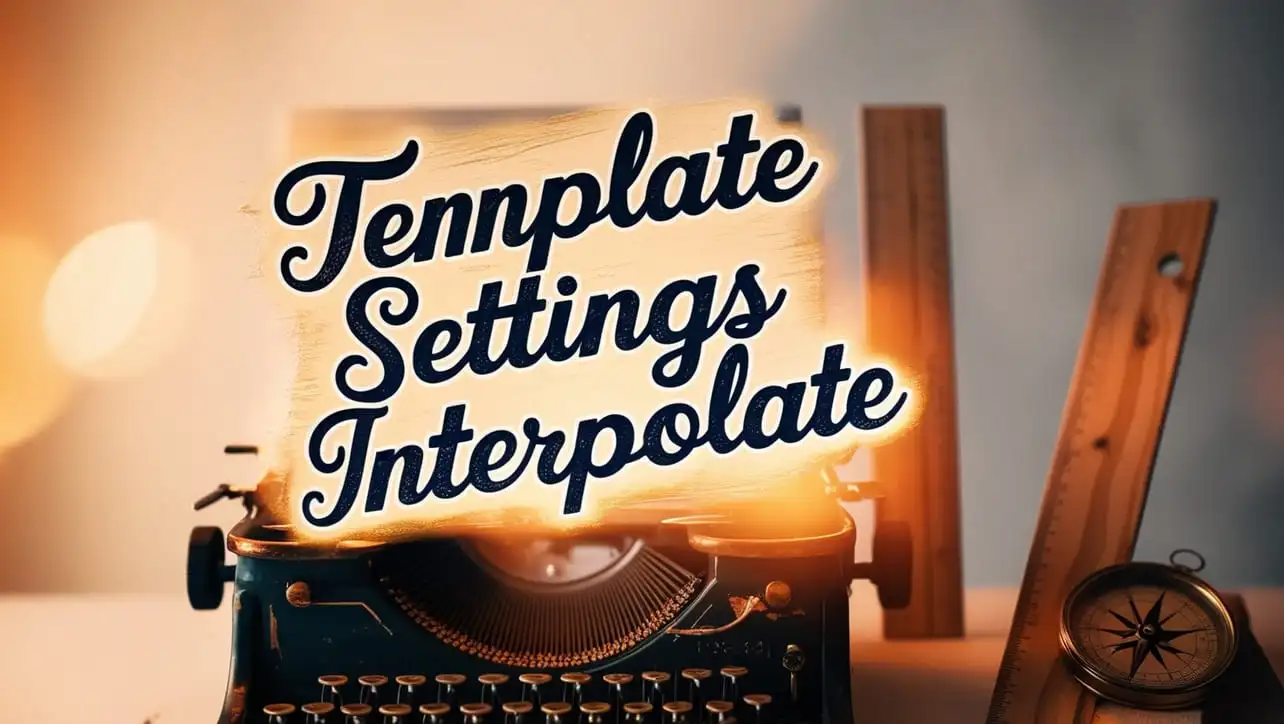
Lodash _.templateSettings.interpolate Property
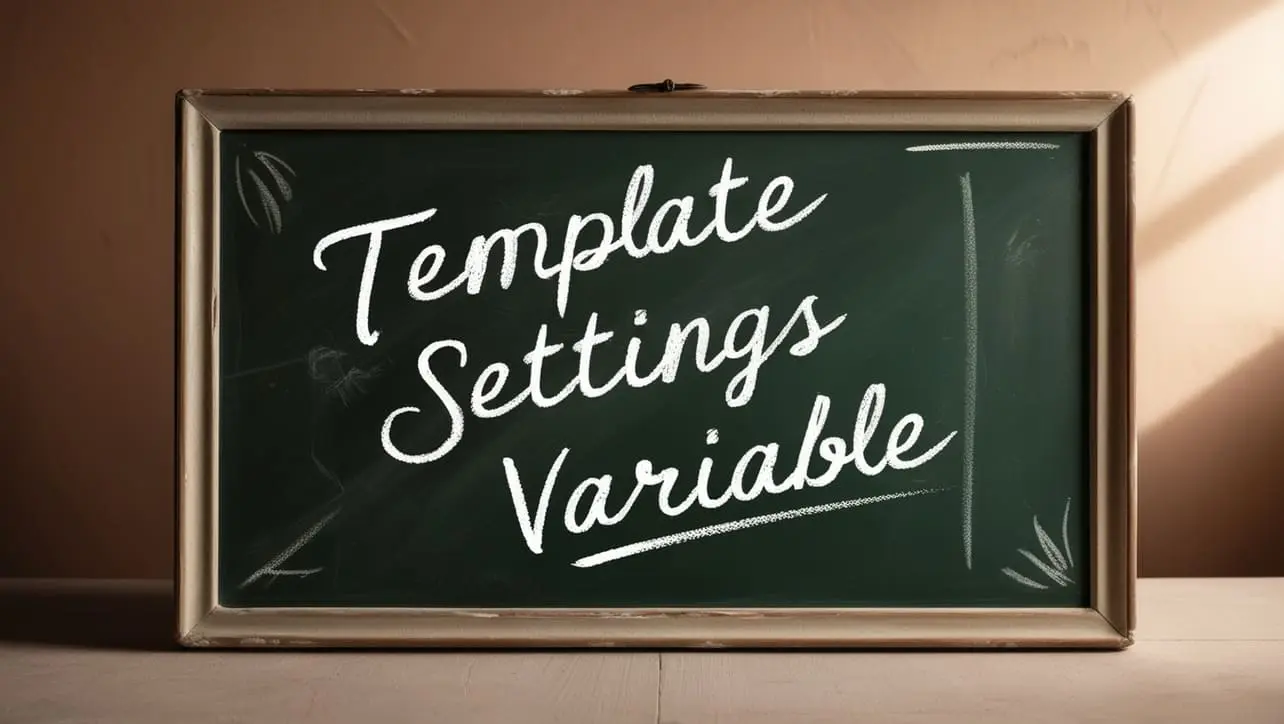
If you have any doubts regarding this article (Lodash _.overArgs() Function Method), please comment here. I will help you immediately.