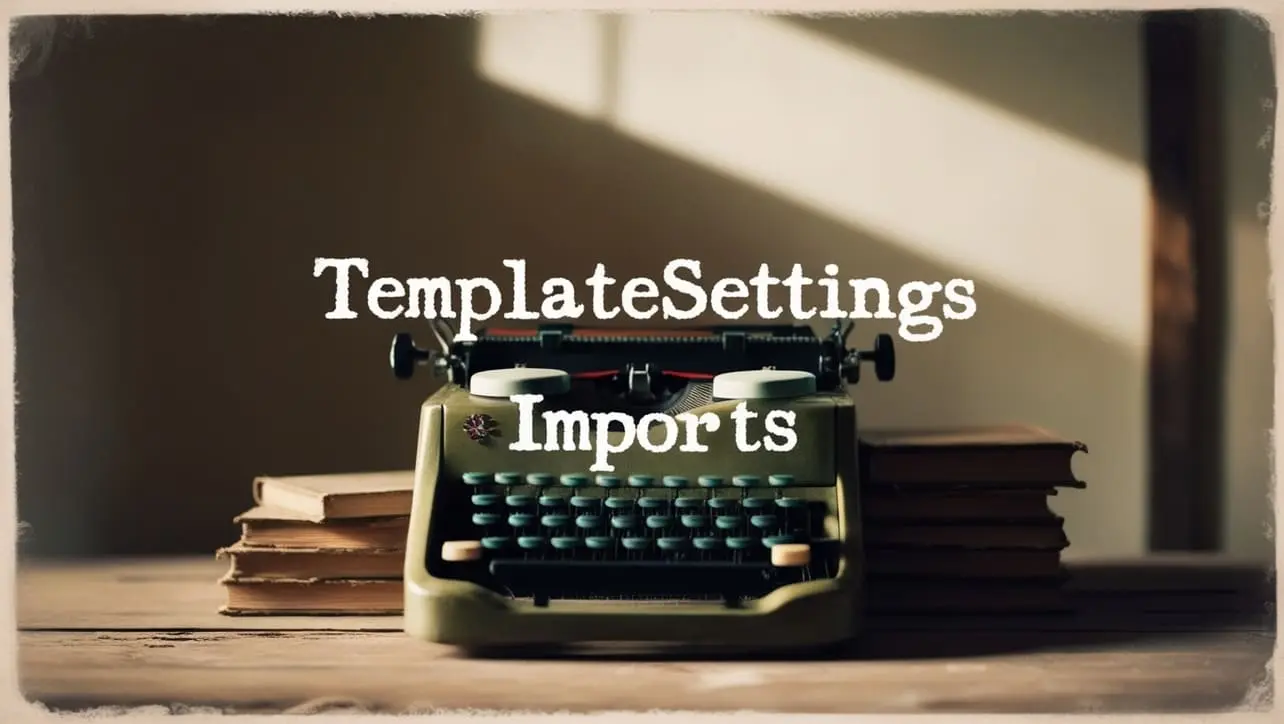
Lodash _.negate() Function Method
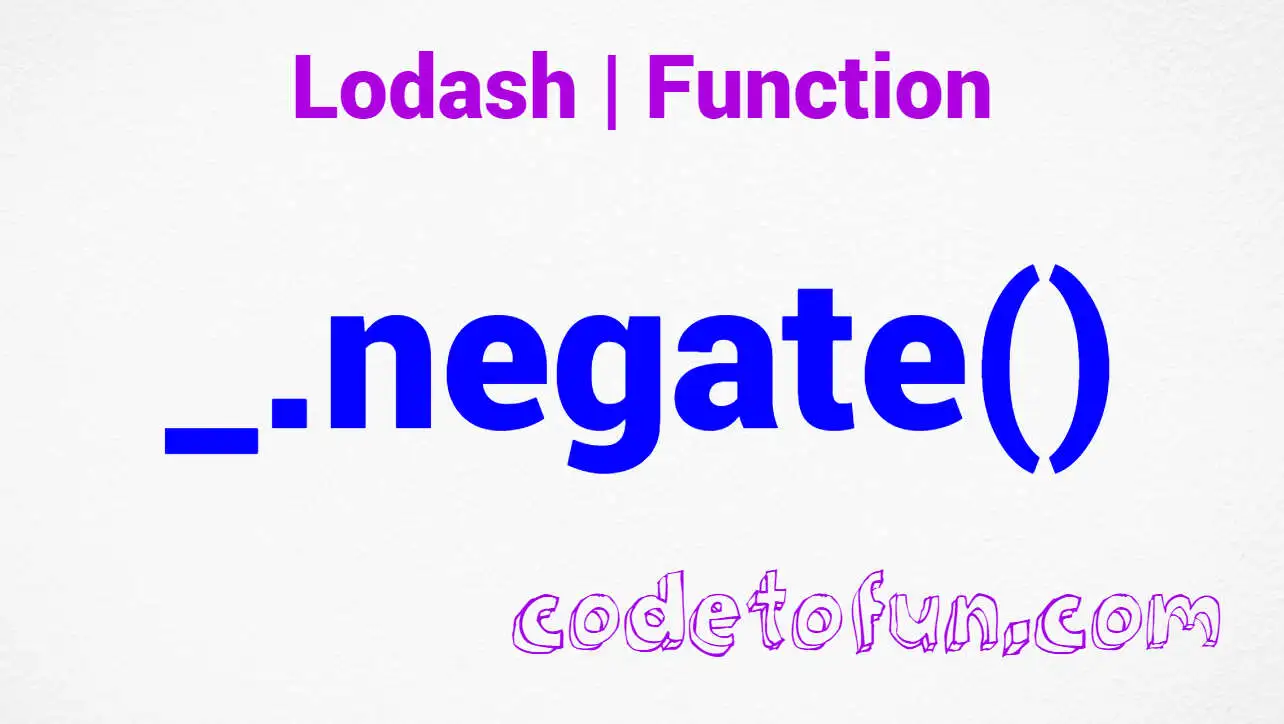
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, effective manipulation of functions is crucial for building robust and flexible applications. Lodash, a powerful utility library, offers a variety of methods to streamline these operations. One such method is _.negate()
, a function utility that creates a negated version of a predicate function.
This method proves invaluable when working with conditions and logical operations in your JavaScript code.
🧠 Understanding _.negate() Method
The _.negate()
function method in Lodash is designed to create a new function that negates the result of the provided predicate function. This means that if the original function returns true, the negated function will return false, and vice versa. This utility is particularly handy when you want to reverse the logic of a given condition or when composing multiple functions.
💡 Syntax
The syntax for the _.negate()
method is straightforward:
_.negate(predicate)
- predicate: The predicate function to negate.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.negate()
method:
const _ = require('lodash');
const isEven = num => num % 2 === 0;
const isOdd = _.negate(isEven);
console.log(isEven(4)); // Output: true
console.log(isOdd(4)); // Output: false
In this example, the isEven function checks if a number is even, and isOdd is the negated version using _.negate()
. It reverses the logic, checking if a number is not even.
🏆 Best Practices
When working with the _.negate()
method, consider the following best practices:
Understanding Predicate Functions:
Ensure the predicate function you intend to negate returns a boolean value.
_.negate()
is designed to work with functions that produce boolean results.example.jsCopiedconst isPositive = num => num > 0; const isNotPositive = _.negate(isPositive); console.log(isPositive(5)); // Output: true console.log(isNotPositive(5)); // Output: false
Composing Functions:
Explore the power of function composition by combining
_.negate()
with other functions. This allows you to build complex conditions in a more readable and modular way.example.jsCopiedconst isPositive = num => num > 0; const isEven = num => num % 2 === 0; const isNotPositiveAndEven = _.negate(_.overSome([isPositive, isEven])); console.log(isNotPositiveAndEven(6)); // Output: false
Reversing Conditions:
Use
_.negate()
to easily reverse the conditions in your code, making it more intuitive and expressive.example.jsCopiedconst hasPermission = user => user.role === 'admin'; const lacksPermission = _.negate(hasPermission); console.log(hasPermission({ role: 'admin' })); // Output: true console.log(lacksPermission({ role: 'admin' })); // Output: false
📚 Use Cases
Conditional Rendering:
In scenarios where you want to conditionally render elements based on the negation of a certain condition,
_.negate()
proves to be a concise and expressive solution.example.jsCopiedconst isVisible = /* ... some condition ... */; const isHidden = _.negate(isVisible); // Use in UI rendering logic if (isHidden()) { // Render hidden element } else { // Render visible element }
Filtering Arrays:
When filtering arrays based on a condition,
_.negate()
can be used to easily switch between including and excluding elements.example.jsCopiedconst numbers = [1, 2, 3, 4, 5]; const isEven = num => num % 2 === 0; const oddNumbers = numbers.filter(_.negate(isEven)); console.log(oddNumbers); // Output: [1, 3, 5]
Validation Logic:
In validation scenarios, where you need to check if a condition is not met,
_.negate()
simplifies the logic.example.jsCopiedconst isEmailValid = /* ... some email validation logic ... */; const isEmailInvalid = _.negate(isEmailValid); // Use in form validation if (isEmailInvalid(userInputEmail)) { // Display error message }
🎉 Conclusion
The _.negate()
function method in Lodash is a valuable tool for simplifying conditional logic in your JavaScript code. Whether you're reversing conditions, composing functions, or filtering arrays, _.negate()
offers a concise and expressive way to handle boolean logic.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.negate()
method in your Lodash projects.
👨💻 Join our Community:
Author
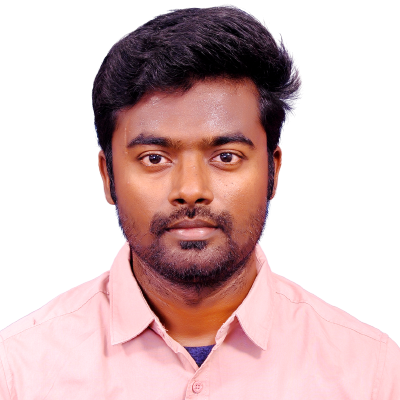
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
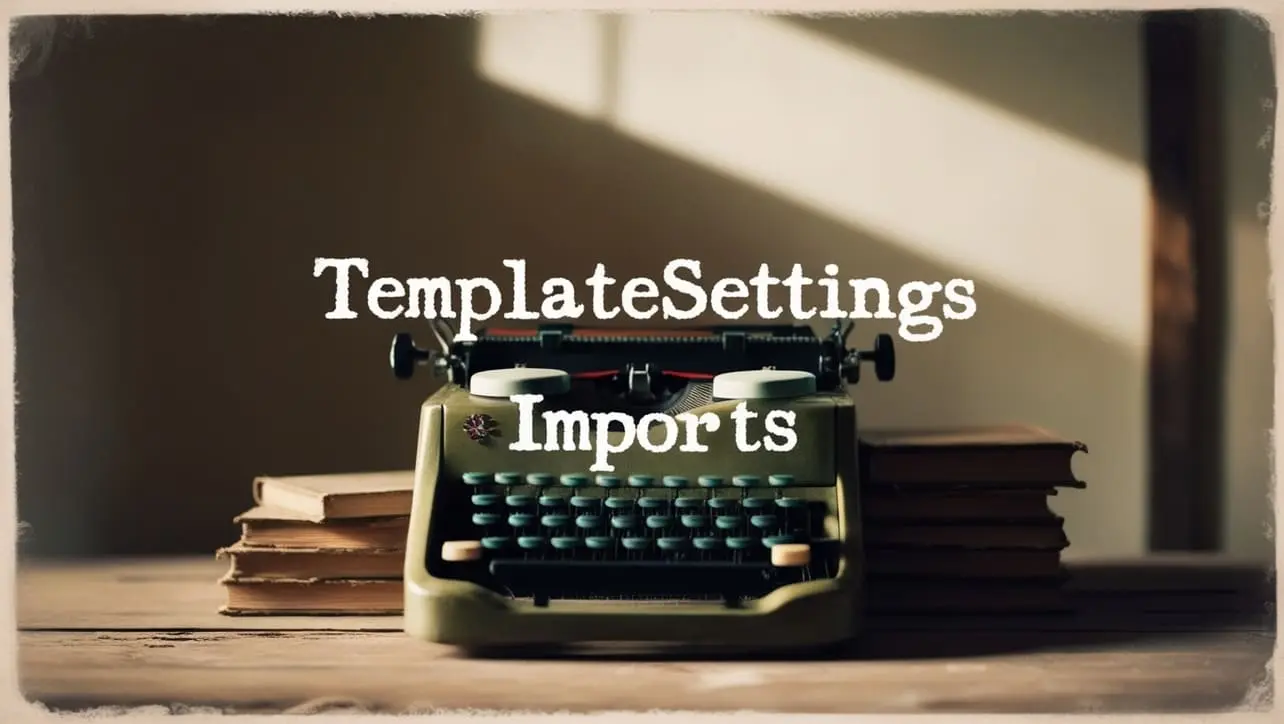
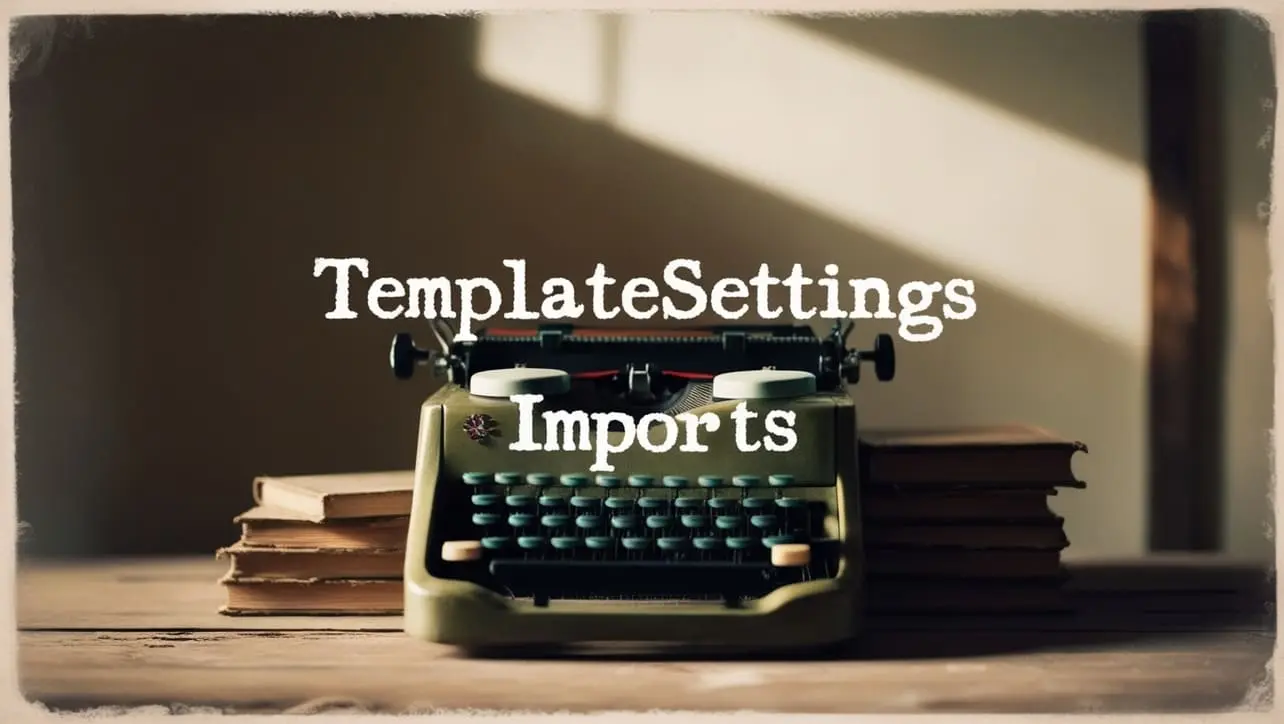
Lodash _.templateSettings.imports Property
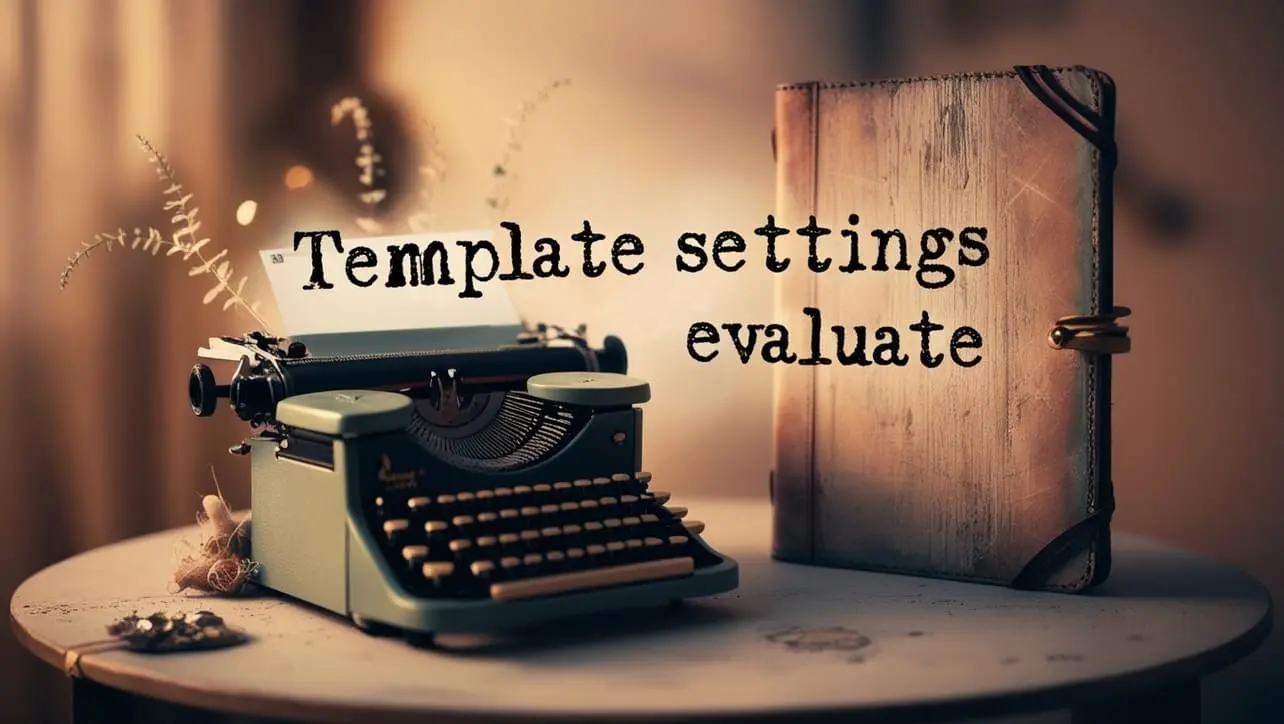
Lodash _.templateSettings.evaluate Property
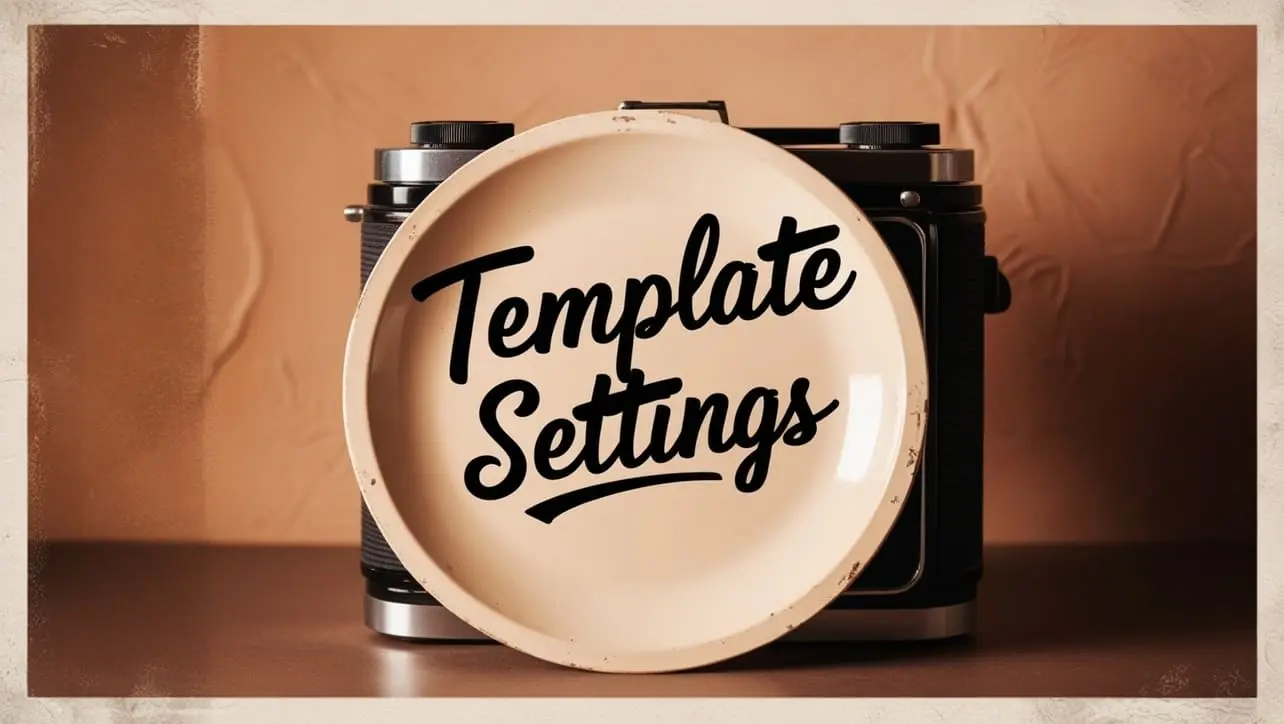
Lodash _.templateSettings Property
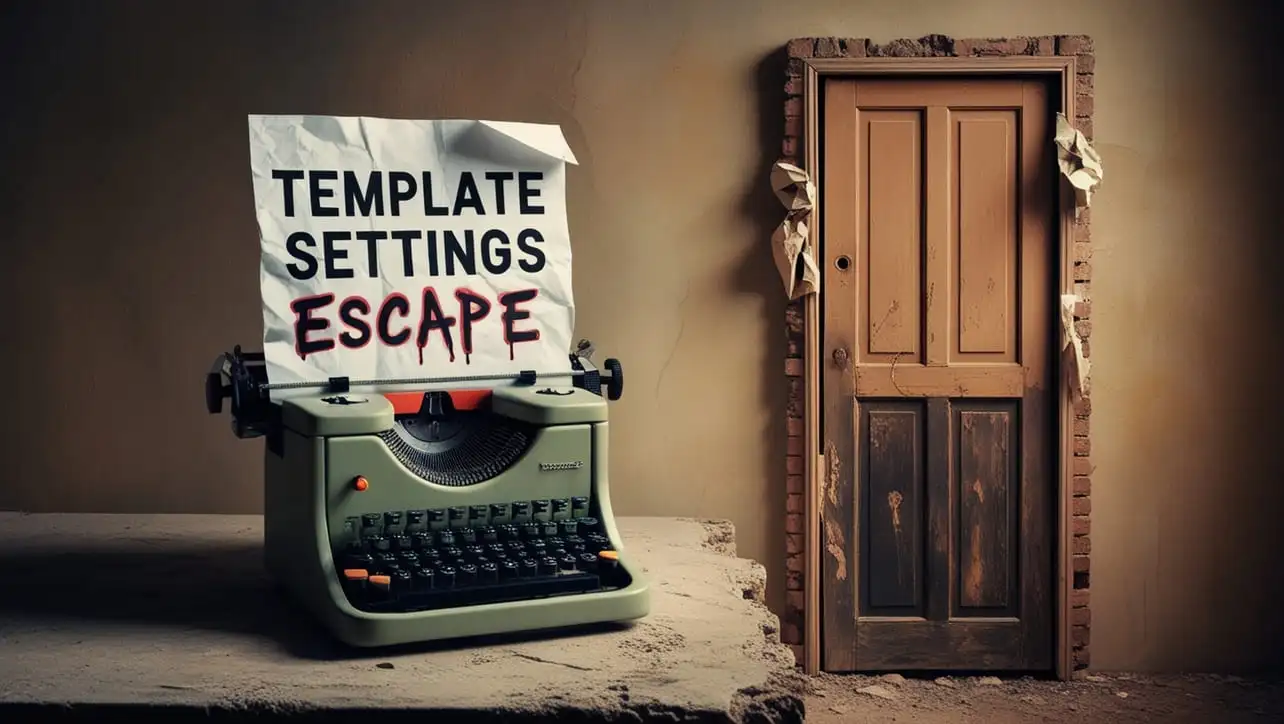
Lodash _.templateSettings.escape Property
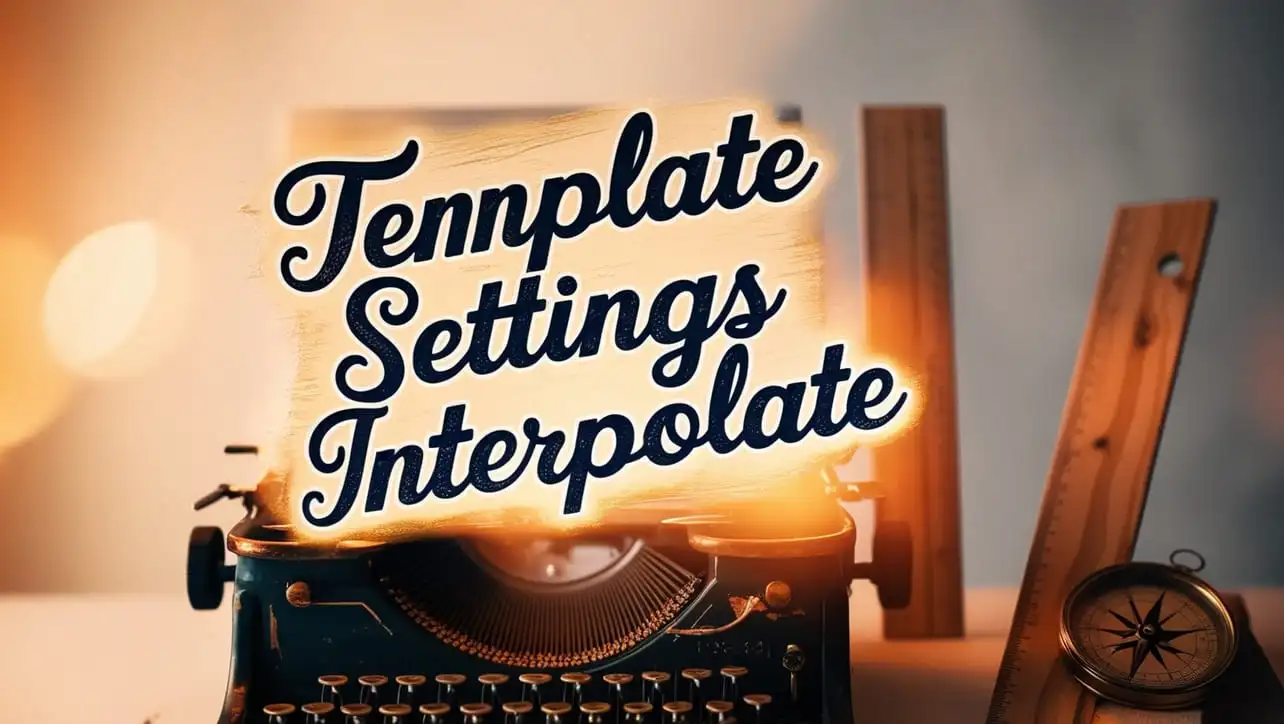
Lodash _.templateSettings.interpolate Property
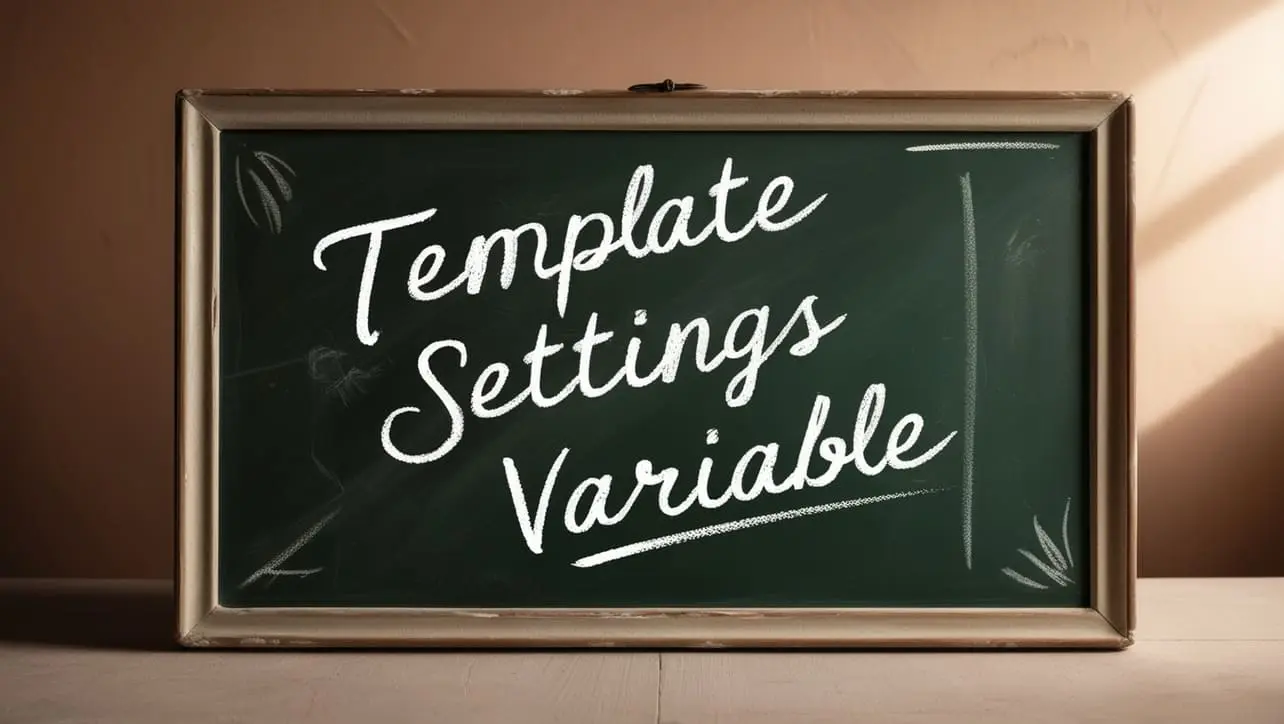
If you have any doubts regarding this article (Lodash _.negate() Function Method), please comment here. I will help you immediately.