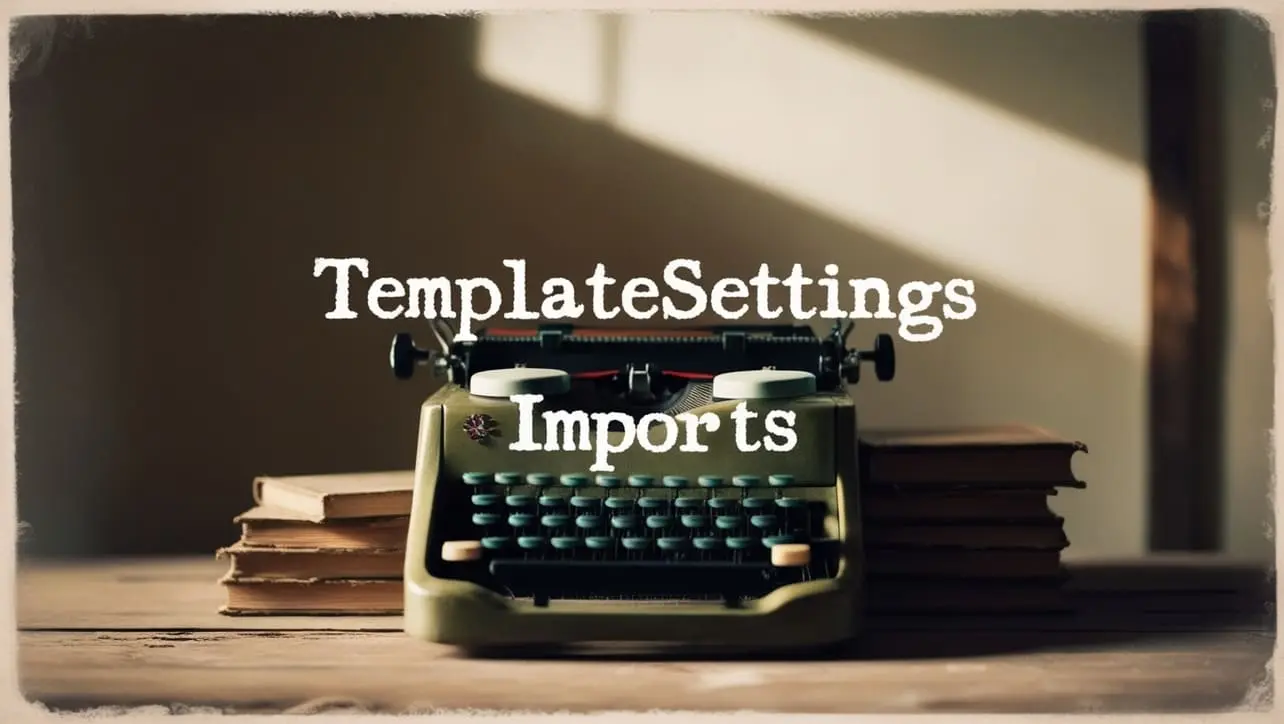
Lodash _.flip() Function Method
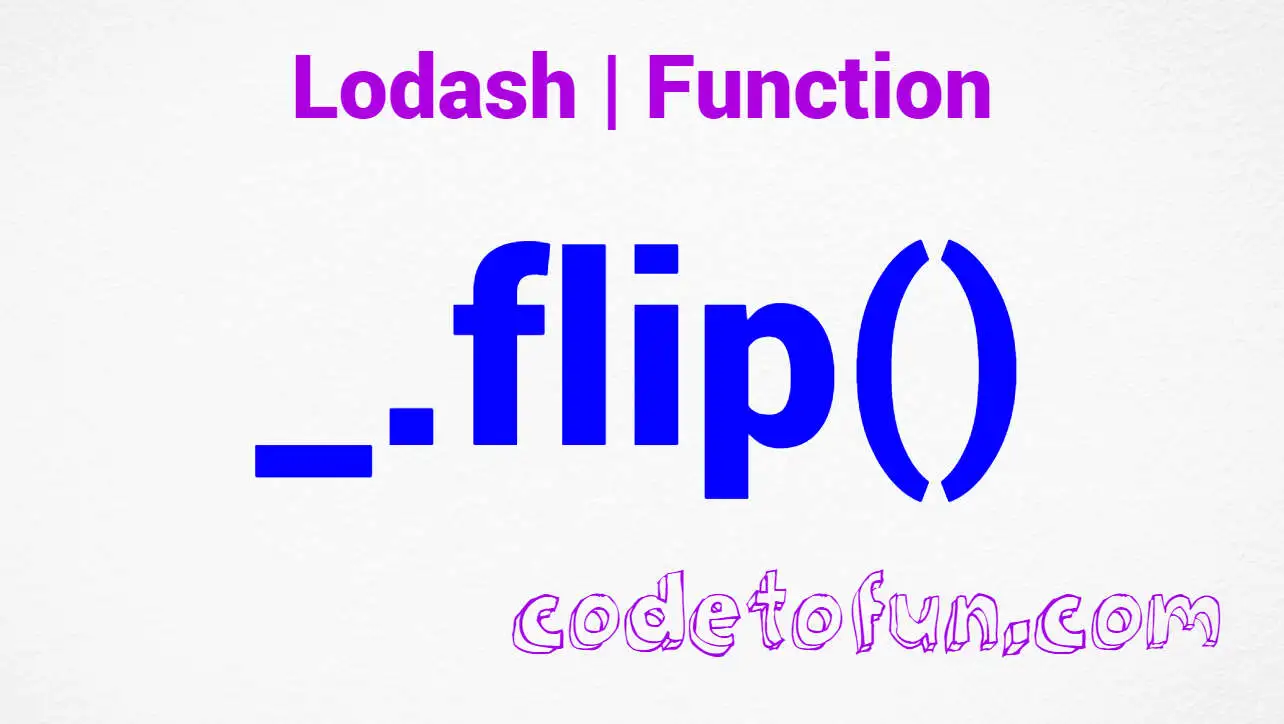
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, manipulating functions can be as crucial as manipulating data. Lodash, a powerful utility library, introduces the _.flip()
method, offering a seamless way to alter the order of arguments in a function.
This can be especially useful when adapting functions or creating new ones, providing flexibility and enhancing code readability.
🧠 Understanding _.flip() Method
The _.flip()
method in Lodash is designed to create a new function that flips the order of its arguments, providing a different perspective on function usage. This can simplify code, improve clarity, and reduce the need for custom functions or workarounds when dealing with various argument orders.
💡 Syntax
The syntax for the _.flip()
method is straightforward:
_.flip(func)
- func: The function to flip the arguments of.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.flip()
method:
const _ = require('lodash');
// Original function
const subtract = (a, b) => a - b;
// Create a new function with flipped arguments
const flippedSubtract = _.flip(subtract);
console.log(subtract(5, 3));
// Output: 2
console.log(flippedSubtract(5, 3));
// Output: -2
In this example, the subtract function subtracts the second argument from the first, and flippedSubtract is a new function created by flipping the arguments. This allows subtracting the first argument from the second.
🏆 Best Practices
When working with the _.flip()
method, consider the following best practices:
Understand Function Arity:
Be aware of the arity (number of arguments) of the original function when using
_.flip()
. Flipping the arguments works best when the original function has two arguments.example.jsCopiedconst multiply = (a, b, c) => a * b * c; // This won't work as expected, as the original function has three arguments const flippedMultiply = _.flip(multiply); console.log(flippedMultiply(2, 3, 4)); // Output: NaN
Adapt Existing Functions:
Consider using
_.flip()
to adapt existing functions with a different argument order, rather than creating entirely new functions.example.jsCopiedconst divide = (a, b) => a / b; // Adapt the existing function for division with flipped arguments const divideFlipped = _.flip(divide); console.log(divide(10, 2)); // Output: 5 console.log(divideFlipped(10, 2)); // Output: 0.2
📚 Use Cases
Custom Argument Orders:
When dealing with functions that have fixed argument orders,
_.flip()
allows you to create variations with custom argument orders.example.jsCopiedconst greet = (greeting, name) => `${greeting}, ${name}!`; // Create a new function with flipped arguments for a different order const greetFlipped = _.flip(greet); console.log(greet('Hello', 'John')); // Output: Hello, John! console.log(greetFlipped('Hello', 'John')); // Output: John, Hello!
Enhancing Function Compositions:
When composing functions,
_.flip()
can be used to seamlessly integrate functions with different argument orders.example.jsCopiedconst add = (a, b) => a + b; const subtractFlipped = _.flip(subtract); // Compose the original function and the flipped function const composedFunction = _.flow(subtractFlipped, add); console.log(composedFunction(5, 3)); // Output: 8
🎉 Conclusion
The _.flip()
method in Lodash provides a versatile solution for altering the order of arguments in functions, offering flexibility and enhancing code readability. Whether you need to adapt existing functions, create variations with custom argument orders, or enhance function compositions, _.flip()
opens up new possibilities in function manipulation within your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.flip()
method in your Lodash projects.
👨💻 Join our Community:
Author
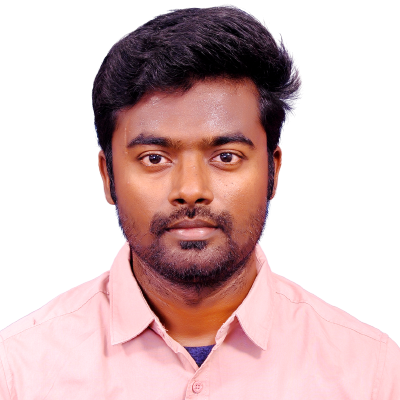
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
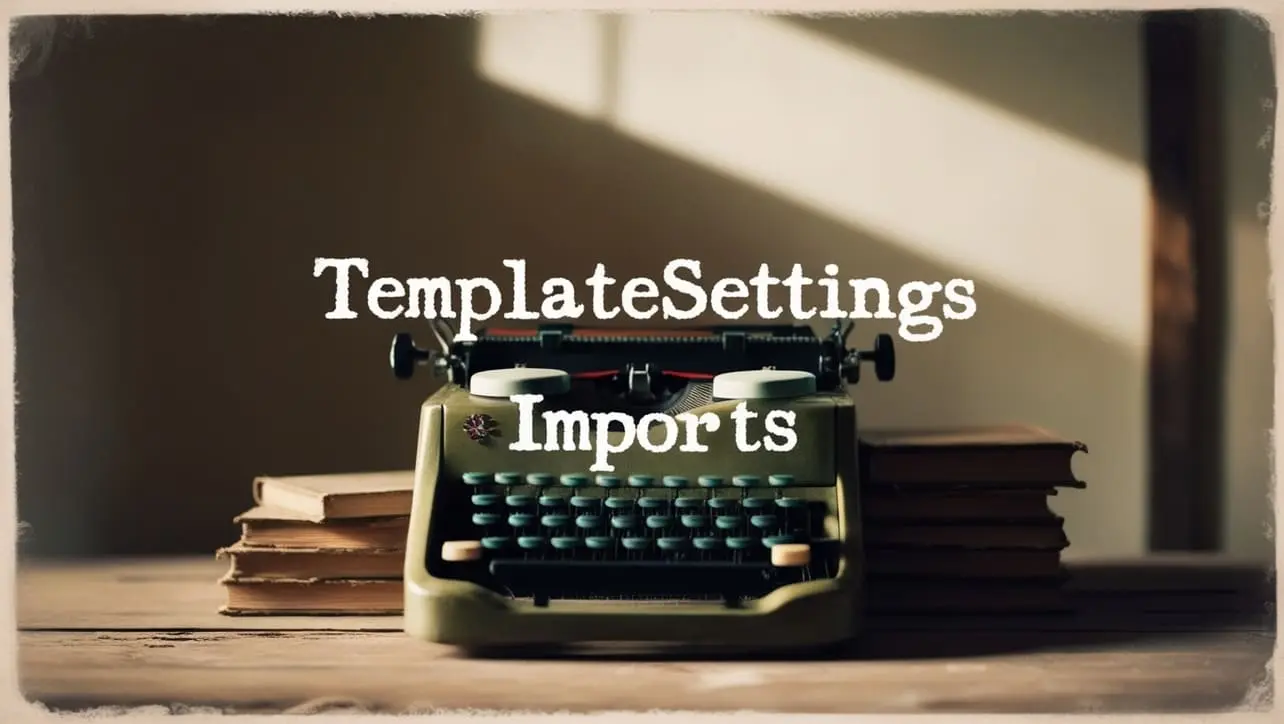
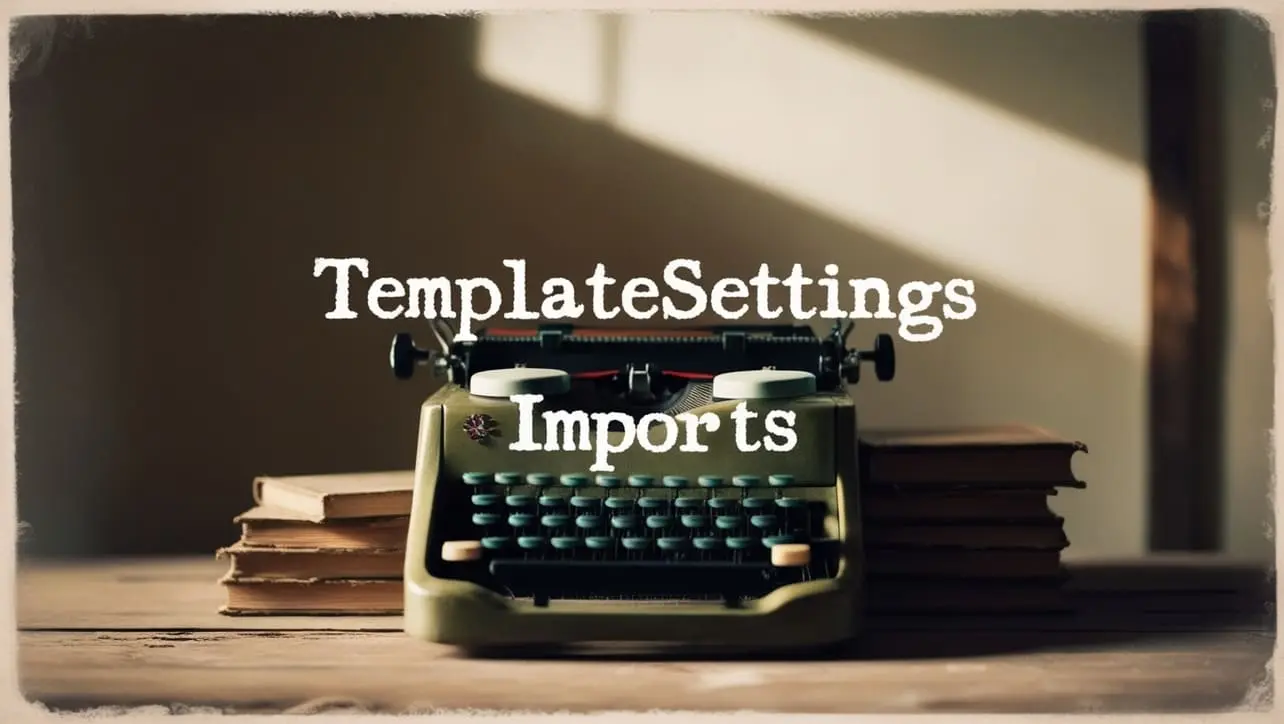
Lodash _.templateSettings.imports Property
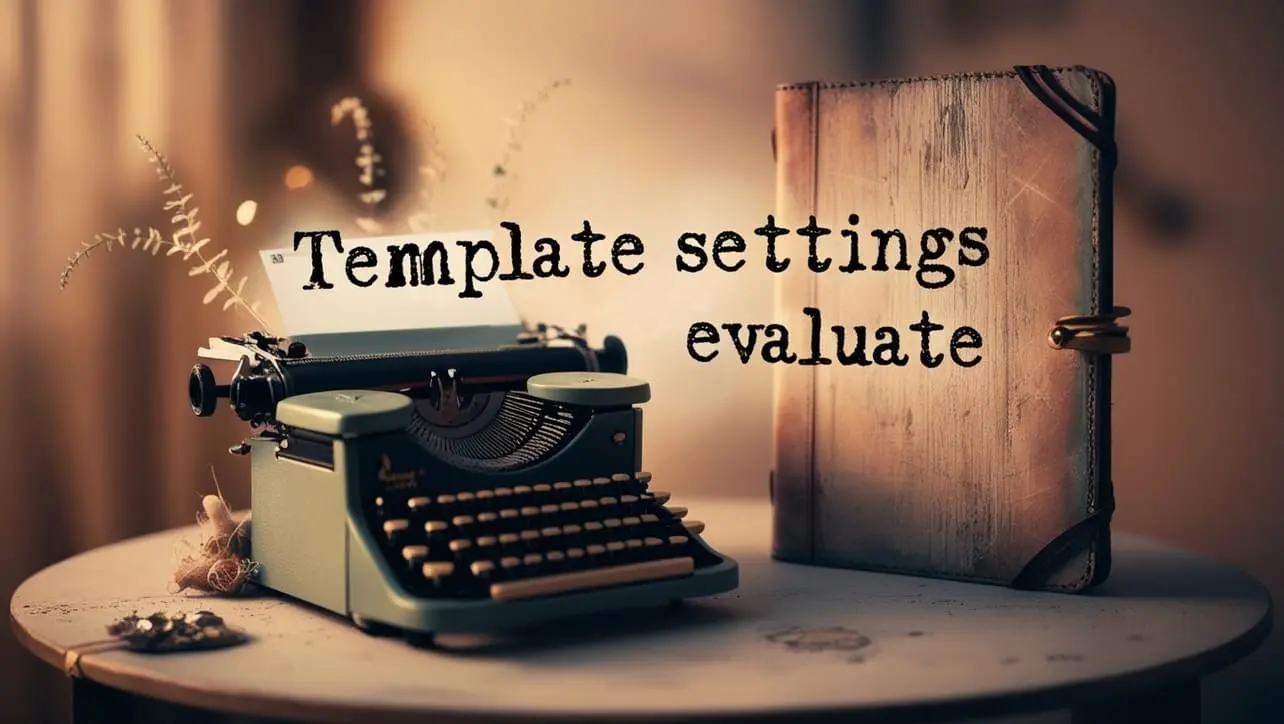
Lodash _.templateSettings.evaluate Property
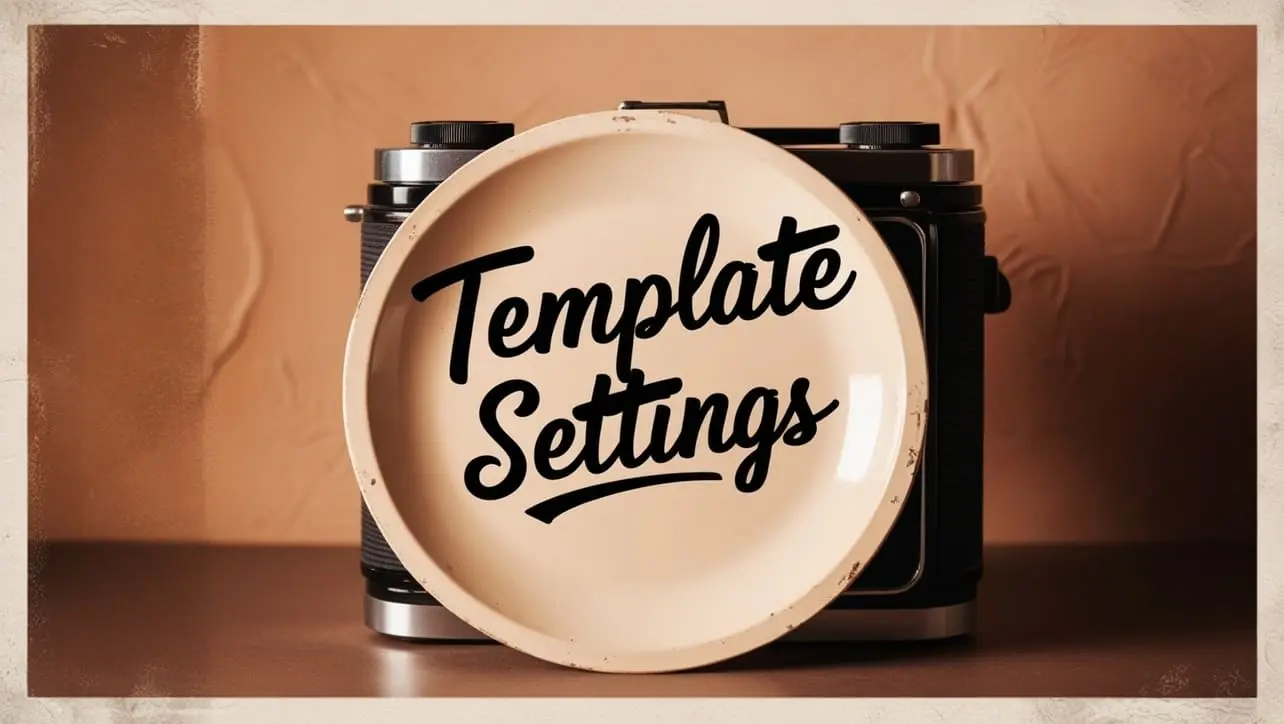
Lodash _.templateSettings Property
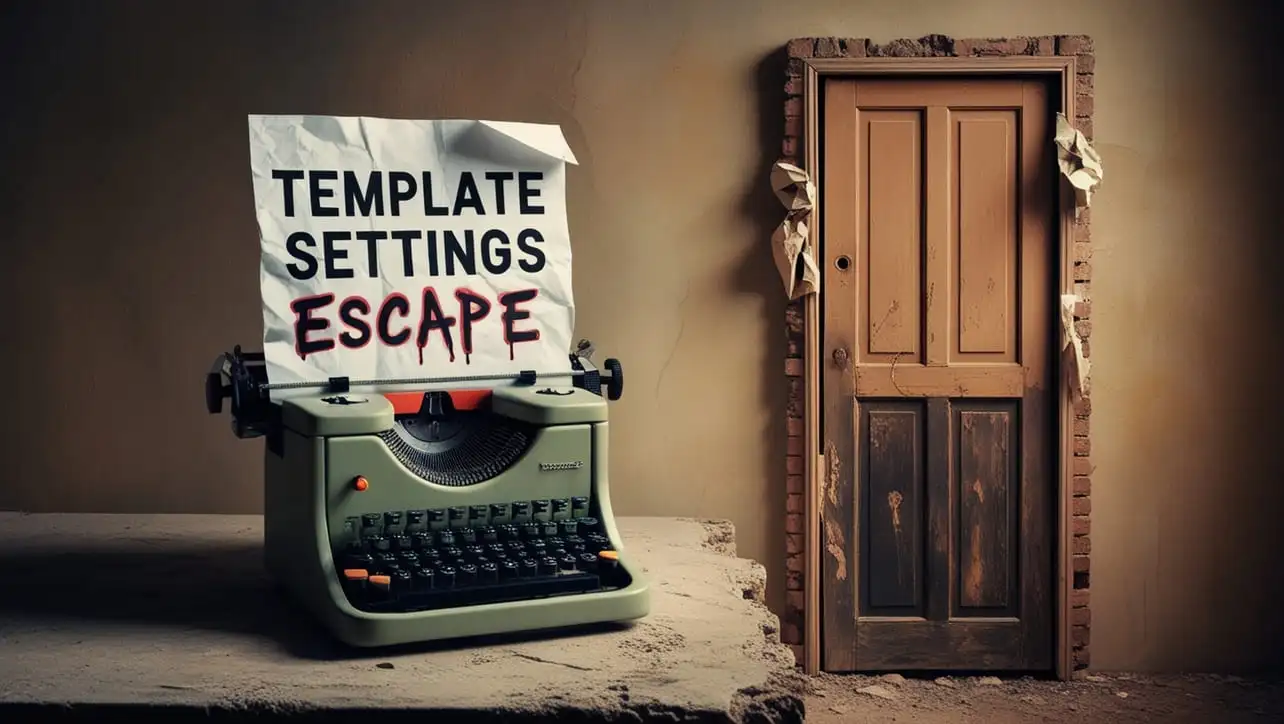
Lodash _.templateSettings.escape Property
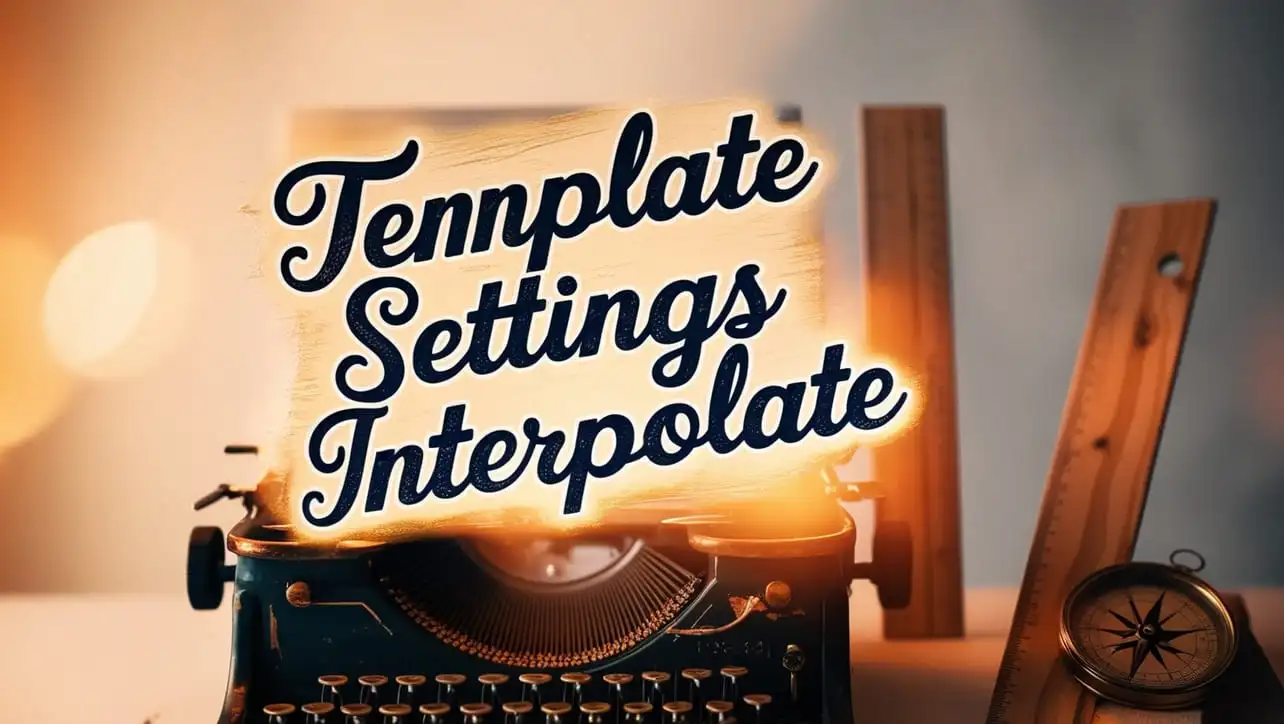
Lodash _.templateSettings.interpolate Property
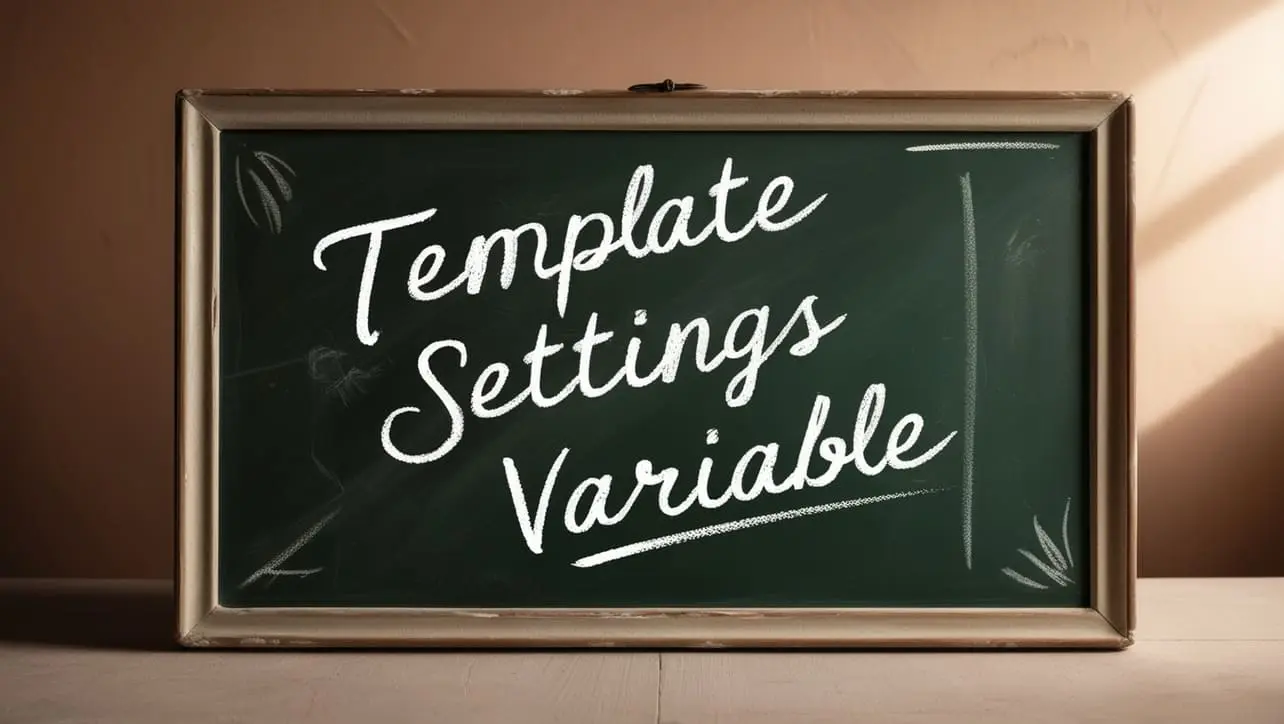
If you have any doubts regarding this article (Lodash _.flip() Function Method), please comment here. I will help you immediately.