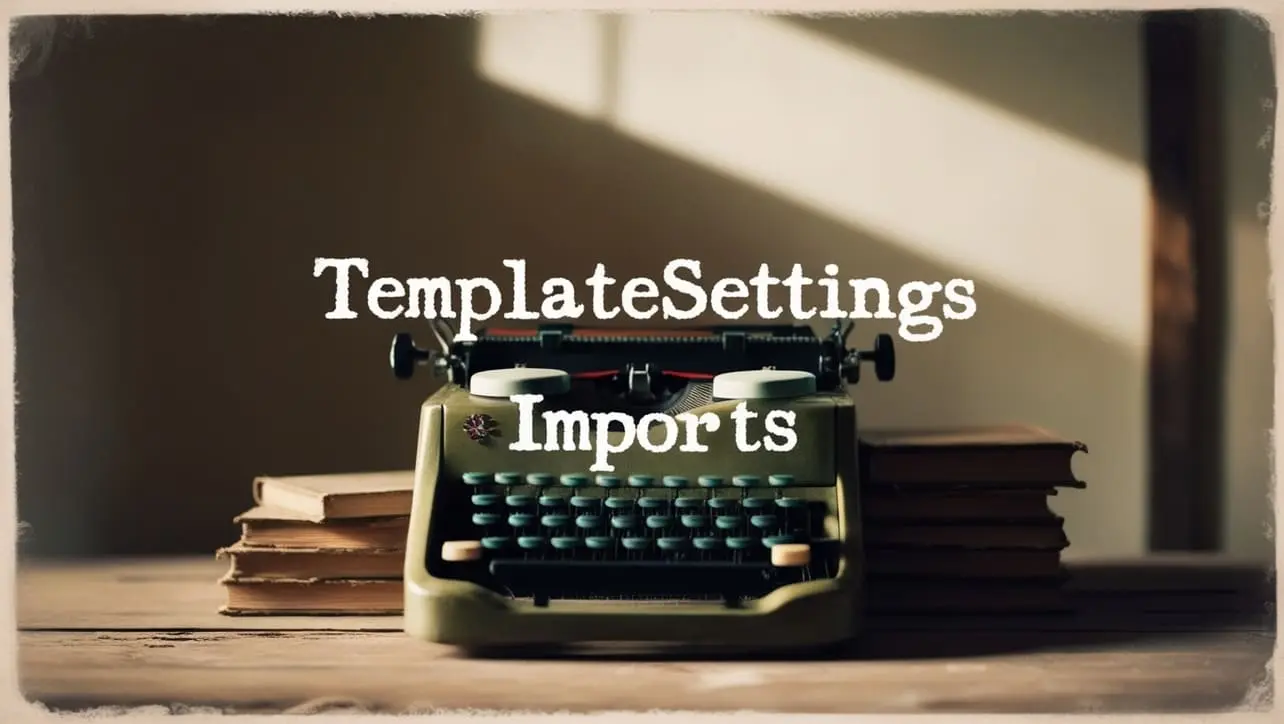
Lodash _.debounce() Function Method
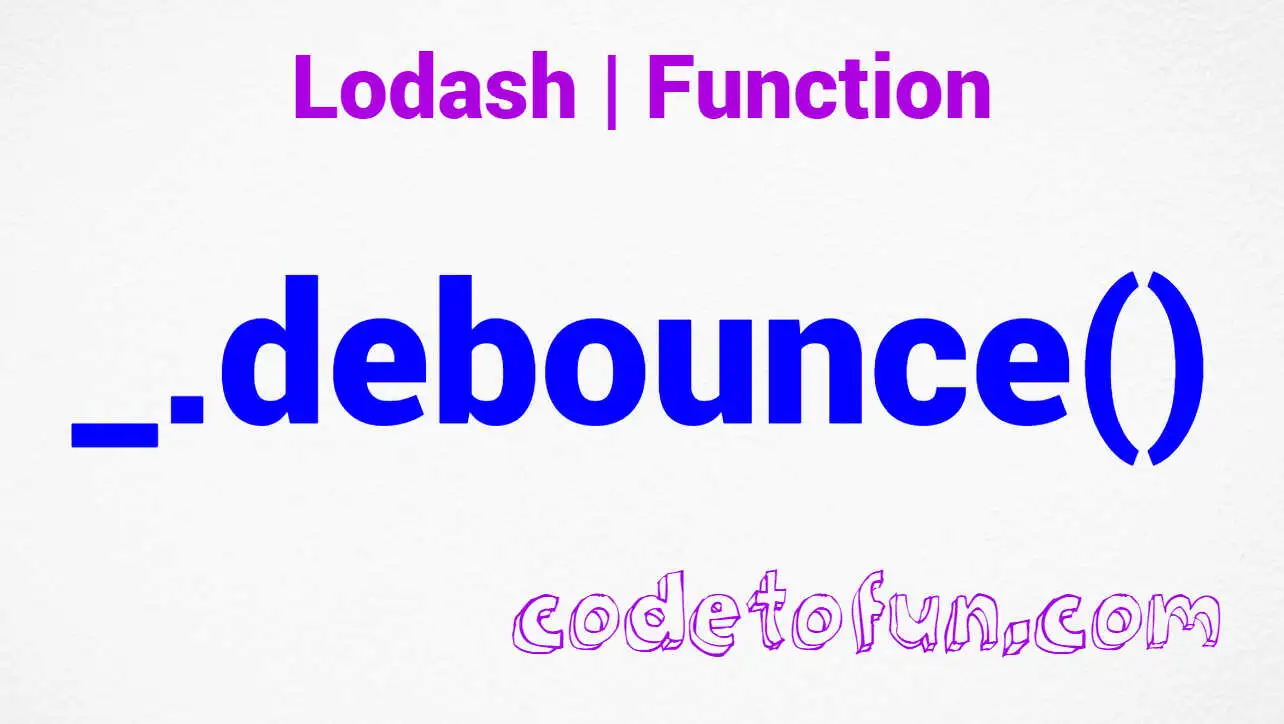
Photo Credit to CodeToFun
🙋 Introduction
Efficiency is key in the world of JavaScript, especially when dealing with functions that are triggered frequently, such as event handlers. Lodash, a robust utility library, provides a solution to this challenge with the _.debounce()
function method.
This method allows developers to control the rate at which a function is executed, preventing it from being called too often and potentially improving performance.
🧠 Understanding _.debounce() Method
The _.debounce()
function in Lodash creates a debounced function that delays invoking the original function until after a specified number of milliseconds have elapsed since the last time the debounced function was invoked. This is particularly useful in scenarios like handling user input or window resize events where you want to wait for a pause in activity before triggering the function.
💡 Syntax
The syntax for the _.debounce()
method is straightforward:
_.debounce(func, [wait=0], [options={}])
- func: The function to debounce.
- wait: The number of milliseconds to delay. Default is 0.
- options: Additional options to customize the debouncing behavior.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.debounce()
method:
const _ = require('lodash');
// Original function to be debounced
const searchFunction = (query) => {
// Perform search operation with the query
console.log(`Searching for: ${query}`);
};
// Debounce the search function with a delay of 500 milliseconds
const debouncedSearch = _.debounce(searchFunction, 500);
// Simulate user input triggering the debounced search
debouncedSearch('Lodash'); // This call will be delayed by 500 milliseconds
debouncedSearch('JavaScript'); // Only this call will be executed after the delay
In this example, the searchFunction is debounced using _.debounce()
with a delay of 500 milliseconds. The debounced function (debouncedSearch) is then called multiple times in quick succession, but the actual search operation only occurs once after the specified delay.
🏆 Best Practices
When working with the _.debounce()
method, consider the following best practices:
Controlling Execution Frequency:
Use
_.debounce()
when you want to control how frequently a function is executed, especially in scenarios like handling user input or window resize events. Adjust the wait parameter to fine-tune the delay.example.jsCopiedconst handleInput = (value) => { // Process input value (e.g., update UI or make API calls) console.log(`Input value: ${value}`); }; // Debounce the input handler with a delay of 300 milliseconds const debouncedInputHandler = _.debounce(handleInput, 300); // Simulate rapid input changes debouncedInputHandler('A'); debouncedInputHandler('AB'); debouncedInputHandler('ABC'); // Only the last call will be executed after a 300-millisecond pause
Optimal Delay Selection:
Experiment with different delay values to find the optimal balance between responsiveness and efficiency. Consider the nature of the task being performed and the expected user experience.
example.jsCopiedconst expensiveOperation = () => { // Perform computationally expensive operation console.log('Executing expensive operation'); }; // Debounce the expensive operation with a delay of 1000 milliseconds const debouncedExpensiveOperation = _.debounce(expensiveOperation, 1000); // Trigger the operation multiple times debouncedExpensiveOperation(); debouncedExpensiveOperation(); debouncedExpensiveOperation(); // The operation will only be executed once after a 1000-millisecond pause
Canceling Debounced Calls:
Use the cancel() method provided by the debounced function to cancel pending debounced calls. This can be useful in scenarios where you want to reset the debounce logic.
example.jsCopiedconst delayedTask = () => { // Perform a delayed task console.log('Executing delayed task'); }; // Debounce the task with a delay of 500 milliseconds const debouncedTask = _.debounce(delayedTask, 500); // Trigger the debounced task debouncedTask(); // Cancel the debounced call (no execution will occur) debouncedTask.cancel();
📚 Use Cases
Search Input:
Implementing a search functionality where you want to wait for users to finish typing before triggering the actual search operation.
example.jsCopiedconst searchInputHandler = (query) => { // Perform search operation with the query console.log(`Searching for: ${query}`); }; // Debounce the search input handler with a delay of 800 milliseconds const debouncedSearchInput = _.debounce(searchInputHandler, 800); // Attach the debounced handler to the search input field document.getElementById('searchInput').addEventListener('input', (event) => { debouncedSearchInput(event.target.value); });
Window Resize Events:
Managing window resize events to update the UI layout but only after a brief pause to avoid unnecessary recalculations.
example.jsCopiedconst handleResize = () => { // Update UI layout or perform responsive actions console.log('Handling window resize'); }; // Debounce the window resize handler with a delay of 500 milliseconds const debouncedResizeHandler = _.debounce(handleResize, 500); // Attach the debounced handler to the window resize event window.addEventListener('resize', debouncedResizeHandler);
Auto-Saving Form Data:
Implementing auto-saving functionality for form data but delaying the actual save operation to avoid frequent API calls.
example.jsCopiedconst autoSaveForm = (formData) => { // Perform auto-save operation with the form data console.log('Auto-saving form data:', formData); }; // Debounce the auto-save function with a delay of 1000 milliseconds const debouncedAutoSave = _.debounce(autoSaveForm, 1000); // Attach the debounced handler to form input events document.getElementById('formInput').addEventListener('input', (event) => { debouncedAutoSave(event.target.value); });
🎉 Conclusion
The _.debounce()
function method in Lodash is a valuable tool for controlling the execution frequency of functions, especially in scenarios where responsiveness and efficiency are crucial. By strategically debouncing functions, developers can enhance user experience and optimize performance in various applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.debounce()
method in your Lodash projects.
👨💻 Join our Community:
Author
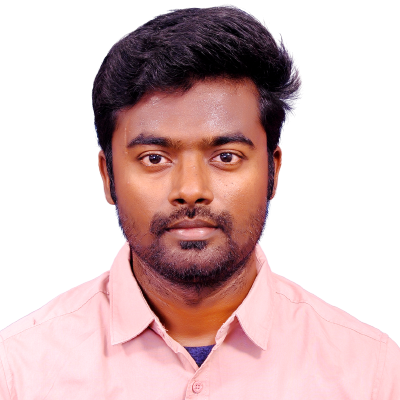
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
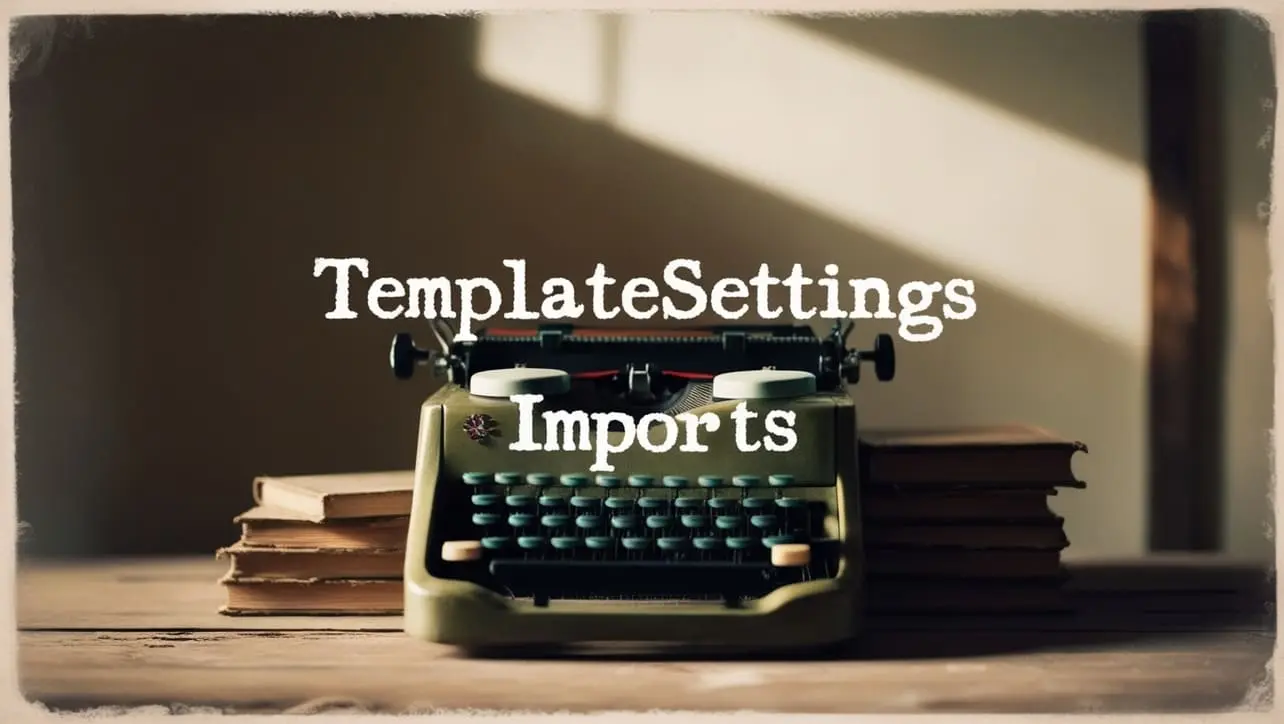
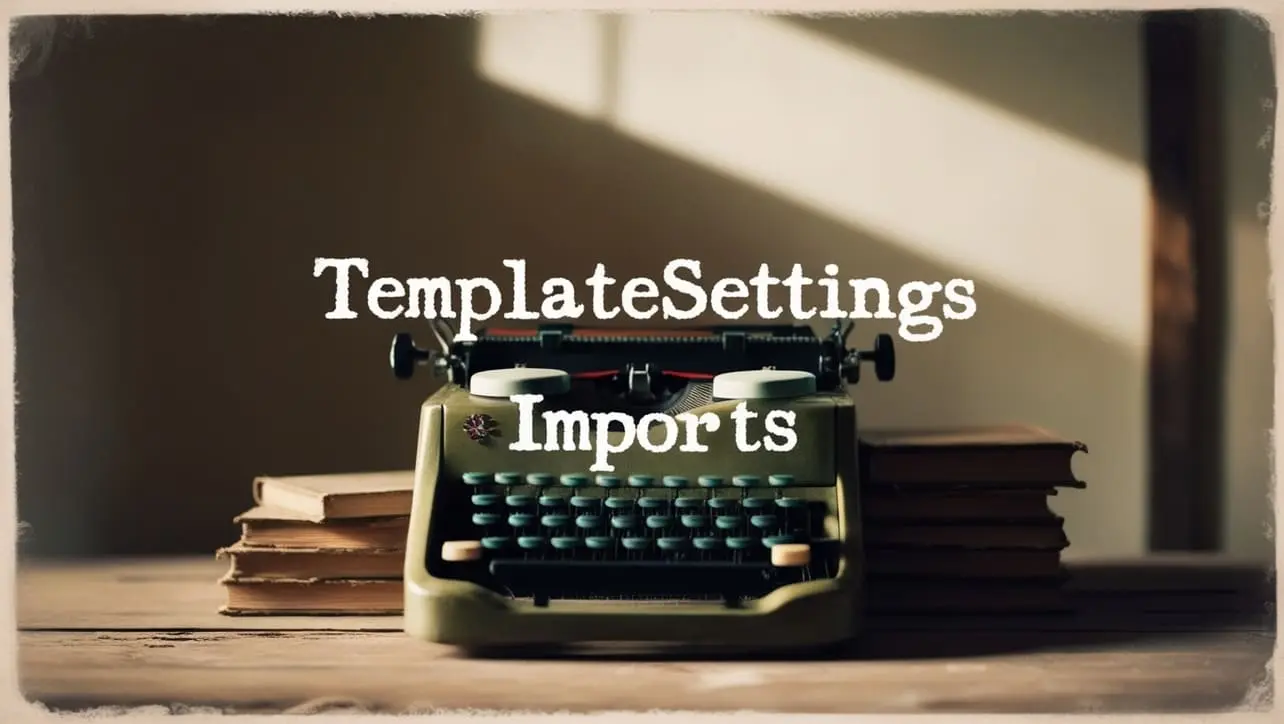
Lodash _.templateSettings.imports Property
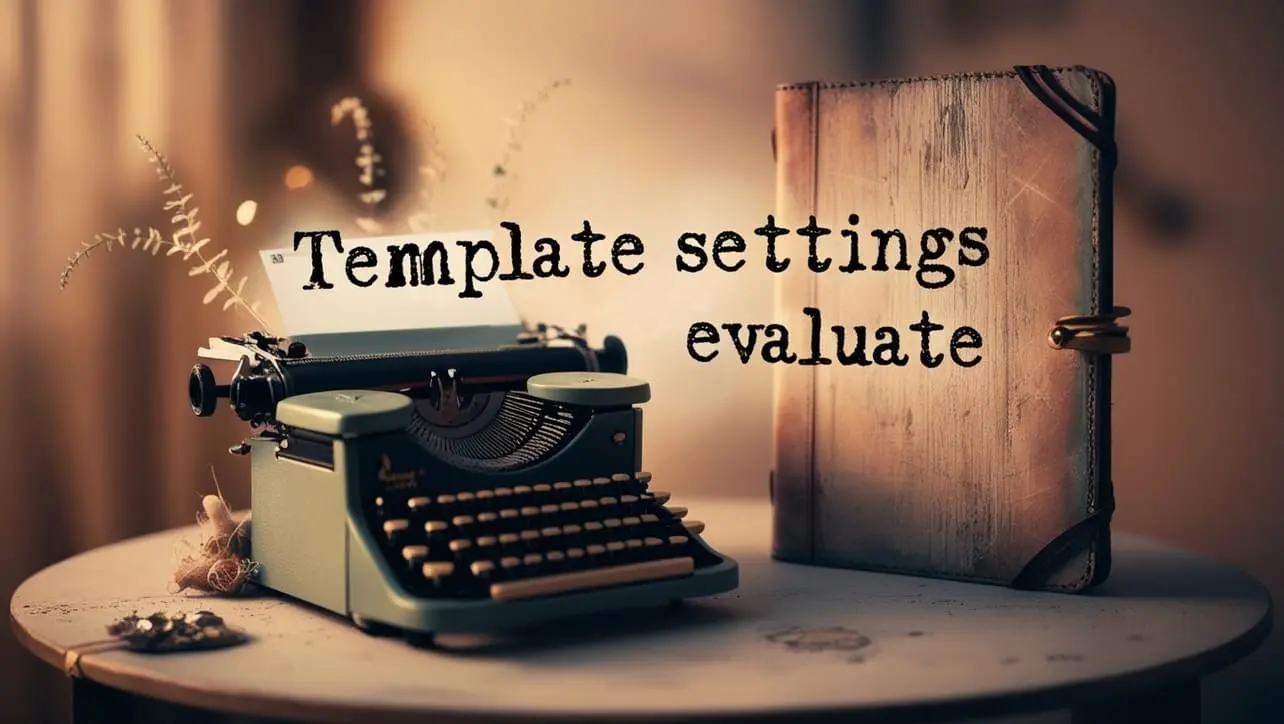
Lodash _.templateSettings.evaluate Property
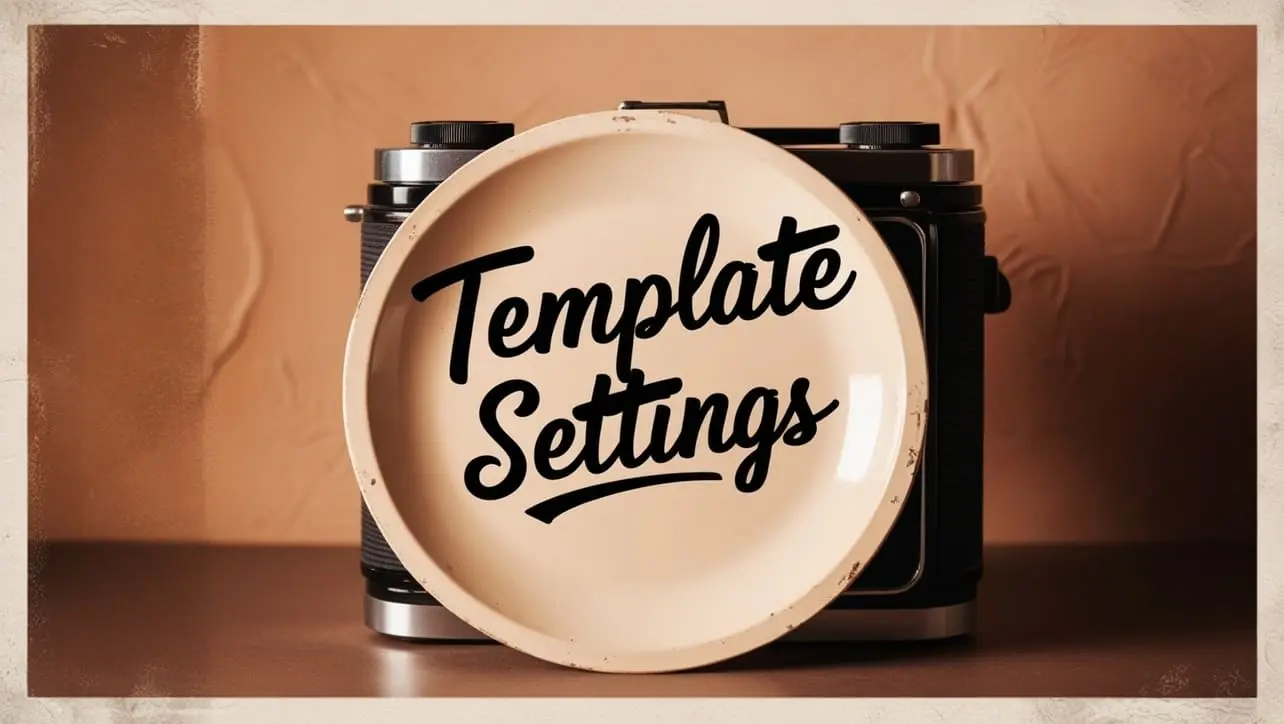
Lodash _.templateSettings Property
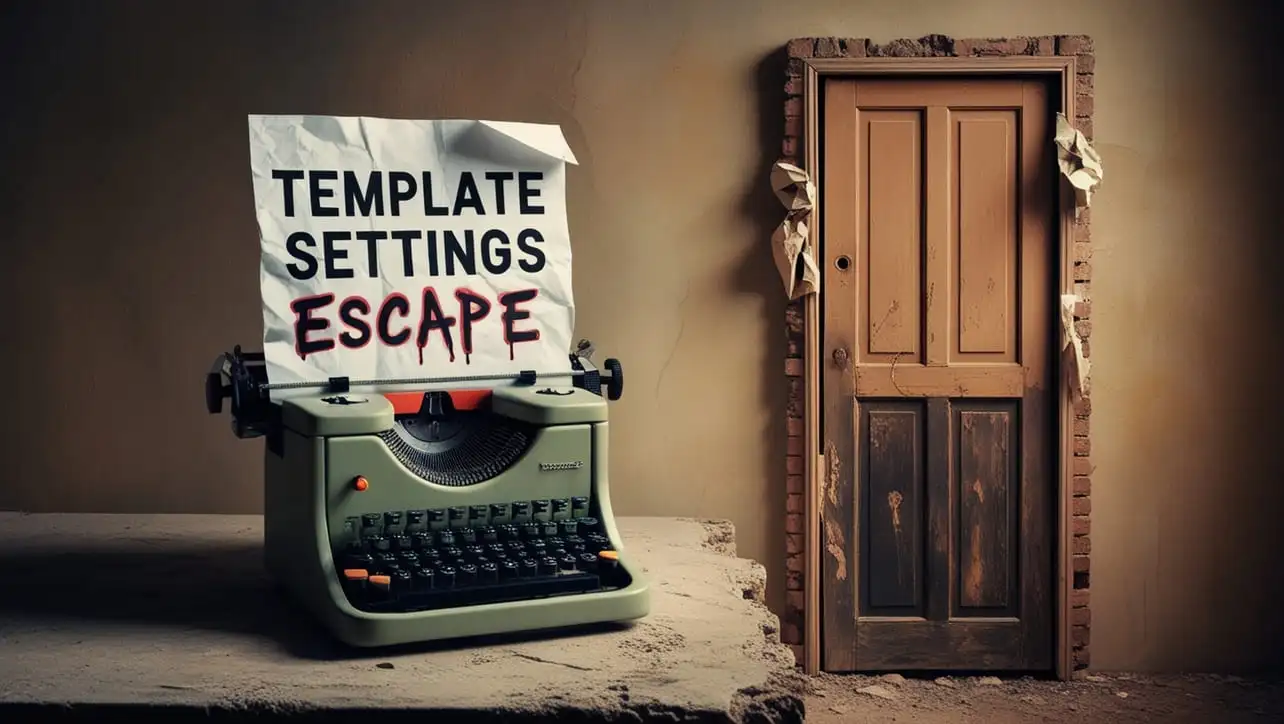
Lodash _.templateSettings.escape Property
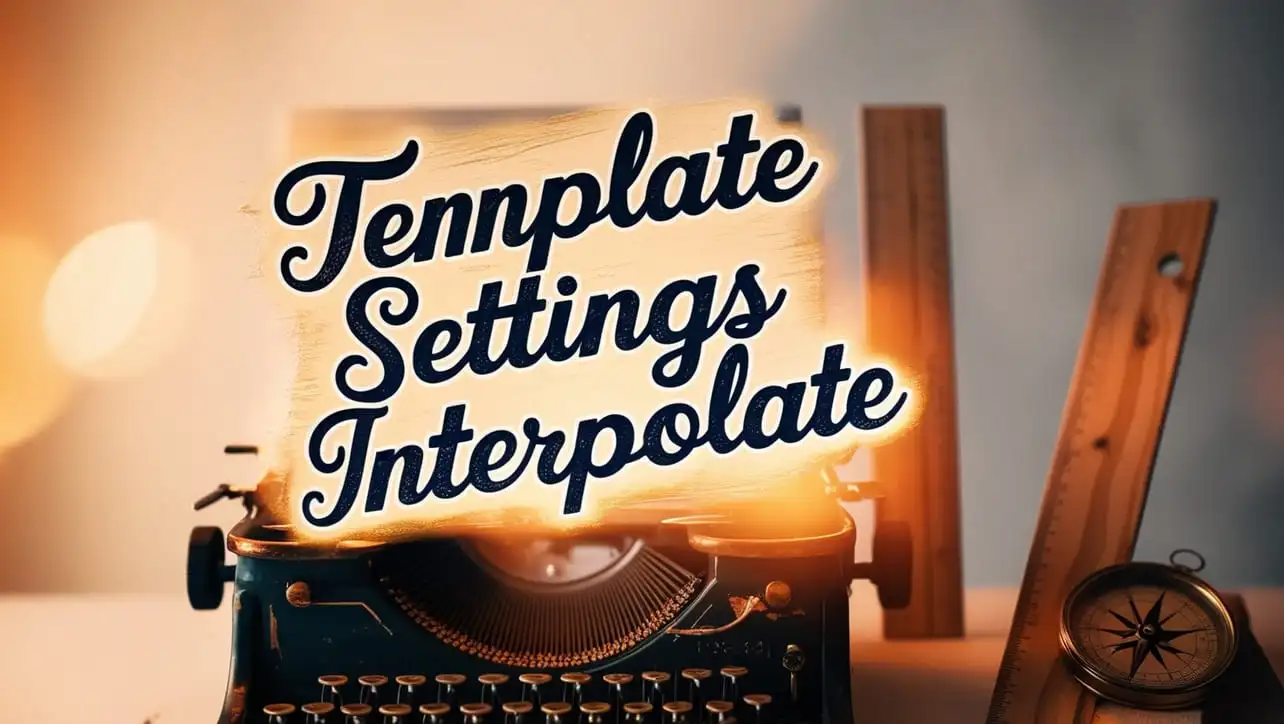
Lodash _.templateSettings.interpolate Property
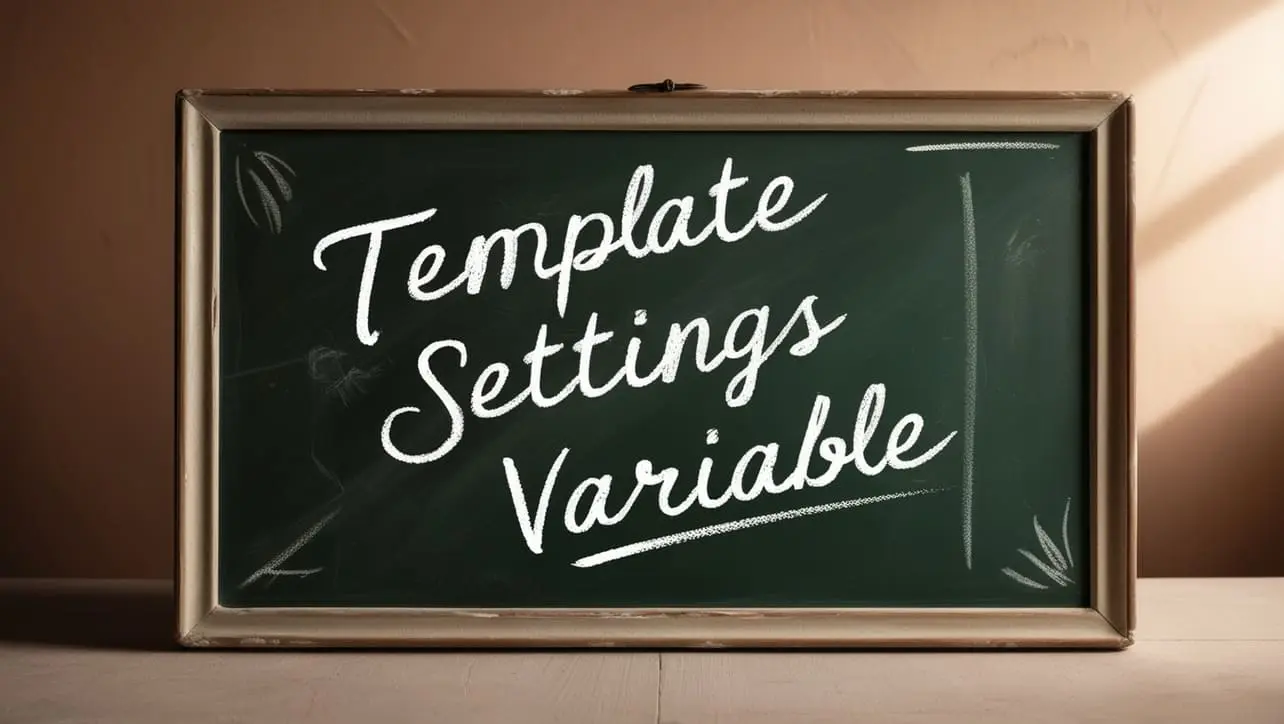
If you have any doubts regarding this article (Lodash _.debounce() Function Method), please comment here. I will help you immediately.