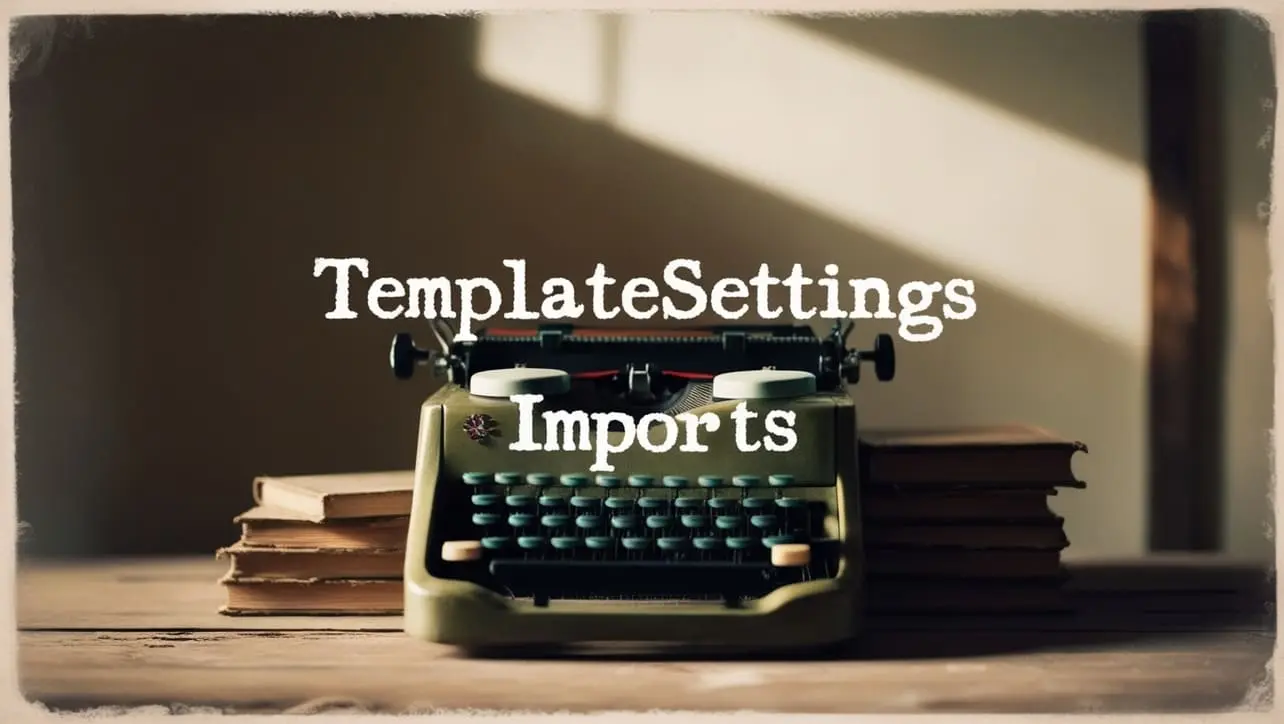
Lodash _.curry() Function Method
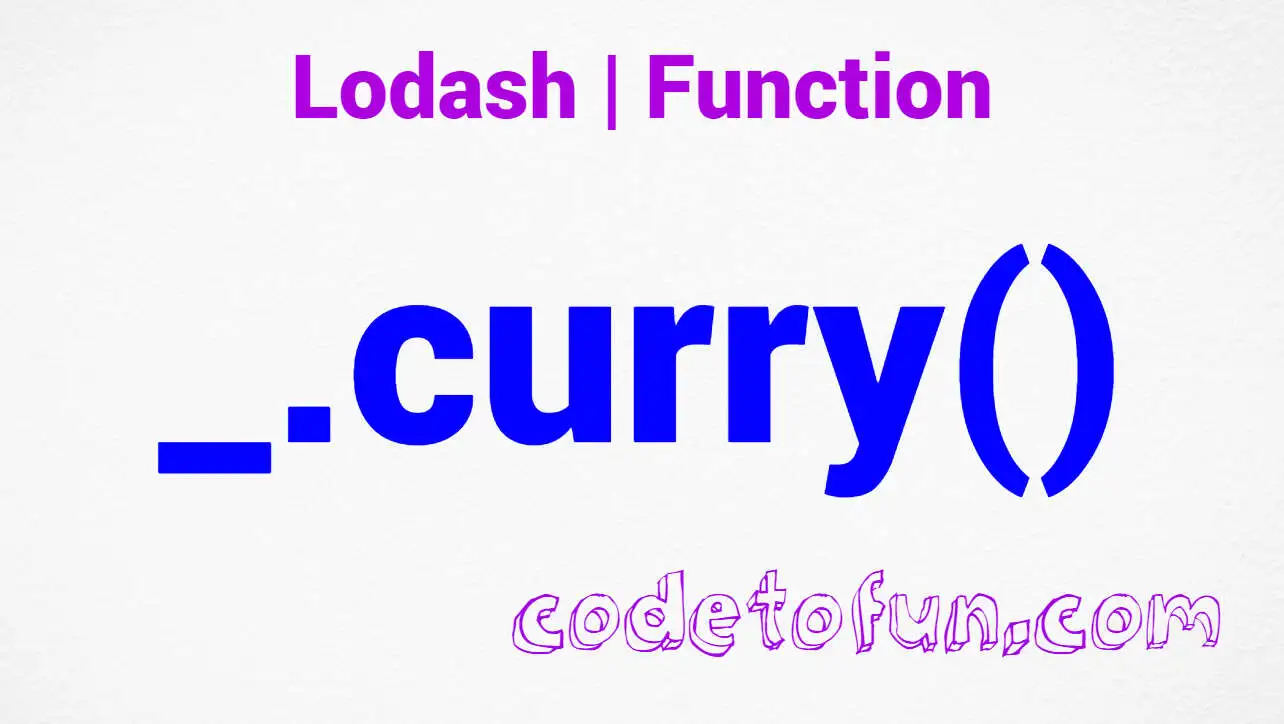
Photo Credit to CodeToFun
🙋 Introduction
Functional programming in JavaScript often involves composing functions and creating more flexible, reusable code. Lodash, a versatile utility library, provides the _.curry()
method, an essential tool for currying functions. Currying allows you to create functions with partial application, enabling better composition and configurability.
In this exploration, we'll delve into the capabilities of _.curry()
and its applications in enhancing functional programming in JavaScript.
🧠 Understanding _.curry() Method
The _.curry()
method in Lodash is designed to curry a given function, transforming it into a sequence of partially applicable functions. This empowers developers to create more versatile and reusable code by partially applying arguments to functions.
💡 Syntax
The syntax for the _.curry()
method is straightforward:
_.curry(func, [arity=func.length])
- func: The function to curry.
- arity (Optional): The number of arguments the curried function should accept.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.curry()
method:
const _ = require('lodash');
// A simple function
const add = (a, b, c) => a + b + c;
// Curry the function
const curriedAdd = _.curry(add);
// Partial application
const addFive = curriedAdd(5);
const result = addFive(3)(2);
console.log(result);
// Output: 10
In this example, the add function is curried using _.curry()
, allowing for partial application and creating a more versatile function.
🏆 Best Practices
When working with the _.curry()
method, consider the following best practices:
Currying Any Function:
Use
_.curry()
to curry any function, making it more flexible and allowing for partial application. This enhances the composability of your code.example.jsCopiedconst multiply = (a, b, c) => a * b * c; const curriedMultiply = _.curry(multiply); const double = curriedMultiply(2); const triple = double(3); console.log(triple(4)); // Output: 24
Specifying Arity:
Consider specifying the arity parameter when currying functions with a specific number of arguments. This allows you to control the behavior of the resulting curried function.
example.jsCopiedconst customCurry = _.curry((a, b, c, d) => a + b + c + d, 3); const partiallyApplied = customCurry(1)(2); console.log(partiallyApplied(3, 4)); // Output: 10
Composing Curried Functions:
Leverage the composability of curried functions by combining them to create more complex functions.
example.jsCopiedconst power = (base, exponent) => Math.pow(base, exponent); const curriedPower = _.curry(power); const square = curriedPower(2); const cube = curriedPower(3); console.log(square(4), cube(3)); // Output: 16 27
📚 Use Cases
Building Configurable Functions:
_.curry()
is particularly useful when building configurable functions. By partially applying arguments, you can create functions with preset configurations.example.jsCopiedconst configLogger = _.curry((prefix, message) => console.log(`${prefix}: ${message}`)); const logInfo = configLogger('INFO'); const logError = configLogger('ERROR'); logInfo('Application started'); logError('Critical error occurred');
Event Handling:
In scenarios where event handlers require additional contextual information, currying can be employed to create specialized event handlers with predefined parameters.
example.jsCopiedconst handleEvent = _.curry((eventType, target, eventData) => { console.log(`${eventType} event on ${target}: ${eventData}`); }); const handleClick = handleEvent('click'); const handleHover = handleEvent('hover'); handleClick('button', 'Button clicked'); handleHover('link', 'Mouse over link');
Functional Composition:
Currying plays a crucial role in functional composition, allowing for the creation of functions that can be easily composed with other functions.
example.jsCopiedconst greet = (greeting, name) => `${greeting}, ${name}!`; const toUpperCase = str => str.toUpperCase(); const greetUpperCase = _.flowRight(toUpperCase, _.curry(greet)('Hello')); console.log(greetUpperCase('John')); // Output: HELLO, JOHN!
🎉 Conclusion
The _.curry()
method in Lodash empowers JavaScript developers with the ability to curry functions, enabling partial application and enhancing code flexibility. Whether you're building configurable functions, handling events, or engaging in functional composition, _.curry()
is a valuable tool in your functional programming toolkit.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.curry()
method in your Lodash projects.
👨💻 Join our Community:
Author
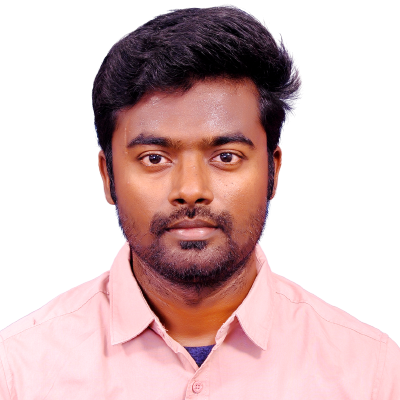
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
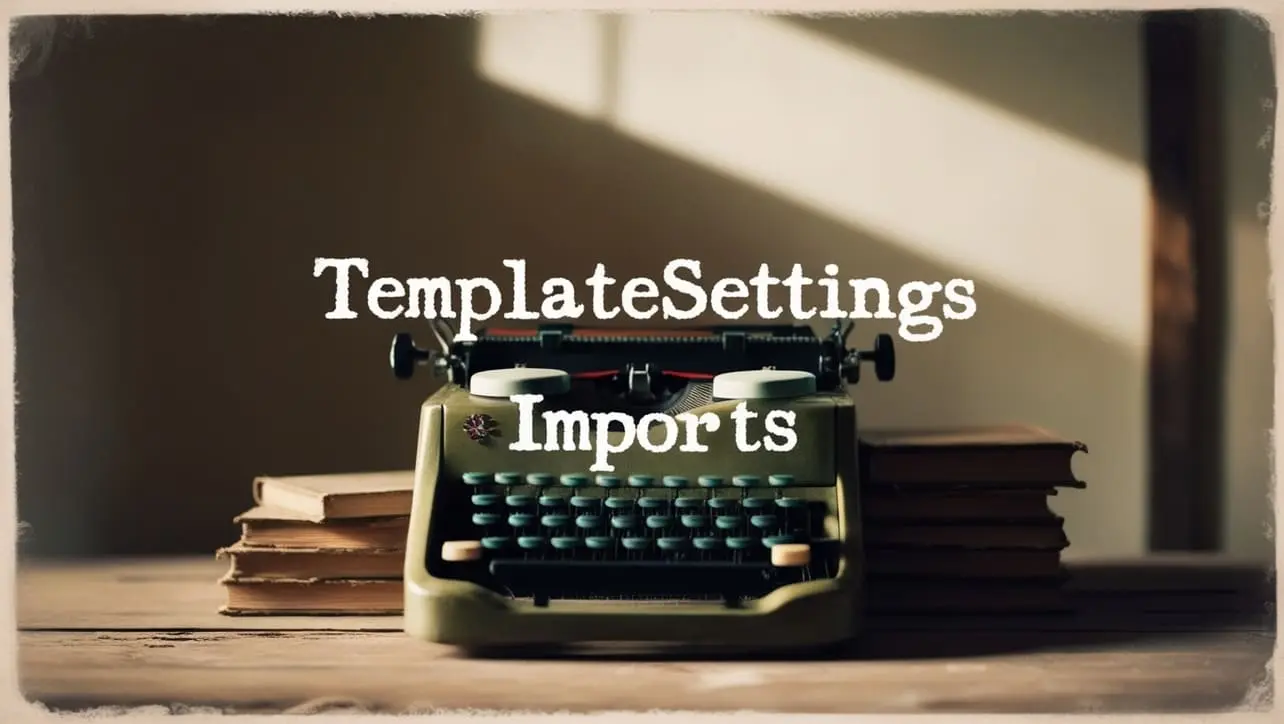
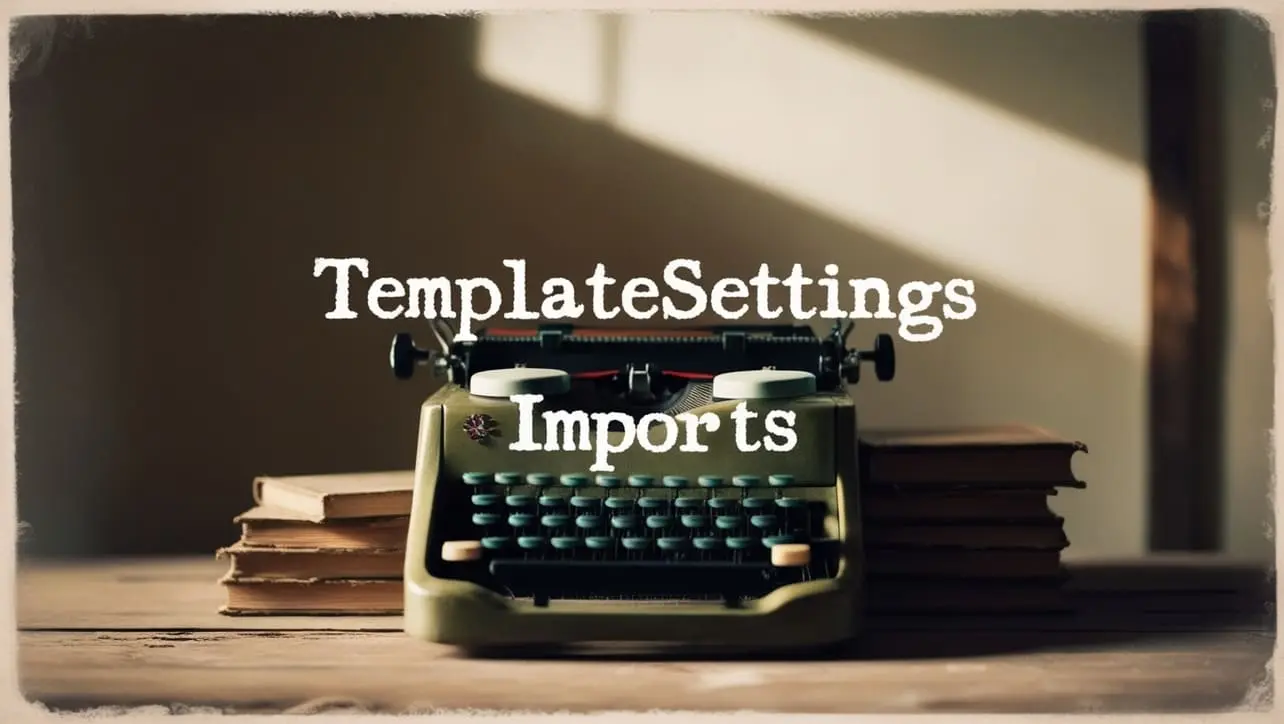
Lodash _.templateSettings.imports Property
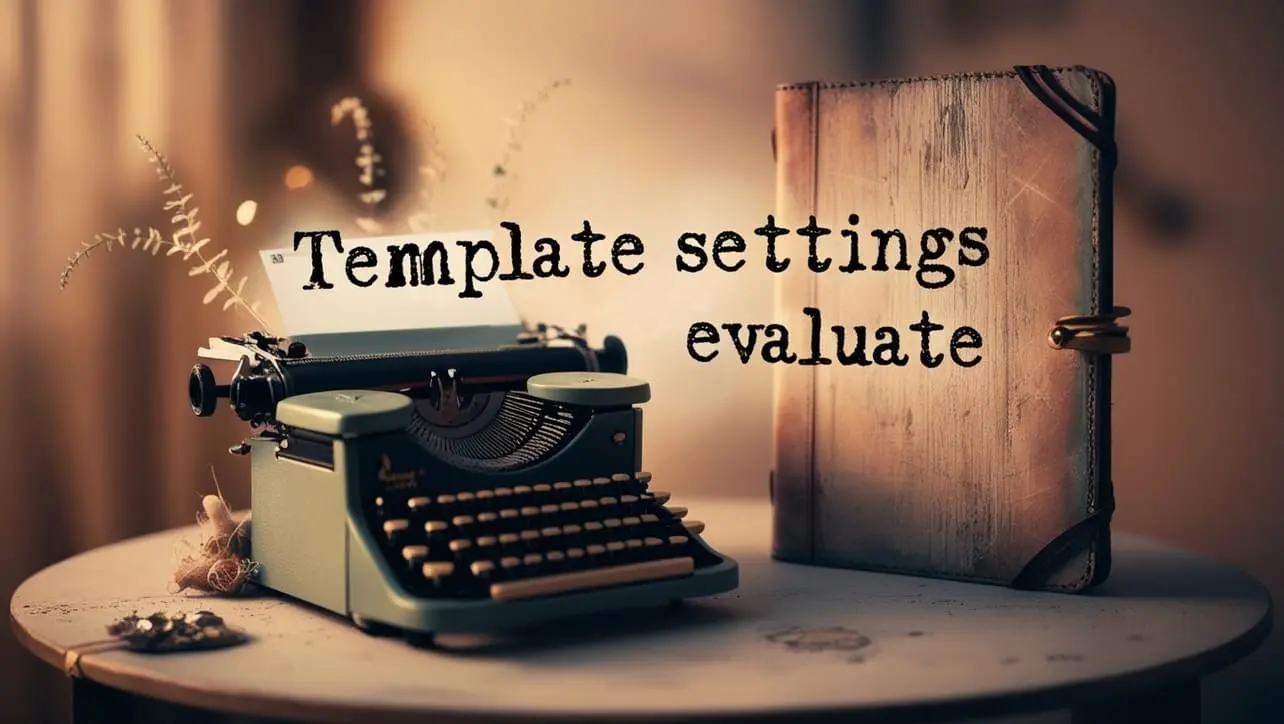
Lodash _.templateSettings.evaluate Property
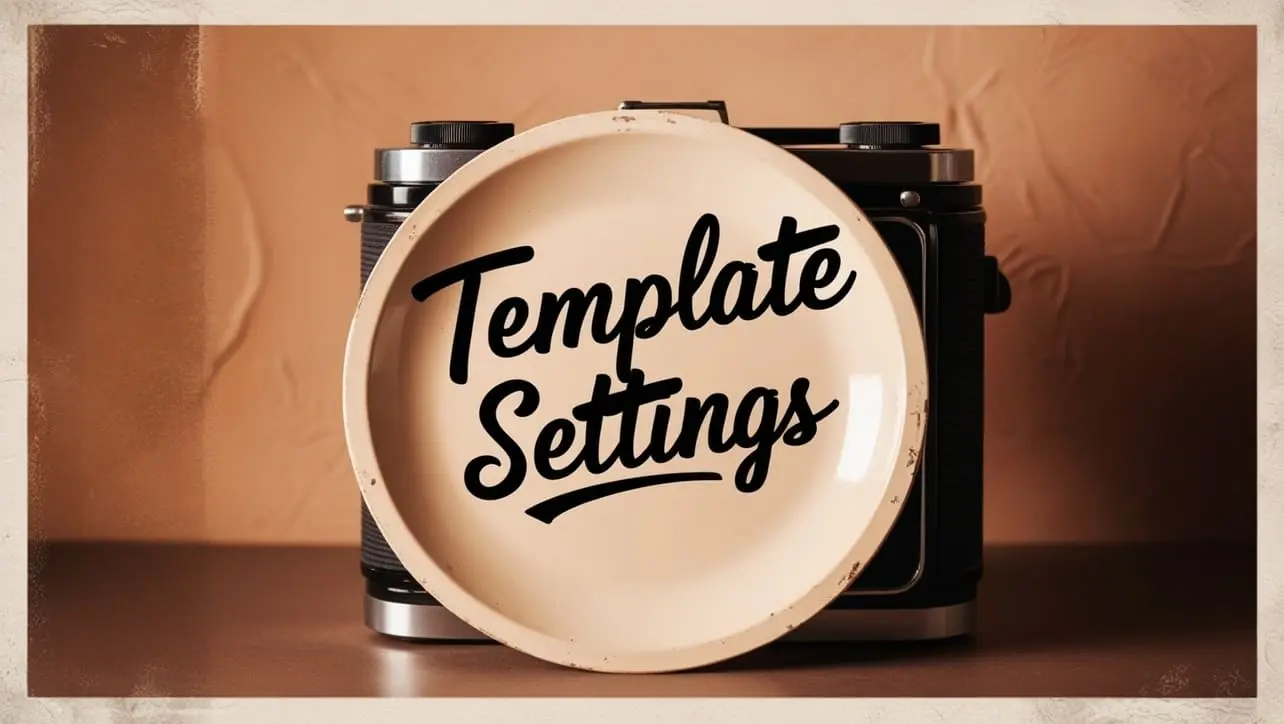
Lodash _.templateSettings Property
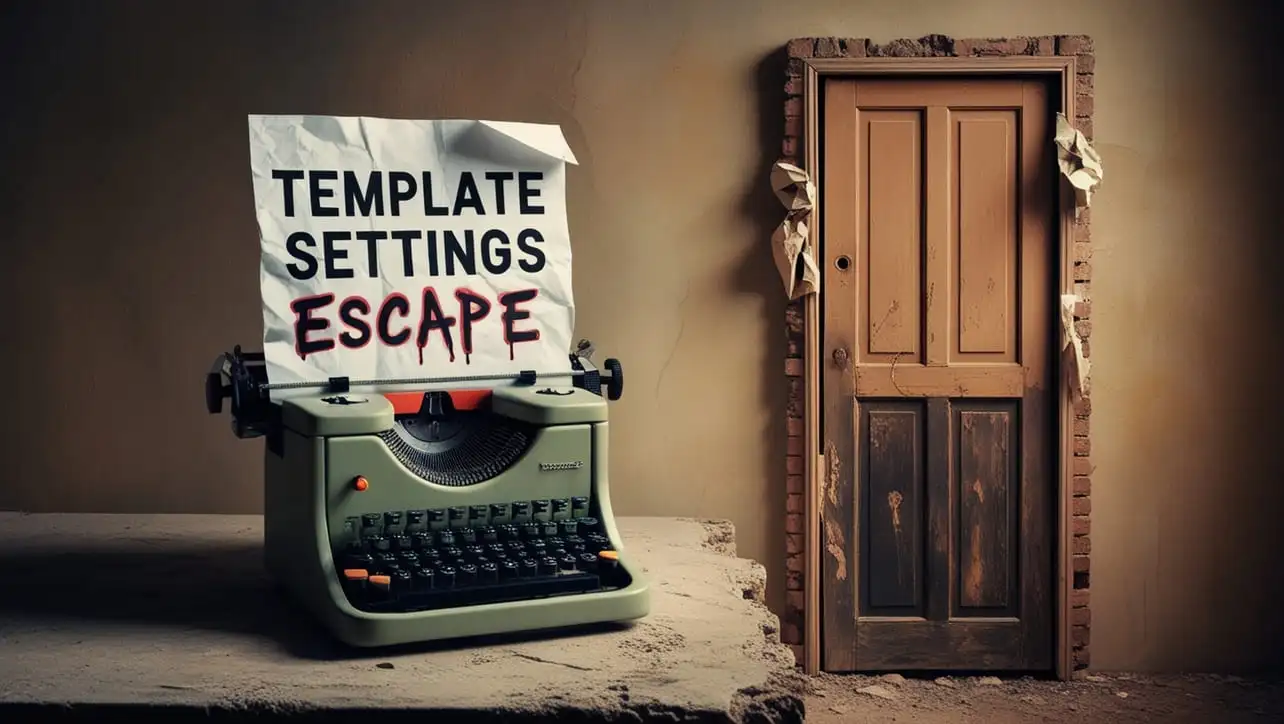
Lodash _.templateSettings.escape Property
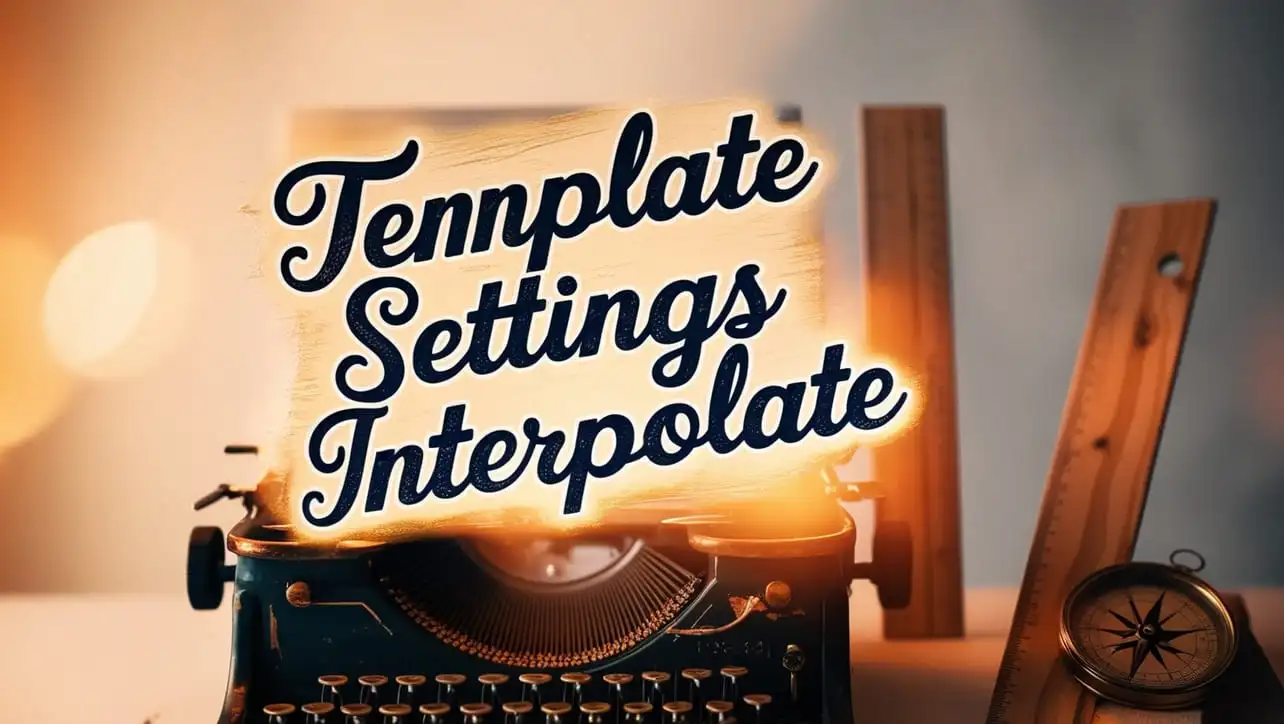
Lodash _.templateSettings.interpolate Property
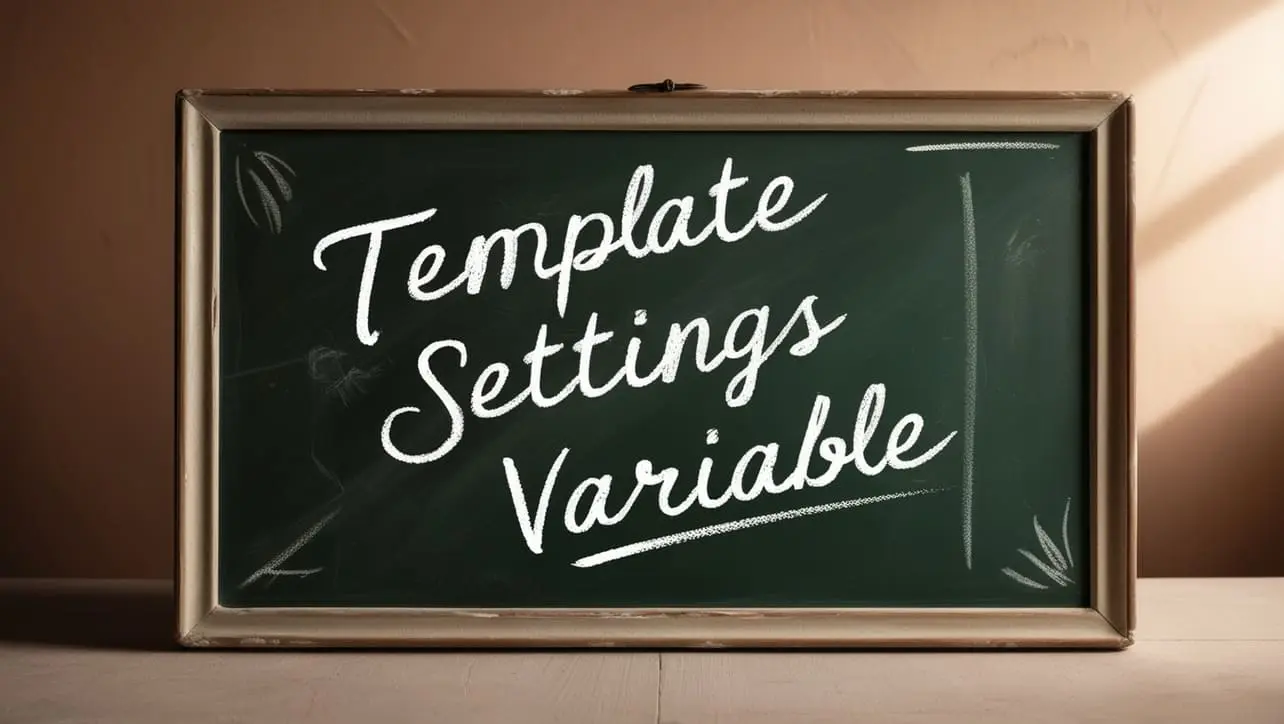
If you have any doubts regarding this article (Lodash _.curry() Function Method), please comment here. I will help you immediately.