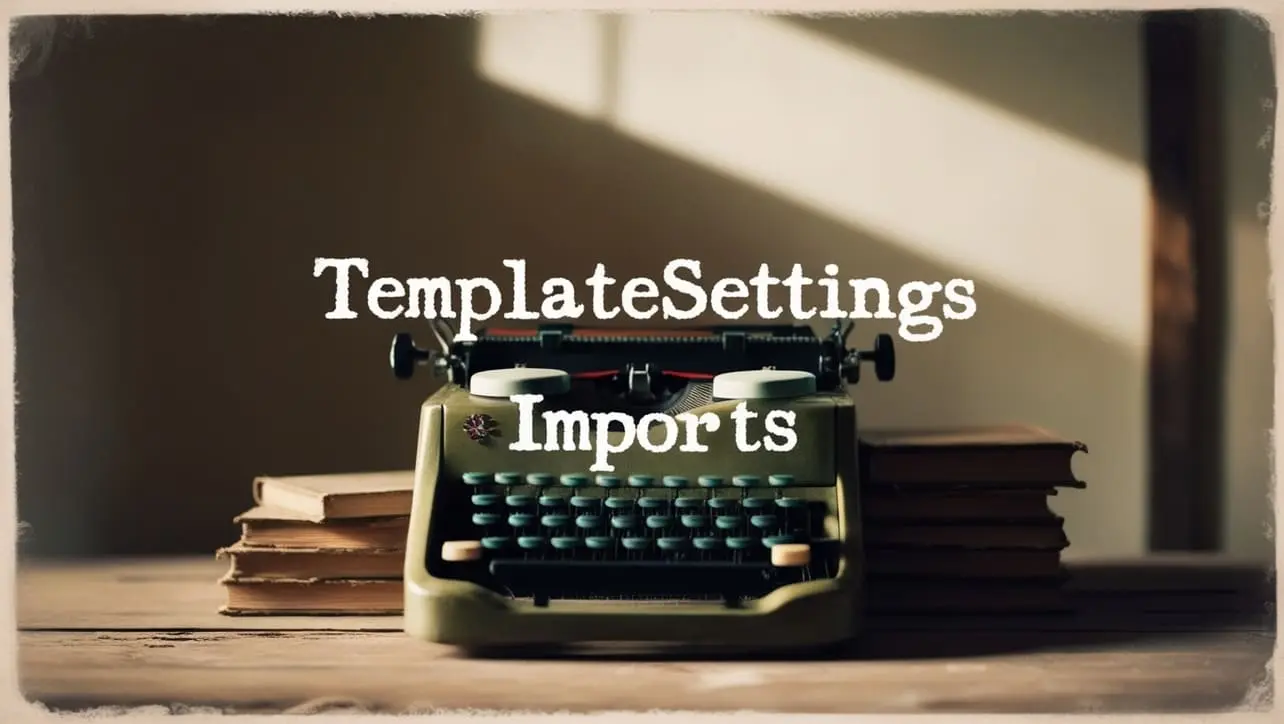
Lodash _.bind() Function Method
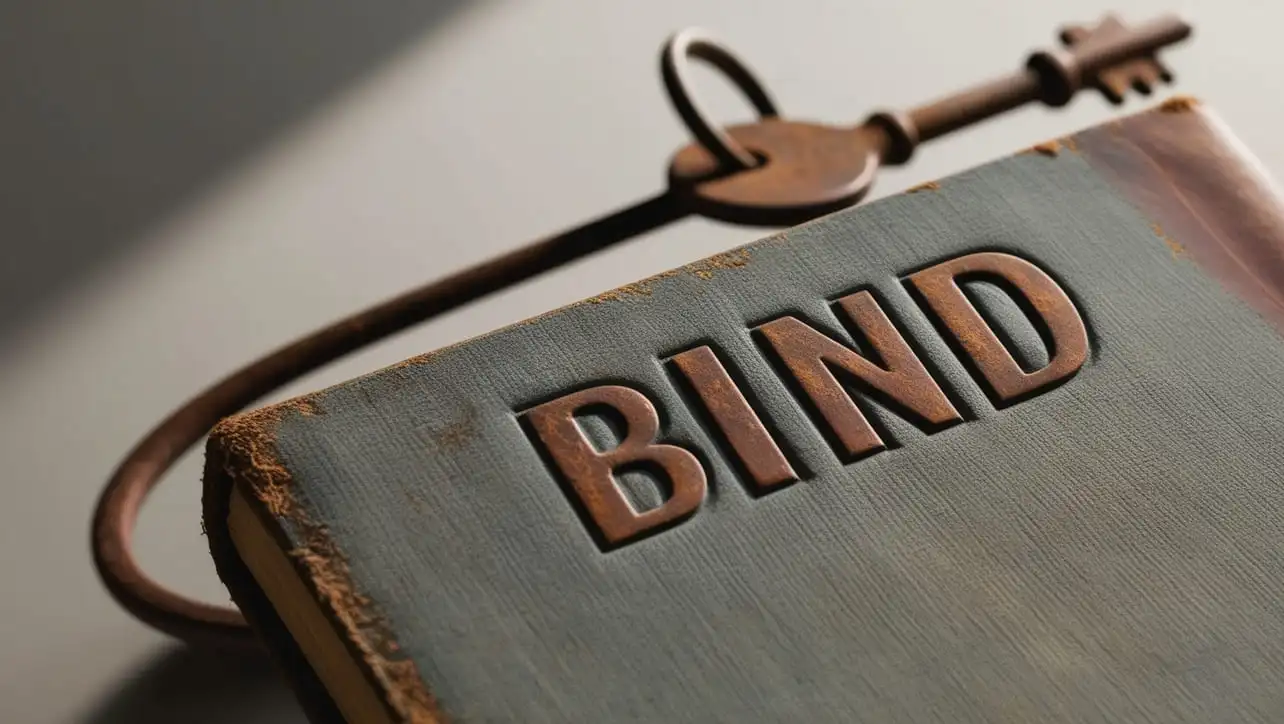
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript, functions play a pivotal role in crafting efficient and reusable code. Lodash, a comprehensive utility library, provides a host of functions to augment JavaScript's capabilities.
Among these functions is _.bind()
, a method that allows developers to create partially applied functions, enabling greater control over the execution context and the ability to preset function arguments.
🧠 Understanding _.bind() Method
The _.bind()
method in Lodash is designed to create a partially applied function by specifying a predefined execution context (this value) and optionally providing one or more arguments. This empowers developers to tailor functions to their specific needs, enhancing code flexibility and maintainability.
💡 Syntax
The syntax for the _.bind()
method is straightforward:
_.bind(func, thisArg, [partials])
- func: The target function to bind.
- thisArg: The context to which the this value is set.
- partials (Optional): Arguments that are partially applied to the function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.bind()
method:
const _ = require('lodash');
function greet(greeting, punctuation) {
return `${greeting}, ${this.name}${punctuation}`;
}
const boundGreet = _.bind(greet, { name: 'John' }, 'Hello');
console.log(boundGreet('!'));
// Output: Hello, John!
In this example, the greet function is bound to the context { name: 'John' } with the partial argument Hello. The resulting boundGreet function can now be invoked with the remaining argument '!' to produce the desired output.
🏆 Best Practices
When working with the _.bind()
method, consider the following best practices:
Understand Execution Context:
Ensure a clear understanding of the execution context when using
_.bind()
. The context (this value) is a crucial aspect that determines how the function behaves.example.jsCopiedconst obj = { value: 42, getValue() { return this.value; }, }; const boundGetValue = _.bind(obj.getValue, { value: 99 }); console.log(boundGetValue()); // Output: 99
Utilize Partial Application:
Take advantage of partial application by supplying arguments to
_.bind()
. This allows you to create functions with predefined values, promoting code reusability.example.jsCopiedfunction multiply(a, b) { return a * b; } const multiplyByTwo = _.bind(multiply, null, 2); console.log(multiplyByTwo(5)); // Output: 10
Consider Use Cases for Partial Application:
Evaluate use cases where partial application with
_.bind()
can simplify code. It's particularly useful in scenarios where certain arguments remain constant across multiple function calls.example.jsCopiedconst logMessage = _.bind(console.log, console, 'Message from _.bind:'); logMessage('Hello, world!'); // Output: 'Message from _.bind: Hello, world!'
📚 Use Cases
Controlling Execution Context:
_.bind()
is instrumental in controlling the execution context of a function. This is particularly useful when dealing with methods that rely on the value of this.example.jsCopiedconst user = { name: 'Alice', greet: function () { console.log(`Hello, ${this.name}!`); }, }; const boundGreet = _.bind(user.greet, user); boundGreet(); // Output: Hello, Alice!
Creating Function Variants:
Use
_.bind()
to create variants of functions with fixed values for some parameters. This promotes code modularity and reusability.example.jsCopiedfunction power(base, exponent) { return Math.pow(base, exponent); } const square = _.bind(power, null, _, 2); const cube = _.bind(power, null, _, 3); console.log(square(4)); // Output: 16 console.log(cube(3)); // Output: 27
Event Handling:
In scenarios involving event handling,
_.bind()
can be employed to ensure the correct context and predefined arguments, facilitating cleaner and more maintainable event-driven code.example.jsCopiedconst button = document.getElementById('myButton'); function handleClick(event, message) { console.log(`${message}: Button clicked!`); } button.addEventListener('click', _.bind(handleClick, null, _, 'Info'));
🎉 Conclusion
The _.bind()
method in Lodash empowers JavaScript developers with the ability to control function execution context and create partially applied functions. Whether you're shaping the behavior of methods or crafting reusable variants of functions, _.bind()
is a versatile tool that enhances code flexibility and maintainability.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.bind()
method in your Lodash projects.
👨💻 Join our Community:
Author
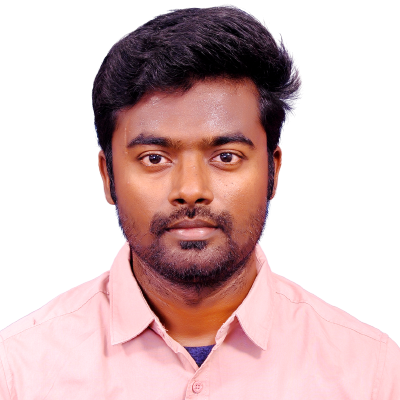
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
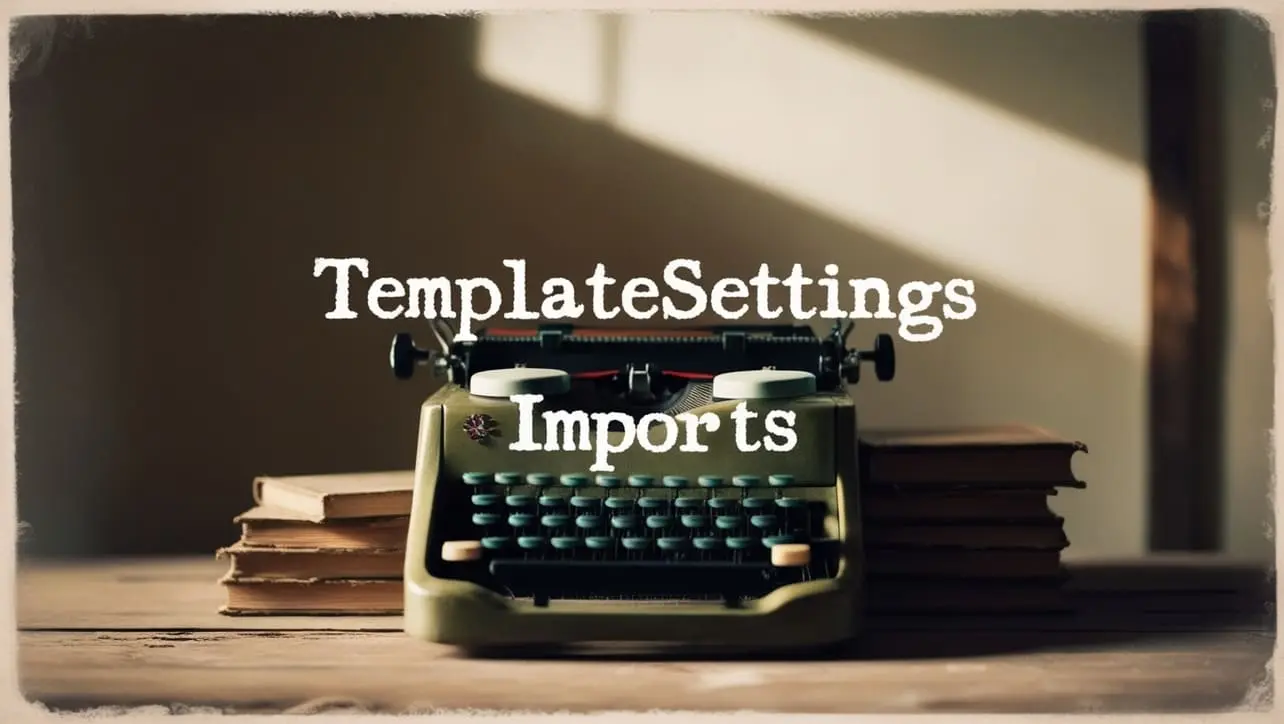
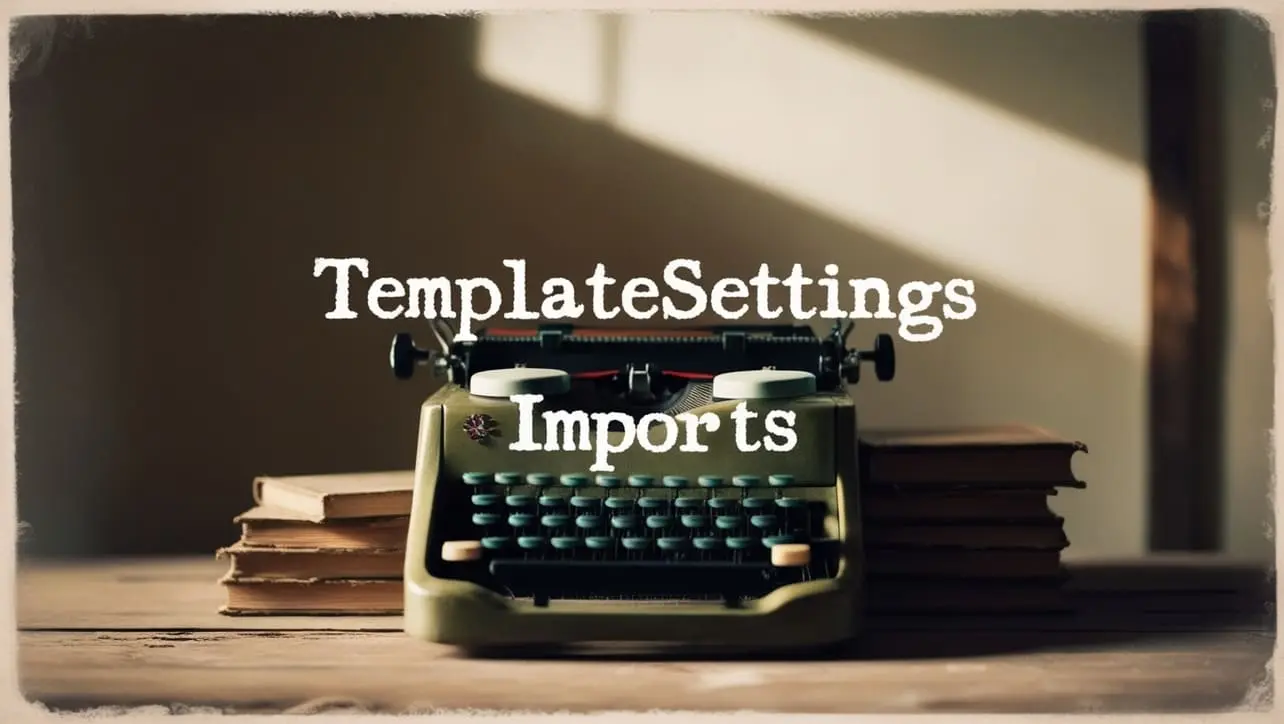
Lodash _.templateSettings.imports Property
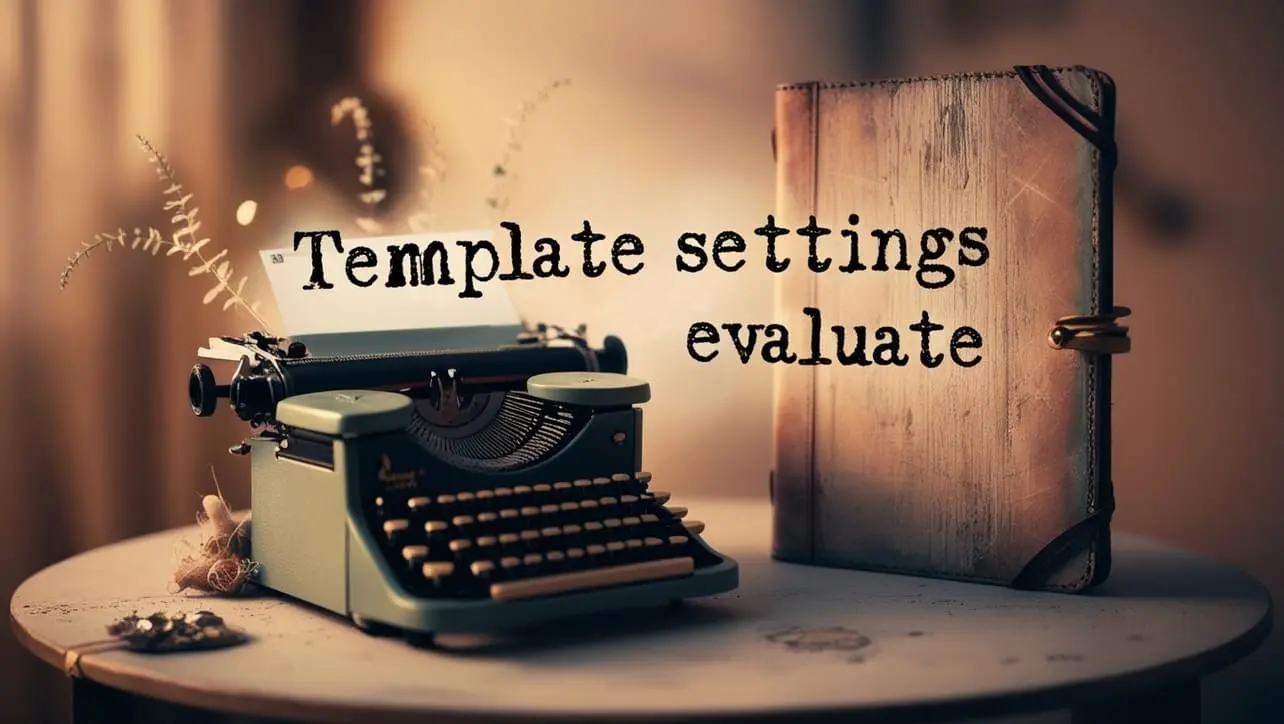
Lodash _.templateSettings.evaluate Property
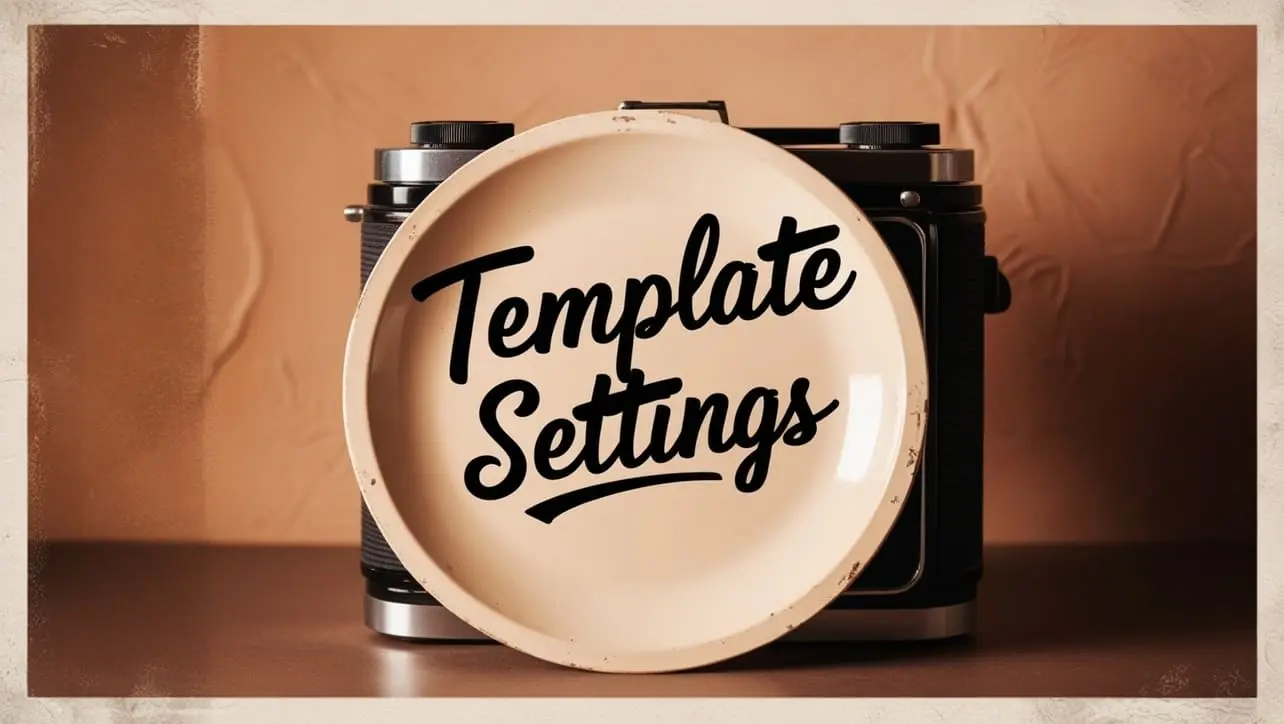
Lodash _.templateSettings Property
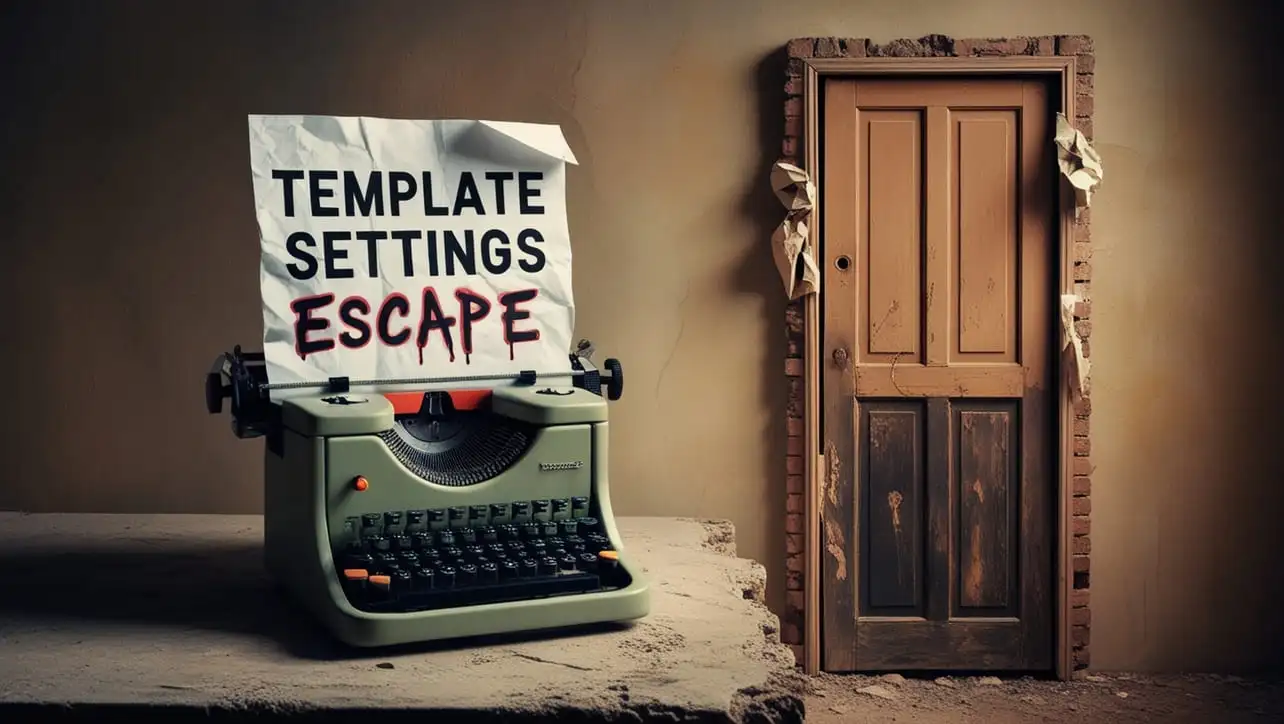
Lodash _.templateSettings.escape Property
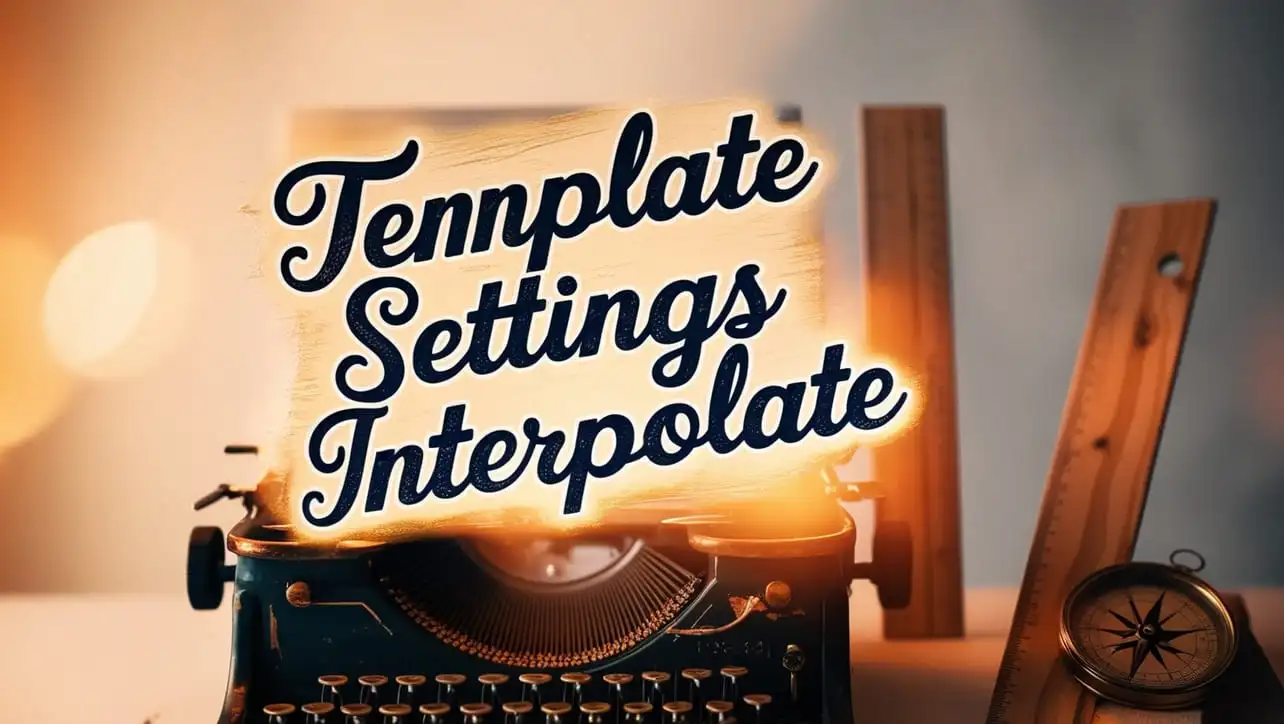
Lodash _.templateSettings.interpolate Property
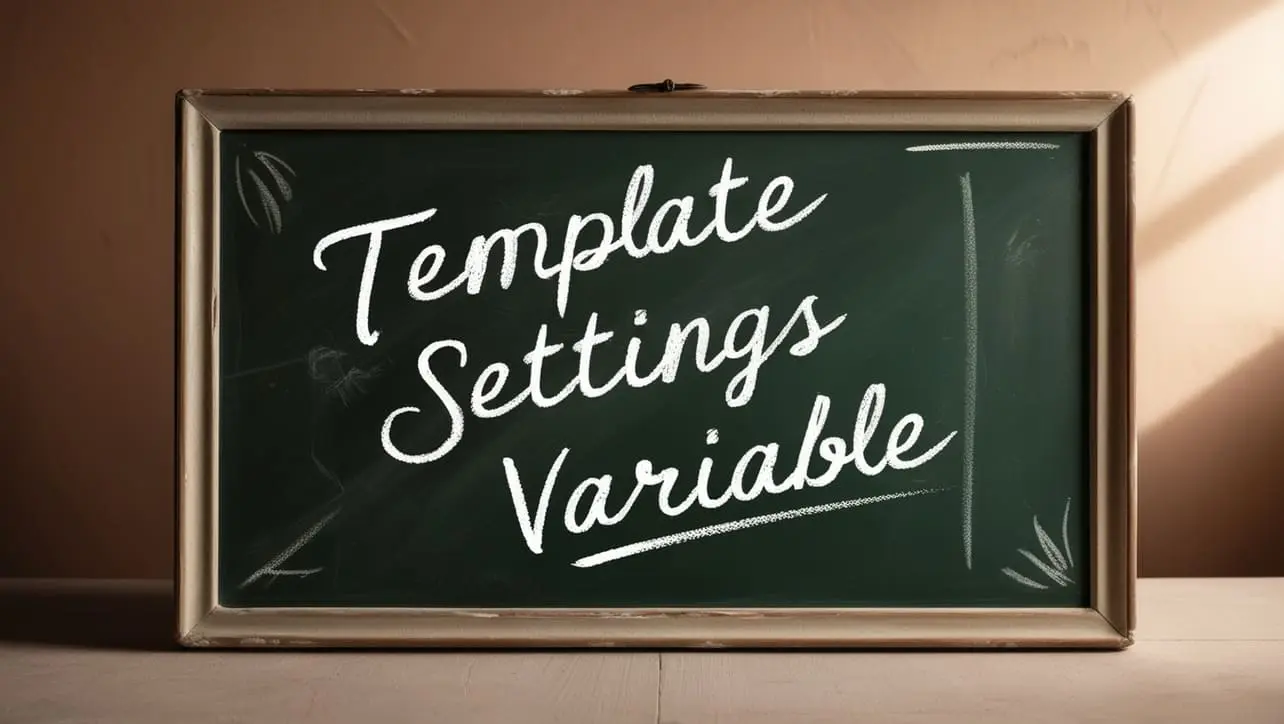
If you have any doubts regarding this article (Lodash _.bind() Function Method), please comment here. I will help you immediately.