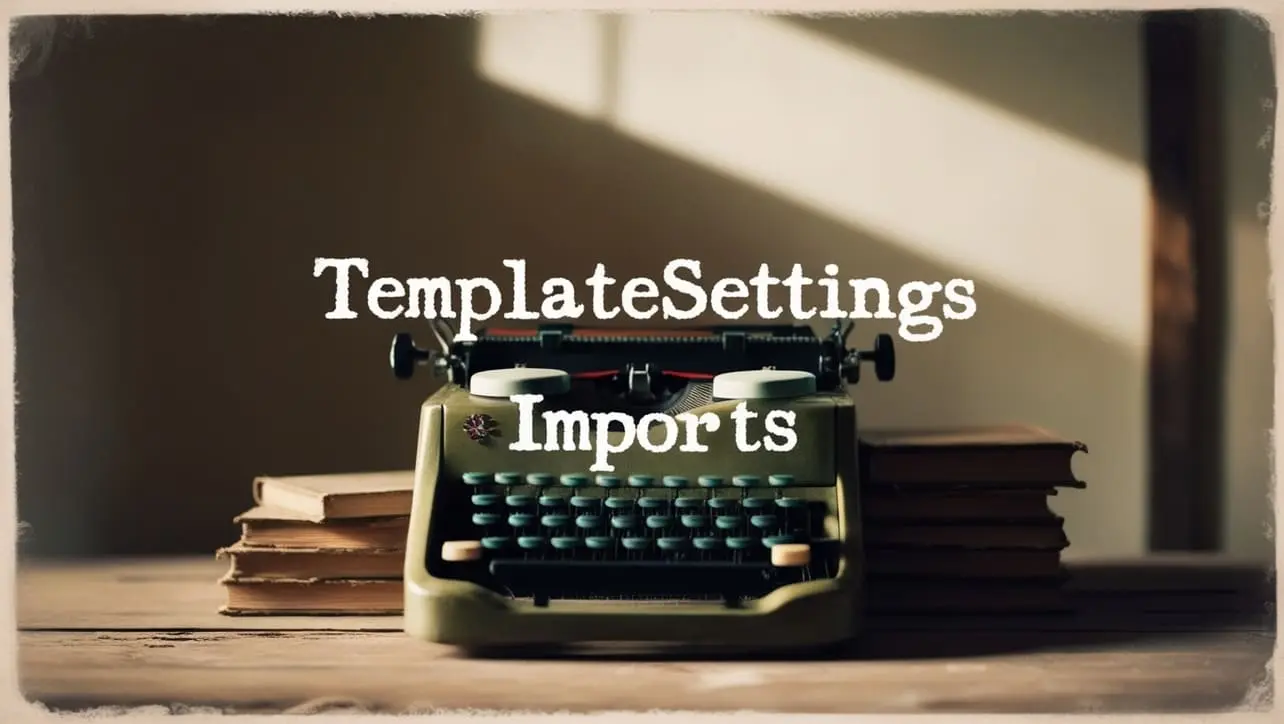
Lodash _.throttle() Function Method
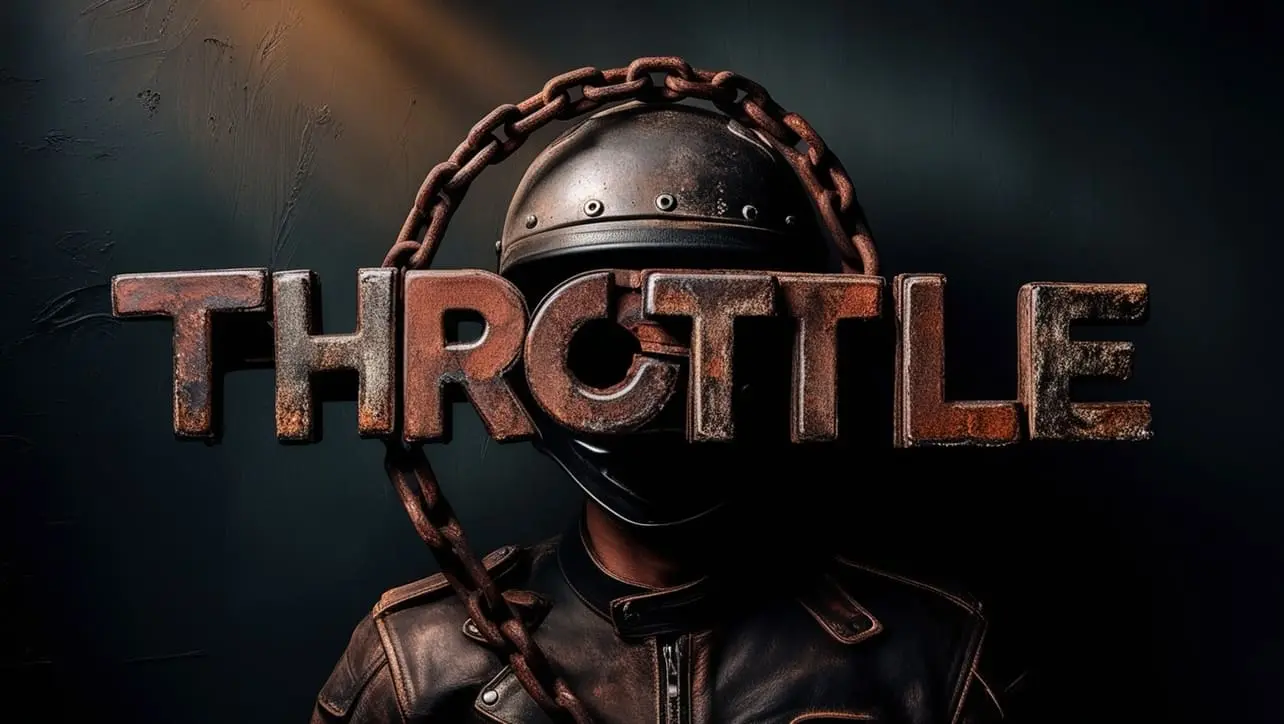
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript development, handling functions and controlling their execution frequency is a common requirement. Enter Lodash, a versatile utility library that provides a myriad of functions to streamline programming tasks. One such function is _.throttle()
, a powerful tool for controlling the rate at which a function is invoked.
This method proves invaluable in scenarios where you need to limit the frequency of function calls, especially in response to rapid events like scrolling or resizing.
🧠 Understanding _.throttle() Method
The _.throttle()
method in Lodash is designed to limit the rate at which a function is executed. This is achieved by ensuring that the function is called at most once in a specified time window, often referred to as the "throttle window" or "throttle interval." This can prevent performance issues and enhance the overall user experience.
💡 Syntax
The syntax for the _.throttle()
method is straightforward:
_.throttle(func, [wait=0], [options={}])
- func: The function to throttle.
- wait: The number of milliseconds to throttle the function (default is 0).
- options: Additional options to customize throttling behavior.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.throttle()
method:
const _ = require('lodash');
// Example function to be throttled
function logMessage(message) {
console.log(message);
}
// Throttle the function to be called at most once every 2000 milliseconds (2 seconds)
const throttledLog = _.throttle(logMessage, 2000);
// Simulate rapid function calls
setInterval(() => throttledLog('Hello, Throttle!'), 500);
// Output: "Hello, Throttle!" every 2000 milliseconds
In this example, the logMessage function is throttled using _.throttle()
to ensure it is called at most once every 2000 milliseconds, even though it's being invoked more frequently.
🏆 Best Practices
When working with the _.throttle()
method, consider the following best practices:
Understanding Throttle Window:
Be mindful of the throttle window (wait parameter) and its impact on function execution. Adjust the throttle window based on the specific use case to strike a balance between responsiveness and performance.
example.jsCopiedconst debouncedSave = _.throttle(saveChanges, 5000); // Adjusting throttle window dynamically based on user interaction someButton.addEventListener('click', () => { debouncedSave(); }); anotherButton.addEventListener('click', () => { // Update the throttle window to a shorter duration debouncedSave.options({ leading: false, trailing: true, wait: 1000 })(); });
Customizing Throttle Options:
Explore and customize the options parameter to tailor the throttling behavior according to your application's requirements.
example.jsCopied// Customizing throttle options const throttledFunction = _.throttle(myFunction, 1000, { leading: false, trailing: true }); // Execute the throttled function throttledFunction();
Use Cases for Throttling:
Identify scenarios where throttling is beneficial, such as handling user input, preventing excessive API requests, or optimizing performance during resource-intensive operations.
example.jsCopied// Throttle user input to improve performance during typing const throttledSearch = _.throttle(searchFunction, 300); searchInput.addEventListener('input', throttledSearch);
📚 Use Cases
Handling User Input:
_.throttle()
is particularly useful when handling user input, such as search queries or interactions that trigger frequent function calls. Throttling ensures that the function is called at a controlled rate, preventing unnecessary computation.example.jsCopiedconst searchInput = document.getElementById('searchInput'); // Throttle the search function to be called at most once every 300 milliseconds const throttledSearch = _.throttle(searchFunction, 300); // Attach the throttled function to the input event searchInput.addEventListener('input', throttledSearch);
Preventing Excessive API Requests:
In scenarios where functions trigger API requests,
_.throttle()
can be employed to prevent excessive requests by limiting the frequency of API calls.example.jsCopied// Throttle the function to fetch data from the API, allowing at most one request every 2000 milliseconds const throttledFetch = _.throttle(fetchDataFromApi, 2000); // Trigger the throttled function based on user interactions userButton.addEventListener('click', throttledFetch);
Optimizing Performance during Scrolling:
During scrolling events, especially in web applications, throttling functions can optimize performance by reducing the frequency of computations.
example.jsCopied// Throttle the function to handle scroll events, ensuring it is called at most once every 500 milliseconds const throttledScrollHandler = _.throttle(handleScroll, 500); // Attach the throttled function to the scroll event window.addEventListener('scroll', throttledScrollHandler);
🎉 Conclusion
The _.throttle()
method in Lodash provides an effective solution for controlling the rate at which functions are executed, offering benefits in scenarios involving user input, API requests, or performance optimization during events like scrolling.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.throttle()
method in your Lodash projects.
👨💻 Join our Community:
Author
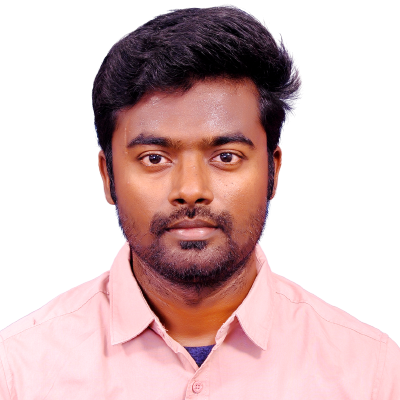
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
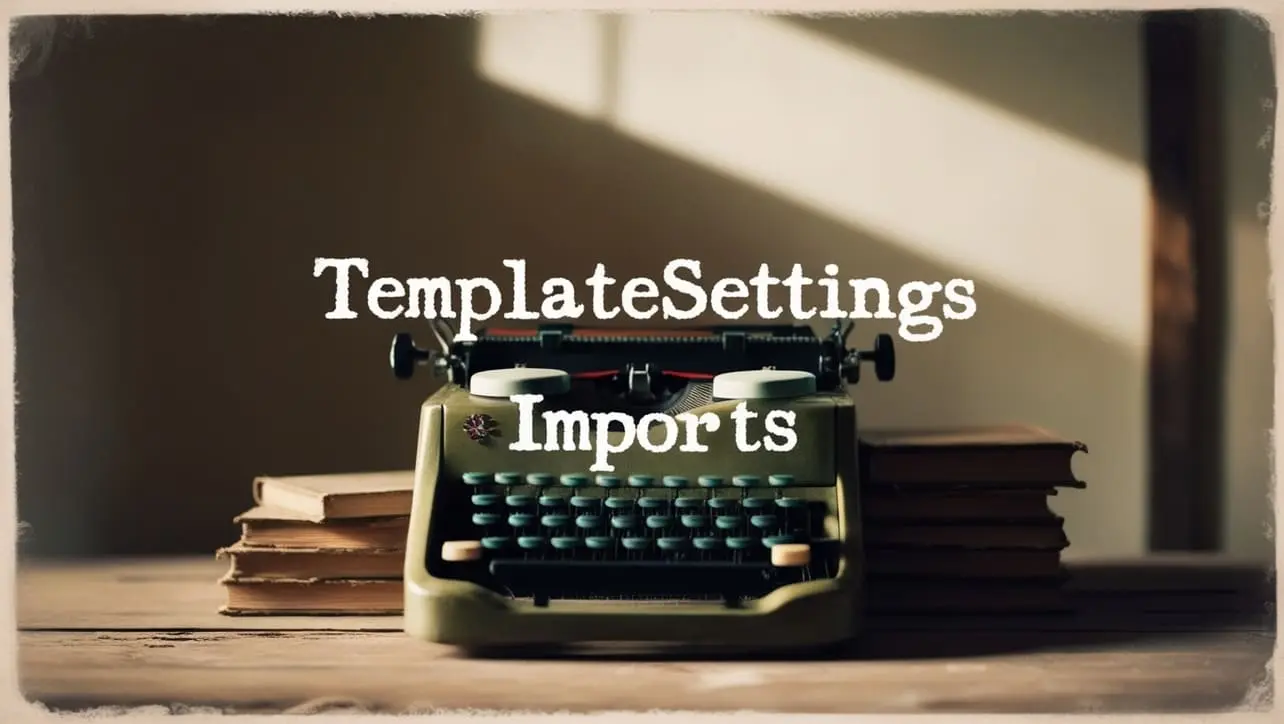
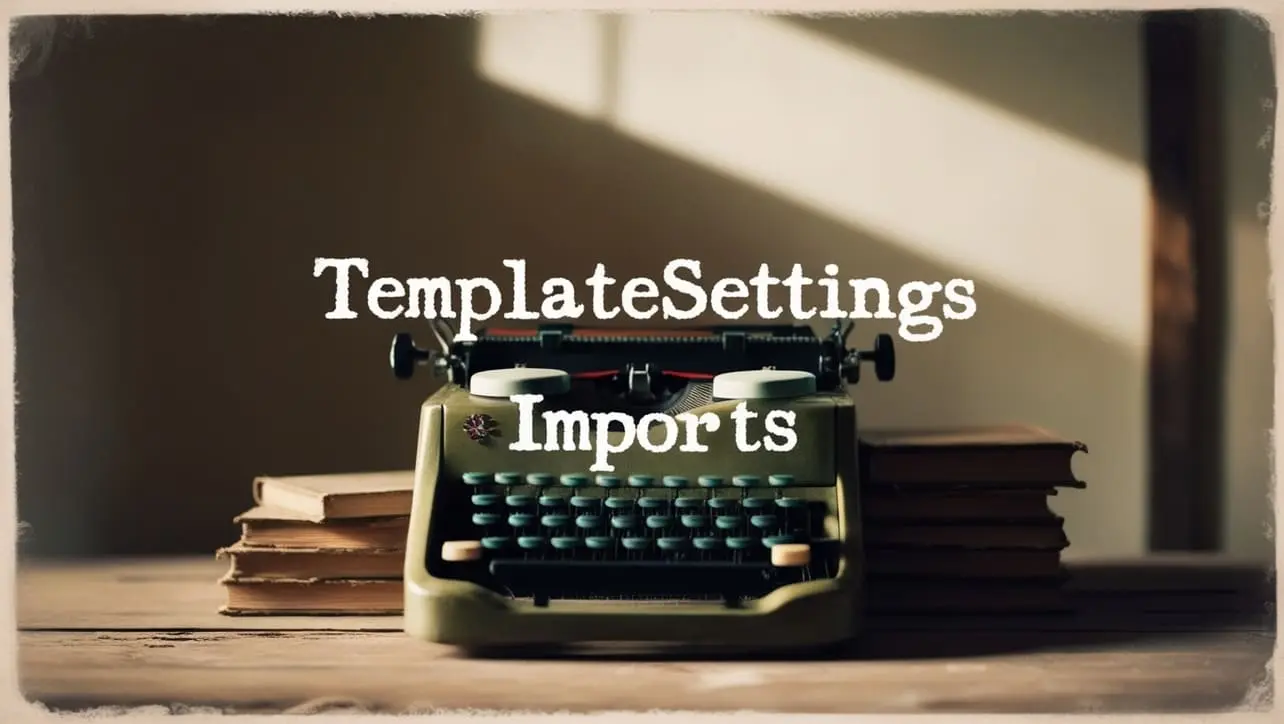
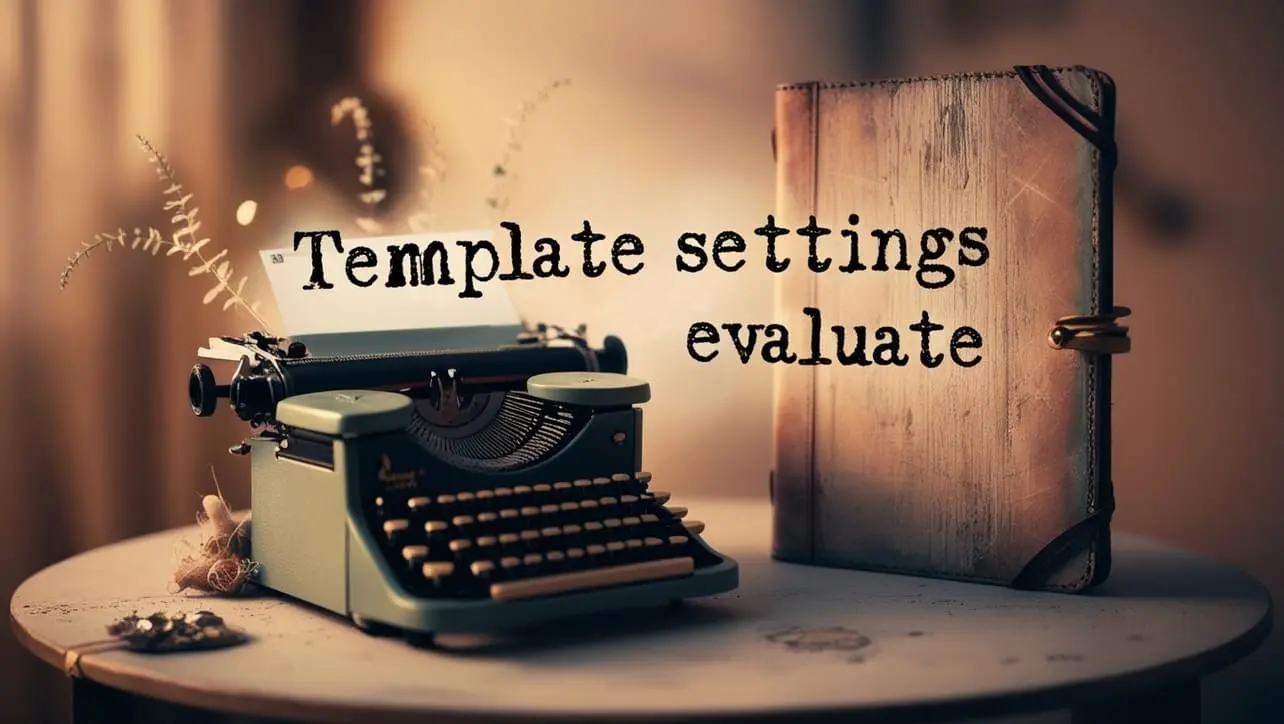
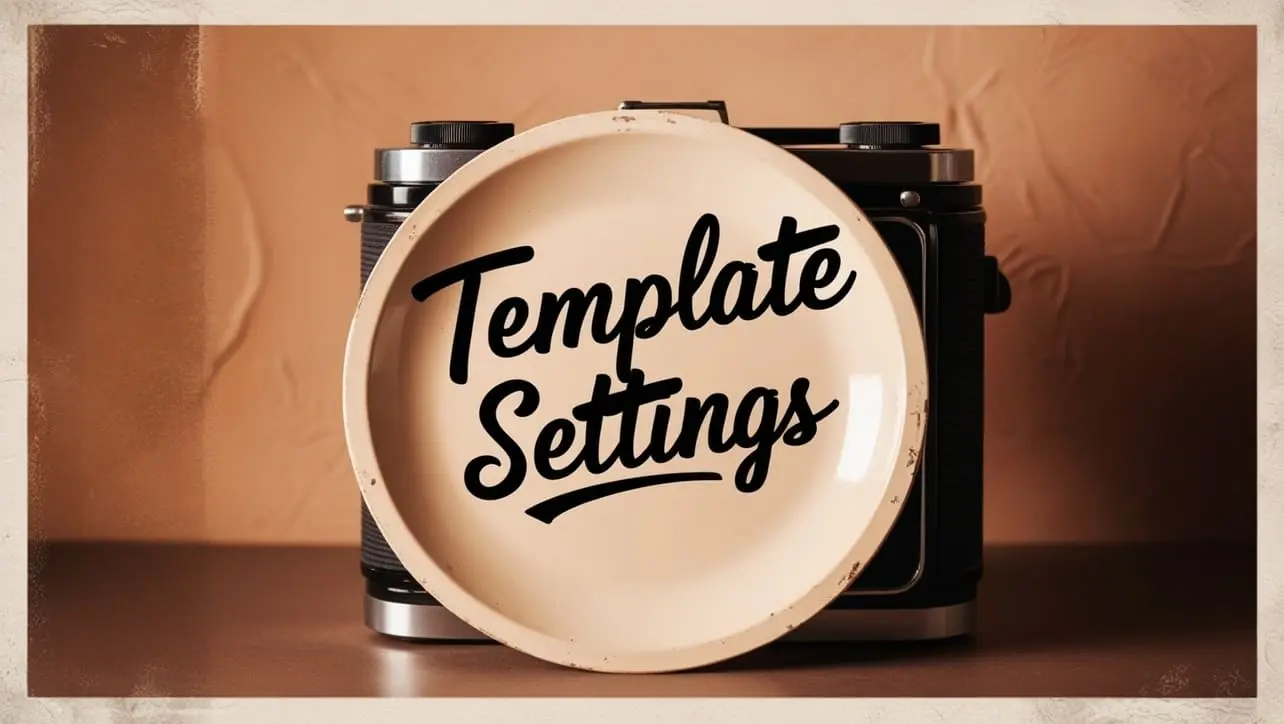
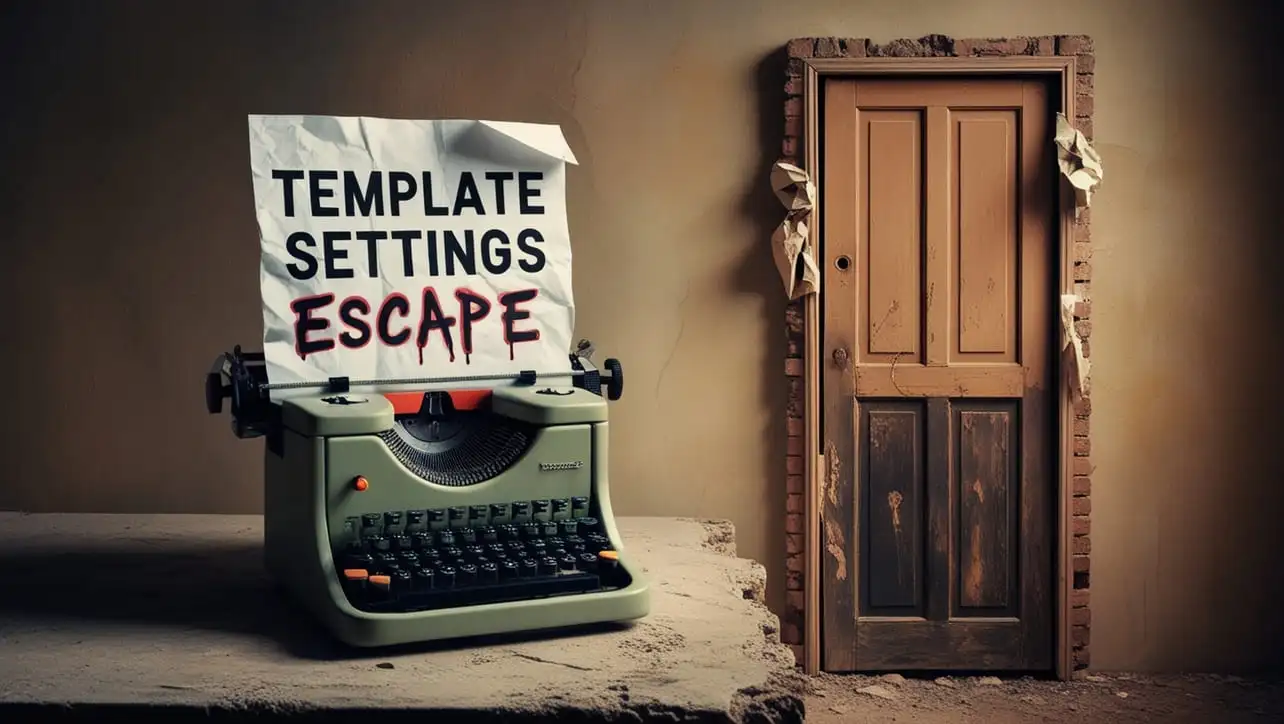
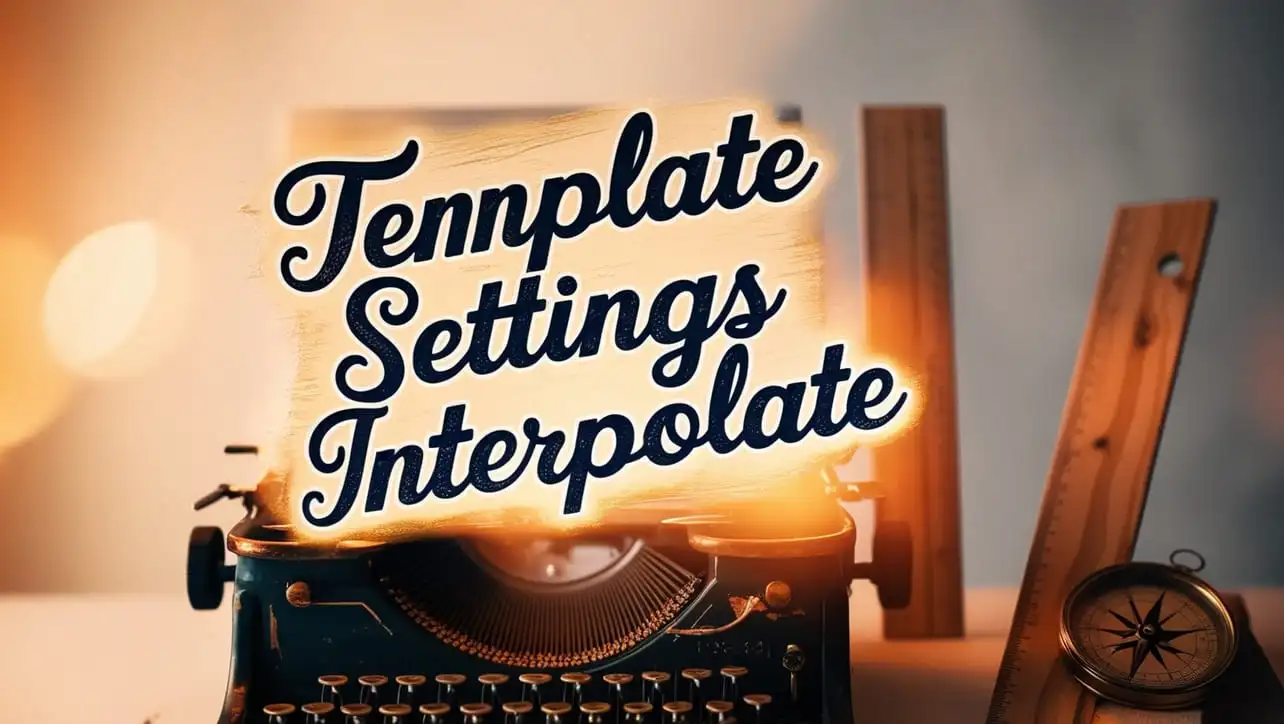
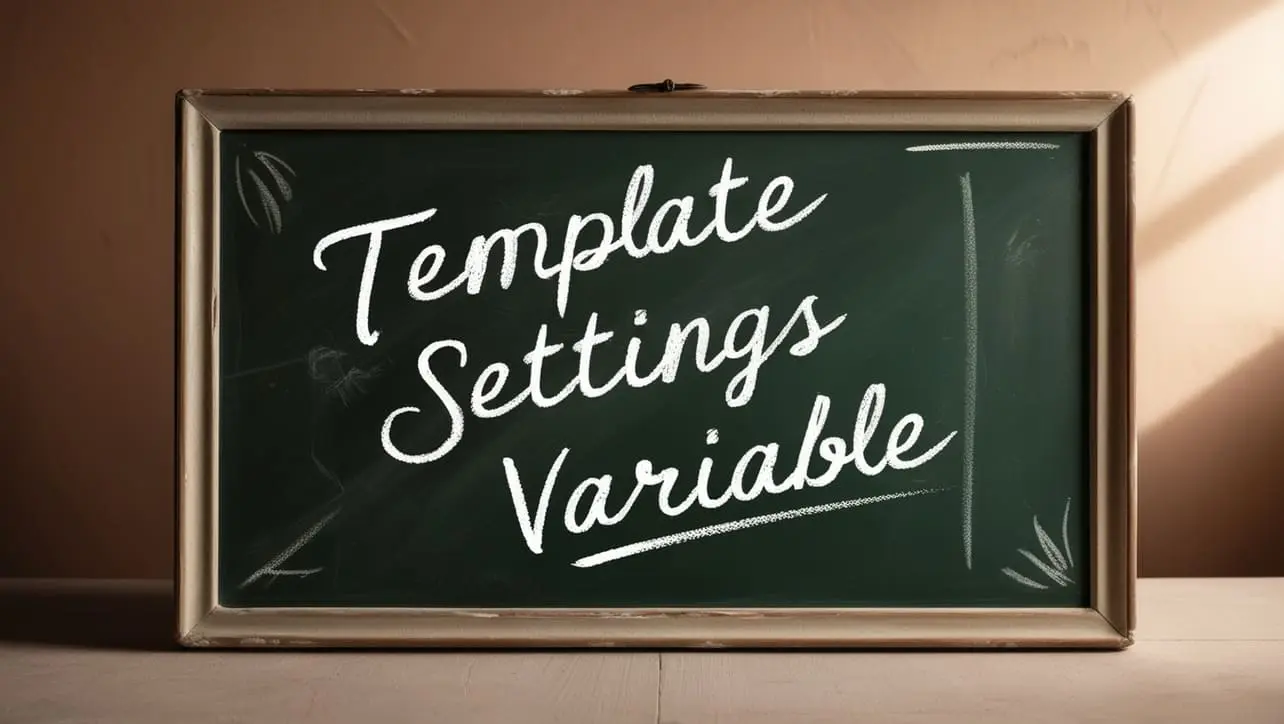
If you have any doubts regarding this article (Lodash _.throttle() Function Method), please comment here. I will help you immediately.