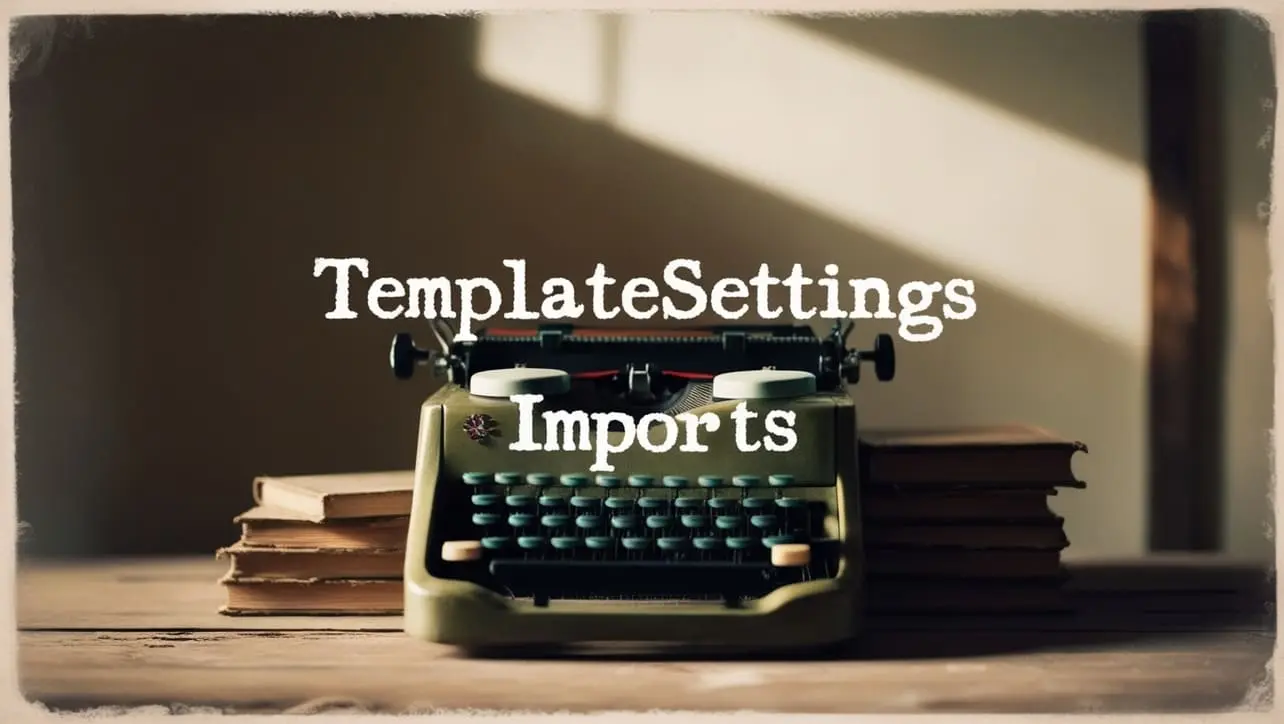
Lodash _.spread() Function Method
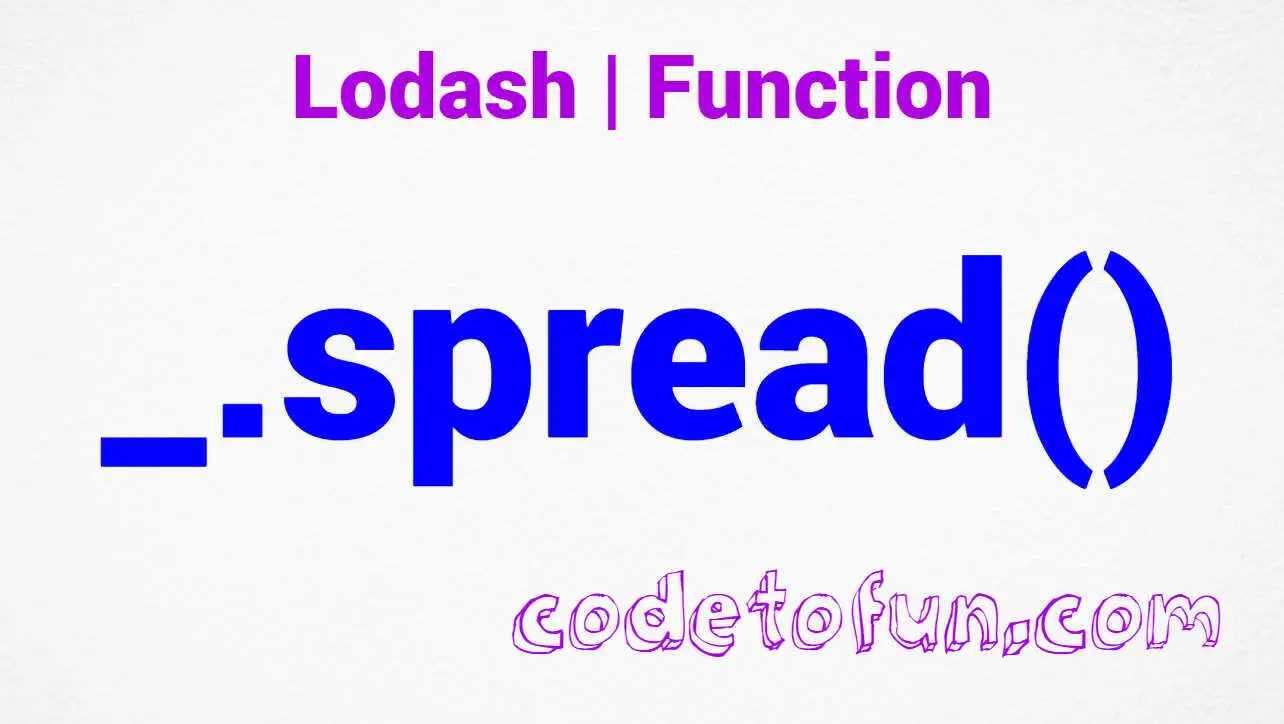
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript development, tools that enhance the flexibility and readability of code are invaluable. The Lodash library, a popular utility library, offers a multitude of functions, and one such gem is the _.spread()
function method. This method plays a key role in simplifying function invocation and improving the overall structure of your code.
In this exploration, we'll delve into the capabilities of _.spread()
and understand how it can be a game-changer in your JavaScript projects.
🧠 Understanding _.spread() Method
The _.spread()
function method in Lodash is designed to transform a function into one that can be called with an array of arguments. This is particularly useful when dealing with functions that expect individual arguments but need to be invoked with an array. _.spread()
facilitates a seamless transition between these scenarios, enhancing the versatility of your code.
💡 Syntax
The syntax for the _.spread()
method is straightforward:
_.spread(func)
- func: The target function to be transformed.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.spread()
method:
const _ = require('lodash');
const greet = (firstName, lastName) => {
console.log(`Hello, ${firstName} ${lastName}!`);
};
const spreadGreet = _.spread(greet);
const namesArray = ['John', 'Doe'];
spreadGreet(namesArray);
// Output: Hello, John Doe!
In this example, the greet function, which expects two separate arguments, is transformed into spreadGreet using _.spread()
. The array namesArray is then spread into individual arguments when invoking spreadGreet.
🏆 Best Practices
When working with the _.spread()
method, consider the following best practices:
Function Compatibility:
Ensure that the target function is compatible with receiving multiple arguments.
_.spread()
is most effective when used with functions that expect individual parameters.example.jsCopiedconst sum = (a, b, c) => a + b + c; const spreadSum = _.spread(sum); console.log(spreadSum([1, 2, 3])); // Output: 6
Handling Varying Argument Lengths:
Consider the variability in the lengths of the argument arrays you might encounter. Use default values or additional checks within your functions to handle different scenarios gracefully.
example.jsCopiedconst printDetails = (name, age) => { console.log(`Name: ${name}, Age: ${age || 'Not specified'}`); }; const spreadPrintDetails = _.spread(printDetails); spreadPrintDetails(['Alice']); // Output: Name: Alice, Age: Not specified
Composing Functions:
Leverage
_.spread()
in conjunction with other Lodash functions, such as _.compose(), to create powerful function compositions.example.jsCopiedconst toUpperCase = str => str.toUpperCase(); const exclaim = str => `${str}!`; const transformAndExclaim = _.compose(exclaim, _.spread(toUpperCase)); console.log(transformAndExclaim(['hello'])); // Output: HELLO!
📚 Use Cases
Array-to-Function Invocation:
_.spread()
is particularly useful when you have an array of values that you want to use as individual arguments when invoking a function.example.jsCopiedconst coordinatesToPosition = (x, y, z) => `Position: (${x}, ${y}, ${z})`; const spreadCoordinates = _.spread(coordinatesToPosition); const coordinates = [10, 20, 30]; console.log(spreadCoordinates(coordinates)); // Output: Position: (10, 20, 30)
Adapting APIs:
When working with APIs that expect individual arguments,
_.spread()
can adapt arrays of data into a format that aligns with the API's requirements.example.jsCopiedconst apiRequest = (endpoint, data) => { // Make API request with endpoint and data console.log(`API request to ${endpoint} with data: ${data}`); }; const spreadApiRequest = _.spread(apiRequest); const requestData = ['/users', { id: 123, name: 'John' }]; spreadApiRequest(requestData); // Output: API request to /users with data: [object Object]
Enhanced Readability:
Improve the readability of your code by using
_.spread()
in situations where the function expects separate arguments, but you have the data in an array.example.jsCopiedconst logDetails = (name, age, city) => { console.log(`Name: ${name}, Age: ${age}, City: ${city}`); }; const spreadLogDetails = _.spread(logDetails); const userDetails = ['Alice', 30, 'Wonderland']; spreadLogDetails(userDetails); // Output: Name: Alice, Age: 30, City: Wonderland
🎉 Conclusion
The _.spread()
function method in Lodash empowers developers to seamlessly transition between array-based and individual argument-based function invocations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.spread()
method in your Lodash projects.
👨💻 Join our Community:
Author
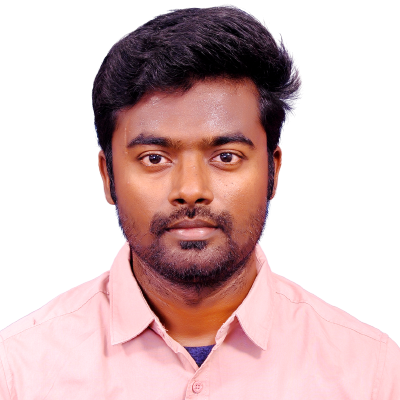
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
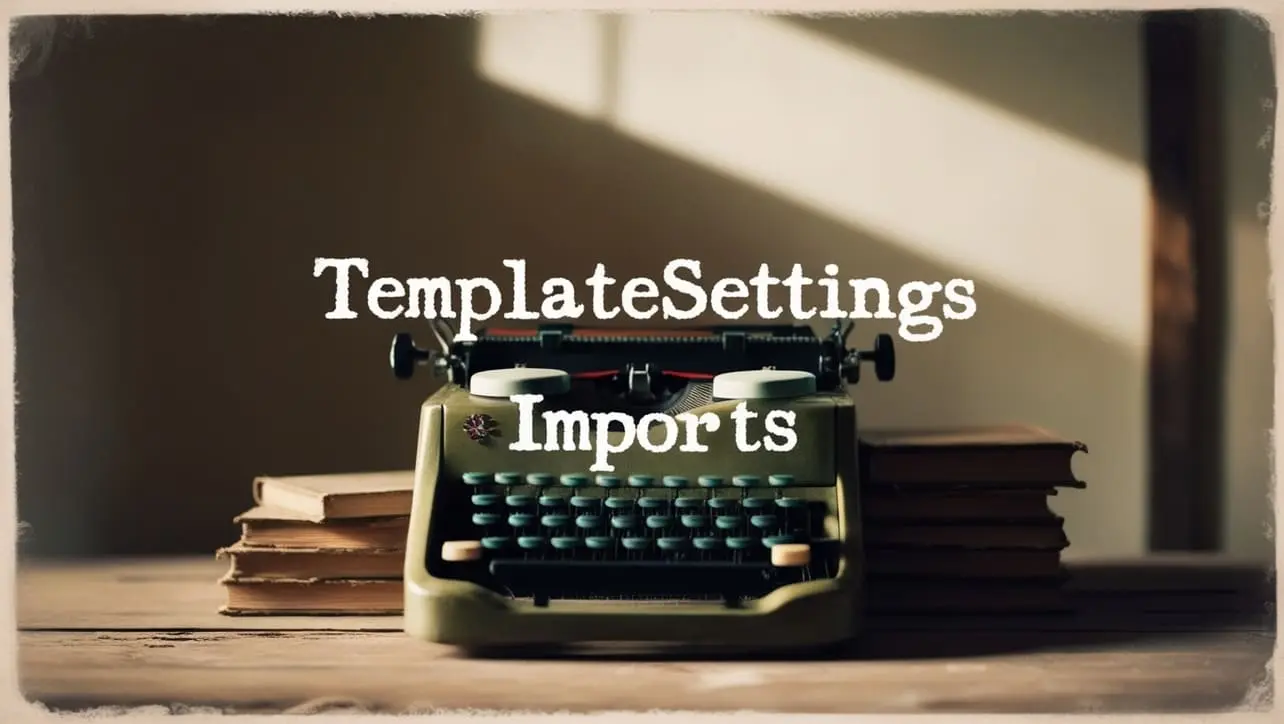
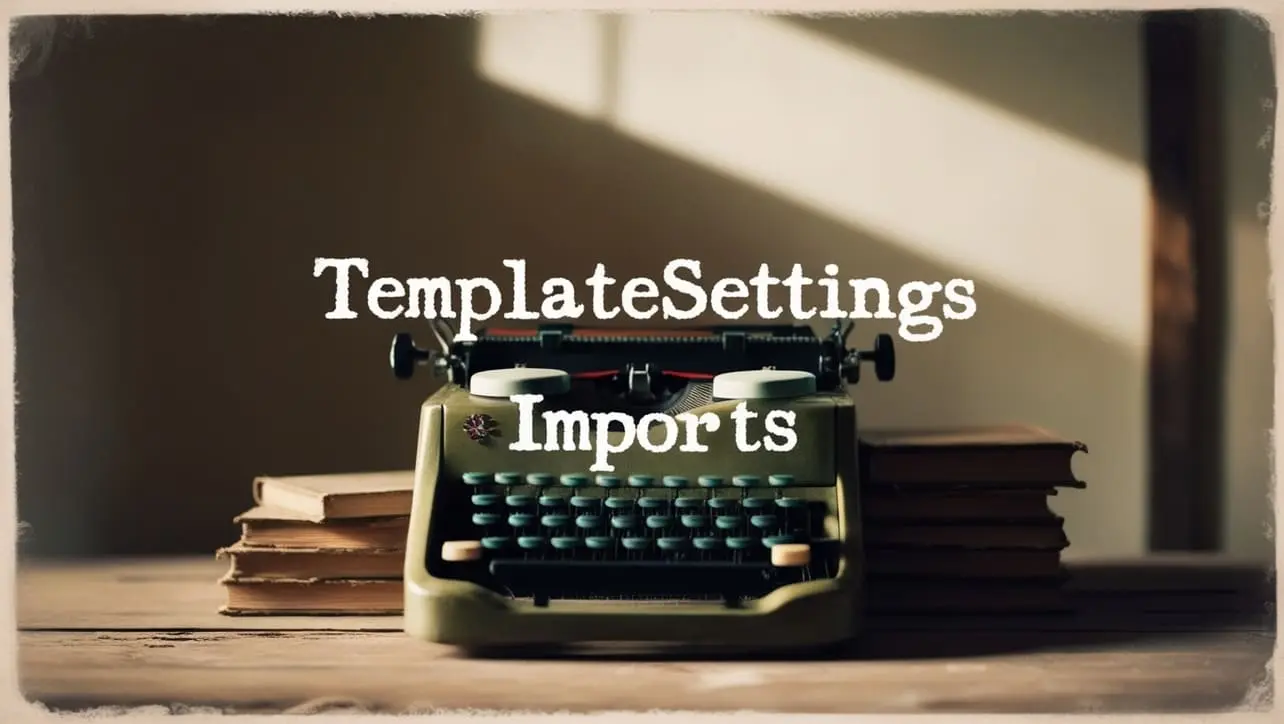
Lodash _.templateSettings.imports Property
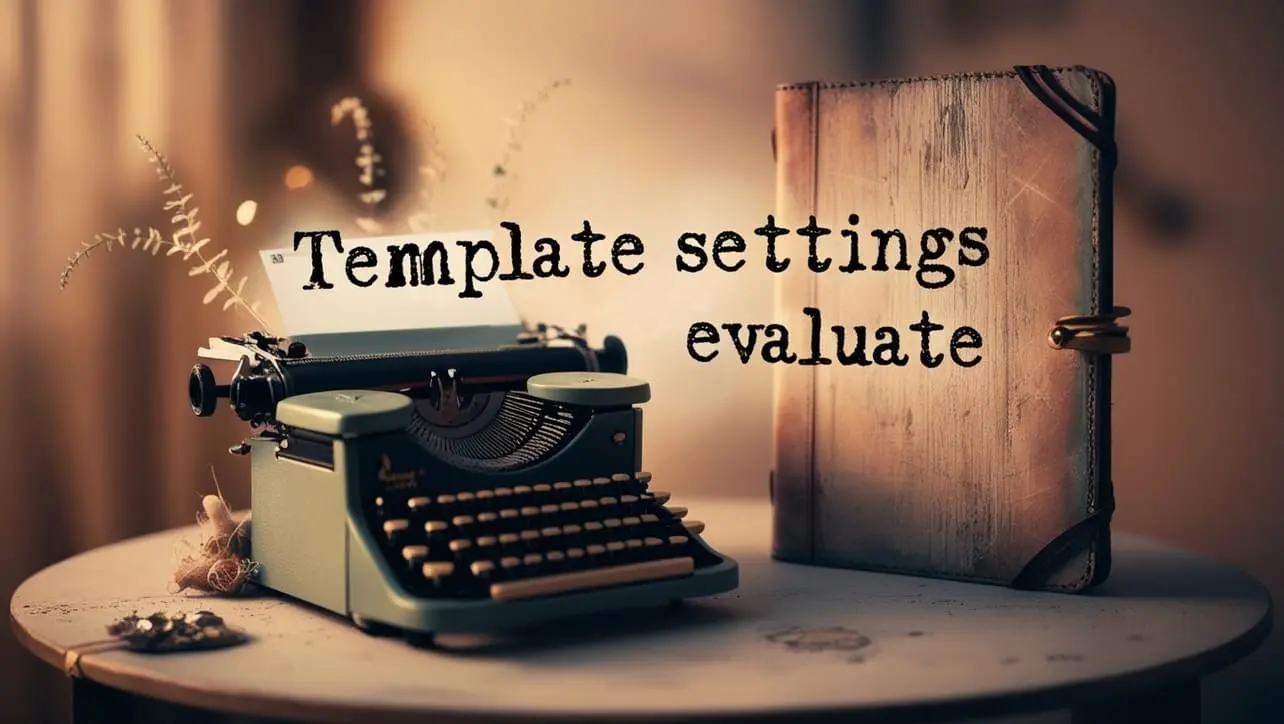
Lodash _.templateSettings.evaluate Property
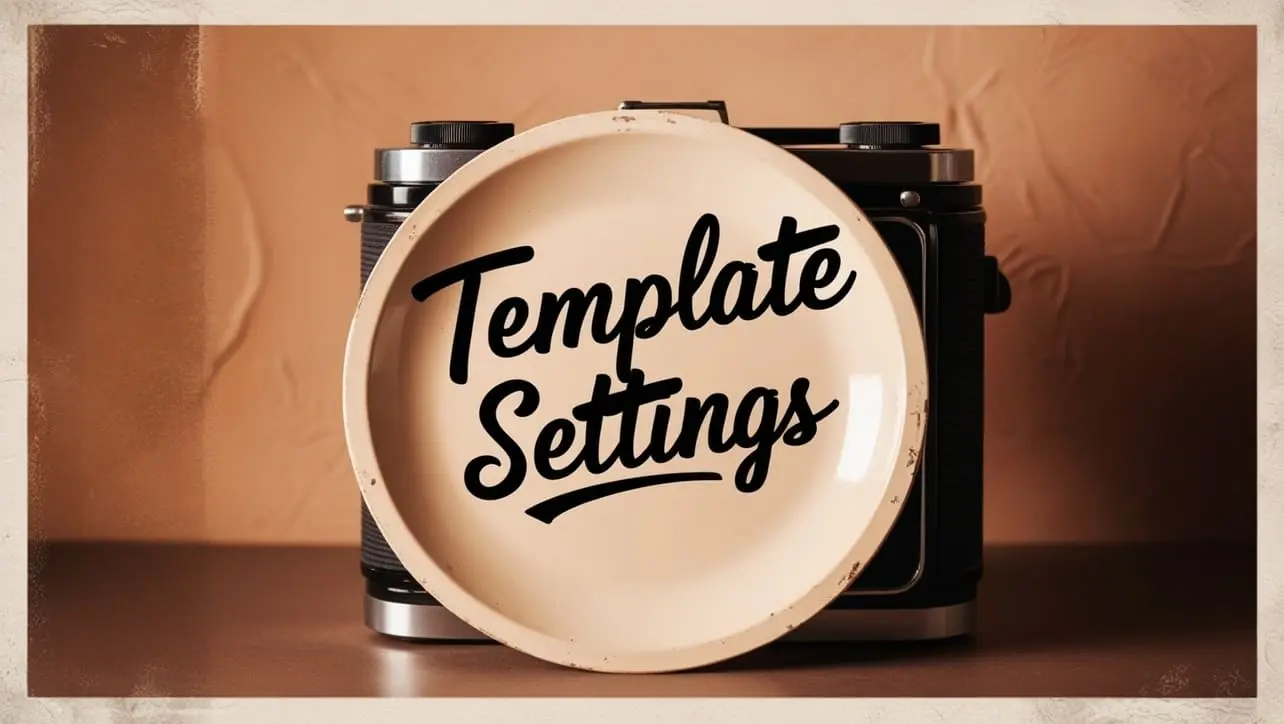
Lodash _.templateSettings Property
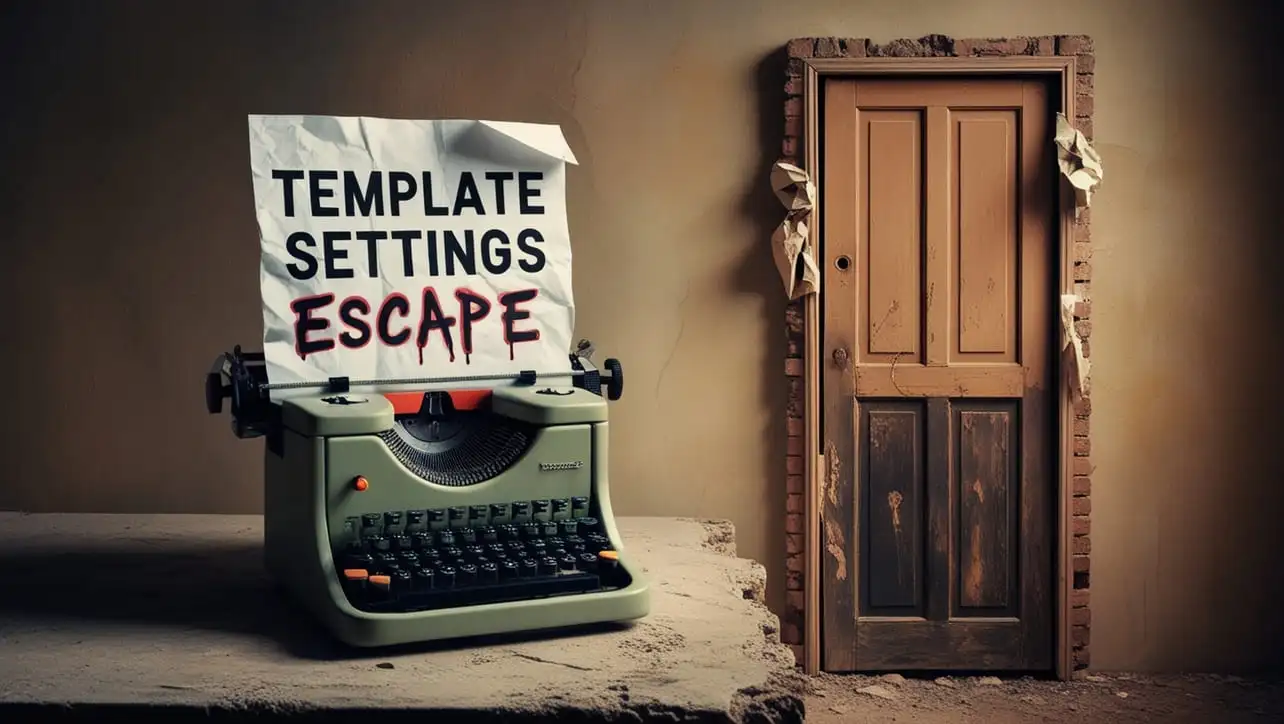
Lodash _.templateSettings.escape Property
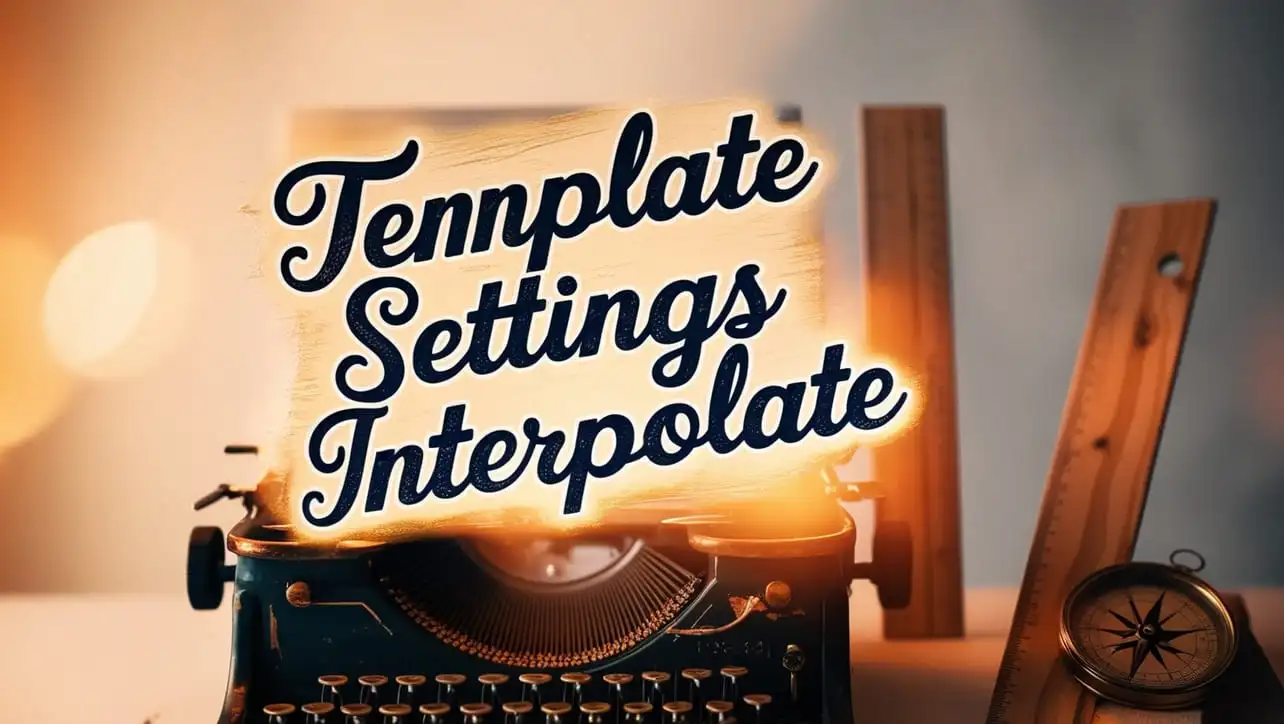
Lodash _.templateSettings.interpolate Property
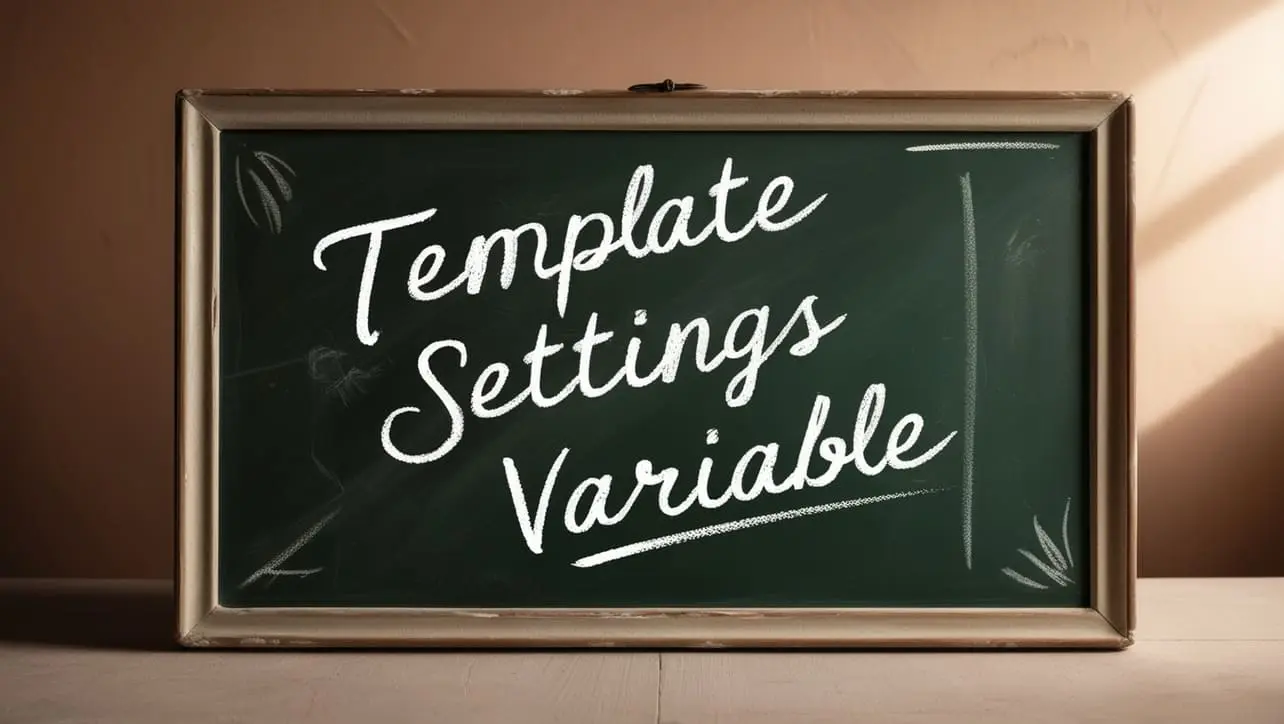
If you have any doubts regarding this article (Lodash _.spread() Function Method), please comment here. I will help you immediately.