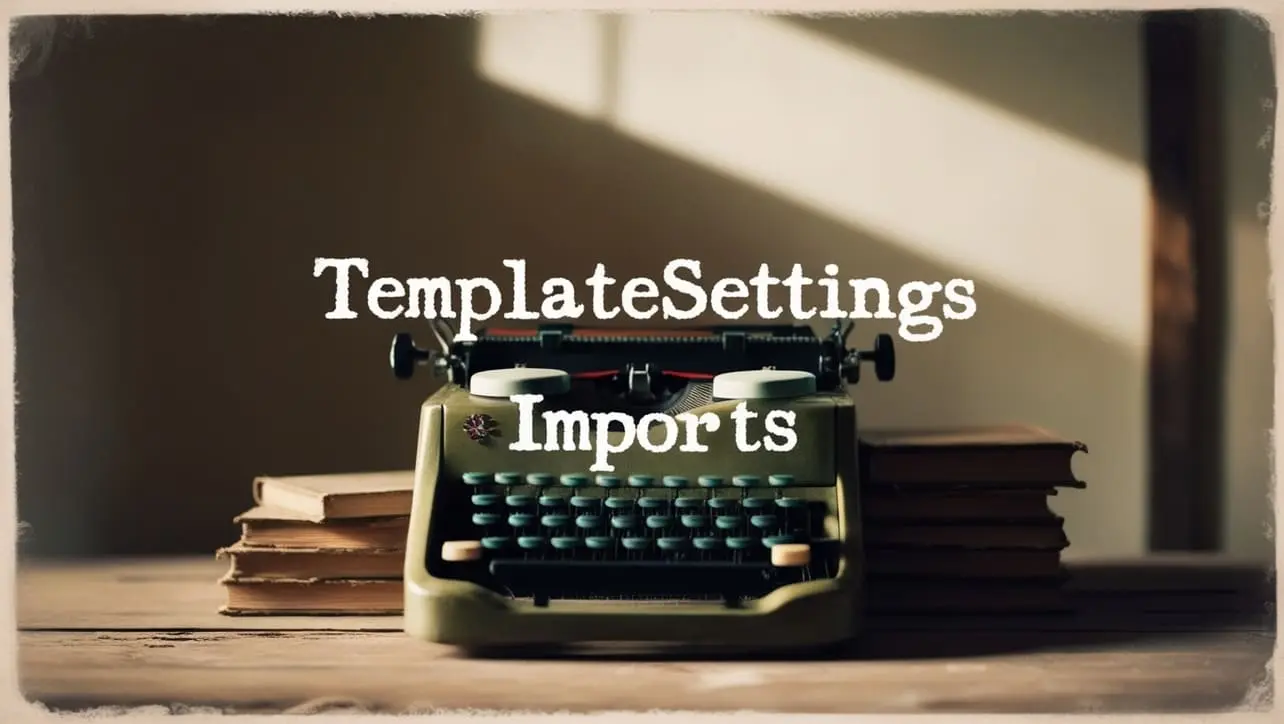
Lodash _.rearg() Function Method
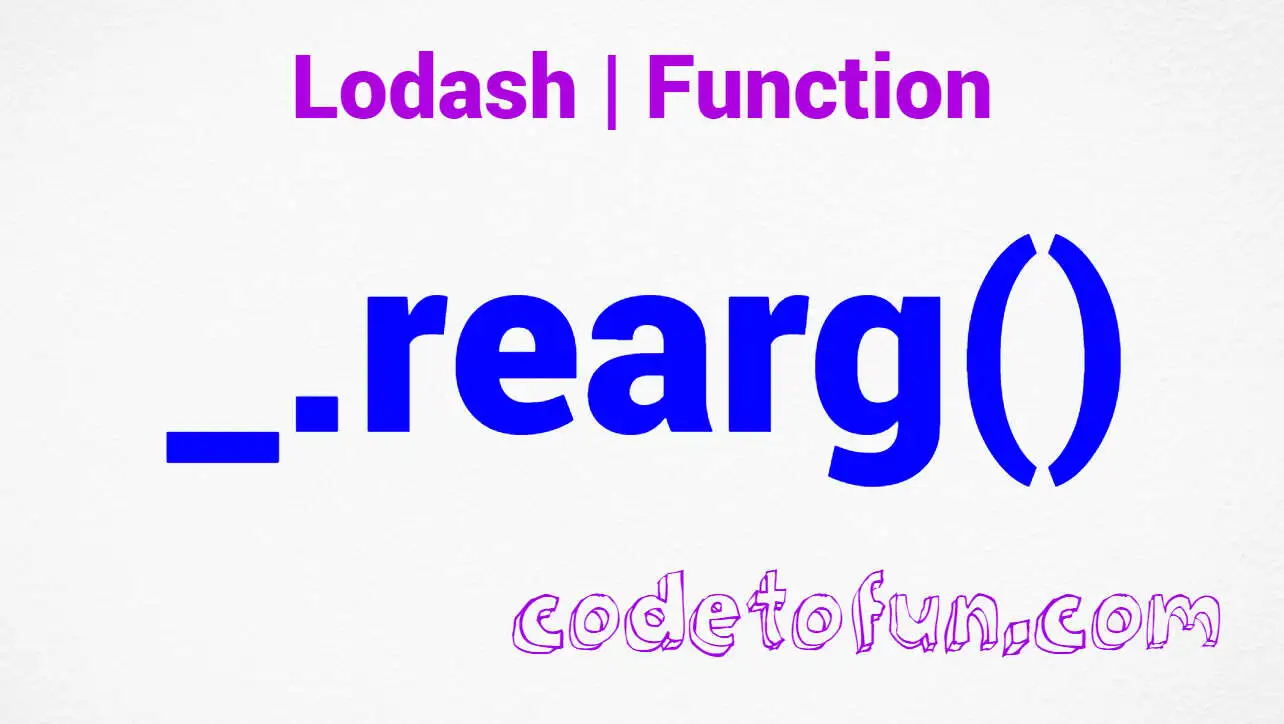
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript programming, functional programming paradigms often play a crucial role. Lodash, a comprehensive utility library, provides an array of methods to facilitate functional programming. Among these, the _.rearg()
method stands out, offering a powerful way to rearrange the arguments of a function.
This can lead to cleaner and more maintainable code, especially when dealing with functions that accept multiple parameters.
🧠 Understanding _.rearg() Method
The _.rearg()
method in Lodash allows you to create a new function with specified argument positions rearranged according to a given order. This can be particularly beneficial when you want to reuse an existing function but need to adjust the order of its arguments.
💡 Syntax
The syntax for the _.rearg()
method is straightforward:
_.rearg(func, indexes)
- func: The function to rearrange.
- indexes: The new order of argument indexes.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.rearg()
method:
const _ = require('lodash');
function originalFunction(a, b, c) {
return [a, b, c];
}
const rearrangedFunction = _.rearg(originalFunction, [2, 0, 1]);
console.log(rearrangedFunction(1, 2, 3));
// Output: [3, 1, 2]
In this example, originalFunction takes three arguments (a, b, c), and _.rearg()
creates a new function (rearrangedFunction) with the order of arguments rearranged to [2, 0, 1].
🏆 Best Practices
When working with the _.rearg()
method, consider the following best practices:
Understand the Original Function:
Before applying
_.rearg()
, thoroughly understand the purpose and argument order of the original function. This ensures that the rearrangement aligns with the intended functionality.example.jsCopiedfunction originalMultiply(a, b) { return a * b; } const rearrangedMultiply = _.rearg(originalMultiply, [1, 0]); console.log(rearrangedMultiply(3, 4)); // Output: 12
Use Meaningful Indexes:
When specifying argument indexes, use meaningful values that clearly indicate the purpose of each argument. This enhances code readability and maintenance.
example.jsCopiedfunction logDetails(name, age, profession) { console.log(`Name: ${name}, Age: ${age}, Profession: ${profession}`); } const rearrangedLog = _.rearg(logDetails, [2, 0, 1]); rearrangedLog('John', 30, 'Engineer'); // Output: Name: Engineer, Age: John, Profession: 30
Consider Currying:
If your function supports currying, take advantage of it alongside
_.rearg()
for even greater flexibility in argument manipulation.example.jsCopiedfunction curryExample(a, b, c) { return a + b + c; } const curriedAndRearranged = _.curry(_.rearg(curryExample, [2, 0, 1])); console.log(curriedAndRearranged(1)(2)(3)); // Output: 6
📚 Use Cases
Adapting to External APIs:
When working with external APIs that expect parameters in a different order,
_.rearg()
can adapt your internal functions to meet the API requirements.example.jsCopiedfunction internalFormatData(name, age, country) { // ...format data... } const externalApiFormat = _.rearg(internalFormatData, [0, 2, 1]); // Now, use externalApiFormat to comply with the API expectations. externalApiFormat('John', 'USA', 30);
Enhancing Readability:
In functions with a large number of parameters, using
_.rearg()
to reorder arguments can significantly enhance code readability by emphasizing the most crucial parameters.example.jsCopiedfunction complexFunction(param1, param2, param3, param4, param5) { // ...function logic... } const simplifiedFunction = _.rearg(complexFunction, [0, 2, 1, 4, 3]); // Use simplifiedFunction with a clearer argument order.
Legacy Code Integration:
When dealing with legacy code that expects parameters in a different order,
_.rearg()
provides a seamless way to integrate and adapt existing functions.example.jsCopiedfunction legacyApiFunction(firstName, lastName, age) { // ...legacy API logic... } const modernizedApiFunction = _.rearg(legacyApiFunction, [0, 2, 1]); // Use modernizedApiFunction with a more modern and intuitive argument order.
🎉 Conclusion
The _.rearg()
method in Lodash is a valuable tool for enhancing the flexibility and readability of your JavaScript code. Whether you're adapting to external APIs, improving readability, or integrating with legacy code, _.rearg()
provides a clean and efficient solution for rearranging function arguments.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.rearg()
method in your Lodash projects.
👨💻 Join our Community:
Author
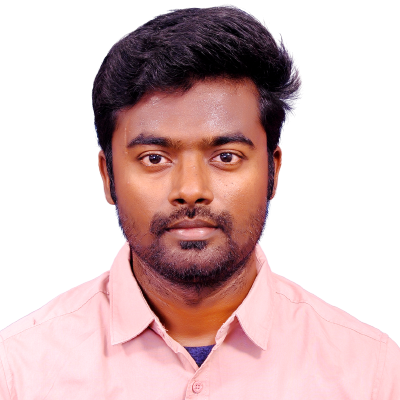
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
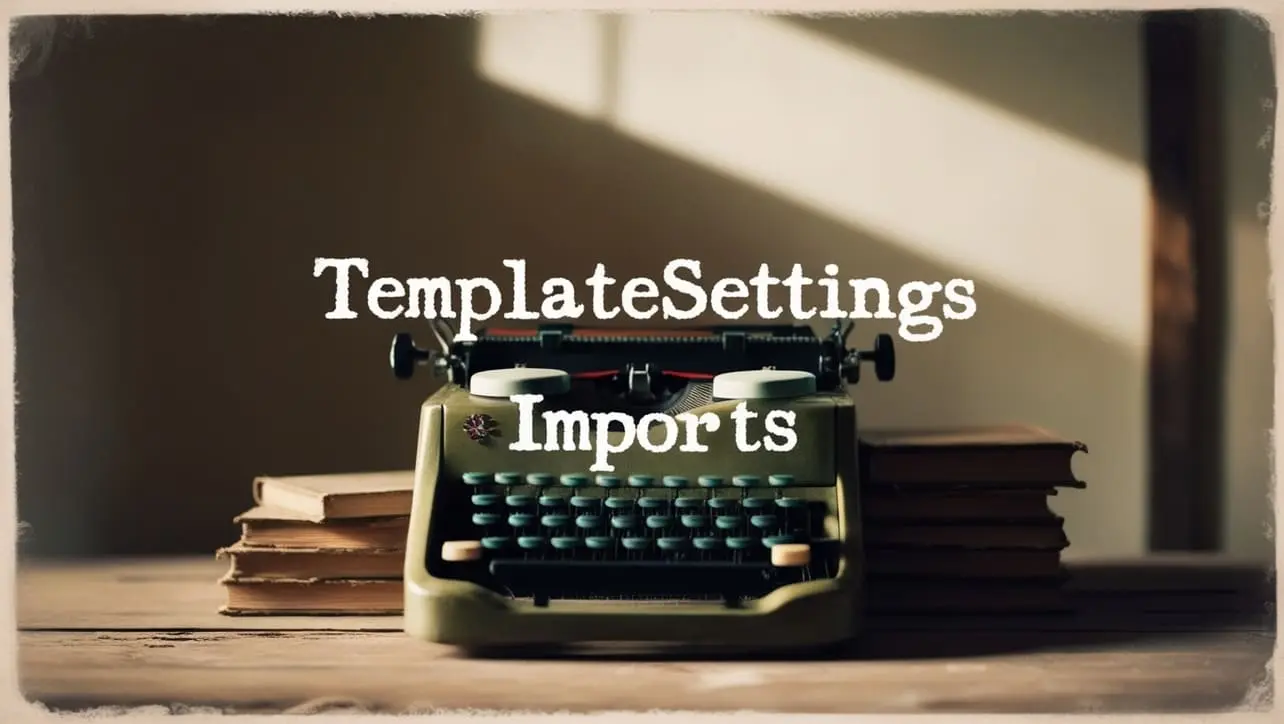
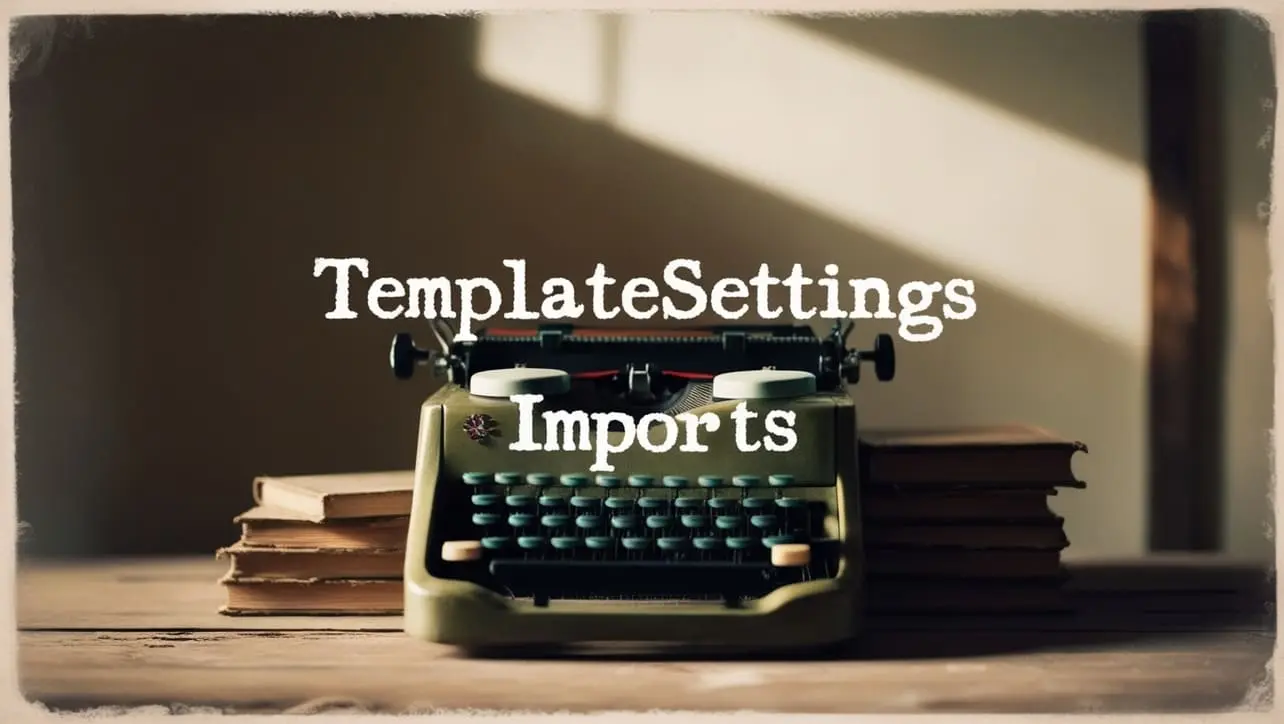
Lodash _.templateSettings.imports Property
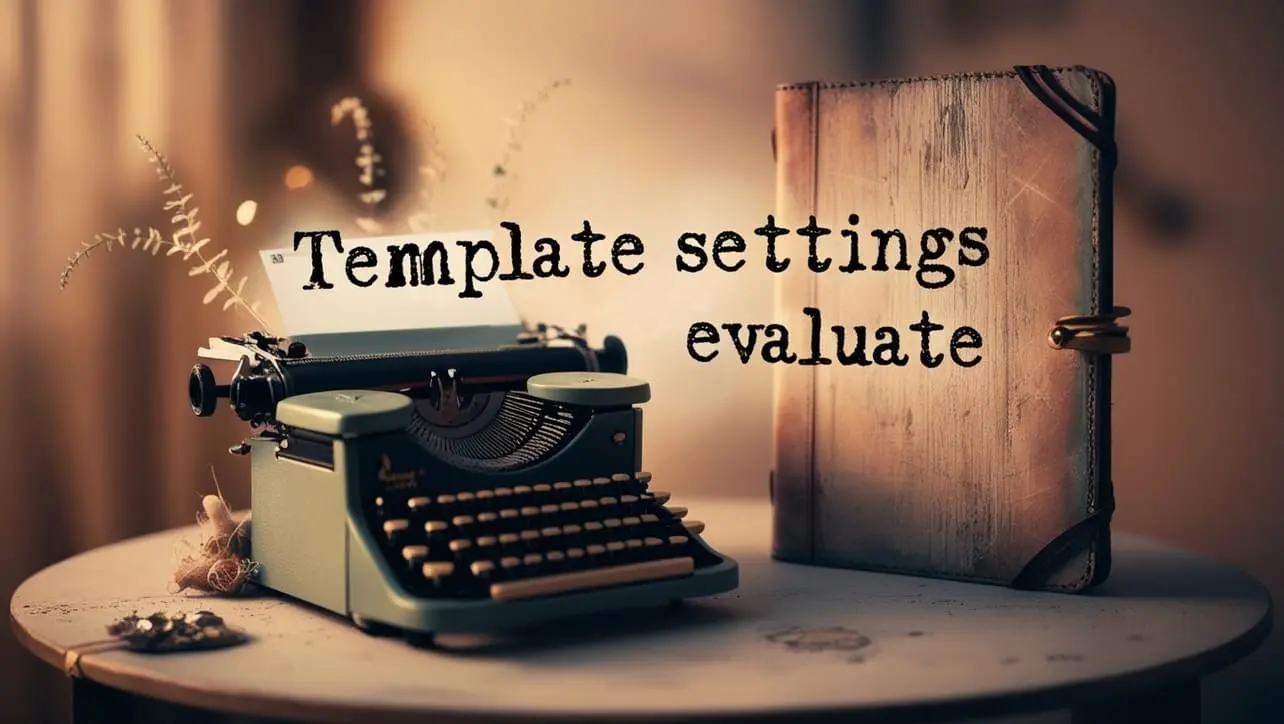
Lodash _.templateSettings.evaluate Property
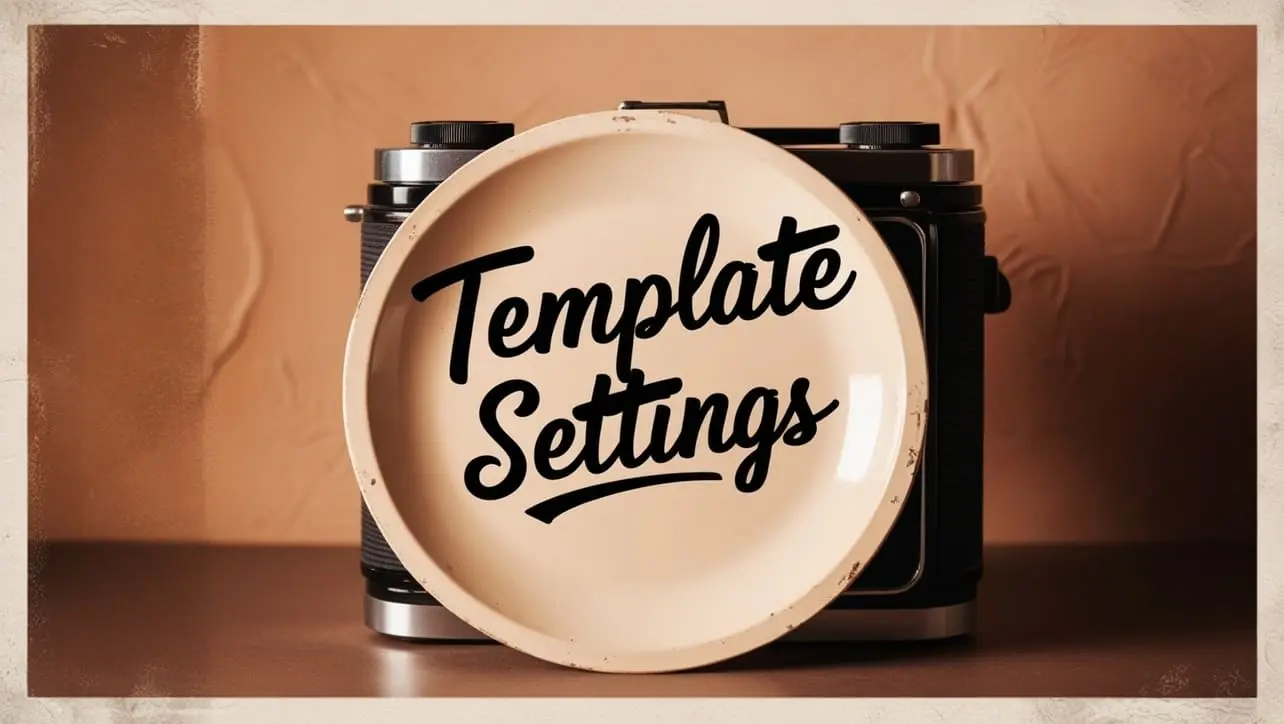
Lodash _.templateSettings Property
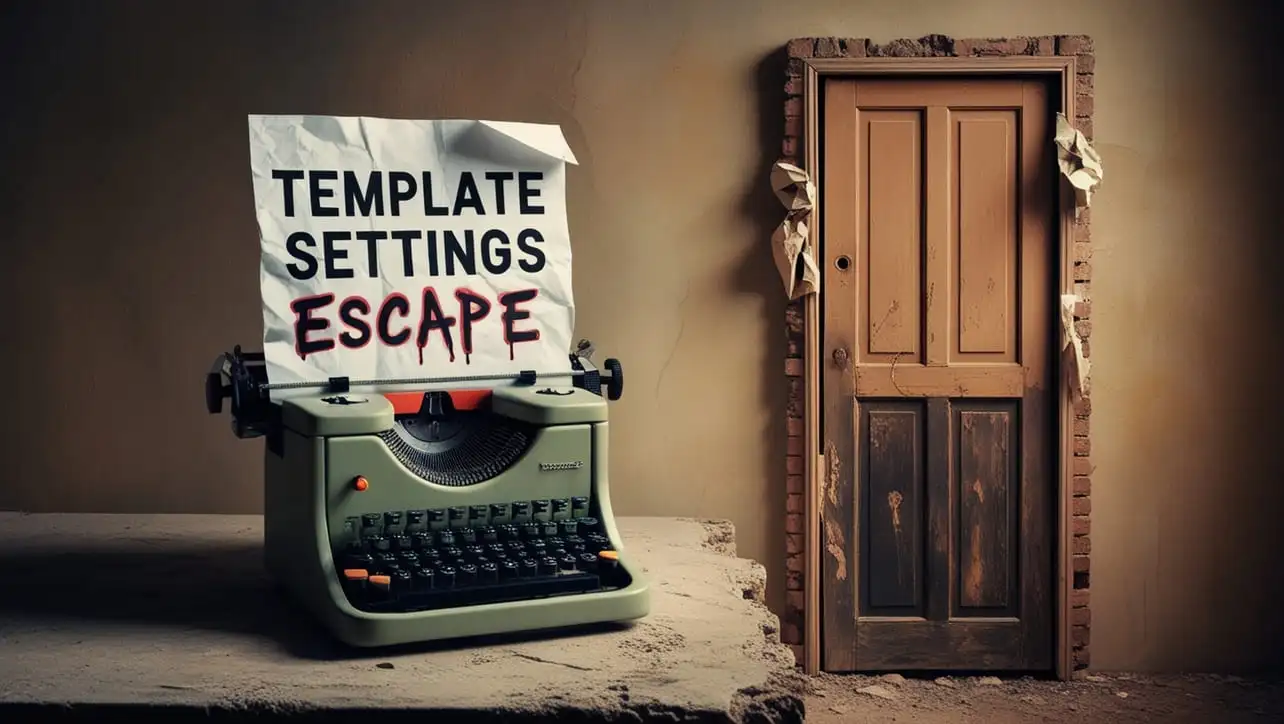
Lodash _.templateSettings.escape Property
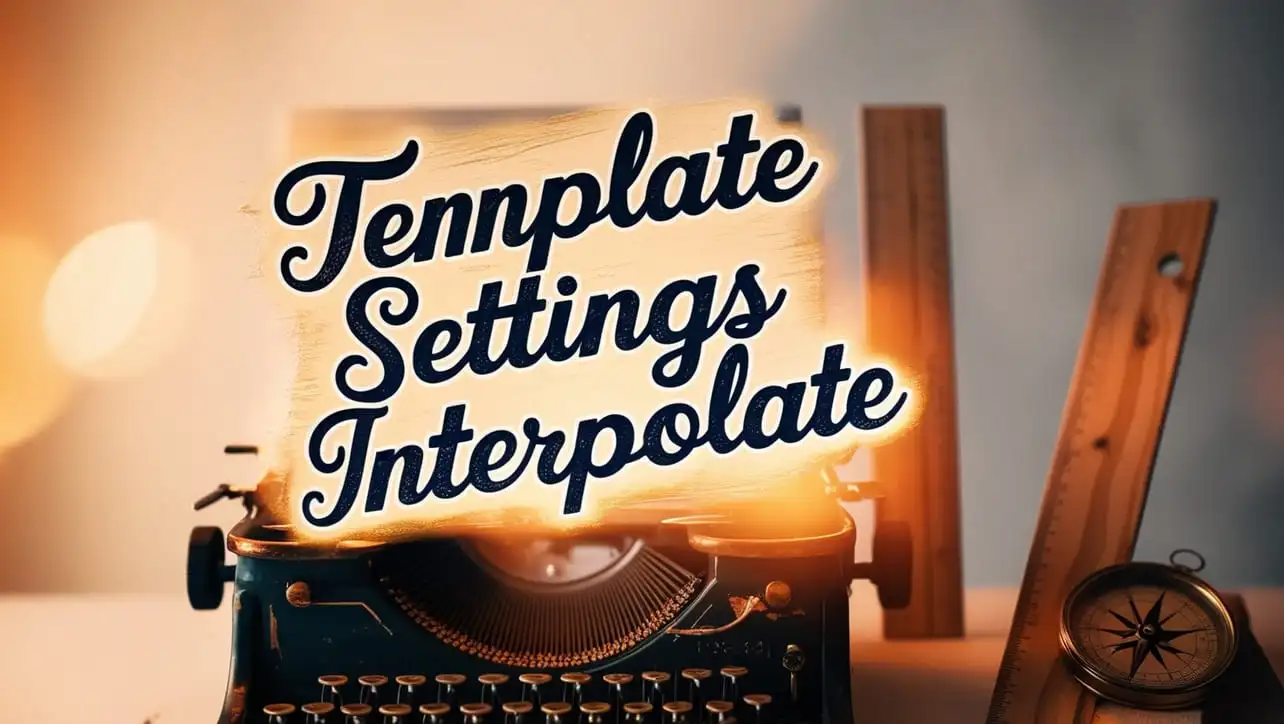
Lodash _.templateSettings.interpolate Property
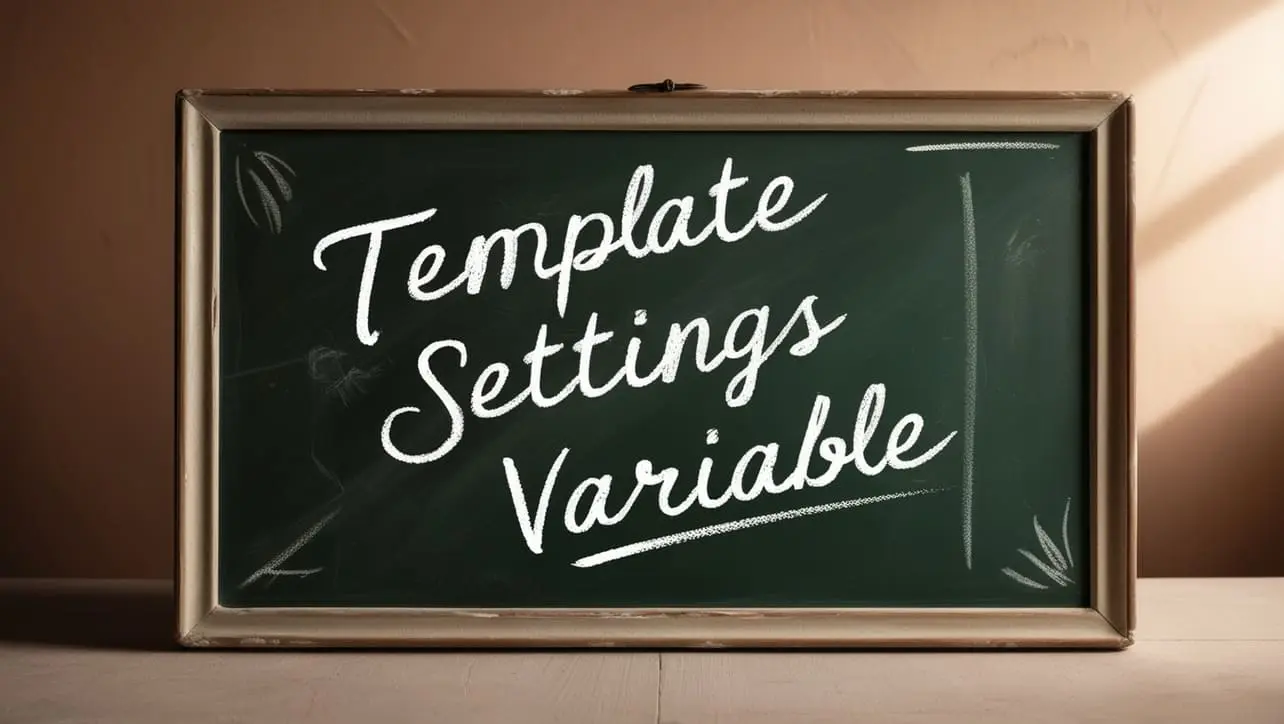
If you have any doubts regarding this article (Lodash _.rearg() Function Method), please comment here. I will help you immediately.