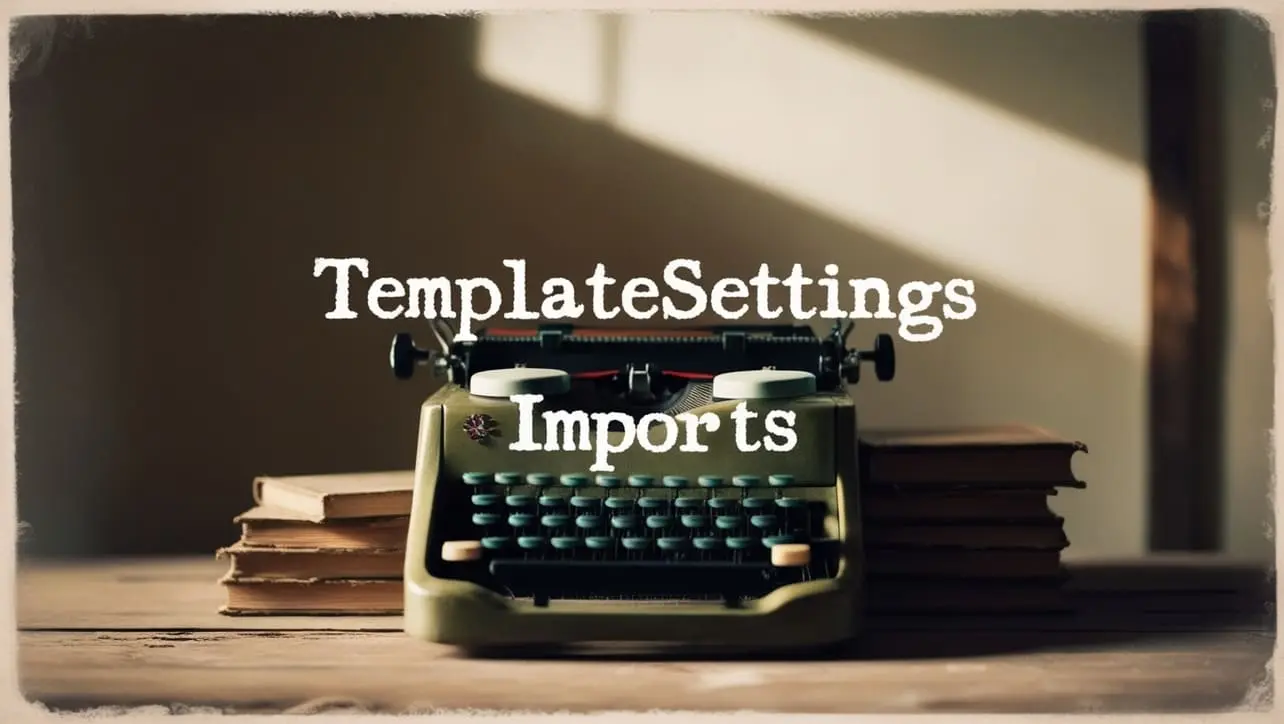
Lodash _.delay() Function Method
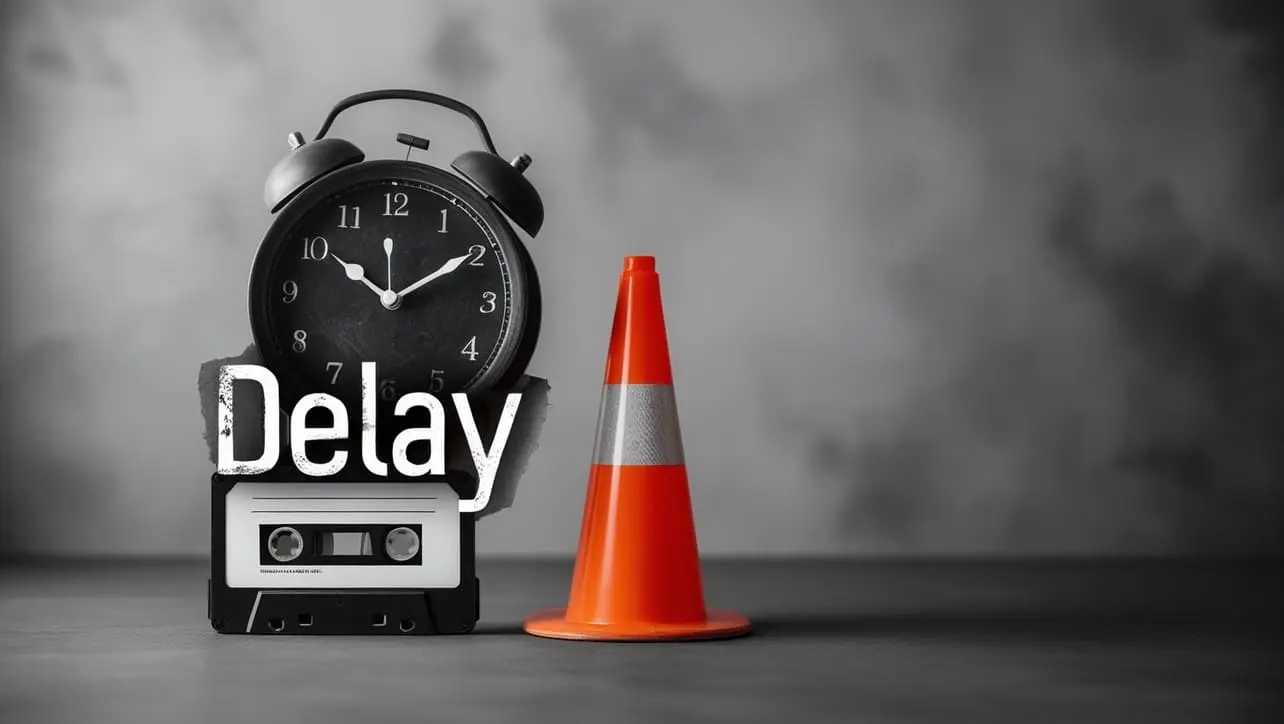
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic world of JavaScript, timing is often critical. Whether you're working with animations, asynchronous operations, or simply want to introduce delays in your code execution, the _.delay()
function method in Lodash can be a valuable tool.
This method allows you to defer the execution of a function, enhancing control over the flow of your code.
🧠 Understanding _.delay()
Method
The _.delay()
function in Lodash is designed to postpone the execution of a given function by a specified duration. This can be particularly useful in scenarios where you need to introduce delays between operations or when dealing with asynchronous tasks.
💡 Syntax
The syntax for the _.delay()
method is straightforward:
_.delay(func, wait, [args])
- func: The function to delay.
- wait: The number of milliseconds to delay the function execution.
- args (Optional): Arguments to pass to the function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.delay()
method:
const _ = require('lodash');
function greet(name) {
console.log(`Hello, ${name}!`);
}
// Delay execution of the greet function by 2000 milliseconds (2 seconds)
_.delay(greet, 2000, 'John');
In this example, the greet function is delayed by 2000 milliseconds, and it will be invoked with the argument 'John' after the specified delay.
🏆 Best Practices
When working with the _.delay()
method, consider the following best practices:
Proper Function Binding:
Ensure that the function passed to
_.delay()
is properly bound if it relies on this context. This ensures that the function behaves as expected when executed after the delay.example.jsCopiedconst _ = require('lodash'); const obj = { name: 'Alice', greet: function () { console.log(`Hello, ${this.name}!`); }, }; // Incorrect: The function loses its context _.delay(obj.greet, 2000); // Correct: Ensure proper binding _.delay(obj.greet.bind(obj), 2000);
Use Arrow Functions for Lexical Scoping:
When using functions that rely on lexical scoping, consider using arrow functions to preserve the context.
example.jsCopiedconst _ = require('lodash'); const obj = { name: 'Bob', greet: function () { _.delay(() => { console.log(`Hello, ${this.name}!`); }, 2000); }, }; obj.greet();
Handle Potential Edge Cases:
Consider potential edge cases, such as delays with very short intervals or negative delays. Understanding the behavior in such cases is crucial for proper usage.
example.jsCopiedconst _ = require('lodash'); // Delay with a very short interval (not recommended) _.delay(() => console.log('Delayed!'), 1); // Negative delay (executes immediately) _.delay(() => console.log('Negative Delay!'), -1000);
📚 Use Cases
User Feedback in UI:
Introduce delays to provide user feedback in the UI. For example, displaying a success message for a few seconds before hiding it.
example.jsCopiedconst _ = require('lodash'); function showSuccessMessage(message) { console.log(`Success: ${message}`); } function hideSuccessMessage() { console.log('Hiding success message'); } // Display success message and hide it after 3000 milliseconds (3 seconds) showSuccessMessage('Task completed successfully'); _.delay(hideSuccessMessage, 3000);
Debouncing User Input:
Use
_.delay()
in combination with user input to debounce function calls. This is beneficial when dealing with rapid user input, such as in search boxes.example.jsCopiedconst _ = require('lodash'); function performSearch(query) { // Perform search operation with the provided query console.log(`Searching for: ${query}`); } // Delay search execution by 1000 milliseconds (1 second) after user stops typing const debounceSearch = _.debounce(performSearch, 1000); // Simulate user input debounceSearch('L'); debounceSearch('Lo'); debounceSearch('Lod'); debounceSearch('Lodas'); _.delay(() => debounceSearch('Lodash'), 1500);
Asynchronous Operations:
Integrate delays when dealing with asynchronous operations to control the timing of function executions.
example.jsCopiedconst _ = require('lodash'); function fetchData() { // Simulate fetching data asynchronously return new Promise(resolve => { _.delay(() => { resolve({ data: 'Fetched data' }); }, 2000); }); } async function processFetchedData() { const result = await fetchData(); console.log(result.data); } processFetchedData();
🎉 Conclusion
The _.delay()
function method in Lodash is a versatile tool for introducing delays in JavaScript code. Whether you're enhancing user experience in the UI, debouncing user input, or managing asynchronous operations, _.delay()
provides a straightforward way to control the timing of function executions.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.delay()
method in your Lodash projects.
👨💻 Join our Community:
Author
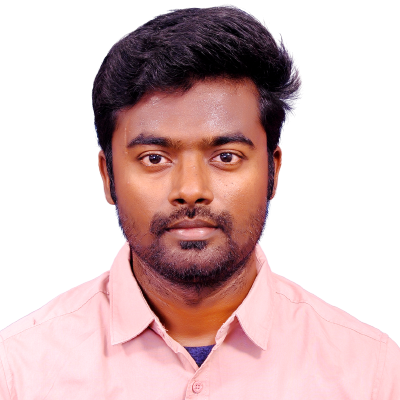
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
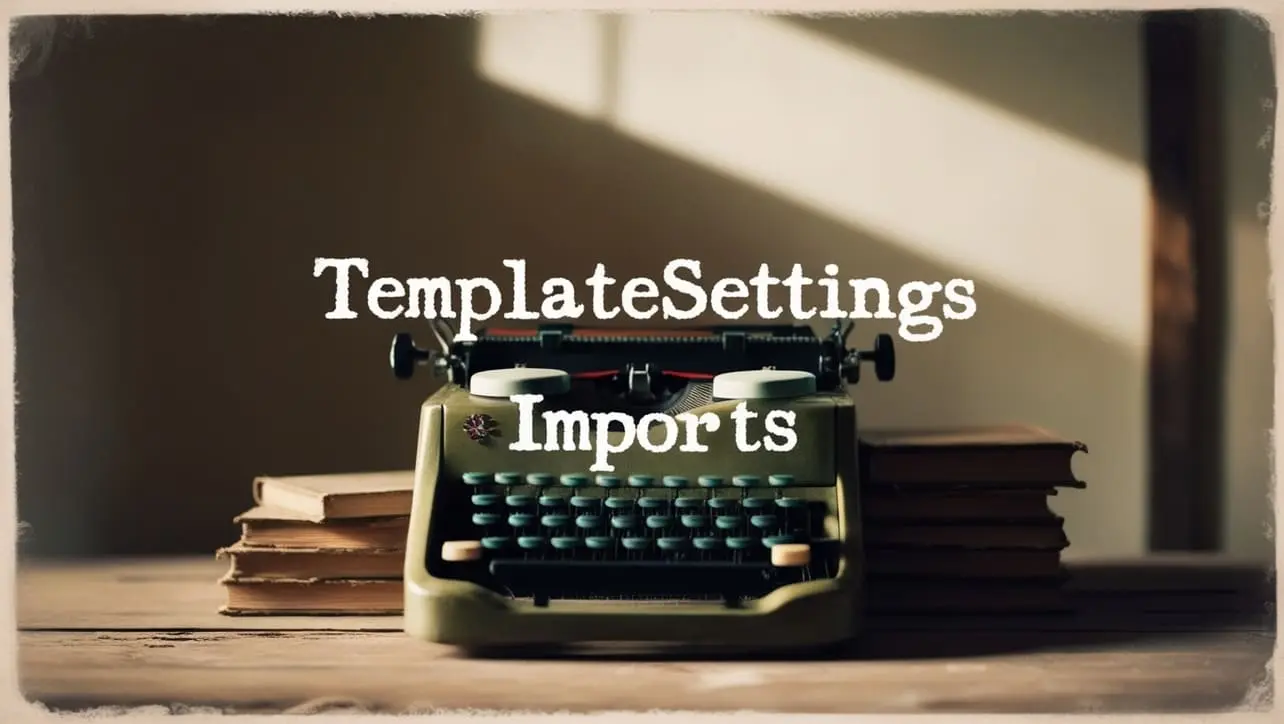
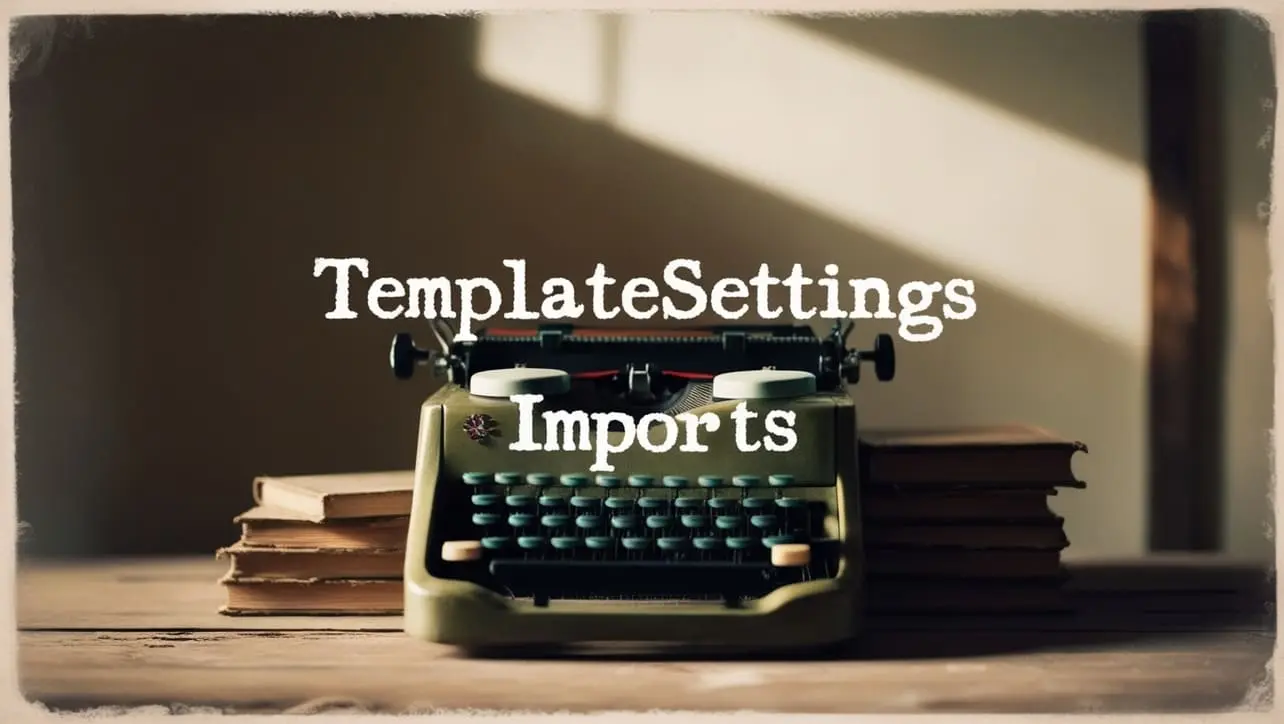
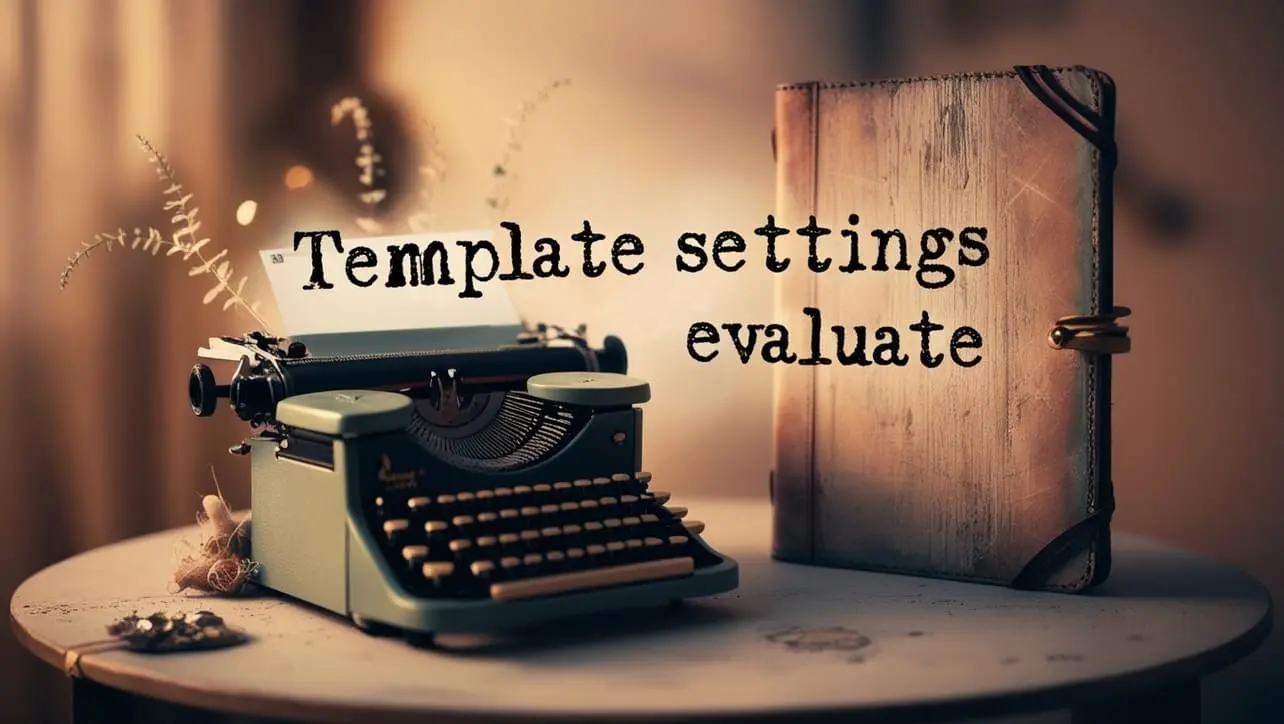
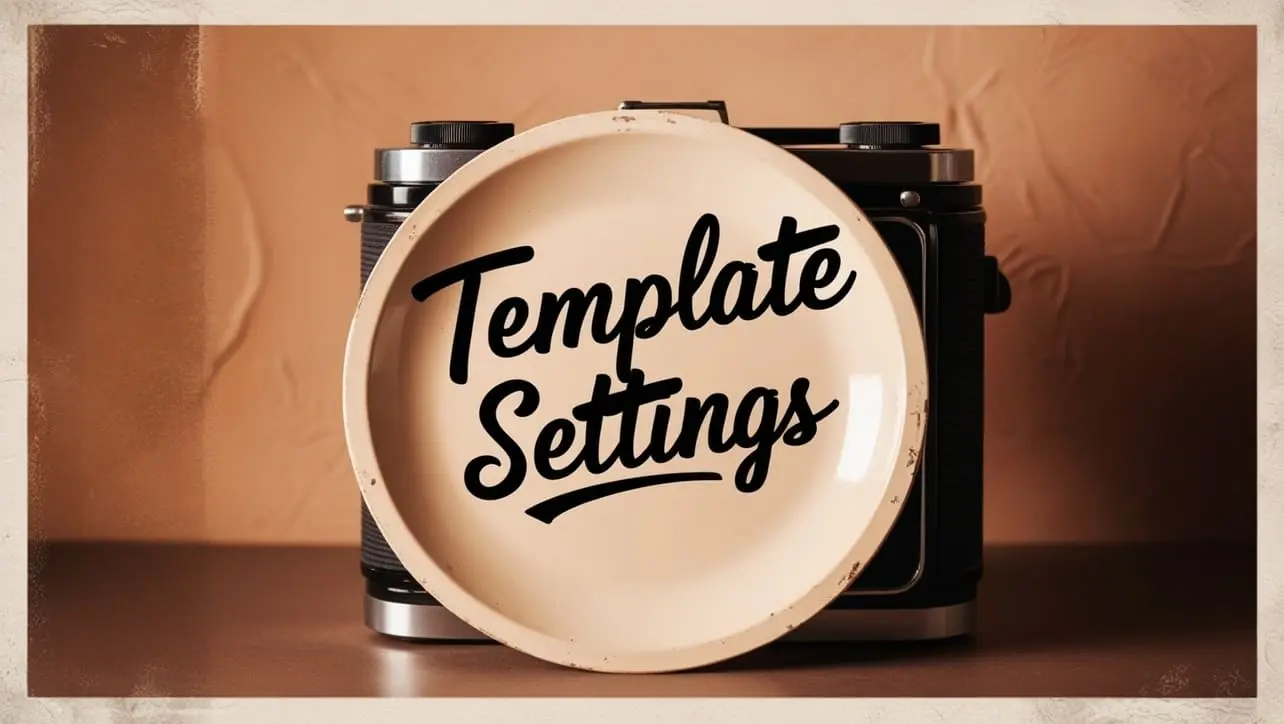
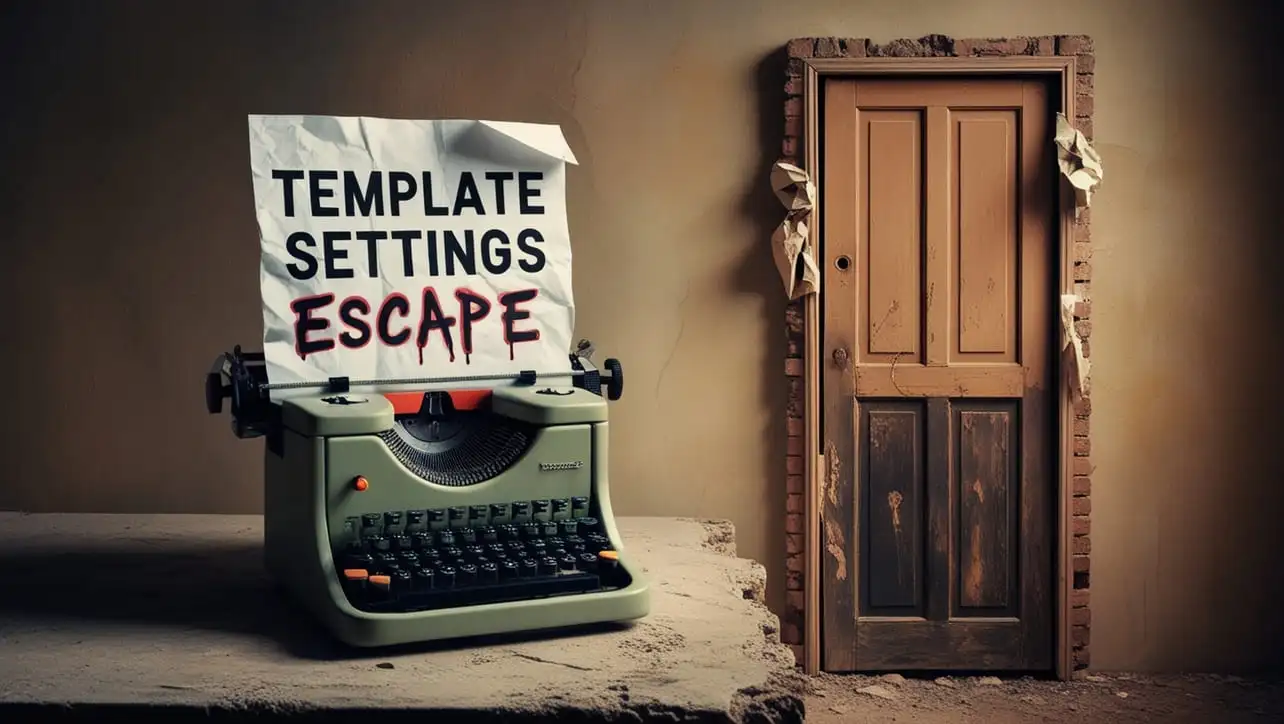
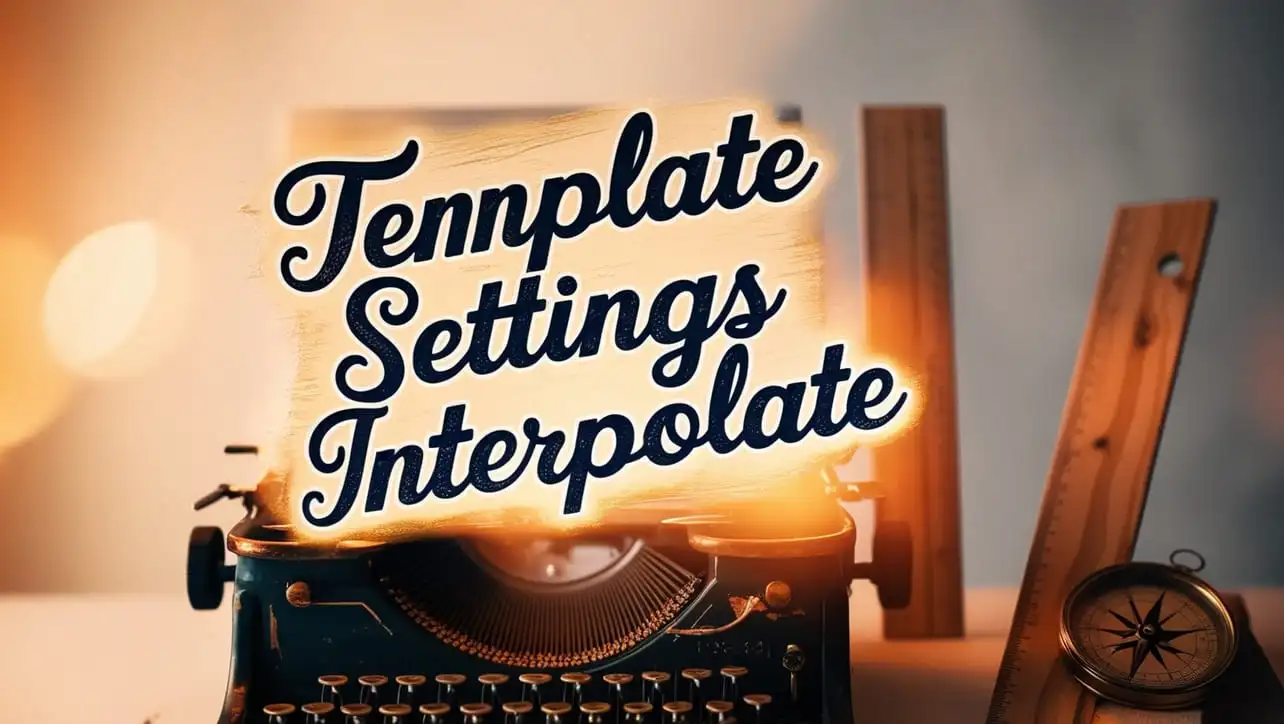
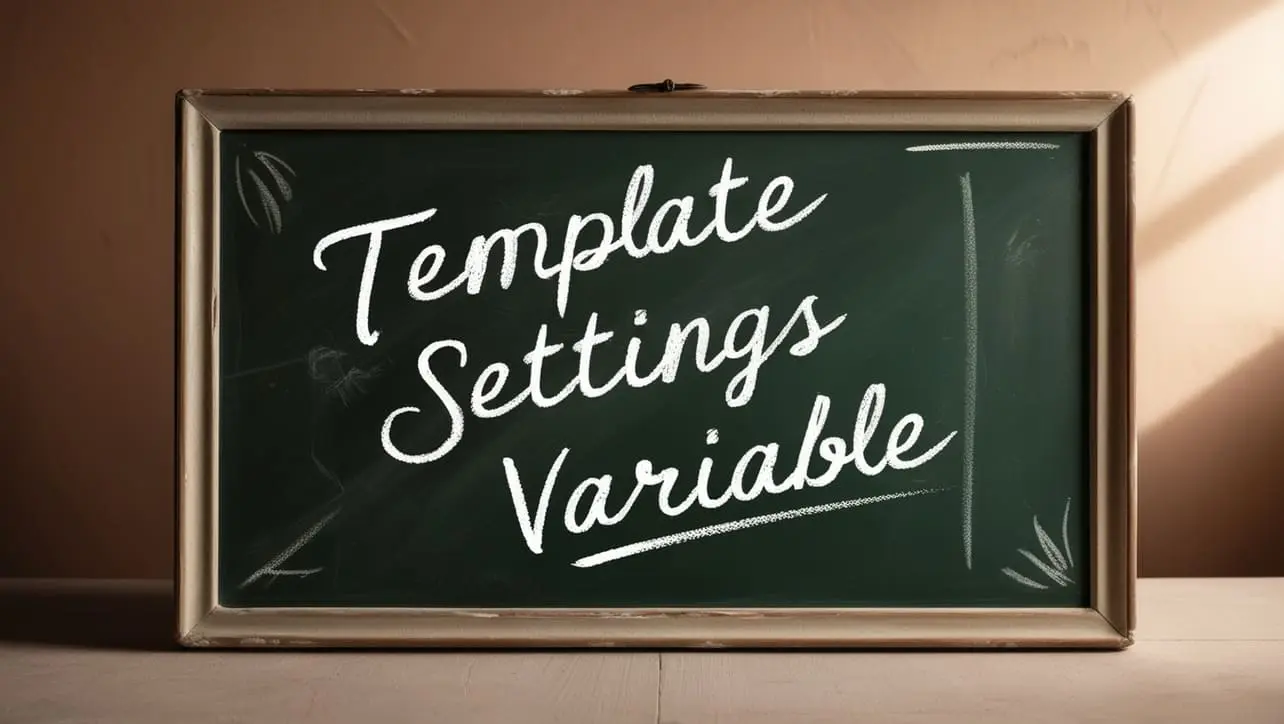
If you have any doubts regarding this article (Lodash _.delay() Function Method), please comment here. I will help you immediately.