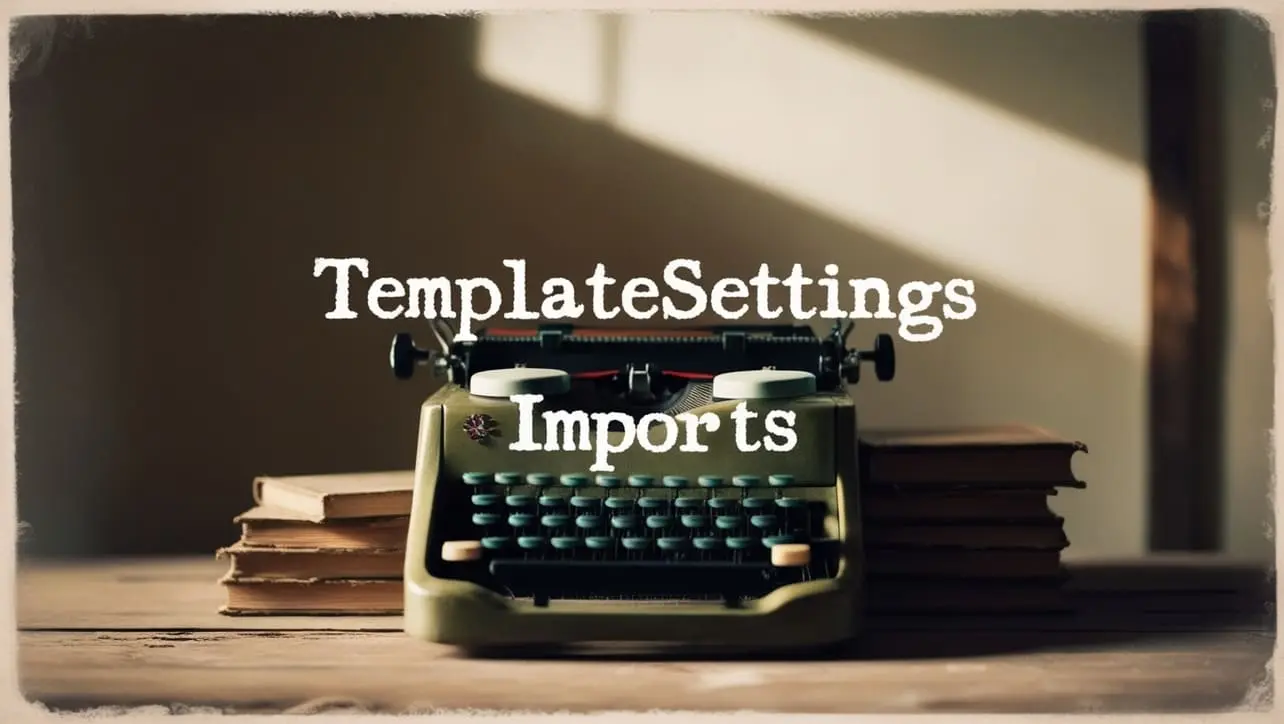
Lodash _.defer() Function Method
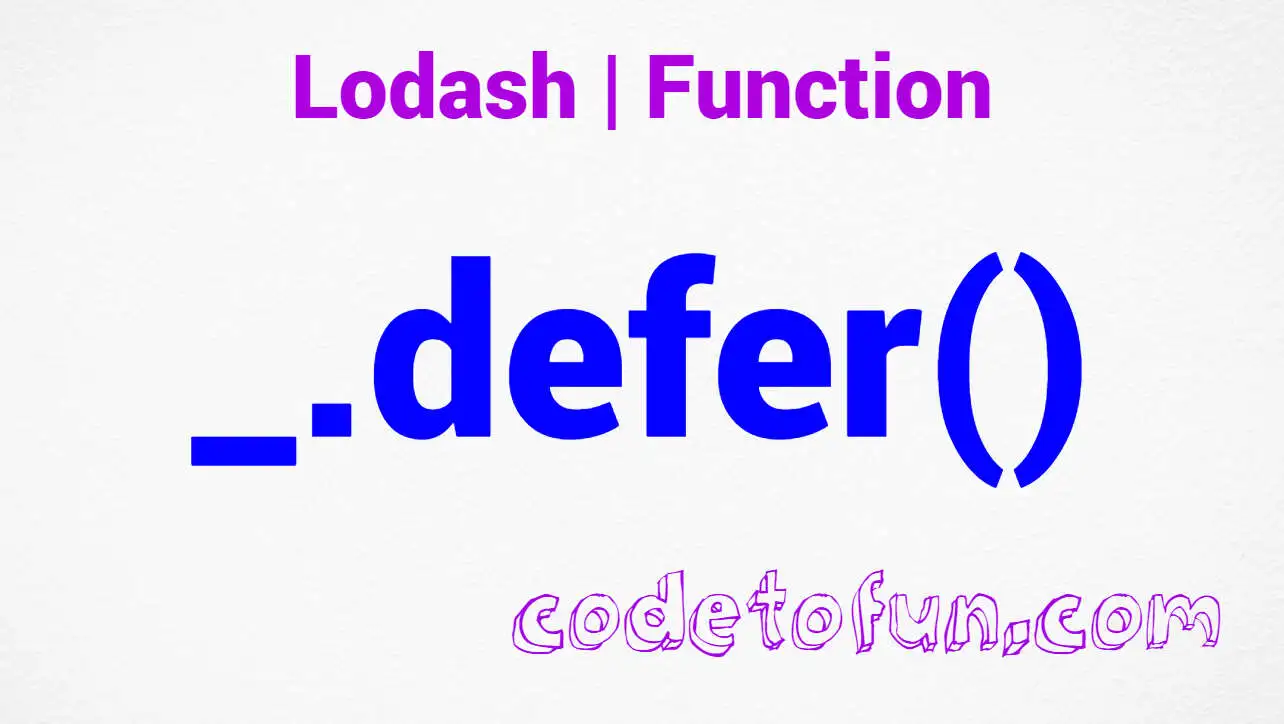
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript development, managing asynchronous operations is a common challenge. Lodash, a powerful utility library, comes to the rescue with functions like _.defer()
. This method allows developers to defer the execution of a function to the next event loop cycle, enhancing control over the timing of function invocation.
Understanding and leveraging _.defer()
can contribute to more efficient and responsive code.
🧠 Understanding _.defer() Method
The _.defer()
method in Lodash is designed to delay the execution of a function until the current call stack has cleared, effectively deferring its invocation. This is particularly useful for scenarios where you want a function to run asynchronously, avoiding blocking the main thread.
💡 Syntax
The syntax for the _.defer()
method is straightforward:
_.defer(func, [args])
- func: The function to defer.
- args (Optional): The arguments to pass to the deferred function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.defer()
method:
const _ = require('lodash');
function delayedFunction(message) {
console.log(`Delayed Execution: ${message}`);
}
_.defer(delayedFunction, 'Hello, Lodash!');
// Output: (output will be logged in the next event loop cycle)
In this example, the delayedFunction is deferred using _.defer()
, allowing the rest of the code to continue executing without waiting for the function to complete.
🏆 Best Practices
When working with the _.defer()
method, consider the following best practices:
Asynchronous Execution:
Use
_.defer()
when you want to ensure that a function runs asynchronously, especially when dealing with operations that could potentially block the main thread.example.jsCopiedconst asyncOperation = () => { // ... perform asynchronous task ... }; _.defer(asyncOperation); // Continue with other operations without waiting for asyncOperation to complete
Reducing Main Thread Blocking:
Employ
_.defer()
to move non-critical or time-consuming tasks to the background, reducing the risk of blocking the main thread and improving the overall responsiveness of your application.example.jsCopiedconst timeConsumingTask = () => { // ... perform a time-consuming task ... }; // Execute the time-consuming task in the background _.defer(timeConsumingTask); // Continue with other operations without waiting for timeConsumingTask to complete
Function with Arguments:
When deferring a function that requires arguments, pass the arguments as the second parameter to
_.defer()
.example.jsCopiedfunction deferredFunctionWithArgs(arg1, arg2) { console.log(`Deferred Execution with Arguments: ${arg1}, ${arg2}`); } _.defer(deferredFunctionWithArgs, 'arg1Value', 'arg2Value'); // Output: (output will be logged in the next event loop cycle)
📚 Use Cases
UI Rendering:
Deferred execution is beneficial when updating the UI after a user action. Use
_.defer()
to ensure that the UI updates occur asynchronously, preventing delays in responsiveness.example.jsCopiedconst updateUserInterface = () => { // ... update UI elements ... }; // Trigger UI update after user action _.defer(updateUserInterface); // Continue with other user interactions without waiting for the UI update to complete
Batch Processing:
When dealing with batch processing of data,
_.defer()
can be employed to process chunks of data in the background, avoiding interruptions to the main thread.example.jsCopiedconst batchProcessData = (dataChunk) => { // ... process a chunk of data ... }; const dataToProcess = /* ... fetch data ... */; // Process data in the background in batches dataToProcess.forEach(chunk => _.defer(batchProcessData, chunk)); // Continue with other operations without waiting for data processing to complete
Timed Execution:
Use
_.defer()
in combination with timers to create delays in function execution, achieving timed asynchronous behavior.example.jsCopiedconst delayedLog = () => { console.log('Logged after a delay'); }; // Log after a delay of 1 second setTimeout(() => { _.defer(delayedLog); }, 1000);
🎉 Conclusion
The _.defer()
method in Lodash provides a valuable tool for managing asynchronous operations in JavaScript. By deferring the execution of functions, you can optimize the responsiveness of your code, ensuring a smooth user experience and efficient handling of background tasks.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.defer()
method in your Lodash projects.
👨💻 Join our Community:
Author
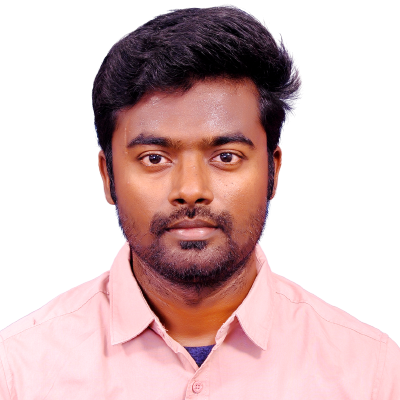
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
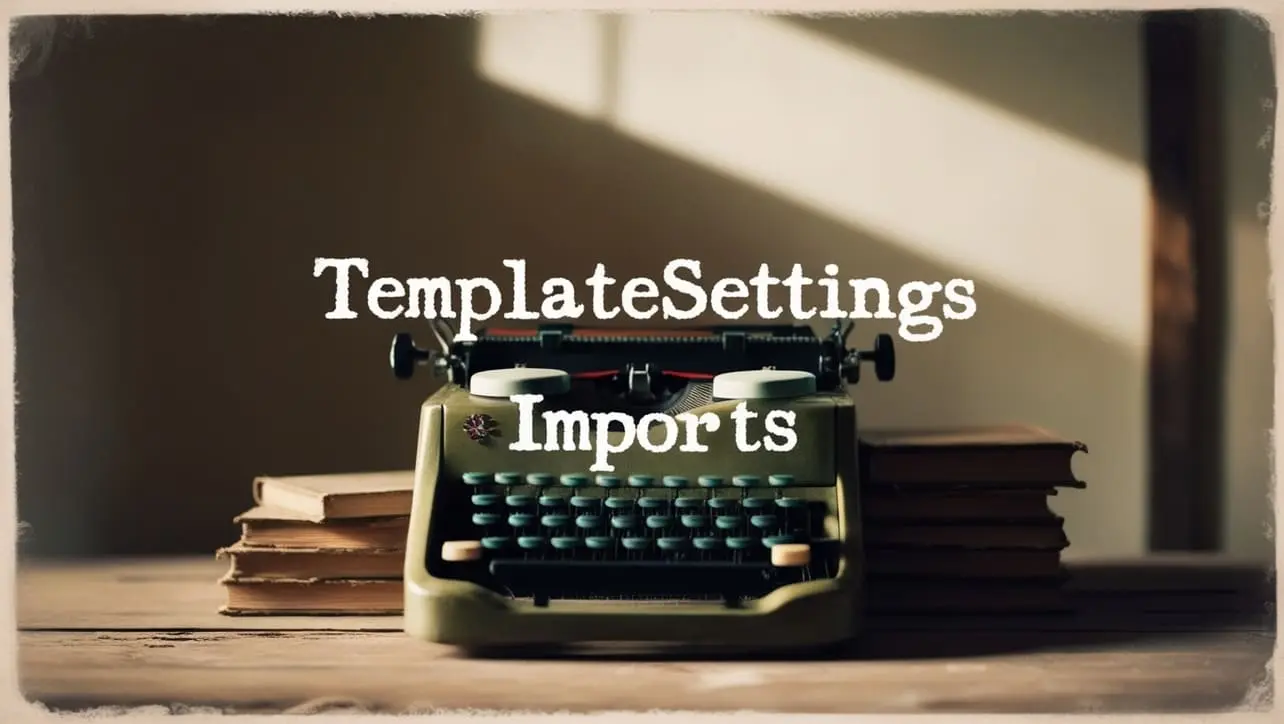
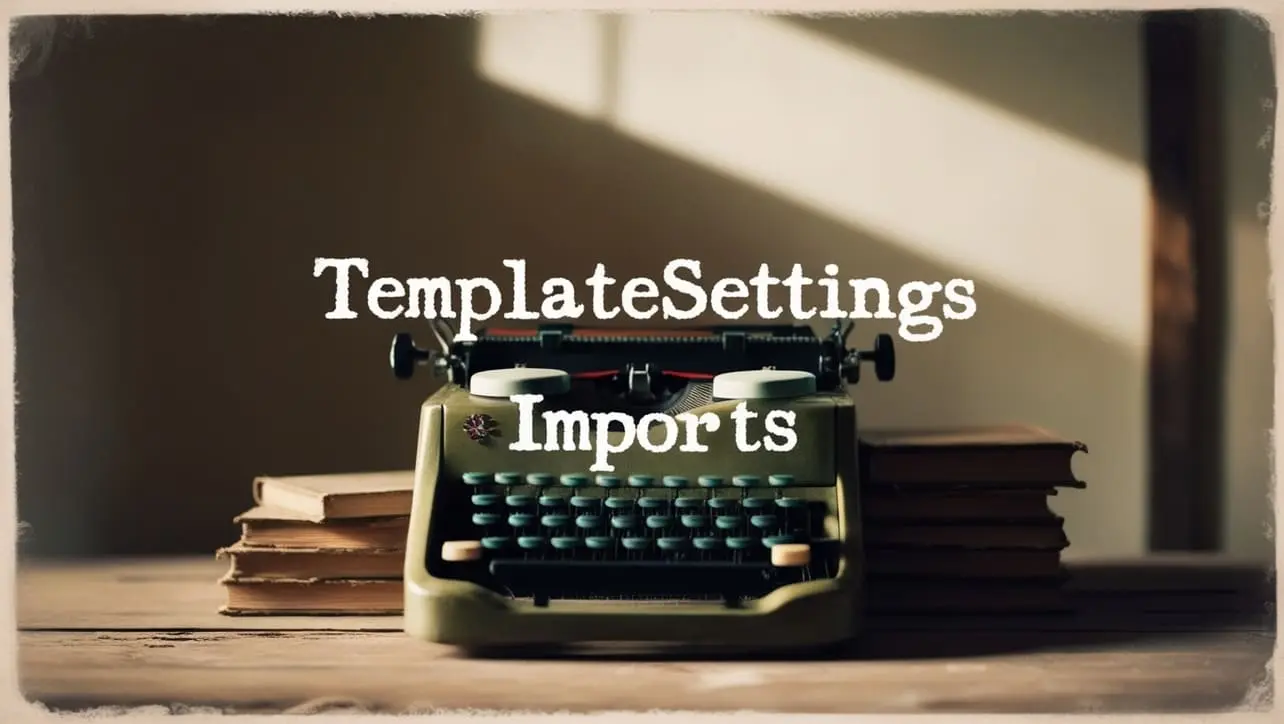
Lodash _.templateSettings.imports Property
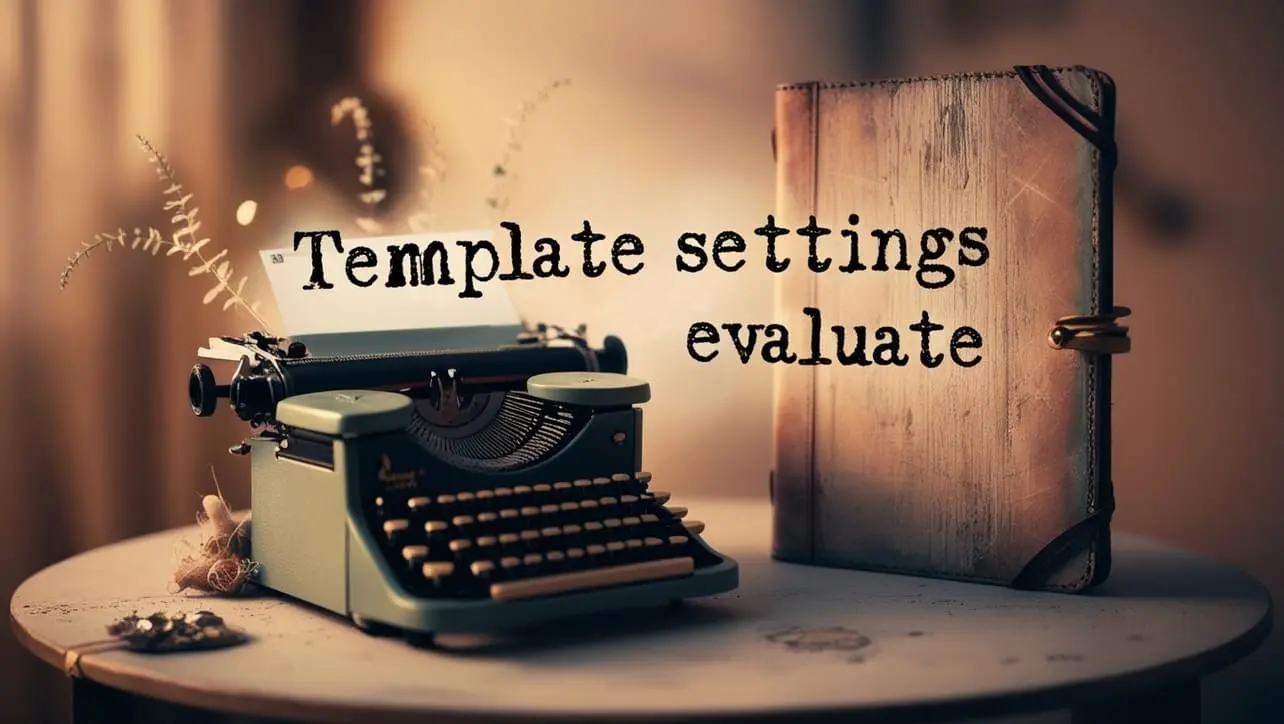
Lodash _.templateSettings.evaluate Property
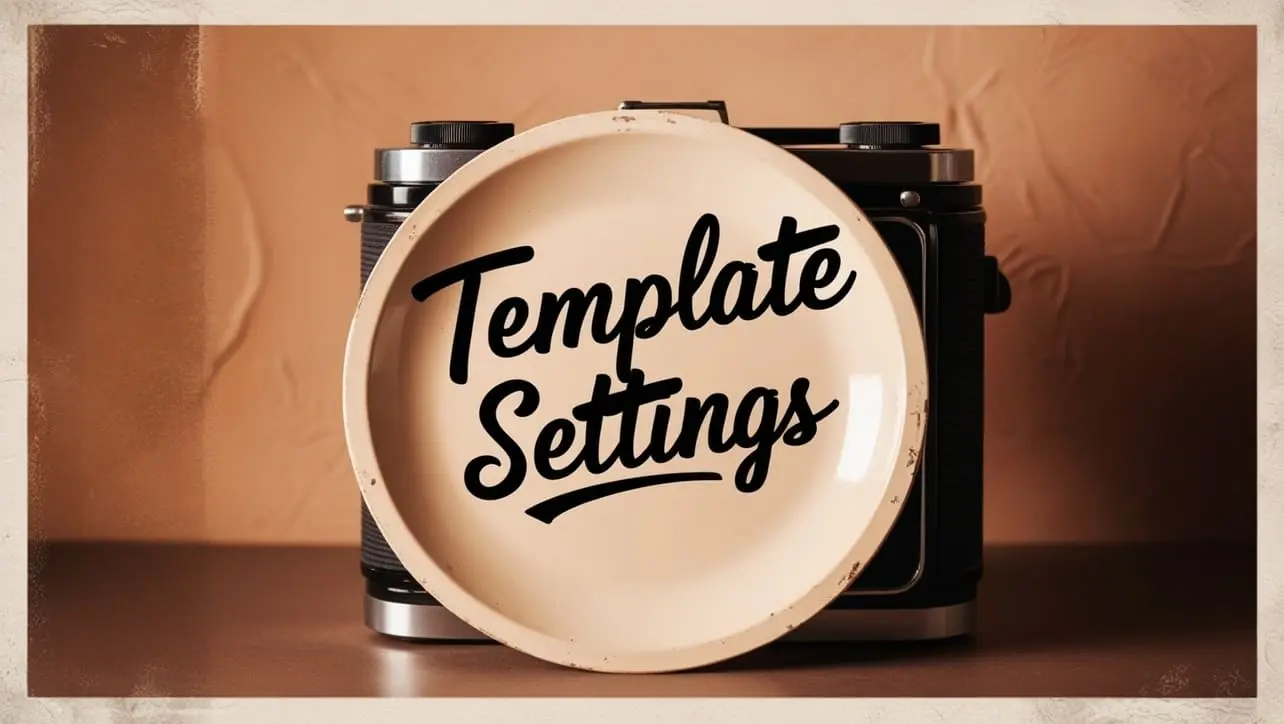
Lodash _.templateSettings Property
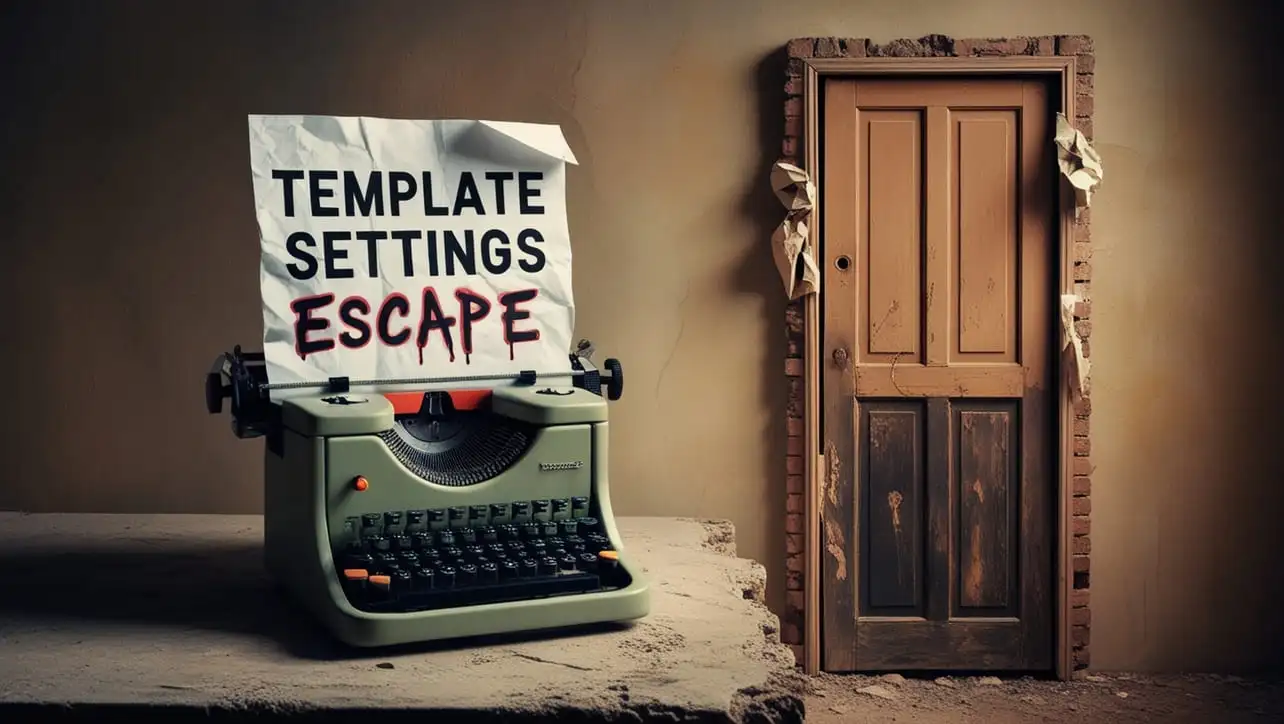
Lodash _.templateSettings.escape Property
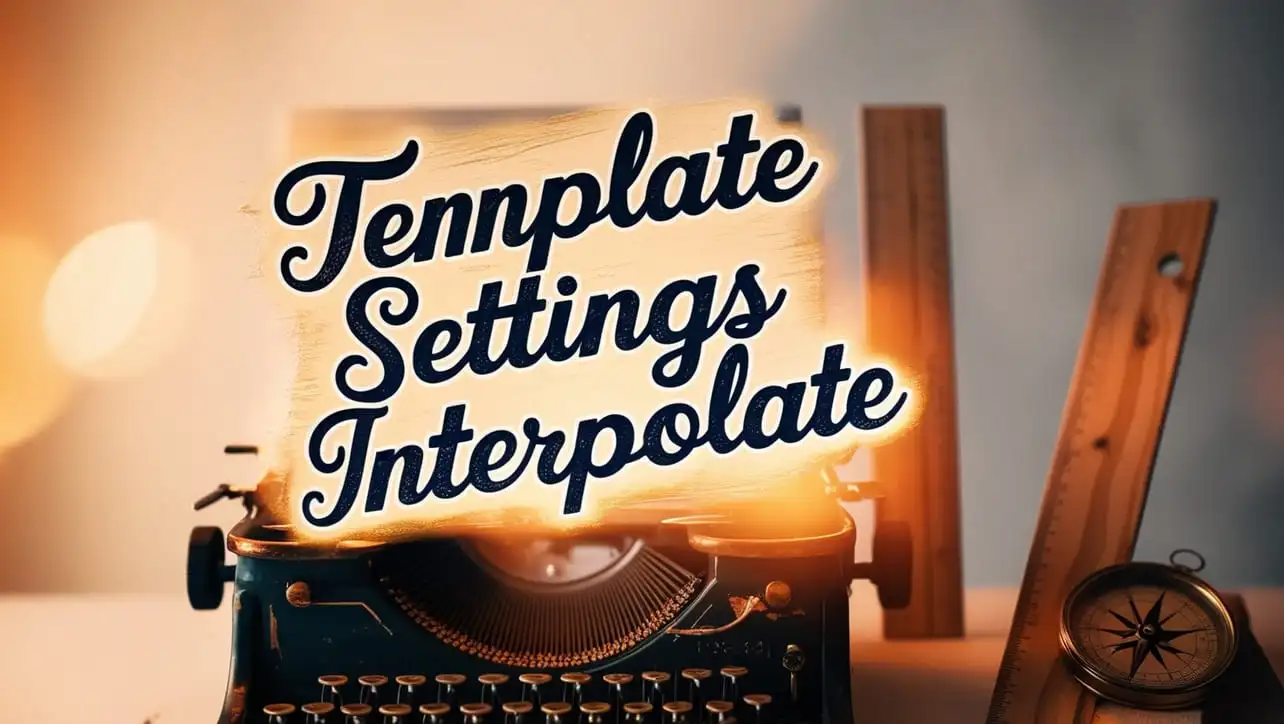
Lodash _.templateSettings.interpolate Property
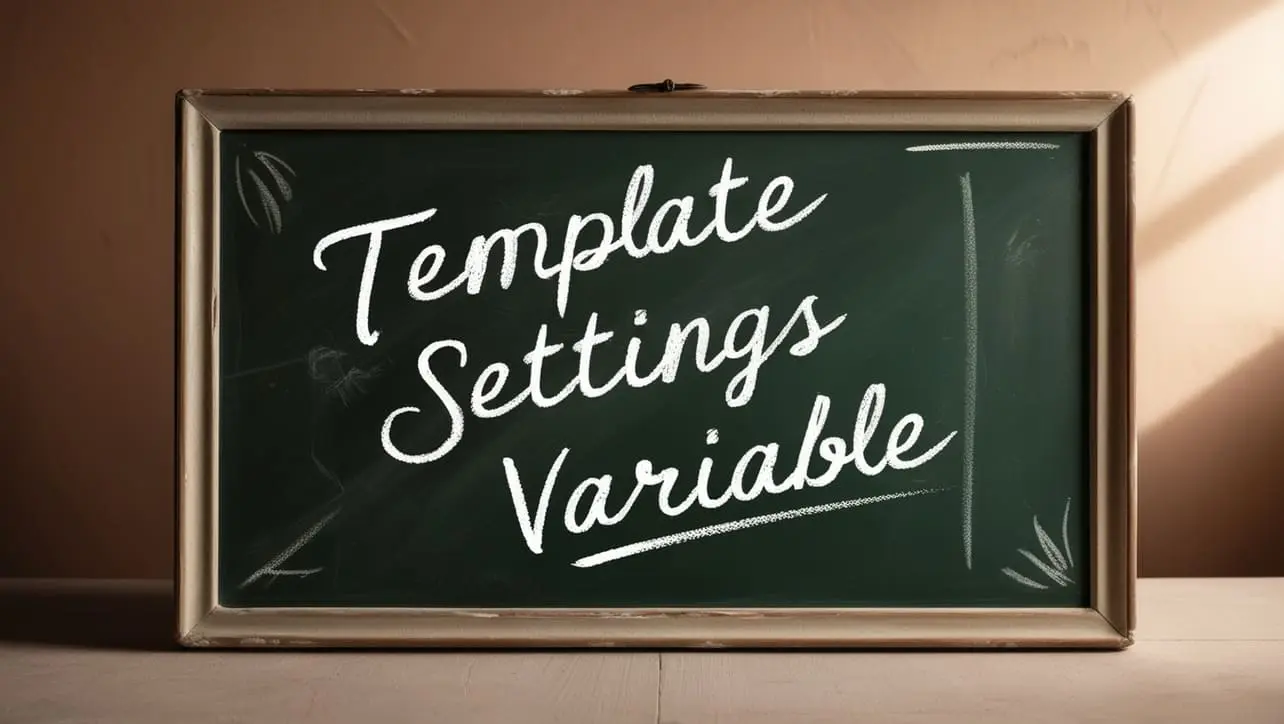
If you have any doubts regarding this article (Lodash _.defer() Function Method), please comment here. I will help you immediately.