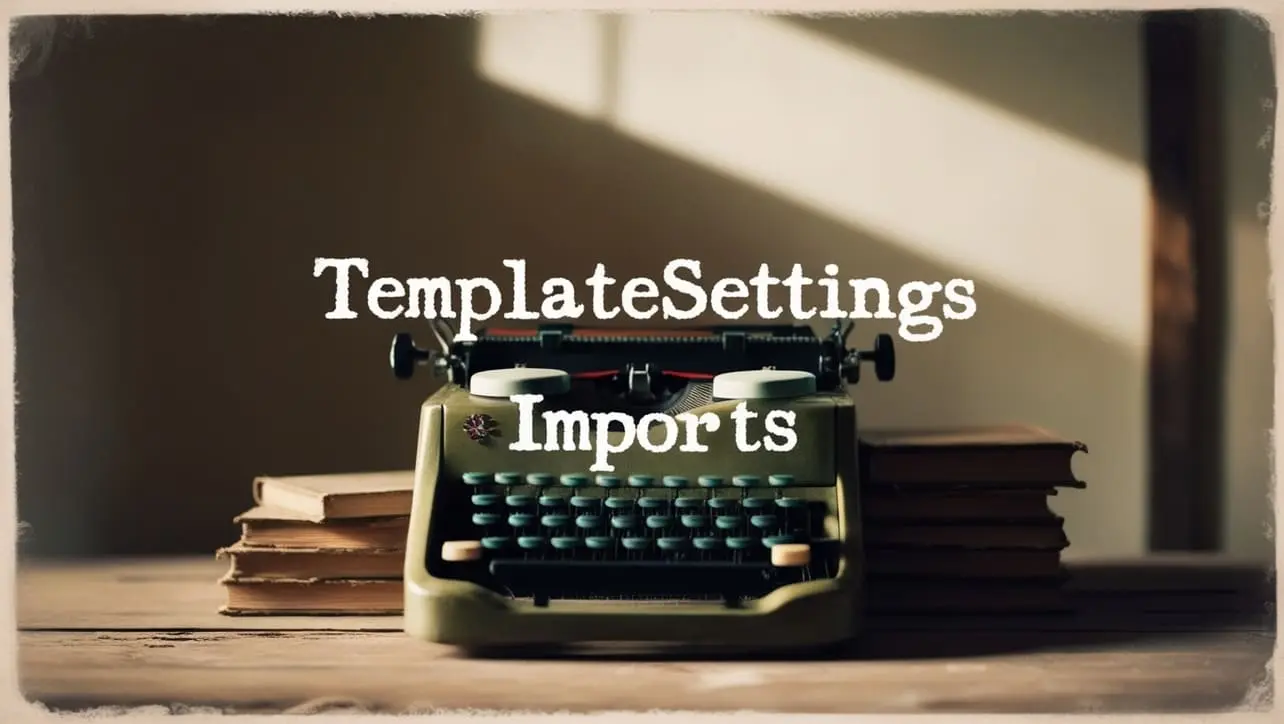
Lodash _.ary() Function Method
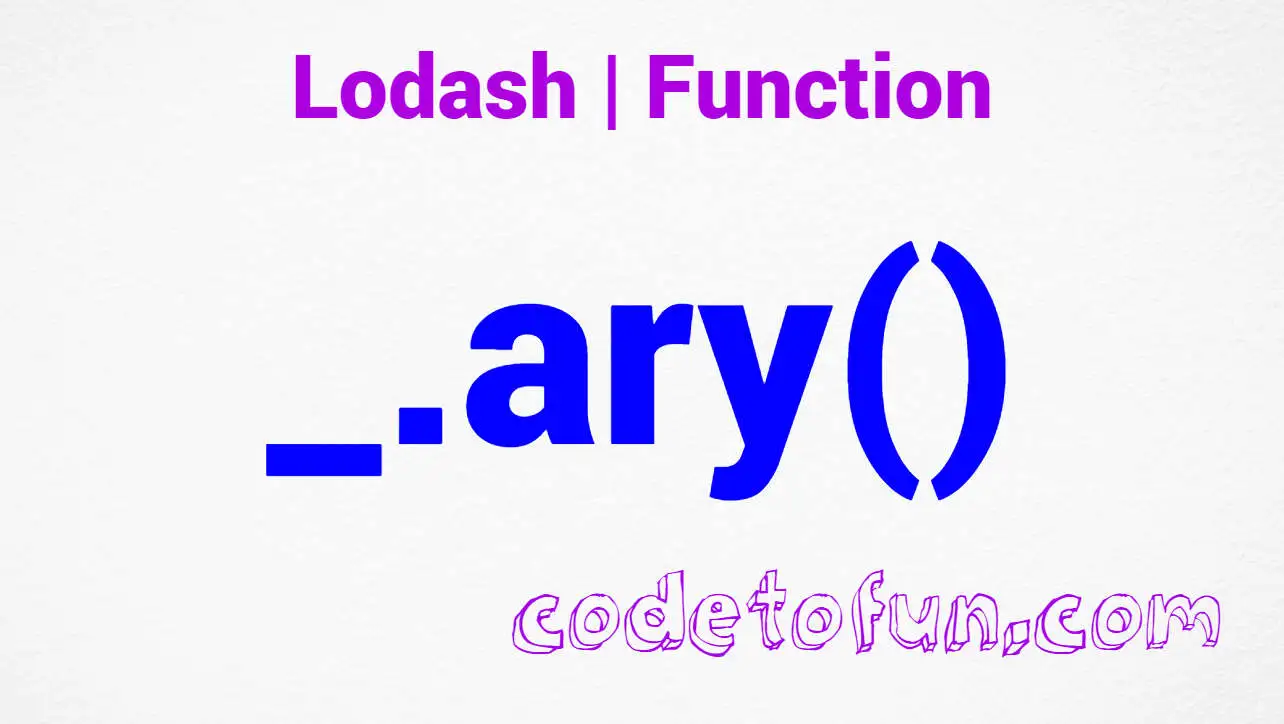
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, functional programming plays a vital role, and Lodash provides a rich set of tools to enhance functional programming practices. Among these tools is the _.ary()
method, a versatile function modifier that allows developers to control the arity (number of expected arguments) of a given function.
This method empowers developers to tailor functions to their specific needs, promoting flexibility and clarity in code.
🧠 Understanding _.ary() Method
The _.ary()
method in Lodash is designed to create a function with a specified arity, ensuring that it only accepts the desired number of arguments. This can be particularly useful when working with functions that may receive more arguments than necessary, providing a concise way to manage input parameters.
💡 Syntax
The syntax for the _.ary()
method is straightforward:
_.ary(func, [n=func.length])
- func: The target function.
- n: The desired arity of the new function (default is func.length).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.ary()
method:
const _ = require('lodash');
// Original function with three parameters
const originalFunction = (a, b, c) => a + b + c;
// Create a new function with only two parameters
const modifiedFunction = _.ary(originalFunction, 2);
console.log(modifiedFunction(1, 2, 3));
// Output: 3 (ignores the third argument)
In this example, the modifiedFunction is created from originalFunction with an arity of 2, allowing it to ignore the third argument.
🏆 Best Practices
When working with the _.ary()
method, consider the following best practices:
Controlling Function Arity:
Use
_.ary()
when you need to control the number of arguments a function accepts. This can be especially helpful when working with higher-order functions or callback functions where the arity might vary.example.jsCopiedconst originalCallback = (a, b, c) => /* ... */; const controlledCallback = _.ary(originalCallback, 2); // Use controlledCallback in a higher-order function [1, 2, 3].map(controlledCallback);
Default to Function's Length:
When not specifying the arity (n),
_.ary()
defaults to the length of the original function. This provides a convenient way to create modified functions that maintain the same arity.example.jsCopiedconst originalFunc = (a, b, c, d) => /* ... */; const defaultAryFunc = _.ary(originalFunc); // defaultAryFunc has the same arity as originalFunc
Compose with Other Lodash Methods:
Combine
_.ary()
with other Lodash methods, such as _.map() or _.filter(), to create powerful compositions tailored to your specific requirements.example.jsCopiedconst square = n => n * n; const squaredNumbers = _.map([1, 2, 3], _.ary(square, 1)); console.log(squaredNumbers); // Output: [1, 4, 9]
📚 Use Cases
Callbacks in Array Methods:
Use
_.ary()
to control callbacks in array methods like _.map() or _.filter(), ensuring that they receive only the necessary arguments.example.jsCopiedconst array = ['apple', 'banana', 'cherry']; // Use _.ary() to create a callback that ignores the index const logWithoutIndex = _.ary(console.log, 1); // Log each item without the index _.map(array, logWithoutIndex);
Partial Application:
Leverage
_.ary()
in partial application scenarios, where you want to fix a specific number of arguments in a function.example.jsCopiedconst multiply = (a, b, c) => a * b * c; // Create a partially applied function with only two arguments const partiallyApplied = _.ary(multiply, 2); console.log(partiallyApplied(2, 3)); // Output: 12 (ignores the third argument)
Adapting Functions in Functional Programming:
In functional programming, use
_.ary()
to adapt functions to specific contexts, ensuring they conform to expected argument counts.example.jsCopiedconst unaryFunction = n => n * 2; const adaptedFunction = _.ary(unaryFunction, 1); // Use adaptedFunction in a context that expects a unary function
🎉 Conclusion
The _.ary()
method in Lodash provides a valuable tool for controlling the arity of functions in JavaScript. Whether you're working with array methods, applying partial application, or adapting functions in a functional programming paradigm, _.ary()
offers a concise and flexible solution to tailor functions to your specific needs.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.ary()
method in your Lodash projects.
👨💻 Join our Community:
Author
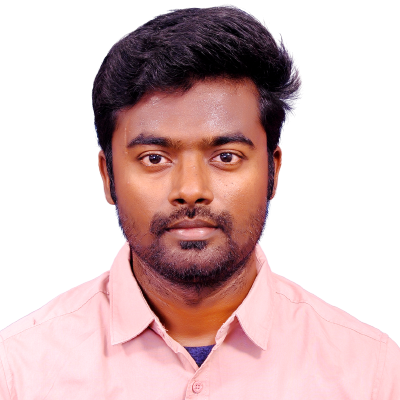
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
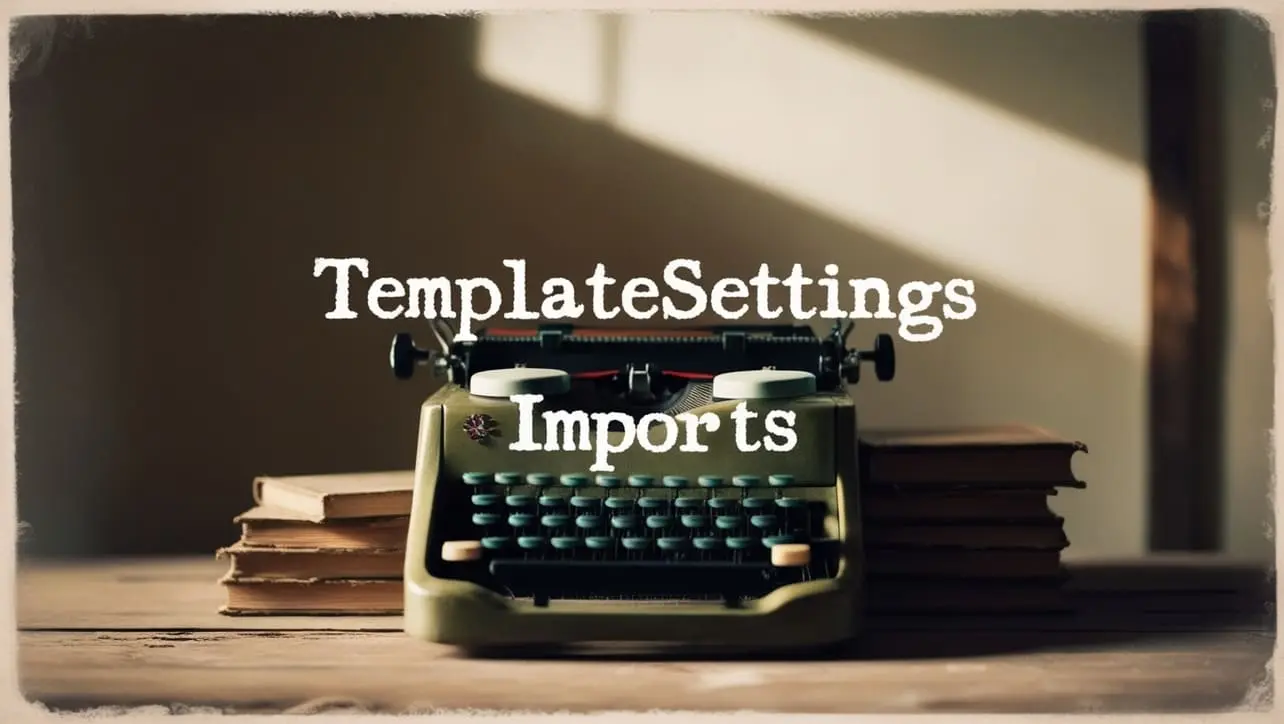
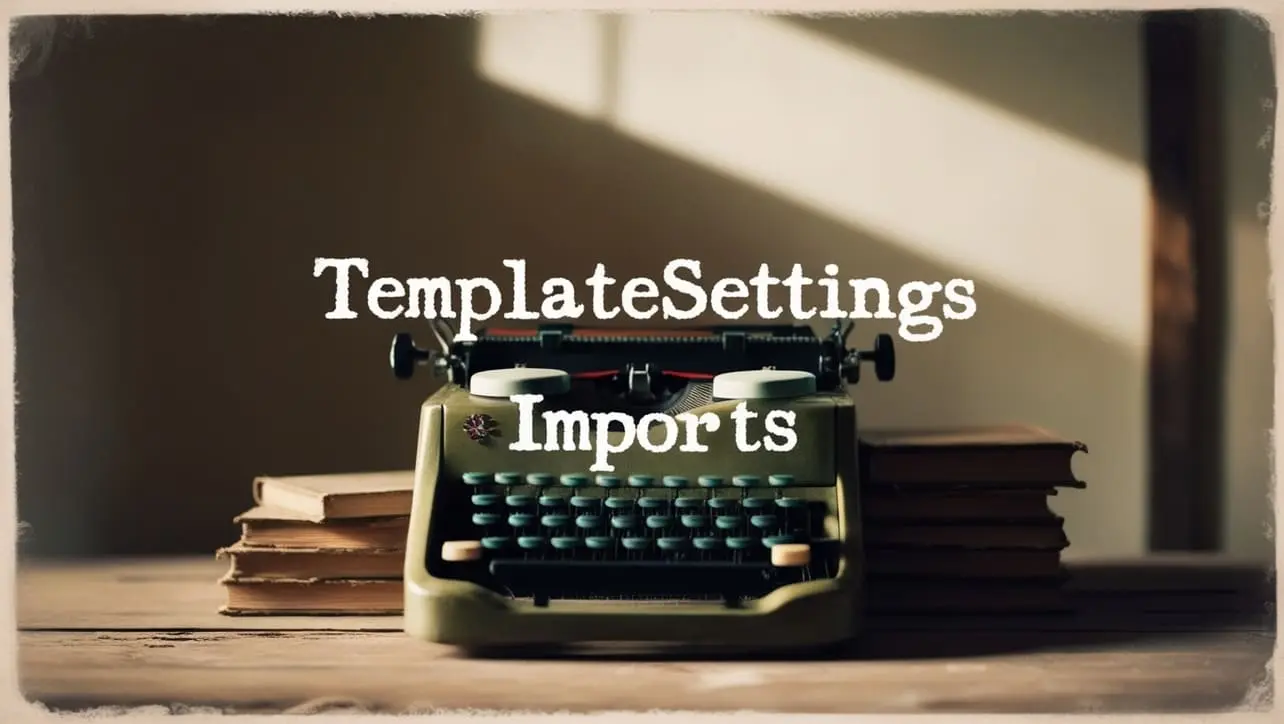
Lodash _.templateSettings.imports Property
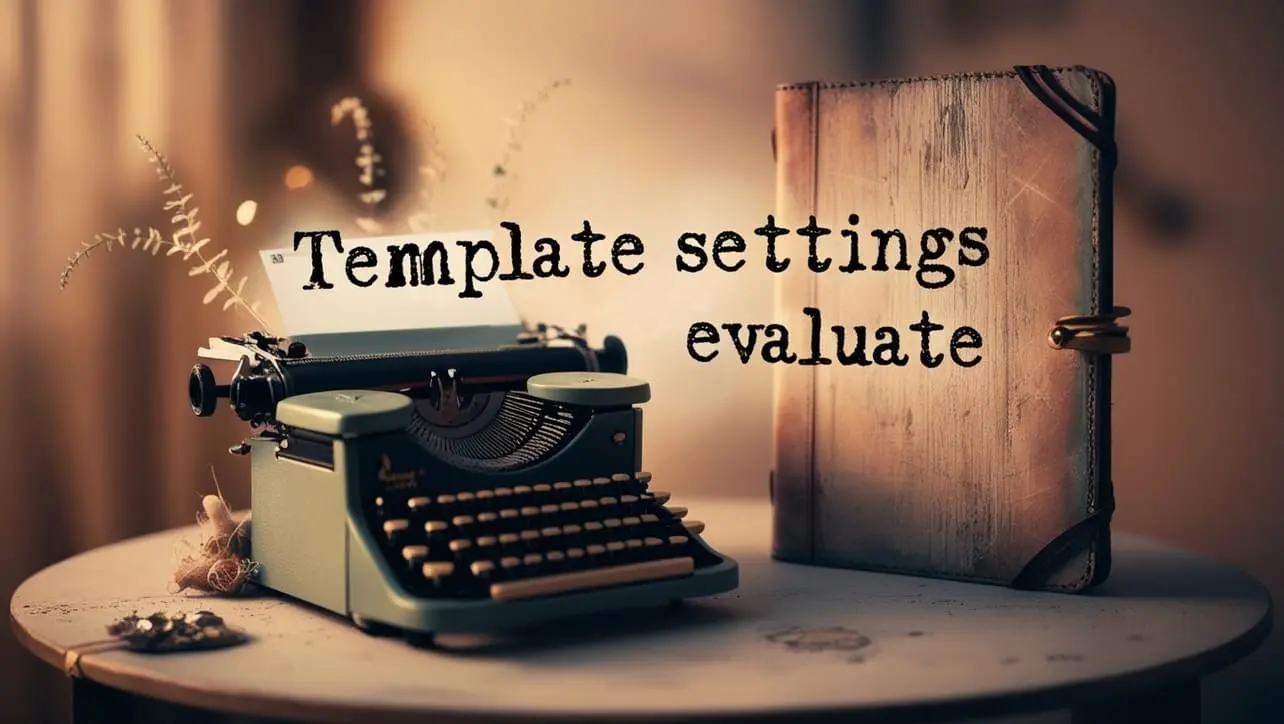
Lodash _.templateSettings.evaluate Property
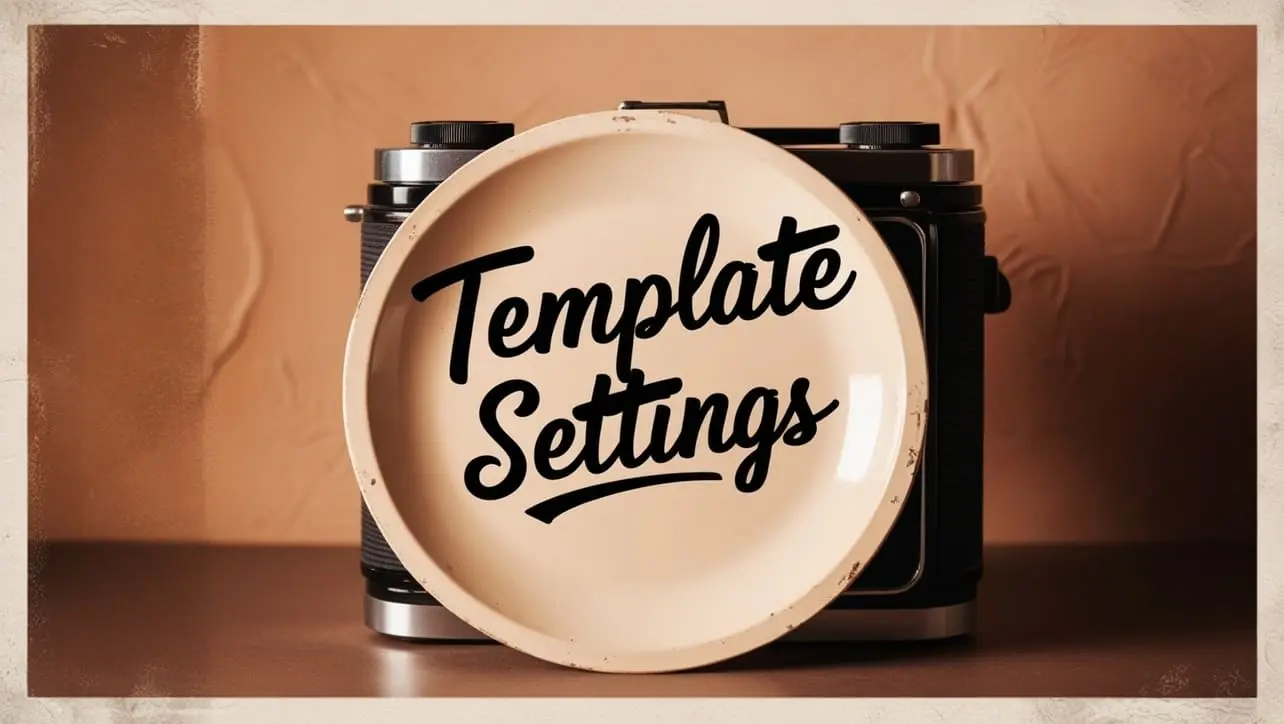
Lodash _.templateSettings Property
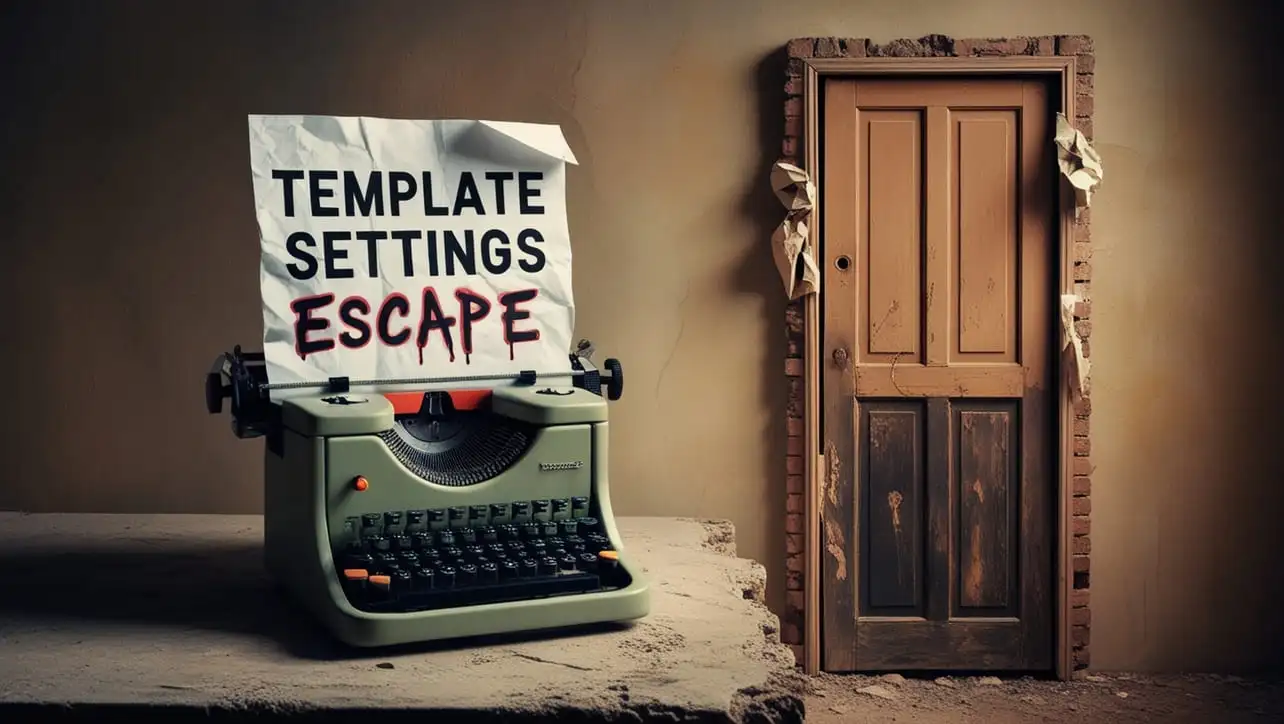
Lodash _.templateSettings.escape Property
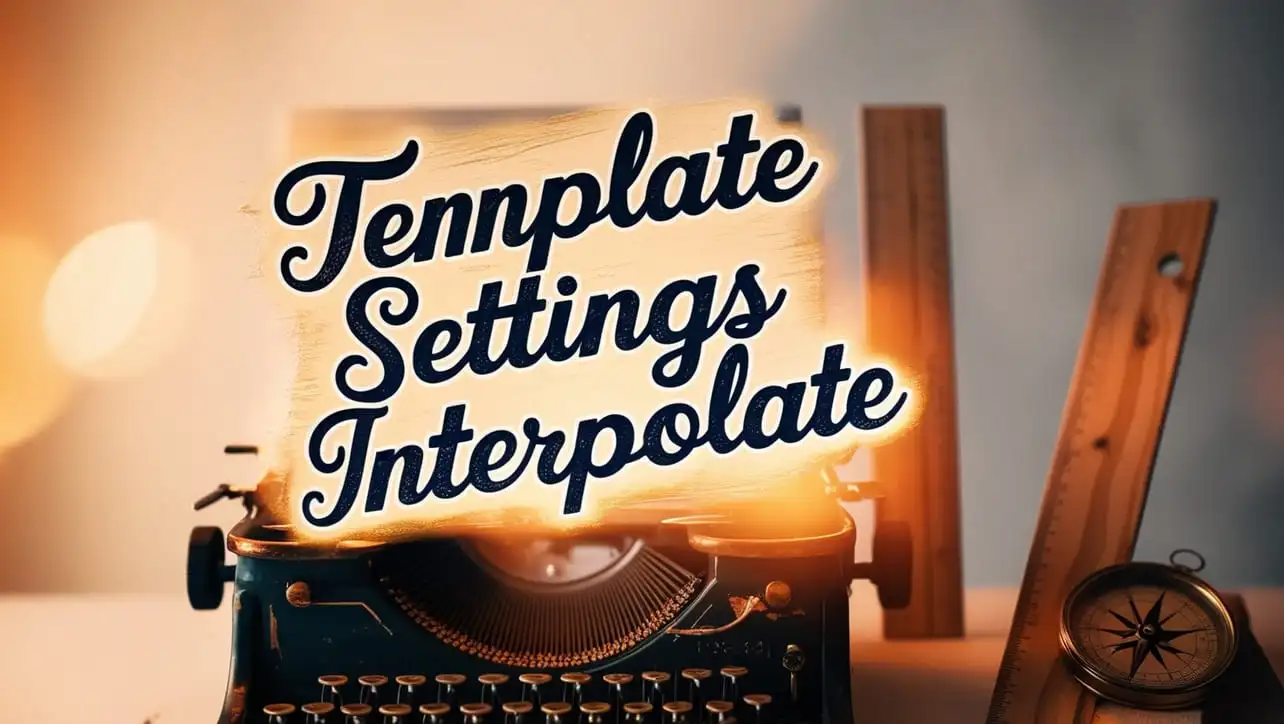
Lodash _.templateSettings.interpolate Property
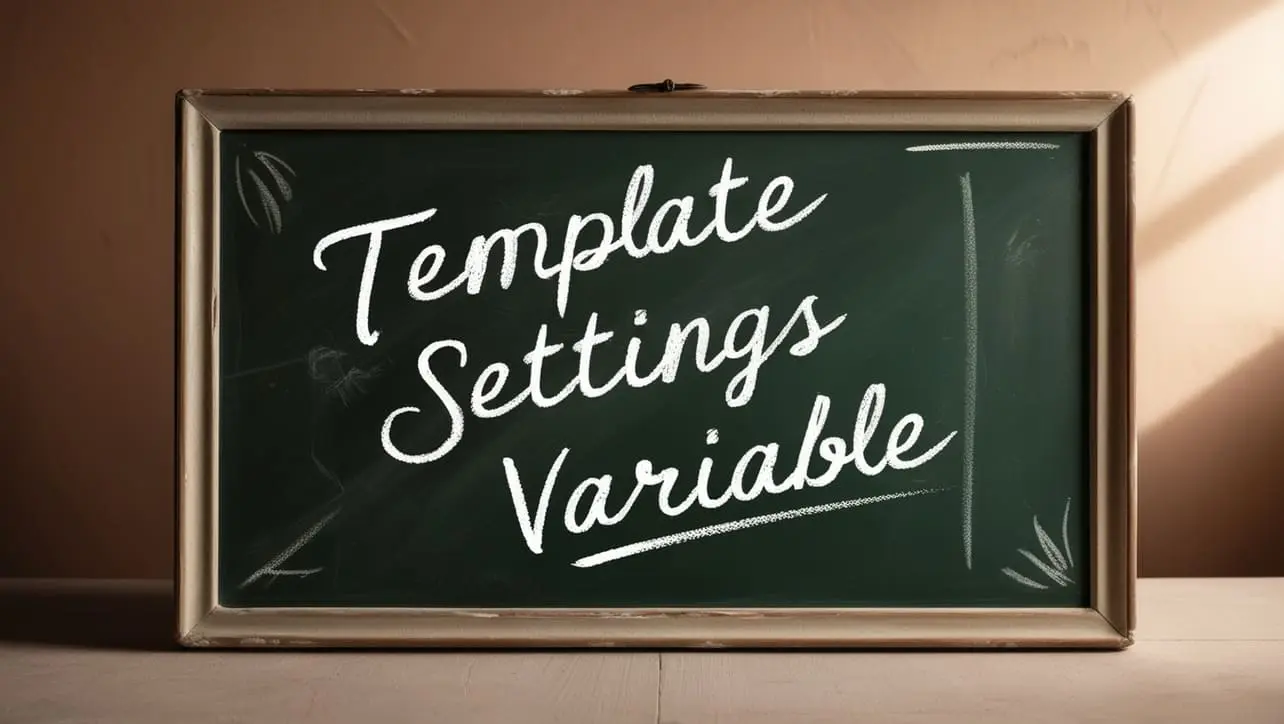
If you have any doubts regarding this article (Lodash _.ary() Function Method), please comment here. I will help you immediately.