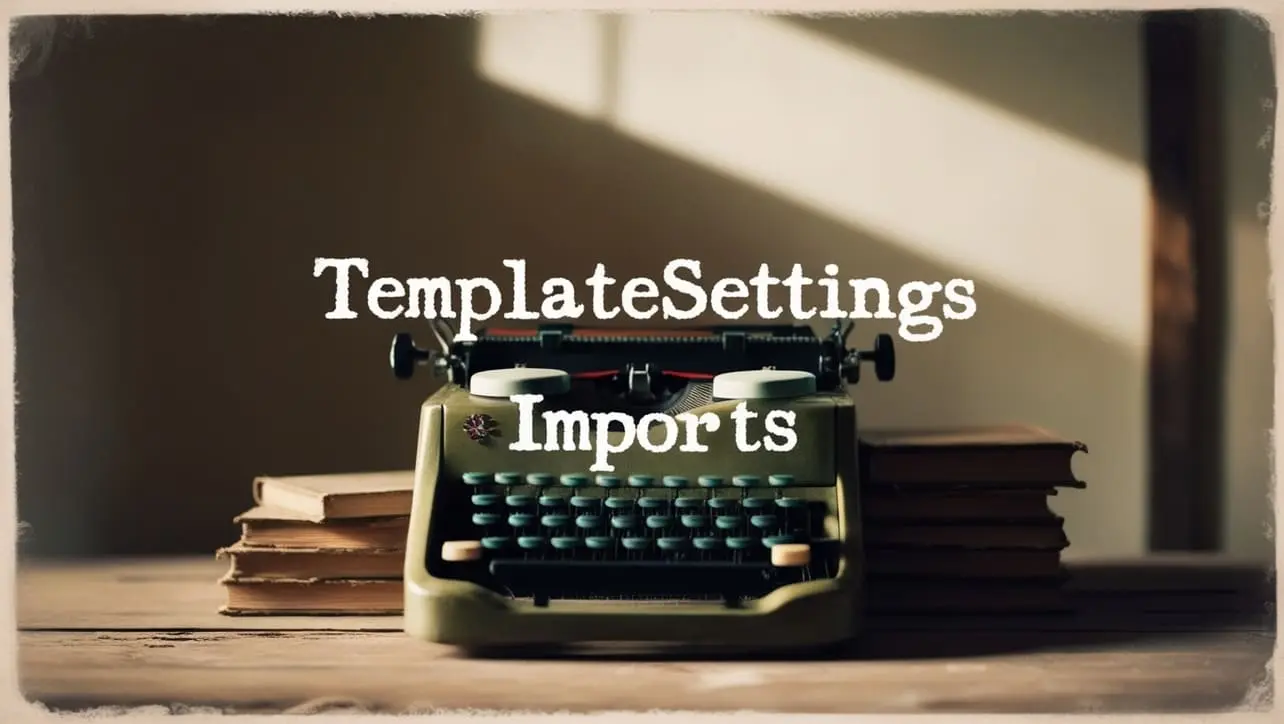
Lodash _.now() Date Method
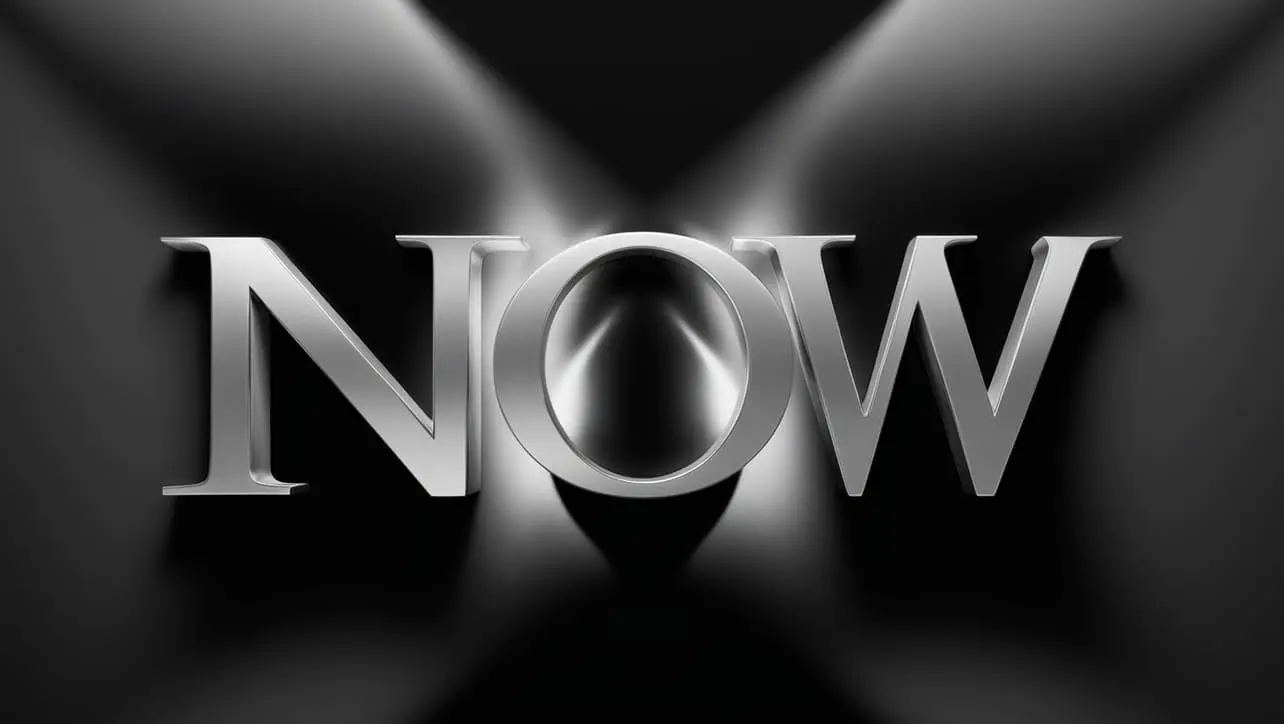
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript, dealing with dates and time is a common requirement for many developers. Lodash, a versatile utility library, offers a solution to simplify the process with the _.now()
method.
This method provides a quick and efficient way to obtain the current timestamp, enabling developers to handle time-related tasks with ease.
🧠 Understanding _.now() Method
The _.now()
method in Lodash is a straightforward utility that returns the current timestamp in milliseconds. It's a lightweight alternative to the native Date.now() method, offering consistent performance across different JavaScript environments.
💡 Syntax
The syntax for the _.now()
method is straightforward:
_.now()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.now()
method:
const _ = require('lodash');
const currentTimestamp = _.now();
console.log(currentTimestamp);
// Output: Current timestamp in milliseconds
In this example, _.now()
is used to obtain the current timestamp, which can be useful in various scenarios, such as measuring performance or tracking time-based events.
🏆 Best Practices
When working with the _.now()
method, consider the following best practices:
Consistency with Native Method:
While
_.now()
is a convenient utility, consider using the native Date.now() method for consistency if your project doesn't rely heavily on Lodash. Both methods serve the same purpose of providing the current timestamp.example.jsCopiedconst nativeTimestamp = Date.now(); console.log(nativeTimestamp);
Time Measurement:
Use
_.now()
for measuring the execution time of code blocks. Capture the timestamp before and after the code execution to calculate the elapsed time.example.jsCopiedconst startTime = _.now(); // ... Code to measure ... const endTime = _.now(); const elapsedTime = endTime - startTime; console.log(`Elapsed Time: ${elapsedTime} milliseconds`);
📚 Use Cases
Benchmarking:
_.now()
is valuable for benchmarking and performance measurement. Use it to capture timestamps before and after the execution of a specific task to assess its efficiency.example.jsCopiedconst startBenchmark = _.now(); // ... Code to benchmark ... const endBenchmark = _.now(); const benchmarkDuration = endBenchmark - startBenchmark; console.log(`Benchmark Duration: ${benchmarkDuration} milliseconds`);
Event Timestamps:
When working with events or logs,
_.now()
can be used to timestamp events for tracking and analysis purposes.example.jsCopiedconst logEvent = (message) => { const timestamp = _.now(); console.log(`[${timestamp}] ${message}`); }; logEvent('User logged in');
Timeout Management:
For precise timeout management,
_.now()
can help calculate the elapsed time since a specific point, aiding in accurate timeout adjustments.example.jsCopiedconst startTime = _.now(); // ... Code execution ... const elapsedTime = _.now() - startTime; // Adjust timeout based on elapsedTime const adjustedTimeout = 1000 - elapsedTime; setTimeout(() => { // ... Code to execute after adjusted timeout ... }, adjustedTimeout);
🎉 Conclusion
The _.now()
method in Lodash is a handy utility for obtaining the current timestamp in milliseconds. Whether you're benchmarking code performance, tracking events, or managing timeouts, _.now()
provides a concise and efficient solution for handling time-related tasks in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.now()
method in your Lodash projects.
👨💻 Join our Community:
Author
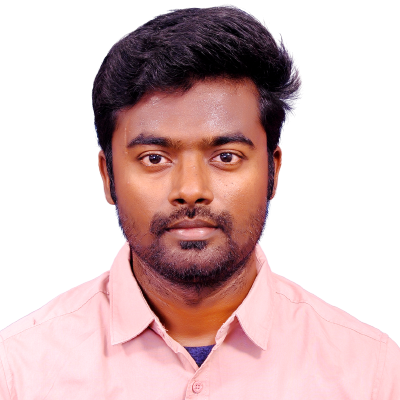
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
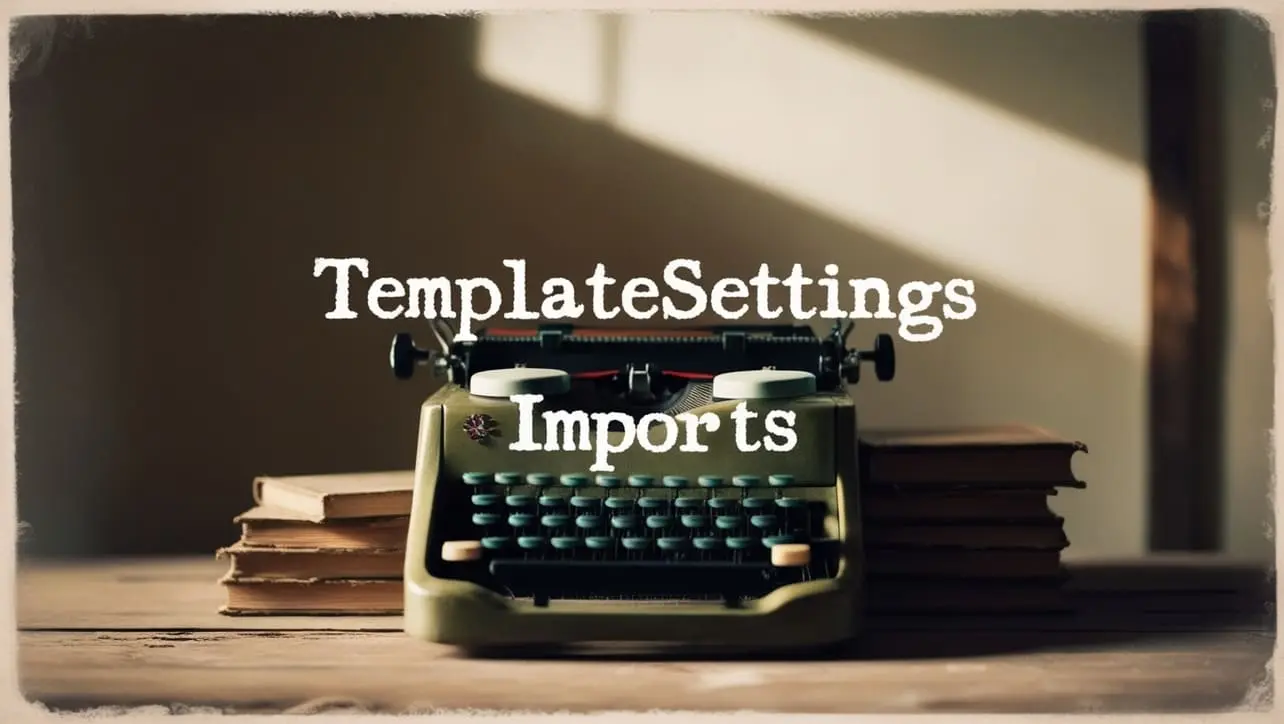
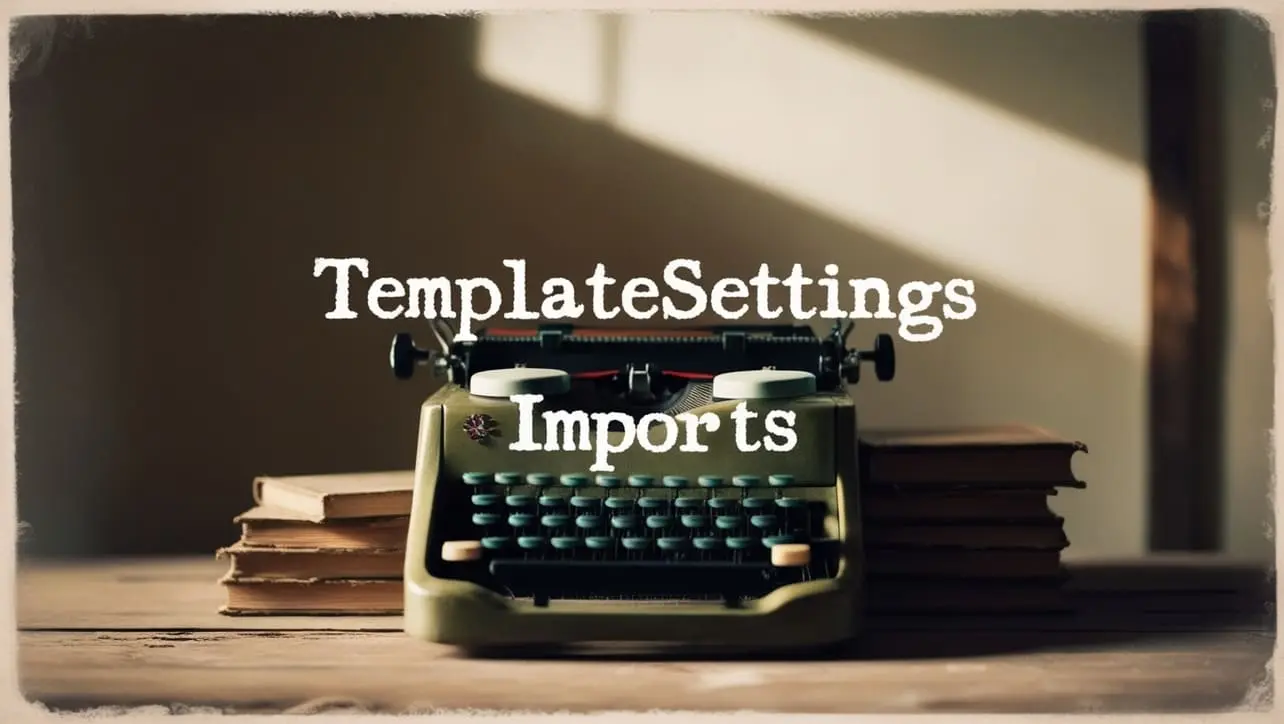
Lodash _.templateSettings.imports Property
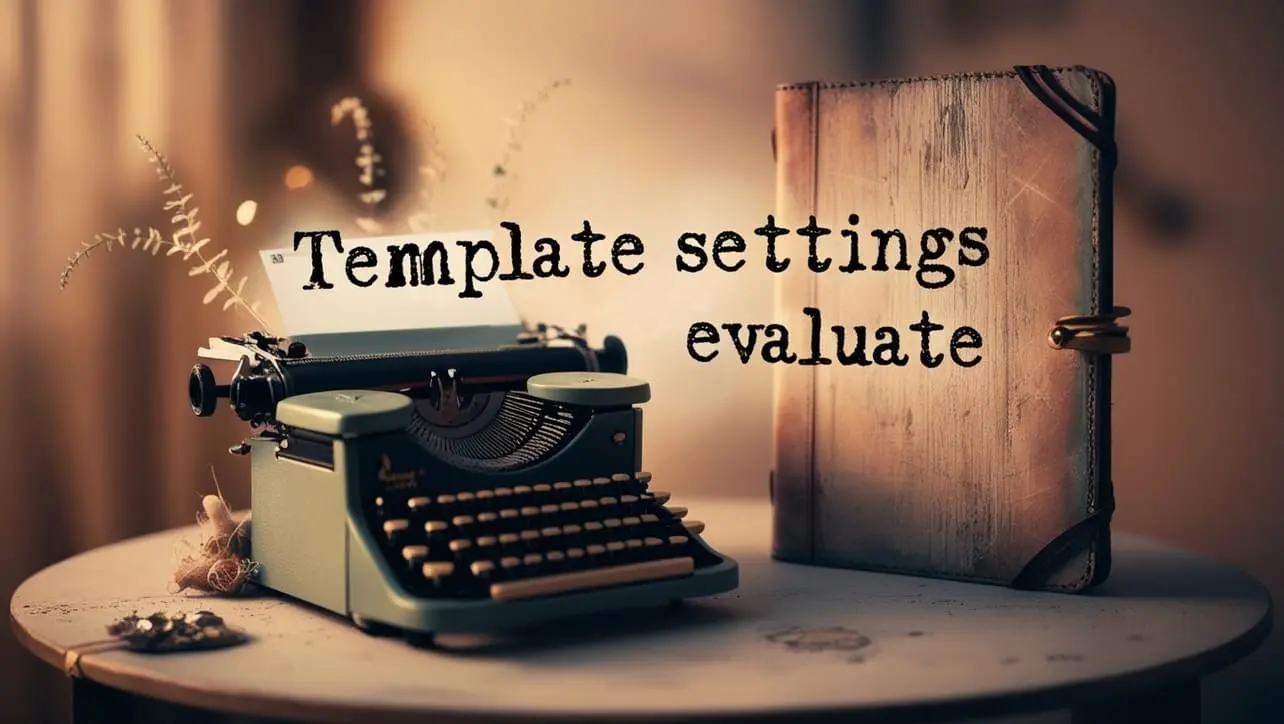
Lodash _.templateSettings.evaluate Property
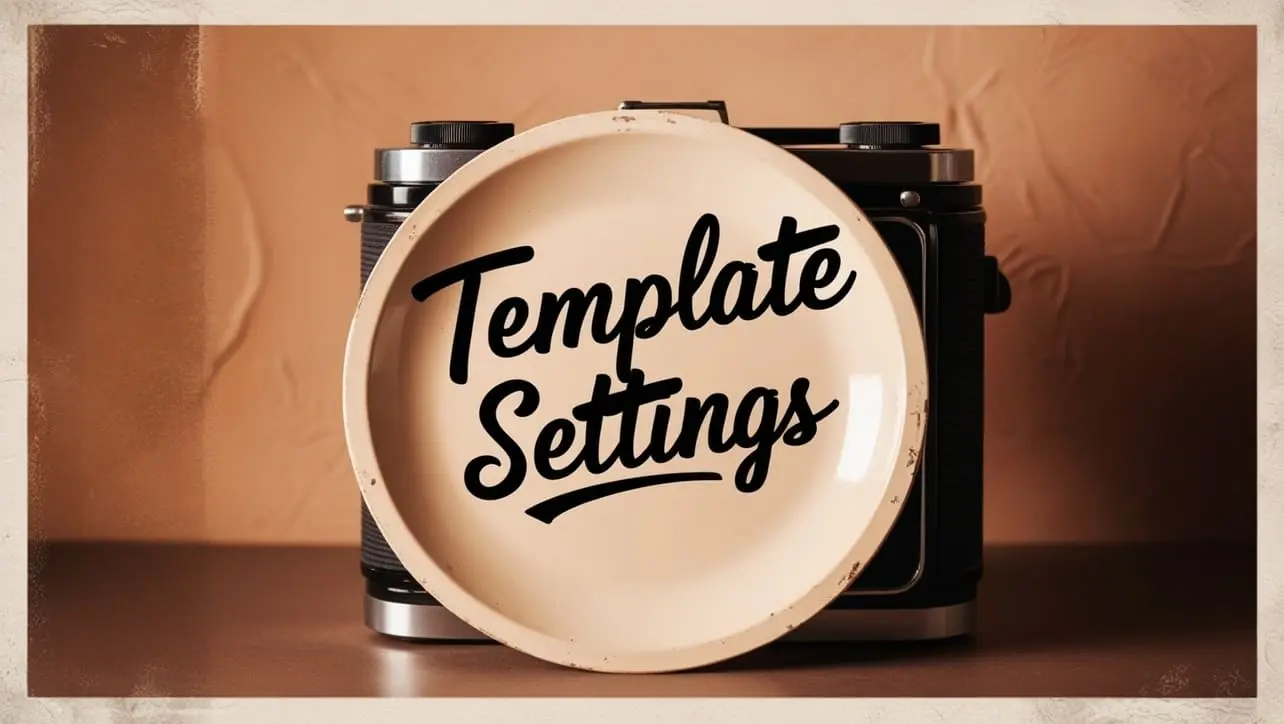
Lodash _.templateSettings Property
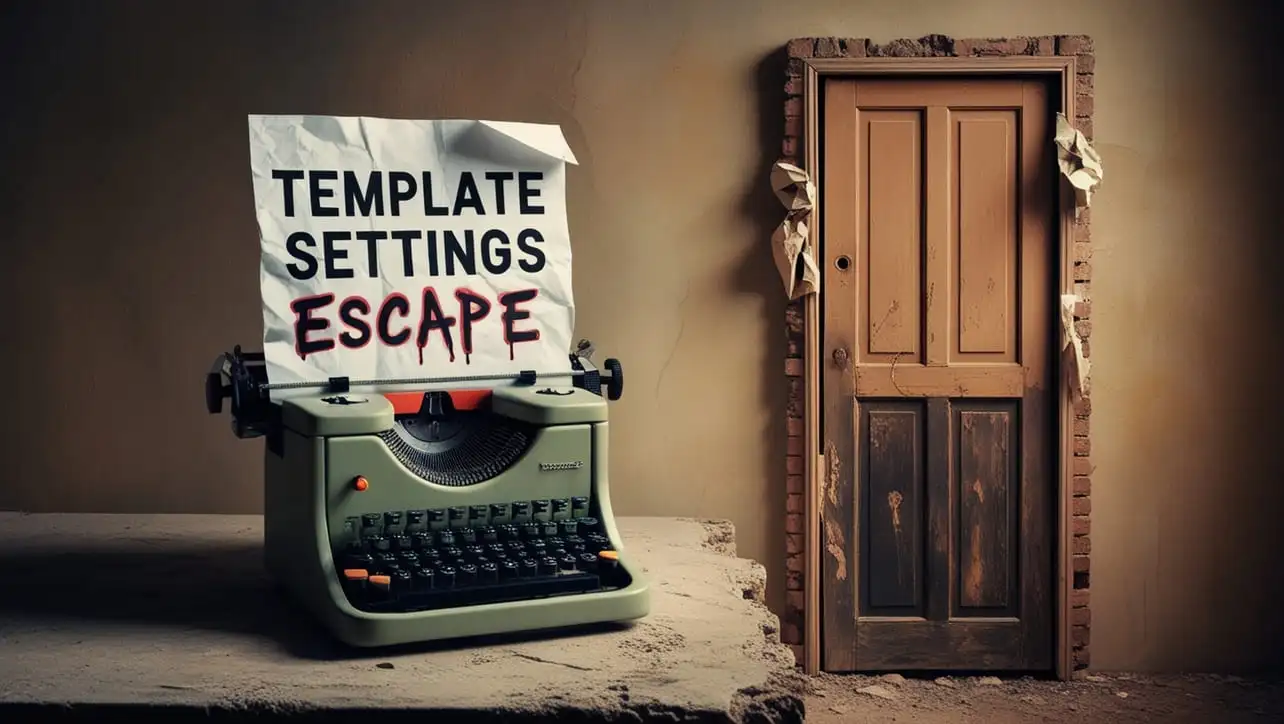
Lodash _.templateSettings.escape Property
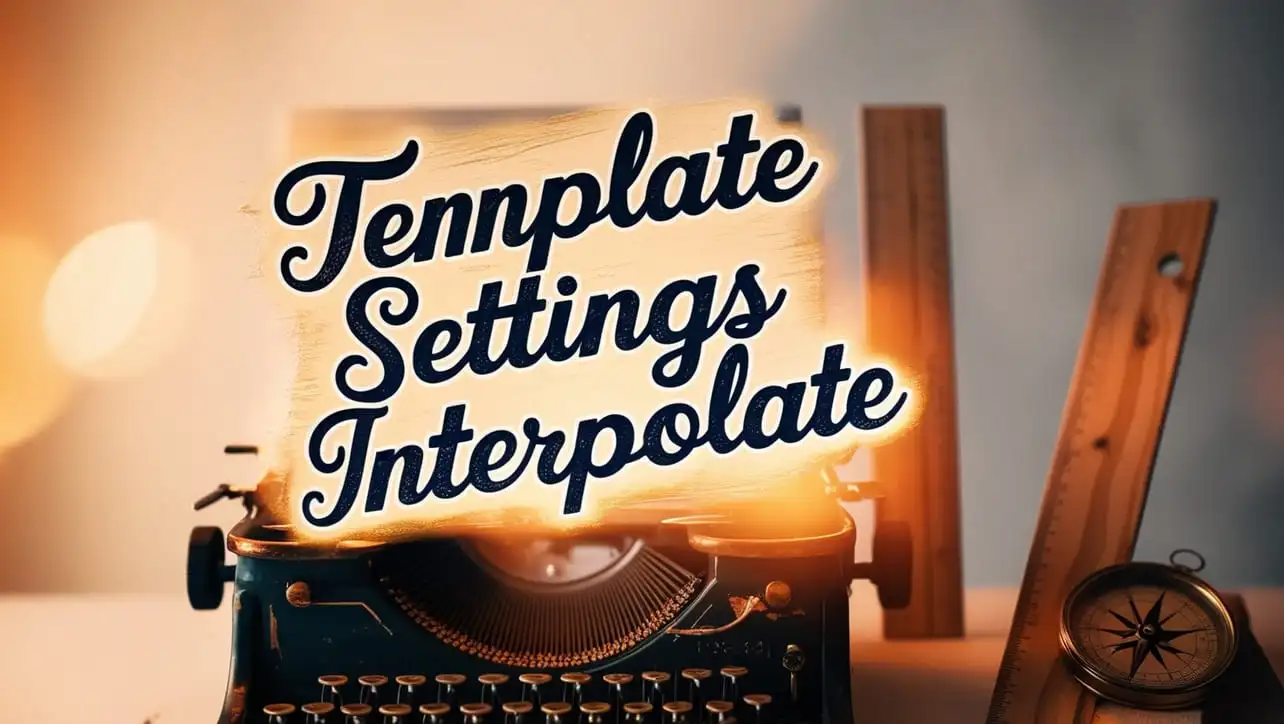
Lodash _.templateSettings.interpolate Property
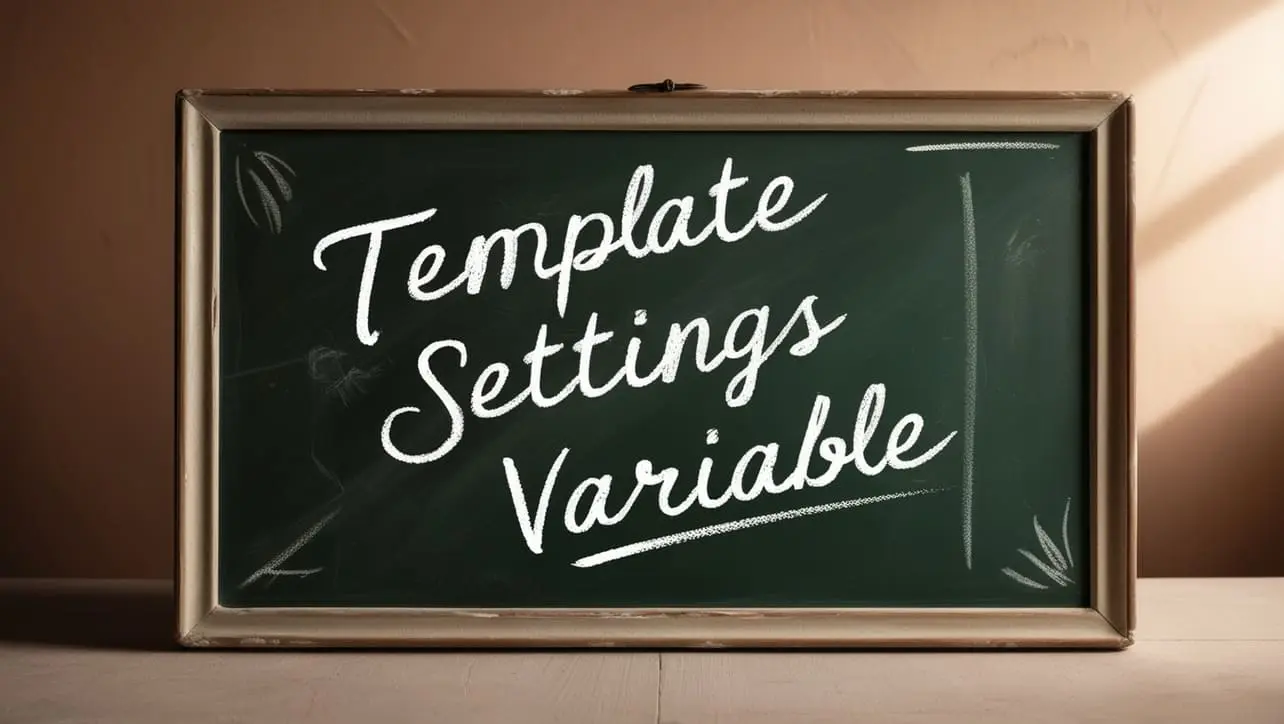
If you have any doubts regarding this article (Lodash _.now() Date Method), please comment here. I will help you immediately.