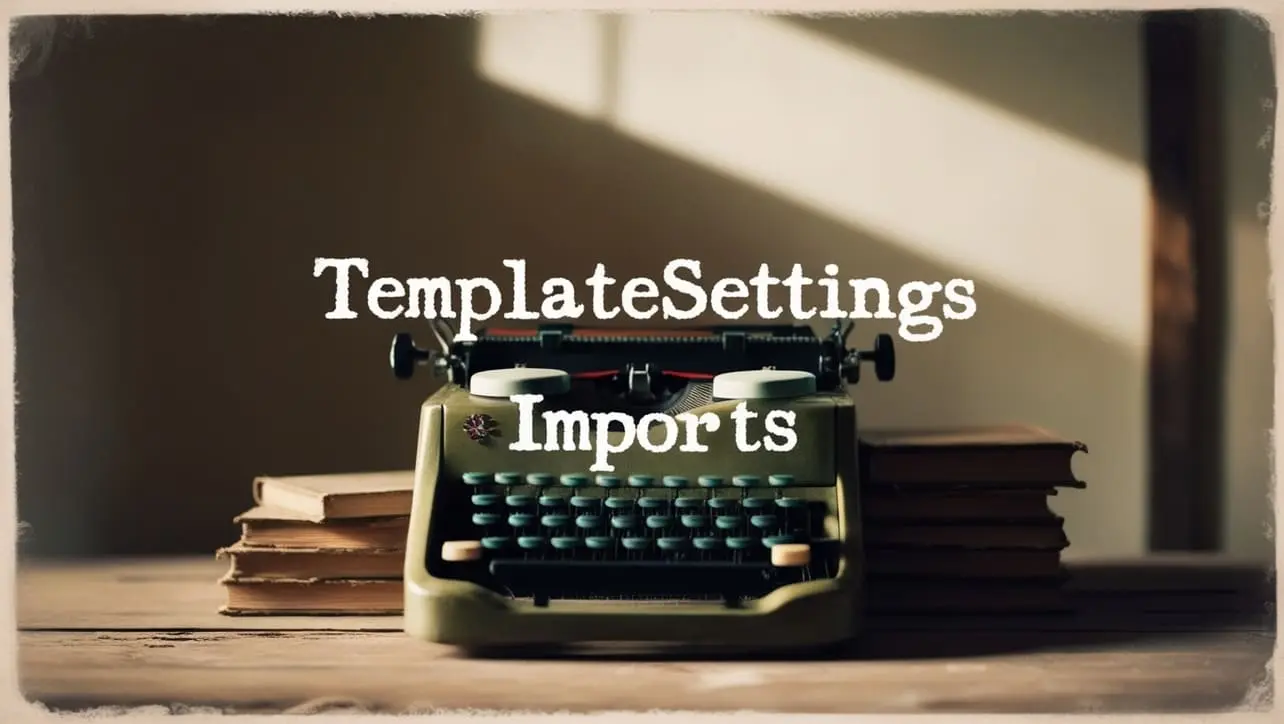
Lodash _.some() Collection Method
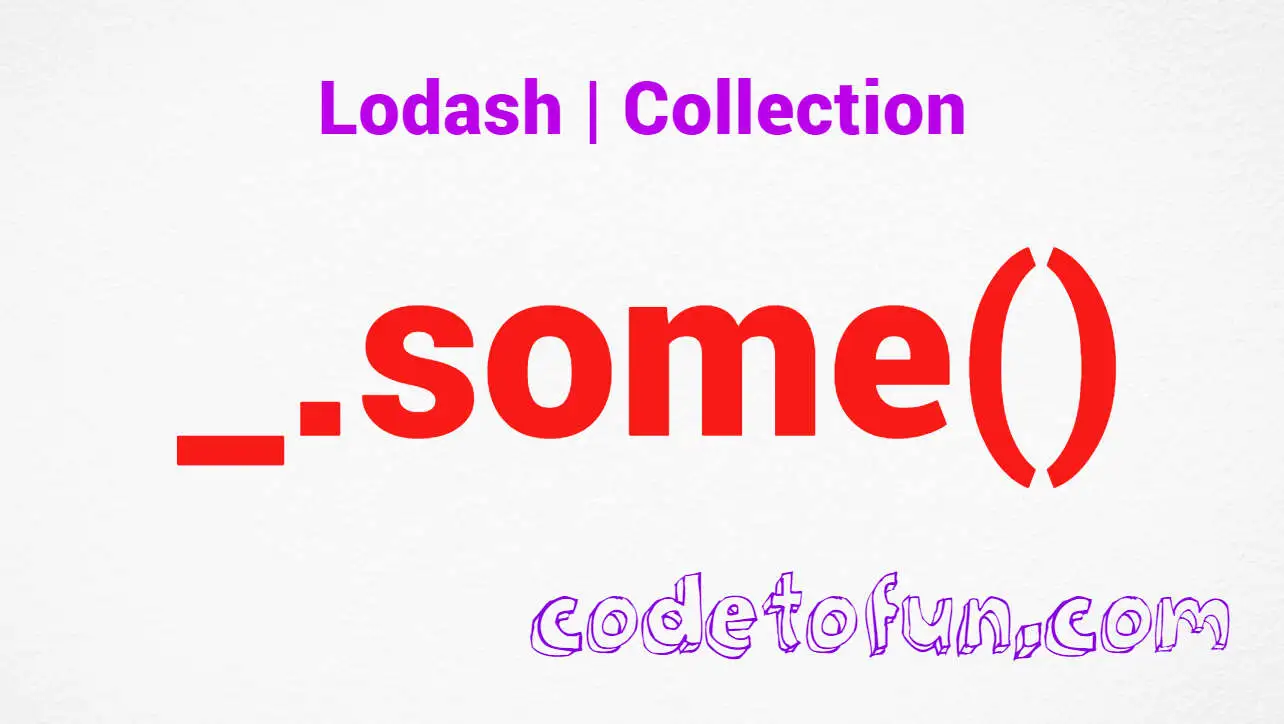
Photo Credit to CodeToFun
🙋 Introduction
Efficiently traversing and validating collections is a common task in JavaScript development. Lodash, a versatile utility library, provides the _.some()
method, offering a concise and powerful solution to check if at least one element in a collection satisfies a given condition.
This method enhances the expressiveness of your code and simplifies the process of collection evaluation.
🧠 Understanding _.some() Method
The _.some()
method in Lodash is designed to determine whether at least one element in a collection meets a specified condition. It stops iteration once a truthy value is encountered, providing early exit functionality and improving performance.
💡 Syntax
The syntax for the _.some()
method is straightforward:
_.some(collection, [predicate])
- collection: The collection to iterate over.
- predicate (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.some()
method:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const hasEvenNumber = _.some(numbers, num => num % 2 === 0);
console.log(hasEvenNumber);
// Output: true
In this example, the _.some()
method checks if at least one element in the numbers array is an even number.
🏆 Best Practices
When working with the _.some()
method, consider the following best practices:
Understand Truthy Conditions:
Be mindful of the truthy conditions when using
_.some()
. It stops iteration when it encounters the first truthy value, which may not always be a strict boolean true.example.jsCopiedconst mixedData = [0, '', false, 4, 'lodash']; const hasTruthyValue = _.some(mixedData); console.log(hasTruthyValue); // Output: true (because 'lodash' is truthy)
Leverage Predicate Function:
Utilize the predicate parameter to define custom conditions. This allows you to tailor the behavior of
_.some()
based on your specific requirements.example.jsCopiedconst users = [ { id: 1, name: 'Alice', isActive: true }, { id: 2, name: 'Bob', isActive: false }, { id: 3, name: 'Charlie', isActive: true }, ]; const isActiveUser = _.some(users, user => user.isActive); console.log(isActiveUser); // Output: true
Early Exit Optimization:
Take advantage of the early exit functionality provided by
_.some()
. If the condition is met early in the collection, further iterations are skipped, enhancing performance.example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; const hasDesiredData = _.some(largeDataset, data => /* ...custom condition... */); console.log(hasDesiredData);
📚 Use Cases
Validation in Forms:
_.some()
is useful for form validation scenarios, where you need to check if at least one input meets certain criteria.example.jsCopiedconst formInputs = [ { name: 'username', value: 'john_doe', isValid: true }, { name: 'password', value: 'secure123', isValid: false }, { name: 'email', value: 'john@example.com', isValid: true }, ]; const isFormValid = _.some(formInputs, input => !input.isValid); console.log(isFormValid); // Output: false
Permission Checks:
When dealing with user permissions,
_.some()
can be employed to determine if a user has at least one required permission.example.jsCopiedconst userPermissions = ['read', 'write']; const hasWritePermission = _.some(userPermissions, permission => permission === 'write'); console.log(hasWritePermission); // Output: true
Data Filtering:
Use
_.some()
to filter data based on a specific condition, allowing you to retrieve elements that meet a particular criterion.example.jsCopiedconst data = [ { id: 1, category: 'fruit', inStock: false }, { id: 2, category: 'vegetable', inStock: true }, { id: 3, category: 'fruit', inStock: true }, ]; const hasOutOfStockItem = _.some(data, item => !item.inStock); console.log(hasOutOfStockItem); // Output: true
🎉 Conclusion
The _.some()
method in Lodash is a valuable tool for efficiently checking if at least one element in a collection satisfies a given condition. Whether you're performing form validation, permission checks, or data filtering, _.some()
provides a succinct and performant solution for collection evaluation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.some()
method in your Lodash projects.
👨💻 Join our Community:
Author
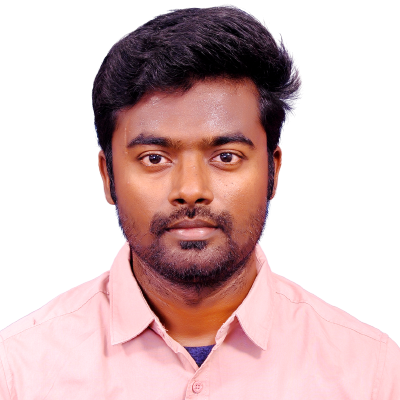
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
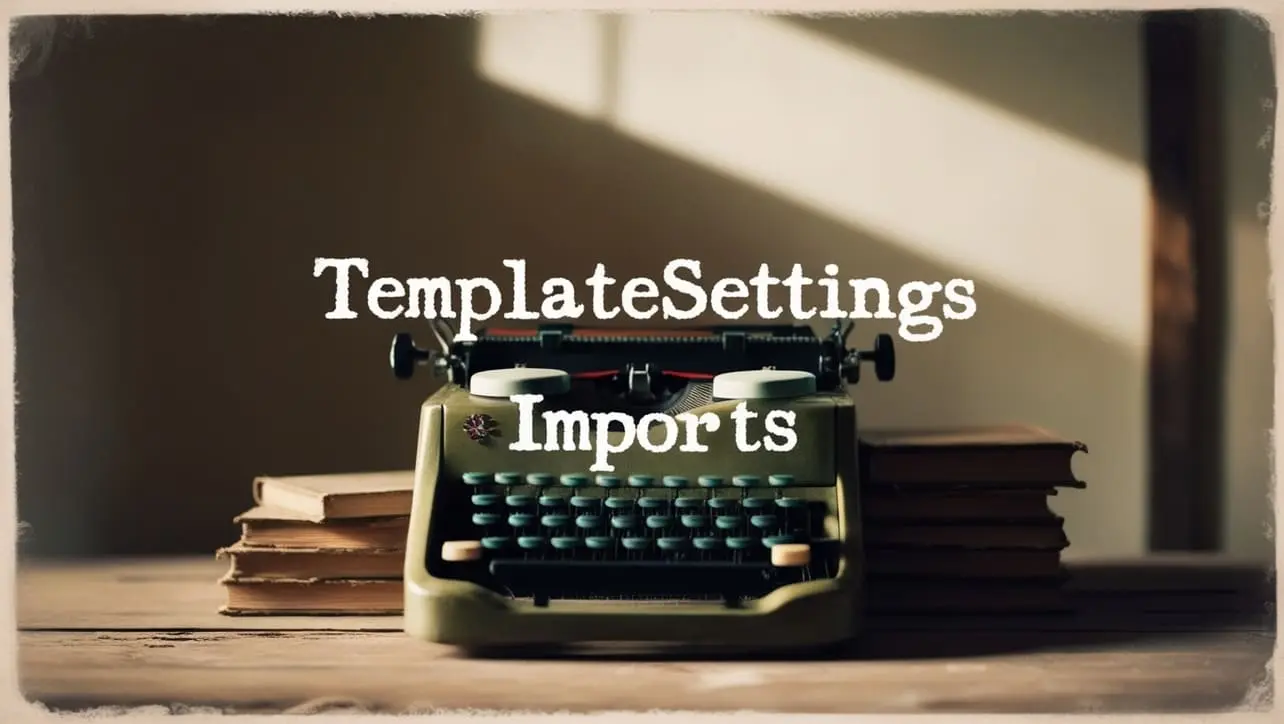
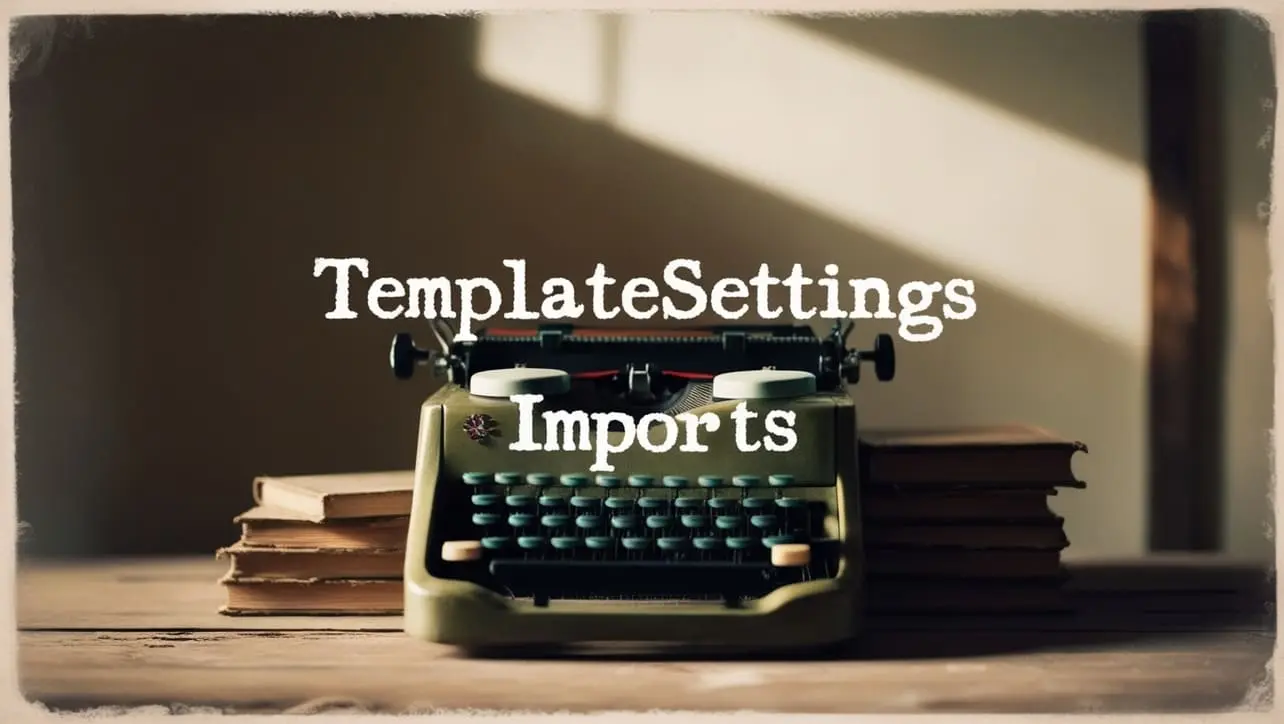
Lodash _.templateSettings.imports Property
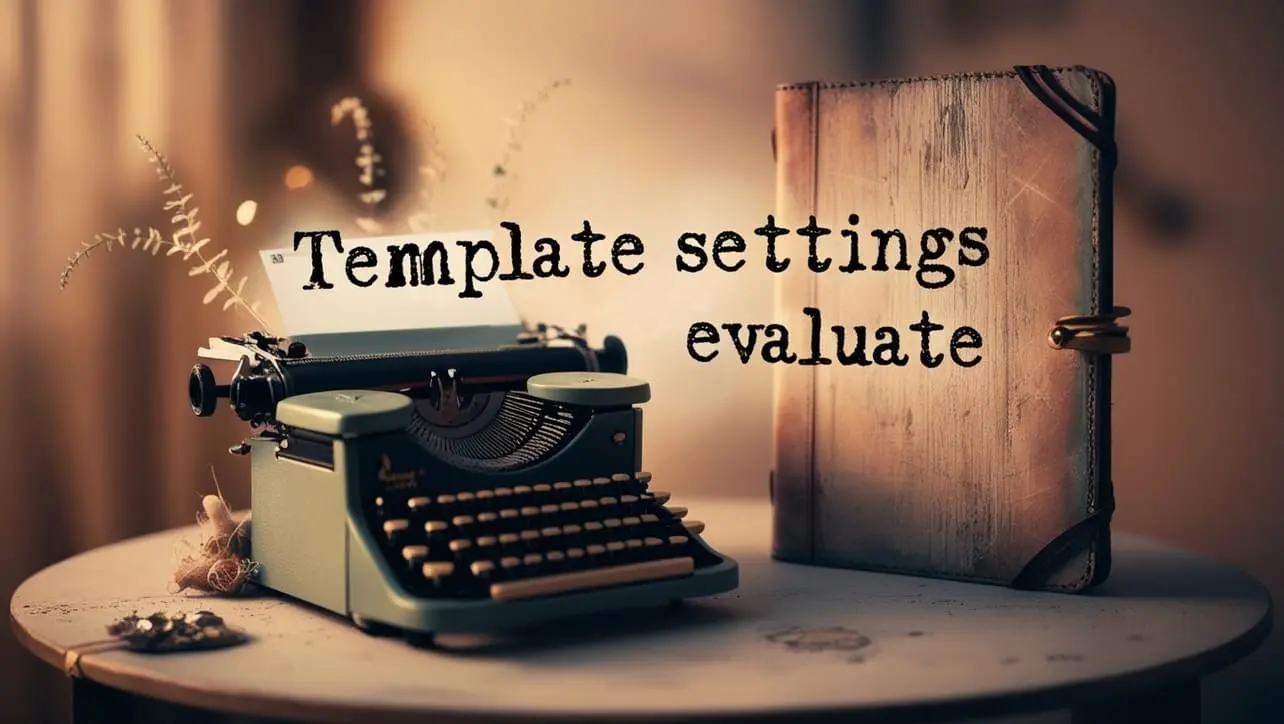
Lodash _.templateSettings.evaluate Property
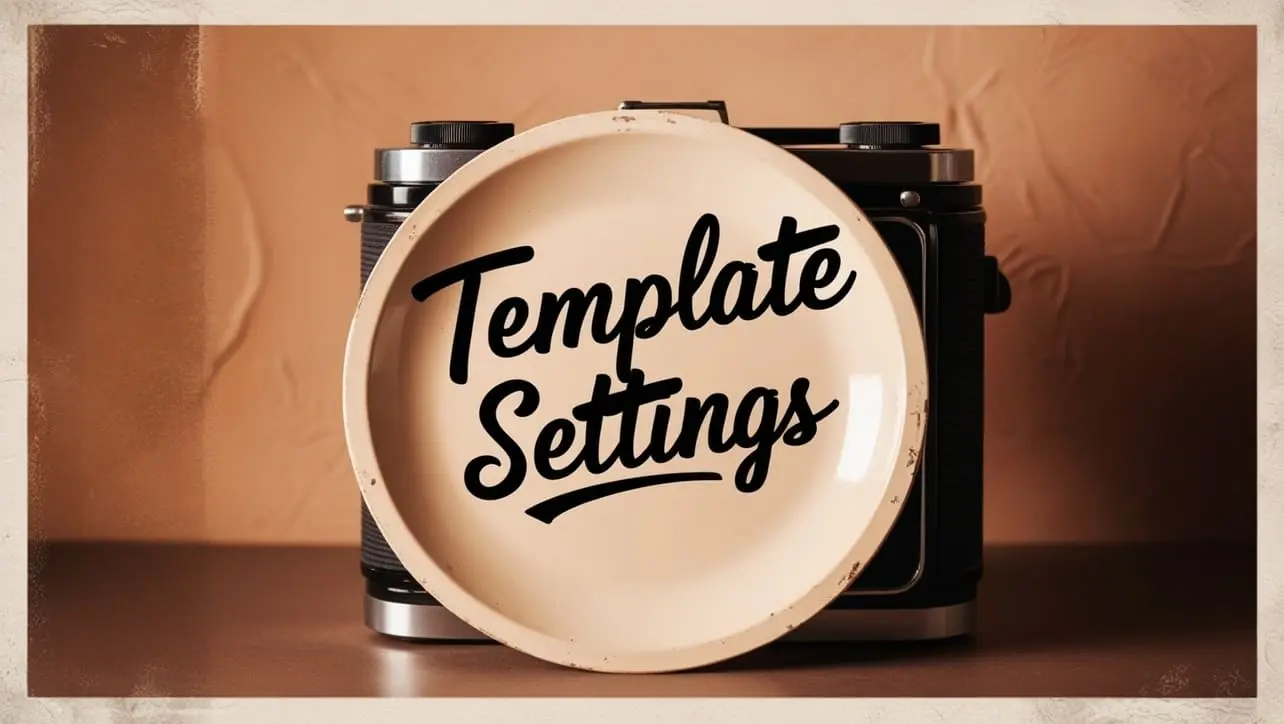
Lodash _.templateSettings Property
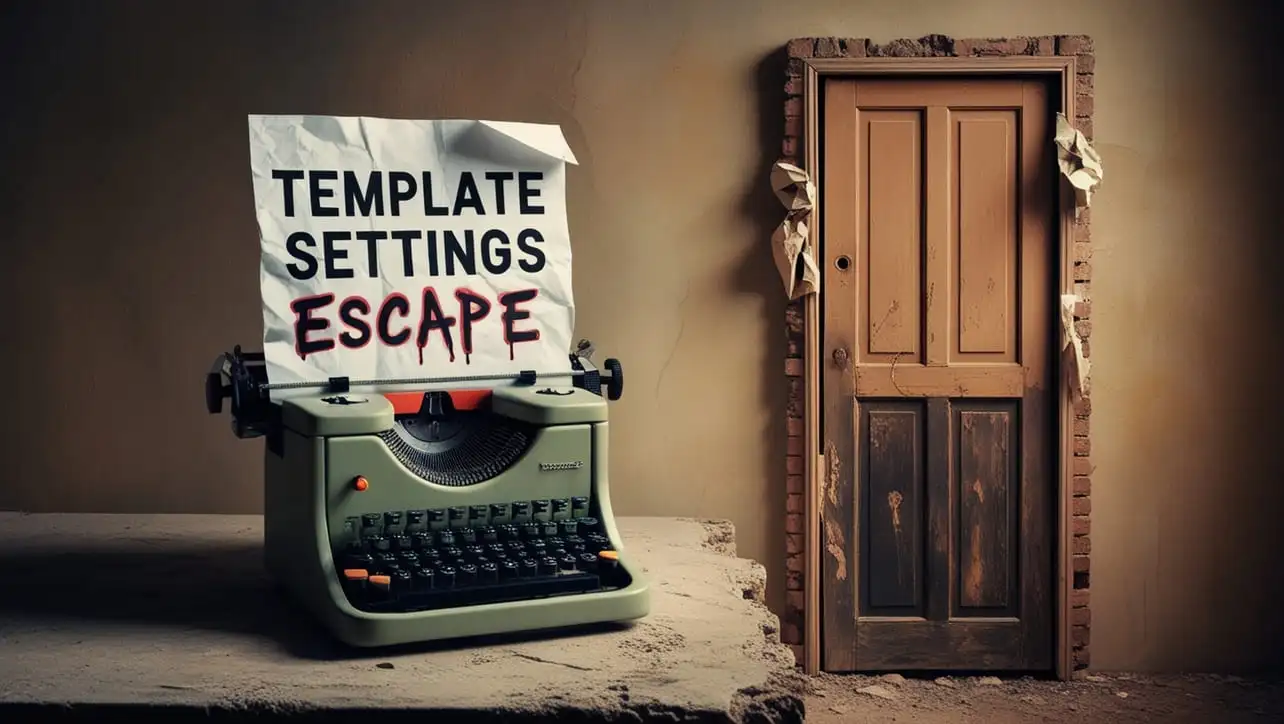
Lodash _.templateSettings.escape Property
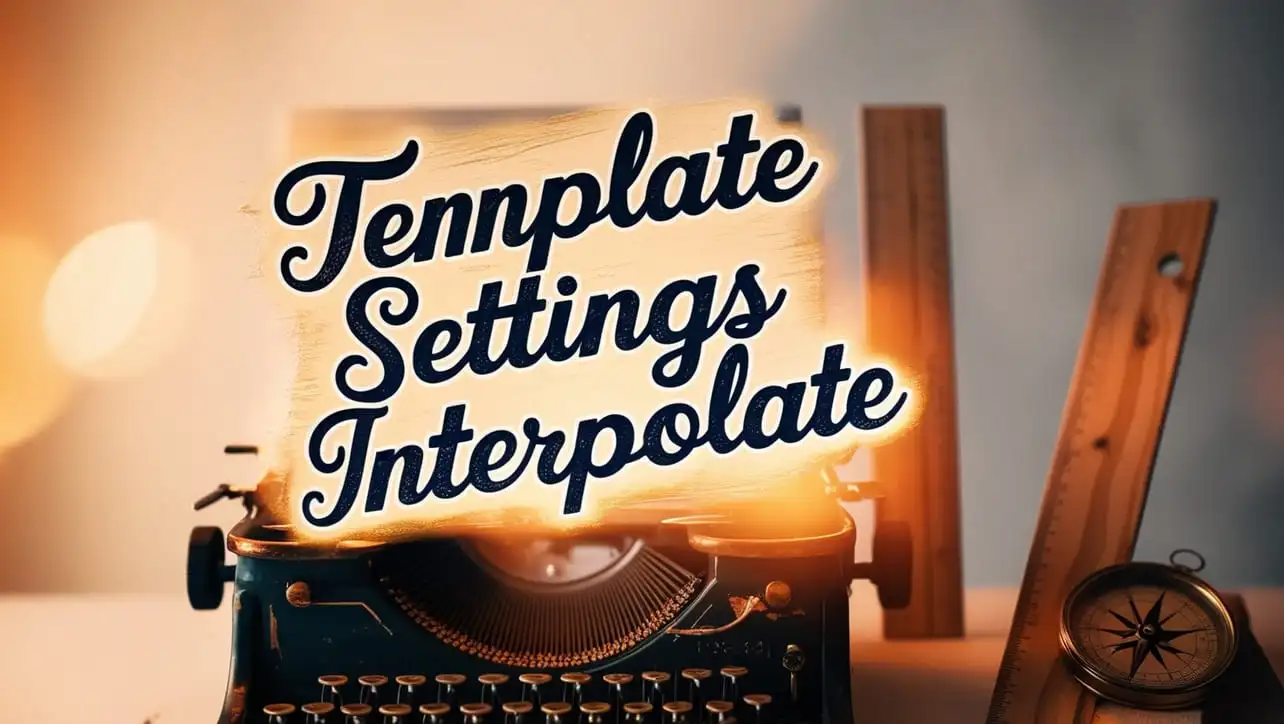
Lodash _.templateSettings.interpolate Property
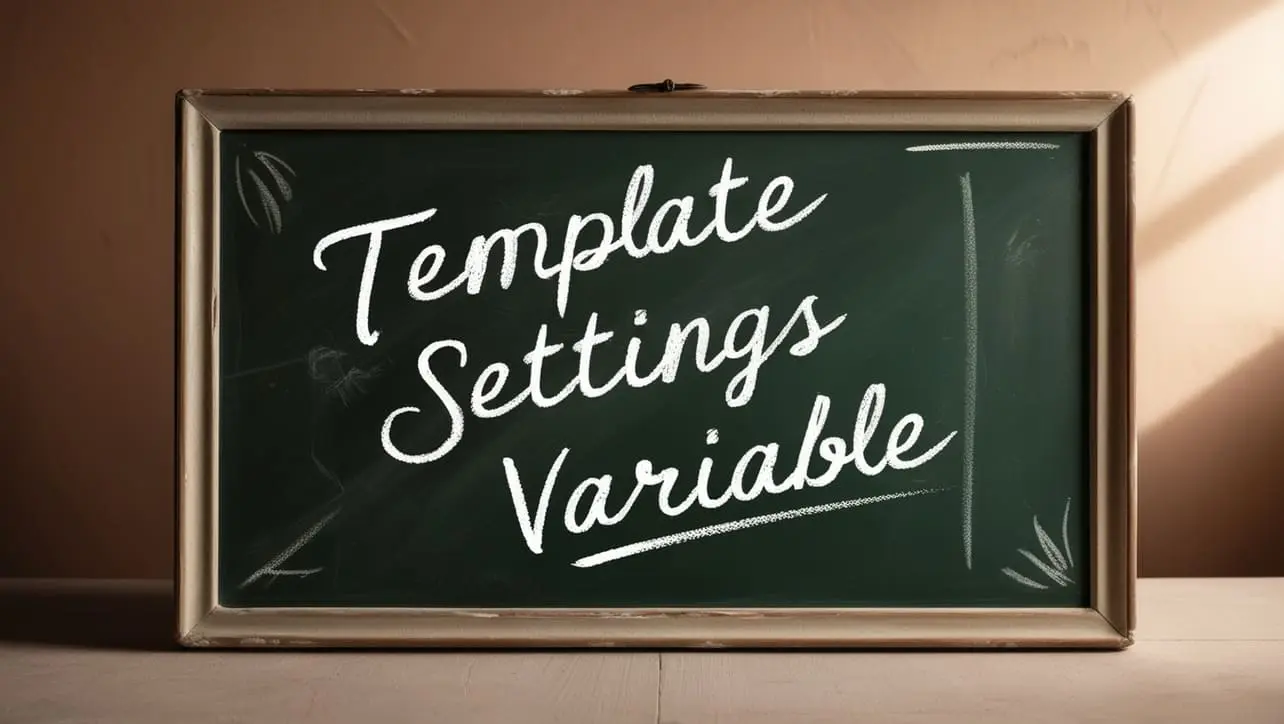
If you have any doubts regarding this article (Lodash _.some() Collection Method), please comment here. I will help you immediately.