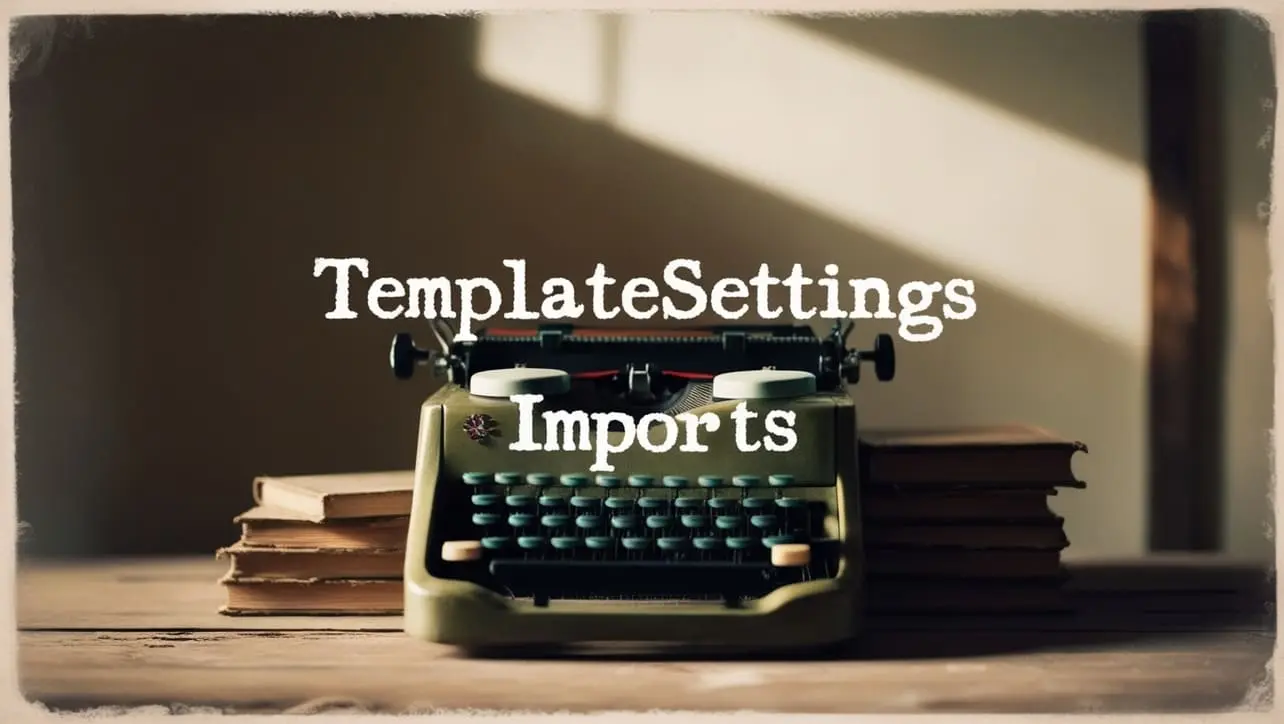
Lodash _.sample() Collection Method
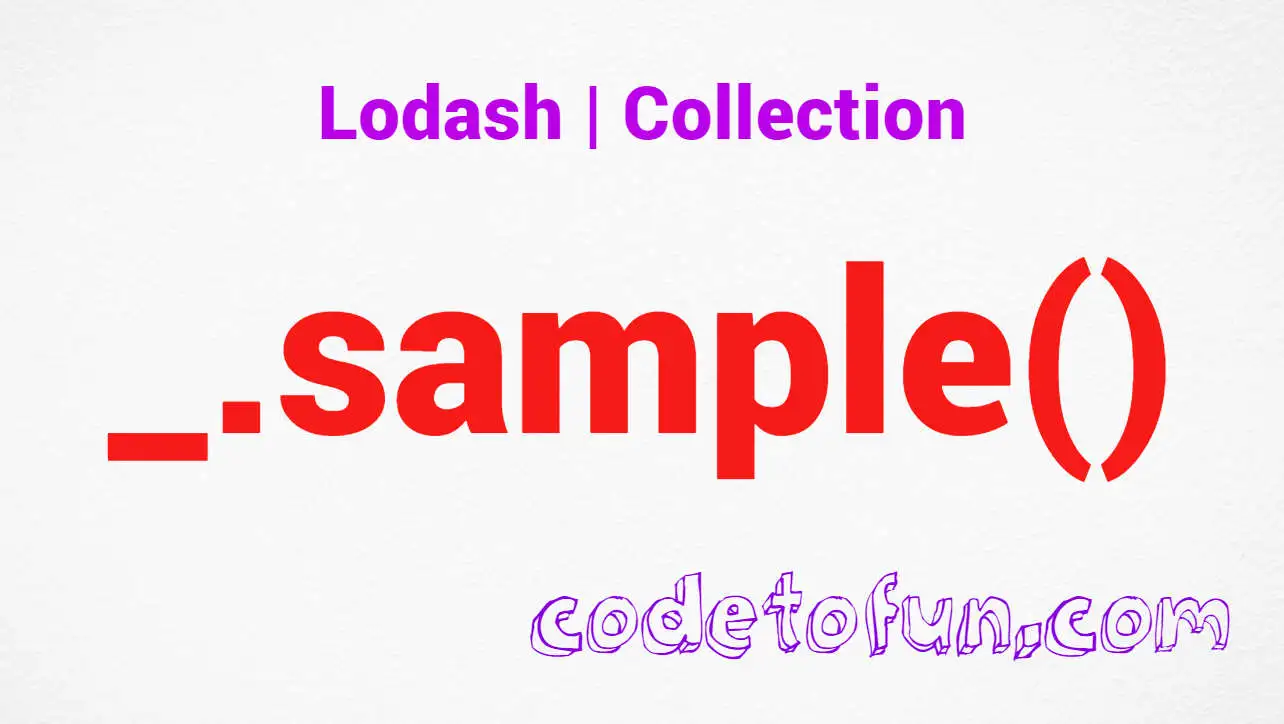
Photo Credit to CodeToFun
🙋 Introduction
In the diverse landscape of JavaScript development, manipulating collections with efficiency is key. Lodash, a comprehensive utility library, provides an array of functions to simplify common programming tasks. Among these functions, the _.sample()
method stands out as a versatile tool for extracting random elements from a collection.
This method is valuable for scenarios where randomization is essential, offering developers a straightforward way to introduce unpredictability into their code.
🧠 Understanding _.sample() Method
The _.sample()
method in Lodash is designed to retrieve a random element from a collection. Whether you're working with arrays, objects, or other iterable structures, _.sample()
adds a touch of randomness to your application, making it useful for scenarios like shuffling content, creating randomized quizzes, or any situation where randomness is a desirable feature.
💡 Syntax
The syntax for the _.sample()
method is straightforward:
_.sample(collection)
- collection: The collection from which to sample.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.sample()
method:
const _ = require('lodash');
const colors = ['red', 'blue', 'green', 'yellow', 'orange'];
const randomColor = _.sample(colors);
console.log(randomColor);
// Output: (Random color from the 'colors' array)
In this example, _.sample()
randomly selects a color from the colors array, providing an element of unpredictability to the code.
🏆 Best Practices
When working with the _.sample()
method, consider the following best practices:
Validate Collection:
Before applying
_.sample()
, ensure that the collection is valid and contains elements. This practice prevents errors that may occur if attempting to sample from an empty or undefined collection.example.jsCopiedconst emptyCollection = []; const randomElement = _.sample(emptyCollection); if (randomElement === undefined) { console.error('Cannot sample from an empty collection'); } else { console.log(randomElement); }
Use Cases of Random Sampling:
Identify scenarios where random sampling enhances your application. Whether it's creating randomized quizzes, shuffling content, or introducing variety into user experiences, understanding the purpose of random sampling guides its effective application.
example.jsCopiedconst quizQuestions = /* ...fetch questions from a database or API... */; const randomQuestion = _.sample(quizQuestions); console.log(randomQuestion); // Output: (Random quiz question)
Repeatable Randomness:
If you need repeatable randomness (e.g., for testing), consider using a seeded random number generator in conjunction with
_.sample()
.example.jsCopiedconst seedrandom = require('seedrandom'); const rng = seedrandom('hello'); const randomValue = _.sample([1, 2, 3], rng); console.log(randomValue); // Output: (Deterministic random value based on the seed 'hello')
📚 Use Cases
Dynamic User Experience:
Implement dynamic and unpredictable user experiences by incorporating
_.sample()
in scenarios like rotating banners, changing background colors, or displaying random content.example.jsCopiedconst bannerImages = /* ...fetch images for banners... */; const randomBanner = _.sample(bannerImages); // Display 'randomBanner' to the user
Game Development:
Incorporate randomness into game development by using
_.sample()
to select items, enemies, or events with an element of unpredictability.example.jsCopiedconst gameEnemies = ['goblin', 'skeleton', 'dragon', 'zombie']; const randomEnemy = _.sample(gameEnemies); // Spawn 'randomEnemy' in the game
Content Shuffling:
Shuffle content on a webpage or application to keep the user experience fresh and engaging by randomly selecting elements for display.
example.jsCopiedconst featuredArticles = /* ...fetch articles for display... */; const shuffledArticles = _.shuffle(featuredArticles); const randomArticle = _.sample(shuffledArticles); // Display 'randomArticle' to the user
🎉 Conclusion
The _.sample()
method in Lodash provides a straightforward and versatile solution for introducing randomness into your JavaScript applications. Whether you're building dynamic user experiences, developing games, or shuffling content, _.sample()
empowers you to bring an element of unpredictability to your code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.sample()
method in your Lodash projects.
👨💻 Join our Community:
Author
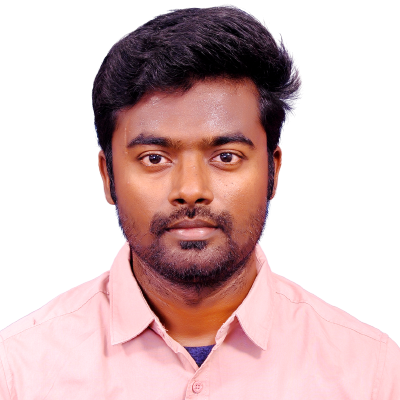
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
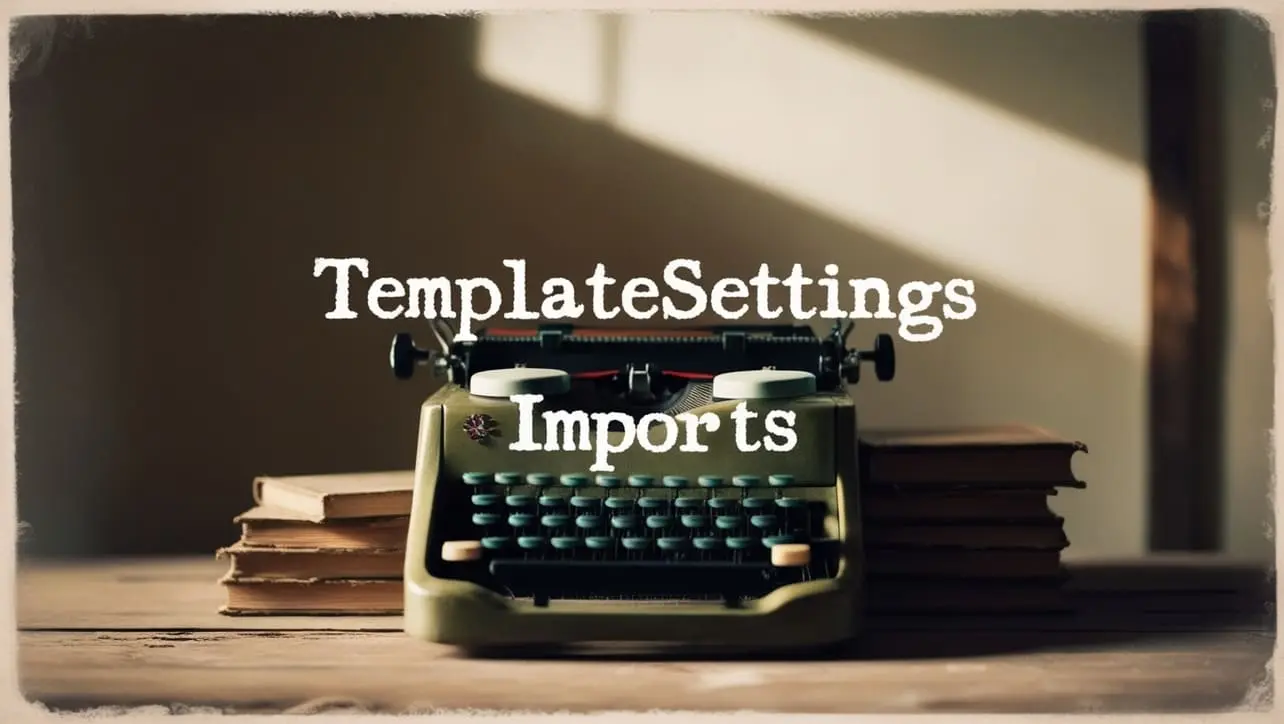
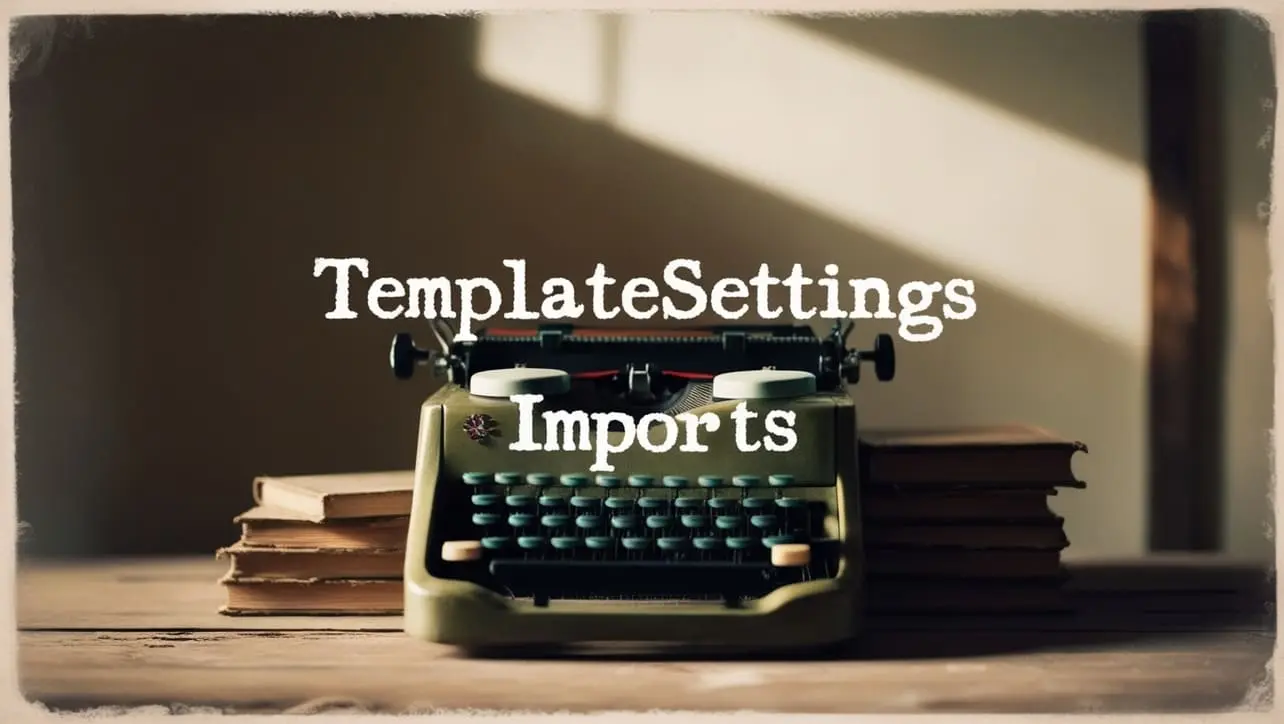
Lodash _.templateSettings.imports Property
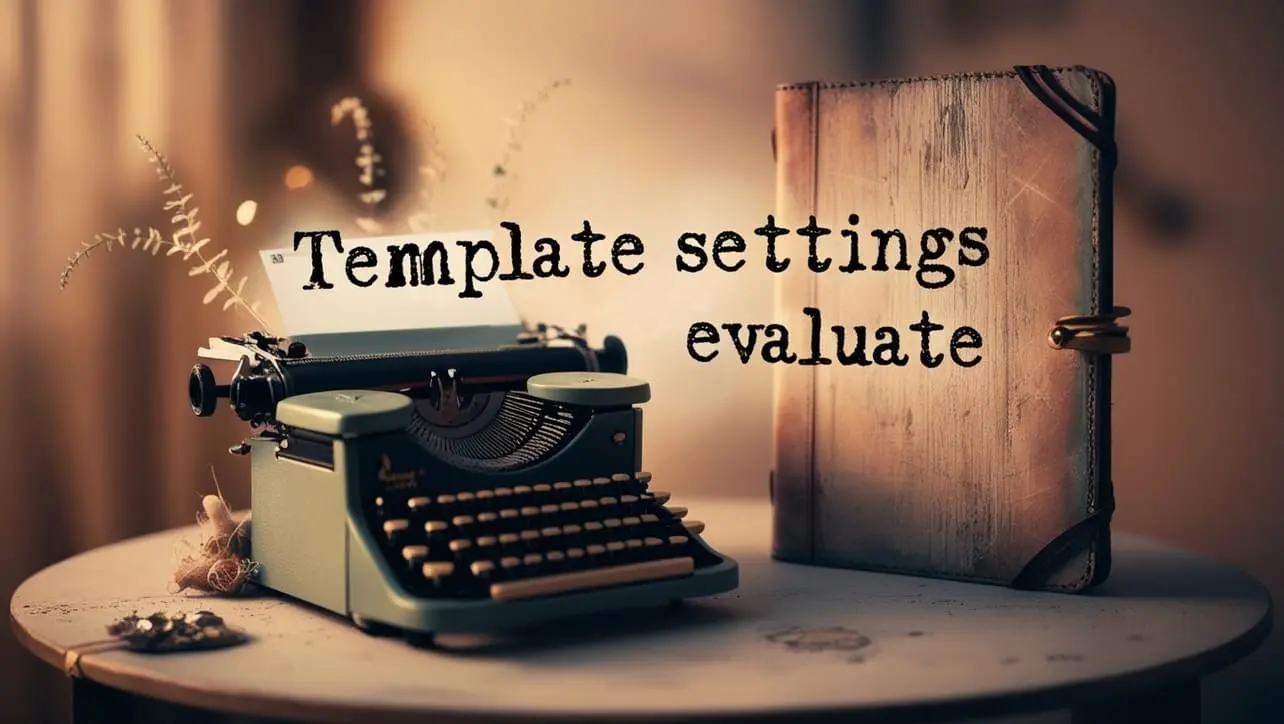
Lodash _.templateSettings.evaluate Property
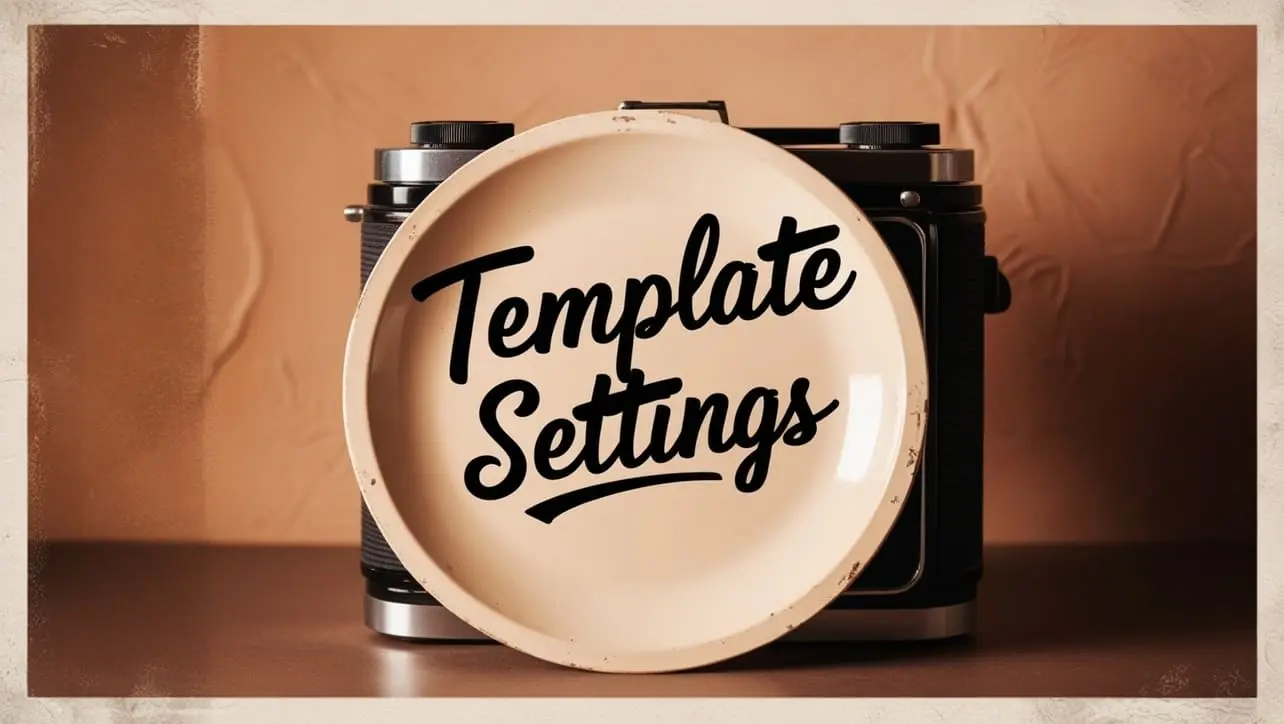
Lodash _.templateSettings Property
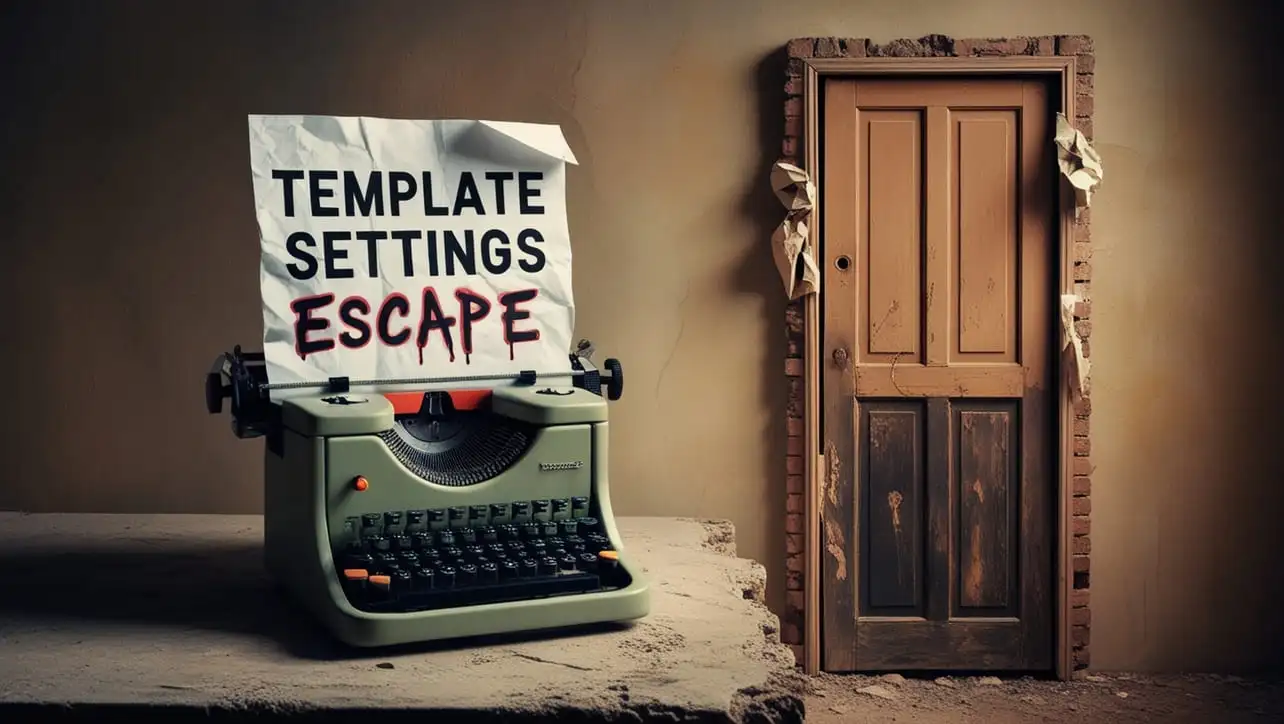
Lodash _.templateSettings.escape Property
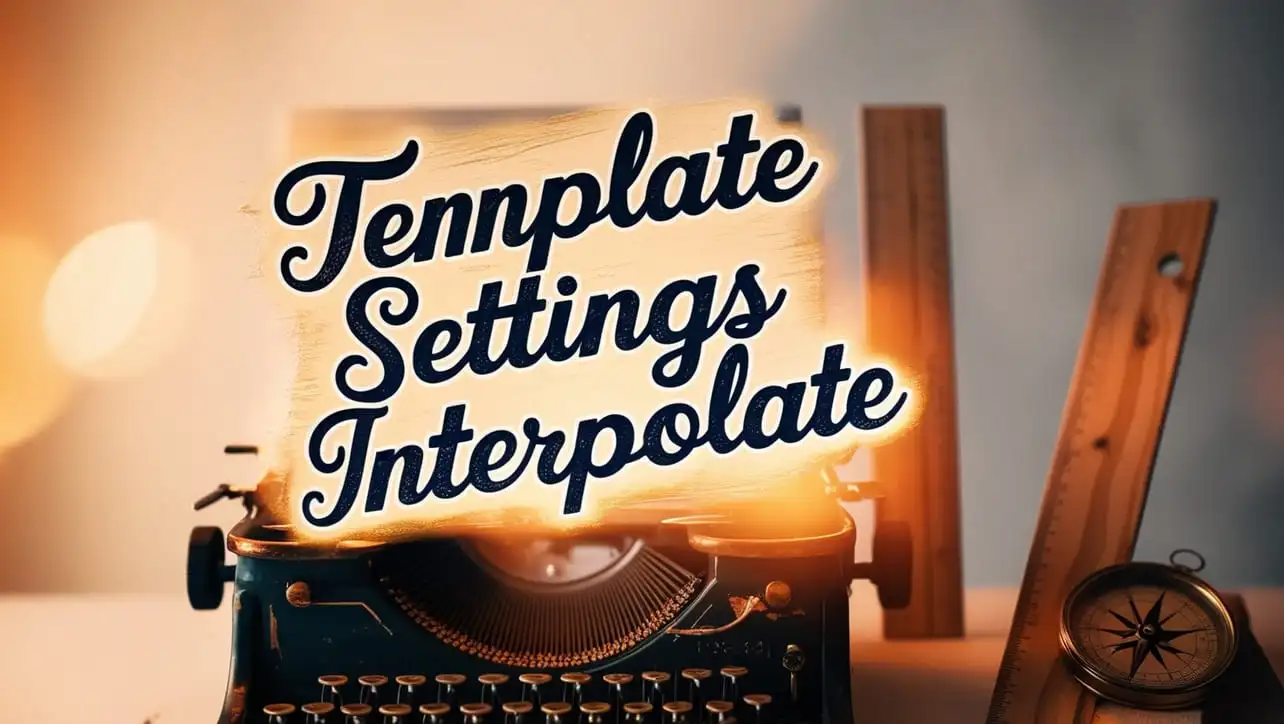
Lodash _.templateSettings.interpolate Property
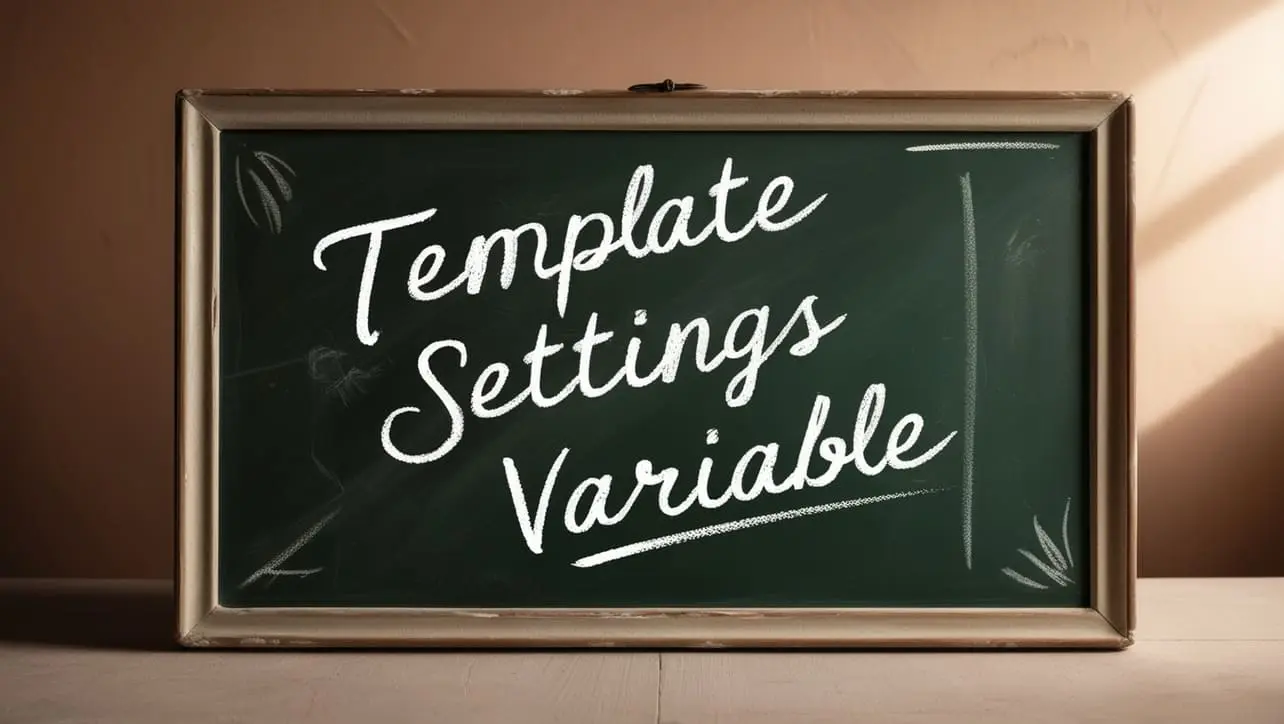
If you have any doubts regarding this article (Lodash _.sample() Collection Method), please comment here. I will help you immediately.