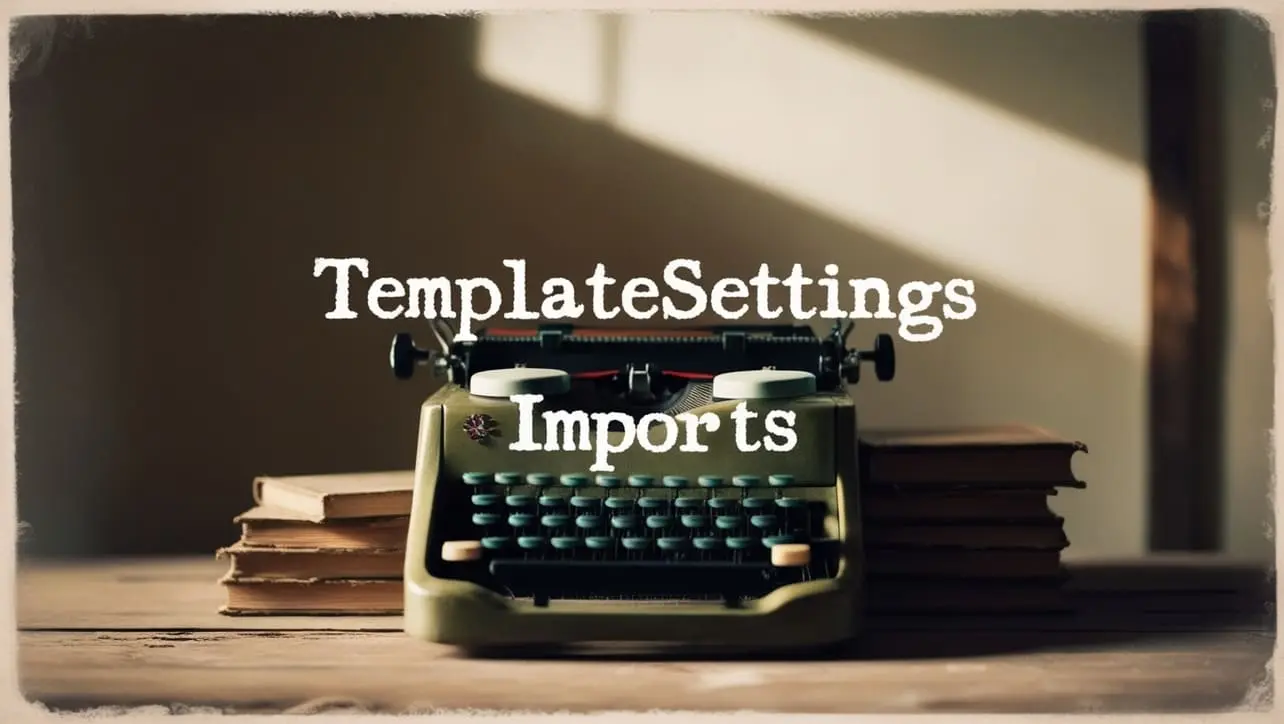
Lodash _.reduce() Collection Method
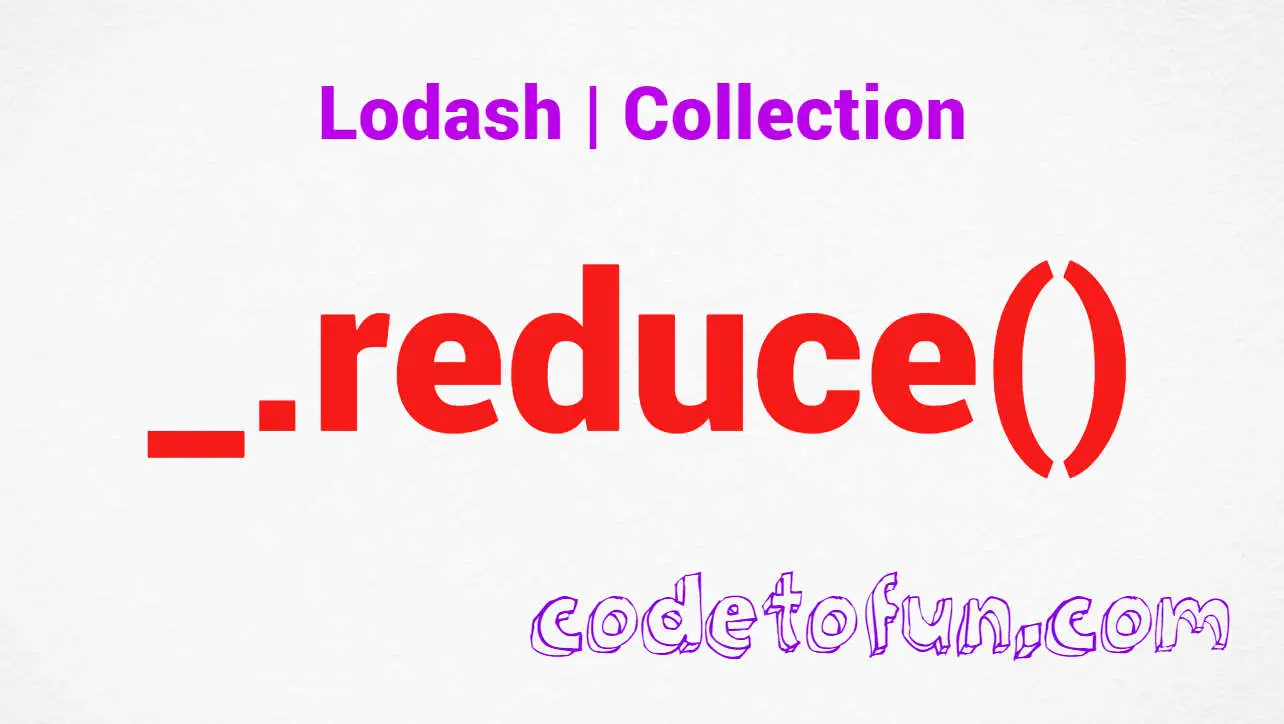
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript development, efficient manipulation of collections is a fundamental skill. Lodash, a powerful utility library, provides developers with a plethora of tools, and among them is the _.reduce()
method. This method offers a versatile and concise way to iterate over a collection, accumulating values and producing a single result.
Whether you're working with arrays or objects, _.reduce()
is a valuable asset for tackling complex data processing tasks.
🧠 Understanding _.reduce() Method
The _.reduce()
method in Lodash is designed for iterative reduction of a collection into a single value. It takes an iteratee function that is applied to each element of the collection, accumulating a result that is ultimately returned. This method is flexible and can be used for a variety of scenarios, from summing up numeric values to constructing complex data structures.
💡 Syntax
The syntax for the _.reduce()
method is straightforward:
_.reduce(collection, iteratee, [accumulator])
- collection: The collection to iterate over.
- iteratee: The function invoked per iteration.
- accumulator (Optional): The initial value of the accumulator.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.reduce()
method:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const sum = _.reduce(numbers, (acc, num) => acc + num, 0);
console.log(sum);
// Output: 15
In this example, the _.reduce()
method is used to calculate the sum of all numbers in the array.
🏆 Best Practices
When working with the _.reduce()
method, consider the following best practices:
Specify an Initial Accumulator Value:
When using
_.reduce()
, it's a good practice to provide an initial value for the accumulator. This ensures predictable behavior, especially when dealing with empty collections.example.jsCopiedconst emptyArray = []; const defaultValue = 10; const result = _.reduce(emptyArray, (acc, value) => acc + value, defaultValue); console.log(result); // Output: 10
Utilize the Third Parameter for Initial Accumulator:
Take advantage of the optional third parameter, the initial value of the accumulator. This can simplify your code and make it more readable.
example.jsCopiedconst data = [10, 20, 30, 40, 50]; const calculateSum = (acc, num) => acc + num; const sumUsingThirdParameter = _.reduce(data, calculateSum, 0); const sumWithoutThirdParameter = _.reduce(data, calculateSum); console.log(sumUsingThirdParameter); console.log(sumWithoutThirdParameter); // Both outputs: 150
Choose an Appropriate Iteratee Function:
Select or create an iteratee function that suits your specific task. The iteratee function defines how each element contributes to the accumulator.
example.jsCopiedconst words = ['hello', 'world', 'lodash']; const concatenateWords = (acc, word) => acc + ' ' + word; const sentence = _.reduce(words, concatenateWords, ''); console.log(sentence); // Output: ' hello world lodash'
📚 Use Cases
Summing Numeric Values:
One of the most common use cases for
_.reduce()
is summing up numeric values in an array.example.jsCopiedconst expenses = [100, 200, 50, 75, 120]; const totalExpenses = _.reduce(expenses, (acc, expense) => acc + expense, 0); console.log(totalExpenses); // Output: 545
Building Objects:
_.reduce()
can be utilized to construct objects from an array of key-value pairs.example.jsCopiedconst keyValuePairs = [['name', 'John'], ['age', 30], ['city', 'New York']]; const person = _.reduce(keyValuePairs, (acc, [key, value]) => ({ ...acc, [key]: value }), {}); console.log(person); // Output: { name: 'John', age: 30, city: 'New York' }
Data Transformation:
Transform data within a collection using
_.reduce()
to achieve a specific structure or format.example.jsCopiedconst rawData = [1, 2, 3, 4, 5]; const transformedData = _.reduce(rawData, (acc, num) => [...acc, { value: num * 2 }], []); console.log(transformedData); // Output: [ { value: 2 }, { value: 4 }, { value: 6 }, { value: 8 }, { value: 10 } ]
🎉 Conclusion
The _.reduce()
method in Lodash provides a powerful mechanism for iterating over collections and accumulating values. Its flexibility makes it a valuable tool for a wide range of tasks, from simple numeric calculations to complex data transformations. Incorporate _.reduce()
into your JavaScript arsenal to streamline your code and tackle collection manipulation with ease.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.reduce()
method in your Lodash projects.
👨💻 Join our Community:
Author
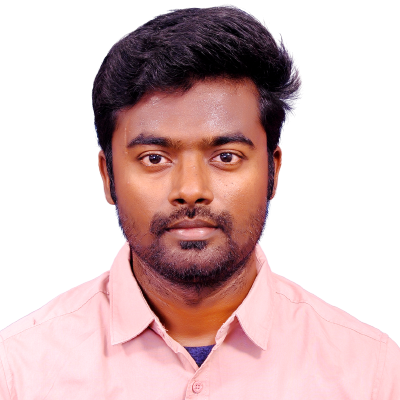
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
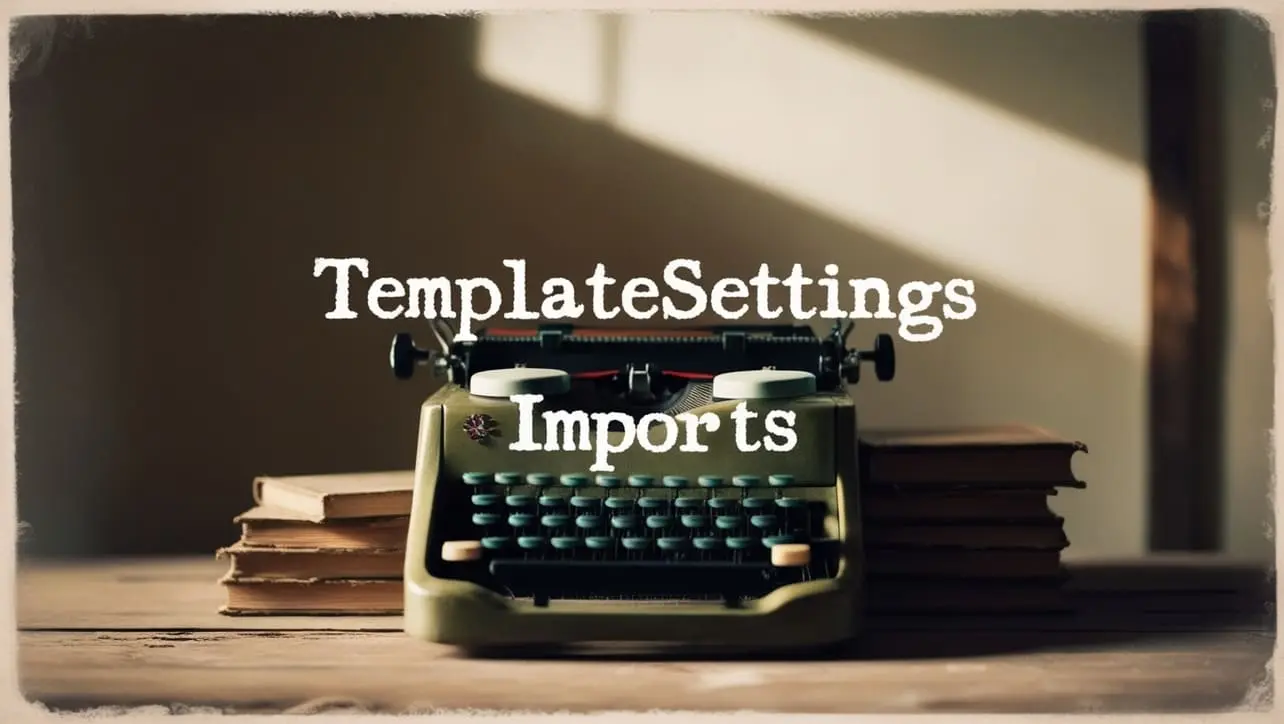
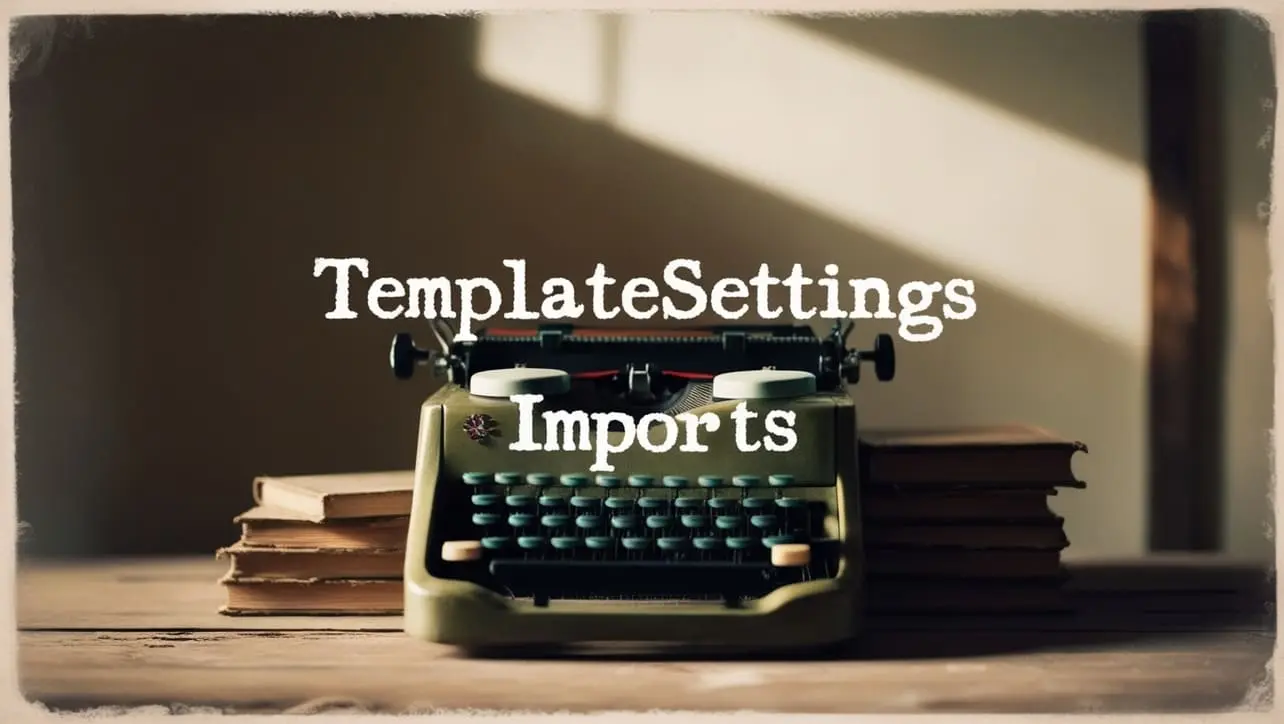
Lodash _.templateSettings.imports Property
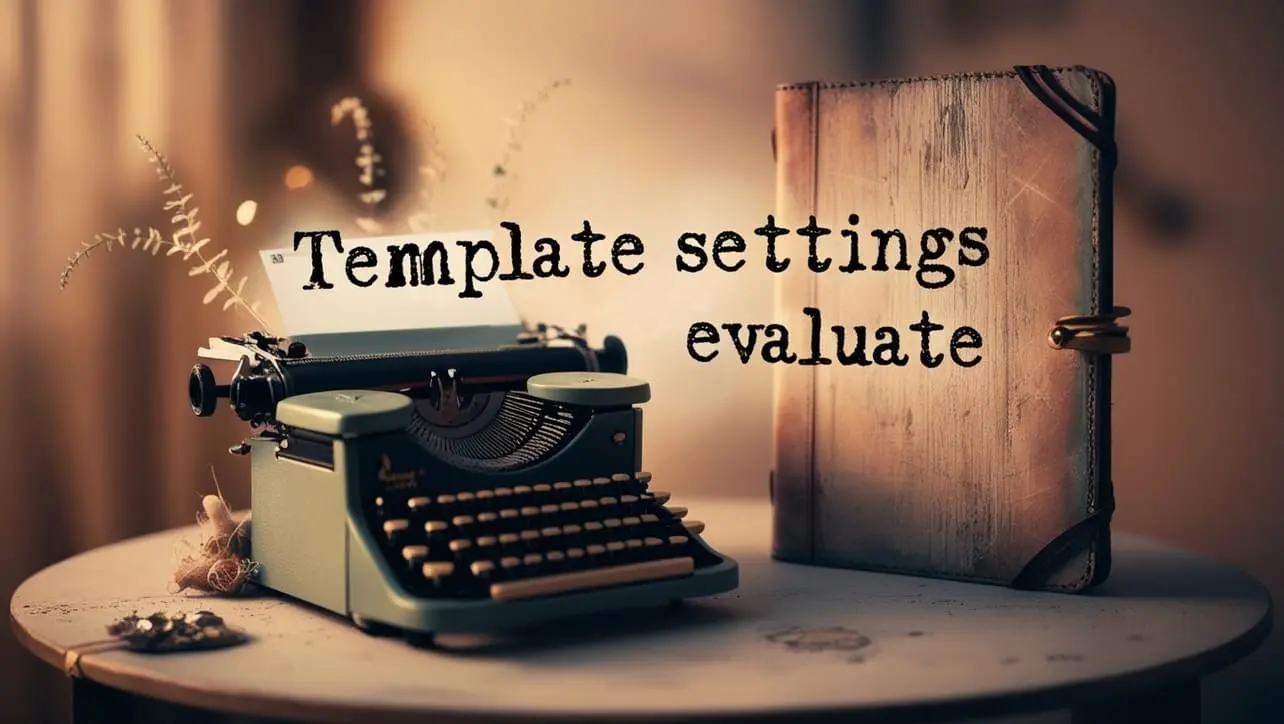
Lodash _.templateSettings.evaluate Property
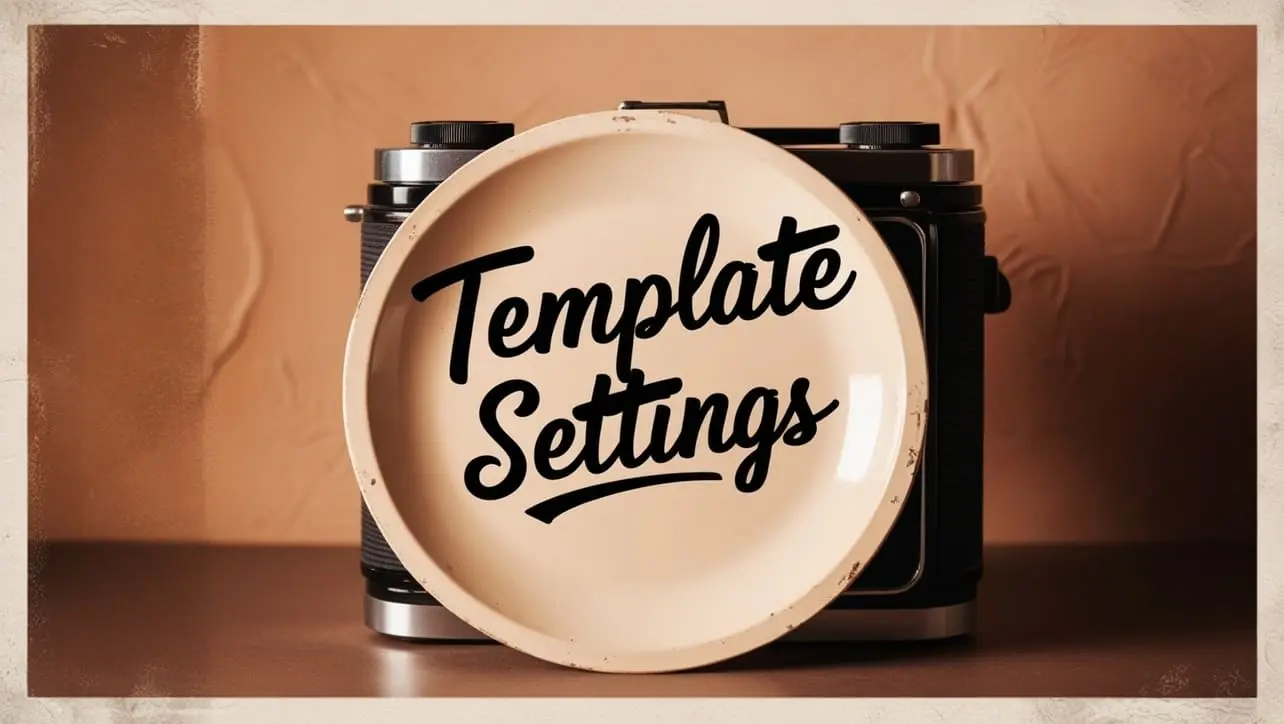
Lodash _.templateSettings Property
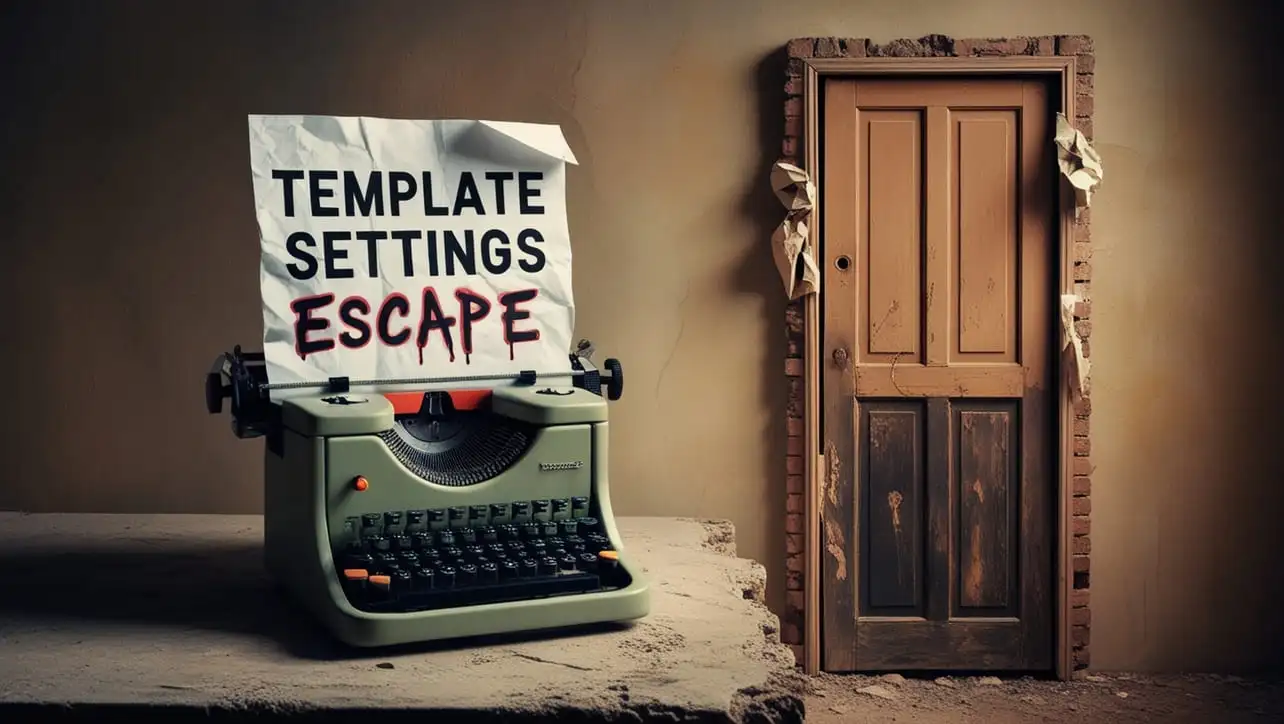
Lodash _.templateSettings.escape Property
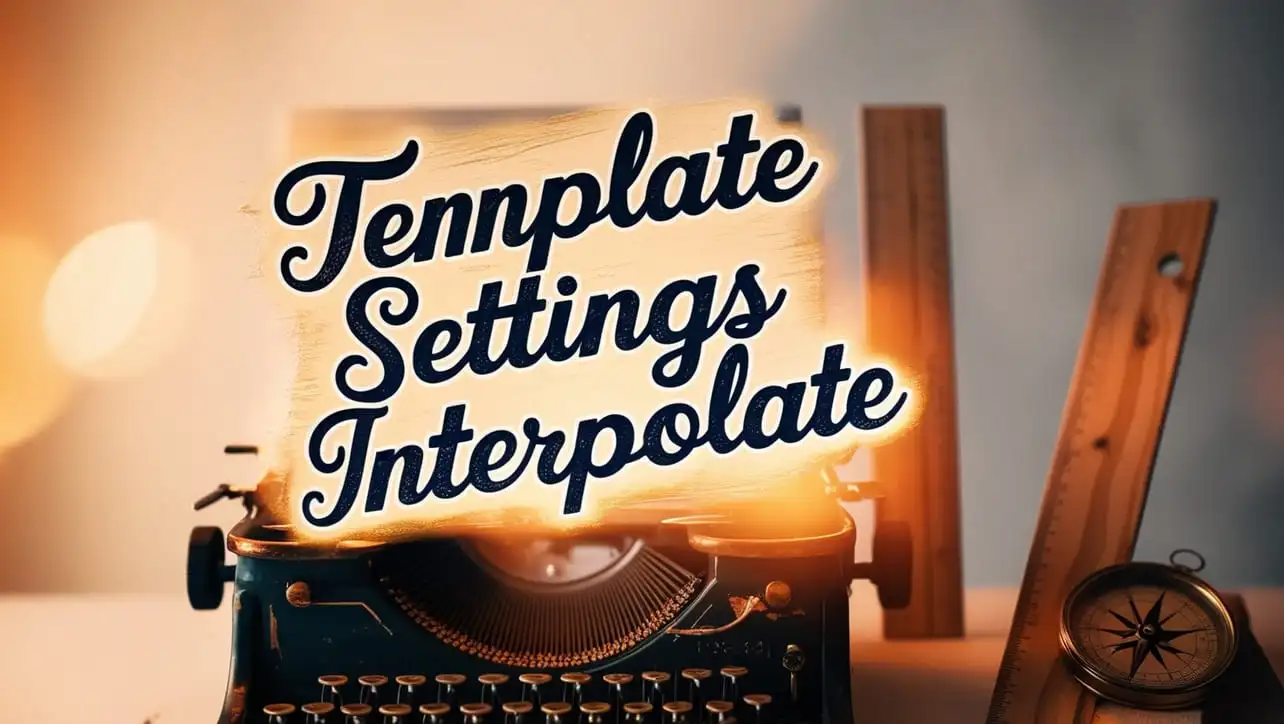
Lodash _.templateSettings.interpolate Property
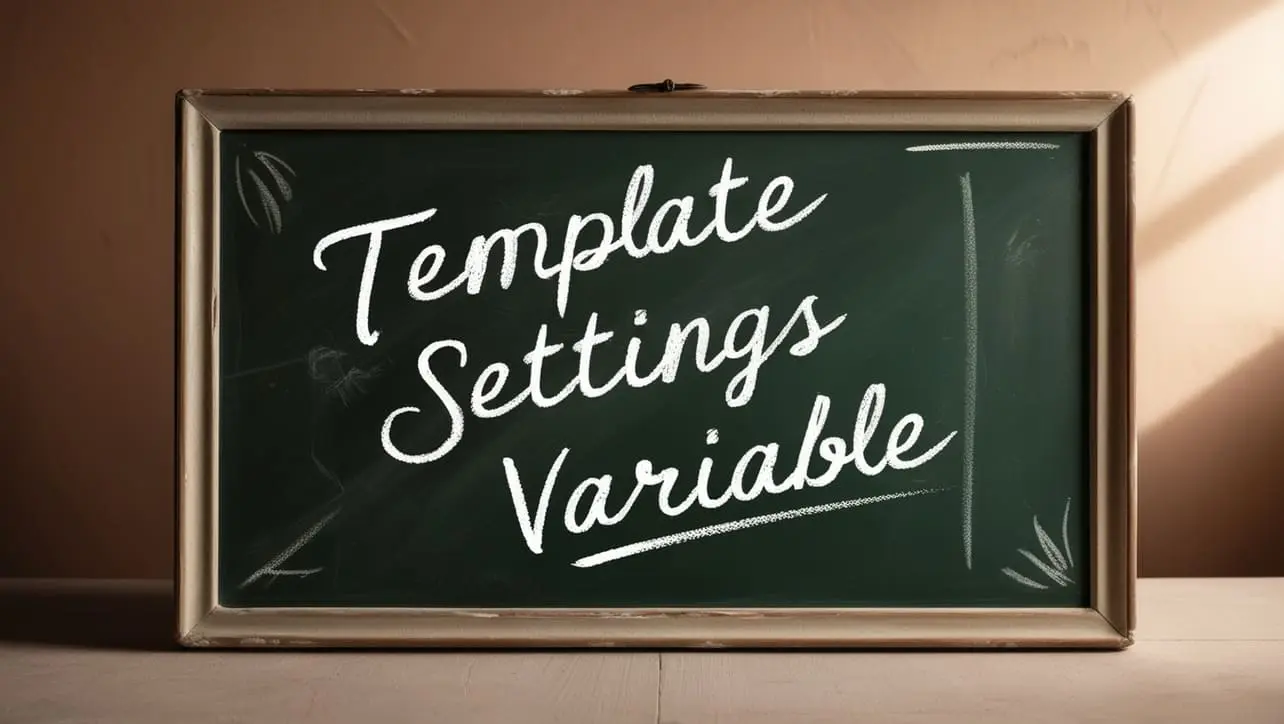
If you have any doubts regarding this article (Lodash _.reduce() Collection Method), please comment here. I will help you immediately.