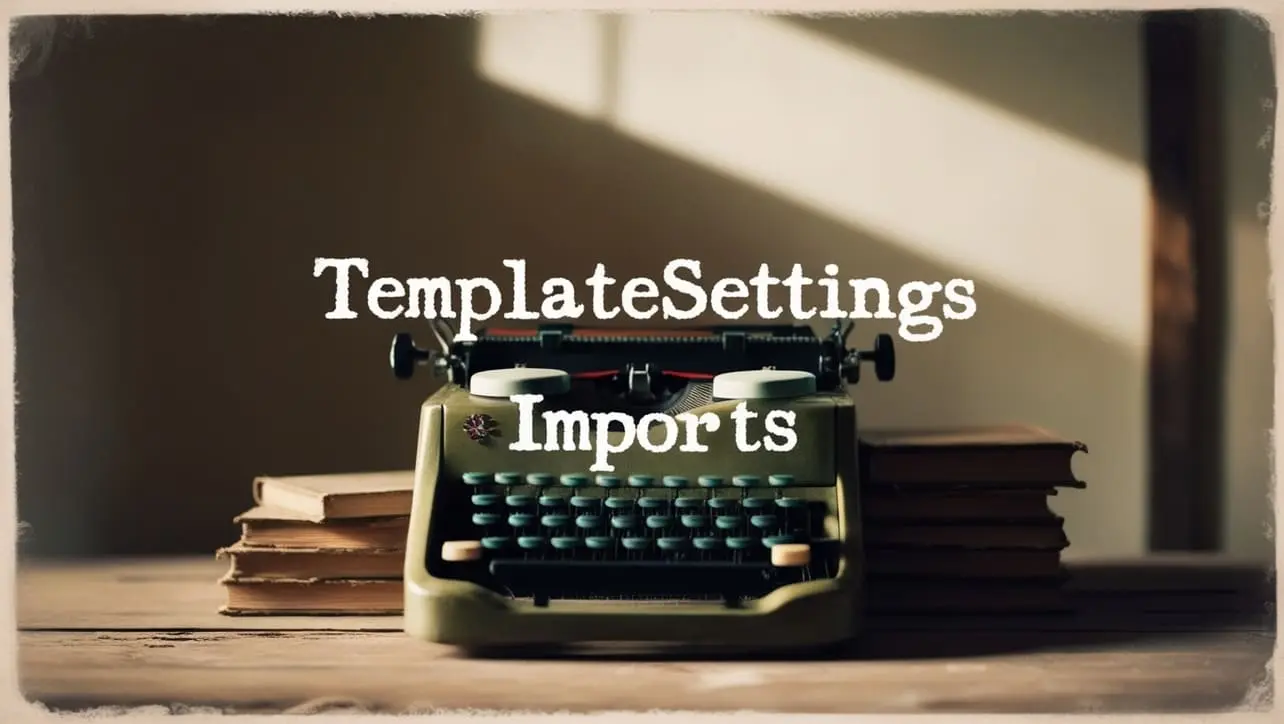
Lodash _.invokeMap() Collection Method
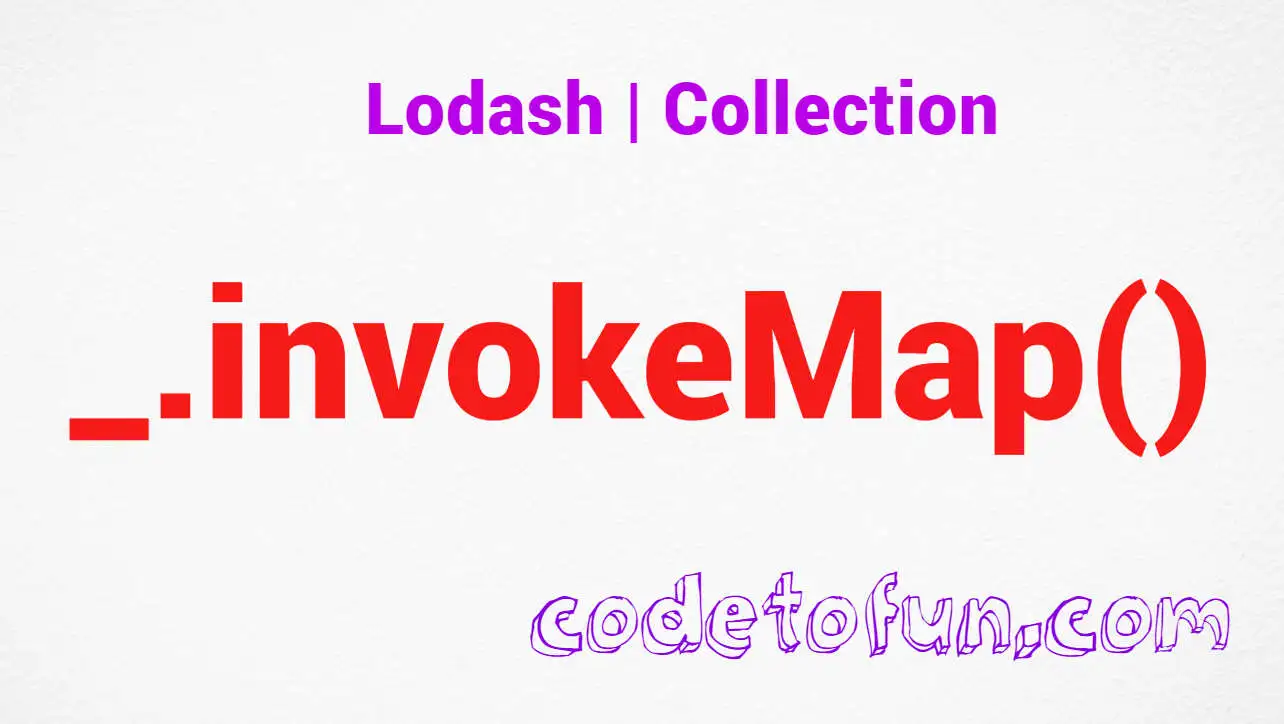
Photo Credit to CodeToFun
🙋 Introduction
When it comes to efficiently manipulating collections in JavaScript, Lodash stands out as a powerful utility library. Among its arsenal of functions, the _.invokeMap()
method is a versatile tool for invoking a specified method on each element of a collection.
This method simplifies code and enhances readability, making it an invaluable asset for developers working with diverse datasets.
🧠 Understanding _.invokeMap()
The _.invokeMap()
method in Lodash is designed for invoking the same method on each element of a collection, whether it's an array or an object. This can be particularly useful when working with a collection of objects and needing to perform a uniform operation on each item.
💡 Syntax
_.invokeMap(collection, path, [args])
- collection: The collection to iterate over.
- path: The path of the method to invoke.
- args (Optional): The arguments to invoke the method with.
📝 Example
Let's delve into a practical example to understand the functionality of _.invokeMap()
:
const _ = require('lodash');
const arrayOfObjects = [
{ name: 'John', age: 30, greet: function(greeting) { return `${greeting}, ${this.name}!`; } },
{ name: 'Alice', age: 25, greet: function(greeting) { return `${greeting}, ${this.name}!`; } },
];
const greetings = _.invokeMap(arrayOfObjects, 'greet', 'Hello');
console.log(greetings);
// Output: ['Hello, John!', 'Hello, Alice!']
In this example, the greet method is invoked on each object in the arrayOfObjects, and the result is an array of greetings.
🏆 Best Practices
Check for Method Existence:
Before applying
_.invokeMap()
, ensure that the specified method exists on each element of the collection. This prevents errors and ensures a smooth execution.example.jsCopiedconst arrayOfObjects = [ { name: 'John', greet: function(greeting) { return `${greeting}, ${this.name}!`; } }, { name: 'Alice' }, ]; const greetings = _.invokeMap(arrayOfObjects, 'greet', 'Hello'); console.log(greetings); // Output: ['Hello, John!', undefined]
Utilize Context:
Leverage the context (this) within the invoked method to access and modify properties of each element in the collection.
example.jsCopiedconst arrayOfObjects = [ { name: 'John', age: 30, greet: function() { return `Hello, ${this.name}! I'm ${this.age} years old.`; } }, { name: 'Alice', age: 25, greet: function() { return `Hello, ${this.name}! I'm ${this.age} years old.`; } }, ]; const greetingsWithAge = _.invokeMap(arrayOfObjects, 'greet'); console.log(greetingsWithAge); /* Output: [ "Hello, John! I'm 30 years old.", "Hello, Alice! I'm 25 years old." ] */
Handle Edge Cases:
Consider potential edge cases, such as empty collections or variations in the structure of objects within the collection. Implement appropriate checks and fallbacks as needed.
example.jsCopiedconst emptyArray = []; const emptyResult = _.invokeMap(emptyArray, 'someMethod'); console.log(emptyResult); // Output: []
📚 Use Cases
Method Invocation on Objects:
_.invokeMap()
is particularly useful when dealing with collections of objects, allowing you to invoke a specific method on each object uniformly.example.jsCopiedconst users = [ { name: 'John', isAdmin: true, activate: function() { this.isActive = true; } }, { name: 'Alice', isAdmin: false, activate: function() { this.isActive = true; } }, ]; _.invokeMap(users, 'activate'); console.log(users); /* Output: [ { name: 'John', isAdmin: true, isActive: true, activate: [Function: activate] }, { name: 'Alice', isAdmin: false, isActive: true, activate: [Function: activate] } ] */
Batch Processing:
When you need to perform a common operation on each element of a collection,
_.invokeMap()
can streamline the process, offering concise and readable code.example.jsCopiedconst tasks = [ { title: 'Task 1', execute: function() { /* ... */ } }, { title: 'Task 2', execute: function() { /* ... */ } }, // ... ]; _.invokeMap(tasks, 'execute');
Dynamic Method Invocation:
By dynamically specifying the method to invoke,
_.invokeMap()
allows for flexibility in code execution based on runtime conditions.example.jsCopiedconst shapes = [ { type: 'circle', calculateArea: function() { /* ... */ } }, { type: 'rectangle', calculateArea: function() { /* ... */ } }, // ... ]; const areaResults = _.invokeMap(shapes, 'calculateArea'); console.log(areaResults); /* Output: [ /* result of calculateArea for circle */, /* result of calculateArea for rectangle */, // ... ] */
🎉 Conclusion
The _.invokeMap()
method in Lodash provides a powerful and efficient way to invoke a specified method on each element of a collection. Whether you're working with arrays of objects or need to perform batch operations, this method streamlines your code and enhances readability.
Embrace the capabilities of _.invokeMap()
and elevate your collection manipulation in JavaScript!
👨💻 Join our Community:
Author
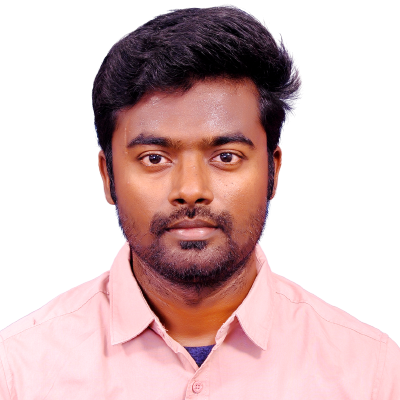
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
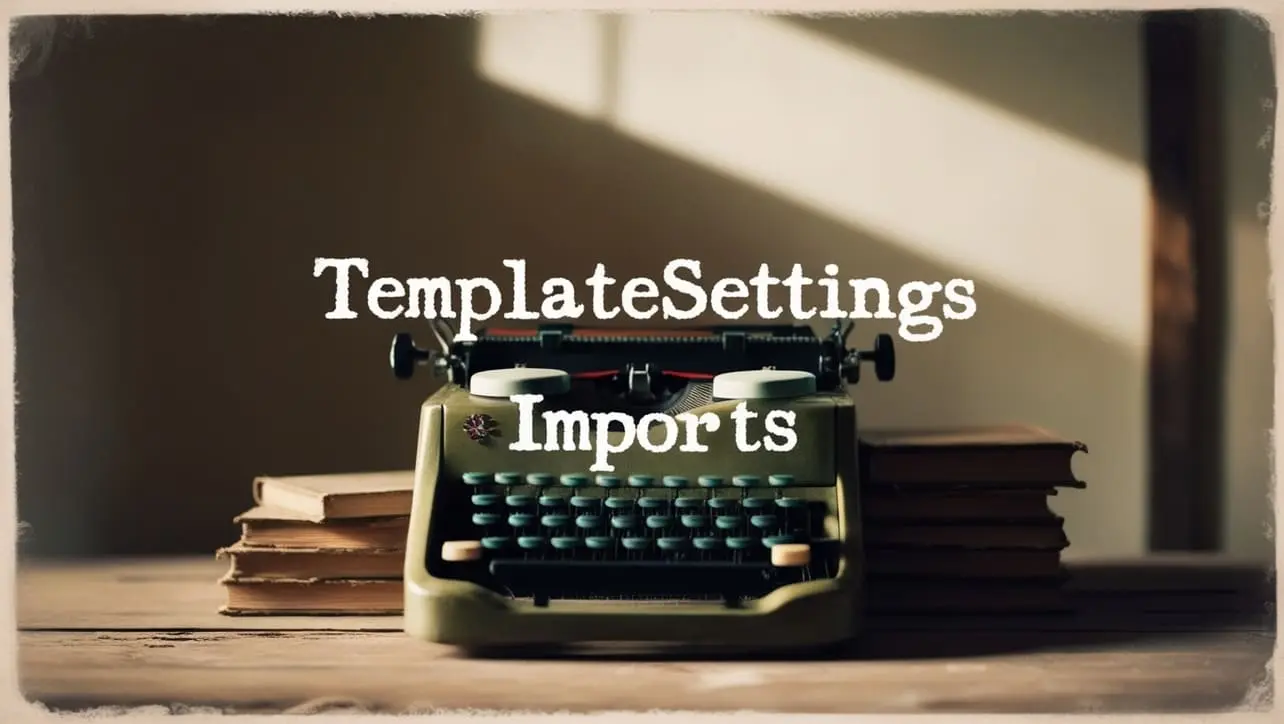
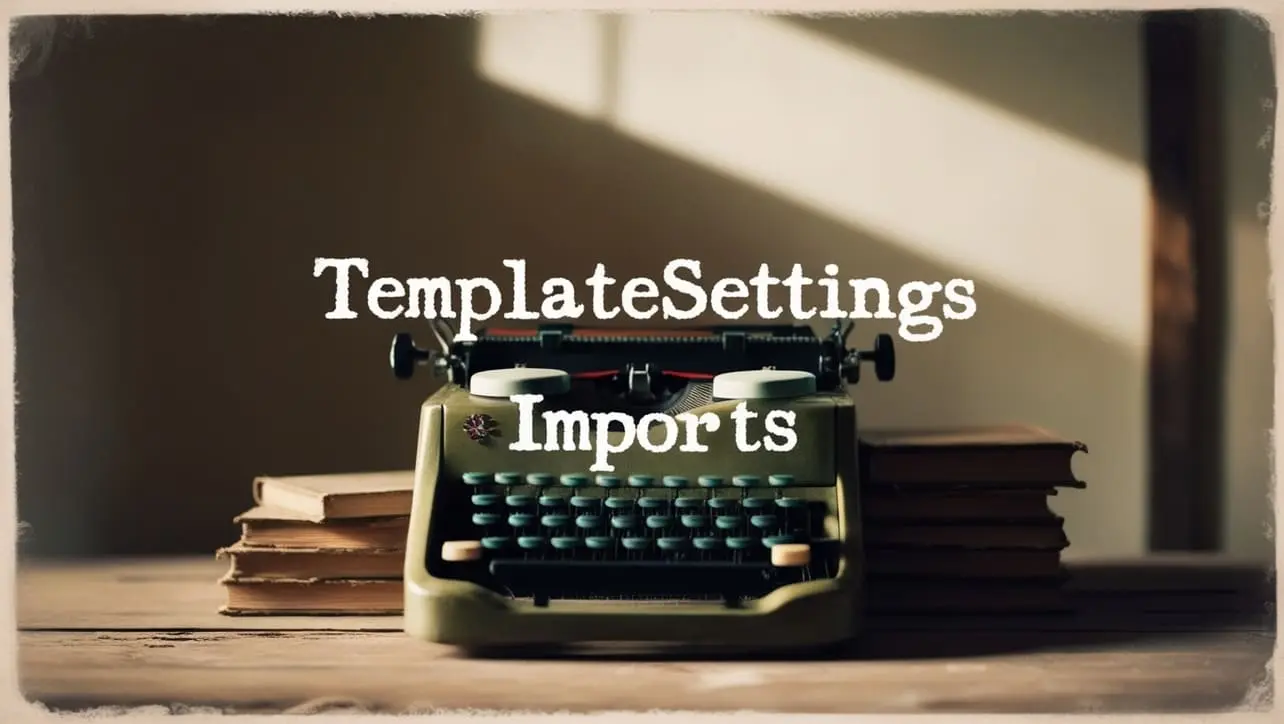
Lodash _.templateSettings.imports Property
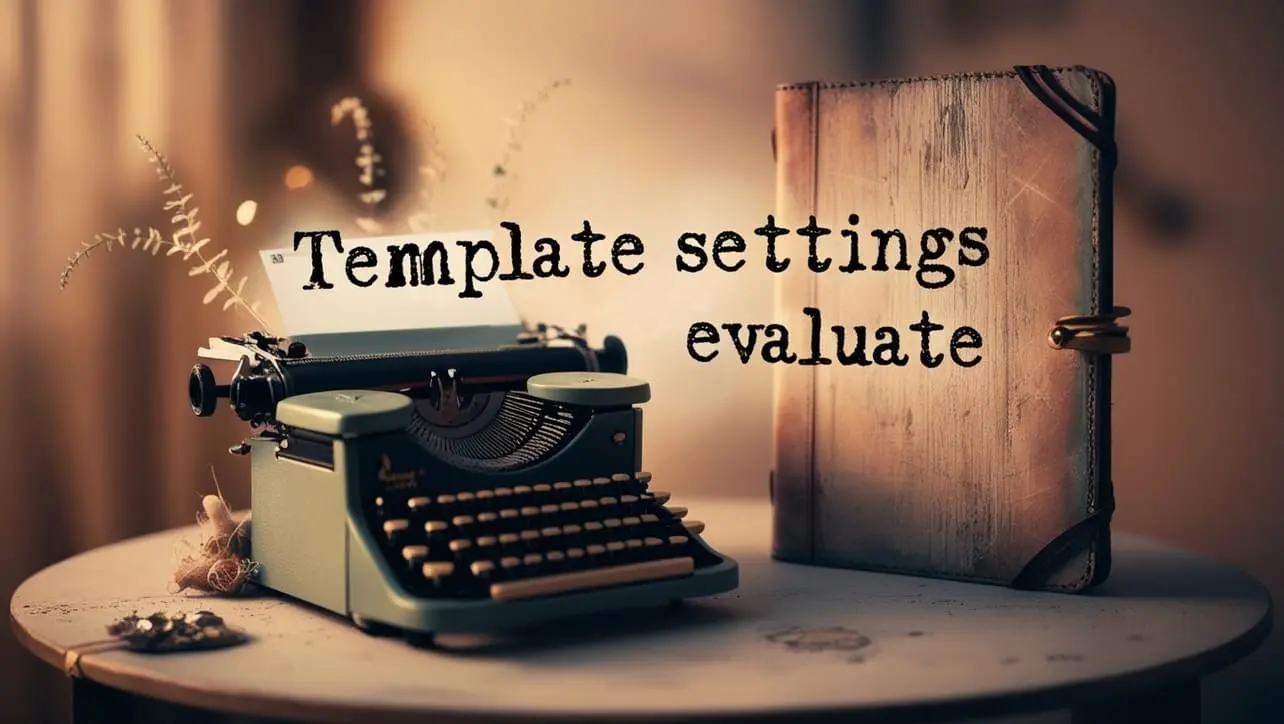
Lodash _.templateSettings.evaluate Property
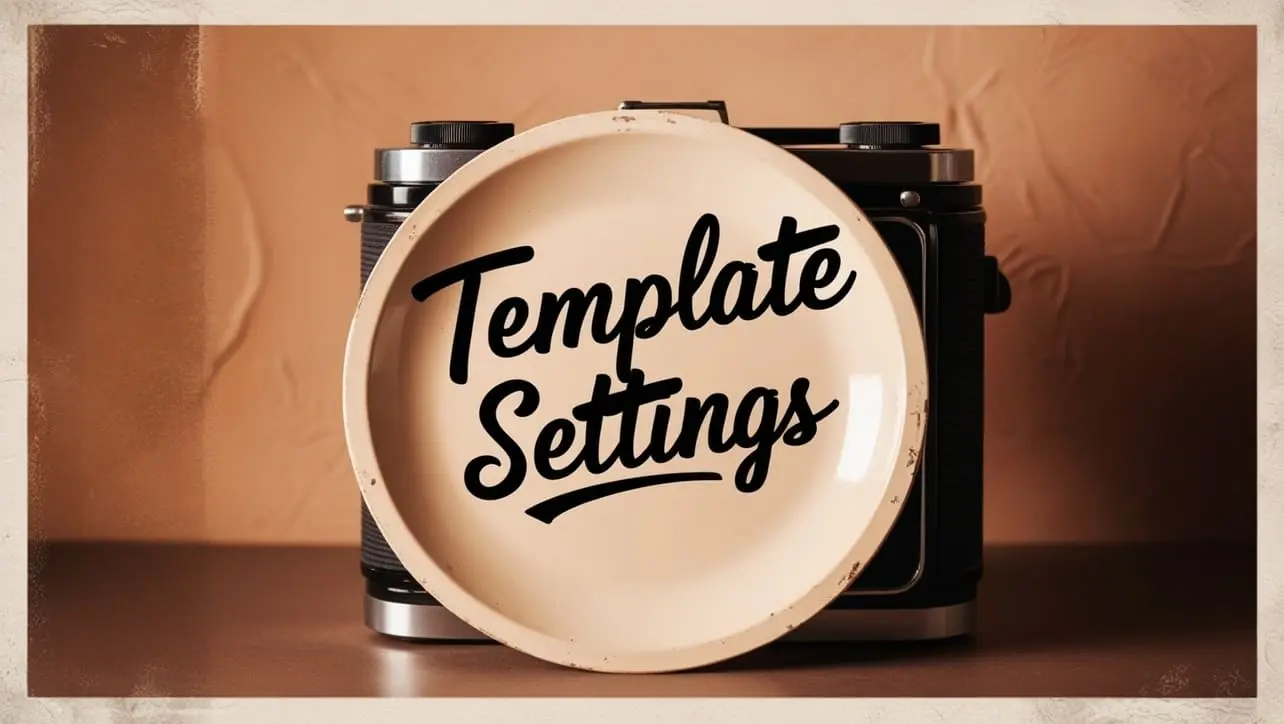
Lodash _.templateSettings Property
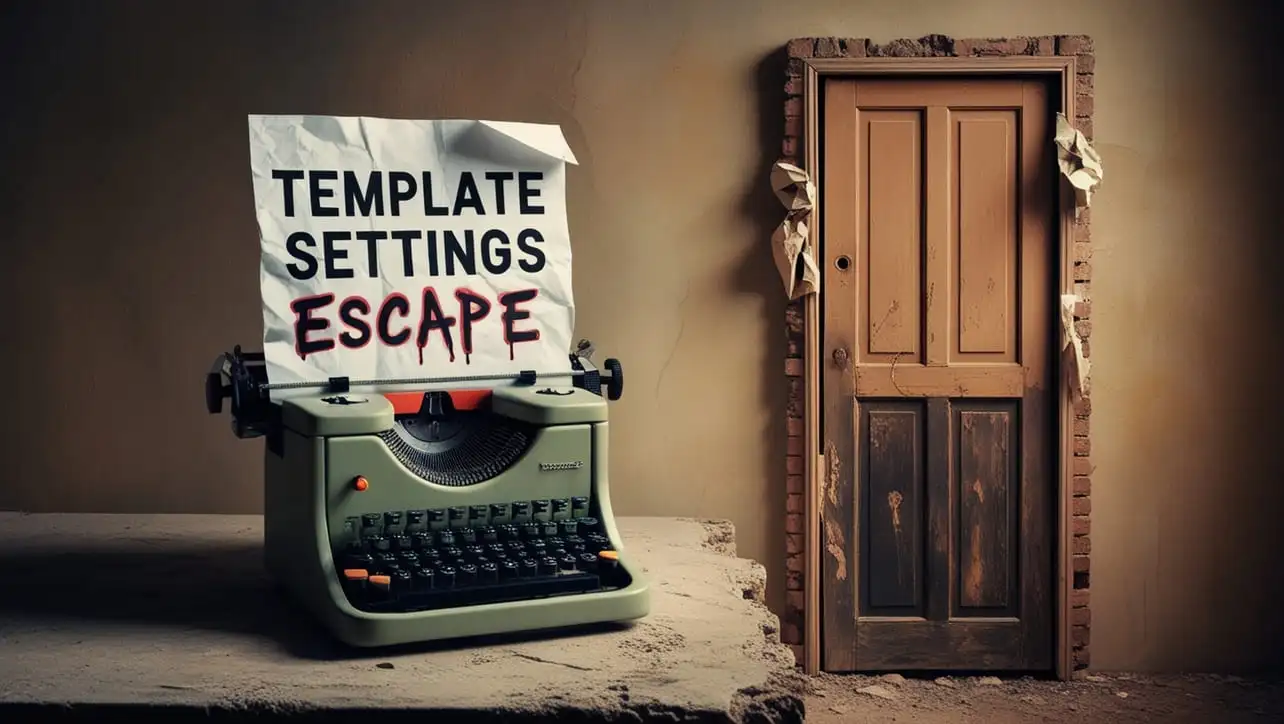
Lodash _.templateSettings.escape Property
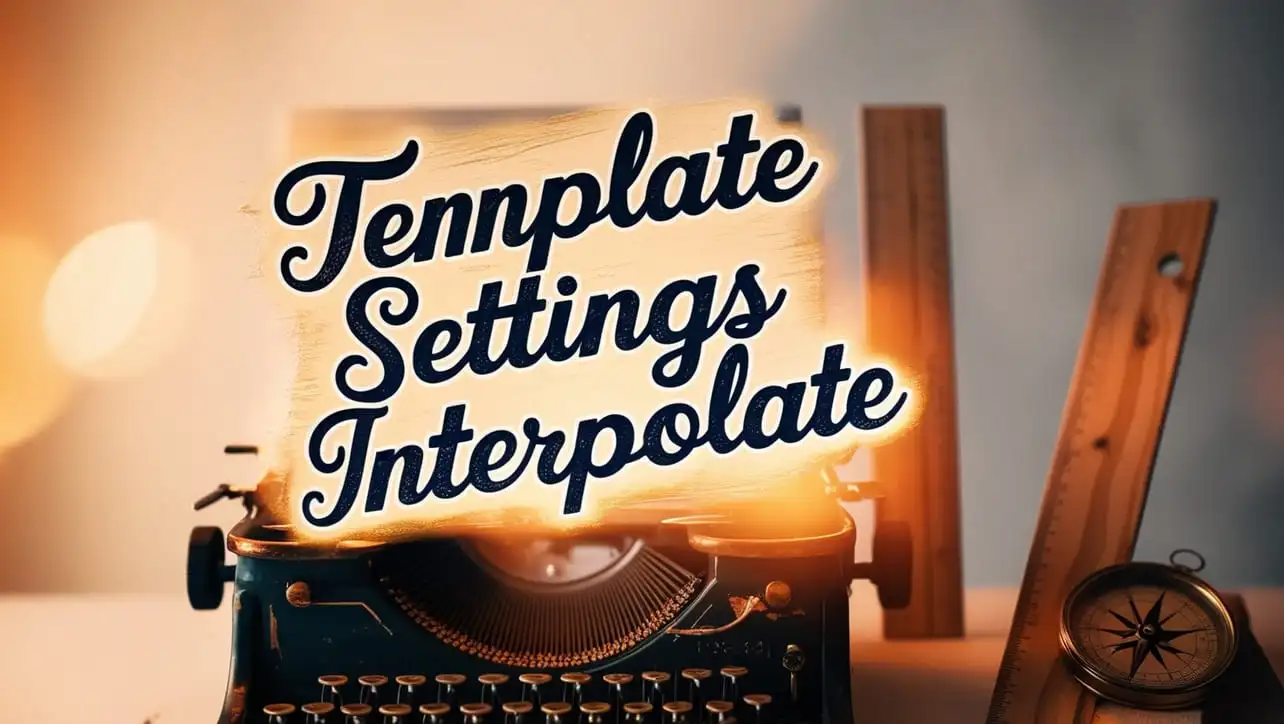
Lodash _.templateSettings.interpolate Property
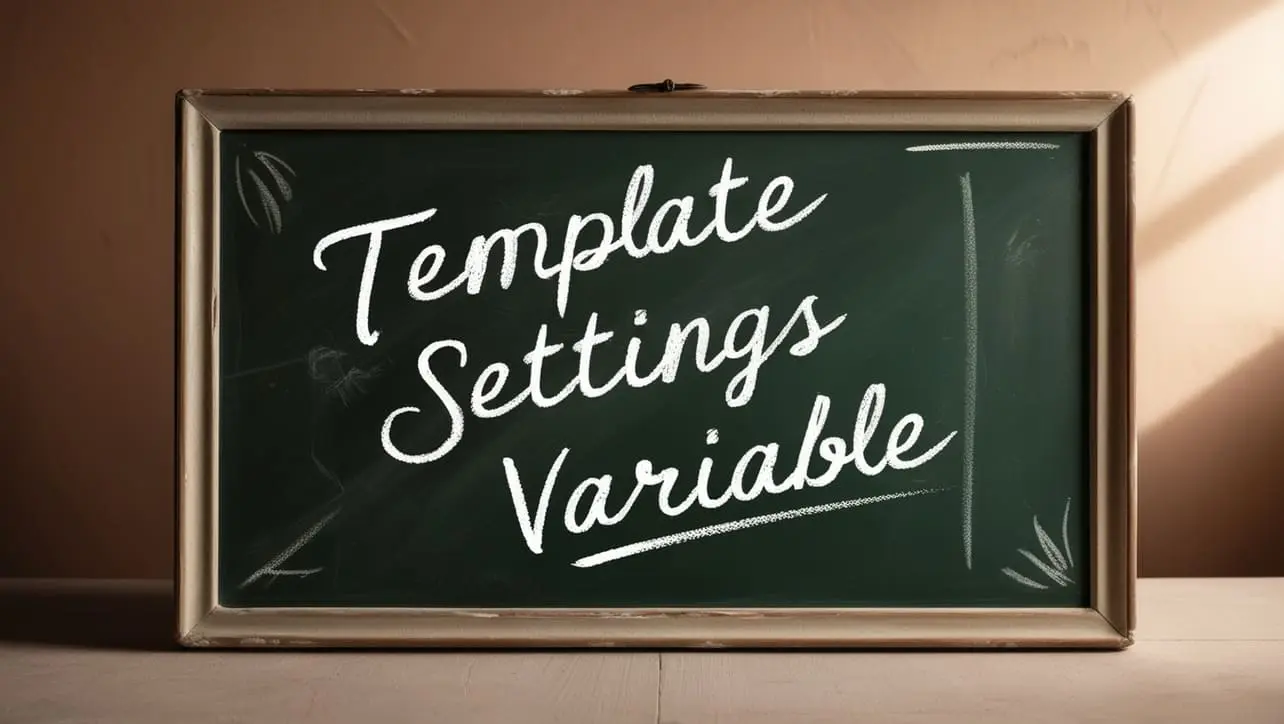
If you have any doubts regarding this article (Lodash _.invokeMap() Collection Method), please comment here. I will help you immediately.