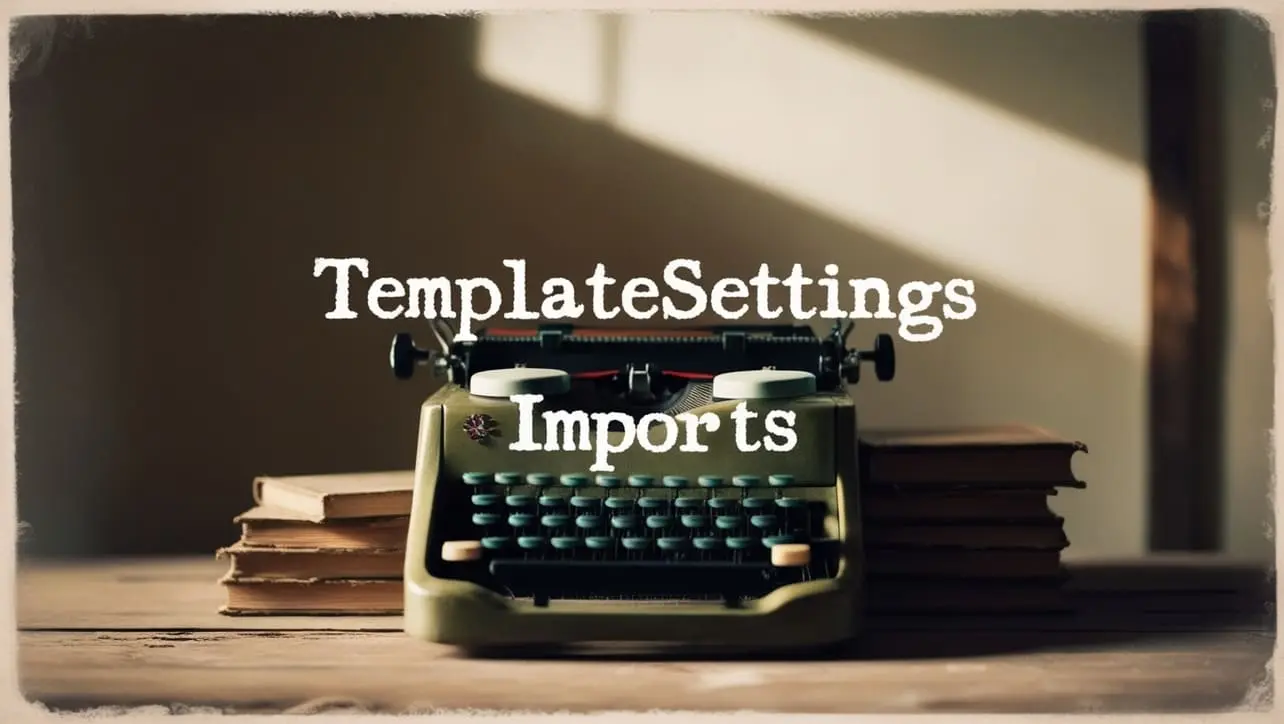
Lodash _.includes() Collection Method
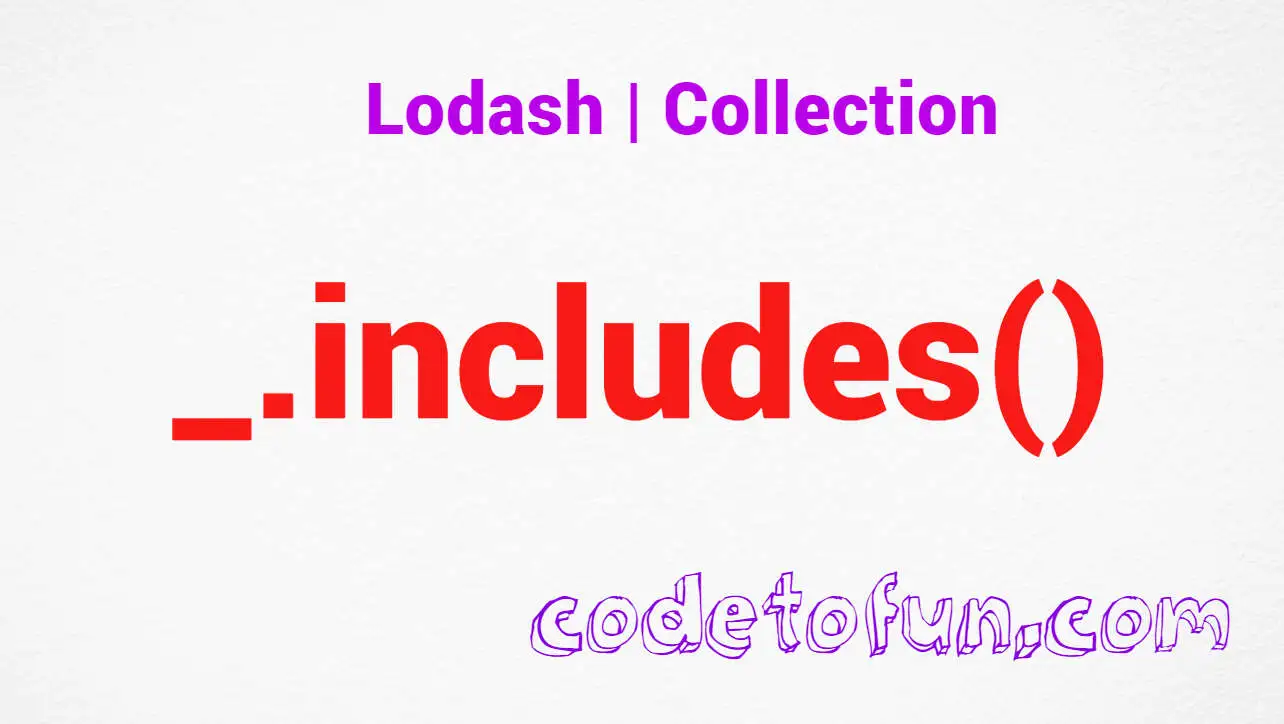
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript programming, efficiently searching for elements in collections is a common requirement. Lodash, a powerful utility library, provides the _.includes()
method to simplify this task.
Whether you are working with arrays or objects, this method proves to be a valuable tool for checking the presence of a specified value within a collection, offering enhanced control and readability to your code.
🧠 Understanding _.includes()
The _.includes()
method in Lodash is designed to determine if a given value exists within a collection, such as an array or an object. It provides a straightforward way to perform inclusion checks, helping developers write more expressive and concise code.
💡 Syntax
_.includes(collection, value, [fromIndex=0])
- collection: The collection to search.
- value: The value to check for inclusion.
- fromIndex (Optional): The index to start the search from.
📝 Example
Let's explore a simple example to illustrate the functionality of _.includes()
:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const includesThree = _.includes(numbers, 3);
console.log(includesThree);
// Output: true
In this example, the numbers array is searched for the value 3 using _.includes()
, which returns true as the value is present in the array.
🏆 Best Practices
Understanding fromIndex:
Take advantage of the fromIndex parameter to control where the search starts within the collection. This can be useful when looking for multiple occurrences or when skipping elements at the beginning of the collection.
example.jsCopiedconst fruits = ['apple', 'orange', 'banana', 'apple', 'kiwi']; const includesAppleFromIndex2 = _.includes(fruits, 'apple', 2); console.log(includesAppleFromIndex2); // Output: true
Handle NaN Values:
Be aware that
_.includes()
performs strict equality checks. When working with collections that may contain NaN (Not a Number) values, ensure you handle them appropriately.example.jsCopiedconst values = [1, 2, NaN, 4, 5]; const includesNaN = _.includes(values, NaN); console.log(includesNaN); // Output: true
Check for Object Values:
_.includes()
is not limited to arrays; it can also be applied to objects. However, keep in mind that it checks for the presence of values, not keys.example.jsCopiedconst person = { name: 'John', age: 30, city: 'New York' }; const includesAge30 = _.includes(person, 30); console.log(includesAge30); // Output: true
📚 Use Cases
Validation in Forms:
Use
_.includes()
to check if a user's input matches predefined options in forms, providing instant feedback to users.example.jsCopiedconst validColors = ['red', 'blue', 'green']; const userInput = /* ...get user input... */; if (_.includes(validColors, userInput)) { console.log('Valid color!'); } else { console.log('Invalid color!'); }
Filtering Data:
Employ
_.includes()
in filtering scenarios, where you want to select elements based on whether they include certain values.example.jsCopiedconst data = /* ...array of objects... */; const selectedData = data.filter(item => _.includes(item.tags, 'important')); console.log(selectedData);
Search Functionality:
Implement search functionality in your applications by utilizing
_.includes()
to check if the entered search term is present in your dataset.example.jsCopiedconst searchQuery = /* ...get user's search query... */; const products = /* ...array of product objects... */; const searchResults = products.filter(product => _.includes(product.name.toLowerCase(), searchQuery.toLowerCase())); console.log(searchResults);
🎉 Conclusion
The _.includes()
method in Lodash is a versatile tool for checking the presence of a value within a collection, providing developers with a simple and efficient solution. Whether you are performing validation, filtering data, or implementing search functionality, _.includes()
offers a straightforward approach to inclusion checks in JavaScript.
Incorporate _.includes()
into your code and elevate the way you handle collections in your JavaScript applications!
👨💻 Join our Community:
Author
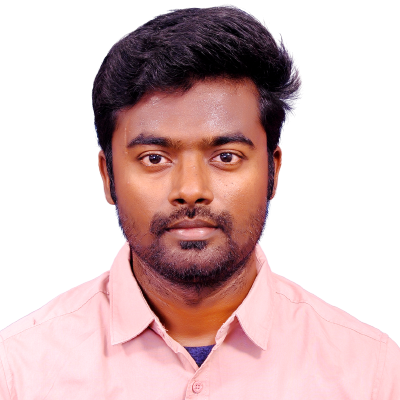
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
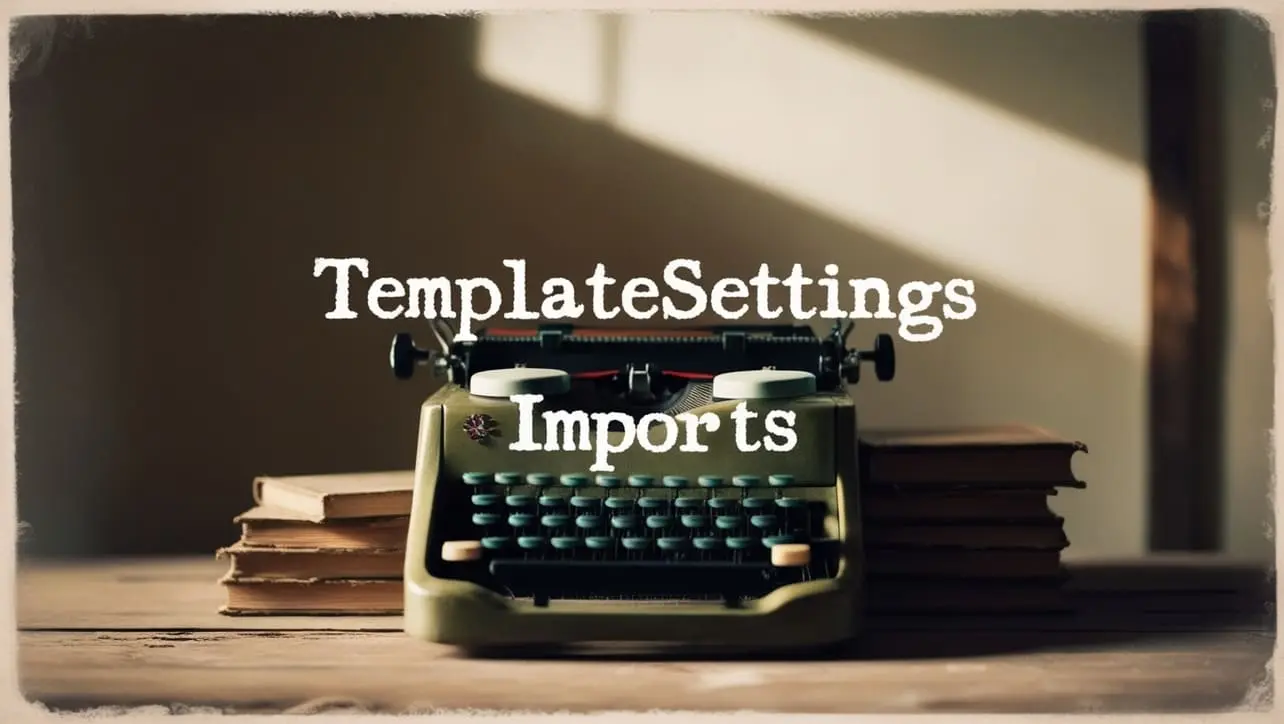
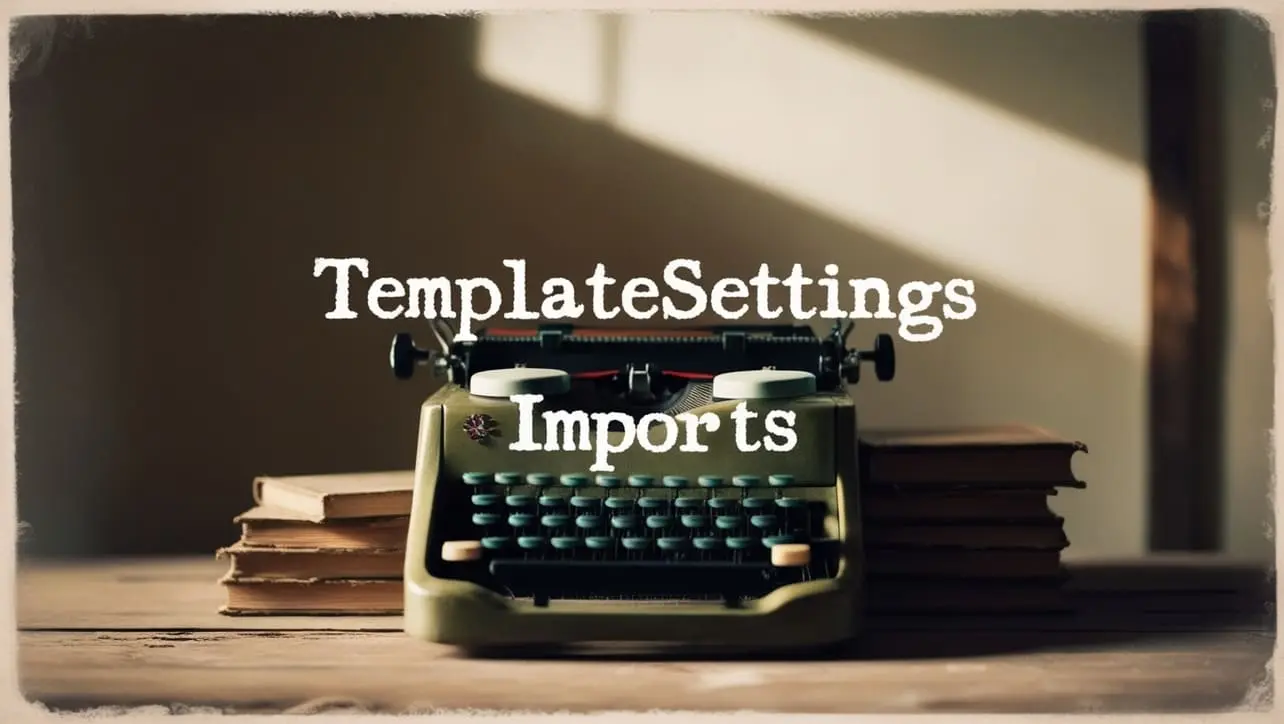
Lodash _.templateSettings.imports Property
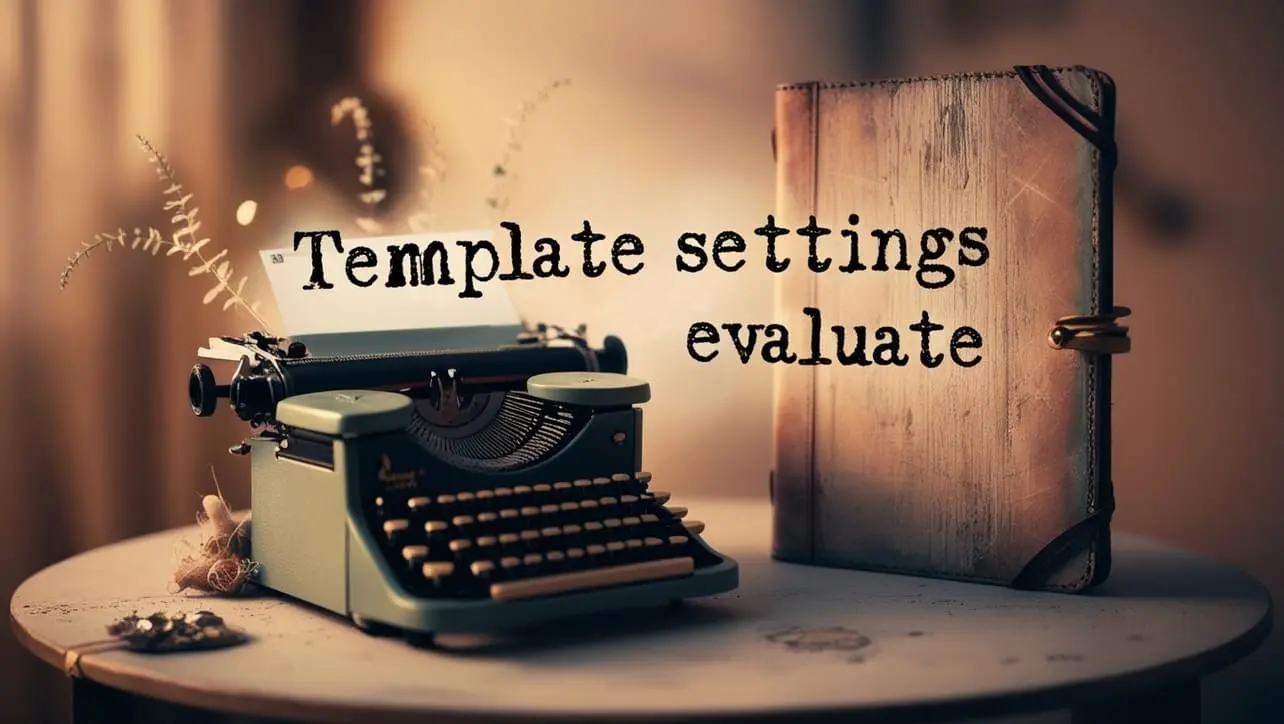
Lodash _.templateSettings.evaluate Property
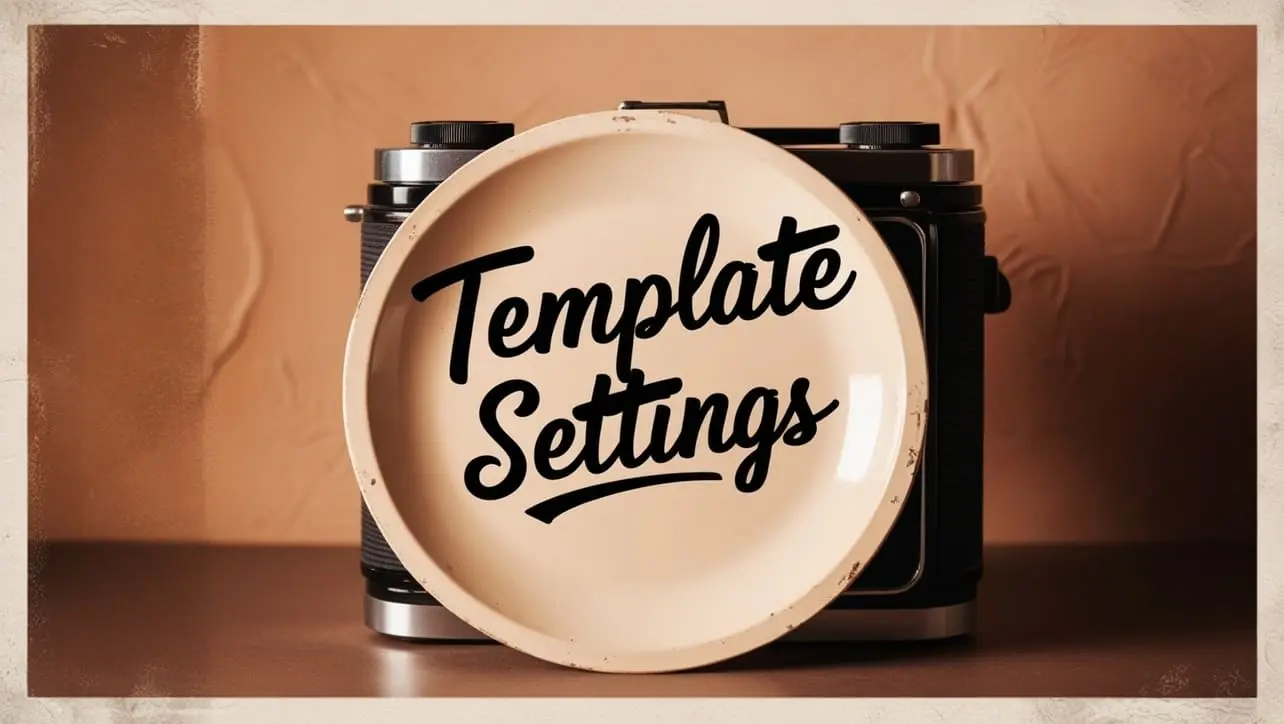
Lodash _.templateSettings Property
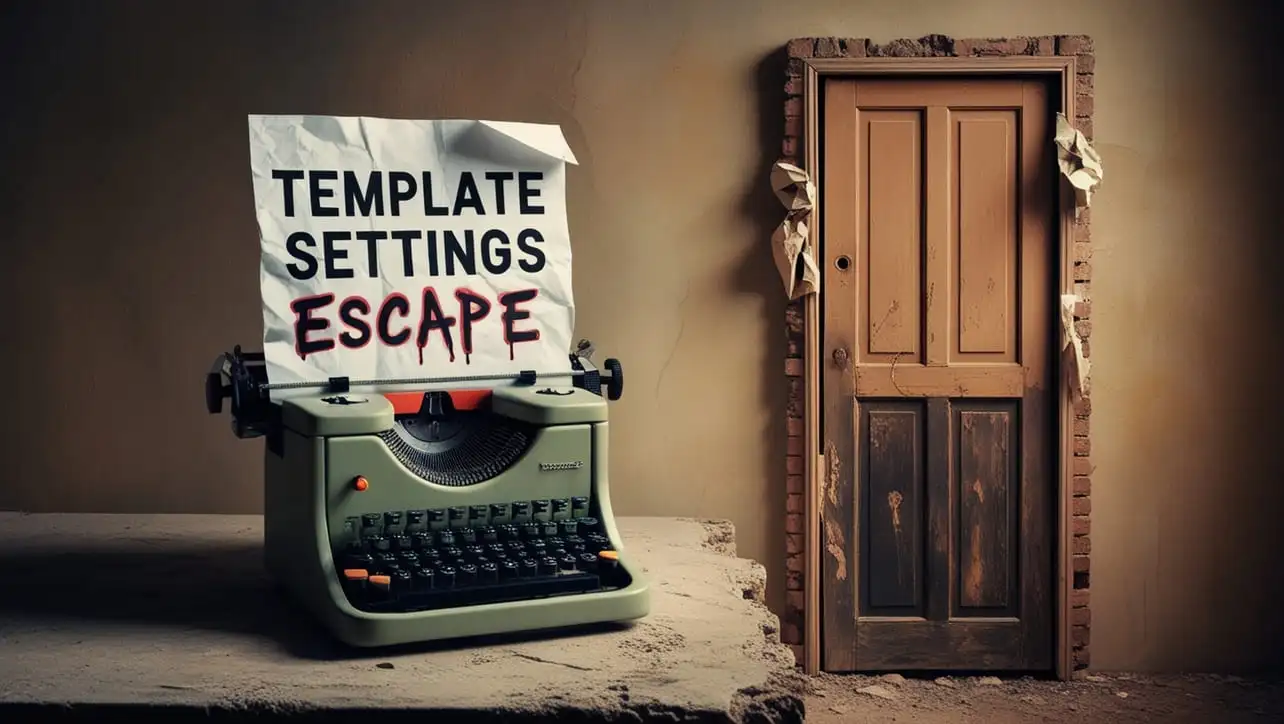
Lodash _.templateSettings.escape Property
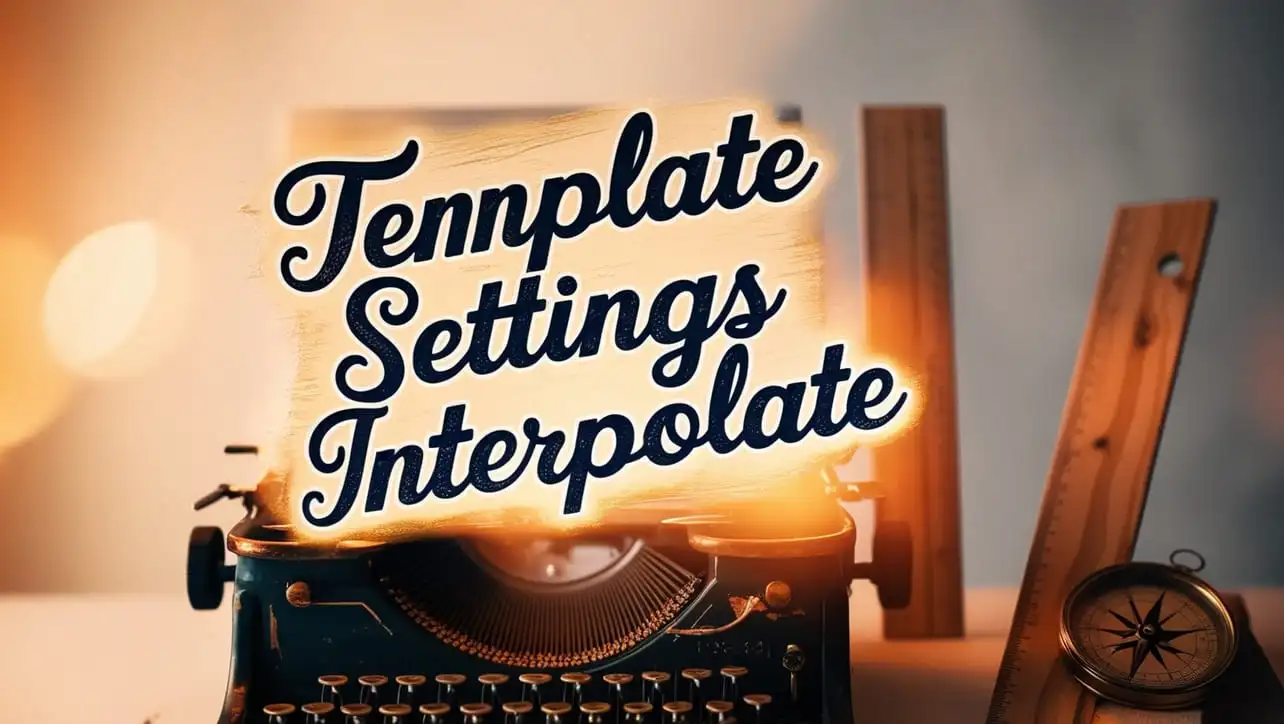
Lodash _.templateSettings.interpolate Property
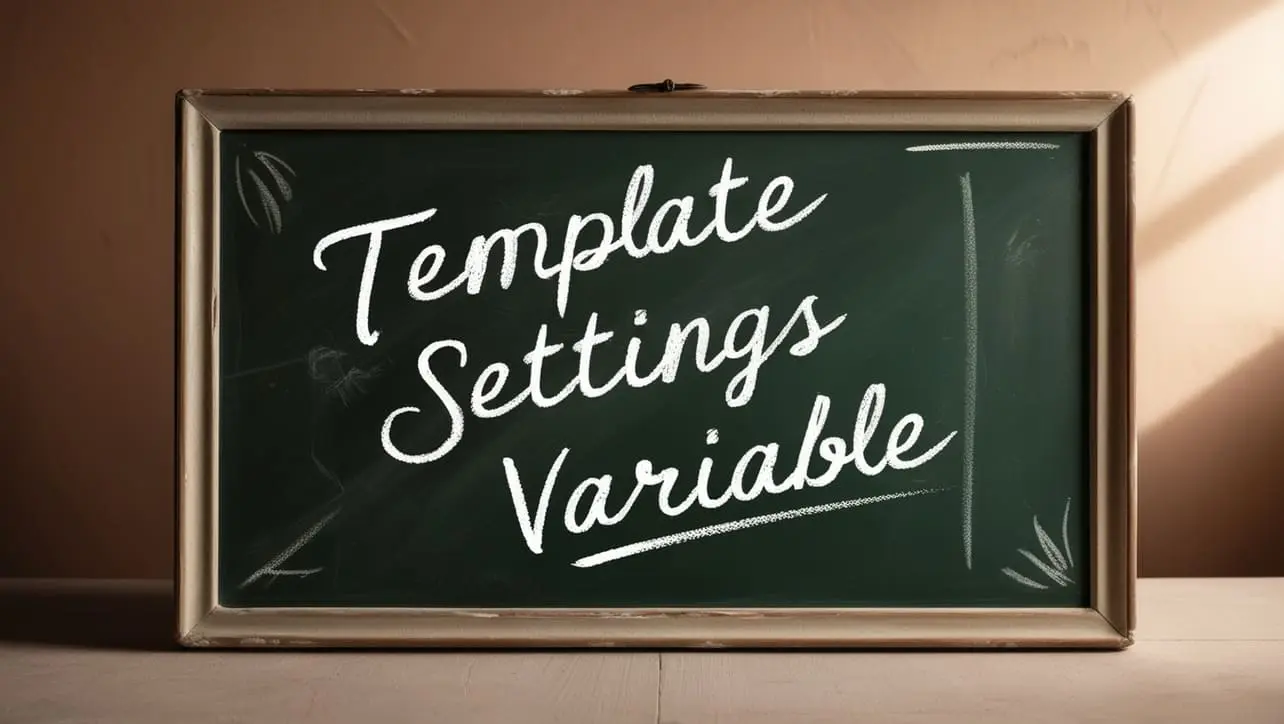
If you have any doubts regarding this article (Lodash _.includes() Collection Method), please comment here. I will help you immediately.