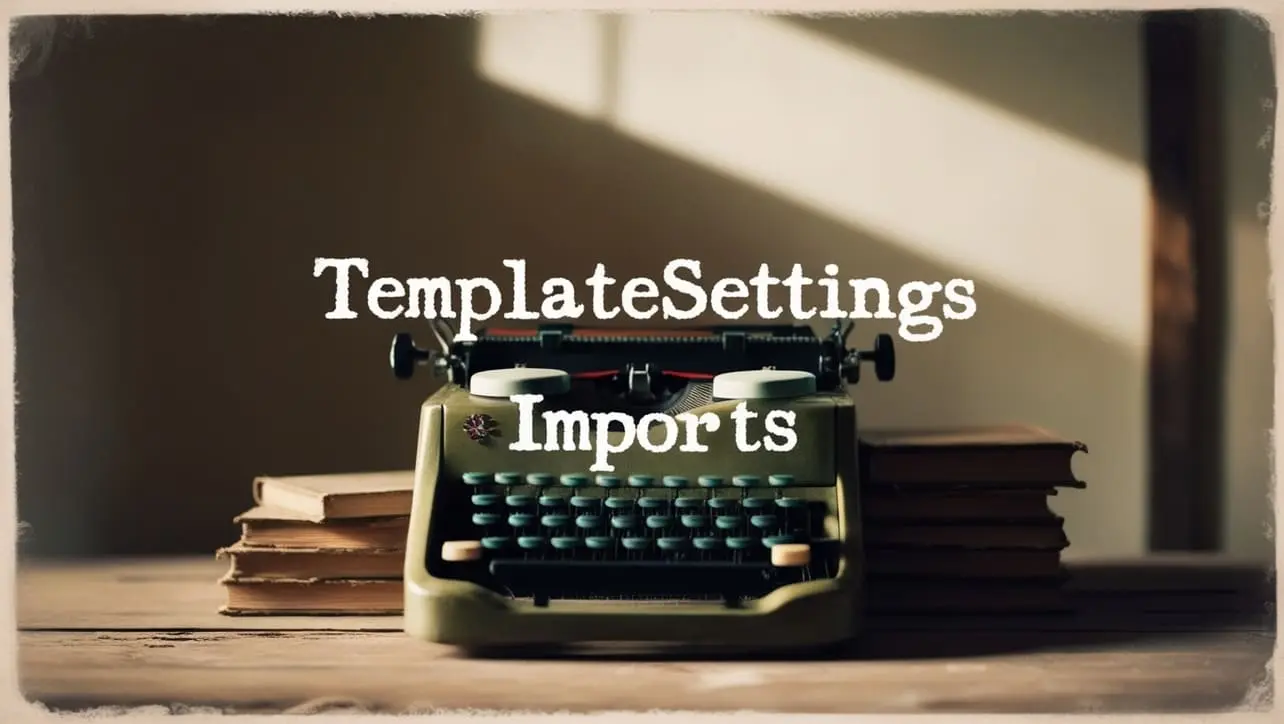
Lodash _.find() Collection Method
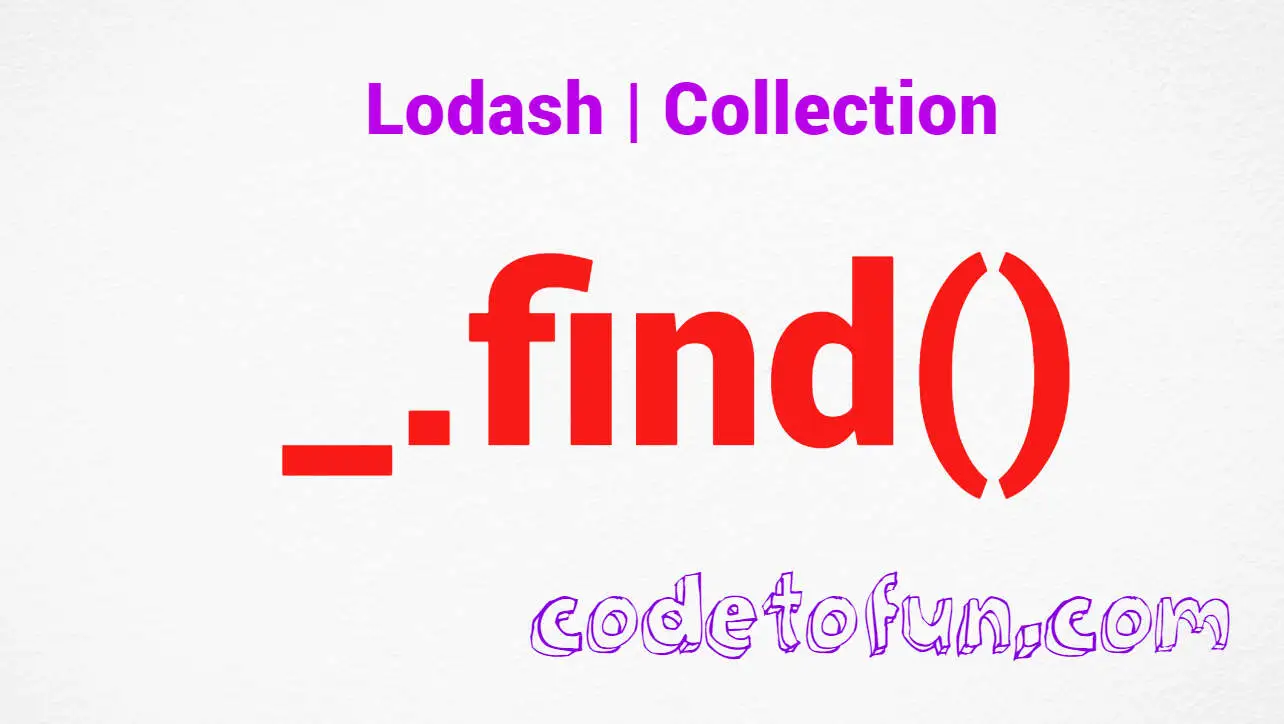
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, working with collections is a common task. Lodash, a powerful utility library, provides a variety of methods to simplify collection manipulation. One such essential function is the _.find()
method. This method proves invaluable when searching through arrays or objects to locate the first element that satisfies a given condition.
Join us as we explore the versatility and utility of _.find()
in enhancing your JavaScript development experience.
🧠 Understanding _.find()
The _.find()
method in Lodash is designed to locate the first element in a collection (array or object) that satisfies a specified condition. This method streamlines the process of searching through data structures, offering a clean and efficient solution.
💡 Syntax
_.find(collection, [predicate=_.identity], [fromIndex=0])
- collection: The collection to iterate over.
- predicate (Optional): The function invoked per iteration.
- fromIndex (Optional): The index to start searching from.
📝 Example
Let's delve into a practical example to understand how _.find()
works:
const _ = require('lodash');
const users = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Alice', age: 30 },
{ id: 3, name: 'Bob', age: 22 },
];
const foundUser = _.find(users, user => user.age > 25);
console.log(foundUser);
// Output: { id: 2, name: 'Alice', age: 30 }
In this example, _.find()
is used to locate the first user in the array whose age is greater than 25.
🏆 Best Practices
Define a Clear Predicate:
When using
_.find()
, ensure the predicate function clearly defines the condition you're searching for. This helps maintain code clarity and avoids unexpected results.example.jsCopiedconst usersWithSpecificName = _.find(users, { name: 'Alice' }); console.log(usersWithSpecificName); // Output: { id: 2, name: 'Alice', age: 30 }
Handle No Match:
Consider handling scenarios where no match is found. Check the result of
_.find()
and implement appropriate actions for cases where the predicate doesn't match any elements.example.jsCopiedconst notFoundMessage = 'No user found with age greater than 35'; const foundUser = _.find(users, user => user.age > 35); if (foundUser) { console.log(foundUser); } else { console.log(notFoundMessage); }
Utilize fromIndex:
Take advantage of the fromIndex parameter to specify the starting index for the search. This can be useful when searching a portion of the collection.
example.jsCopiedconst startingIndex = 1; const secondUserOnwards = _.find(users, user => user.age > 25, startingIndex); console.log(secondUserOnwards); // Output: { id: 2, name: 'Alice', age: 30 }
📚 Use Cases
User Authentication:
In scenarios like user authentication,
_.find()
can be used to locate a user by their username or email address in a collection of users.example.jsCopiedconst users = /* ...fetch users from database... */; const userInput = /* ...get user input (username or email)... */; const authenticatedUser = _.find(users, user => user.username === userInput || user.email === userInput); console.log(authenticatedUser);
Filtering Data:
When working with datasets,
_.find()
is handy for filtering data based on specific criteria, returning the first element that satisfies the condition.example.jsCopiedconst products = /* ...fetch products from API... */; const desiredProduct = _.find(products, product => product.price < 50); console.log(desiredProduct);
Configurations and Options:
In settings or configuration objects,
_.find()
can be employed to locate the option or configuration that matches a certain criterion.example.jsCopiedconst configurations = /* ...retrieve configurations... */; const desiredConfig = _.find(configurations, config => config.isActive); console.log(desiredConfig);
🎉 Conclusion
The _.find()
method in Lodash provides a powerful and efficient way to locate the first element in a collection that meets a specified condition. Whether you're searching for users in an authentication system, filtering data, or finding configurations, _.find()
proves to be a versatile tool for collection manipulation in JavaScript.
Explore the capabilities of _.find()
and elevate your JavaScript development experience by efficiently searching through collections with ease!
👨💻 Join our Community:
Author
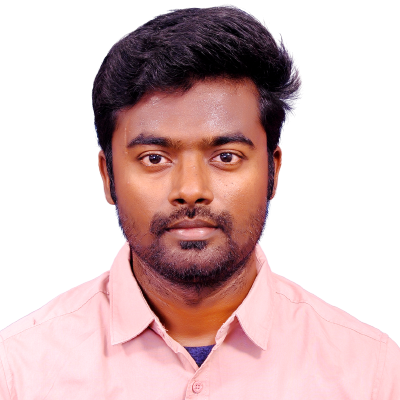
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
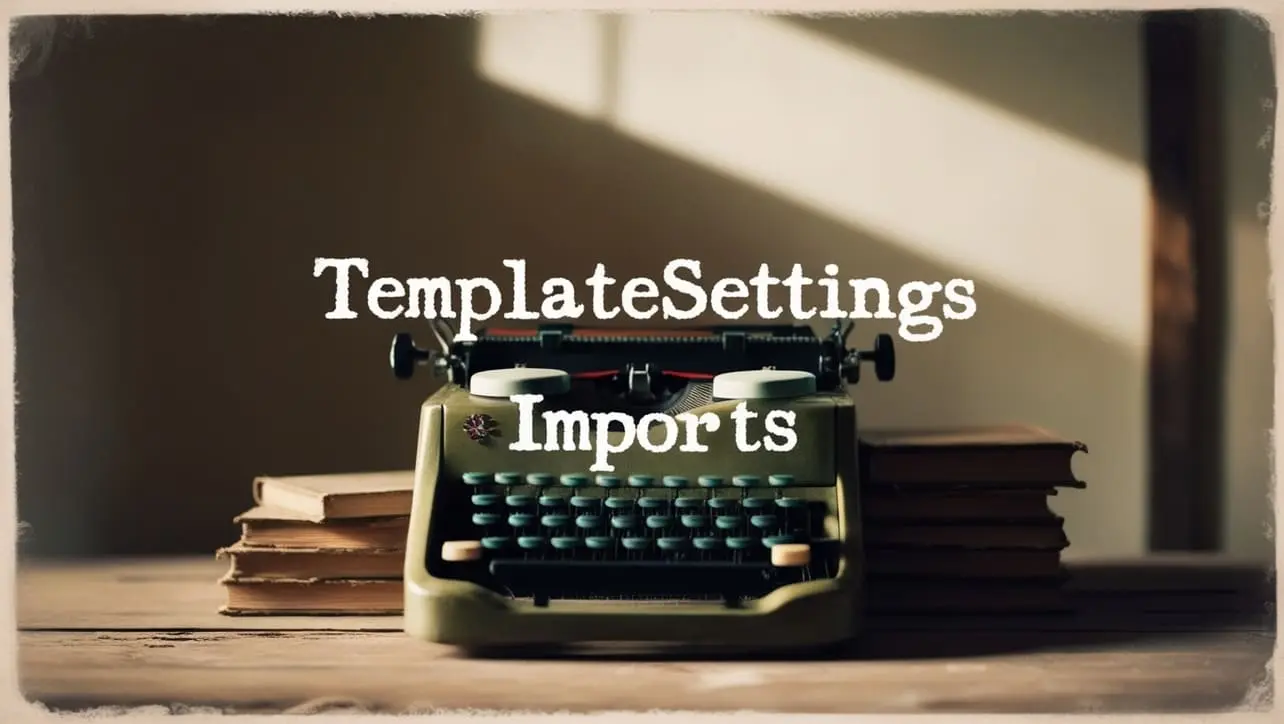
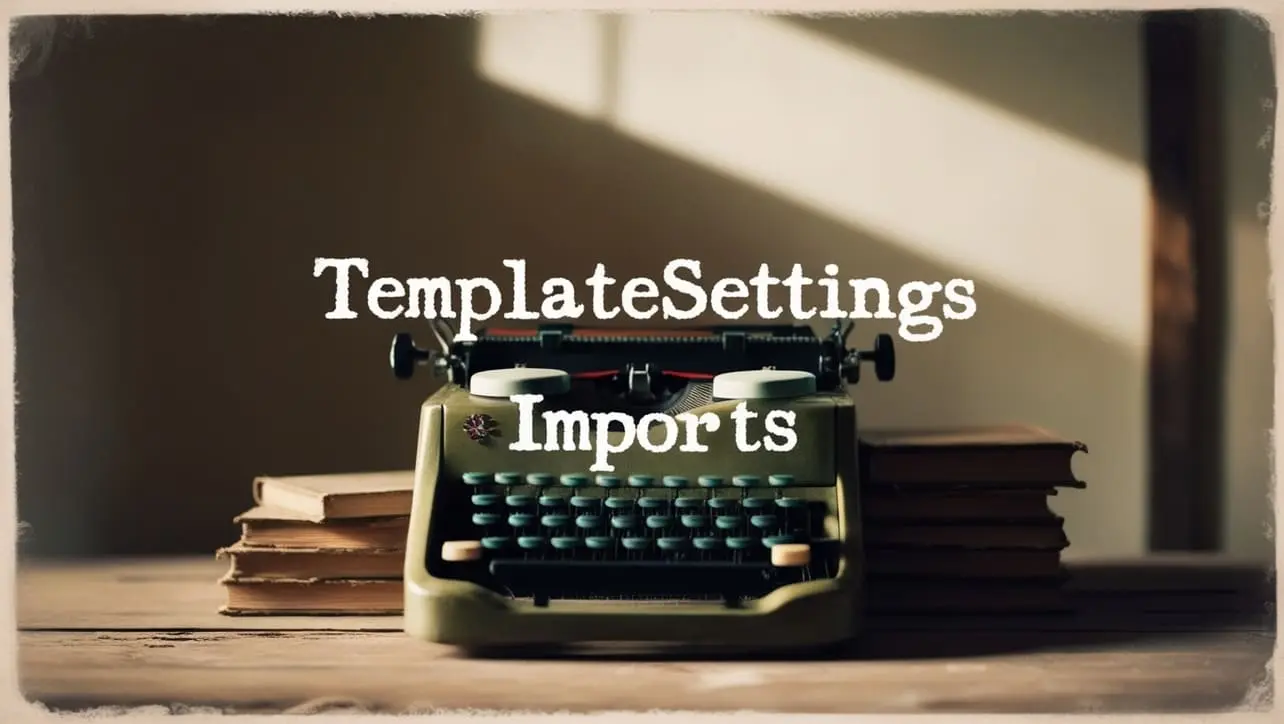
Lodash _.templateSettings.imports Property
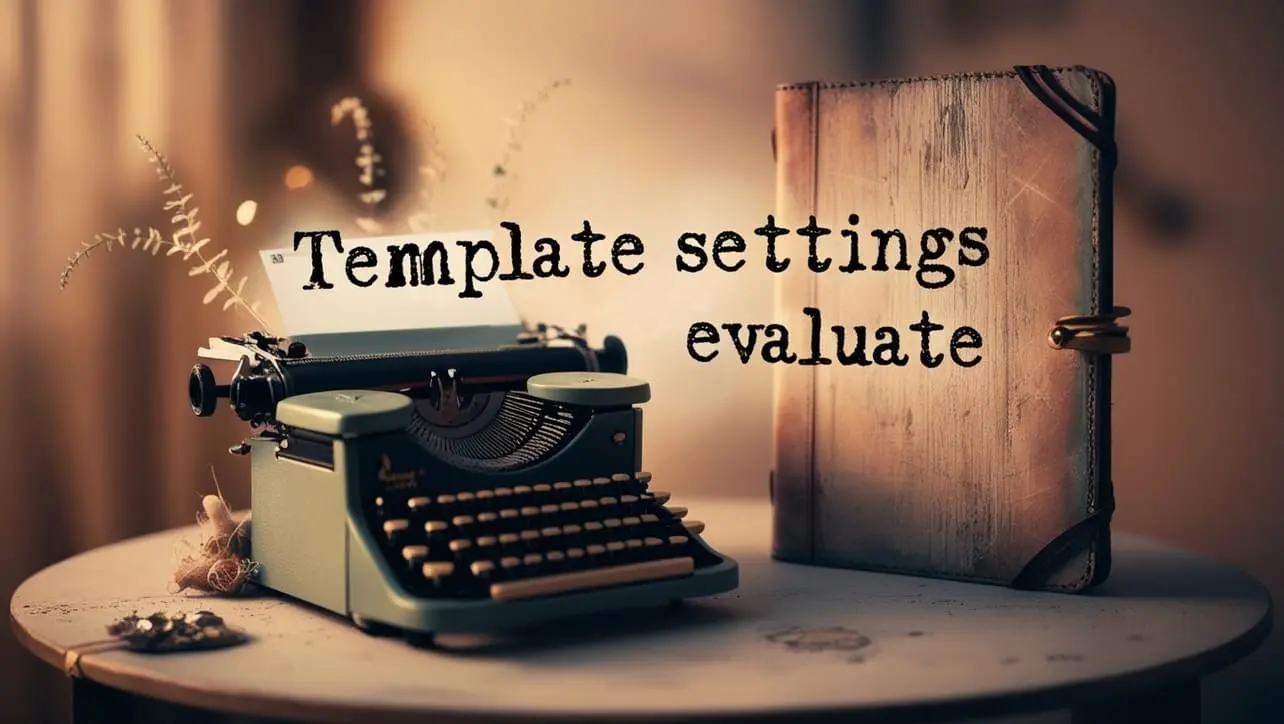
Lodash _.templateSettings.evaluate Property
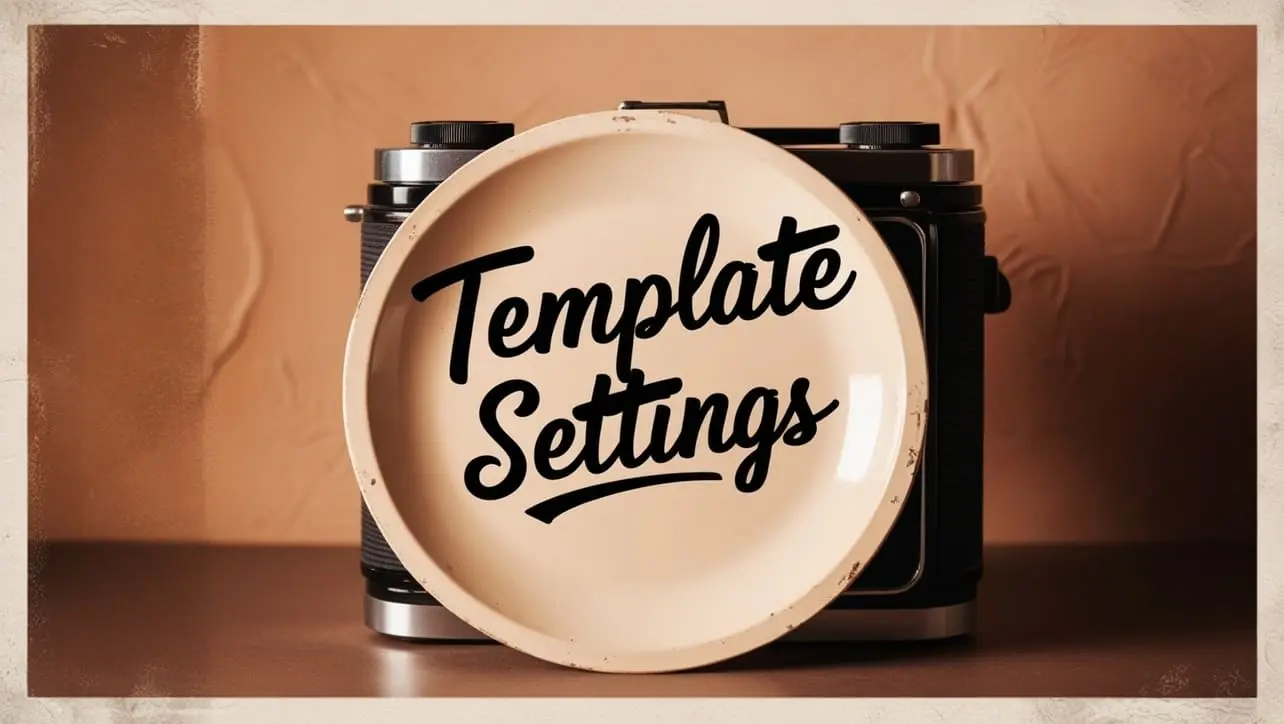
Lodash _.templateSettings Property
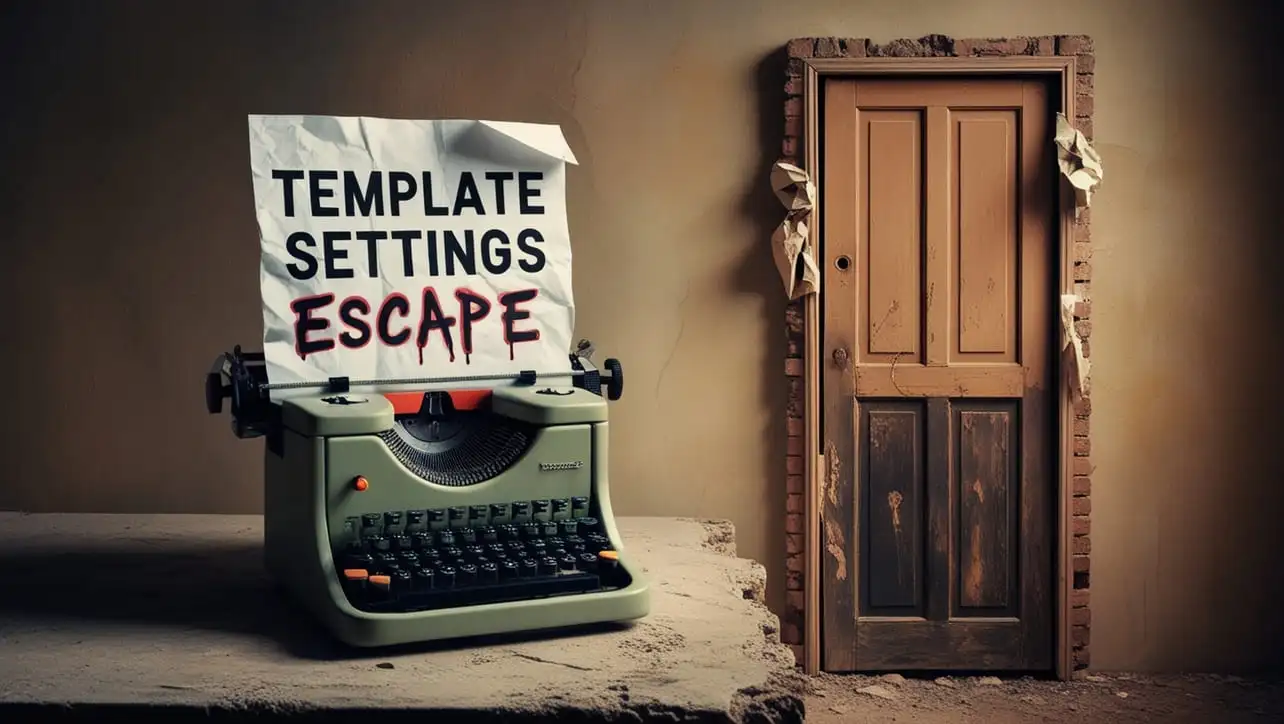
Lodash _.templateSettings.escape Property
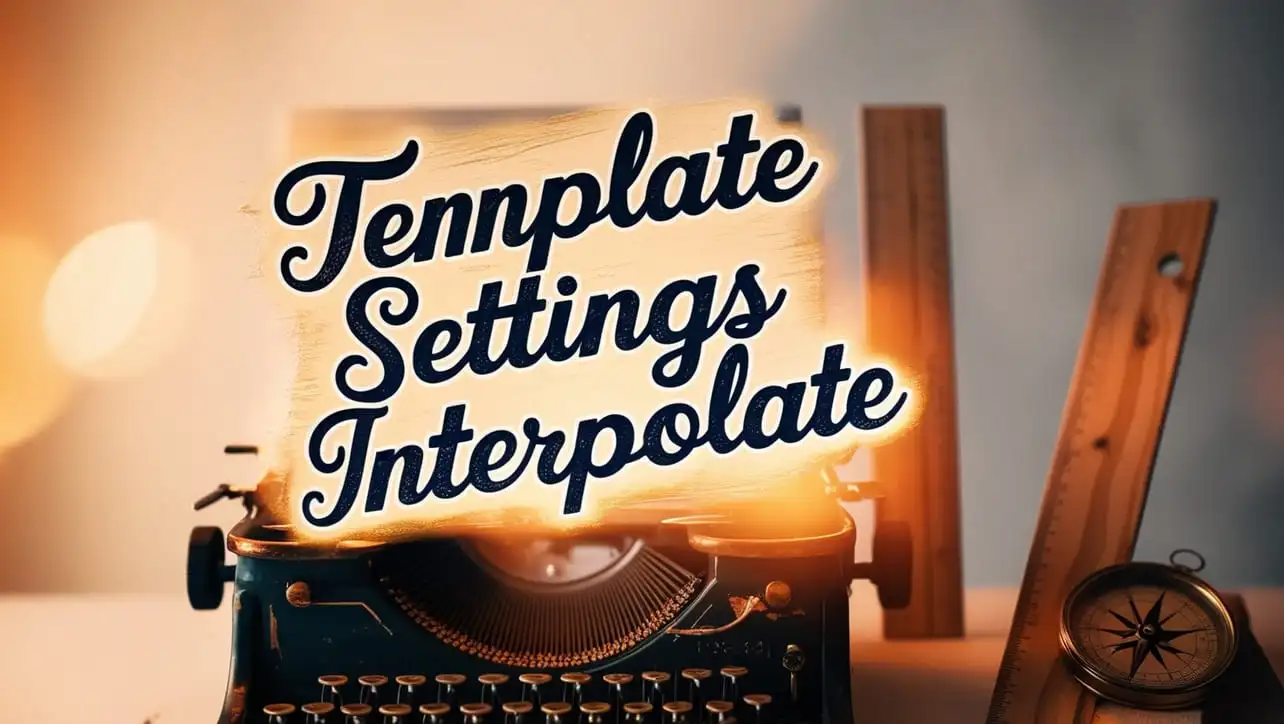
Lodash _.templateSettings.interpolate Property
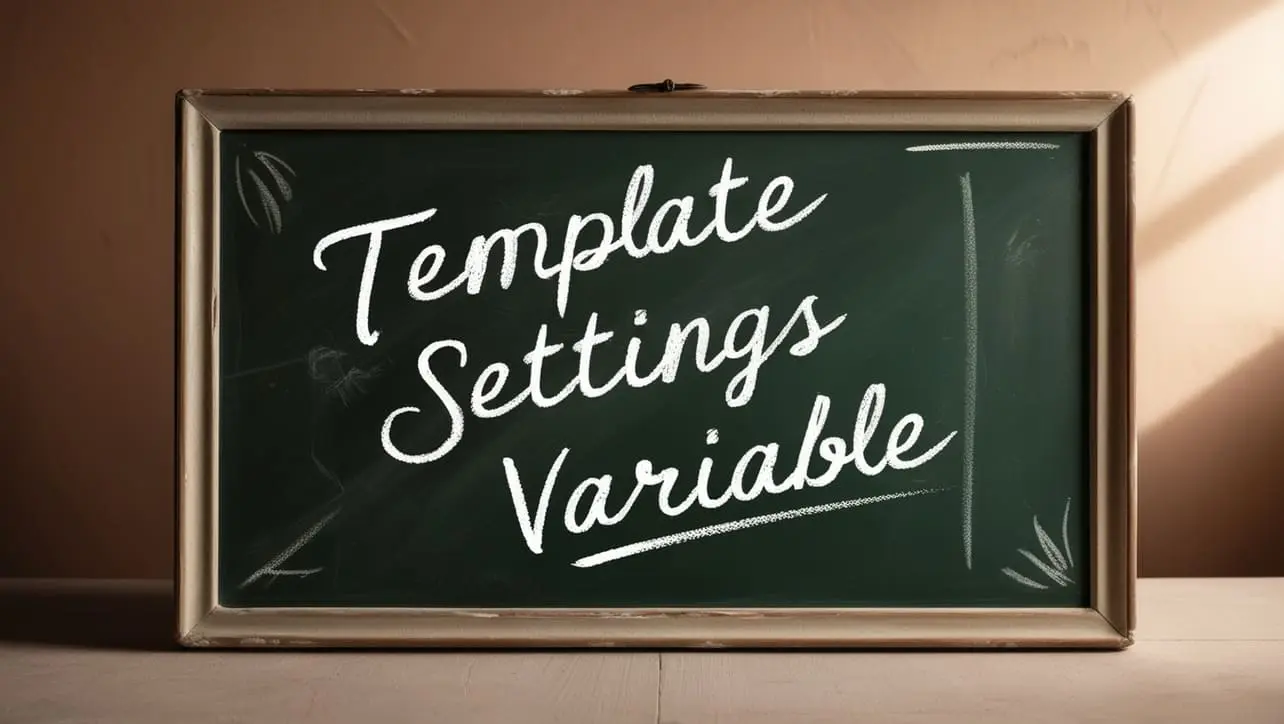
If you have any doubts regarding this article (Lodash _.find() Collection Method), please comment here. I will help you immediately.