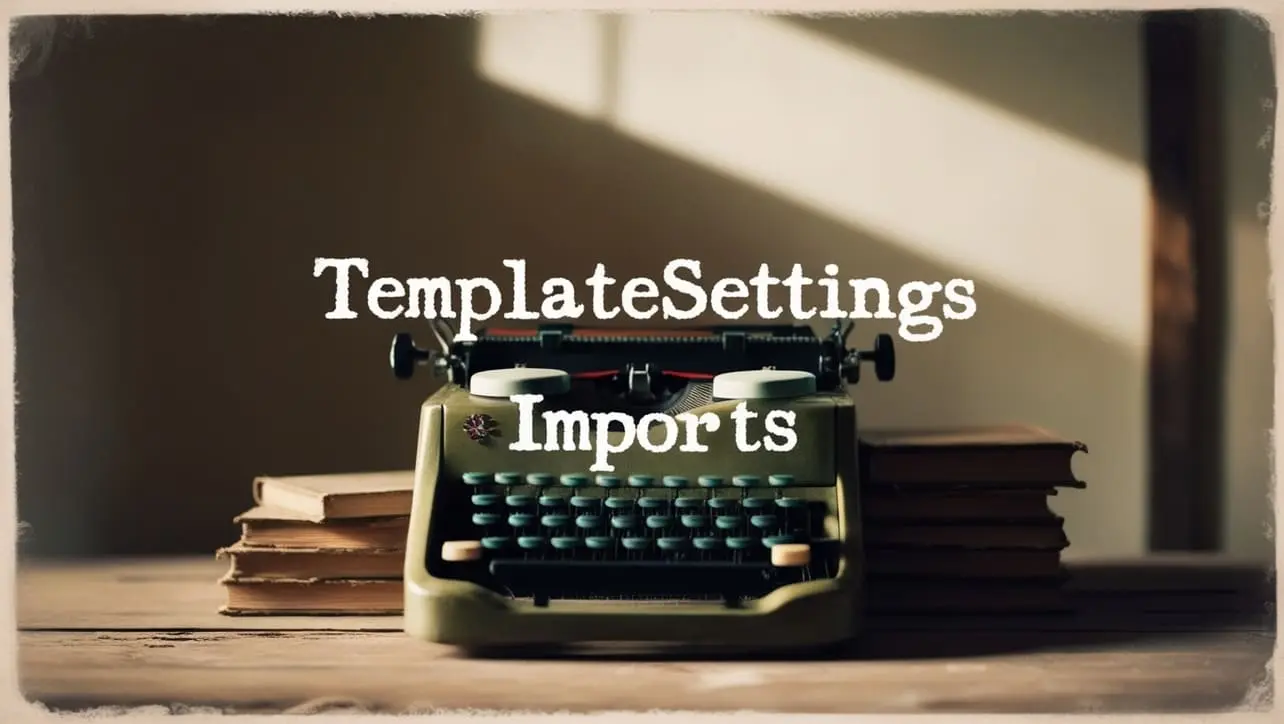
Lodash _.every() Collection Method
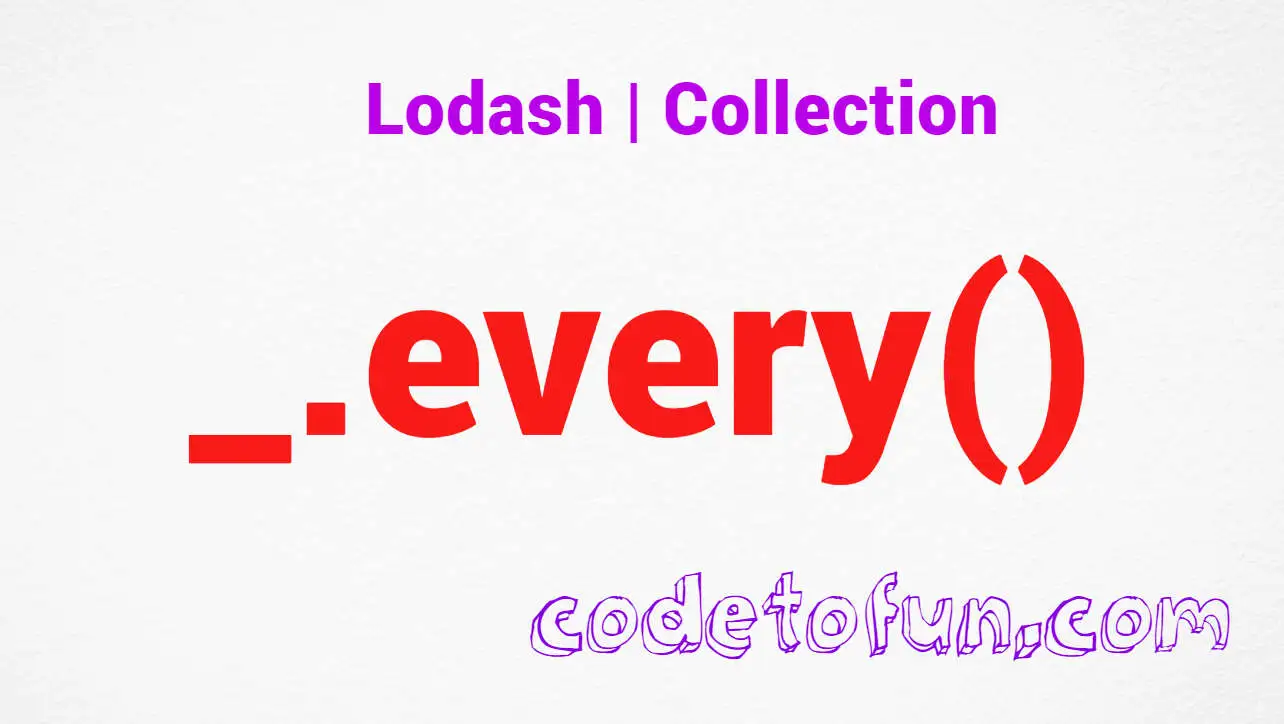
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript, effective handling of collections is fundamental to writing robust and reliable code. Lodash, a versatile utility library, offers a multitude of functions for working with collections, and among them is the _.every()
method.
This method provides an elegant and concise way to check if all elements in a collection satisfy a given condition. As a result, it becomes an indispensable tool for developers striving for code clarity and efficiency.
🧠 Understanding _.every()
The _.every()
method in Lodash is designed to determine whether all elements in a collection meet a specified condition. It iterates through each element in the collection and returns true if the condition is satisfied for every element, otherwise, it returns false.
💡 Syntax
_.every(collection, [predicate])
- collection: The collection to iterate over.
- predicate (Optional): The function invoked per iteration.
📝 Example
Let's delve into a practical example to grasp the functionality of _.every()
:
const _ = require('lodash');
const numbers = [2, 4, 6, 8, 10];
const allEven = _.every(numbers, num => num % 2 === 0);
console.log(allEven);
// Output: true
In this example, the _.every()
method is used to check if all numbers in the numbers array are even. The result is true since every element satisfies the given condition.
🏆 Best Practices
Understand the Predicate Function:
Ensure a clear understanding of the predicate function. The predicate is crucial as it defines the condition that each element must satisfy.
example.jsCopiedconst students = [ { name: 'Alice', grade: 90 }, { name: 'Bob', grade: 85 }, { name: 'Charlie', grade: 95 }, ]; const allHighAchievers = _.every(students, student => student.grade >= 90); console.log(allHighAchievers); // Output: false
Handle Edge Cases:
Consider edge cases to handle situations where the collection is empty or the predicate function might throw errors. Implement appropriate checks and default behaviors.
example.jsCopiedconst emptyCollection = []; const isEmpty = _.every(emptyCollection); console.log(isEmpty); // Output: true
Leverage Short-Circuiting:
Take advantage of the short-circuiting behavior of
_.every()
. As soon as a falsy value is encountered, the iteration stops, improving performance for large collections.example.jsCopiedconst mixedNumbers = [2, 4, 6, 7, 8, 10]; const allEven = _.every(mixedNumbers, num => { if (num % 2 === 0) { return true; // Continue iteration } else { console.log(`${num} is not even.`); return false; // Short-circuiting } }); console.log(allEven); // Output: false
📚 Use Cases
Validation:
Use
_.every()
for validating whether all elements in a collection meet specific criteria, such as form input validation or configuration checks.example.jsCopiedconst formFields = [/* ...array of form field values... */]; const allFieldsValid = _.every(formFields, field => isValid(field)); console.log(allFieldsValid);
Permission Checks:
Determine if all users in a collection have a certain level of permission by leveraging
_.every()
.example.jsCopiedconst users = [ { name: 'Alice', isAdmin: true }, { name: 'Bob', isAdmin: false }, { name: 'Charlie', isAdmin: true }, ]; const allAdmins = _.every(users, user => user.isAdmin); console.log(allAdmins); // Output: false
Data Consistency:
Ensure data consistency across a collection by checking if all elements adhere to a specific format or structure.
example.jsCopiedconst dataRecords = [/* ...array of data records... */]; const isConsistentFormat = _.every(dataRecords, record => hasRequiredFields(record)); console.log(isConsistentFormat);
🎉 Conclusion
The _.every()
method in Lodash provides a concise and effective way to check if all elements in a collection satisfy a given condition. Whether you're performing data validation, permission checks, or ensuring data consistency, this method proves to be a valuable asset in your JavaScript toolkit.
Embrace the power of collection manipulation with _.every()
and elevate your code to new levels of clarity and efficiency!
👨💻 Join our Community:
Author
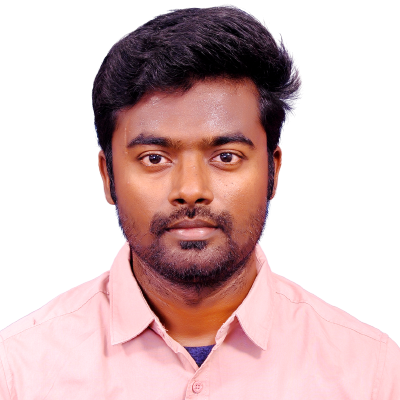
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
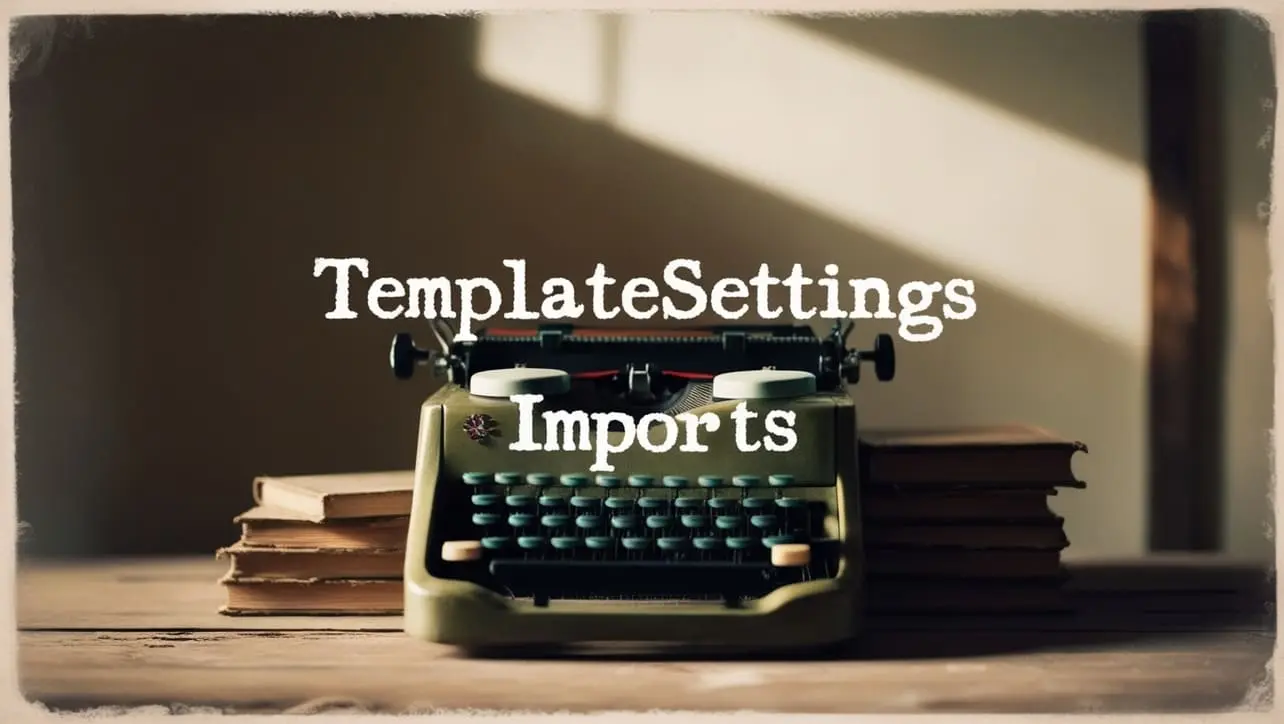
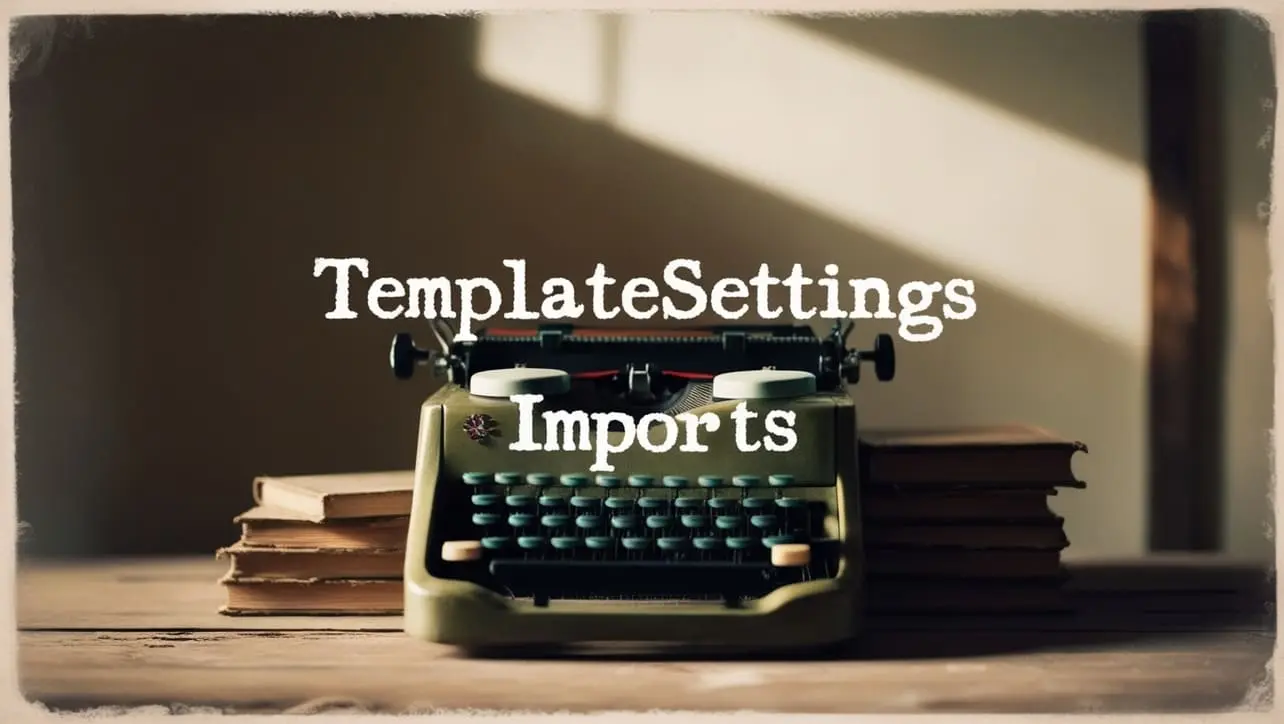
Lodash _.templateSettings.imports Property
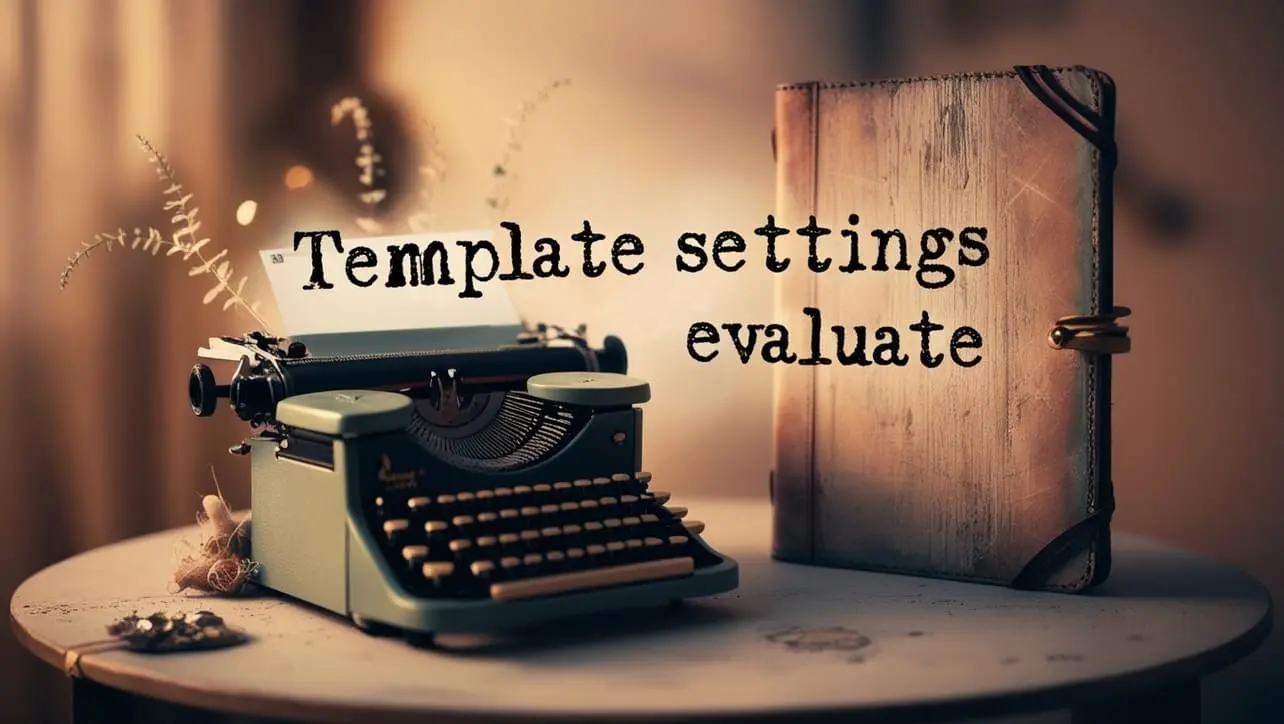
Lodash _.templateSettings.evaluate Property
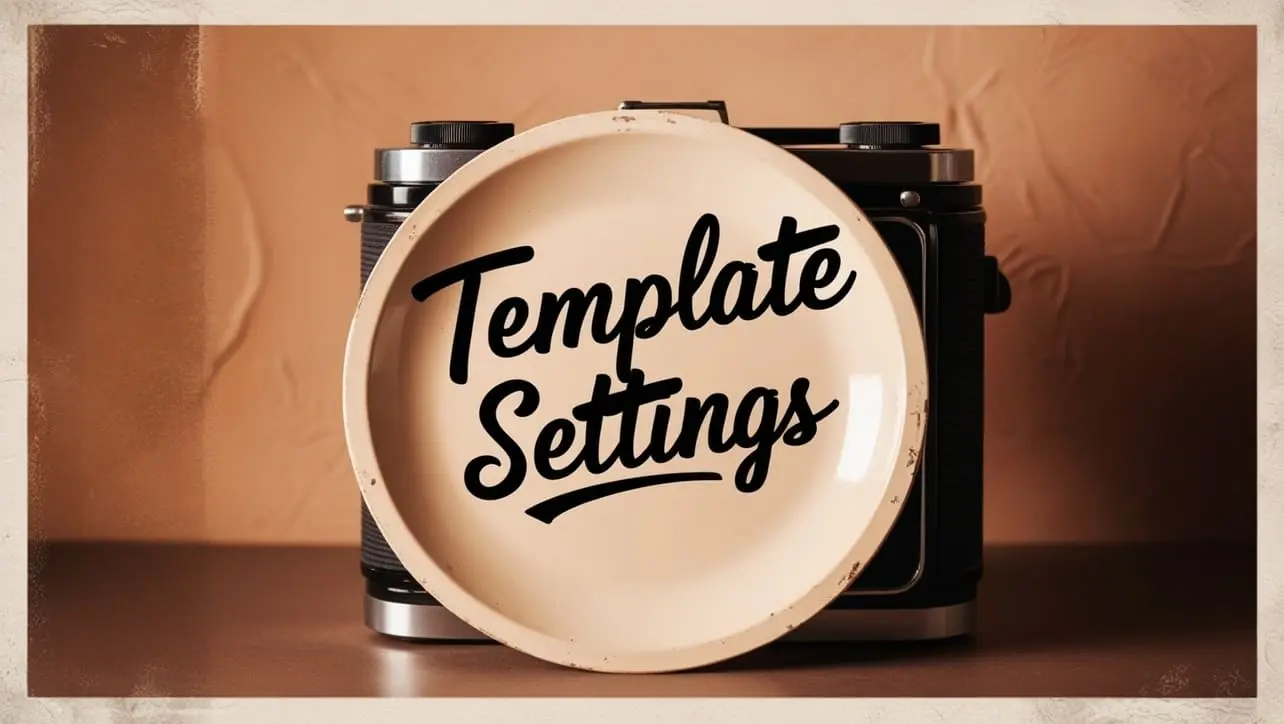
Lodash _.templateSettings Property
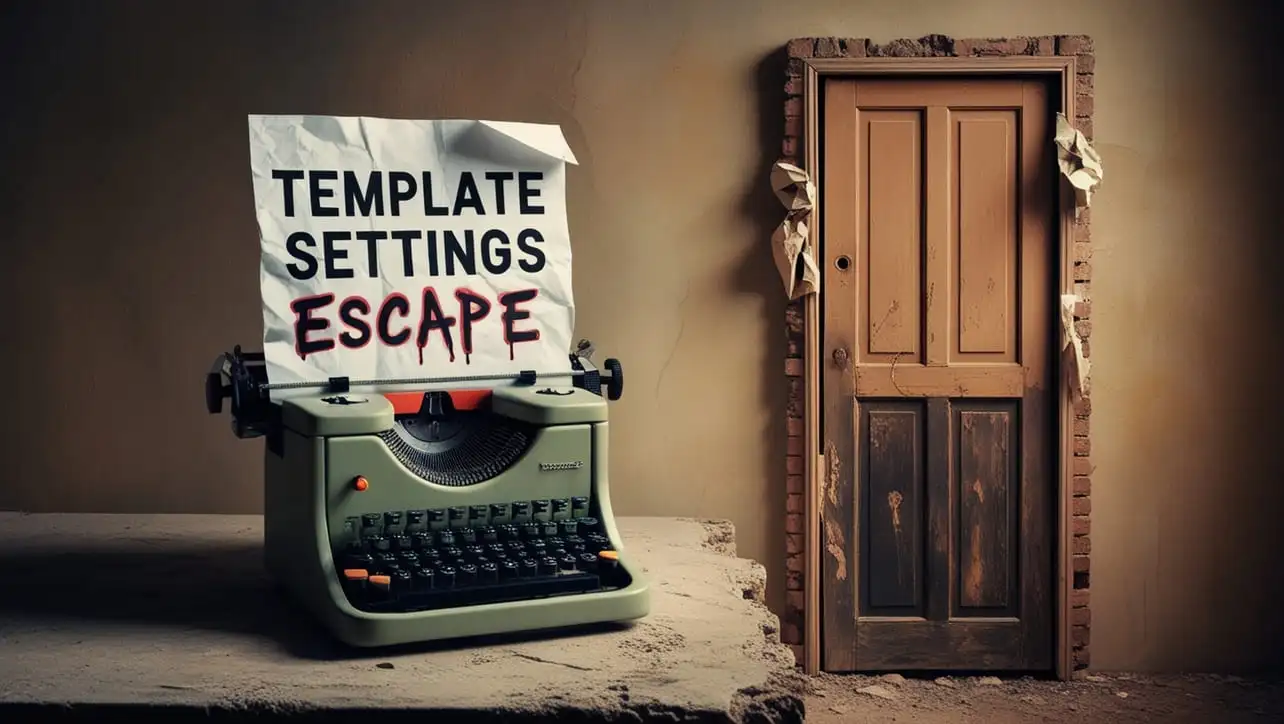
Lodash _.templateSettings.escape Property
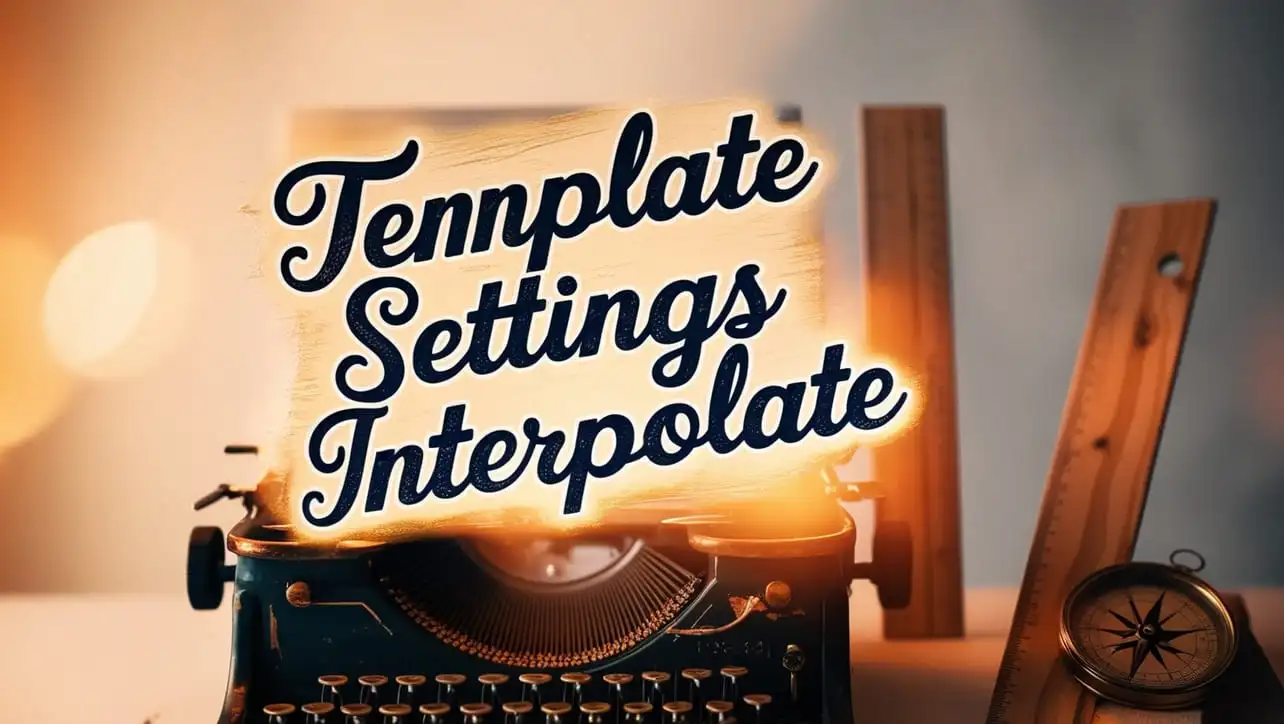
Lodash _.templateSettings.interpolate Property
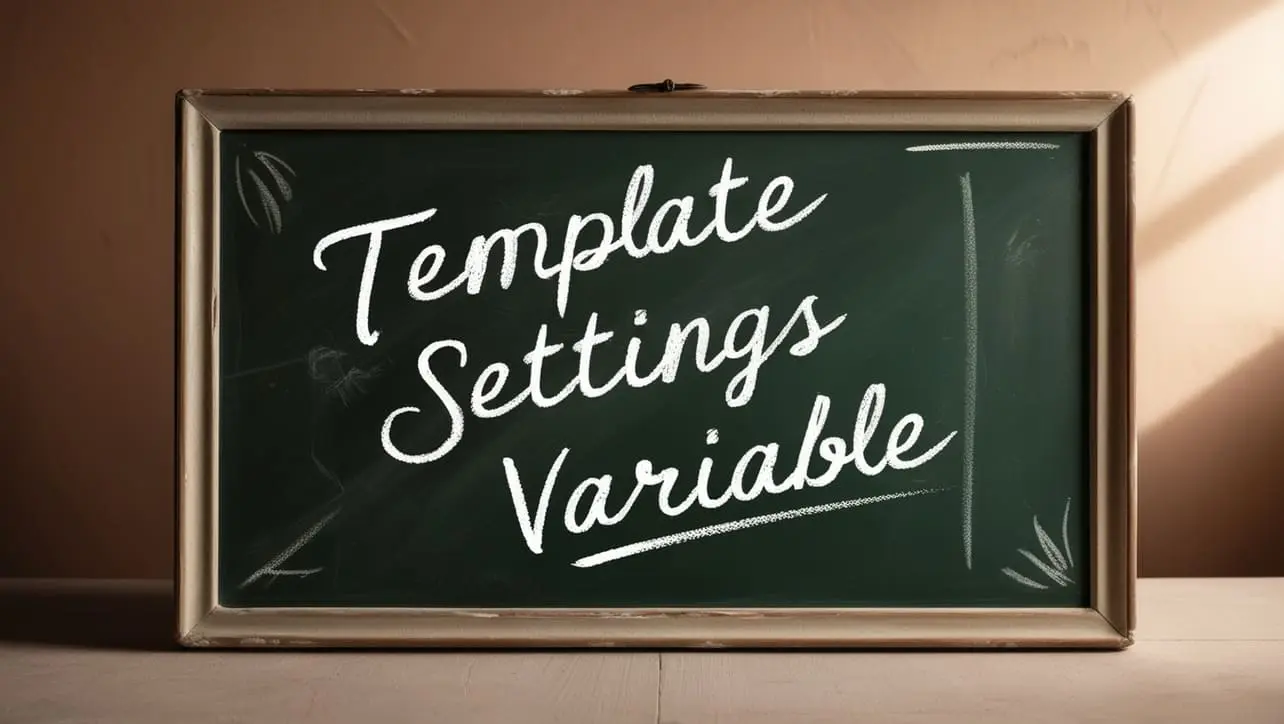
If you have any doubts regarding this article (Lodash _.every() Collection Method), please comment here. I will help you immediately.