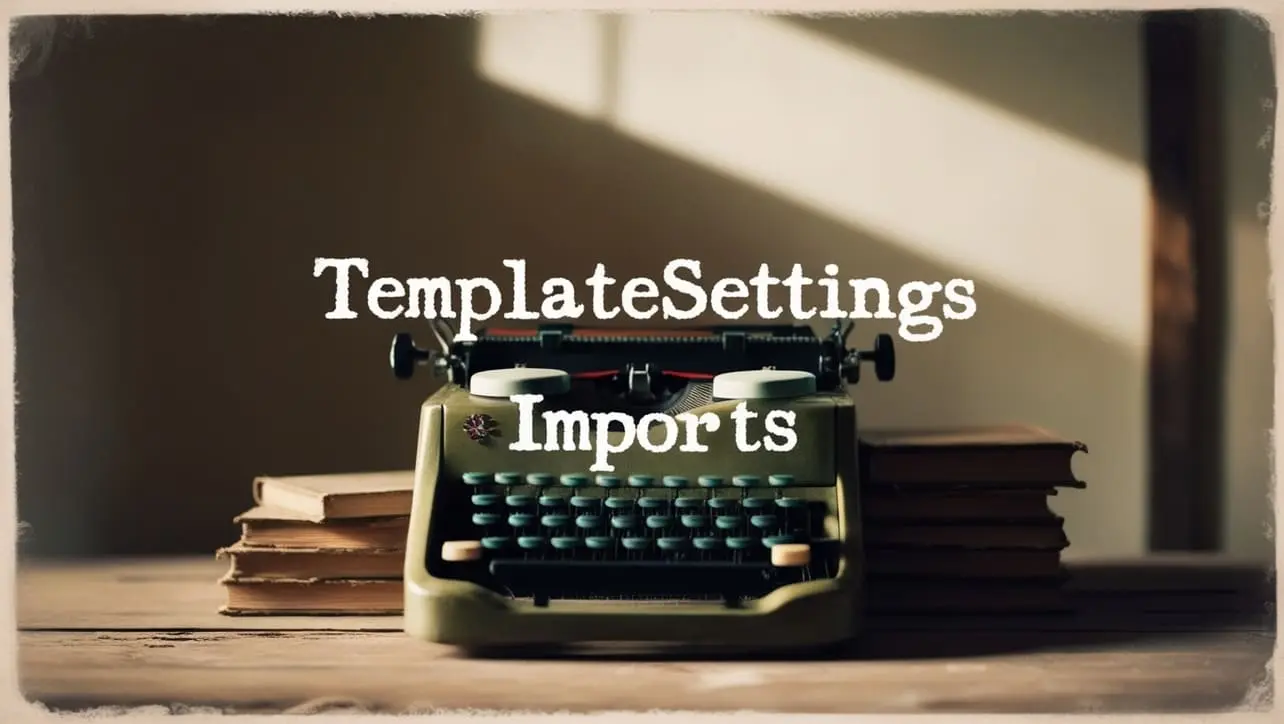
Lodash _.reduceRight() Collection Method
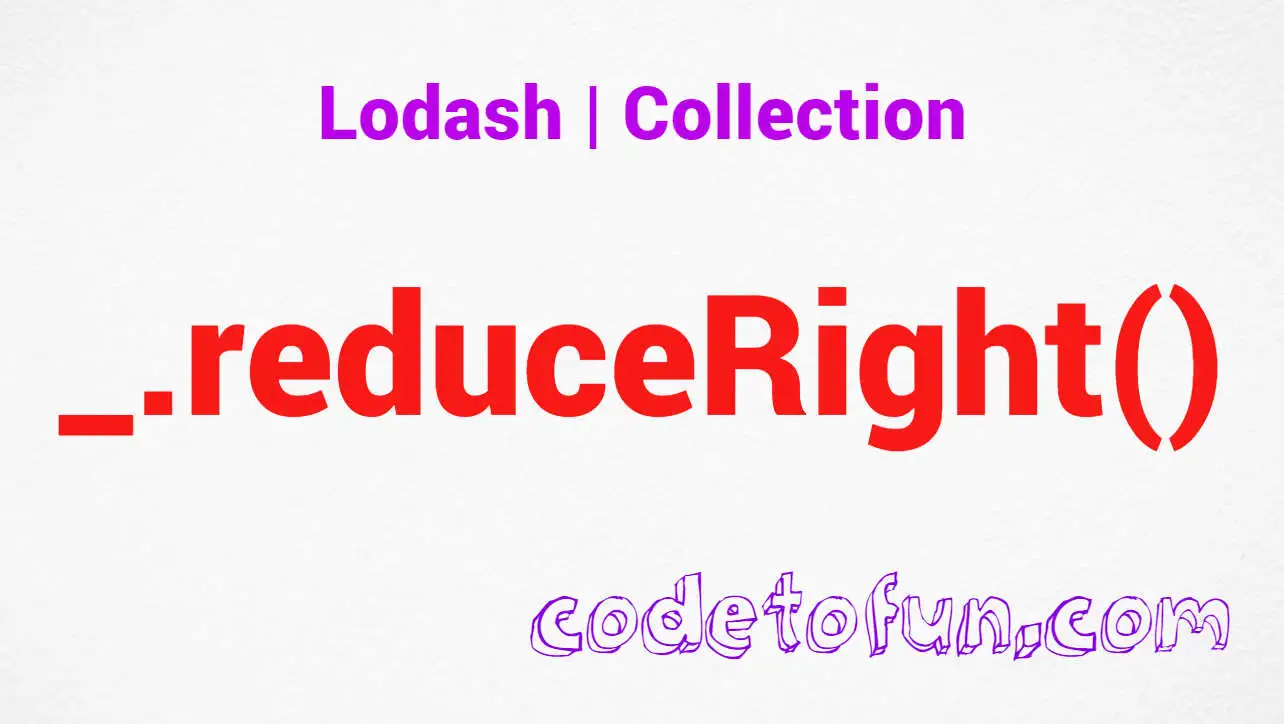
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, the ability to iterate and transform collections is a fundamental aspect of writing efficient and expressive code. Lodash, a comprehensive utility library, offers a variety of functions to simplify collection manipulation. One such versatile function is _.reduceRight()
.
This method allows developers to perform a right-to-left reduction on a collection, gradually transforming each element and accumulating a result. Let's explore the intricacies of _.reduceRight()
and its applications in JavaScript programming.
🧠 Understanding _.reduceRight() Method
The _.reduceRight()
method in Lodash is designed for efficiently reducing a collection from right to left. This means that the iteration starts from the last element of the collection and moves towards the first. During each iteration, a callback function is applied to the current accumulator and element, resulting in a cumulative value.
💡 Syntax
The syntax for the _.reduceRight()
method is straightforward:
_.reduceRight(collection, iteratee, [accumulator])
- collection: The collection to iterate over.
- iteratee: The function invoked per iteration.
- accumulator (Optional): The initial value of the accumulator.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.reduceRight()
method:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
const sum = _.reduceRight(numbers, (acc, num) => acc + num, 0);
console.log(sum);
// Output: 15
In this example, the _.reduceRight()
method is used to calculate the sum of the elements in the numbers array from right to left.
🏆 Best Practices
When working with the _.reduceRight()
method, consider the following best practices:
Choose an Appropriate Initial Value:
When using
_.reduceRight()
, select a suitable initial value for the accumulator. The initial value determines the type and structure of the final result.example.jsCopiedconst words = ['Lodash', 'reduceRight', 'example']; const reversedString = _.reduceRight(words, (acc, word) => acc + ' ' + word, ''); console.log(reversedString.trim()); // Output: 'example reduceRight Lodash'
Utilize the Iteratee Function Effectively:
The iteratee function in
_.reduceRight()
is crucial for defining the transformation logic. Utilize it effectively based on your specific requirements.example.jsCopiedconst array = [1, 2, 3, 4, 5]; const transformedArray = _.reduceRight(array, (acc, num) => { acc.push(num * 2); return acc; }, []); console.log(transformedArray); // Output: [10, 8, 6, 4, 2]
Be Mindful of Side Effects:
Avoid side effects within the iteratee function, as they can lead to unexpected behavior. The goal is to perform a clean and predictable transformation.
example.jsCopiedconst items = [1, 2, 3, 4, 5]; const result = _.reduceRight(items, (acc, item) => { // Avoid side effects like console.log() within the iteratee console.log(`Processing item: ${item}`); return acc + item; }, 0); console.log(result);
📚 Use Cases
Reversing Arrays:
_.reduceRight()
is particularly useful for reversing arrays. By accumulating elements from right to left, you can effectively reverse the order.example.jsCopiedconst originalArray = [1, 2, 3, 4, 5]; const reversedArray = _.reduceRight(originalArray, (acc, num) => { acc.push(num); return acc; }, []); console.log(reversedArray); // Output: [5, 4, 3, 2, 1]
Complex Data Transformations:
Performing complex data transformations, such as combining and reformatting data, becomes more straightforward with
_.reduceRight()
.example.jsCopiedconst data = [ { category: 'fruit', items: ['apple', 'banana'] }, { category: 'vegetable', items: ['carrot', 'spinach'] }, ]; const flattenedData = _.reduceRight(data, (acc, entry) => { acc.push(...entry.items); return acc; }, []); console.log(flattenedData); // Output: ['spinach', 'carrot', 'banana', 'apple']
Calculating Cumulative Values:
Calculate cumulative values, such as running totals or products, by leveraging the power of
_.reduceRight()
.example.jsCopiedconst values = [2, 3, 4, 5]; const cumulativeProduct = _.reduceRight(values, (acc, value) => acc * value, 1); console.log(cumulativeProduct); // Output: 120
🎉 Conclusion
The _.reduceRight()
method in Lodash serves as a valuable tool for performing right-to-left reductions on collections in JavaScript. Whether you're reversing arrays, conducting complex data transformations, or calculating cumulative values, this method provides a concise and efficient solution.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.reduceRight()
method in your Lodash projects.
👨💻 Join our Community:
Author
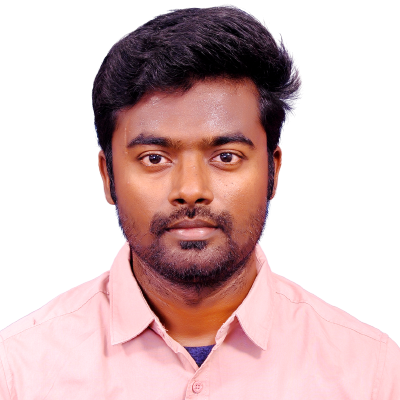
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
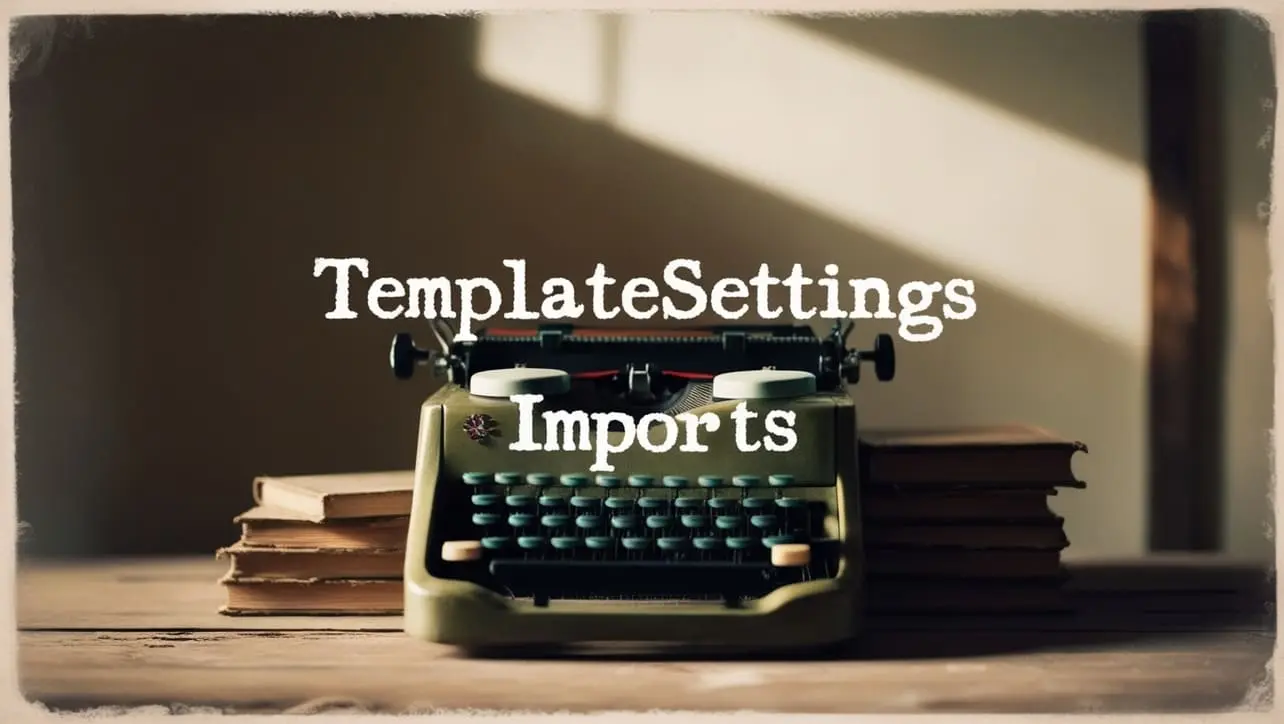
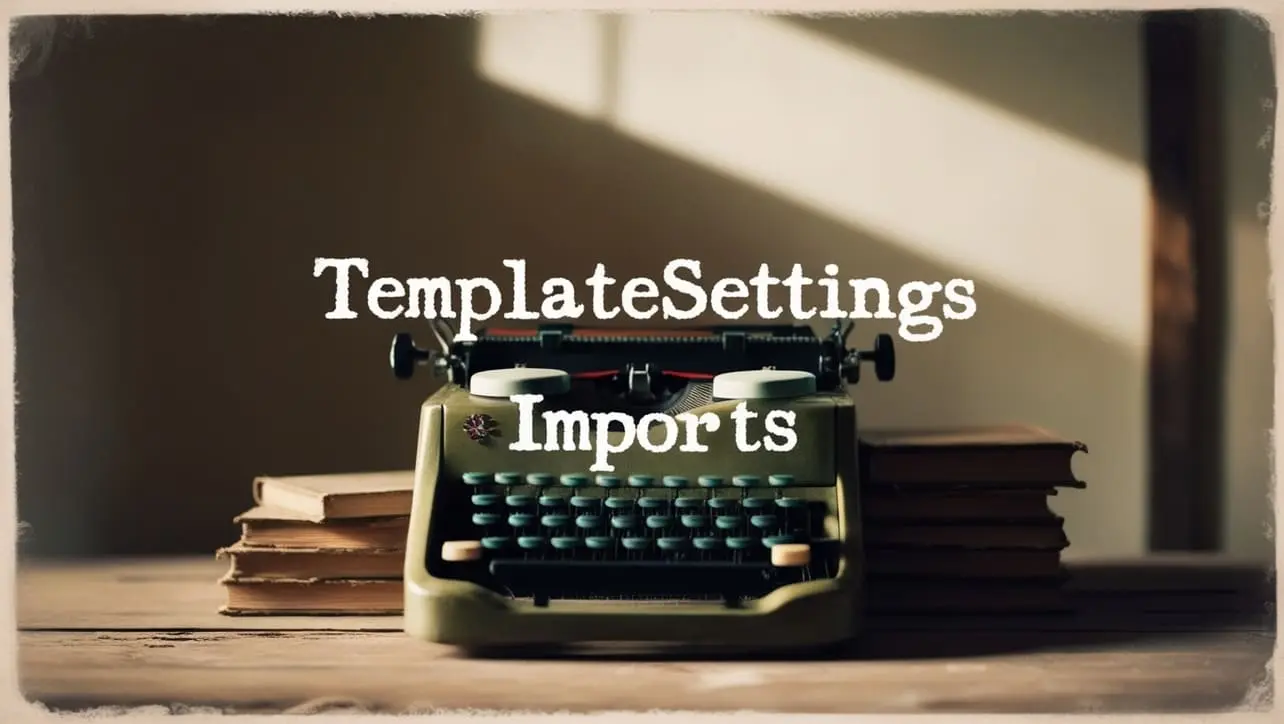
Lodash _.templateSettings.imports Property
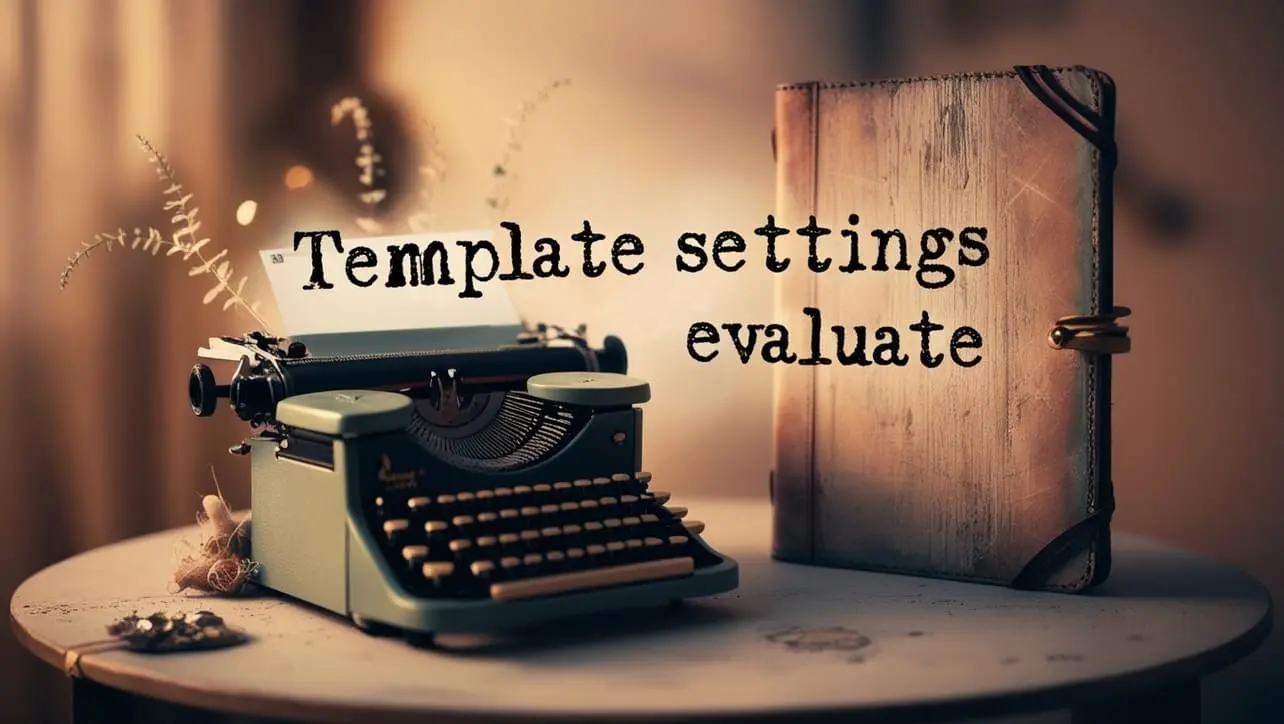
Lodash _.templateSettings.evaluate Property
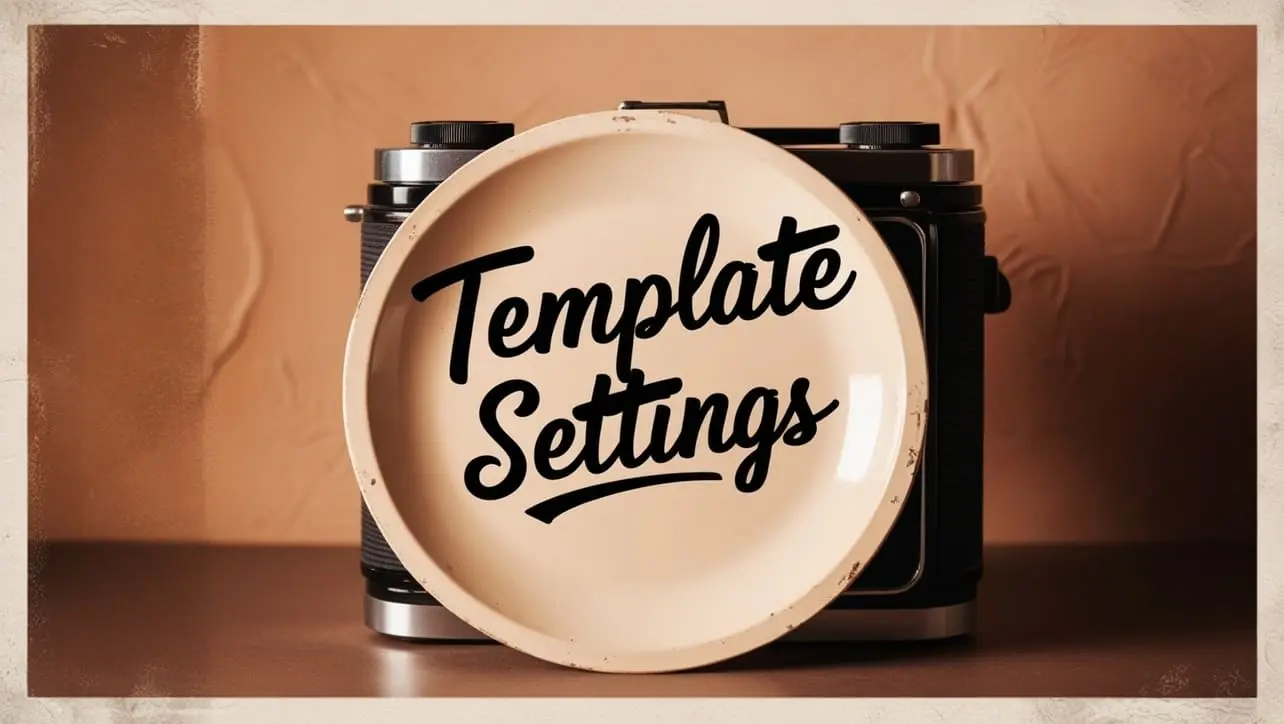
Lodash _.templateSettings Property
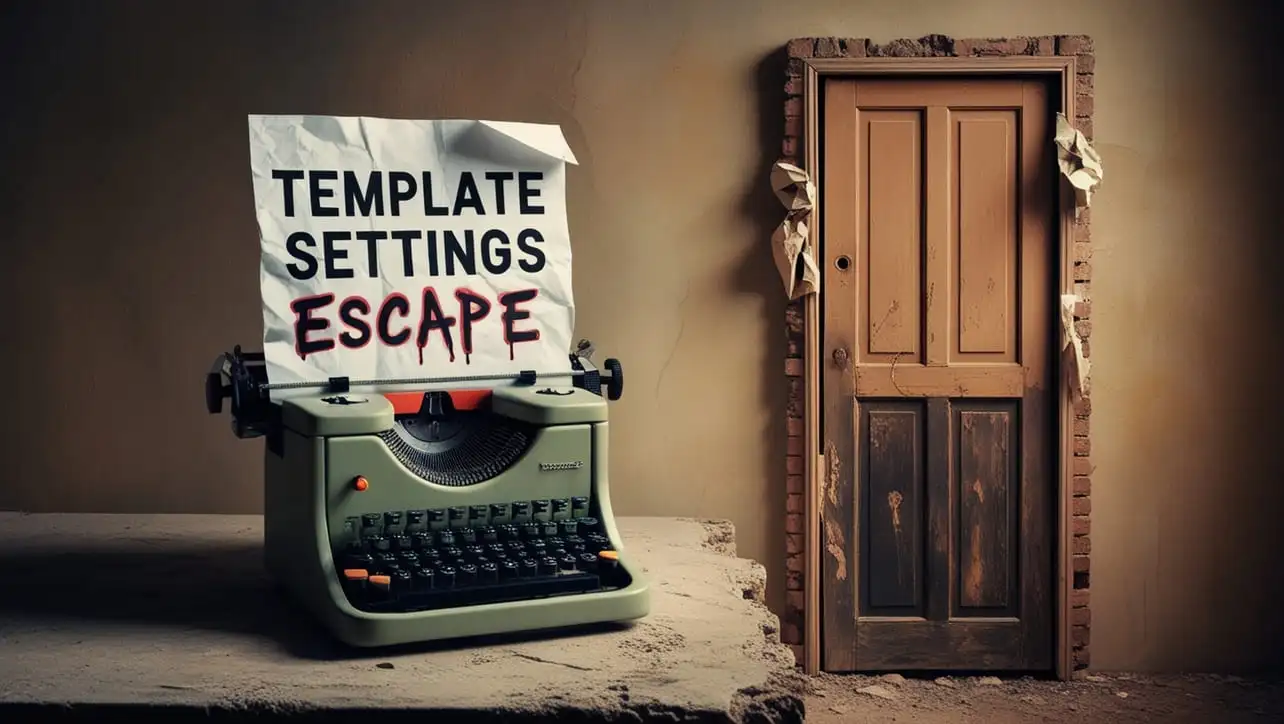
Lodash _.templateSettings.escape Property
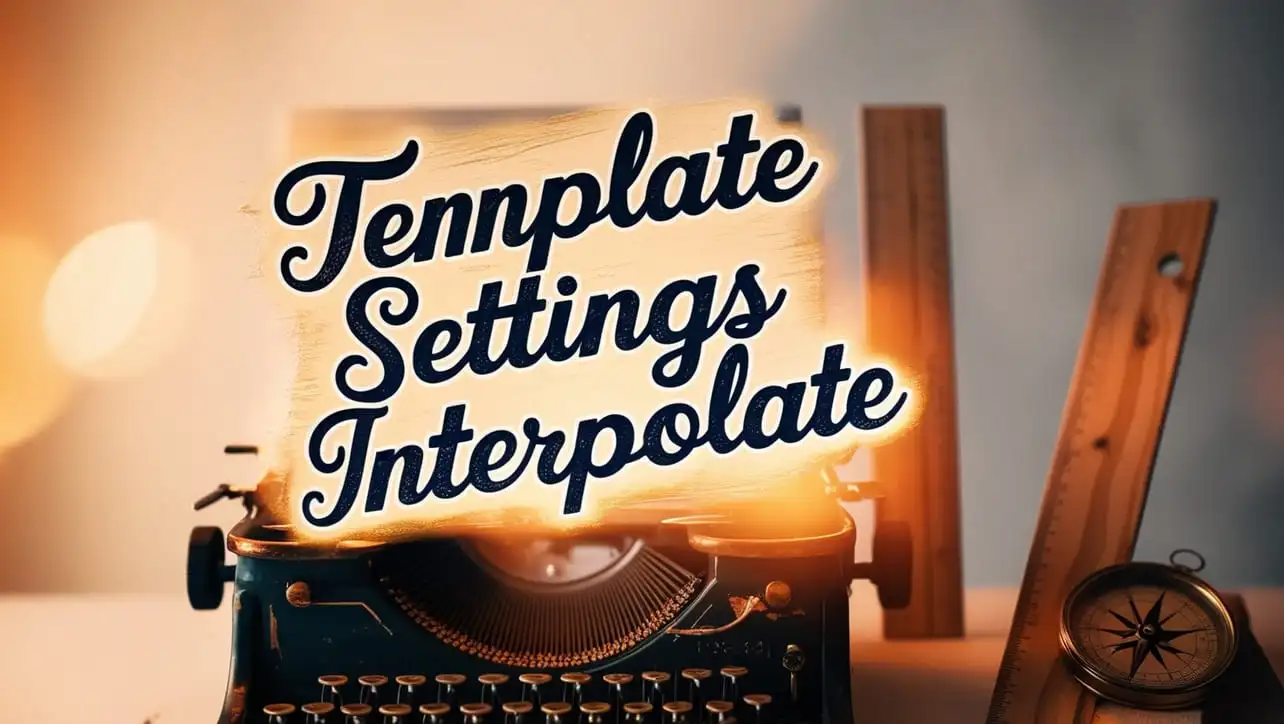
Lodash _.templateSettings.interpolate Property
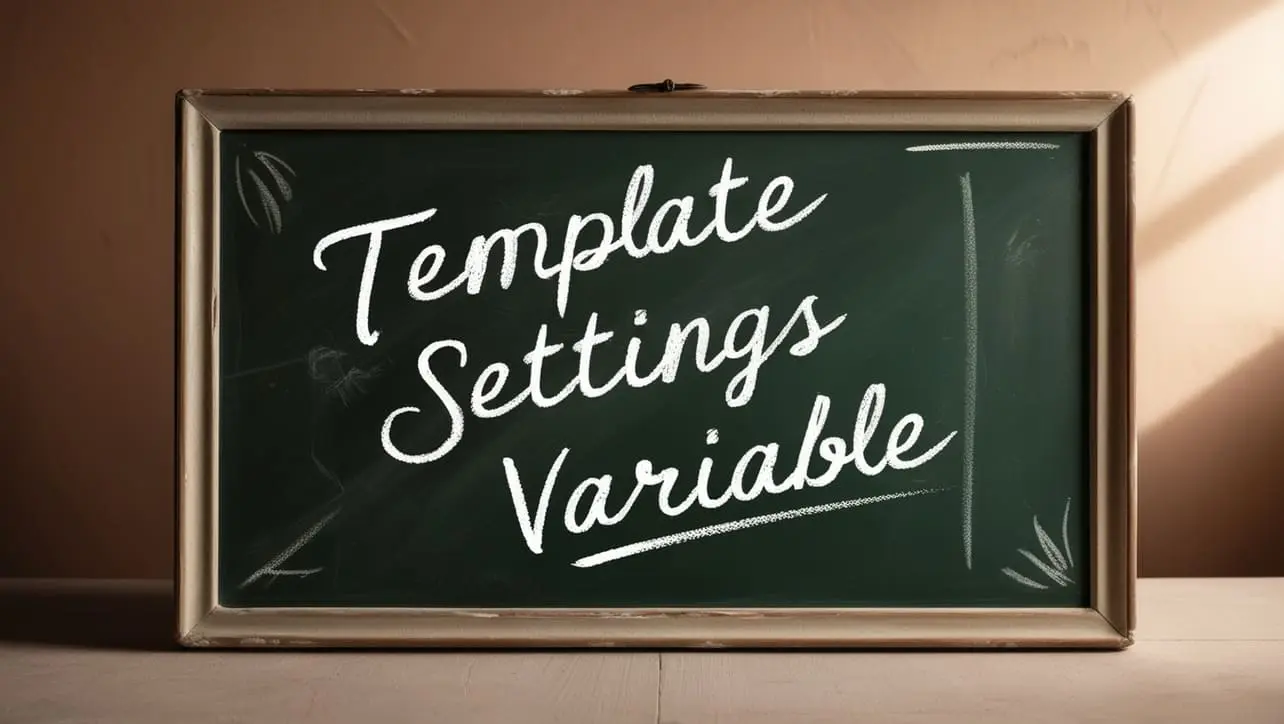
If you have any doubts regarding this article (Lodash _.reduceRight() Collection Method), please comment here. I will help you immediately.