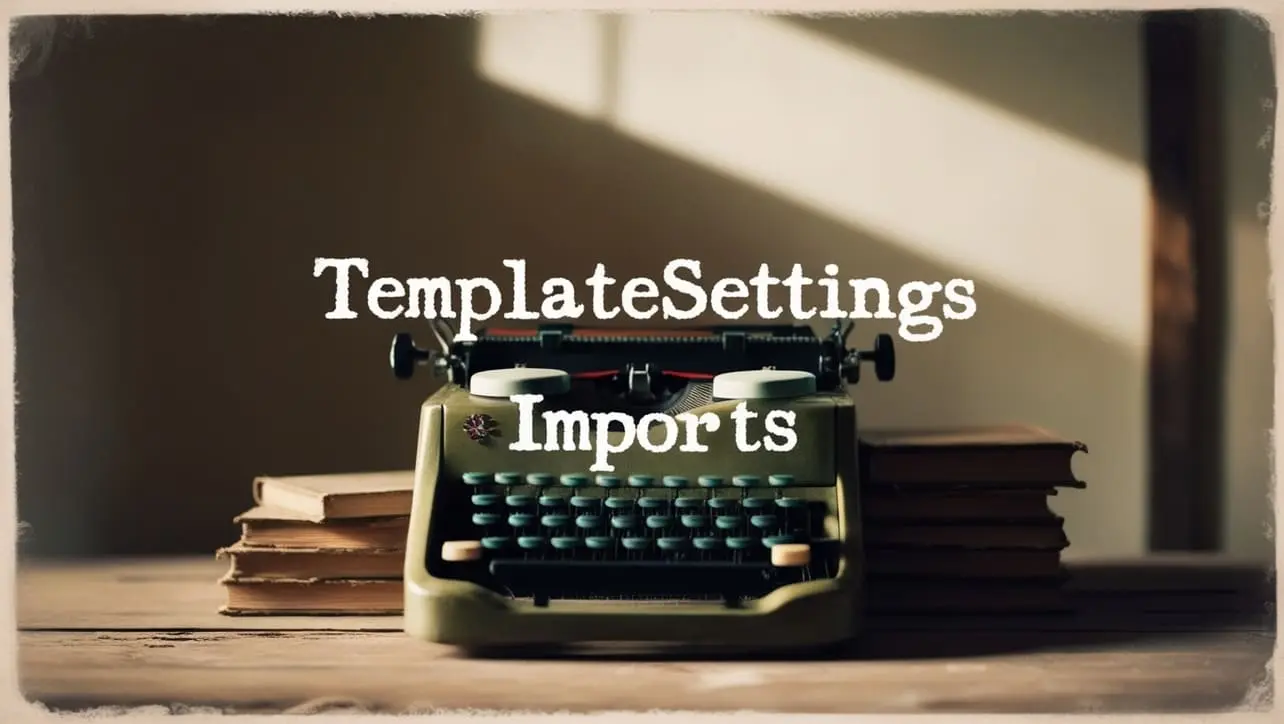
Lodash _.orderBy() Collection Method
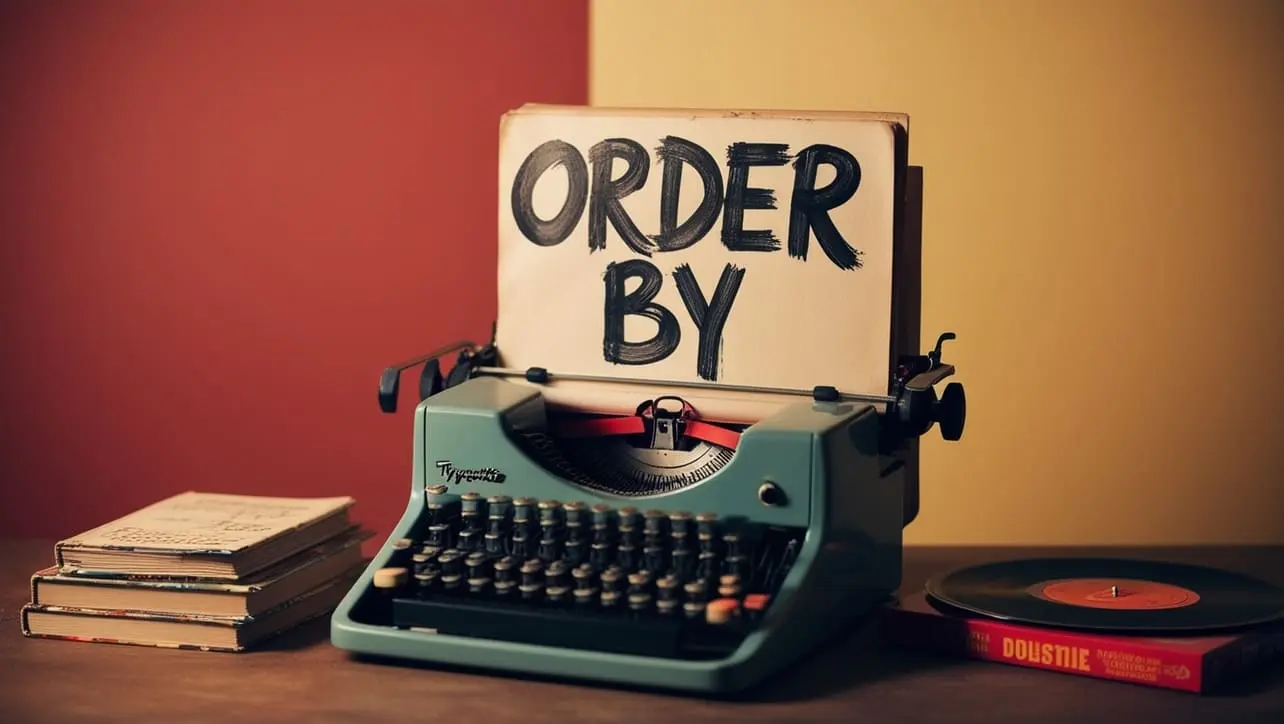
Photo Credit to CodeToFun
🙋 Introduction
In the ever-expanding world of JavaScript development, efficient sorting and ordering of data is a common challenge. Enter Lodash, a versatile utility library that simplifies array and collection manipulation. One of its standout features is the _.orderBy()
method, a powerful tool for sorting collections based on specified criteria.
This method empowers developers to handle complex sorting requirements with ease, enhancing the overall flexibility and functionality of their applications.
🧠 Understanding _.orderBy()
The _.orderBy()
method in Lodash facilitates the sorting of a collection (array of objects) based on specified criteria, such as sorting by one or more properties and in ascending or descending order. This flexibility makes it a valuable asset for developers working with dynamic datasets that require diverse sorting options.
💡 Syntax
_.orderBy(collection, [iteratees], [orders])
- collection: The collection to sort.
- iteratees (Optional): The property names or functions to sort by.
- orders (Optional): The sort orders for corresponding iteratees.
📝 Example
Let's explore a practical example to showcase the functionality of _.orderBy()
:
const _ = require('lodash');
const users = [
{ id: 1, name: 'John', age: 28 },
{ id: 2, name: 'Alice', age: 24 },
{ id: 3, name: 'Bob', age: 32 },
];
const sortedUsers = _.orderBy(users, ['age', 'name'], ['asc', 'desc']);
console.log(sortedUsers);
// Output: [ { id: 2, name: 'Alice', age: 24 },
// { id: 1, name: 'John', age: 28 },
// { id: 3, name: 'Bob', age: 32 } ]
In this example, the users collection is sorted first by the 'age' property in ascending order and then, for elements with equal 'age', by the 'name' property in descending order.
🏆 Best Practices
Define Sorting Criteria:
Clearly define the sorting criteria using the iteratees parameter. This can be a single property name, an array of property names, or functions to extract values for sorting.
example.jsCopiedconst products = [ { id: 1, name: 'Laptop', price: 1200 }, { id: 2, name: 'Phone', price: 800 }, { id: 3, name: 'Tablet', price: 500 }, ]; const sortedProducts = _.orderBy(products, 'price', 'desc'); console.log(sortedProducts); // Output: [ { id: 1, name: 'Laptop', price: 1200 }, // { id: 2, name: 'Phone', price: 800 }, // { id: 3, name: 'Tablet', price: 500 } ]
Specify Sort Orders:
When sorting by multiple criteria, explicitly specify the sort orders using the orders parameter. This ensures that the sorting behavior aligns with your expectations.
example.jsCopiedconst books = [ { id: 1, title: 'The Catcher in the Rye', author: 'J.D. Salinger', publicationYear: 1951 }, { id: 2, title: 'To Kill a Mockingbird', author: 'Harper Lee', publicationYear: 1960 }, { id: 3, title: '1984', author: 'George Orwell', publicationYear: 1949 }, ]; const sortedBooks = _.orderBy(books, ['publicationYear', 'title'], ['desc', 'asc']); console.log(sortedBooks); // Output: [ { id: 2, title: 'To Kill a Mockingbird', author: 'Harper Lee', publicationYear: 1960 }, // { id: 1, title: 'The Catcher in the Rye', author: 'J.D. Salinger', publicationYear: 1951 }, // { id: 3, title: '1984', author: 'George Orwell', publicationYear: 1949 } ]
Performance Considerations:
Be mindful of the performance implications, especially when dealing with large collections. If performance is a concern, consider whether a more targeted sorting approach or optimization is needed.
example.jsCopiedconst largeCollection = /* ...fetch data from API or elsewhere... */; console.time('orderBy'); const sortedData = _.orderBy(largeCollection, 'propertyToSort'); console.timeEnd('orderBy'); console.log(sortedData);
📚 Use Cases
Dynamic Sorting in UI:
_.orderBy()
is particularly valuable when implementing dynamic sorting in user interfaces. Users can choose different criteria and orders for sorting, providing a personalized experience.example.jsCopied// Assume user-selected sorting criteria and order const userSortCriteria = 'price'; const userSortOrder = 'asc'; const dynamicallySortedProducts = _.orderBy(products, userSortCriteria, userSortOrder); console.log(dynamicallySortedProducts);
Data Presentation:
When presenting data in a readable and meaningful way,
_.orderBy()
can be utilized to ensure that information is presented in a logical order based on specified criteria.example.jsCopiedconst presentations = [ { slide: 1, content: 'Introduction' }, { slide: 2, content: 'Main Points' }, { slide: 3, content: 'Conclusion' }, ]; const orderedPresentations = _.orderBy(presentations, 'slide', 'asc'); console.log(orderedPresentations); // Output: [ { slide: 1, content: 'Introduction' }, // { slide: 2, content: 'Main Points' }, // { slide: 3, content: 'Conclusion' } ]
Server-Side Sorting:
For applications interacting with server-side data,
_.orderBy()
can be applied to sort the data before rendering it in the user interface.example.jsCopied// Assume data fetched from a server const serverData = /* ...fetch data from server... */; const sortedServerData = _.orderBy(serverData, 'timestamp', 'desc'); console.log(sortedServerData);
🎉 Conclusion
The _.orderBy()
method in Lodash is a powerful and flexible solution for sorting collections based on specified criteria. Whether you're implementing dynamic sorting in a UI, presenting data in a meaningful way, or optimizing server-side data rendering, this method provides a versatile tool for handling diverse sorting requirements in JavaScript.
Unlock the potential of collection sorting with _.orderBy()
and elevate your JavaScript development experience!
👨💻 Join our Community:
Author
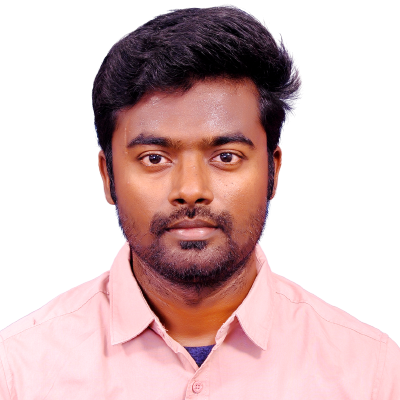
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
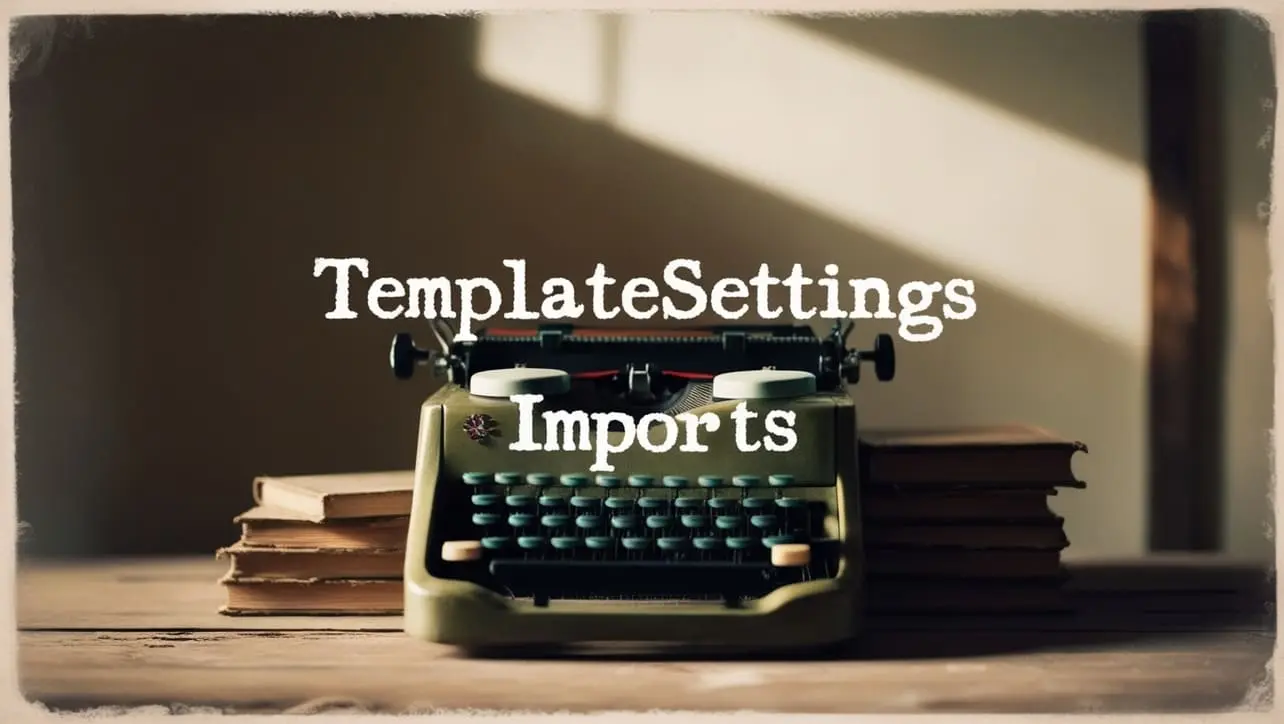
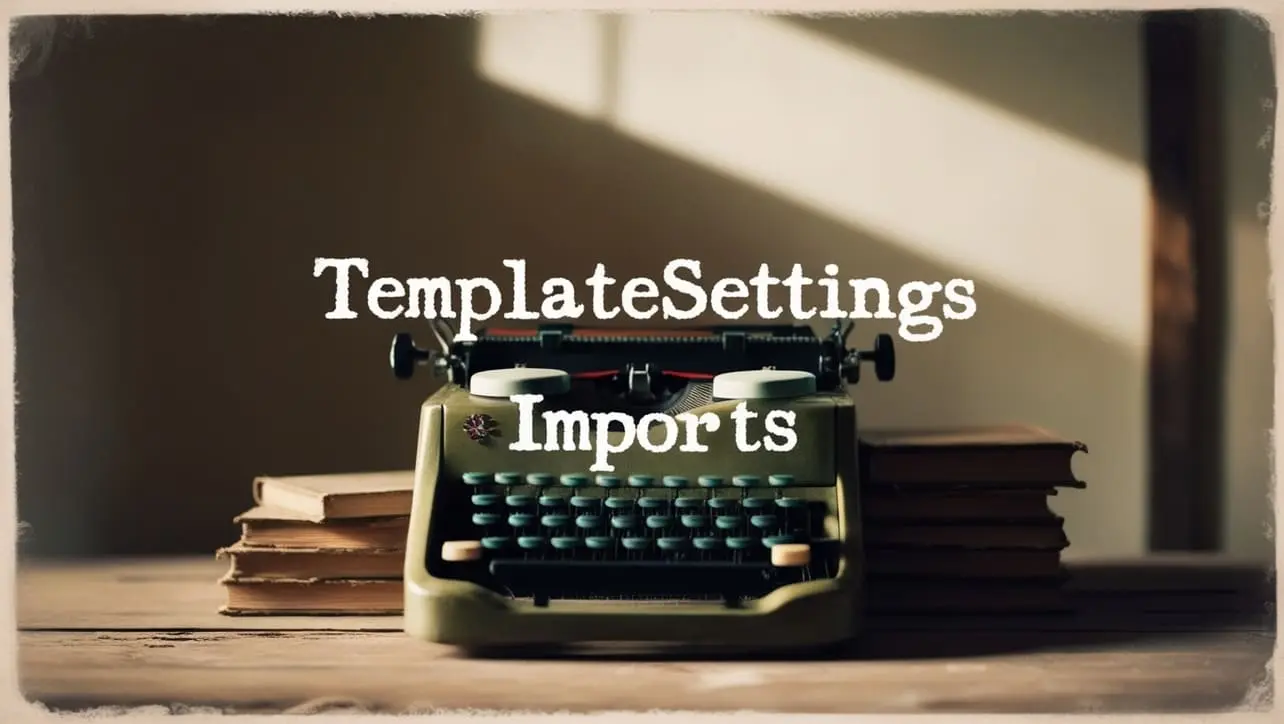
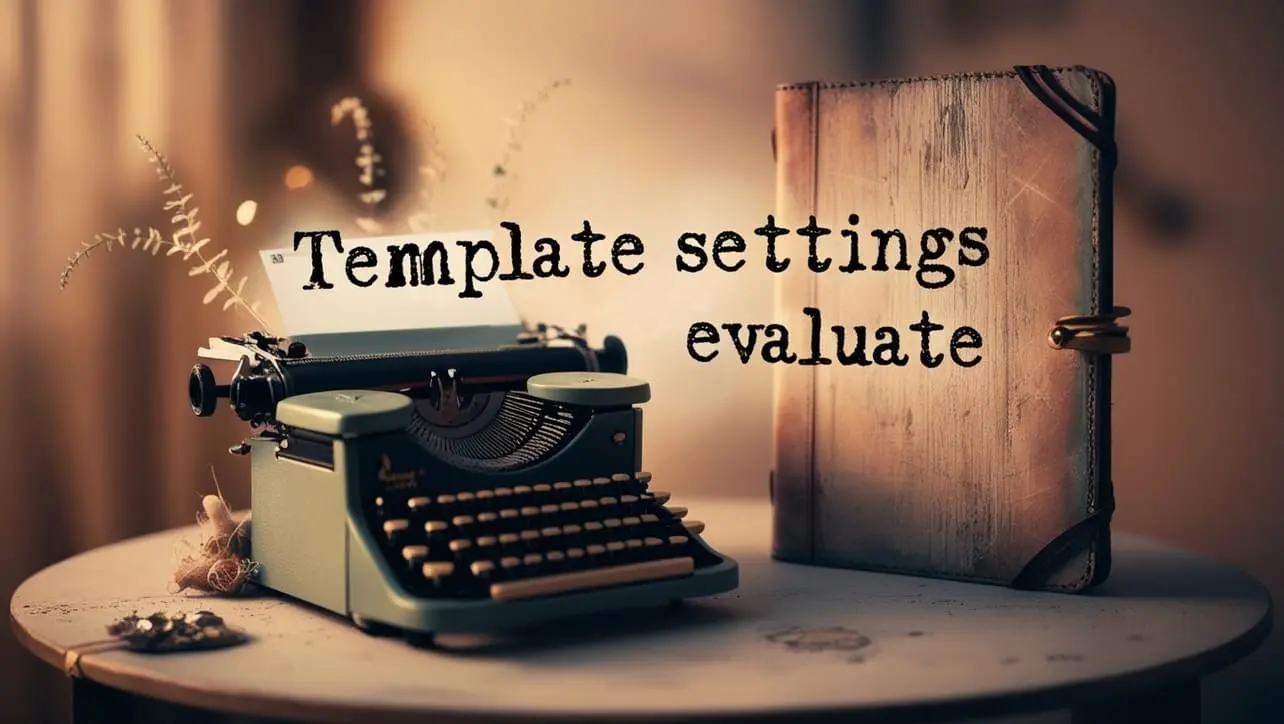
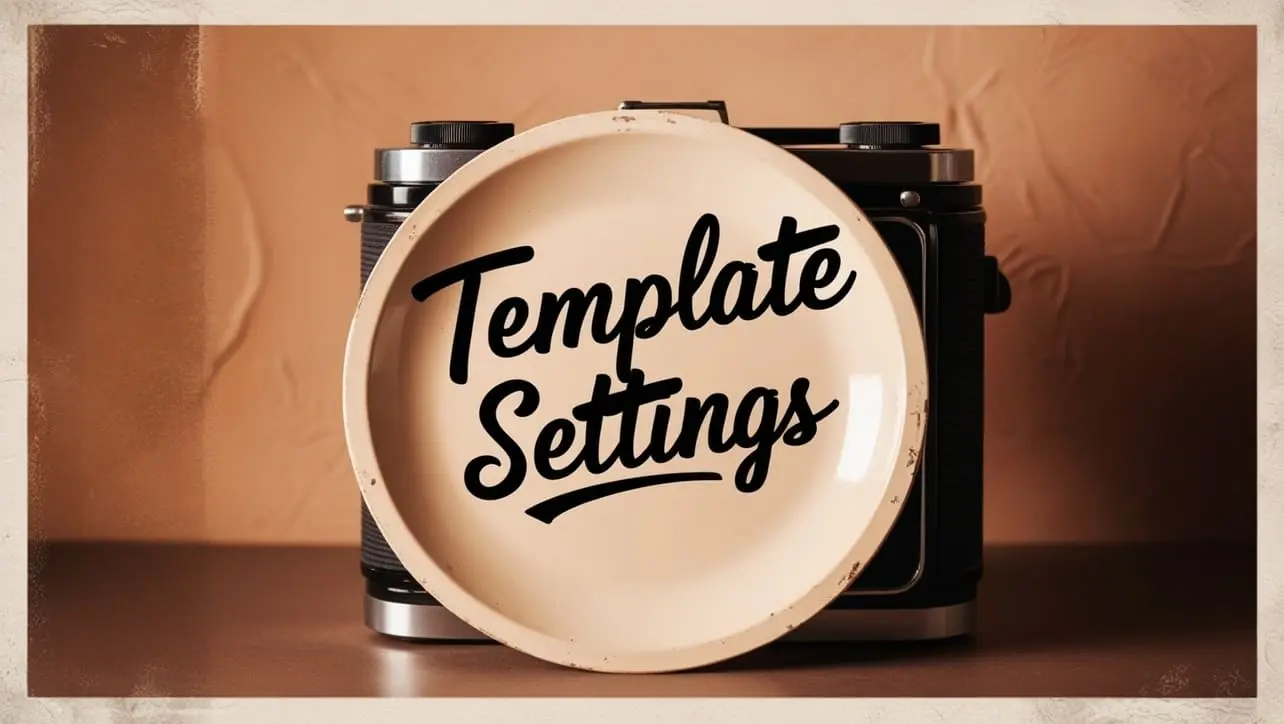
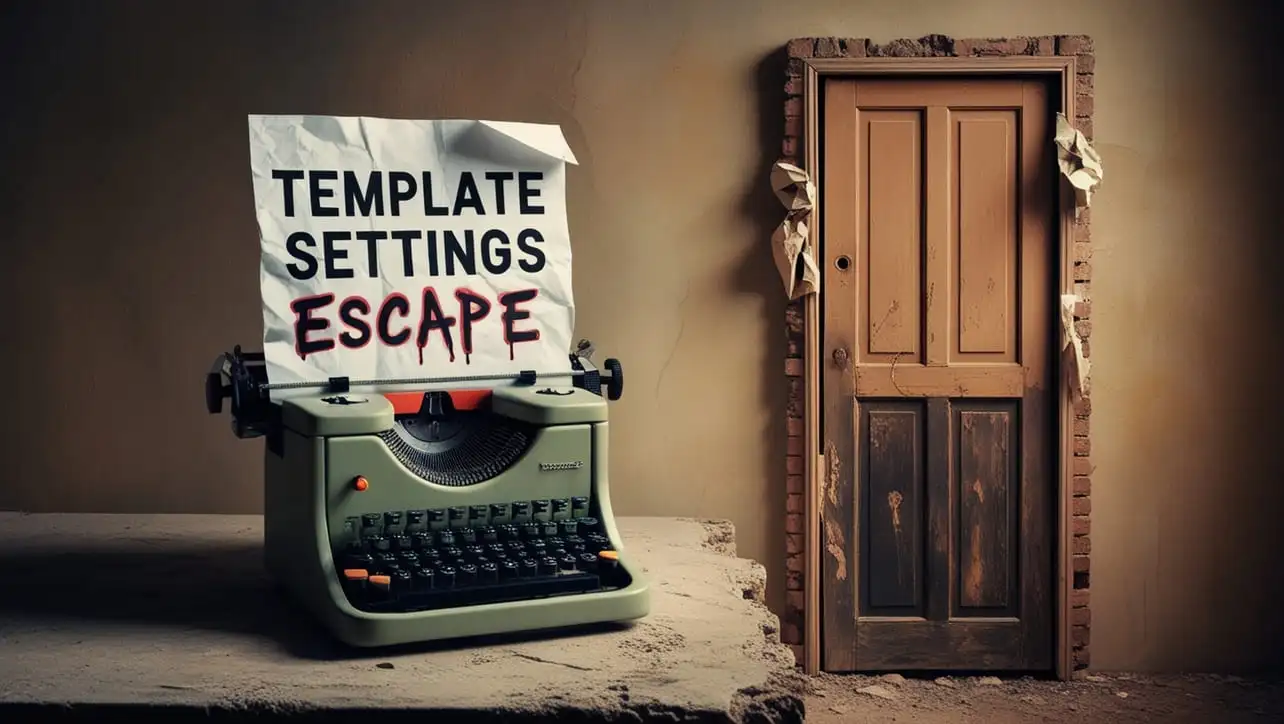
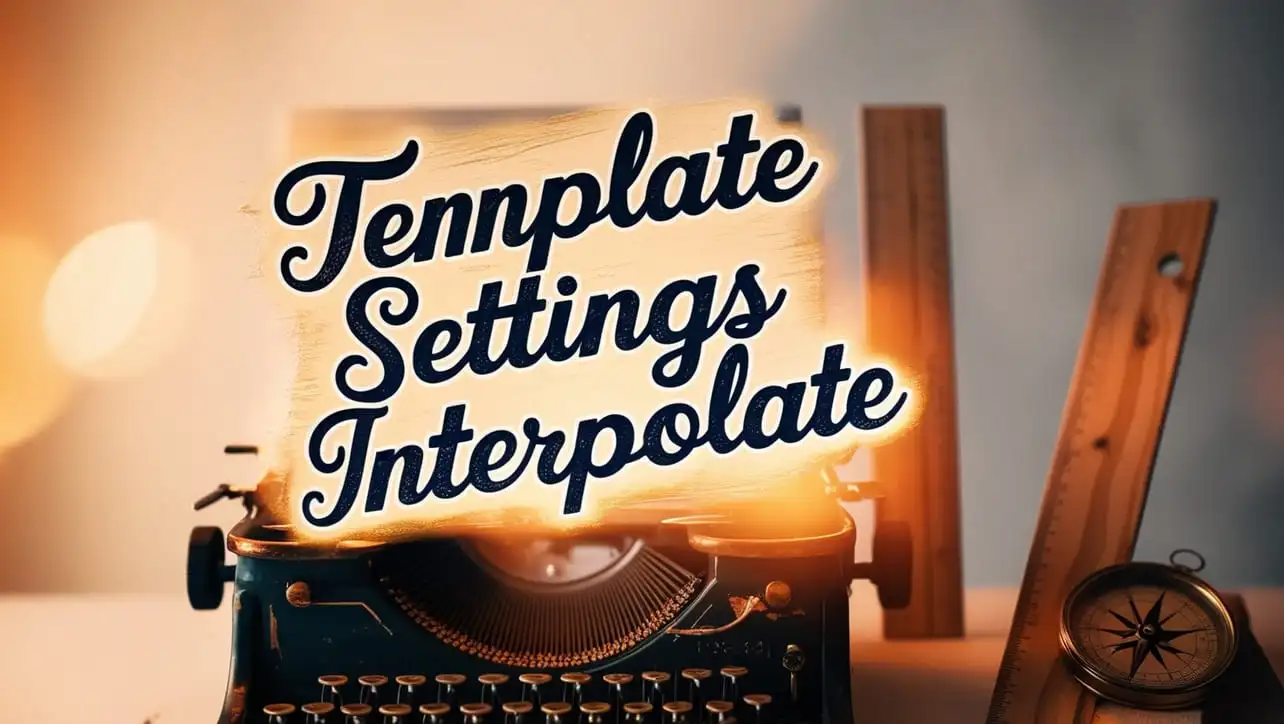
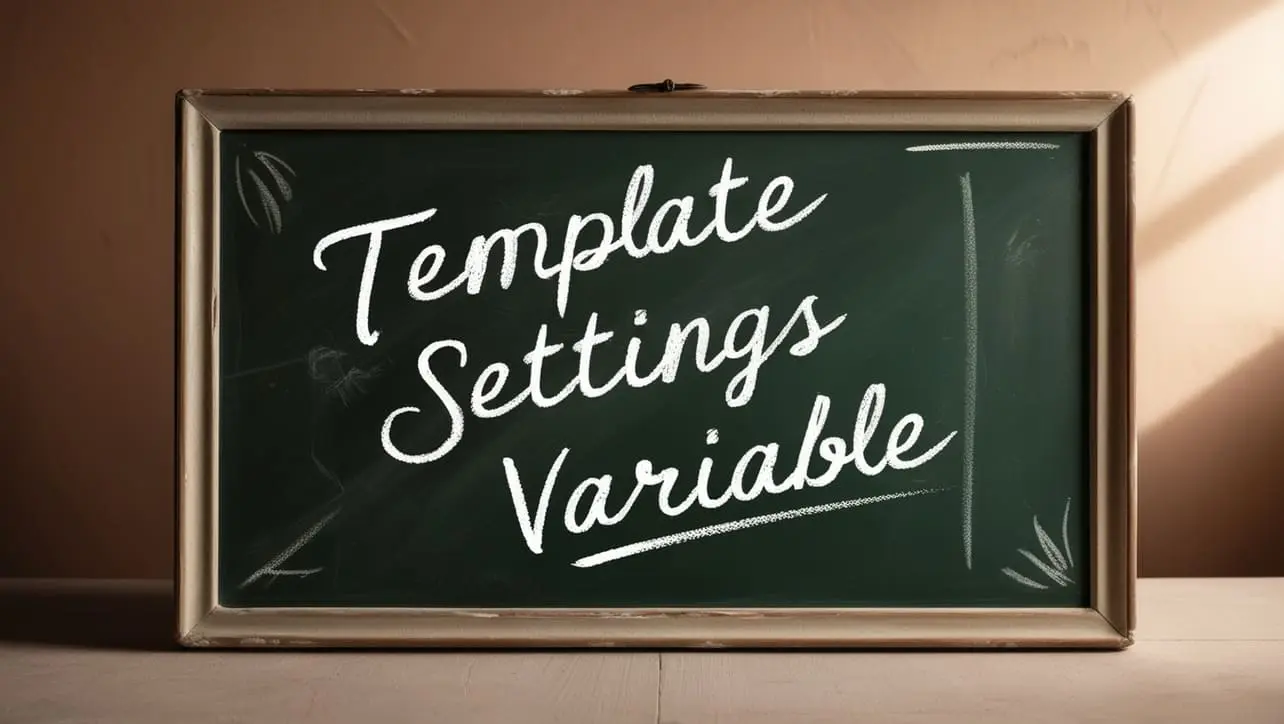
If you have any doubts regarding this article (Lodash _.orderBy() Collection Method), please comment here. I will help you immediately.