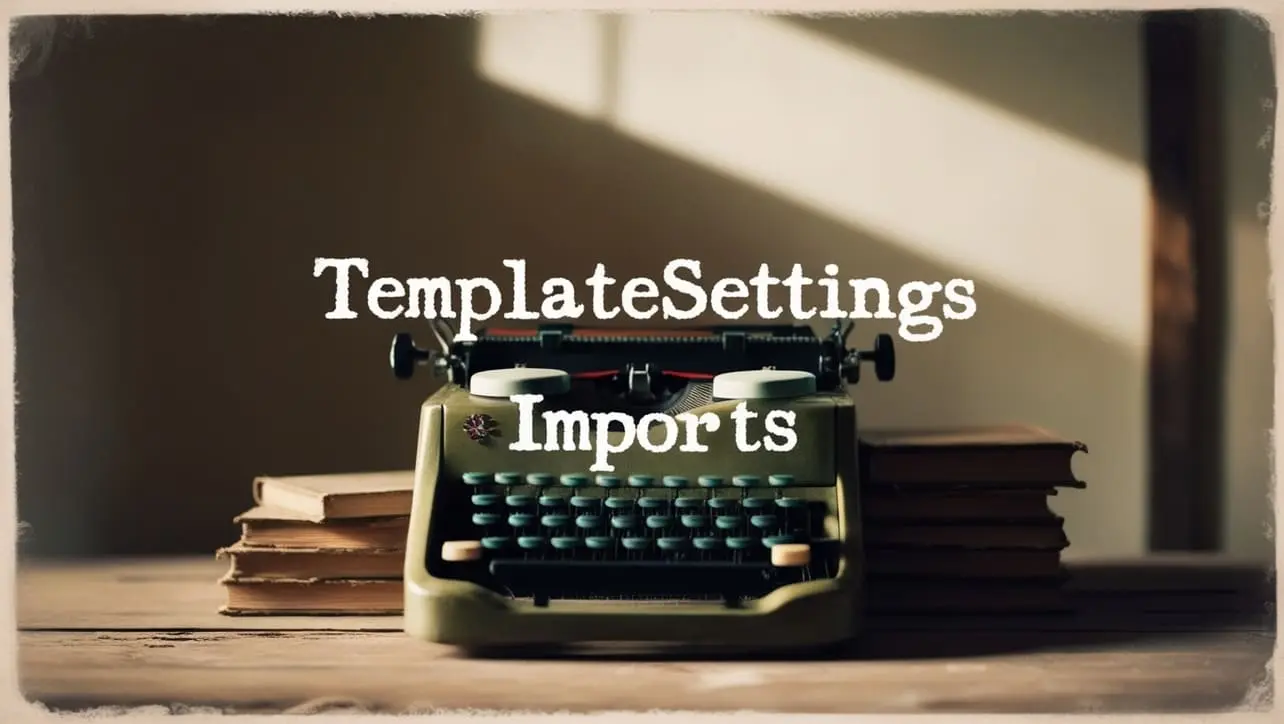
Lodash _.map() Collection Method
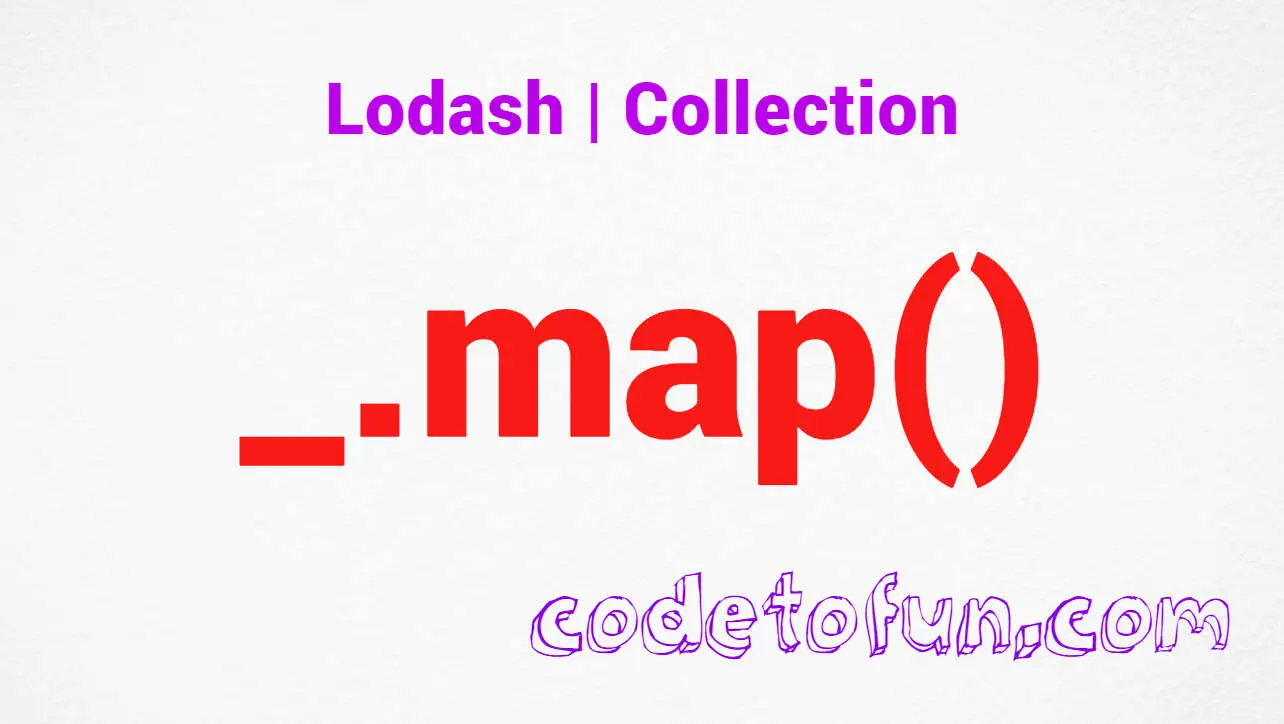
Photo Credit to CodeToFun
🙋 Introduction
Efficiently transforming data is a common task in JavaScript development, and the Lodash library provides a powerful ally in the form of the _.map()
method. This versatile method allows developers to iterate over a collection, applying a function to each element and creating a new array of the results.
Understanding and harnessing the capabilities of _.map()
can greatly enhance code expressiveness and readability.
🧠 Understanding _.map()
The _.map()
method in Lodash is designed for transforming the contents of a collection, such as an array or an object. It iterates over each element of the collection, applies a given function to it, and then constructs a new array containing the results of those function applications.
💡 Syntax
_.map(collection, [iteratee])
- collection: The collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's explore a simple example to illustrate the functionality of _.map()
:
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5];
const squaredArray = _.map(originalArray, num => num * num);
console.log(squaredArray);
// Output: [1, 4, 9, 16, 25]
In this example, the originalArray is transformed using _.map()
to create a new array where each element is squared.
🏆 Best Practices
Define a Clear Iteratee Function:
When using
_.map()
, define a clear and concise iteratee function. This makes the code more readable and ensures that the transformation logic is easy to understand.example.jsCopiedconst words = ['apple', 'banana', 'cherry']; const uppercasedWords = _.map(words, word => word.toUpperCase()); console.log(uppercasedWords); // Output: ['APPLE', 'BANANA', 'CHERRY']
Leverage Iteratee Shorthand:
Take advantage of iteratee shorthand when the transformation logic is a simple operation, such as accessing a property.
example.jsCopiedconst arrayOfObjects = [{ name: 'John' }, { name: 'Alice' }, { name: 'Bob' }]; const namesArray = _.map(arrayOfObjects, 'name'); console.log(namesArray); // Output: ['John', 'Alice', 'Bob']
Handle Undefined Values:
Be cautious when the iteratee function may result in undefined values. Consider using conditional checks or providing a default value to ensure the output array is consistent.
example.jsCopiedconst numbers = [1, 2, 3, 4, 5]; const transformedArray = _.map(numbers, num => (num % 2 === 0 ? num * 2 : undefined)).filter(Boolean); console.log(transformedArray); // Output: [4, 8]
📚 Use Cases
Data Transformation:
_.map()
is ideal for transforming data within a collection. It allows you to easily modify each element based on a specified logic, creating a new array with the transformed values.example.jsCopiedconst temperaturesInCelsius = [0, 25, 100]; const temperaturesInFahrenheit = _.map(temperaturesInCelsius, celsius => (celsius * 9/5) + 32); console.log(temperaturesInFahrenheit); // Output: [32, 77, 212]
Extracting Specific Properties:
When working with an array of objects,
_.map()
simplifies the extraction of specific properties, creating a new array with just the desired values.example.jsCopiedconst users = [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }]; const userIds = _.map(users, 'id'); console.log(userIds); // Output: [1, 2, 3]
Generating HTML Elements:
For dynamic content generation,
_.map()
can be employed to iterate over a collection and generate HTML elements based on the data.example.jsCopiedconst colors = ['red', 'green', 'blue']; const colorListItems = _.map(colors, color => `<li style="color: ${color};">${color}</li>`); console.log(colorListItems); // Output: ['<li style="color: red;">red</li>', '<li style="color: green;">green</li>', '<li style="color: blue;">blue</li>']
🎉 Conclusion
The _.map()
method in Lodash serves as a valuable tool for transforming collections in a concise and readable manner. Whether you are modifying each element, extracting specific properties, or generating dynamic content, _.map()
empowers you to express your logic efficiently.
Explore the capabilities of _.map()
and elevate your JavaScript development by simplifying data transformations in a clean and expressive way!
👨💻 Join our Community:
Author
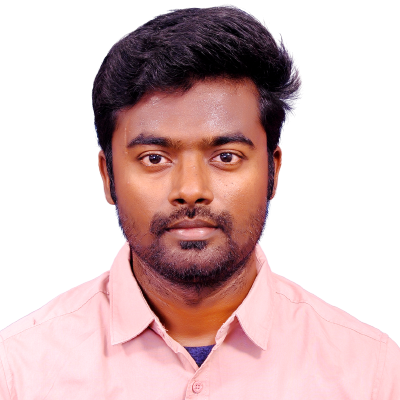
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
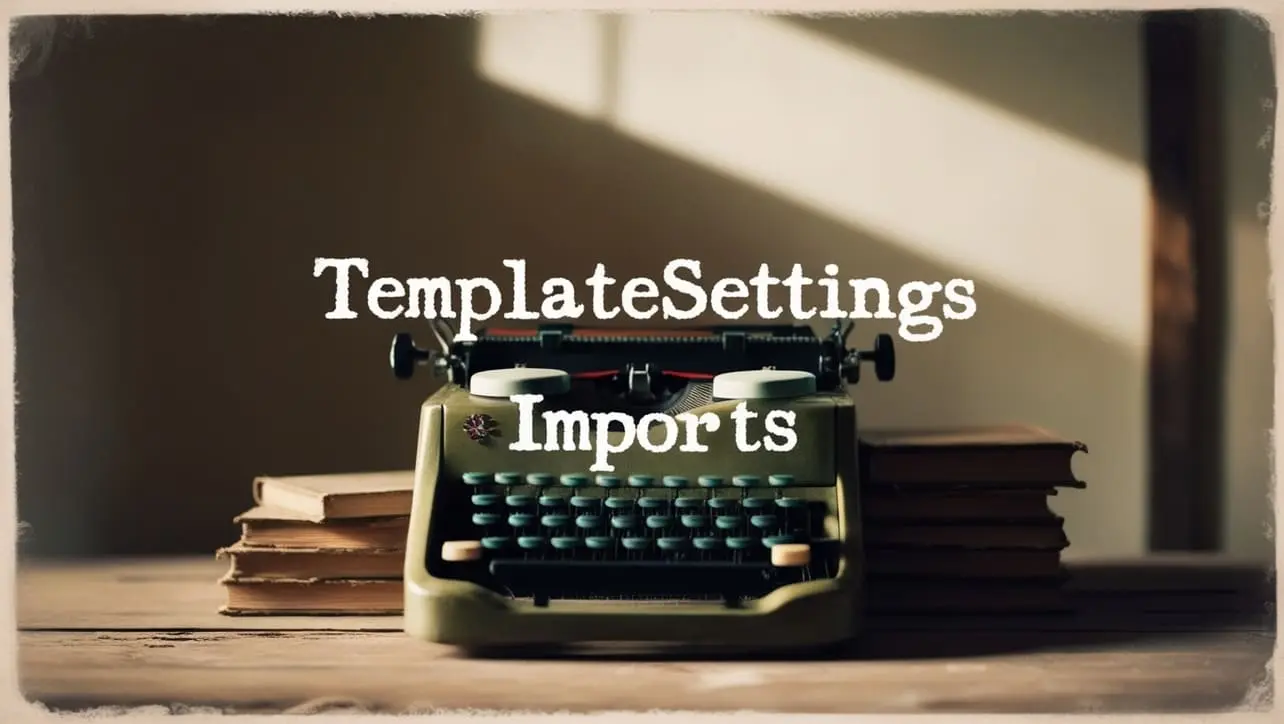
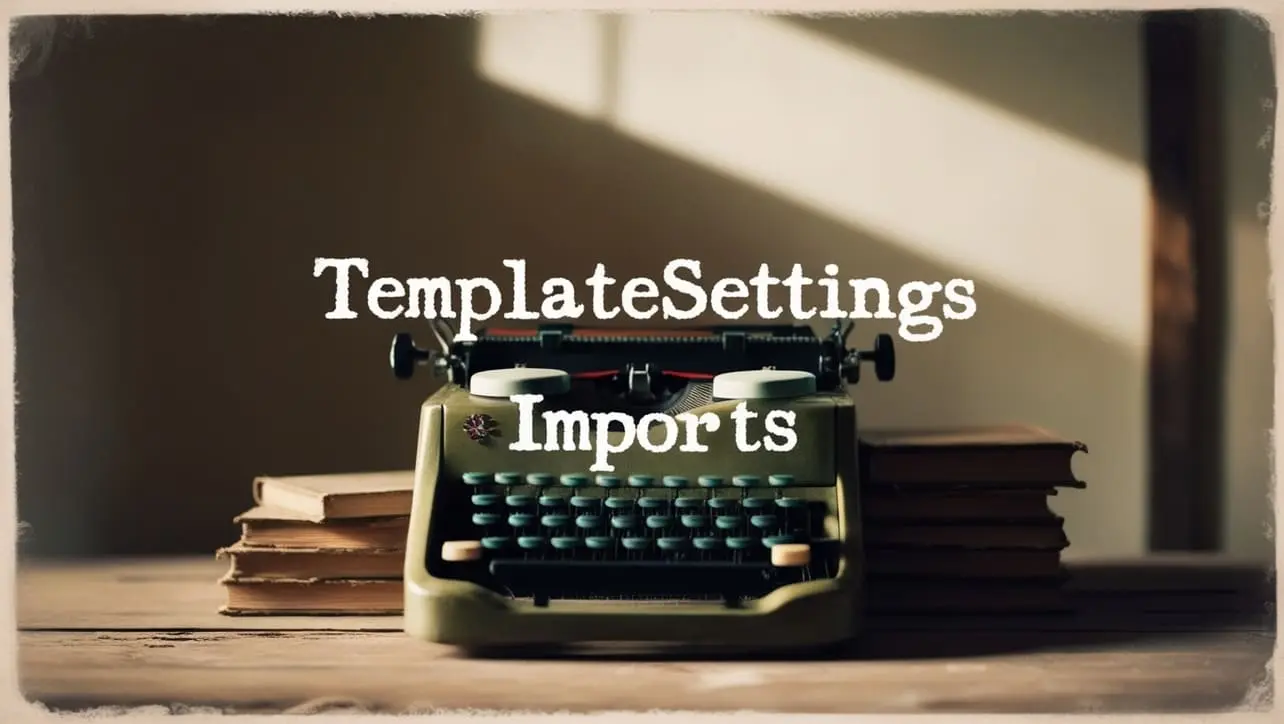
Lodash _.templateSettings.imports Property
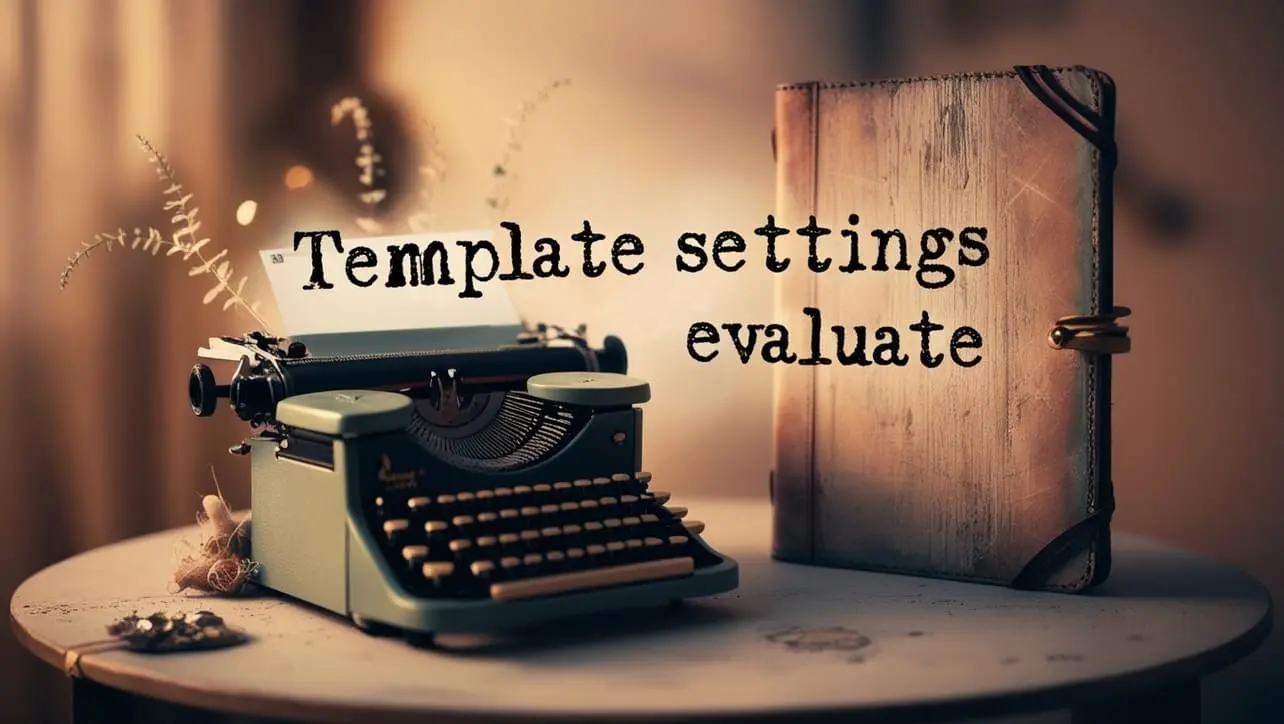
Lodash _.templateSettings.evaluate Property
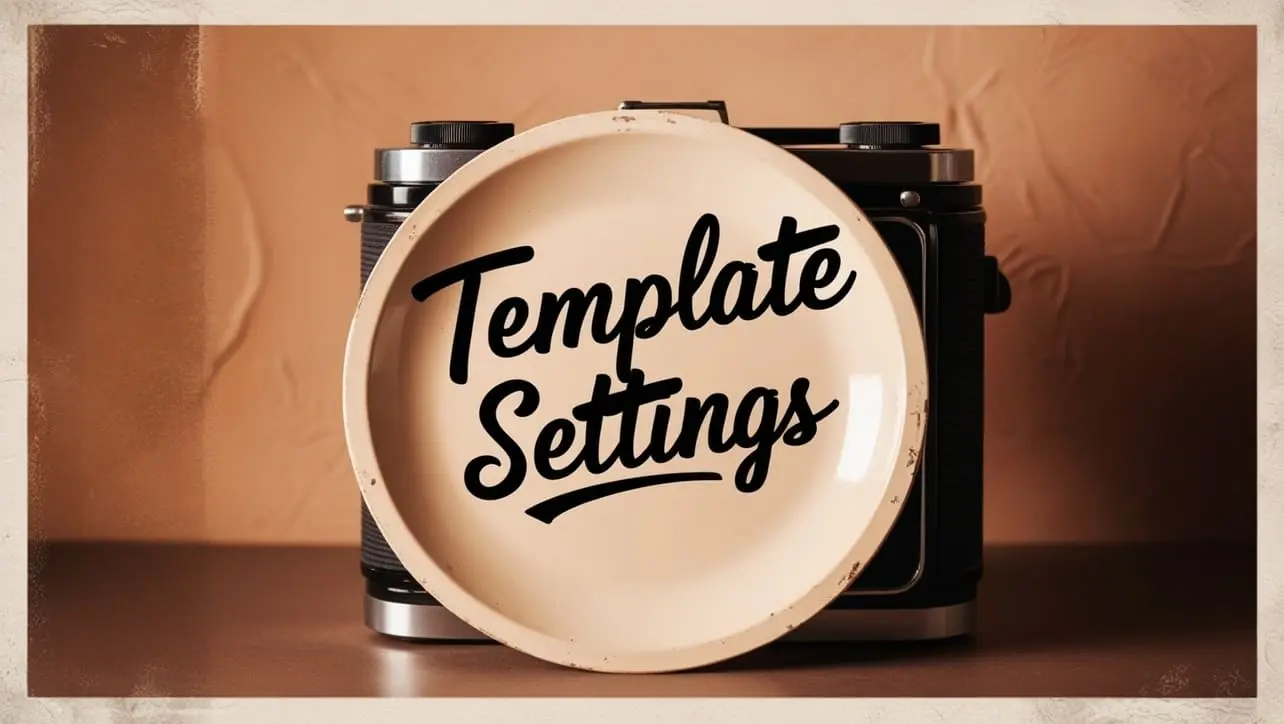
Lodash _.templateSettings Property
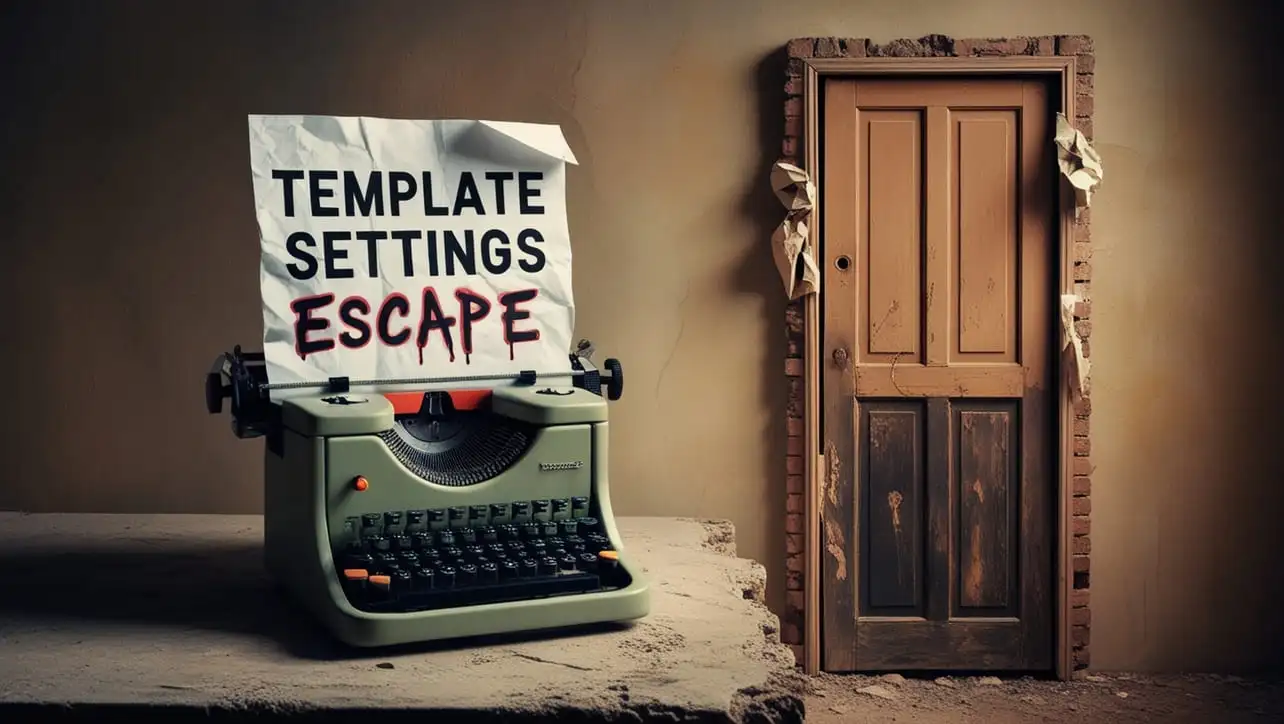
Lodash _.templateSettings.escape Property
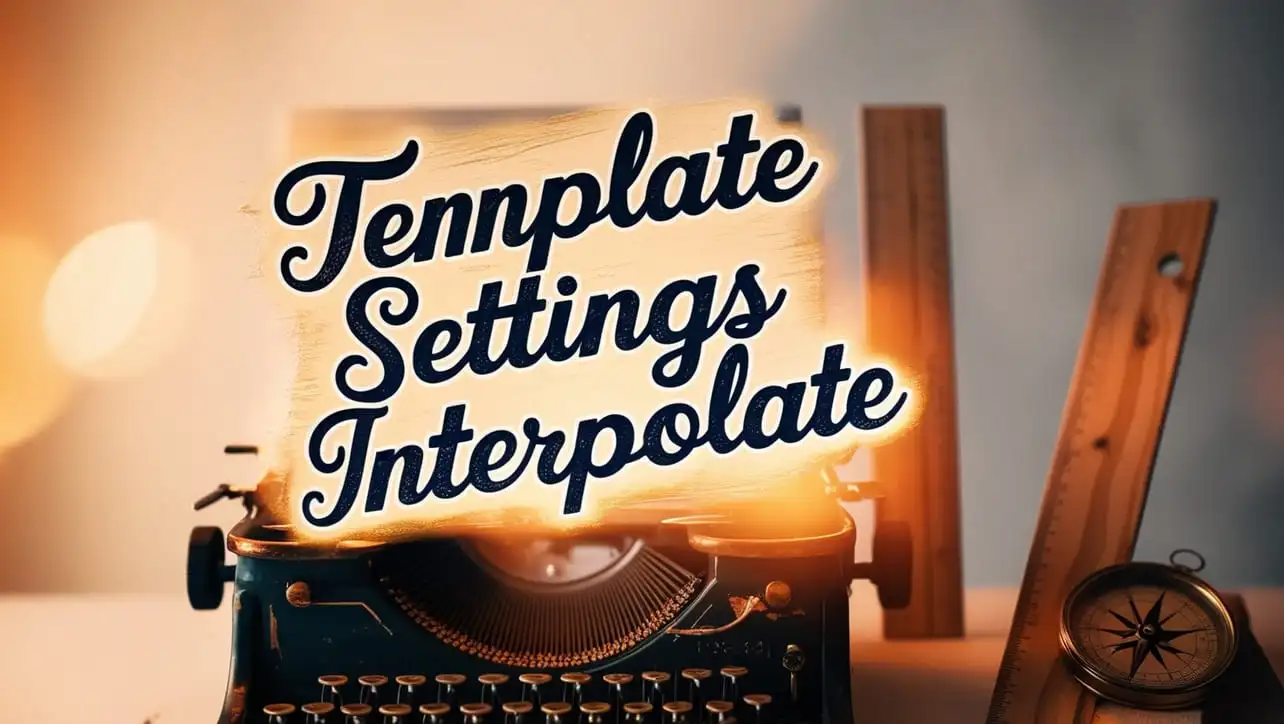
Lodash _.templateSettings.interpolate Property
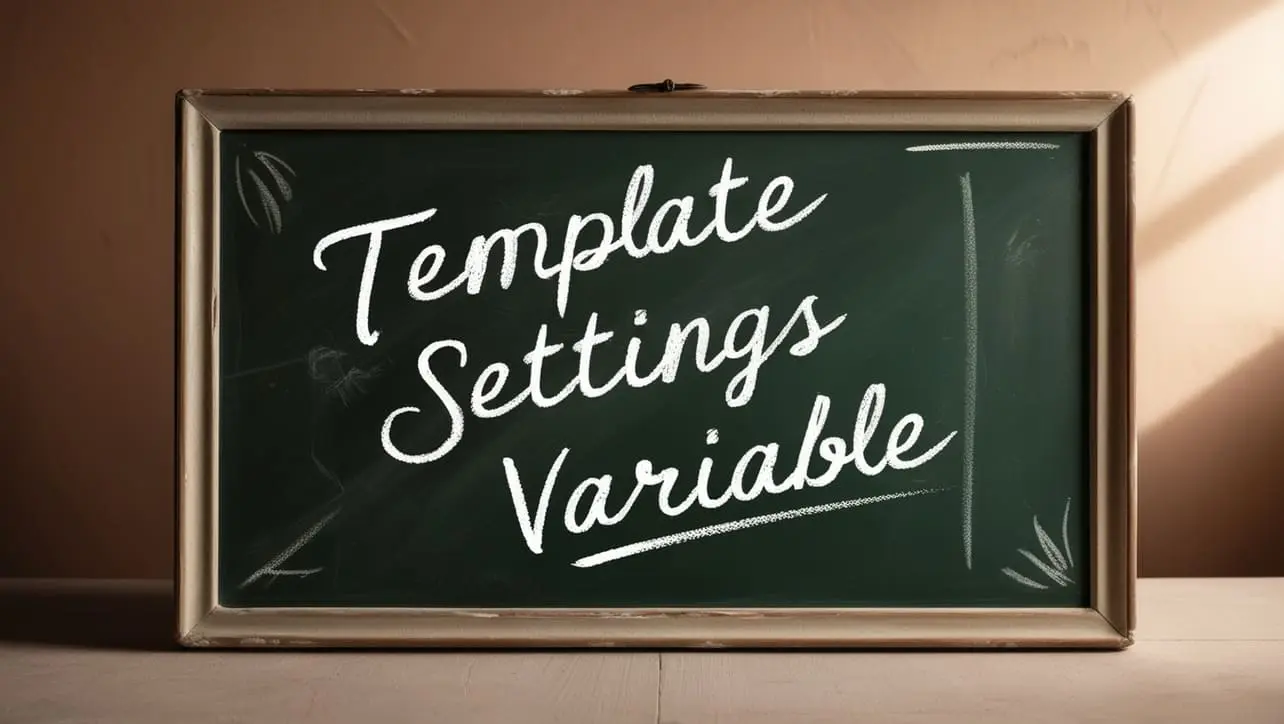
If you have any doubts regarding this article (Lodash _.map() Collection Method), please comment here. I will help you immediately.