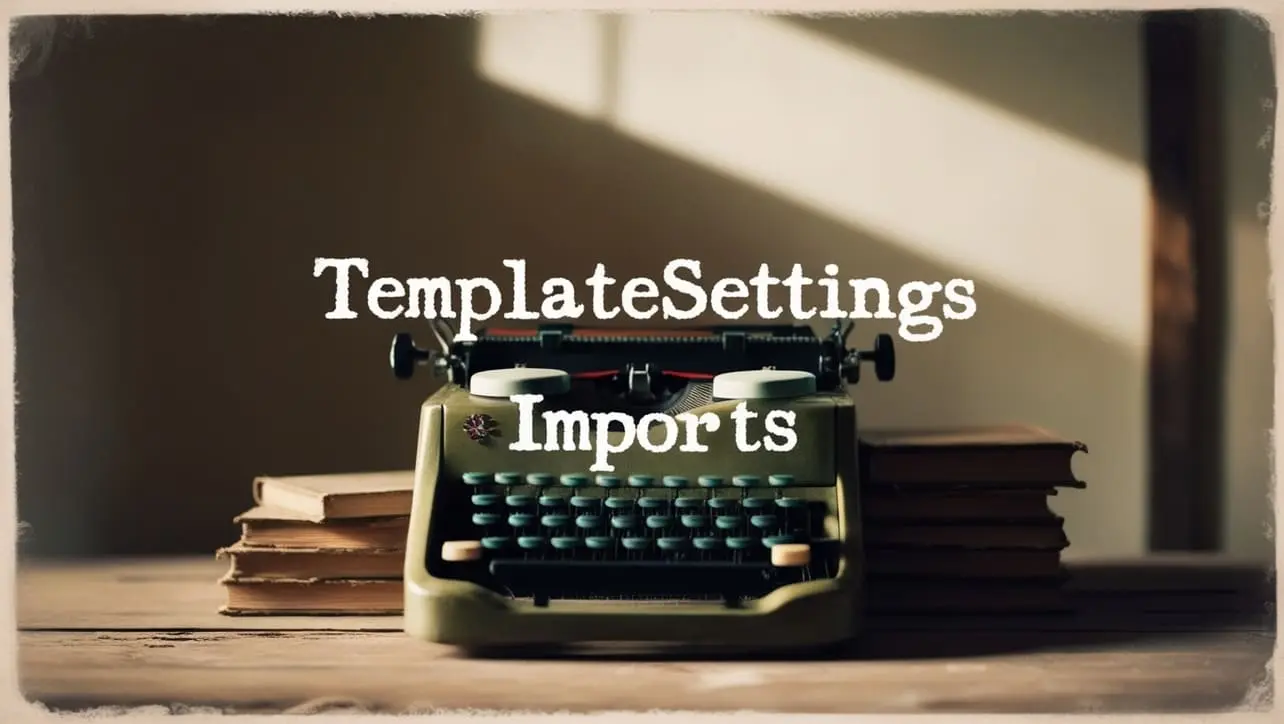
Lodash _.forEachRight() Collection Method
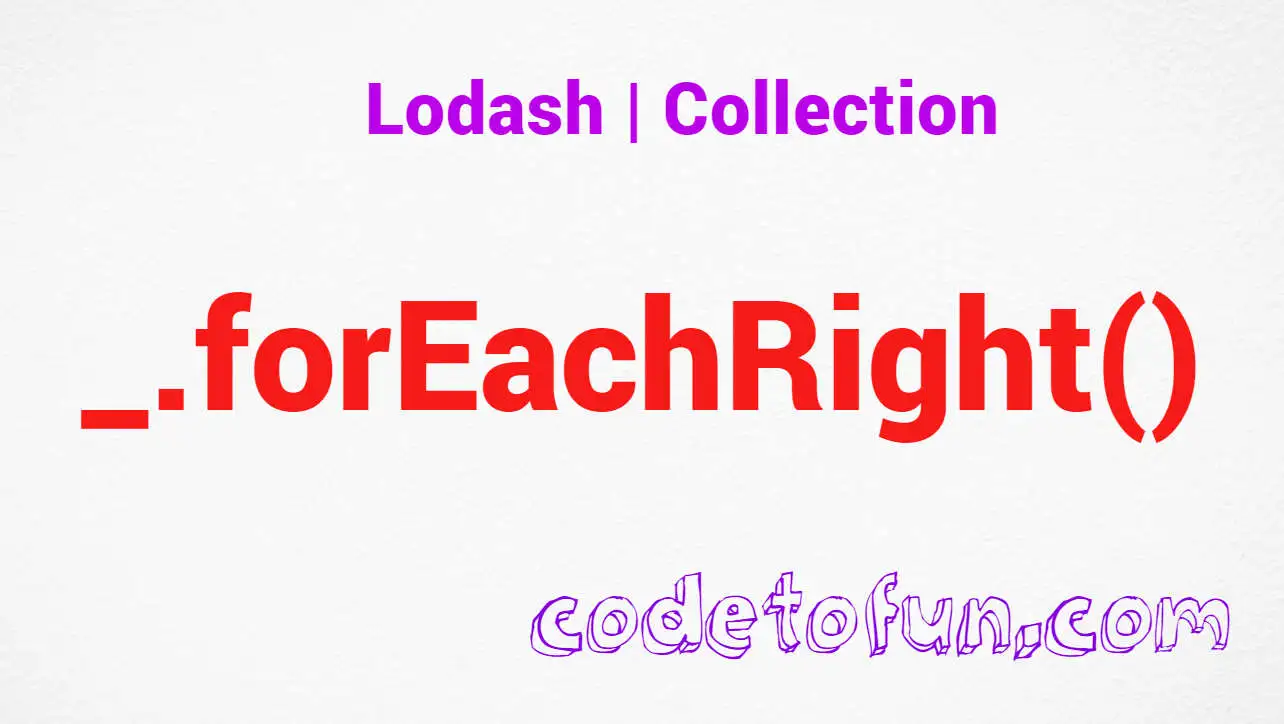
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript, effective iteration over collections is a common necessity. Lodash, a powerful utility library, provides a variety of methods to streamline these tasks. Among them, the _.forEachRight()
method stands out, offering a convenient way to iterate over elements in reverse order.
This method proves invaluable for scenarios where processing elements in a collection from the end to the beginning is crucial.
🧠 Understanding _.forEachRight()
The _.forEachRight()
method in Lodash is tailored for iterating over elements in a collection, such as arrays or objects, in reverse order. It executes a provided function once for each element, providing a flexible and intuitive way to perform operations on the elements.
💡 Syntax
_.forEachRight(collection, iteratee)
- collection: The collection to iterate over.
- iteratee: The function invoked per iteration.
📝 Example
Let's explore a practical example to illustrate the functionality of _.forEachRight()
:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5];
_.forEachRight(numbers, (value, index) => {
console.log(`Element at index ${index}: ${value}`);
});
In this example, the _.forEachRight()
method is used to iterate over the numbers array in reverse order, logging each element along with its index.
🏆 Best Practices
Reverse Iteration:
Leverage
_.forEachRight()
when the order of iteration matters, and processing elements from the end to the beginning is essential.example.jsCopiedconst words = ['apple', 'banana', 'orange']; _.forEachRight(words, word => { console.log(word.split('').reverse().join('')); }); // Output: "egap", "ananab", "egnaro"
Modifying the Original Collection:
Exercise caution when modifying the original collection inside the iteratee function to avoid unexpected behavior.
example.jsCopiedconst items = [1, 2, 3, 4, 5]; _.forEachRight(items, (value, index, collection) => { if (value % 2 === 0) { // Remove even numbers from the original array collection.splice(index, 1); } }); console.log(items); // Output: [5, 3, 1]
Utilizing Index Parameter:
Take advantage of the optional index parameter provided to the iteratee function when the index of the element is needed during processing.
example.jsCopiedconst colors = ['red', 'green', 'blue']; _.forEachRight(colors, (color, index) => { console.log(`Color at position ${index}: ${color}`); }); // Output: "Color at position 2: blue", "Color at position 1: green", "Color at position 0: red"
📚 Use Cases
Reversing Array Elements:
_.forEachRight()
is useful when you need to process array elements in reverse order, which is common in scenarios like reversing strings or arrays.example.jsCopiedconst originalArray = [1, 2, 3, 4, 5]; const reversedArray = []; _.forEachRight(originalArray, value => { reversedArray.push(value); }); console.log(reversedArray); // Output: [5, 4, 3, 2, 1]
Removing Elements Conditionally:
When you want to remove elements from an array based on a condition,
_.forEachRight()
allows you to do so while maintaining correct indices.example.jsCopiedconst numbersToRemove = [1, 2, 3, 4, 5]; const condition = number => number % 2 === 0; _.forEachRight(numbersToRemove, (value, index, collection) => { if (condition(value)) { collection.splice(index, 1); } }); console.log(numbersToRemove); // Output: [5, 3, 1]
Processing Object Properties:
Iterating over object properties in reverse order can be achieved using
_.forEachRight()
, providing flexibility in property processing.example.jsCopiedconst person = { name: 'John', age: 30, city: 'New York', }; _.forEachRight(person, (value, key) => { console.log(`${key}: ${value}`); }); // Output: "city: New York", "age: 30", "name: John"
🎉 Conclusion
The _.forEachRight()
method in Lodash offers a convenient and efficient way to iterate over elements in reverse order within a collection. Whether you need to reverse array elements, conditionally remove elements, or process object properties in reverse, this method provides a versatile solution for diverse iteration needs in JavaScript.
Enhance your collection iteration experience with _.forEachRight()
and unlock new possibilities in your JavaScript development!
👨💻 Join our Community:
Author
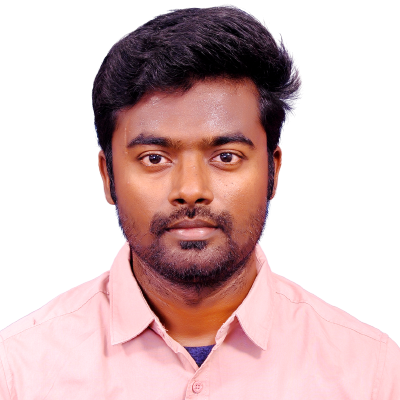
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
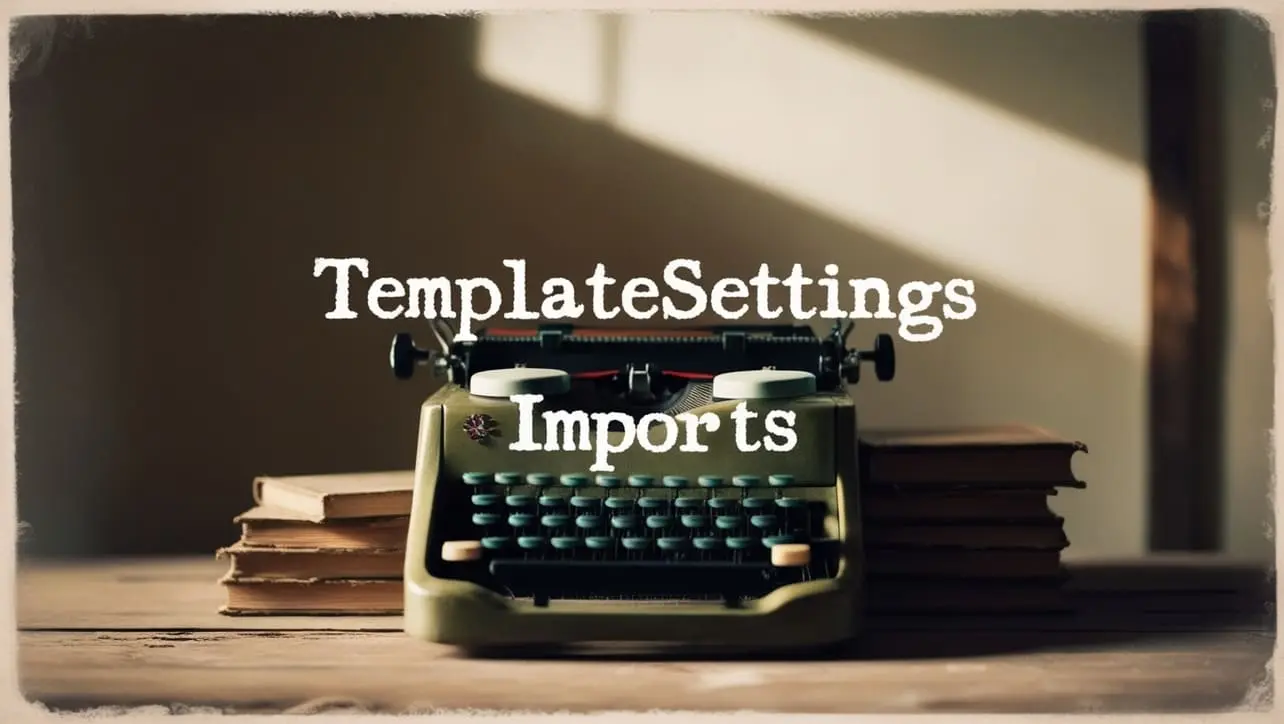
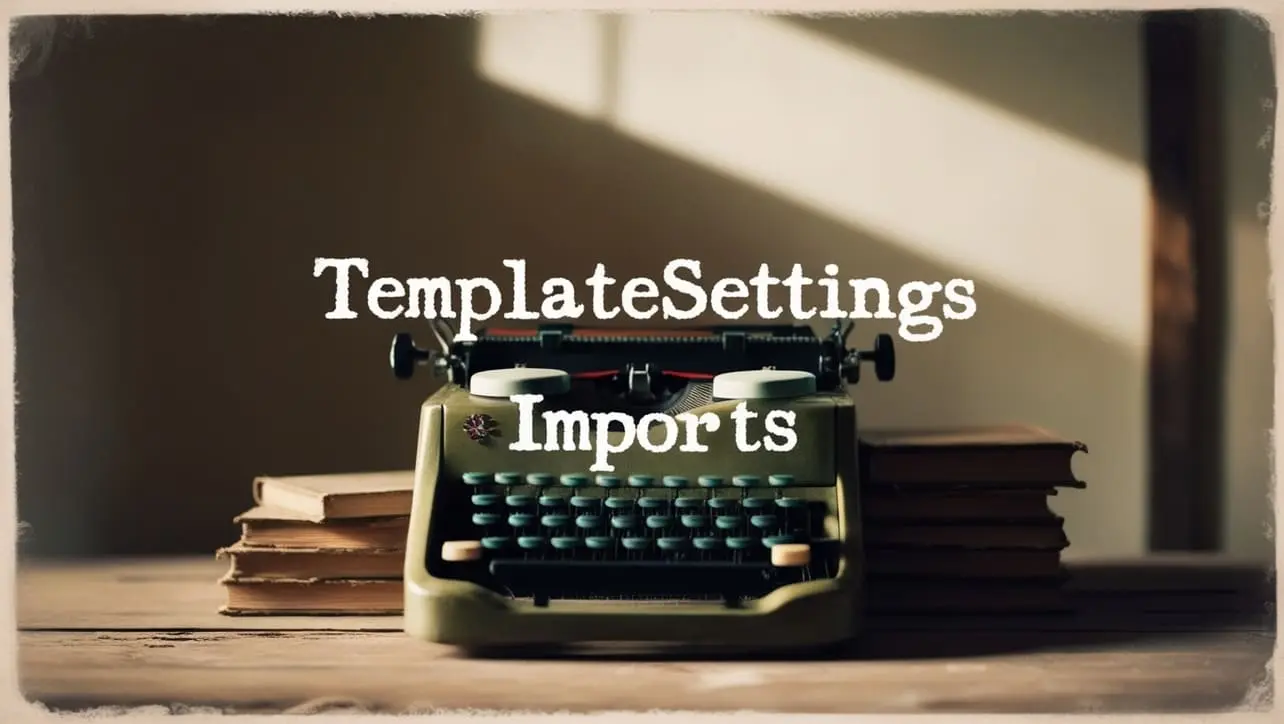
Lodash _.templateSettings.imports Property
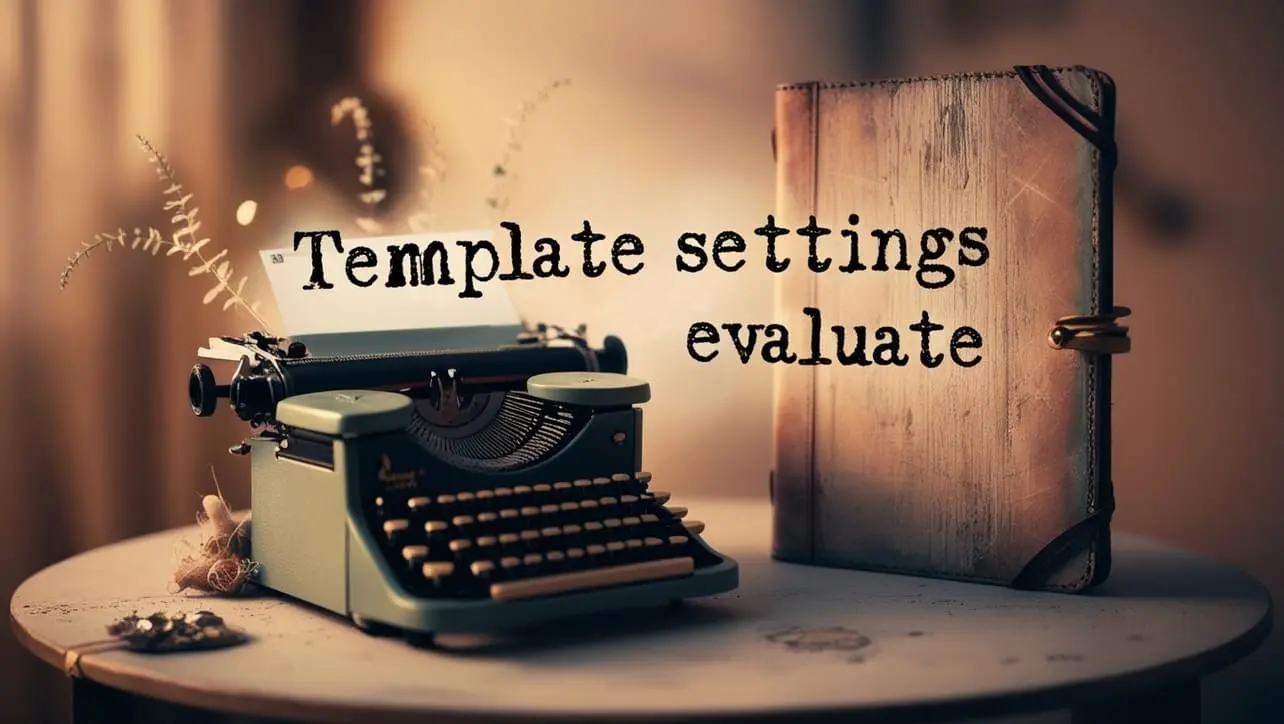
Lodash _.templateSettings.evaluate Property
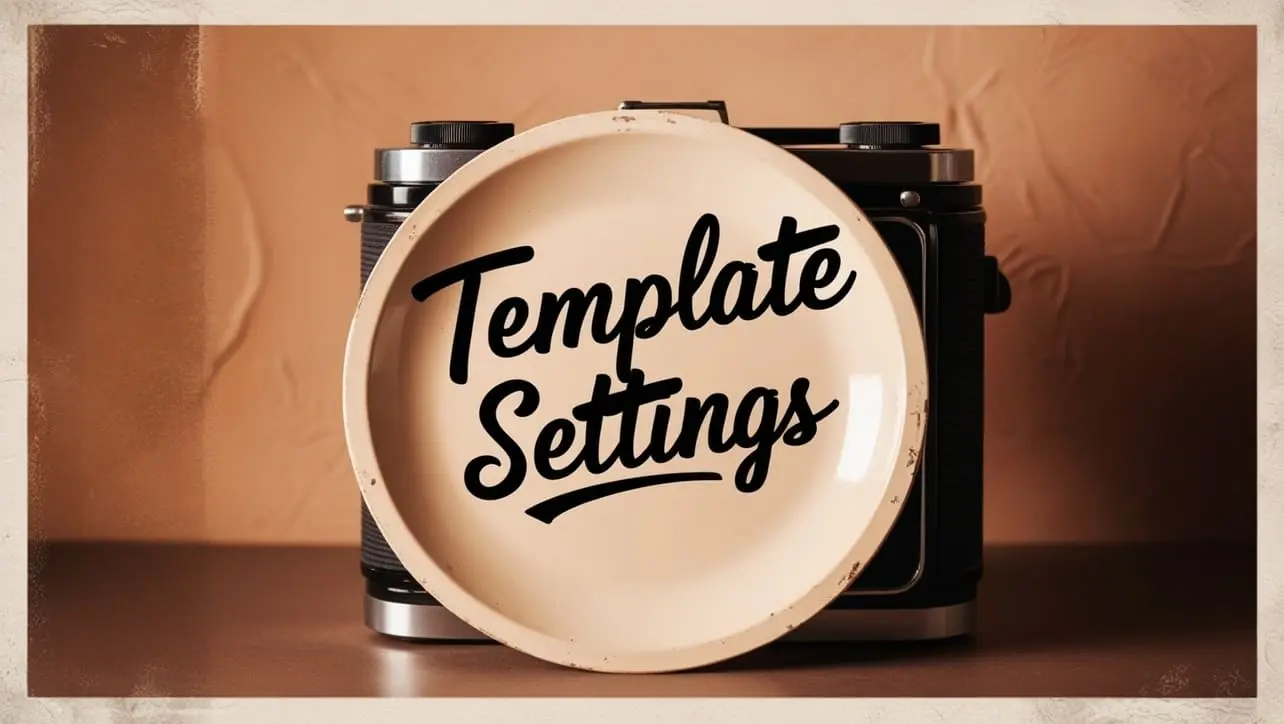
Lodash _.templateSettings Property
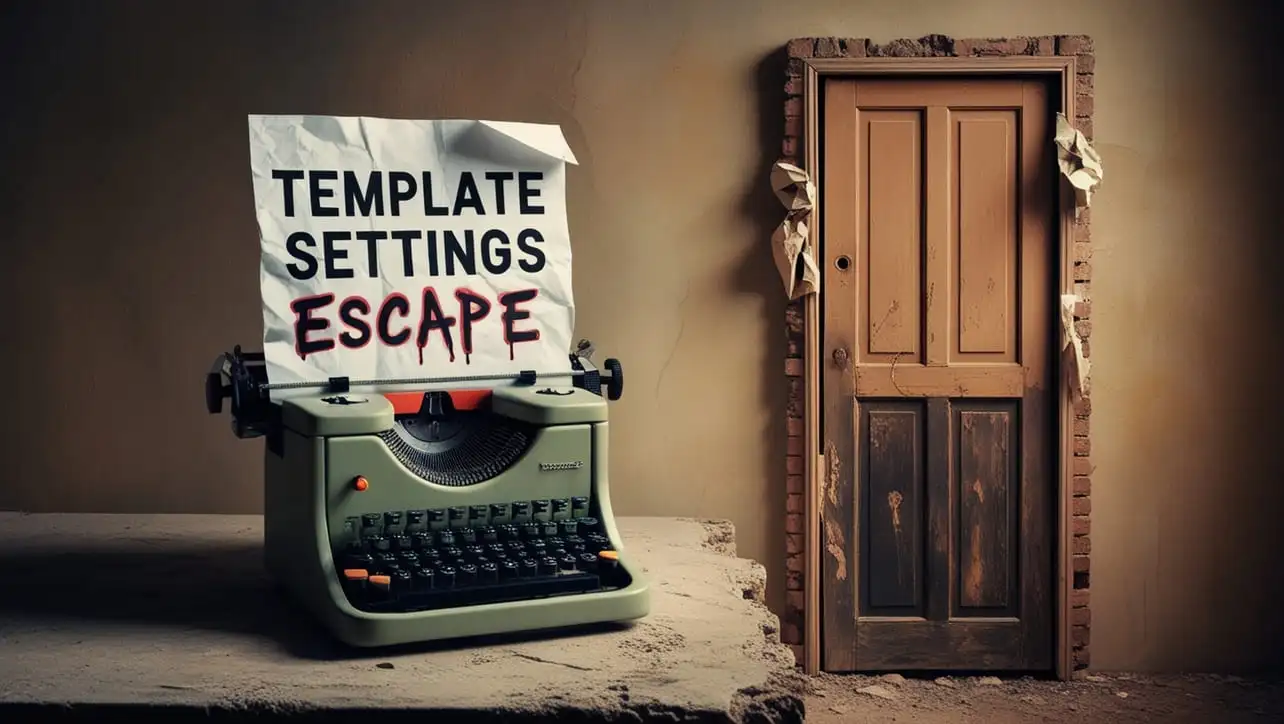
Lodash _.templateSettings.escape Property
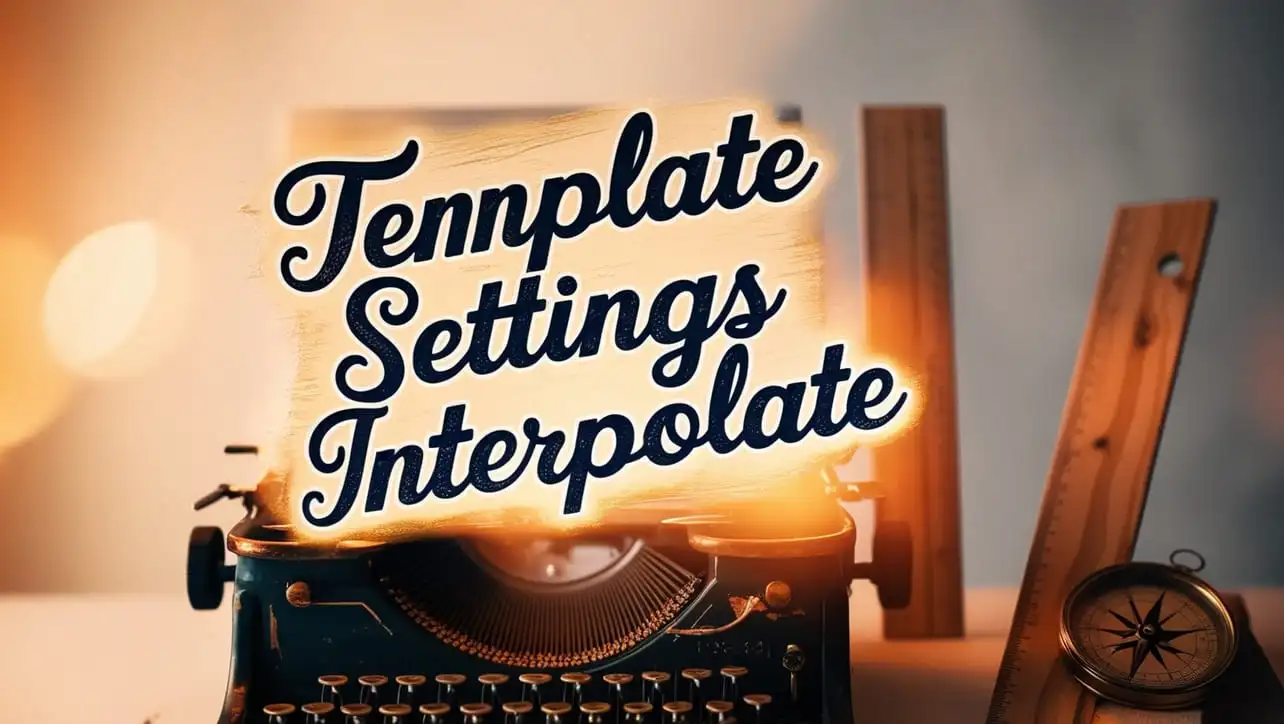
Lodash _.templateSettings.interpolate Property
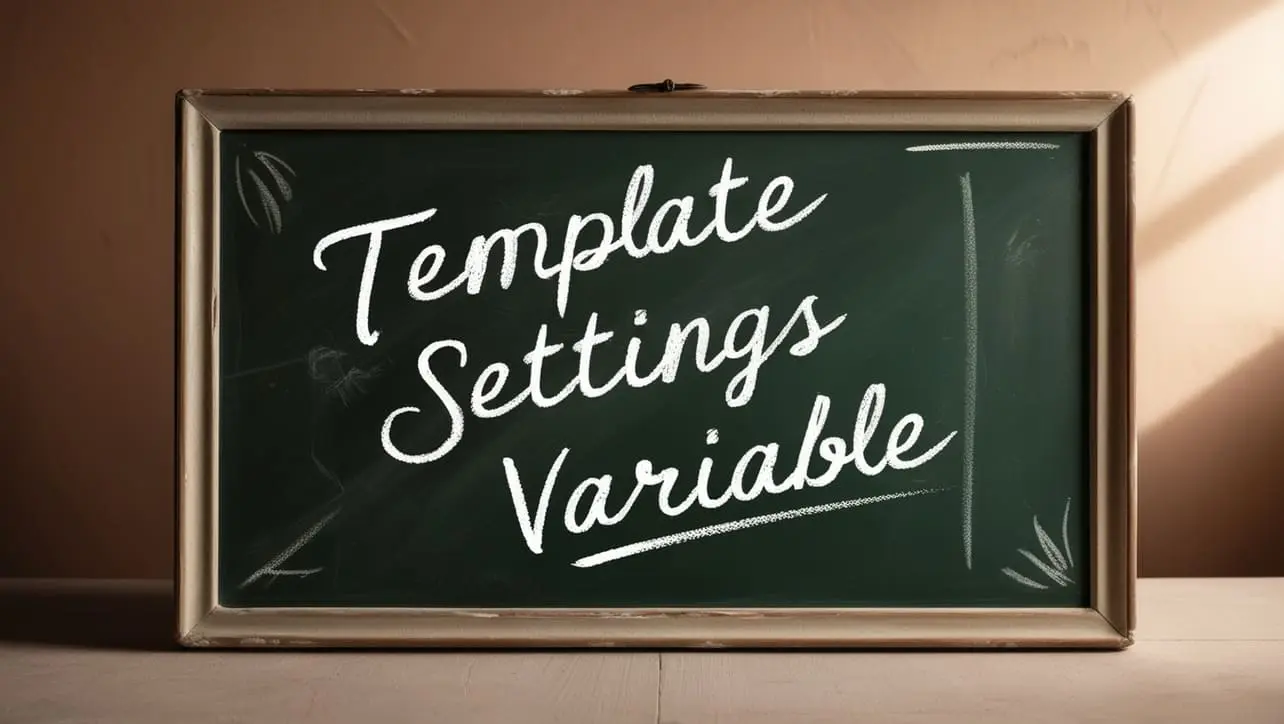
If you have any doubts regarding this article (Lodash _.forEachRight() Collection Method), please comment here. I will help you immediately.