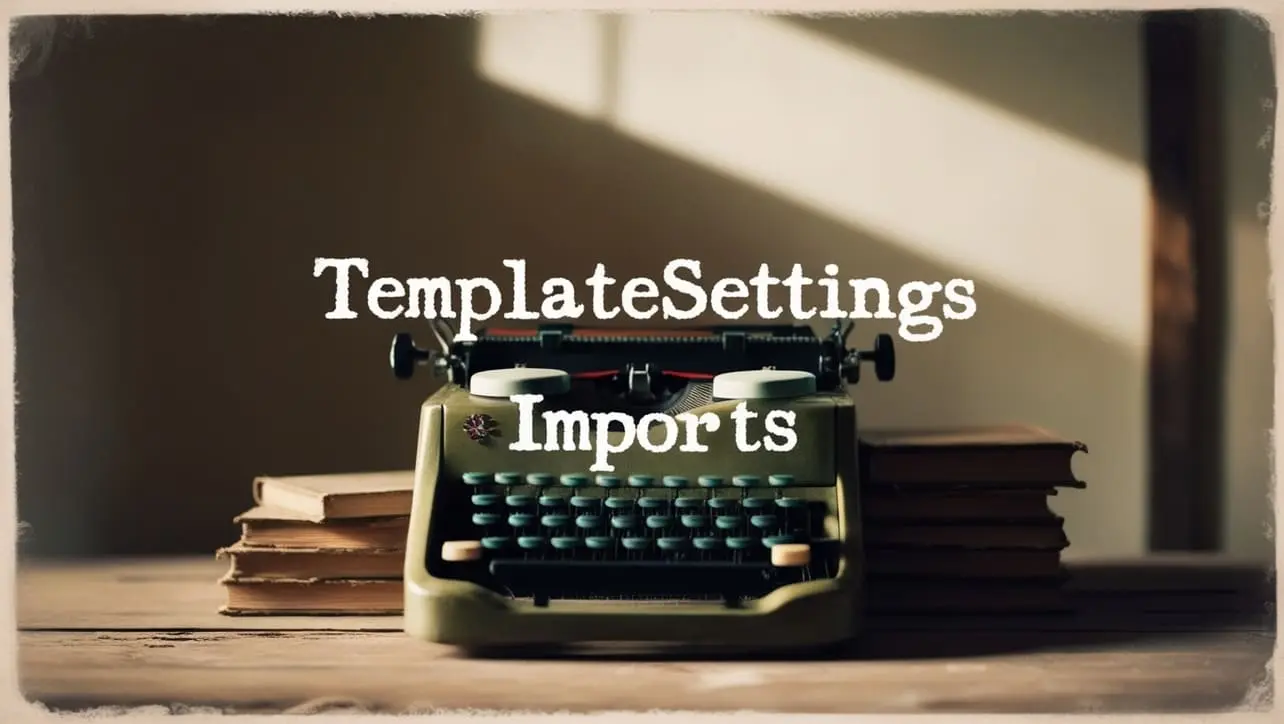
Lodash _.flatMapDeep() Collection Method
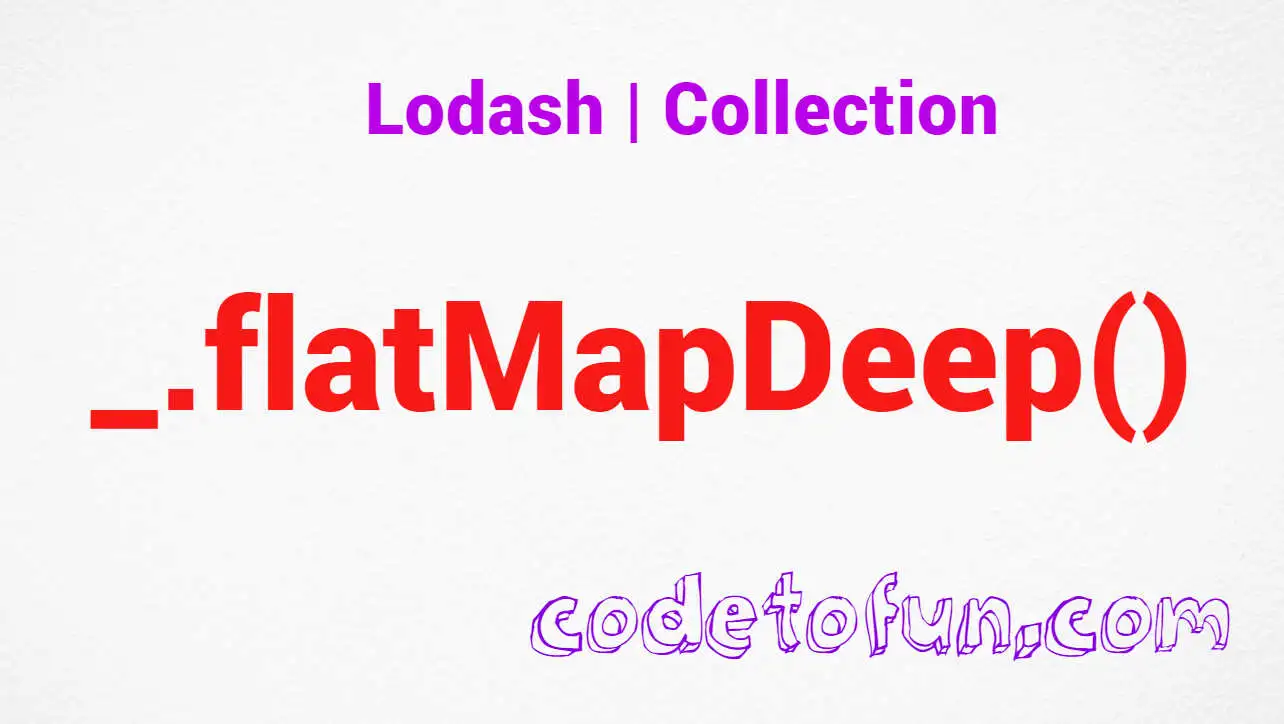
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of JavaScript programming, efficient handling of collections is a common requirement. Lodash, a comprehensive utility library, offers a multitude of functions for simplifying complex tasks. Among these functions, the _.flatMapDeep()
method stands out as a powerful tool for working with nested arrays or collections.
This method not only flattens arrays but also recursively flattens nested arrays, providing flexibility and ease in handling deeply nested structures.
🧠 Understanding _.flatMapDeep()
The _.flatMapDeep()
method in Lodash is designed to both flatten and recursively flatten arrays within arrays, creating a single-level array. This is particularly useful when dealing with deeply nested data structures or arrays of varying depths.
💡 Syntax
_.flatMapDeep(collection, [iteratee])
- collection: The collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's delve into a practical example to understand the power of _.flatMapDeep()
:
const _ = require('lodash');
const nestedArray = [1, [2, [3, [4, 5]]], 6];
const flattenedArray = _.flatMapDeep(nestedArray);
console.log(flattenedArray);
// Output: [1, 2, 3, 4, 5, 6]
In this example, the nestedArray is processed by _.flatMapDeep()
, resulting in a flattened array containing all elements at a single level.
🏆 Best Practices
Understand Nested Structures:
Ensure a clear understanding of your data's nested structures before applying
_.flatMapDeep()
. Knowing the depth and complexity of your data helps you use this method effectively.example.jsCopiedconst deeplyNestedData = [1, [2, [3, [4, [5, [6]]]]]]; const flattenedData = _.flatMapDeep(deeplyNestedData); console.log(flattenedData); // Output: [1, 2, 3, 4, 5, 6]
Utilize Iteratee for Transformation:
Leverage the iteratee function to perform transformations on elements during the flattening process. This is useful when you need to modify or extract specific values.
example.jsCopiedconst dataToTransform = [1, [2, 3], 4]; const transformedData = _.flatMapDeep(dataToTransform, value => [value * 2]); console.log(transformedData); // Output: [2, 4, 6, 8]
Handle Edge Cases:
Consider edge cases, such as empty arrays or arrays with varying depths, and implement appropriate error handling or default behaviors.
example.jsCopiedconst edgeCaseArray = [1, [2, [3, []], 4]]; const handledEdgeCase = _.flatMapDeep(edgeCaseArray, value => { if (Array.isArray(value)) { return value; // Include only non-empty arrays } return [value]; }); console.log(handledEdgeCase); // Output: [1, 2, 3, 4]
📚 Use Cases
Flattening Nested Arrays:
_.flatMapDeep()
is particularly useful when dealing with nested arrays, as it ensures all elements are brought to a single level, simplifying subsequent operations.example.jsCopiedconst deeplyNestedArray = [1, [2, [3, [4, 5]]], 6]; const flattenedArray = _.flatMapDeep(deeplyNestedArray); console.log(flattenedArray); // Output: [1, 2, 3, 4, 5, 6]
Recursive Data Processing:
When working with recursive data structures,
_.flatMapDeep()
provides a convenient way to process each element at all levels of nesting.example.jsCopiedconst recursiveData = [1, [2, [3, [4, [5, [6]]]]]]; const processedData = _.flatMapDeep(recursiveData, value => value * 2); console.log(processedData); // Output: [2, 4, 6, 8, 10, 12]
Extracting Values:
Use
_.flatMapDeep()
to extract specific values from nested arrays, simplifying the process of working with complex data structures.example.jsCopiedconst dataToExtract = [1, [2, { key: 'value' }], 3]; const extractedValues = _.flatMapDeep(dataToExtract, value => (typeof value === 'object' ? Object.values(value) : [value])); console.log(extractedValues); // Output: [1, 2, 'value', 3]
🎉 Conclusion
The _.flatMapDeep()
method in Lodash is a versatile tool for working with nested arrays and complex data structures. Whether you need to flatten deeply nested arrays or perform transformations during the process, this method provides a concise and powerful solution.
Explore the capabilities of _.flatMapDeep()
and streamline your collection manipulation tasks with ease in JavaScript!
👨💻 Join our Community:
Author
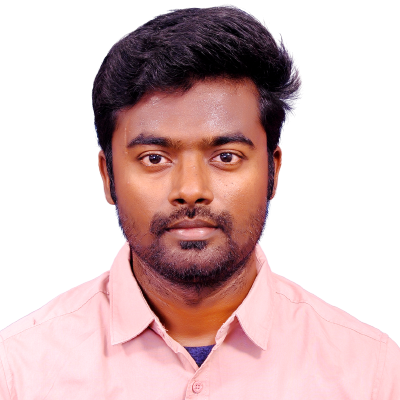
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
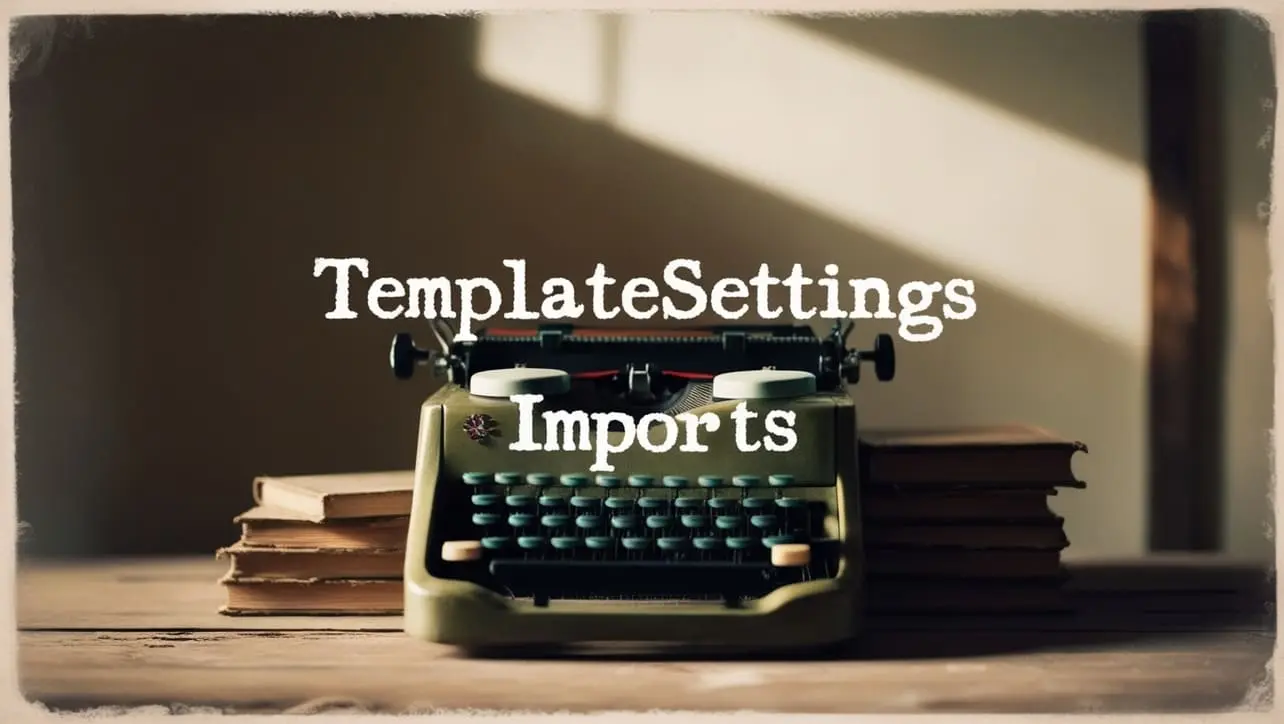
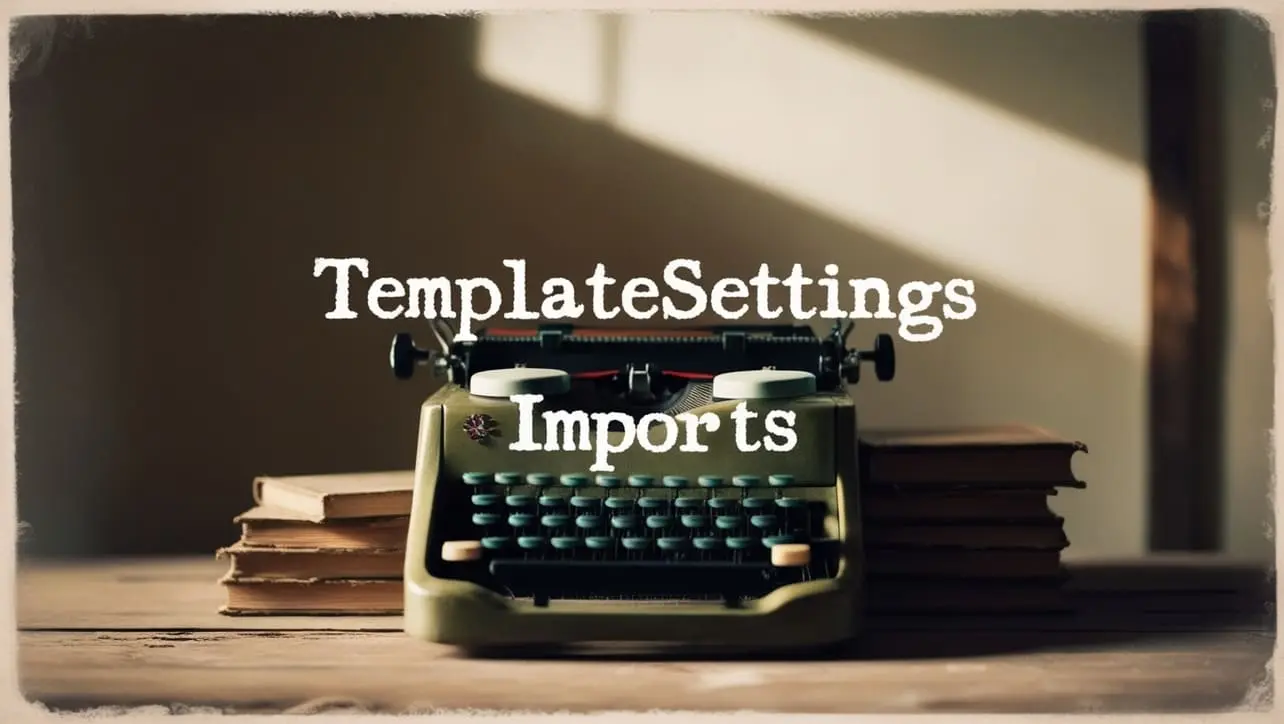
Lodash _.templateSettings.imports Property
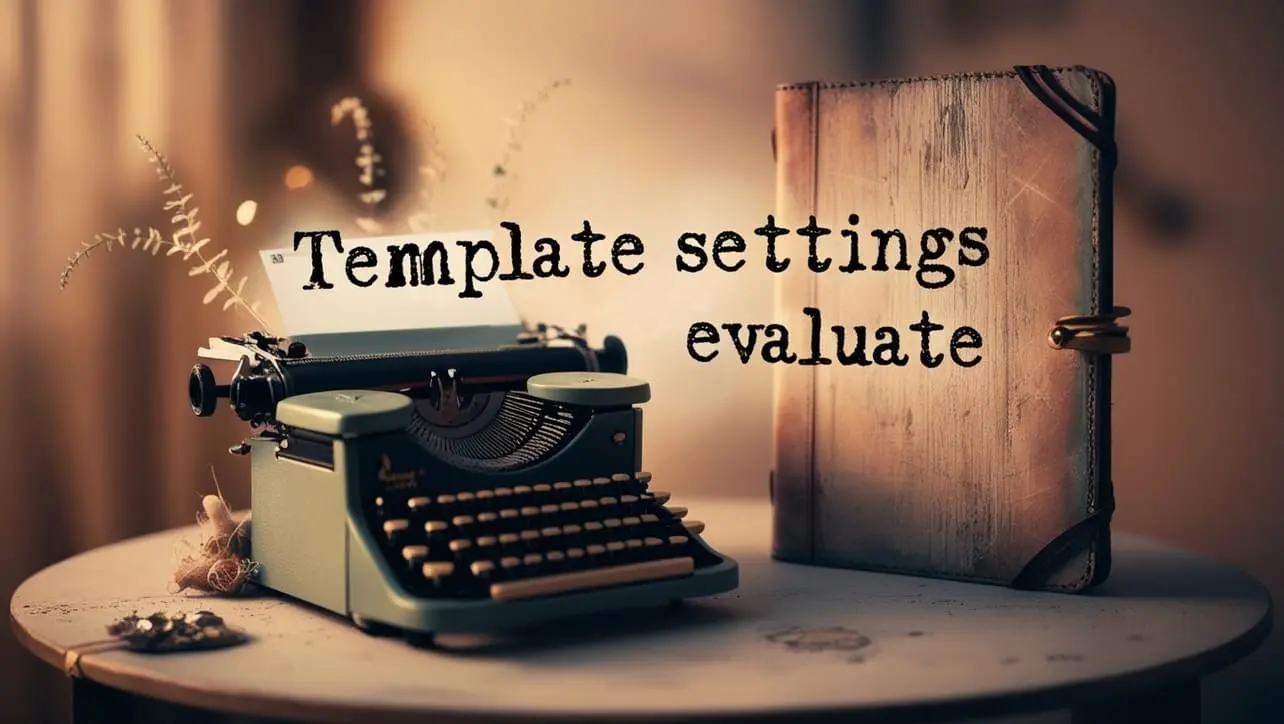
Lodash _.templateSettings.evaluate Property
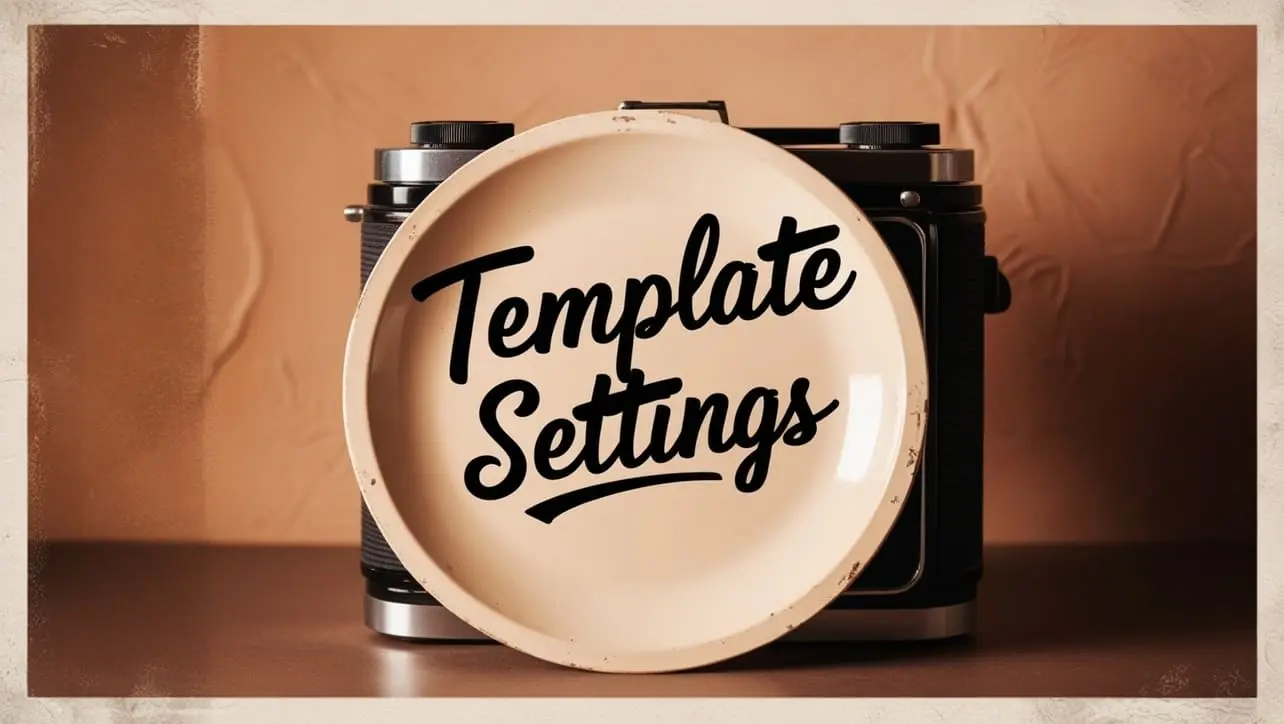
Lodash _.templateSettings Property
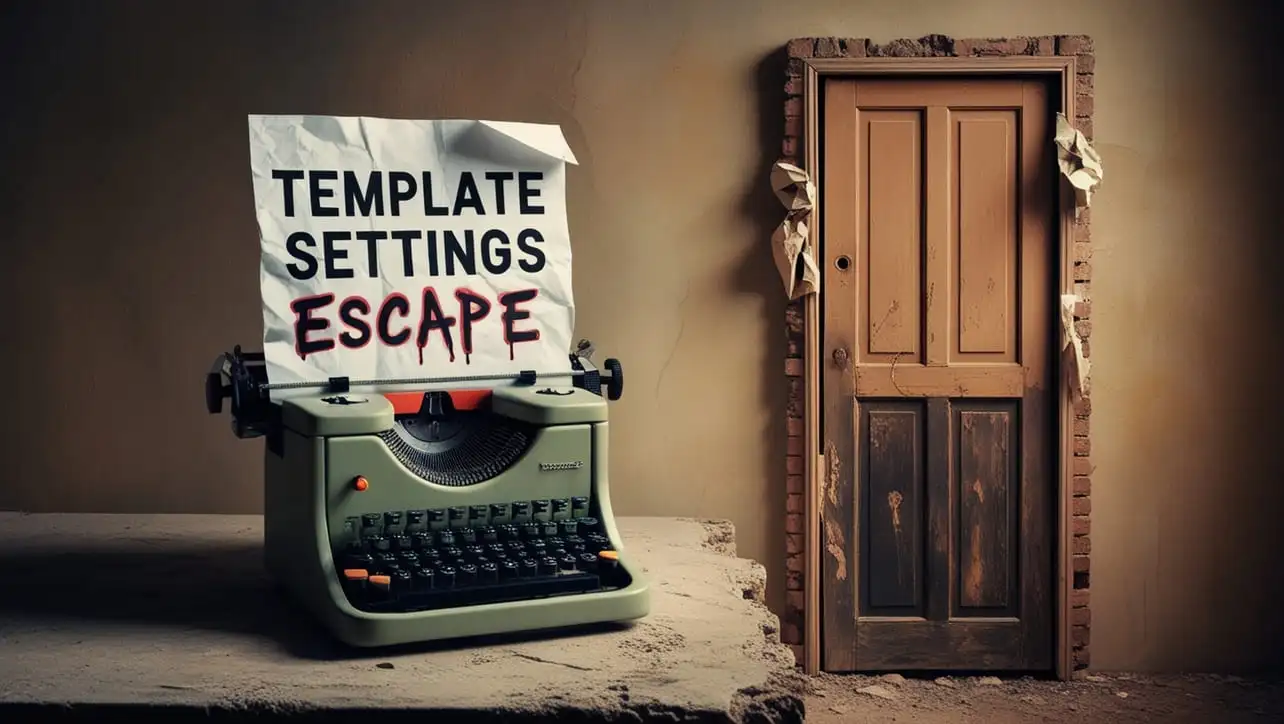
Lodash _.templateSettings.escape Property
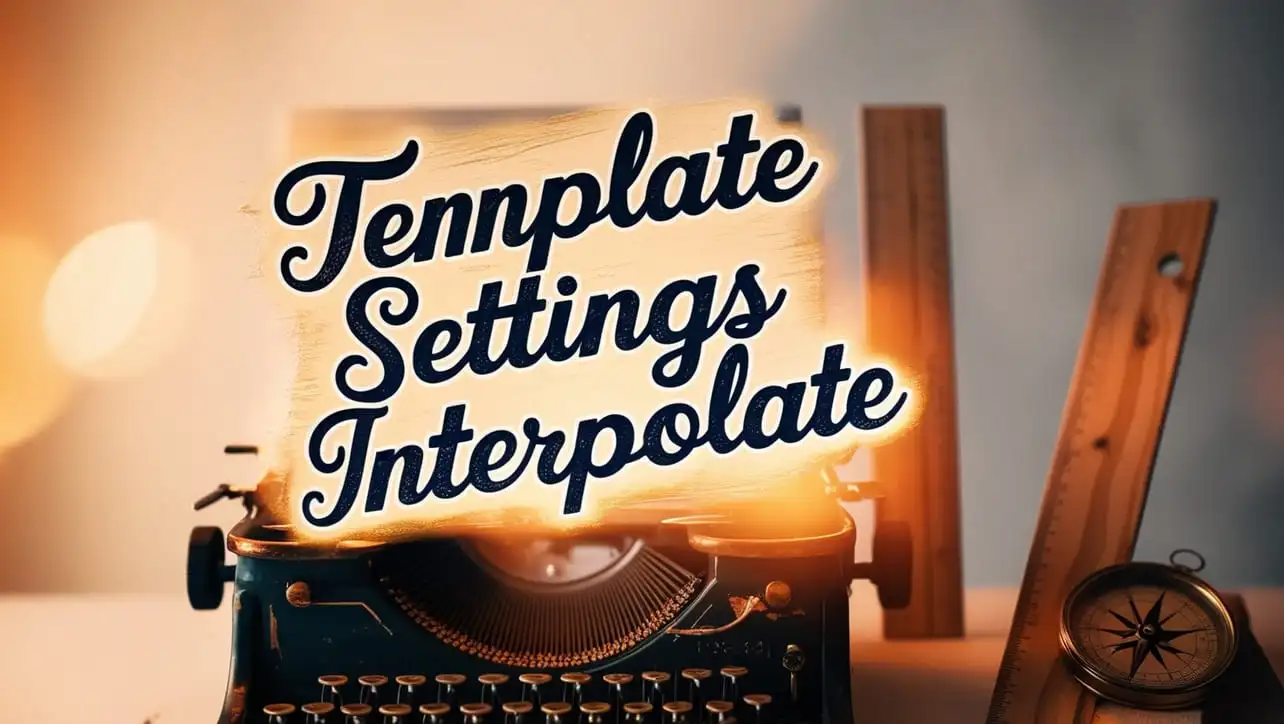
Lodash _.templateSettings.interpolate Property
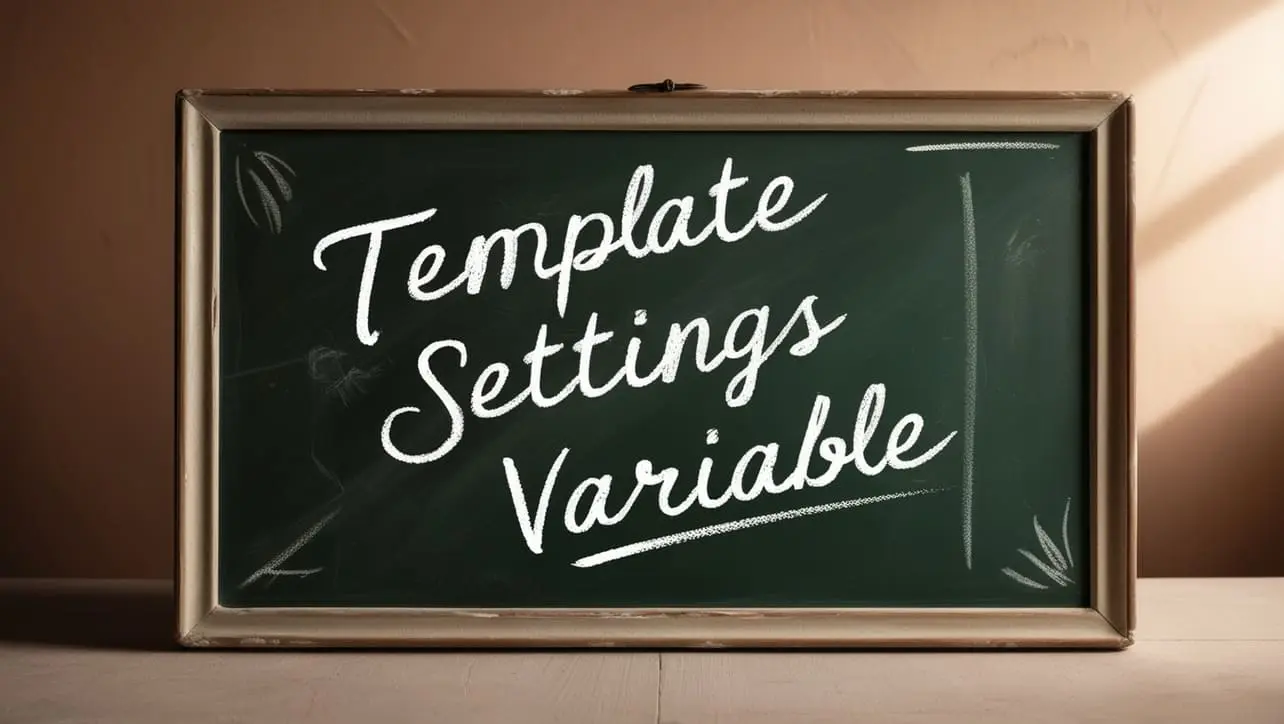
If you have any doubts regarding this article (Lodash _.flatMapDeep() Collection Method), please comment here. I will help you immediately.