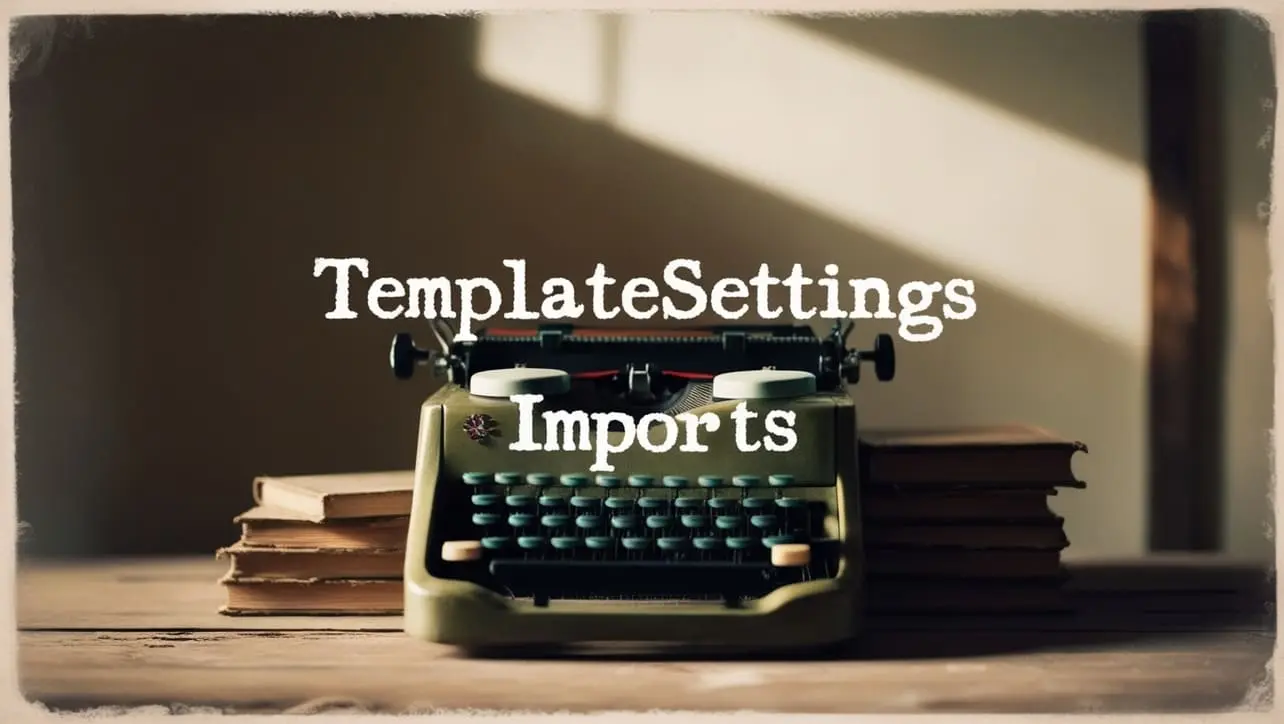
Lodash _.findLast() Collection Method
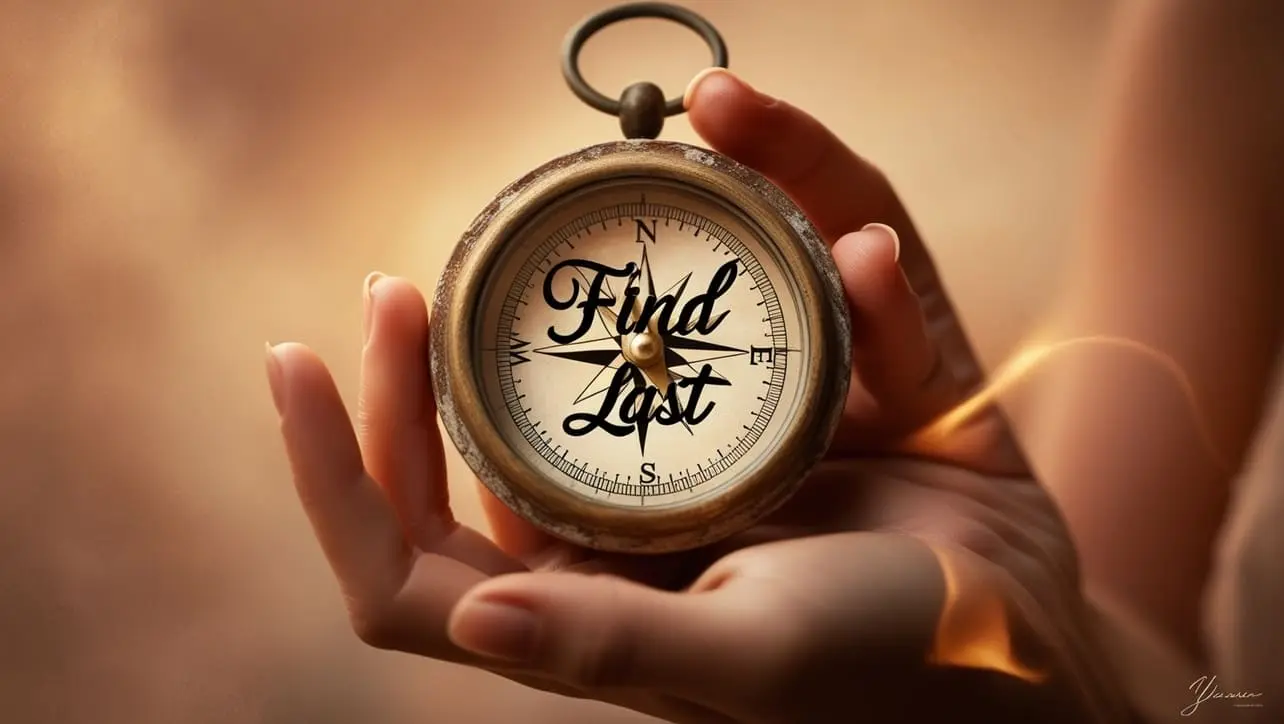
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic world of JavaScript, efficiently searching through collections is a common task. Lodash, a powerful utility library, offers a multitude of functions to streamline such operations. Among these functions is the _.findLast()
method, a versatile tool for searching collections and retrieving the last element that satisfies a given condition.
This method is invaluable for developers looking to enhance their code's readability and efficiency when working with diverse datasets.
🧠 Understanding _.findLast()
The _.findLast()
method in Lodash is designed for searching through a collection and returning the last element that matches a specified condition. Whether you're dealing with arrays of objects or other collections, this method provides a concise and effective way to retrieve the desired element.
💡 Syntax
_.findLast(collection, [predicate], [fromIndex])
- collection: The collection to iterate over.
- predicate (Optional): The function invoked per iteration.
- fromIndex (Optional): The index to start searching from.
📝 Example
Let's explore a practical example to illustrate the functionality of _.findLast()
:
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const lastEvenNumber = _.findLast(numbers, n => n % 2 === 0);
console.log(lastEvenNumber);
// Output: 8
In this example, the _.findLast()
method is applied to the numbers array, searching for the last even number.
🏆 Best Practices
Understand Collection Types:
Be aware of the type of collection you are working with. Whether it's an array, object, or another type of collection, understanding the structure is crucial for effective use of
_.findLast()
.example.jsCopiedconst userProfiles = { alice: { age: 30, active: true }, bob: { age: 25, active: false }, charlie: { age: 35, active: true }, }; const lastActiveUser = _.findLast(userProfiles, user => user.active); console.log(lastActiveUser); // Output: { age: 35, active: true }
Leverage Custom Predicates:
Take advantage of custom predicates to tailor the search criteria according to your requirements. This flexibility allows you to find elements based on specific conditions.
example.jsCopiedconst students = [ { name: 'Alice', grade: 'A' }, { name: 'Bob', grade: 'B' }, { name: 'Charlie', grade: 'A' }, ]; const lastAStudent = _.findLast(students, student => student.grade === 'A'); console.log(lastAStudent); // Output: { name: 'Charlie', grade: 'A' }
Optimize with fromIndex:
When you have information about the collection's structure or know the potential location of the desired element, optimize the search by providing a fromIndex. This can improve performance for large collections.
example.jsCopiedconst numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const lastNumberAfterIndex4 = _.findLast(numbers, n => n > 4, 4); console.log(lastNumberAfterIndex4); // Output: 9
📚 Use Cases
Finding the Latest Element:
_.findLast()
is particularly useful when you need to retrieve the latest or most recent element in a collection based on a specific condition.example.jsCopiedconst logs = [ { timestamp: '2022-01-01', event: 'login' }, { timestamp: '2022-01-02', event: 'logout' }, { timestamp: '2022-01-03', event: 'login' }, ]; const lastLoginEvent = _.findLast(logs, log => log.event === 'login'); console.log(lastLoginEvent); // Output: { timestamp: '2022-01-03', event: 'login' }
Filtering by Condition:
When you need to filter a collection and retrieve the last element that meets a specific condition,
_.findLast()
comes in handy.example.jsCopiedconst temperatures = [20, 22, 25, 18, 23, 20]; const lastHighTemperature = _.findLast(temperatures, temp => temp > 22); console.log(lastHighTemperature); // Output: 23
Conditional Retrieval from Objects:
When working with an array of objects,
_.findLast()
simplifies the process of retrieving the last object that satisfies a particular condition.example.jsCopiedconst products = [ { id: 1, name: 'Laptop', price: 1200 }, { id: 2, name: 'Phone', price: 800 }, { id: 3, name: 'Tablet', price: 400 }, ]; const lastExpensiveProduct = _.findLast(products, product => product.price > 500); console.log(lastExpensiveProduct); // Output: { id: 3, name: 'Tablet', price: 400 }
🎉 Conclusion
The _.findLast()
method in Lodash is a versatile tool for efficiently searching through collections and retrieving the last element that satisfies a given condition. Whether you're working with arrays, objects, or other collections, this method provides a concise and effective solution. By understanding its features and best practices, you can optimize your code for readability and performance.
Harness the power of _.findLast()
in your JavaScript projects and elevate your collection manipulation capabilities!
👨💻 Join our Community:
Author
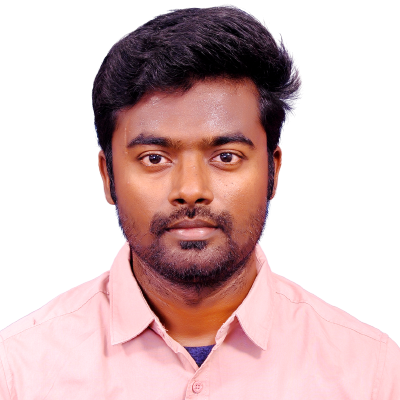
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
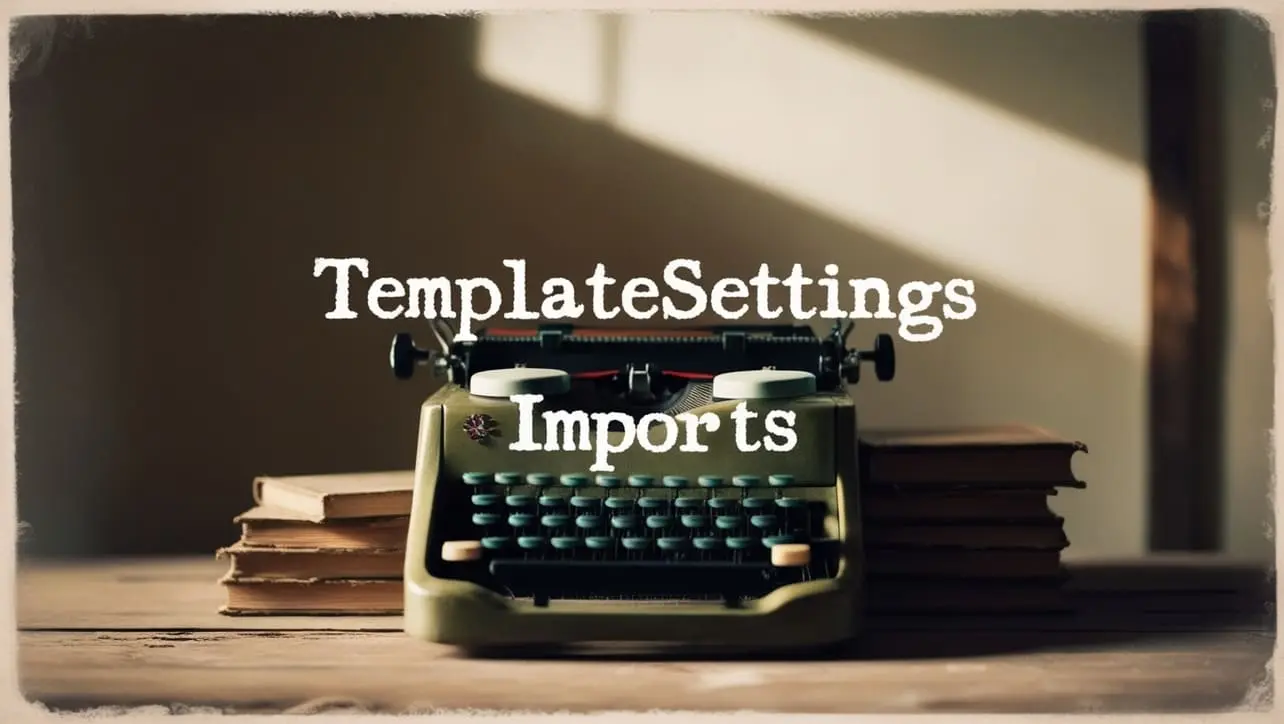
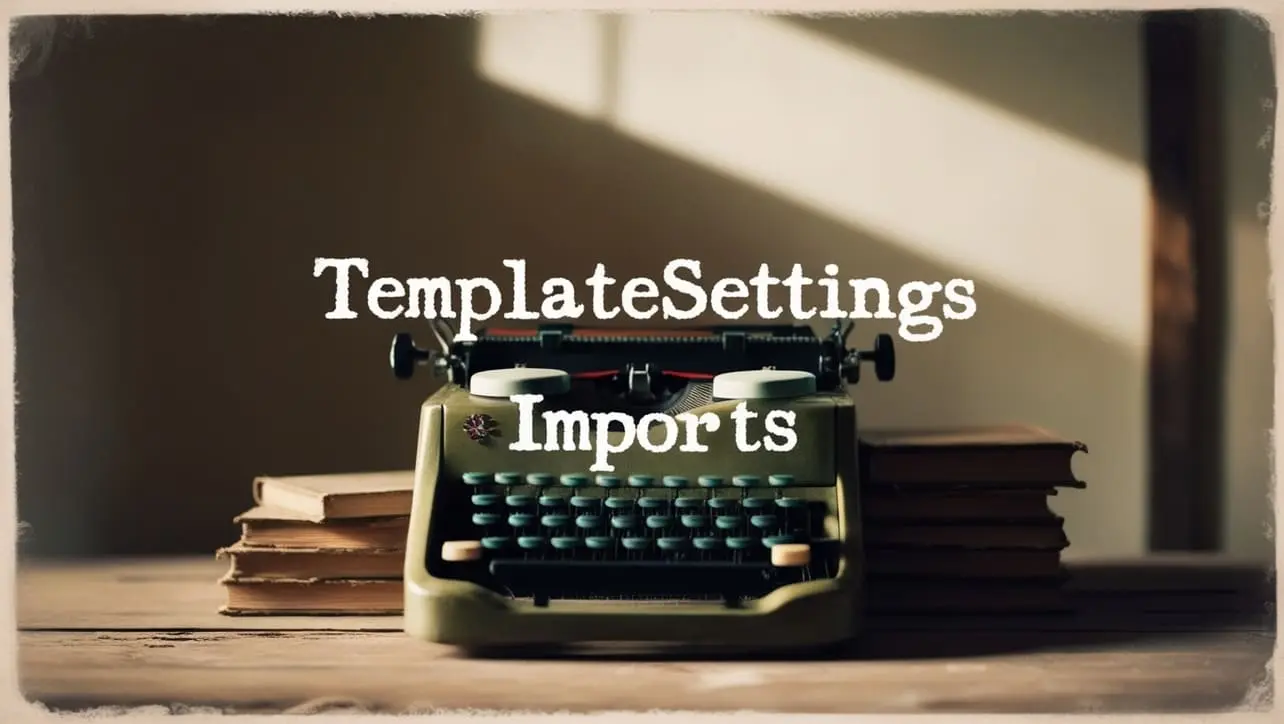
Lodash _.templateSettings.imports Property
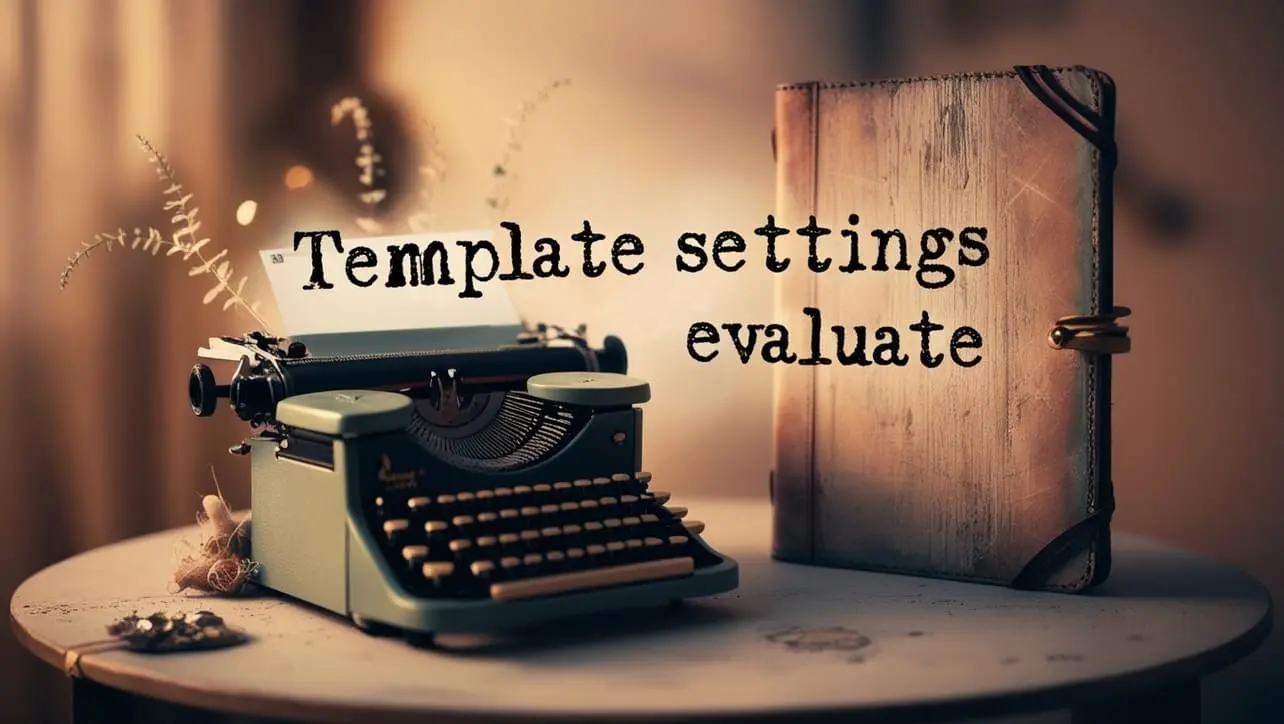
Lodash _.templateSettings.evaluate Property
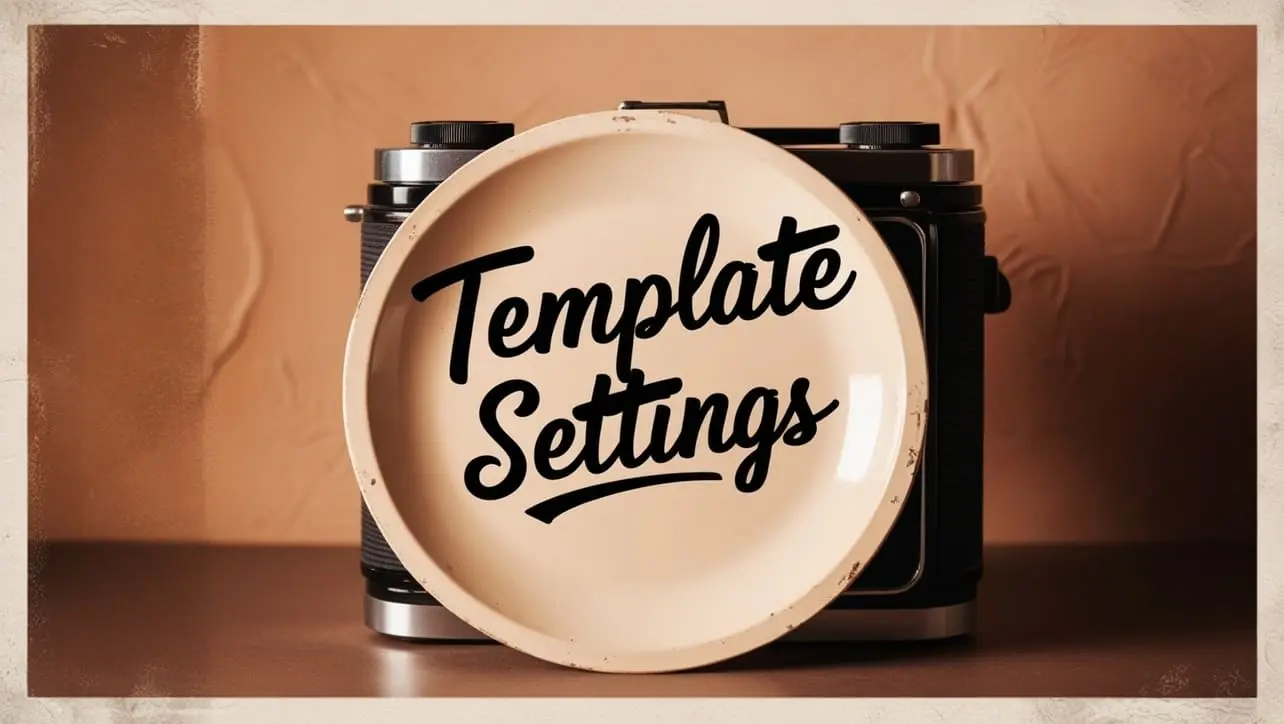
Lodash _.templateSettings Property
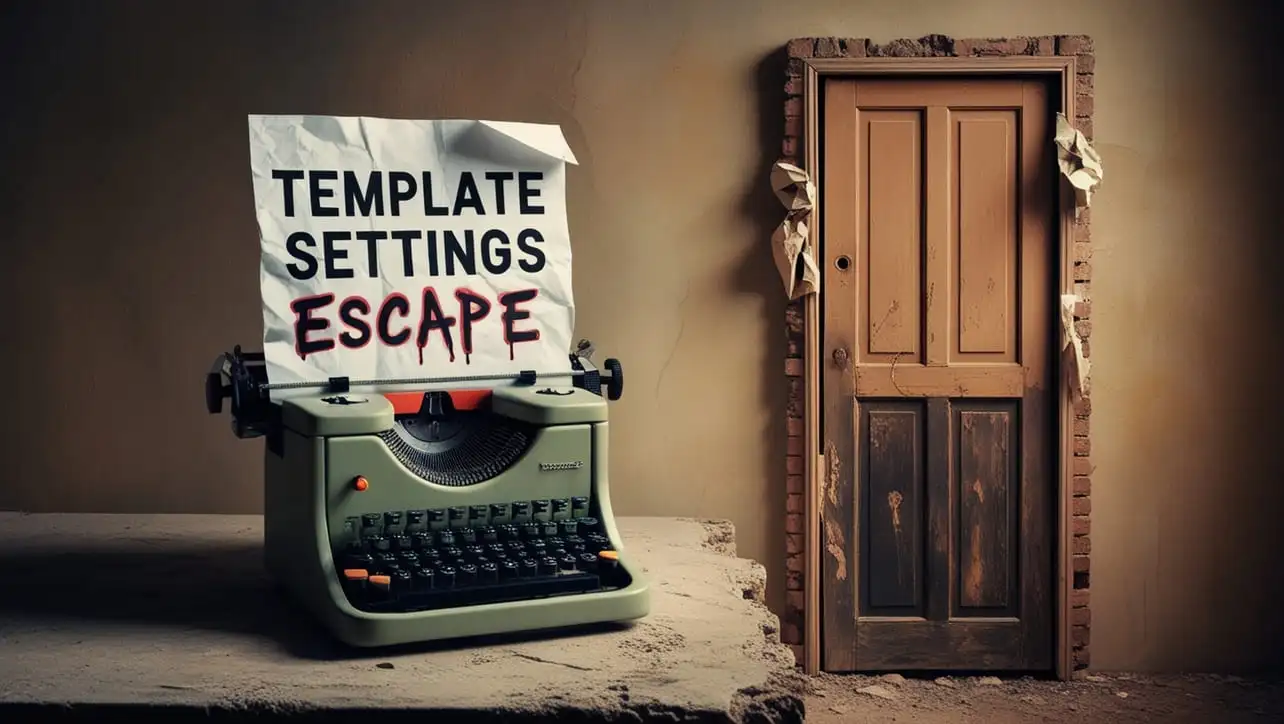
Lodash _.templateSettings.escape Property
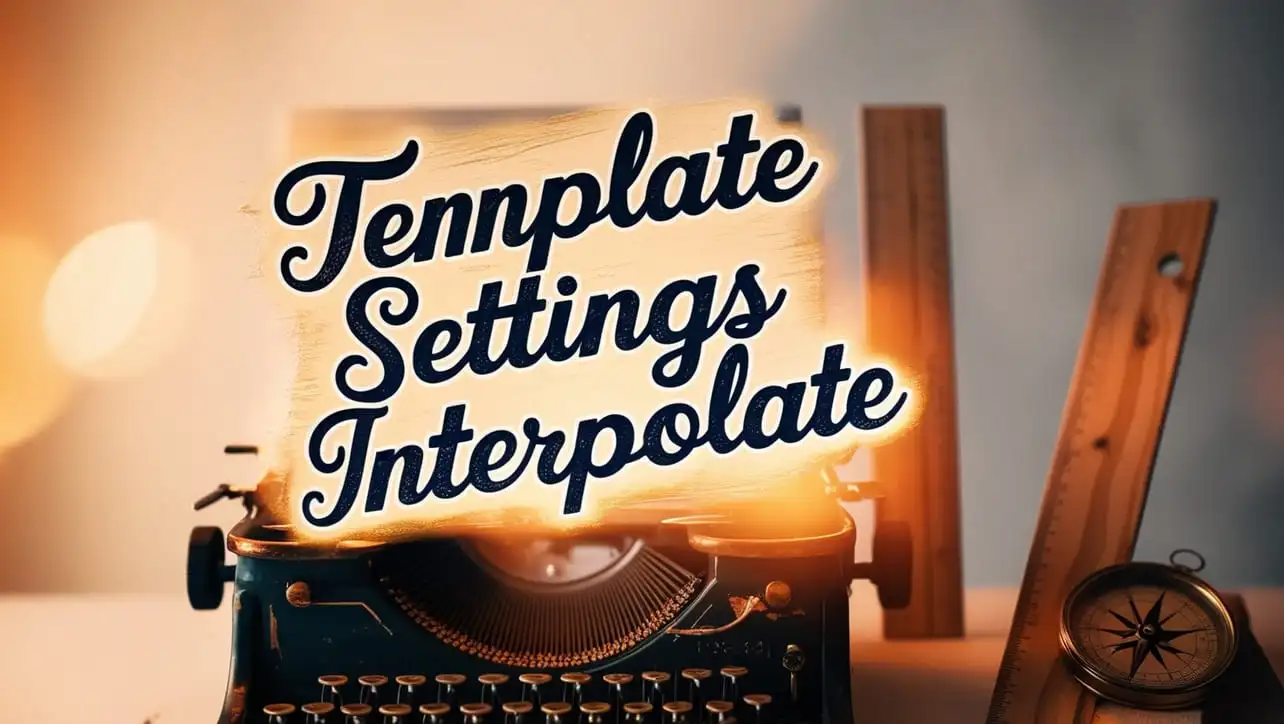
Lodash _.templateSettings.interpolate Property
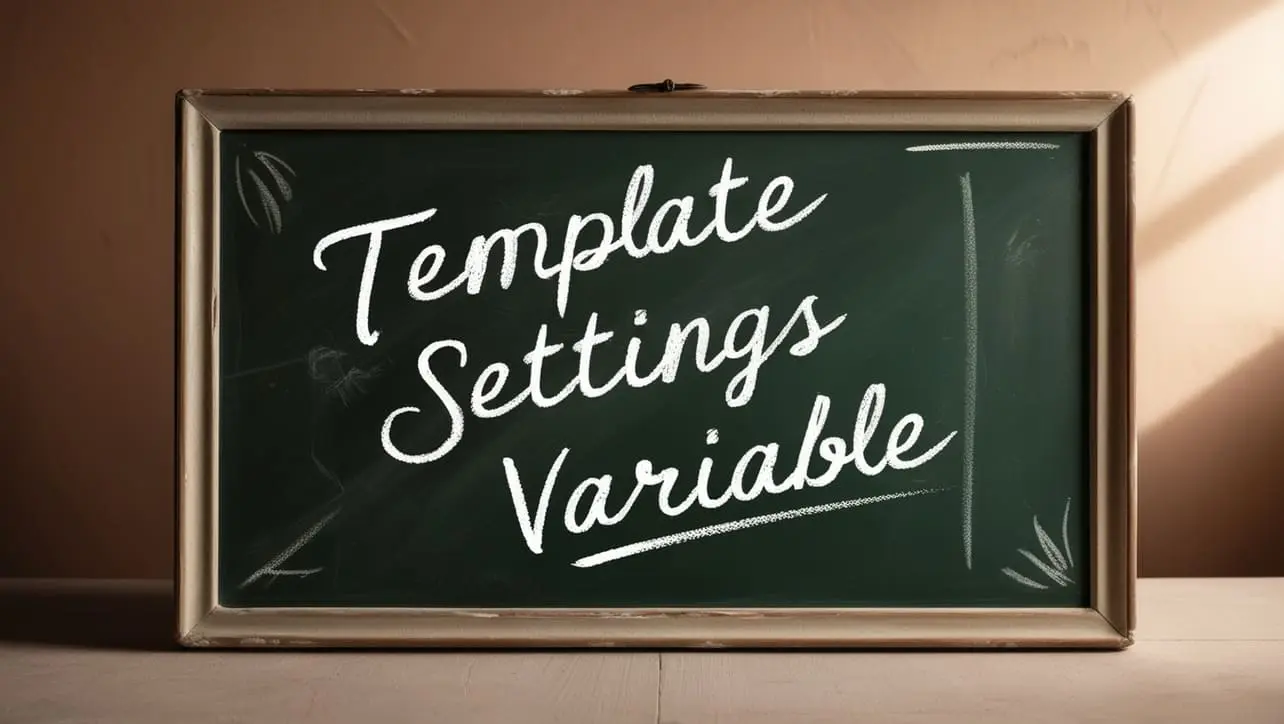
If you have any doubts regarding this article (Lodash _.findLast() Collection Method), please comment here. I will help you immediately.