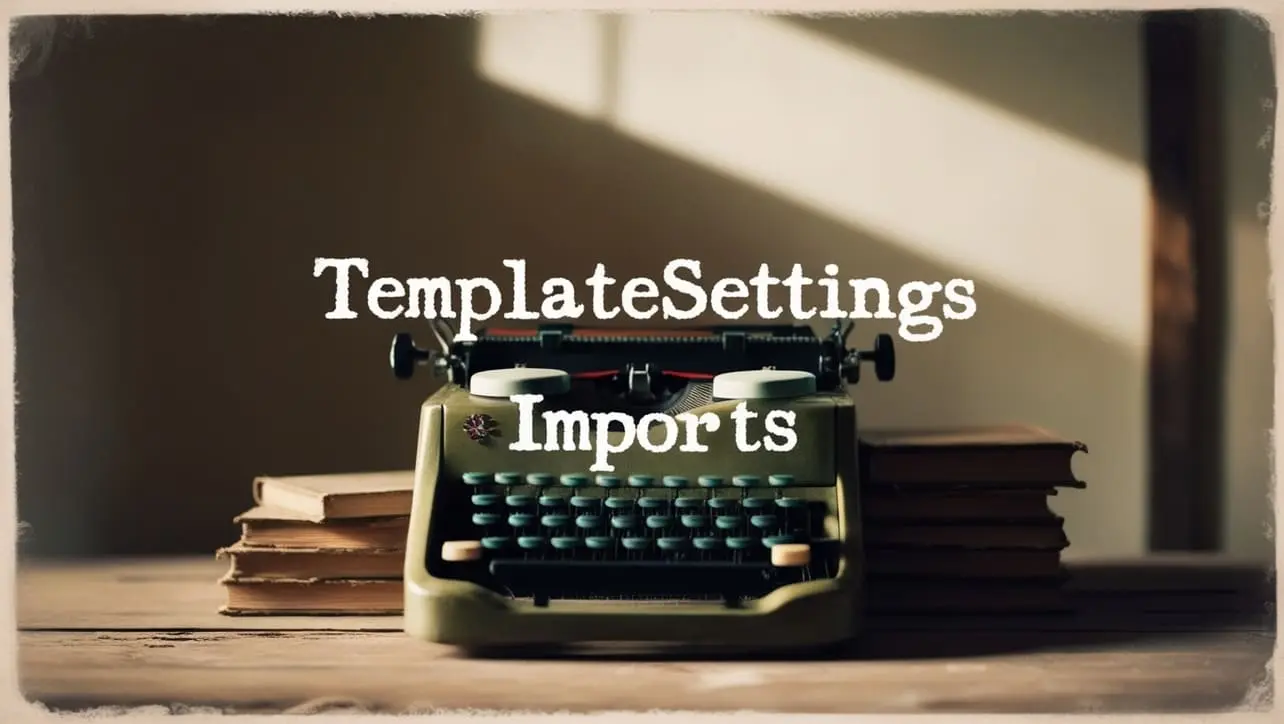
Lodash _.countBy() Collection Method
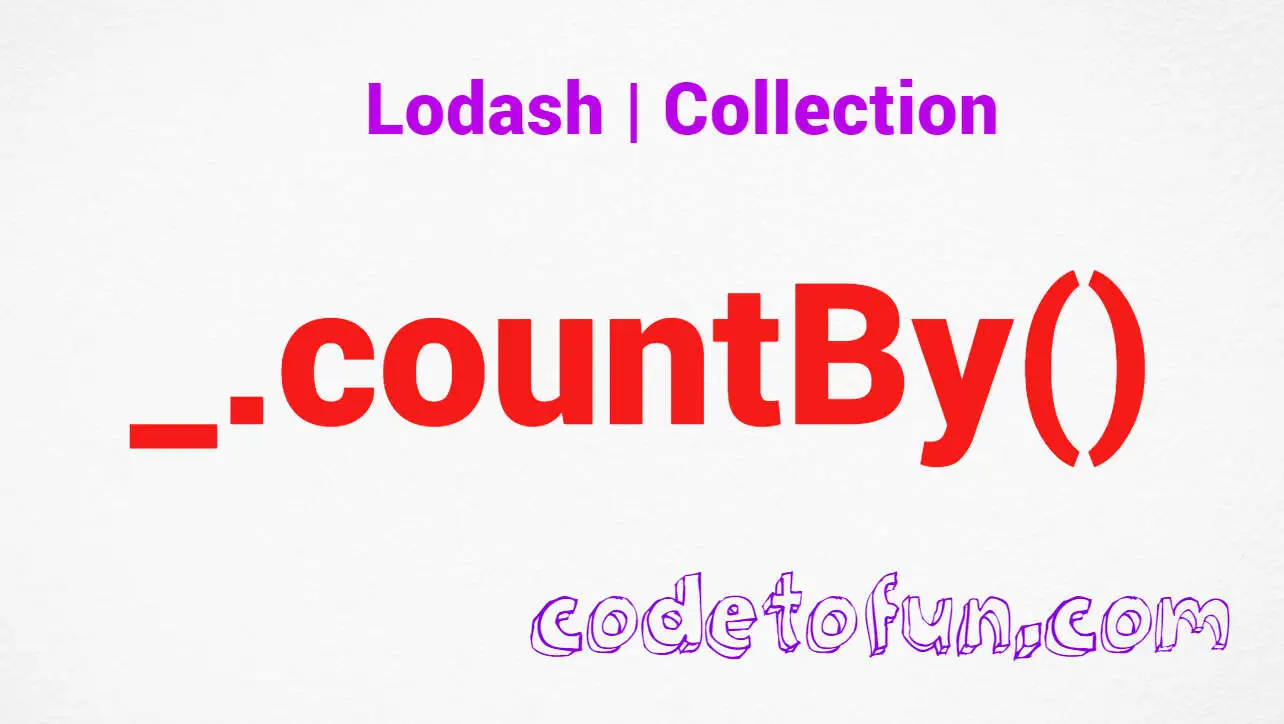
Photo Credit to CodeToFun
🙋 Introduction
Efficiently analyzing and summarizing data is a common task in JavaScript programming. Lodash, a powerful utility library, provides a plethora of functions to streamline these operations. One such gem is the _.countBy()
method, designed for grouping and counting elements in a collection based on a specified criterion.
This method proves invaluable when you need to gain insights into the distribution of values within your data.
🧠 Understanding _.countBy()
The _.countBy()
method in Lodash facilitates the process of grouping elements in a collection and providing a count for each group based on a criterion specified by an iteratee function. This allows developers to quickly gather statistics about the occurrences of different values within their data.
💡 Syntax
_.countBy(collection, [iteratee])
- collection: The collection to iterate over.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's explore a practical example to understand the functionality of _.countBy()
:
const _ = require('lodash');
const data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4];
const counts = _.countBy(data);
console.log(counts);
// Output: { '1': 1, '2': 2, '3': 3, '4': 4 }
In this example, the data array is processed by _.countBy()
, resulting in an object that represents the count of each unique value in the array.
🏆 Best Practices
Utilize Iteratee Function:
Take advantage of the iteratee parameter to customize the criterion for counting. This allows you to group and count elements based on specific properties or conditions.
example.jsCopiedconst arrayOfObjects = [ { type: 'apple', quantity: 2 }, { type: 'orange', quantity: 3 }, { type: 'apple', quantity: 1 }, ]; const countsByType = _.countBy(arrayOfObjects, 'type'); console.log(countsByType); // Output: { 'apple': 2, 'orange': 1 }
Handle Edge Cases:
Be mindful of potential edge cases, such as empty collections or undefined values in the iteratee function. Implement appropriate checks and default behaviors to handle these scenarios.
example.jsCopiedconst emptyArray = []; const countsEmptyArray = _.countBy(emptyArray); console.log(countsEmptyArray); // Output: {}
Explore Nested Structures:
If your collection contains nested structures, consider using a custom iteratee function to access and count values within those structures.
example.jsCopiedconst nestedData = [ { details: { category: 'fruit' } }, { details: { category: 'vegetable' } }, { details: { category: 'fruit' } }, ]; const countsByCategory = _.countBy(nestedData, obj => obj.details.category); console.log(countsByCategory); // Output: { 'fruit': 2, 'vegetable': 1 }
📚 Use Cases
Analyzing Data Distribution:
_.countBy()
is particularly useful when you need to understand the distribution of values within a collection. It provides a quick overview of the frequency of different elements.example.jsCopiedconst surveyResponses = ['Agree', 'Disagree', 'Neutral', 'Agree', 'Strongly Agree', 'Disagree', 'Neutral']; const countsByResponse = _.countBy(surveyResponses); console.log(countsByResponse); // Output: { 'Agree': 2, 'Disagree': 2, 'Neutral': 2, 'Strongly Agree': 1 }
Data Validation:
In scenarios where you are dealing with user input or external data,
_.countBy()
can assist in validating the distribution of values and identifying potential outliers.example.jsCopiedconst userInput = ['Red', 'Blue', 'Green', 'Yellow', 'Purple', 'Pink', 'Orange', 'Red', 'Black']; const colorCounts = _.countBy(userInput); console.log(colorCounts); // Output: { 'Red': 2, 'Blue': 1, 'Green': 1, 'Yellow': 1, 'Purple': 1, 'Pink': 1, 'Orange': 1, 'Black': 1 }
Grouping Data:
When working with datasets,
_.countBy()
can aid in grouping data based on specific criteria, providing a structured summary of the information.example.jsCopiedconst salesData = [ { product: 'Laptop', category: 'Electronics', sales: 20 }, { product: 'Headphones', category: 'Electronics', sales: 15 }, { product: 'Apples', category: 'Groceries', sales: 30 }, { product: 'TV', category: 'Electronics', sales: 10 }, ]; const countsByCategory = _.countBy(salesData, 'category'); console.log(countsByCategory); // Output: { 'Electronics': 3, 'Groceries': 1 }
🎉 Conclusion
The _.countBy()
method in Lodash is a versatile tool for analyzing and summarizing data within a collection. Whether you're examining data distribution, validating inputs, or grouping information, this method provides a concise and efficient solution. Incorporate _.countBy()
into your JavaScript toolkit and gain valuable insights into your datasets!
👨💻 Join our Community:
Author
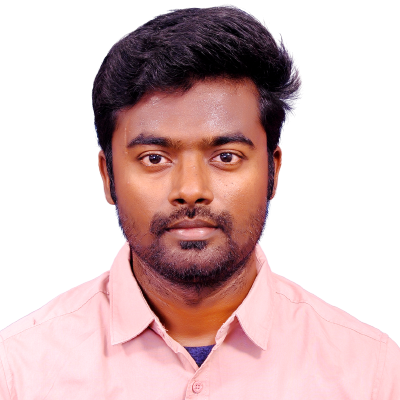
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
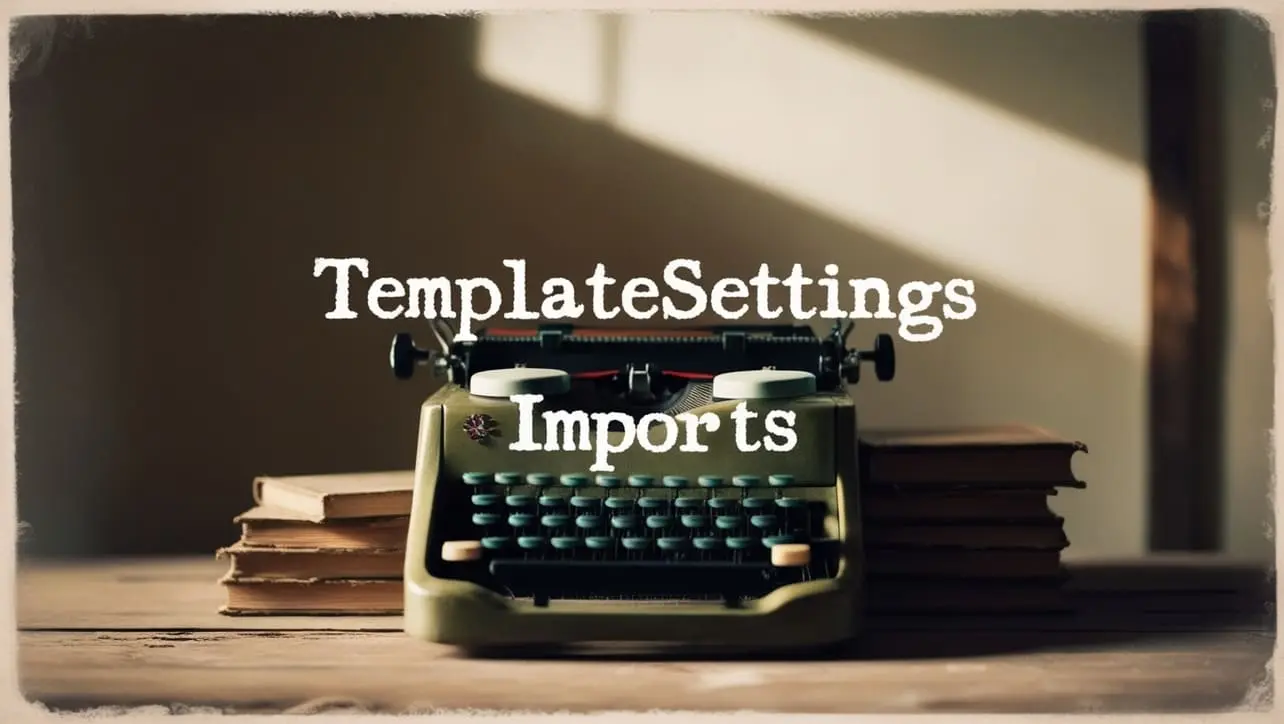
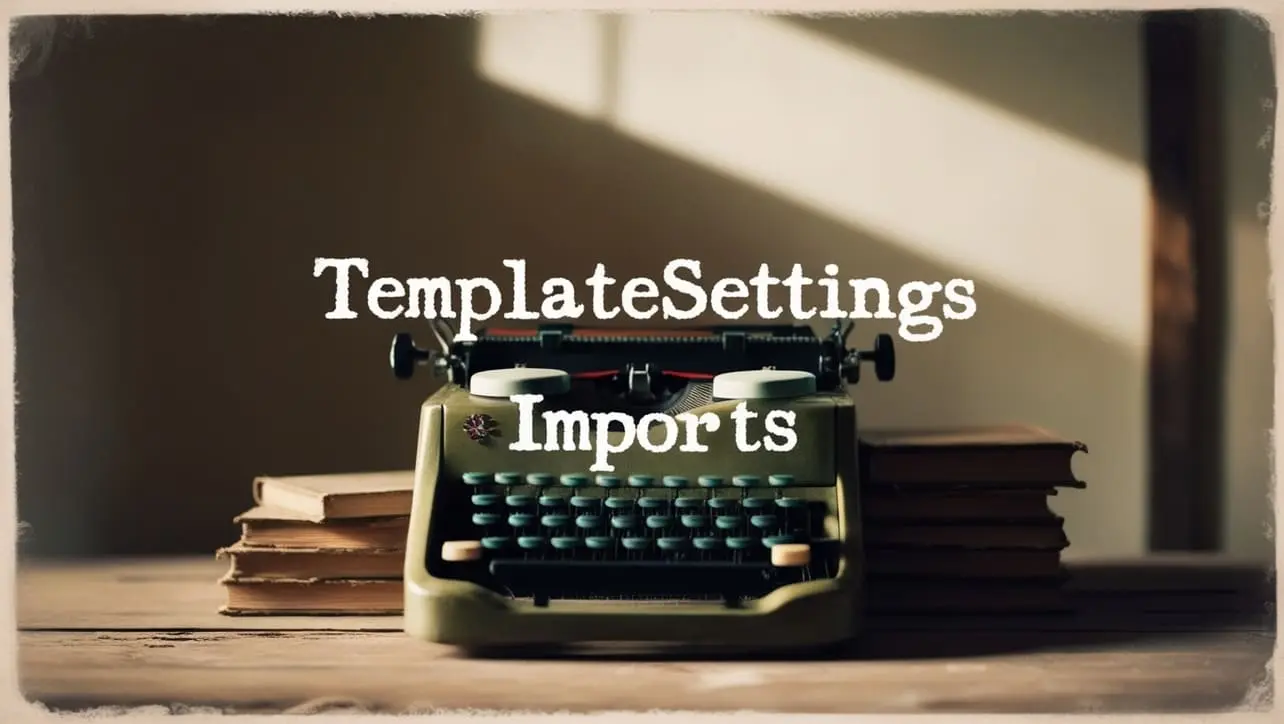
Lodash _.templateSettings.imports Property
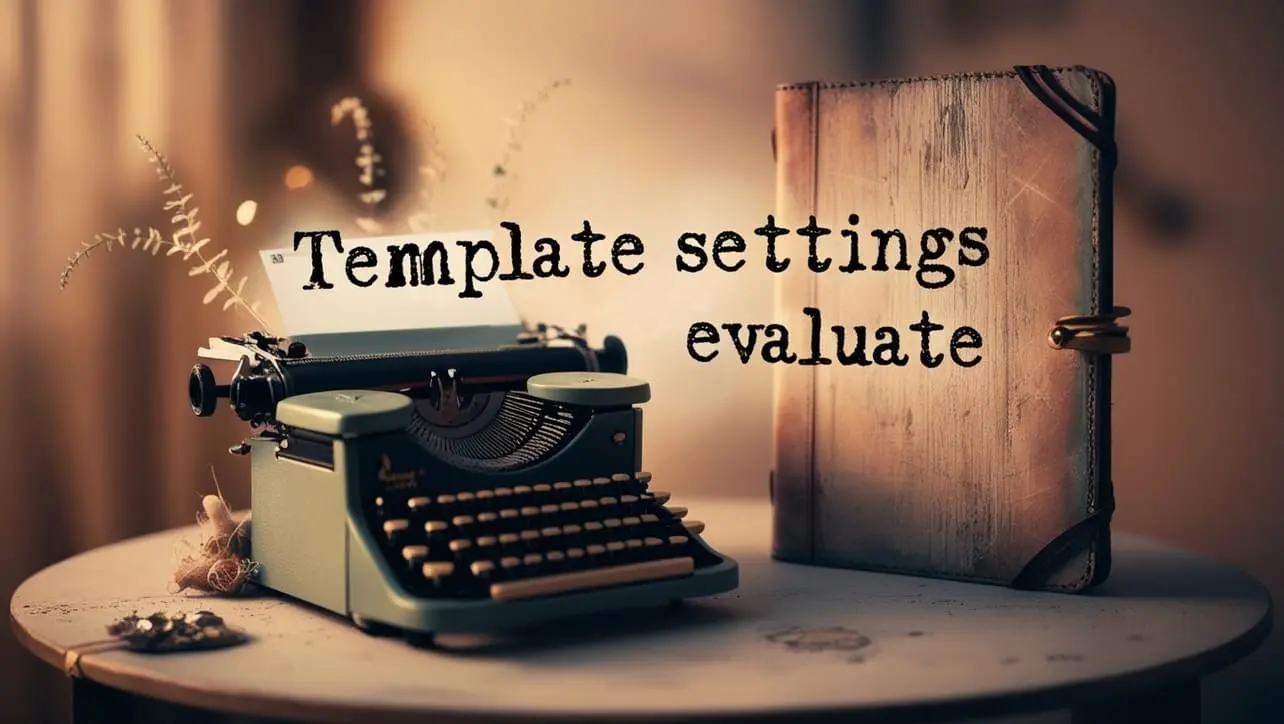
Lodash _.templateSettings.evaluate Property
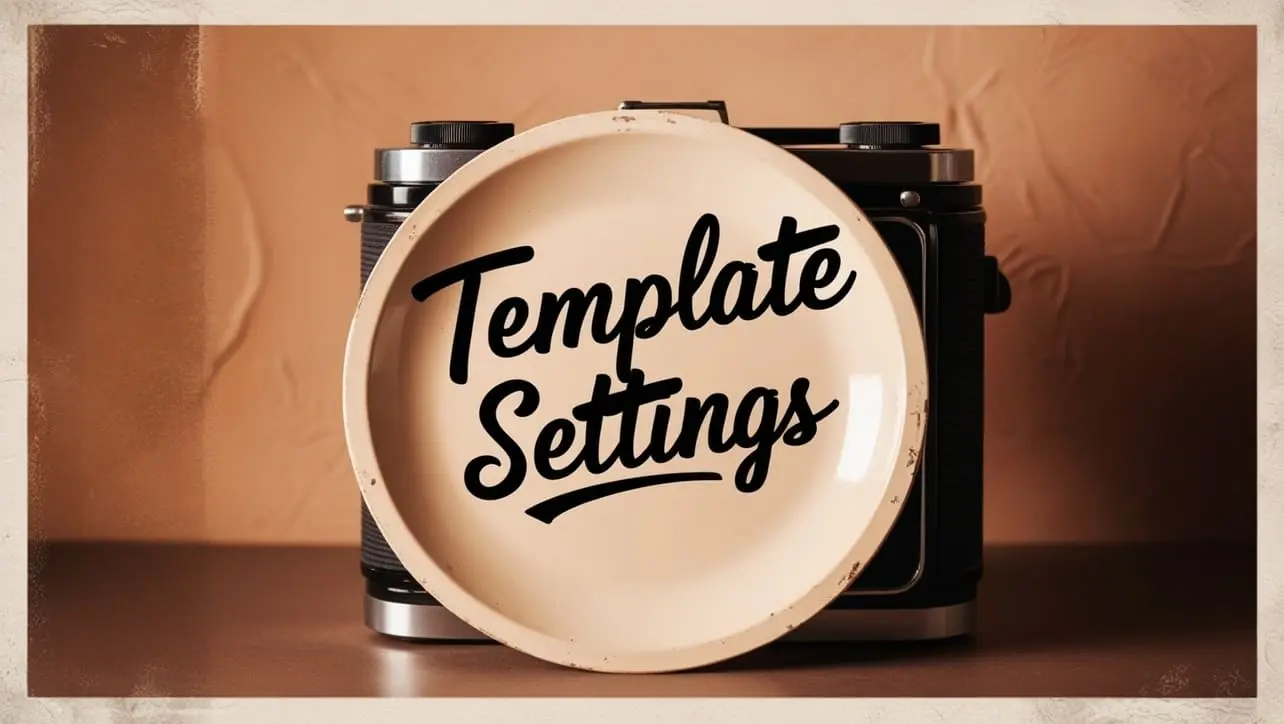
Lodash _.templateSettings Property
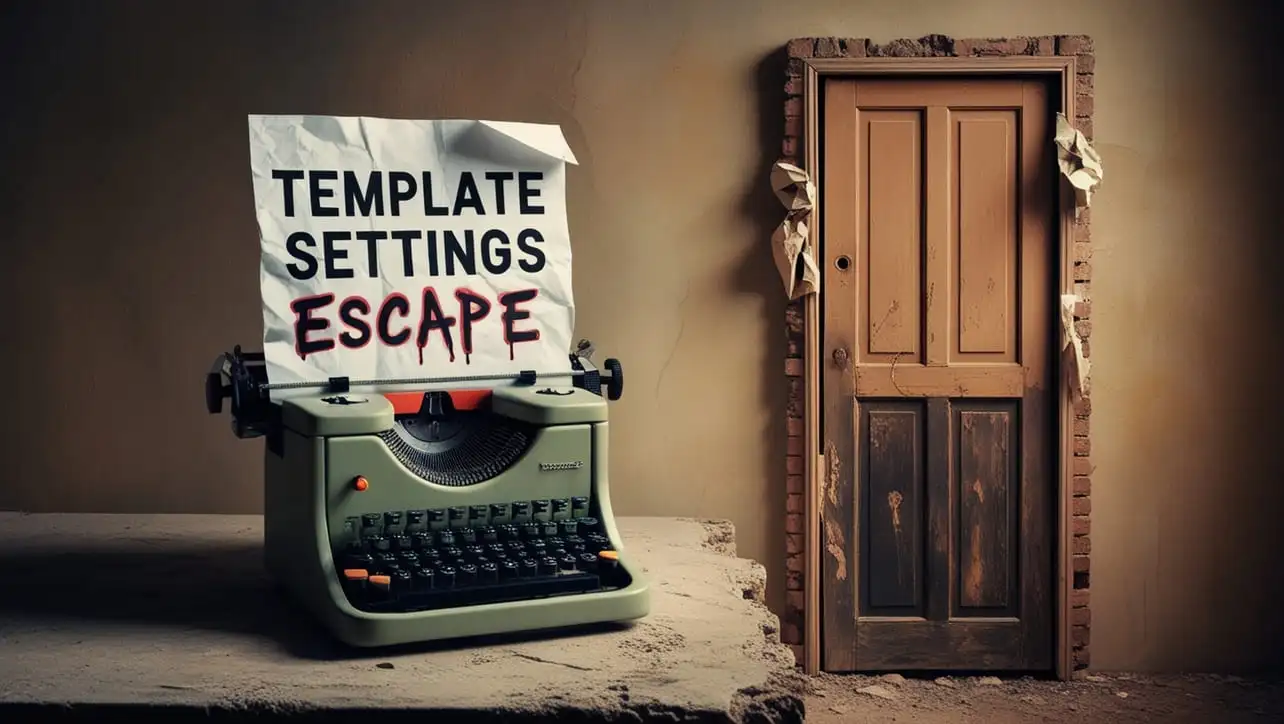
Lodash _.templateSettings.escape Property
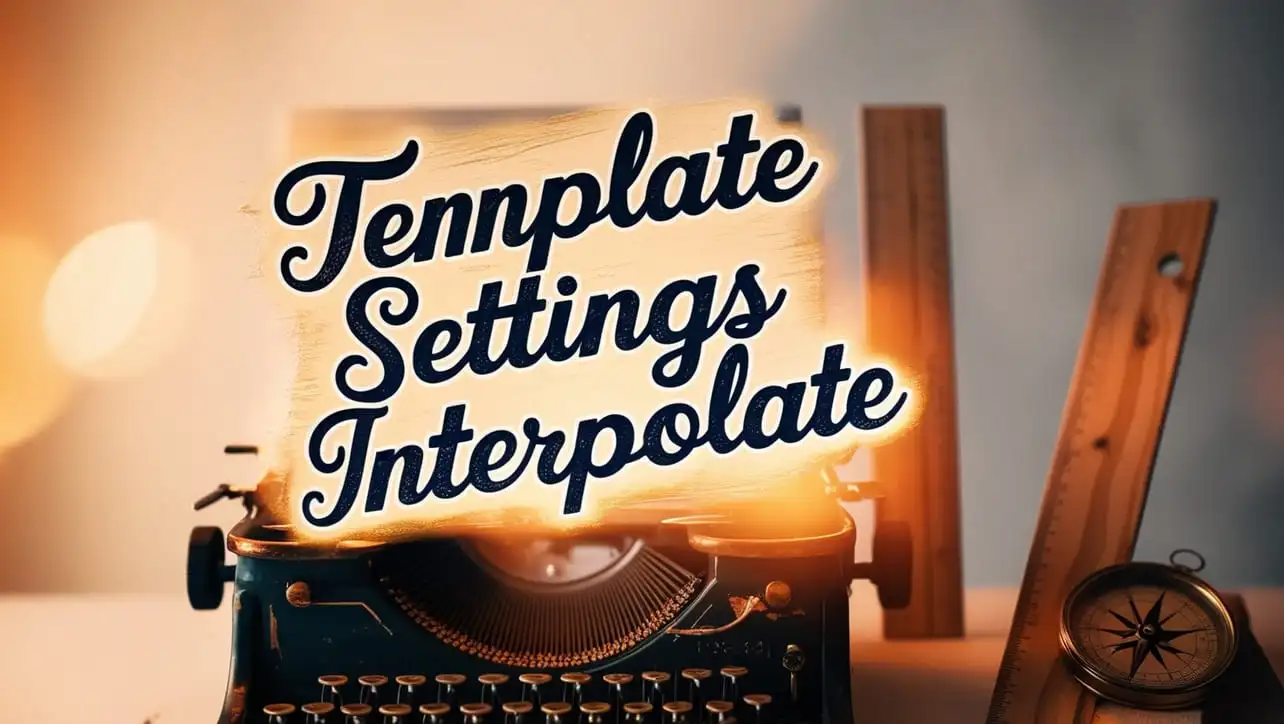
Lodash _.templateSettings.interpolate Property
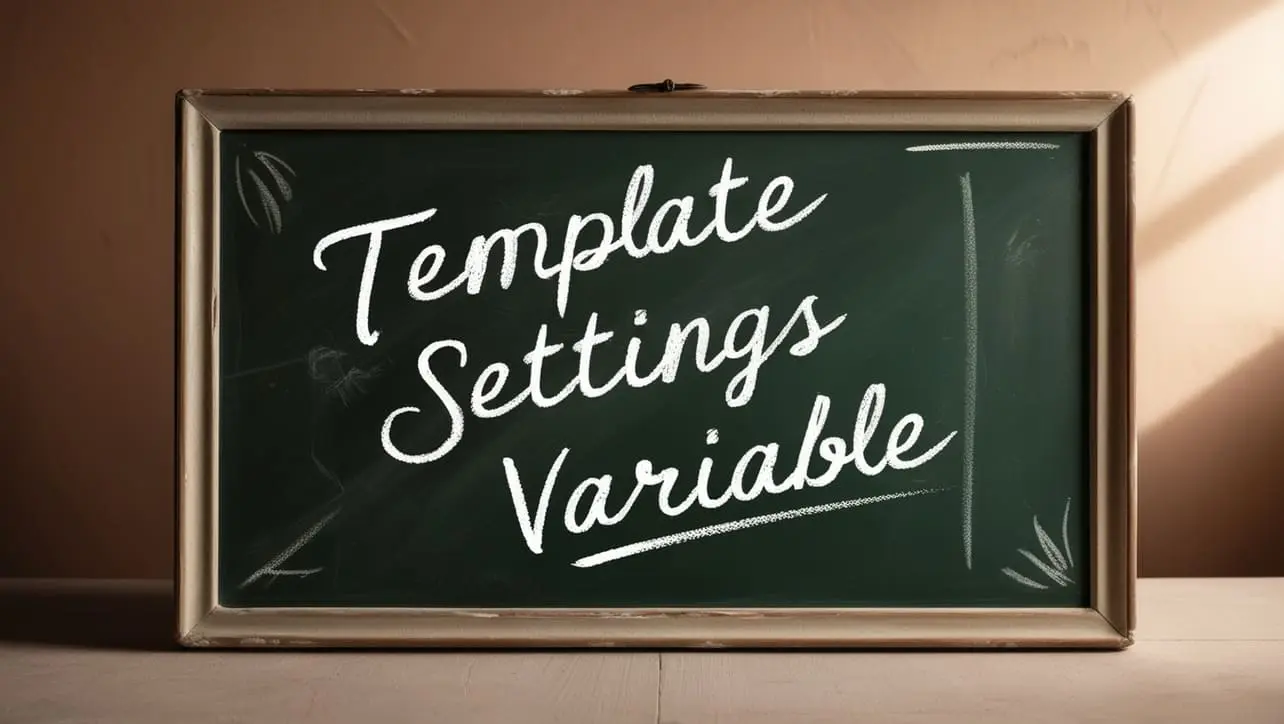
If you have any doubts regarding this article (Lodash _.countBy() Collection Method), please comment here. I will help you immediately.