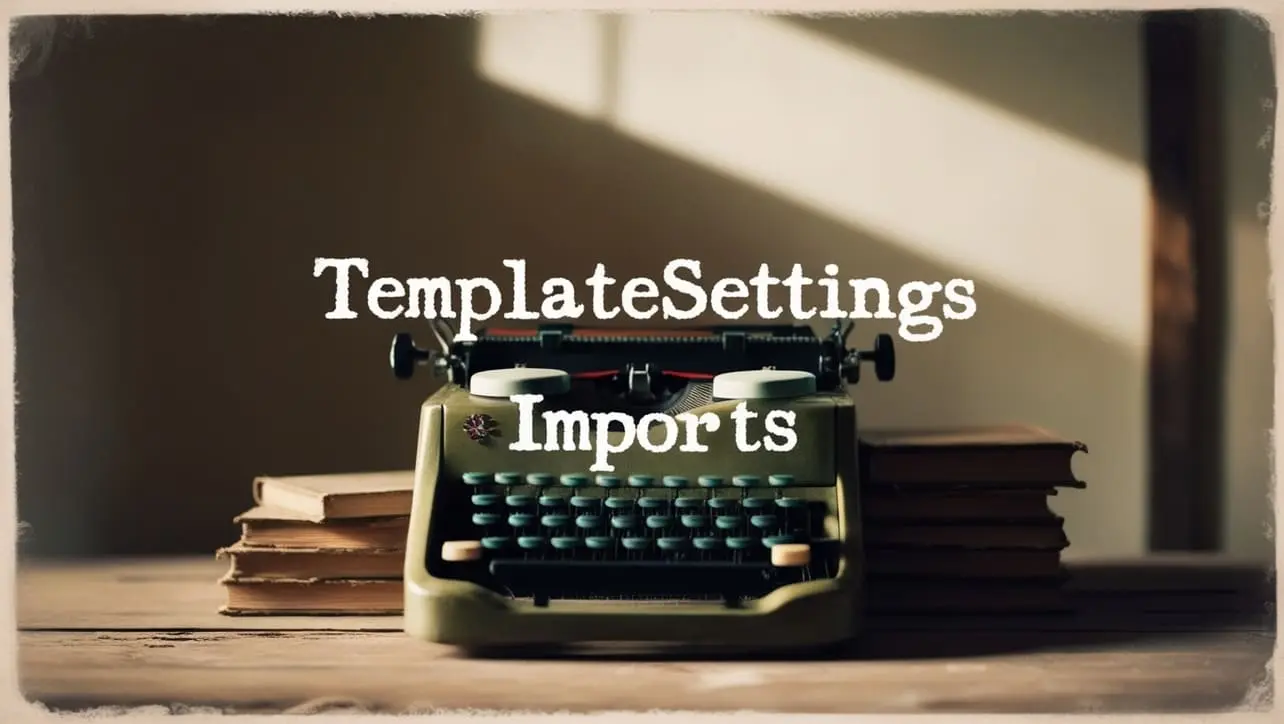
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.uniqWith() Array Method
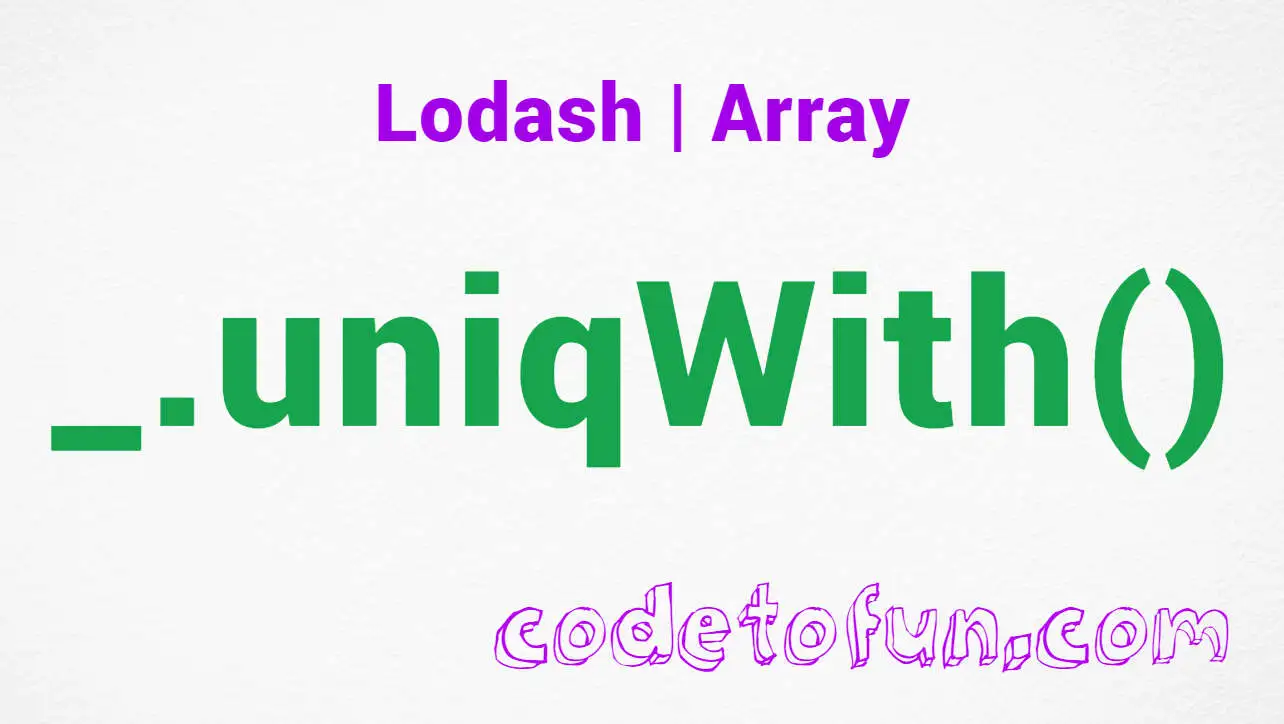
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, managing arrays often involves handling duplicates.
Lodash, a powerful utility library, provides the _.uniqWith()
method to simplify the process of creating a unique array based on custom comparison logic.
This method is a valuable tool for developers aiming to streamline data without relying on standard equality checks.
🧠 Understanding _.uniqWith()
The _.uniqWith()
method in Lodash is designed to create a new array with unique values by utilizing a custom comparator function to determine equality between elements. This offers flexibility in handling complex data structures or custom objects where the default comparison might not suffice.
💡 Syntax
_.uniqWith(array, [comparator])
- array: The array to process.
- comparator: The custom function to compare values (default is a strict equality check).
📝 Example
Let's explore a practical example to illustrate the functionality of _.uniqWith()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const arrayWithDuplicates = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 1, name: 'Alice' }, // Duplicate entry
{ id: 3, name: 'Charlie' },
];
const uniqueArray = _.uniqWith(arrayWithDuplicates, (a, b) => a.id === b.id);
console.log(uniqueArray);
// Output: [ { id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' } ]
In this example, the arrayWithDuplicates is processed, and duplicates are removed based on a custom comparator function comparing the id property.
🏆 Best Practices
Implement a Custom Comparator:
To make the most of
_.uniqWith()
, implement a custom comparator function tailored to your data structure. This ensures accurate and targeted comparison for unique value determination.example.jsCopiedconst customComparator = (a, b) => a.property === b.property; // Customize as per your data structure const uniqueData = _.uniqWith(dataArray, customComparator); console.log(uniqueData);
Choose the Right Data Structure:
Consider the structure of your data and choose appropriate properties for comparison. This ensures that the custom comparator effectively identifies and removes duplicates.
example.jsCopiedconst complexDataArray = [ { id: 1, details: { name: 'John', age: 25 } }, { id: 2, details: { name: 'Jane', age: 30 } }, { id: 1, details: { name: 'John', age: 25 } }, // Duplicate entry ]; const uniqueComplexData = _.uniqWith(complexDataArray, (a, b) => a.id === b.id); console.log(uniqueComplexData);
Understand Comparator Logic:
Be mindful of how the comparator logic operates, especially when dealing with nested objects or arrays. Ensure that the logic aligns with your uniqueness criteria.
example.jsCopiedconst nestedArray = [ [1, 2, 3], [4, 5, 6], [1, 2, 3], // Duplicate entry ]; const uniqueNestedArray = _.uniqWith(nestedArray, (a, b) => _.isEqual(a, b)); console.log(uniqueNestedArray);
📚 Use Cases
Unique Object Array:
_.uniqWith()
is particularly useful when dealing with an array of objects, allowing you to create a unique array based on specific properties.example.jsCopiedconst uniqueUserArray = _.uniqWith(usersArray, (a, b) => a.id === b.id); console.log(uniqueUserArray);
Complex Data Structures:
When working with complex data structures, such as nested objects or arrays,
_.uniqWith()
provides a way to deduplicate based on custom comparison logic.example.jsCopiedconst uniqueNestedData = _.uniqWith(nestedDataArray, (a, b) => _.isEqual(a, b)); console.log(uniqueNestedData);
🎉 Conclusion
The _.uniqWith()
method in Lodash is a powerful tool for creating unique arrays with custom comparison logic. Whether dealing with simple arrays of primitive values or complex data structures, this method provides the flexibility needed to handle duplicates efficiently.
Explore the capabilities of _.uniqWith()
and elevate your array manipulation in JavaScript to new heights. Embrace the versatility of Lodash for streamlined and customized data processing!
👨💻 Join our Community:
Author
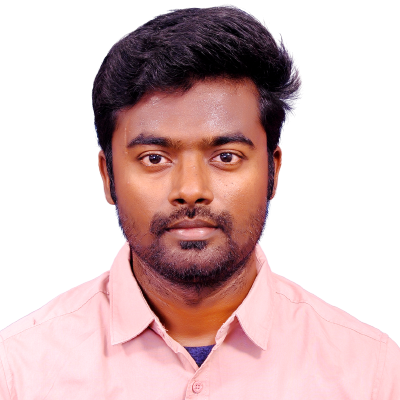
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
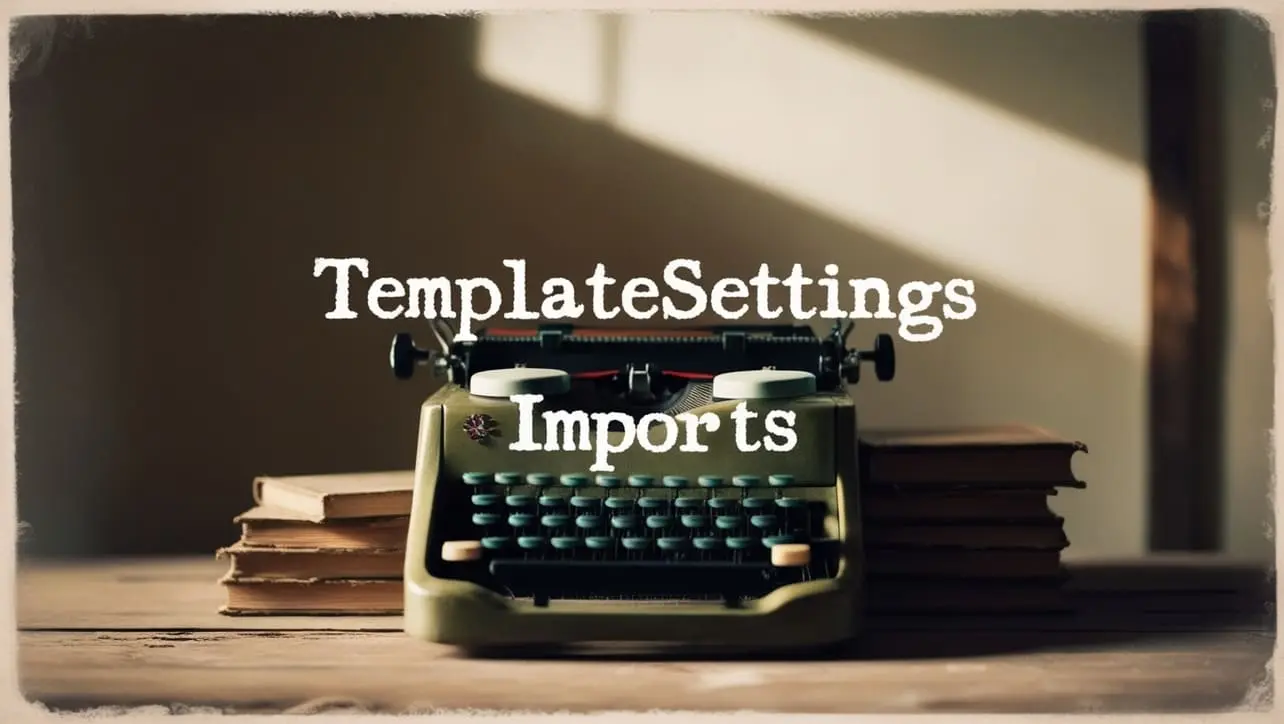
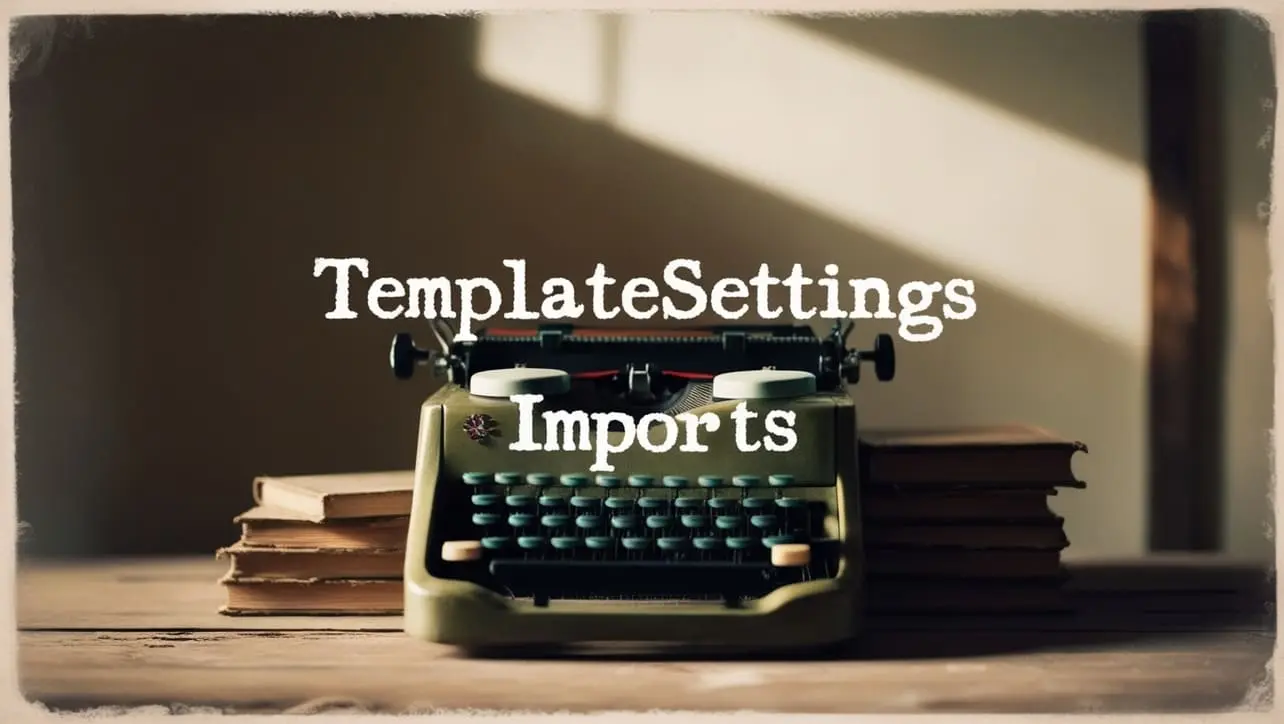
Lodash _.templateSettings.imports Property
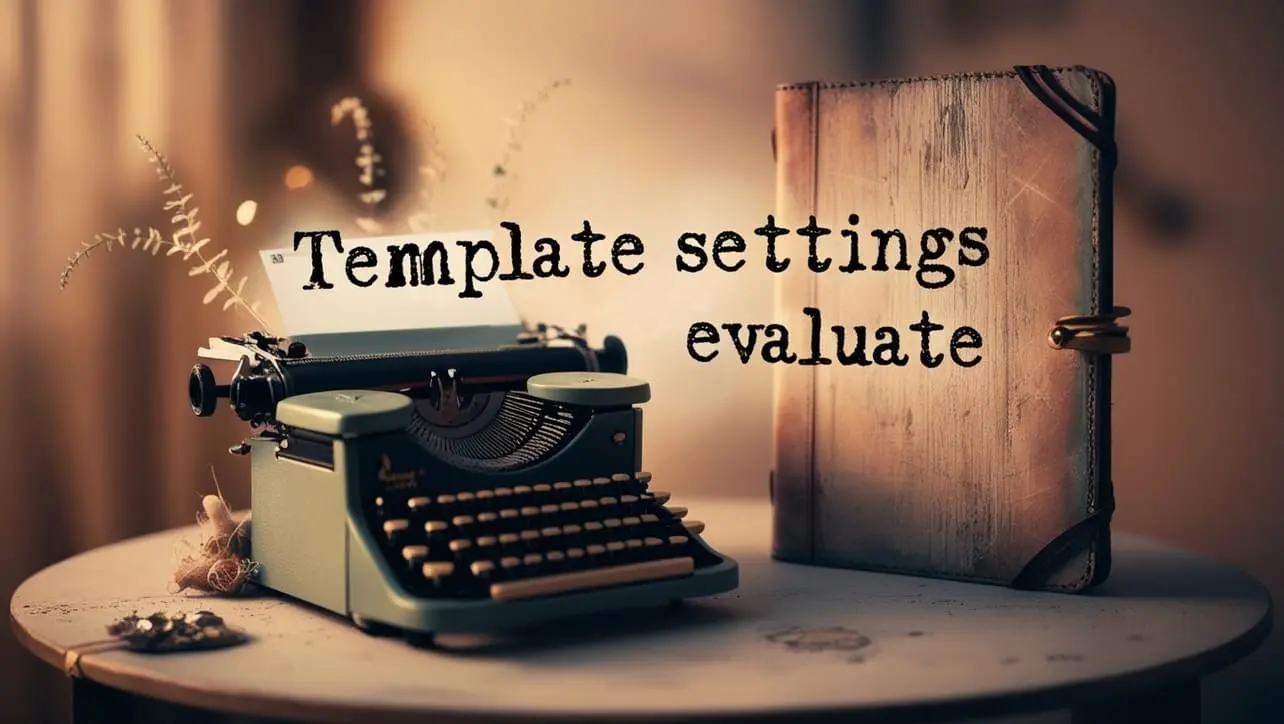
Lodash _.templateSettings.evaluate Property
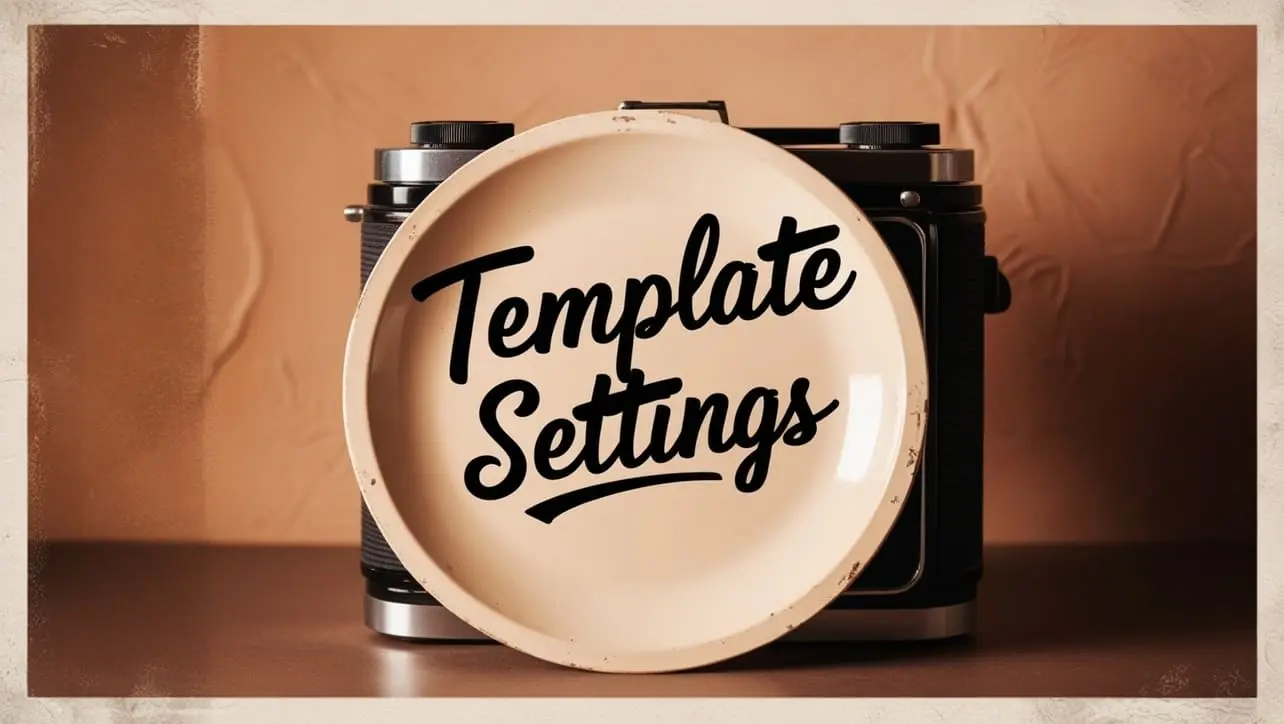
Lodash _.templateSettings Property
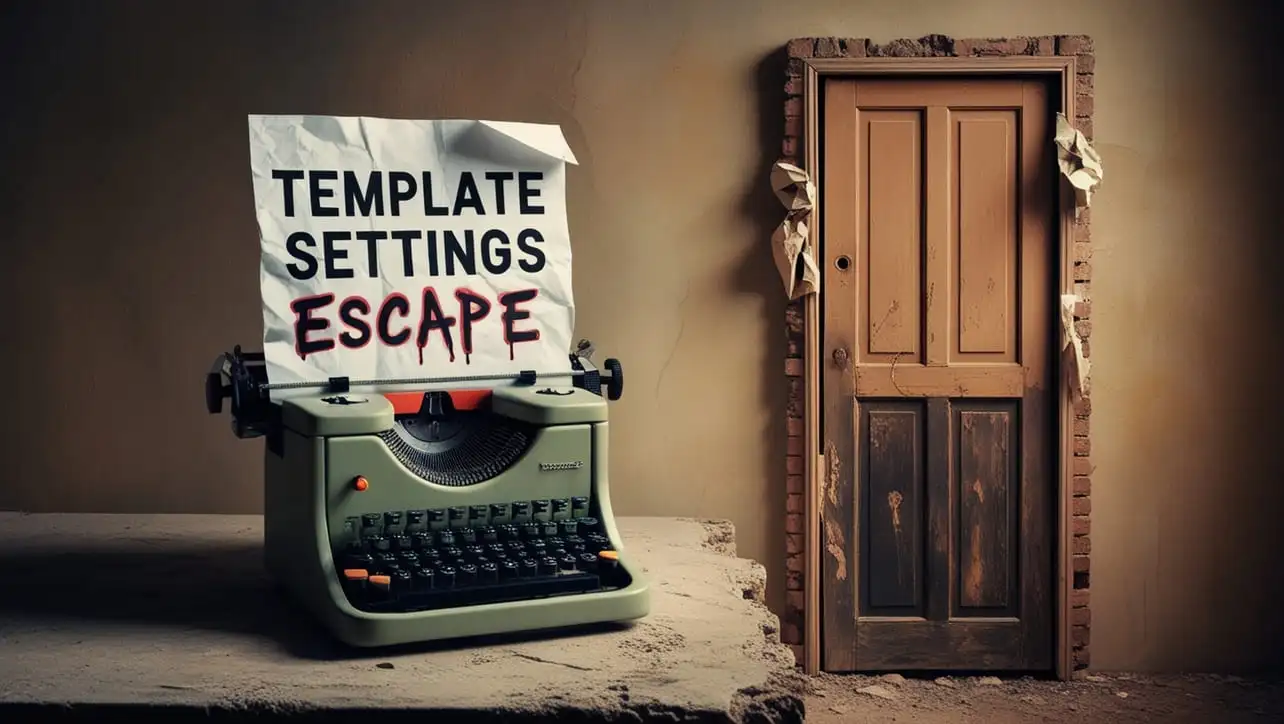
Lodash _.templateSettings.escape Property
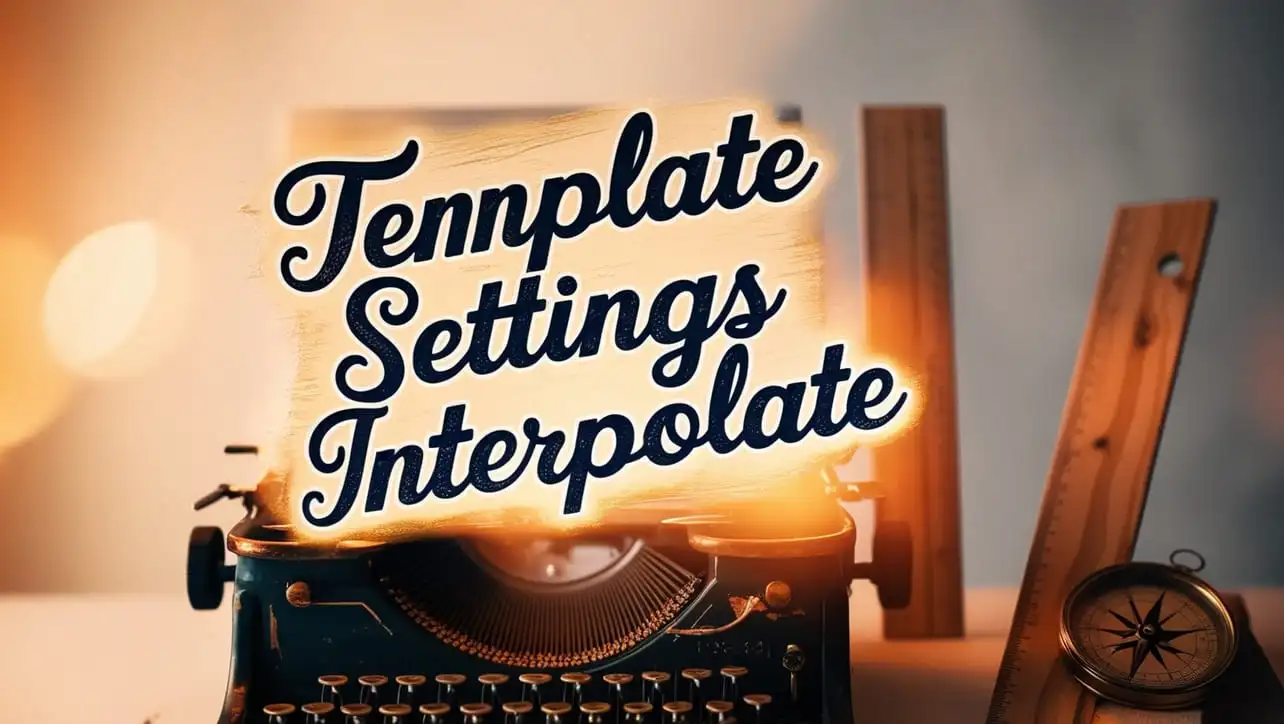
Lodash _.templateSettings.interpolate Property
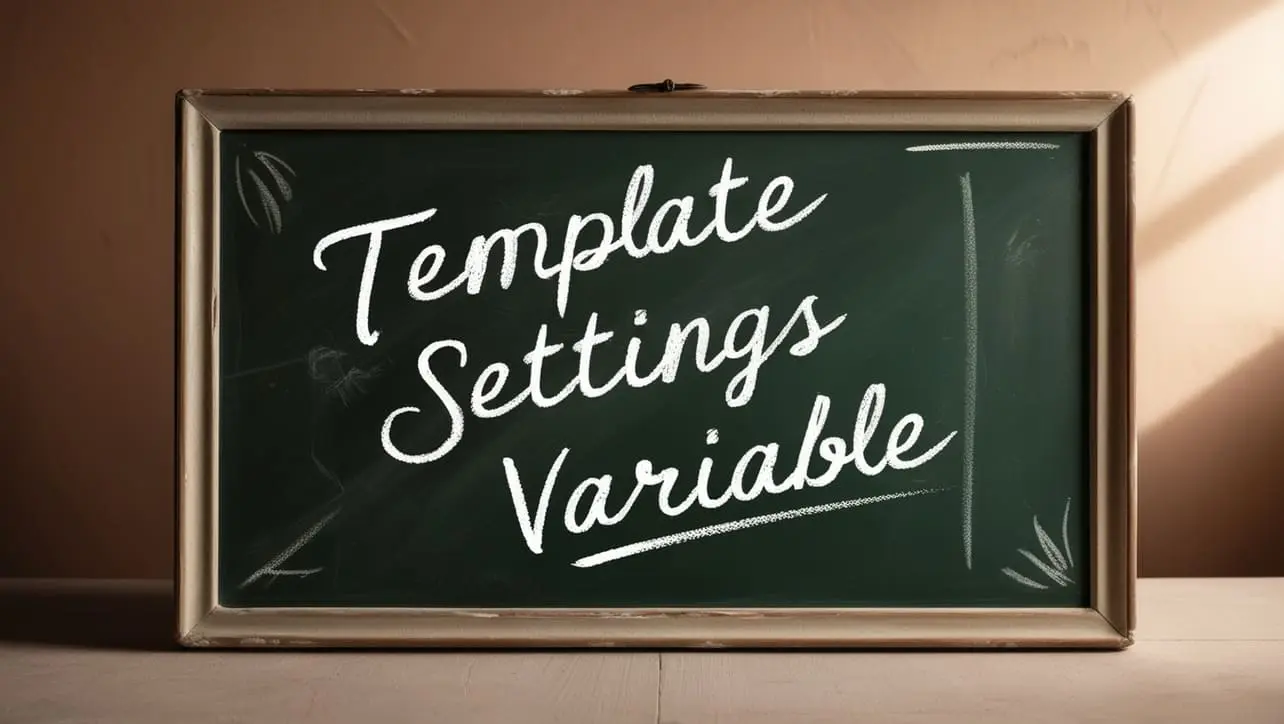
If you have any doubts regarding this article (Lodash _.uniqWith() Array Method), please comment here. I will help you immediately.