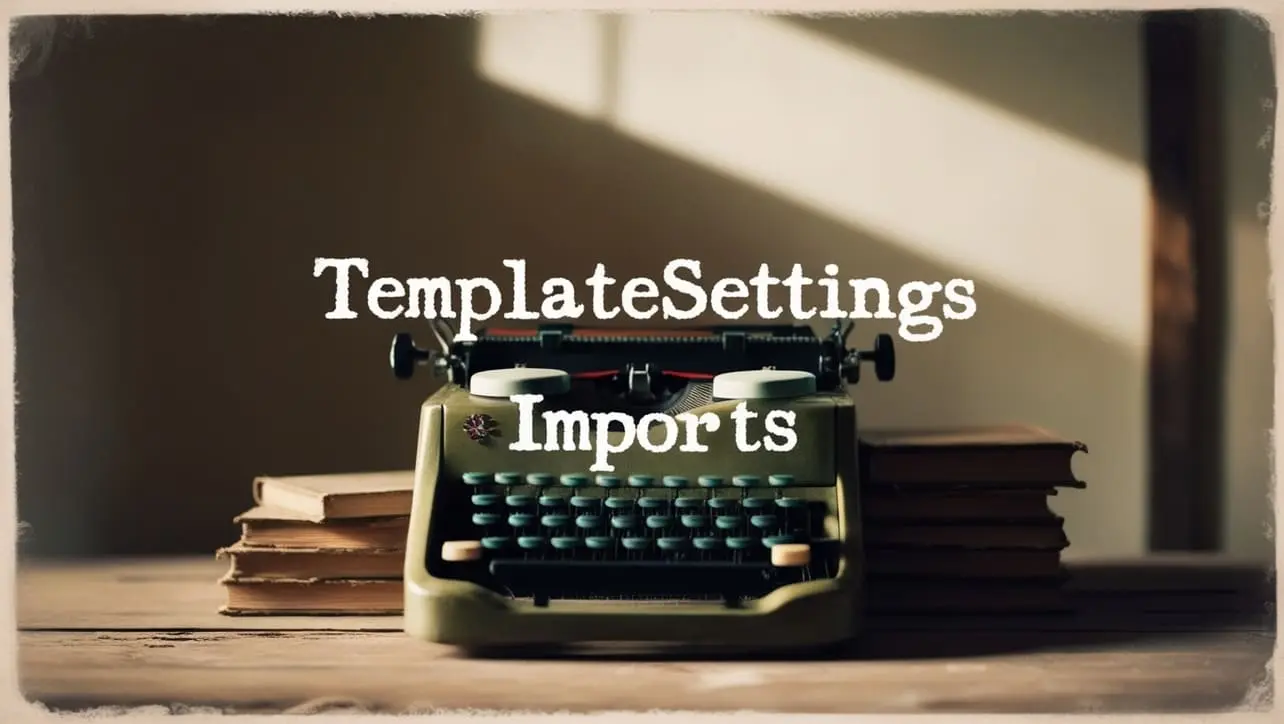
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.unionBy() Array Method
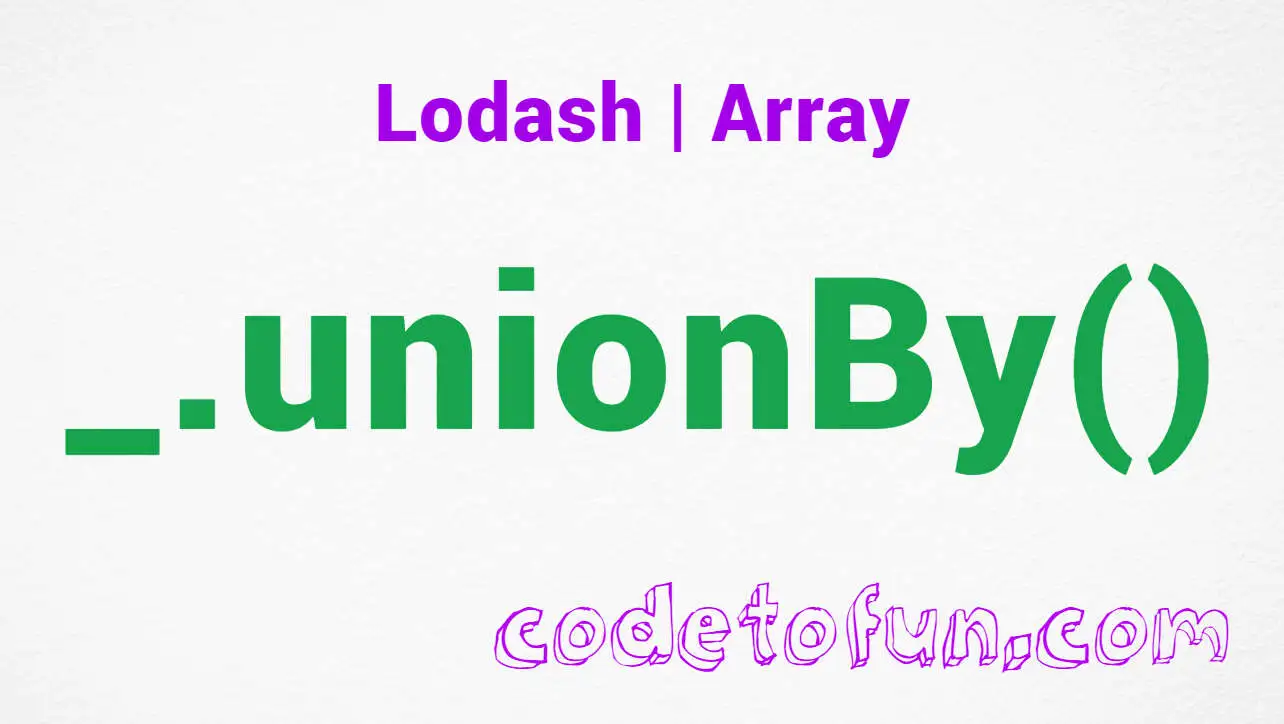
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, managing arrays efficiently is a common challenge. Lodash, a powerful utility library, comes to the rescue with a multitude of functions to simplify array operations. Among these functions is the _.unionBy()
method, a versatile tool for creating a union of arrays while allowing customization based on a specified iteratee function.
This method is invaluable for developers dealing with data integration and manipulation.
🧠 Understanding _.unionBy()
The _.unionBy()
method in Lodash is designed to create a union of arrays while providing flexibility through a user-defined iteratee function. This allows developers to determine uniqueness based on specific criteria, resulting in a merged array containing distinct elements.
💡 Syntax
_.unionBy([arrays], iteratee)
- arrays: The arrays to process.
- iteratee: The function invoked per element.
📝 Example
Let's explore a practical example to illustrate the functionality of _.unionBy()
:
const _ = require('lodash');
const array1 = [1.1, 2.2];
const array2 = [2.3, 3.4];
const array3 = [4.5, 2.6];
const unionArray = _.unionBy([array1, array2, array3], Math.floor);
console.log(unionArray);
// Output: [1.1, 2.2, 3.4, 4.5]
In this example, _.unionBy()
combines the arrays while using the Math.floor function as the iteratee to ensure uniqueness based on the floored values.
🏆 Best Practices
Understanding Uniqueness Criteria:
Clearly define the criteria for uniqueness using the iteratee function. This ensures that the resulting union meets the specific requirements of your application.
example.jsCopiedconst arrays = [ [{ id: 1, value: 'apple' }, { id: 2, value: 'banana' }], [{ id: 2, value: 'banana' }, { id: 3, value: 'orange' }], [{ id: 4, value: 'grape' }], ]; const unionArray = _.unionBy(arrays, 'id'); console.log(unionArray); // Output: [{ id: 1, value: 'apple' }, { id: 2, value: 'banana' }, { id: 3, value: 'orange' }, { id: 4, value: 'grape' }]
Sorting Arrays for Consistency:
Sort the input arrays if consistency in the order of elements is important.
_.unionBy()
processes arrays in the order provided, and sorting them beforehand can lead to predictable results.example.jsCopiedconst arraysToUnion = [ [3, 1, 2], [2, 3, 4], [5, 4, 6], ]; const sortedUnion = _.unionBy(arraysToUnion.map(arr => arr.sort()), Math.floor); console.log(sortedUnion); // Output: [1, 2, 3, 4, 5, 6]
Handling Empty Arrays:
Consider scenarios where input arrays might be empty. Ensure that your code accounts for these cases to avoid unexpected behavior.
example.jsCopiedconst emptyArray = []; const nonEmptyArray = [1, 2, 3]; const unionResult = _.unionBy([emptyArray, nonEmptyArray], Math.floor); console.log(unionResult); // Output: [1, 2, 3]
📚 Use Cases
Data Integration:
_.unionBy()
is ideal for merging arrays containing different datasets, allowing you to integrate data seamlessly.example.jsCopiedconst userData = [{ id: 1, name: 'Alice' }]; const orderData = [{ id: 1, product: 'Laptop' }]; const integratedData = _.unionBy([userData, orderData], 'id'); console.log(integratedData); // Output: [{ id: 1, name: 'Alice' }]
Merging Multiple Sources:
When dealing with multiple sources of data,
_.unionBy()
simplifies the process of merging them into a unified dataset.example.jsCopiedconst source1 = [10, 20, 30]; const source2 = [30, 40, 50]; const source3 = [50, 60, 70]; const mergedData = _.unionBy([source1, source2, source3], value => value % 20); console.log(mergedData); // Output: [10, 20, 30, 40, 50, 60]
Consistent User Lists:
When combining lists of users from different sources,
_.unionBy()
ensures a consistent list based on specified uniqueness criteria.example.jsCopiedconst userList1 = [{ id: 1, name: 'John' }]; const userList2 = [{ id: 2, name: 'Jane' }]; const userList3 = [{ id: 1, name: 'John' }, { id: 3, name: 'Doe' }]; const consistentUserList = _.unionBy([userList1, userList2, userList3], 'id'); console.log(consistentUserList); // Output: [{ id: 1, name: 'John' }, { id: 2, name: 'Jane' }, { id: 3, name: 'Doe' }]
🎉 Conclusion
The _.unionBy()
method in Lodash provides a flexible and powerful solution for creating the union of arrays while allowing customization based on user-defined criteria. Whether you're integrating diverse datasets or merging multiple sources, this method empowers you to handle array operations with ease in JavaScript.
Explore the capabilities of _.unionBy()
and elevate your array manipulation skills with Lodash!
👨💻 Join our Community:
Author
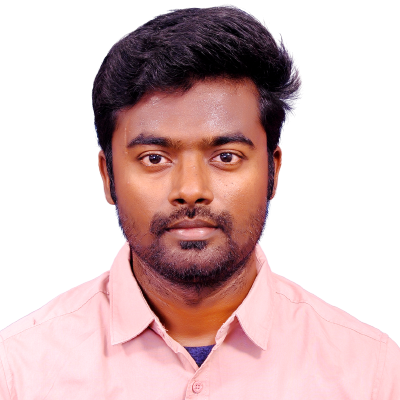
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
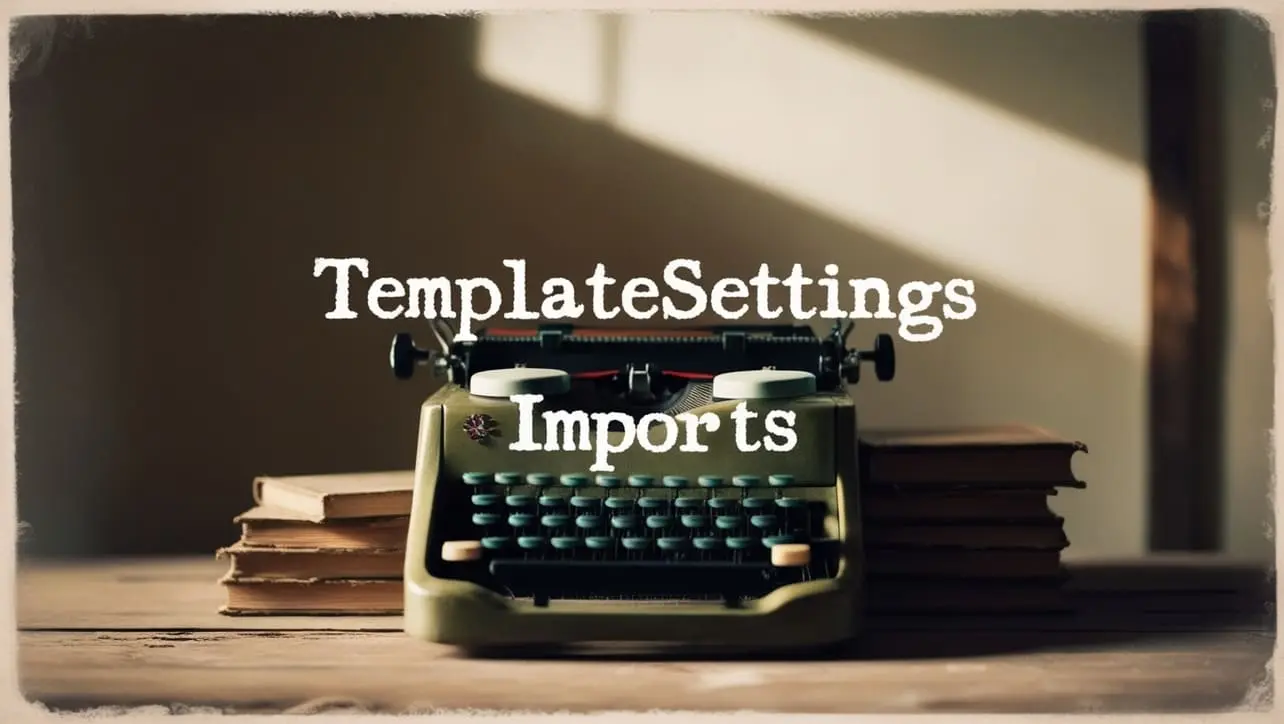
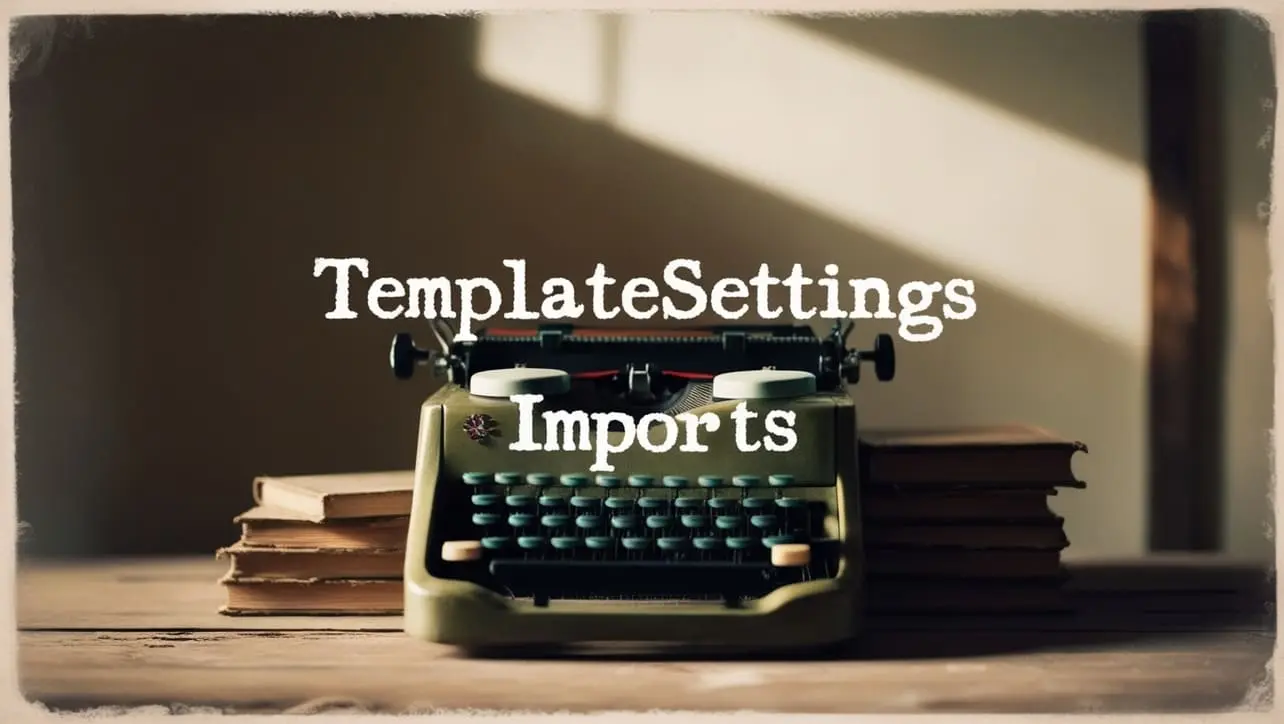
Lodash _.templateSettings.imports Property
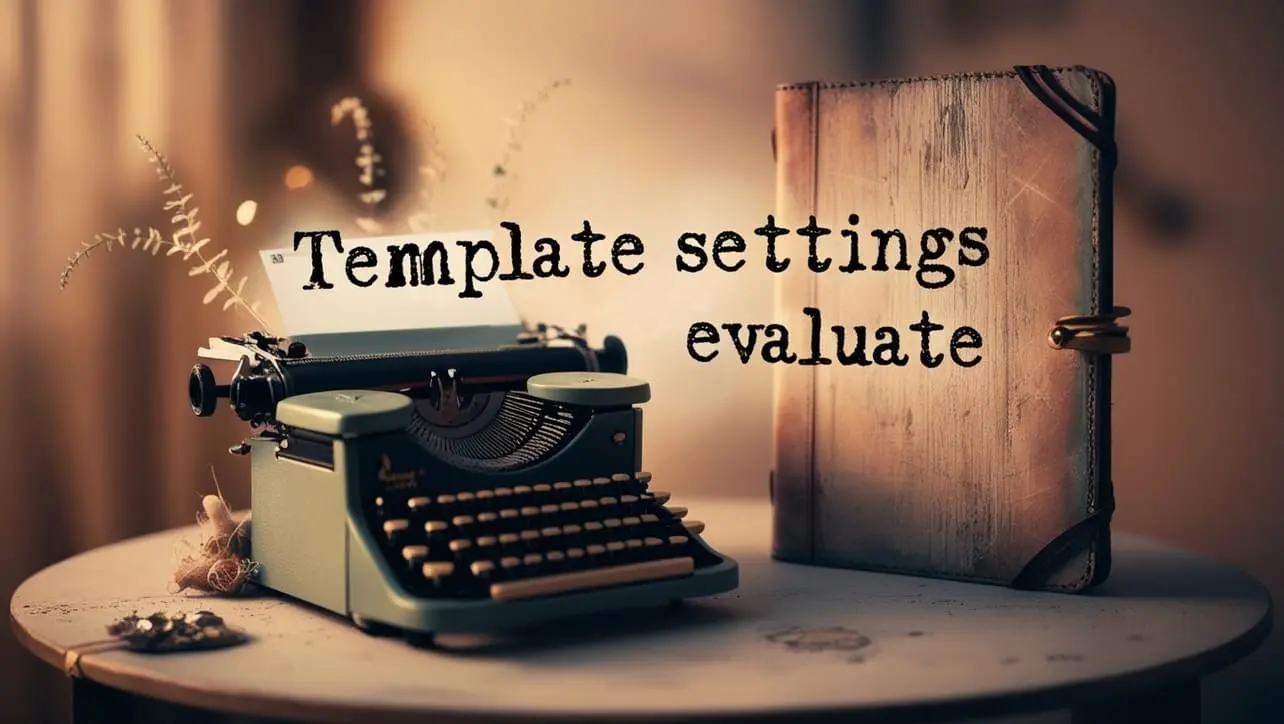
Lodash _.templateSettings.evaluate Property
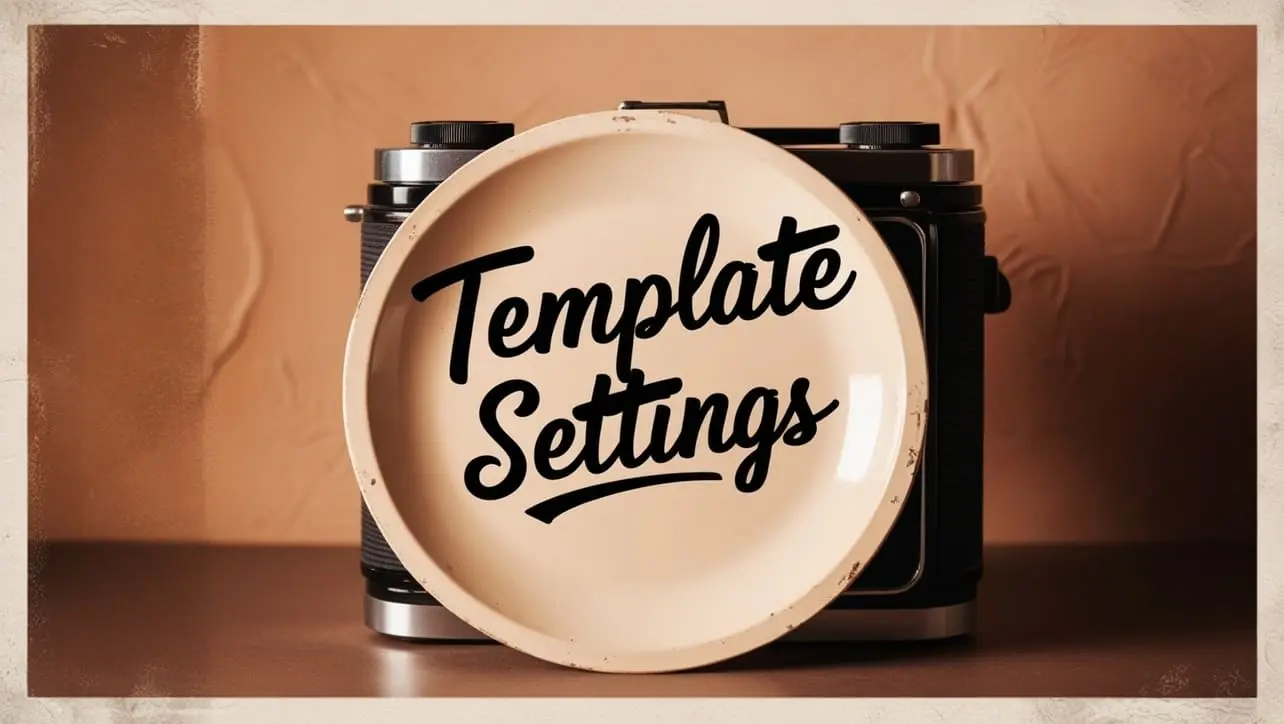
Lodash _.templateSettings Property
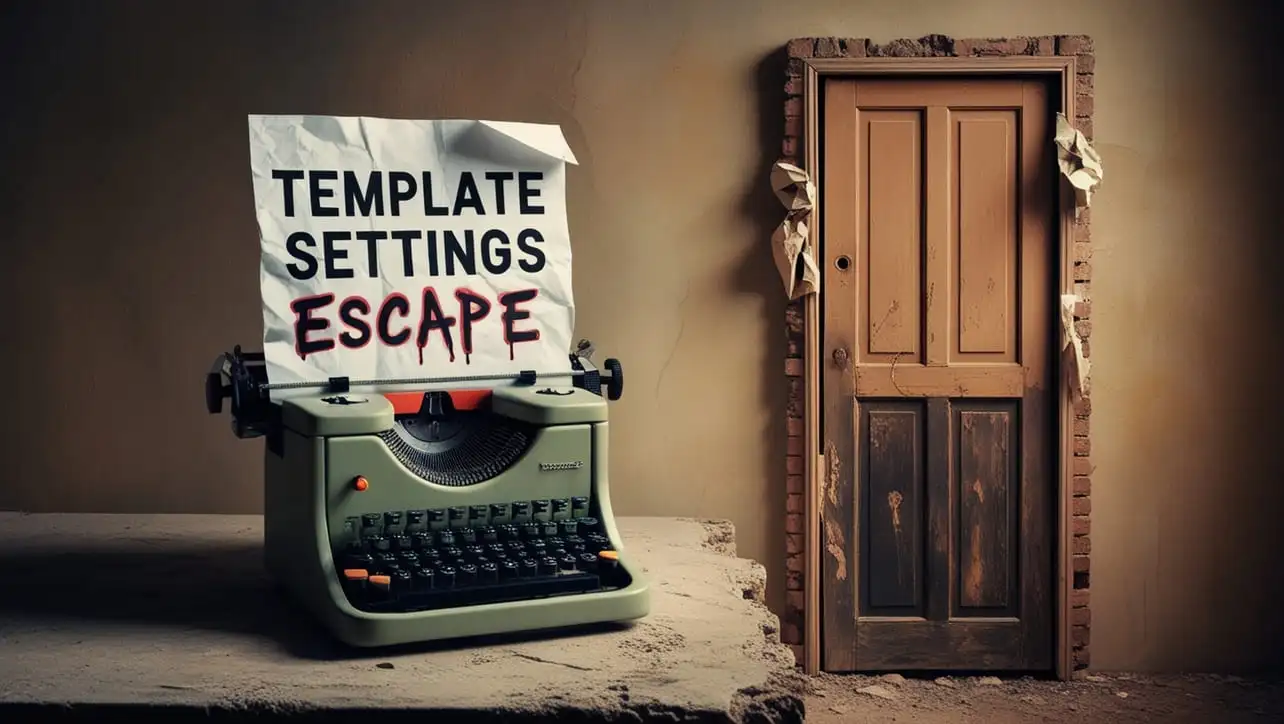
Lodash _.templateSettings.escape Property
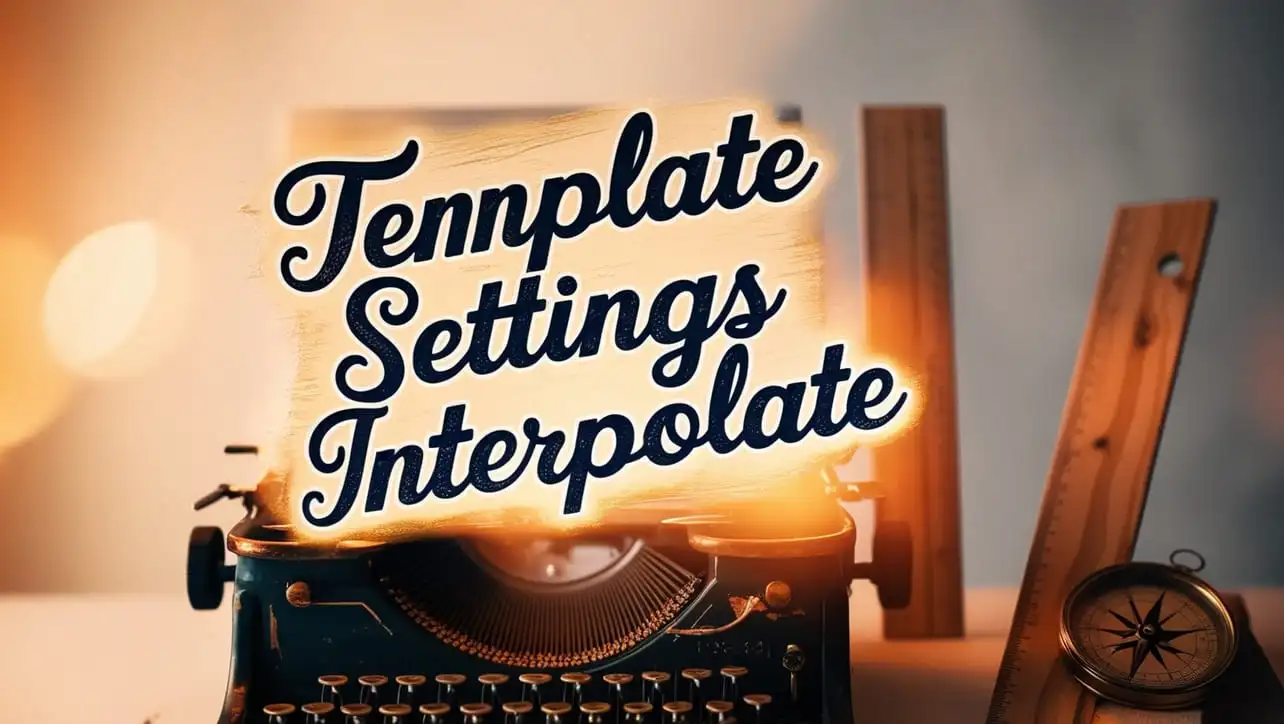
Lodash _.templateSettings.interpolate Property
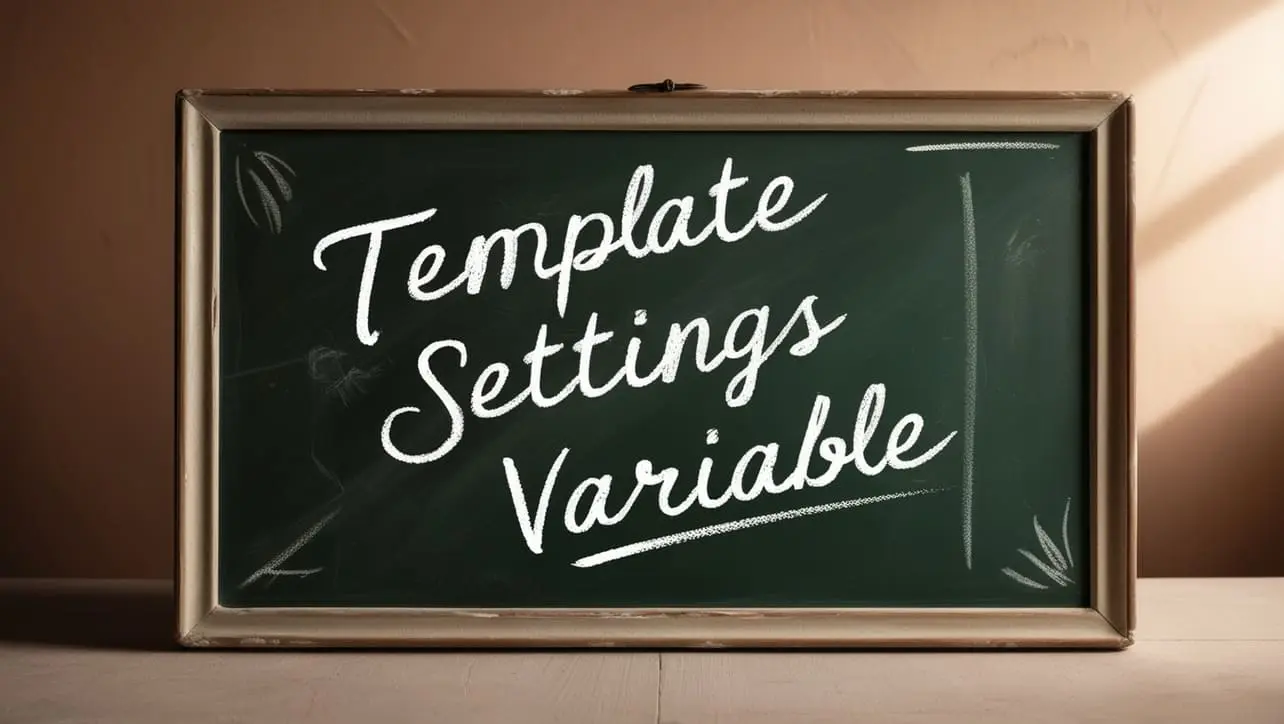
If you have any doubts regarding this article (Lodash _.unionBy() Array Method), please comment here. I will help you immediately.