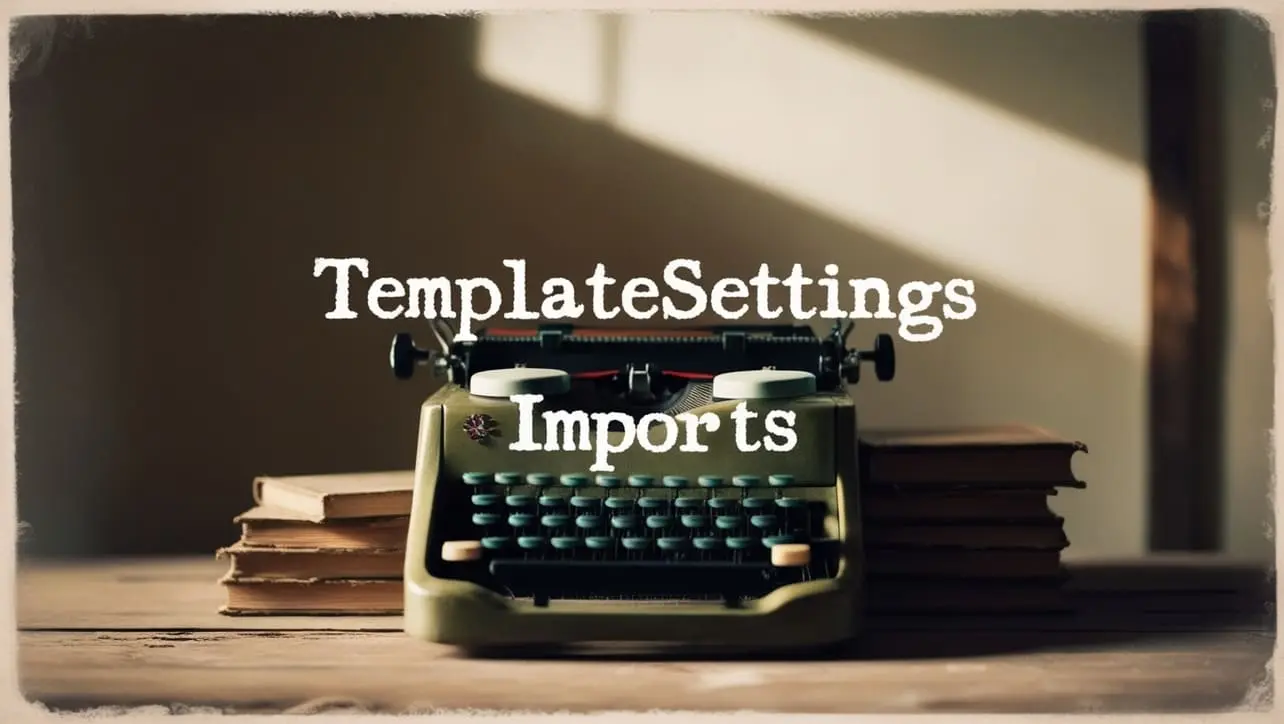
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.union() Array Method
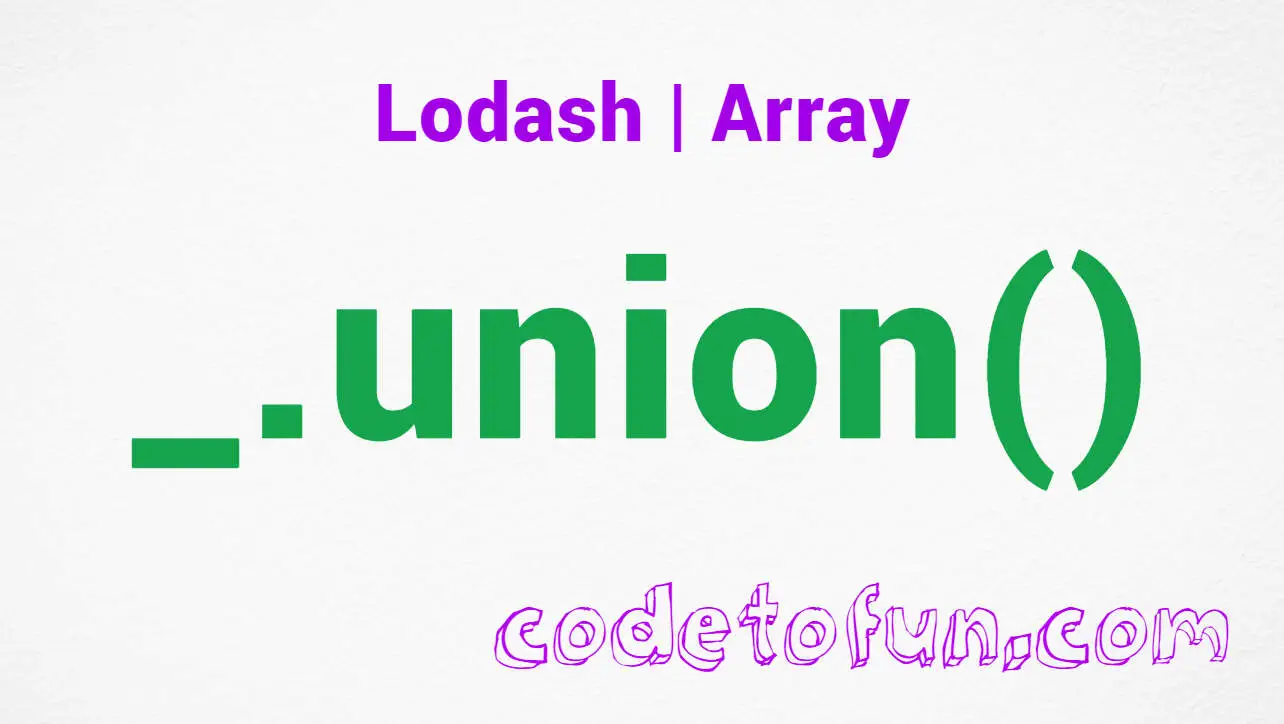
Photo Credit to CodeToFun
🙋 Introduction
Efficiently managing arrays is a common challenge in JavaScript development. Lodash, a powerful utility library, provides a wide array of functions to simplify these tasks. Among them is the _.union()
method, a versatile tool for creating a unique array that contains elements from all given arrays. This method proves invaluable when dealing with diverse datasets or merging arrays without duplicates.
🧠 Understanding _.union()
The _.union()
method in Lodash combines multiple arrays into a new array, excluding duplicate elements. This ensures that the resulting array only contains unique values, making it a reliable choice for array merging operations.
💡 Syntax
_.union([arrays])
- arrays: The arrays to process.
📝 Example
Let's explore a practical example to illustrate the functionality of _.union()
:
const _ = require('lodash');
const array1 = [1, 2, 3];
const array2 = [2, 3, 4, 5];
const array3 = [4, 5, 6];
const combinedArray = _.union(array1, array2, array3);
console.log(combinedArray);
// Output: [1, 2, 3, 4, 5, 6]
In this example, _.union()
merges three arrays (array1, array2, and array3) into a new array containing unique elements.
🏆 Best Practices
Understanding Order Matters:
The order of arrays passed to
_.union()
affects the order of elements in the resulting array. Elements from later arrays override those from earlier arrays.example.jsCopiedconst array1 = [1, 2, 3]; const array2 = [2, 3, 4, 5]; const array3 = [4, 5, 6]; const combinedArray1 = _.union(array1, array2, array3); const combinedArray2 = _.union(array3, array2, array1); console.log(combinedArray1); // Output: [1, 2, 3, 4, 5, 6] console.log(combinedArray2); // Output: [6, 5, 4, 2, 3, 1]
Handling Arrays of Objects:
When working with arrays of objects, use the iteratee function to specify how object uniqueness is determined.
example.jsCopiedconst array1 = [{ id: 1, name: 'Alice' }]; const array2 = [{ id: 2, name: 'Bob' }, { id: 1, name: 'Alice' }]; const combinedArray = _.unionWith(array1, array2, _.isEqual); console.log(combinedArray); // Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }]
Utilizing _.unionWith():
For more control over the comparison of elements, consider using _.unionWith() and providing a custom comparator function.
example.jsCopiedconst array1 = [{ id: 1, name: 'Alice' }]; const array2 = [{ id: 2, name: 'Bob' }, { id: 1, name: 'Alice' }]; const combinedArray = _.unionWith(array1, array2, (a, b) => a.id === b.id); console.log(combinedArray); // Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }]
📚 Use Cases
Merging Arrays Without Duplicates:
The primary use case for
_.union()
is merging arrays while excluding duplicate elements, ensuring a clean and unique result.example.jsCopiedconst userArray = [101, 102, 103]; const adminArray = [102, 103, 104, 105]; const moderatorArray = [103, 104, 105, 106]; const combinedRoles = _.union(userArray, adminArray, moderatorArray); console.log(combinedRoles); // Output: [101, 102, 103, 104, 105, 106]
User Preferences or Settings:
When dealing with user preferences or settings stored in arrays,
_.union()
can be employed to merge these arrays and ensure that only distinct preferences are retained.example.jsCopiedconst user1Preferences = ['dark-mode', 'font-size']; const user2Preferences = ['light-mode', 'font-size', 'language']; const mergedPreferences = _.union(user1Preferences, user2Preferences); console.log(mergedPreferences); // Output: ['dark-mode', 'font-size', 'light-mode', 'language']
Unique Values from Multiple Sources:
In scenarios where data comes from multiple sources,
_.union()
proves useful for creating a consolidated array of unique values.example.jsCopiedconst dataSource1 = [10, 20, 30]; const dataSource2 = [20, 30, 40, 50]; const dataSource3 = [30, 40, 50, 60]; const uniqueValues = _.union(dataSource1, dataSource2, dataSource3); console.log(uniqueValues); // Output: [10, 20, 30, 40, 50, 60]
🎉 Conclusion
The _.union()
method in Lodash provides an effective solution for merging arrays without duplicates. Whether you're consolidating user roles, preferences, or data from various sources, this method offers simplicity and reliability in array manipulation.
Explore the versatility of _.union()
and elevate your JavaScript development experience by efficiently handling arrays with unique values!
👨💻 Join our Community:
Author
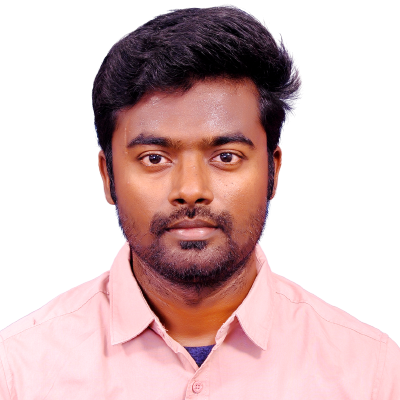
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
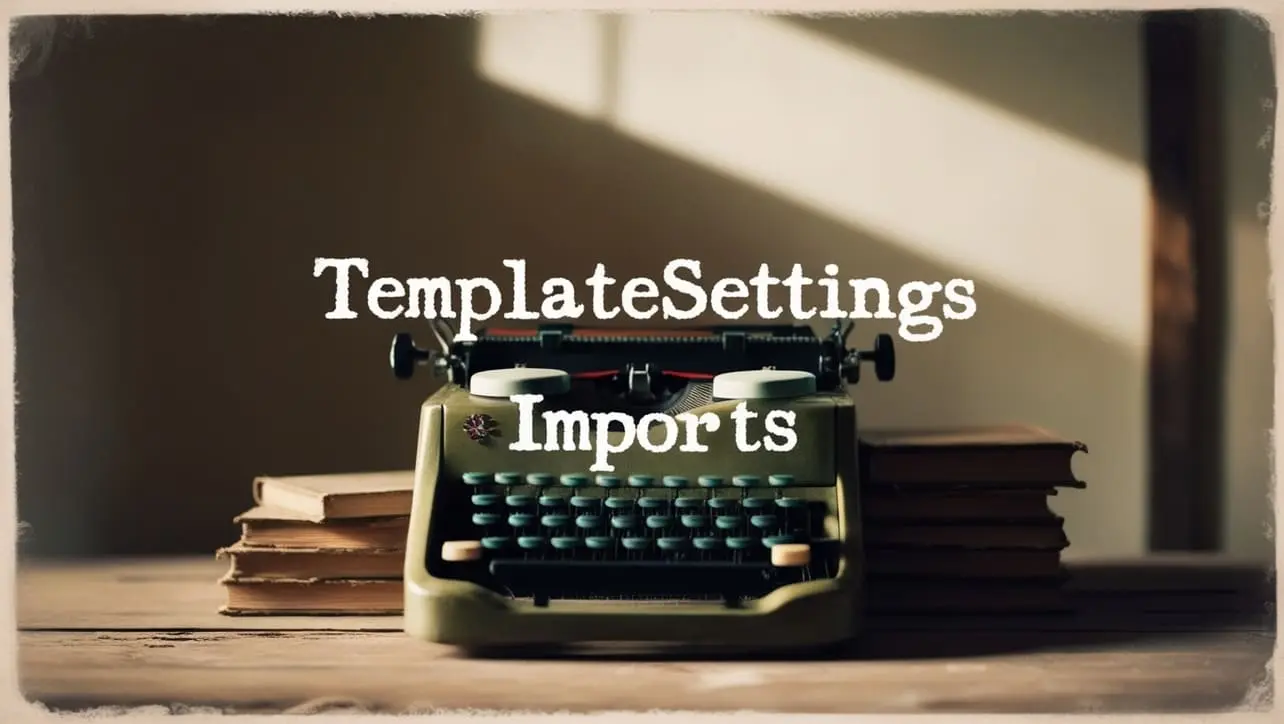
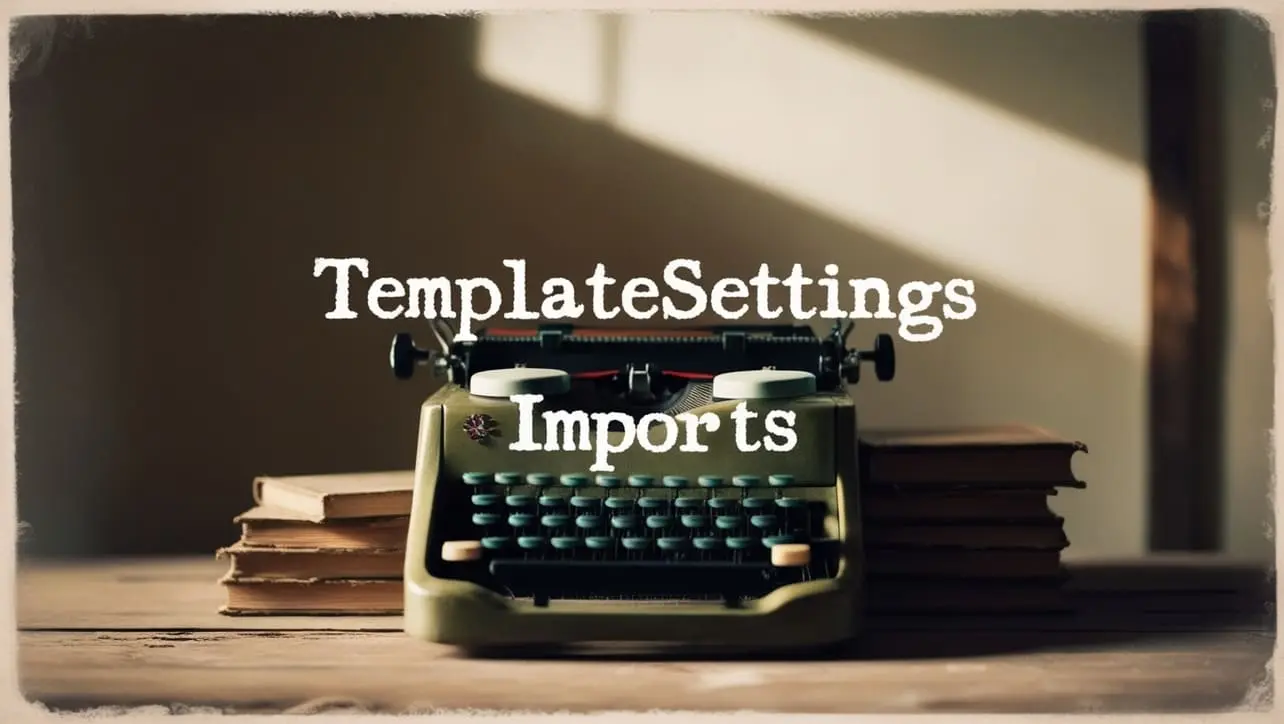
Lodash _.templateSettings.imports Property
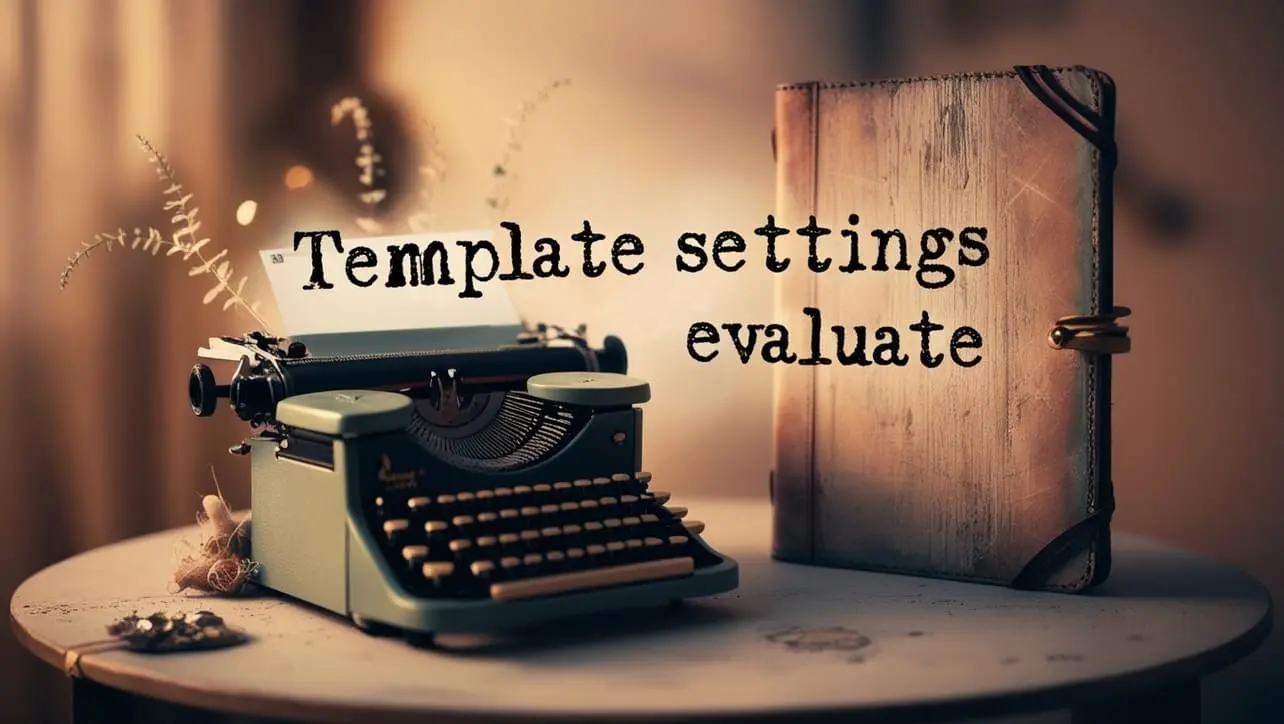
Lodash _.templateSettings.evaluate Property
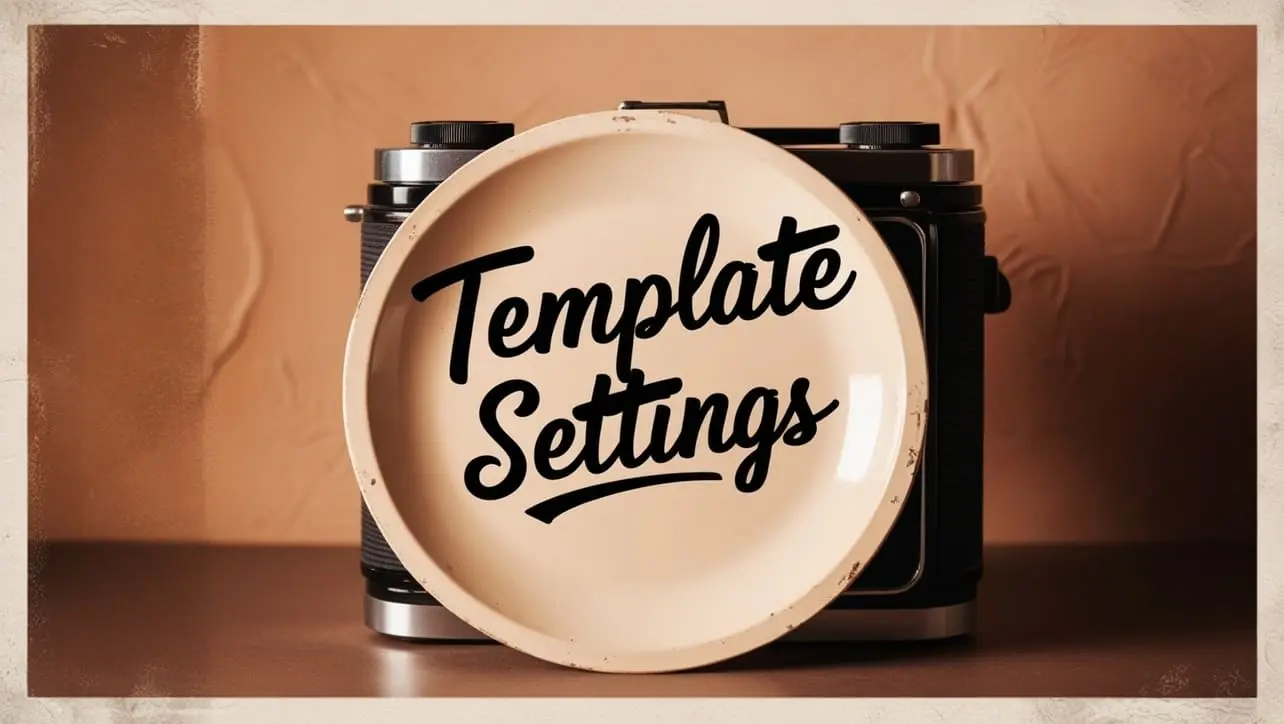
Lodash _.templateSettings Property
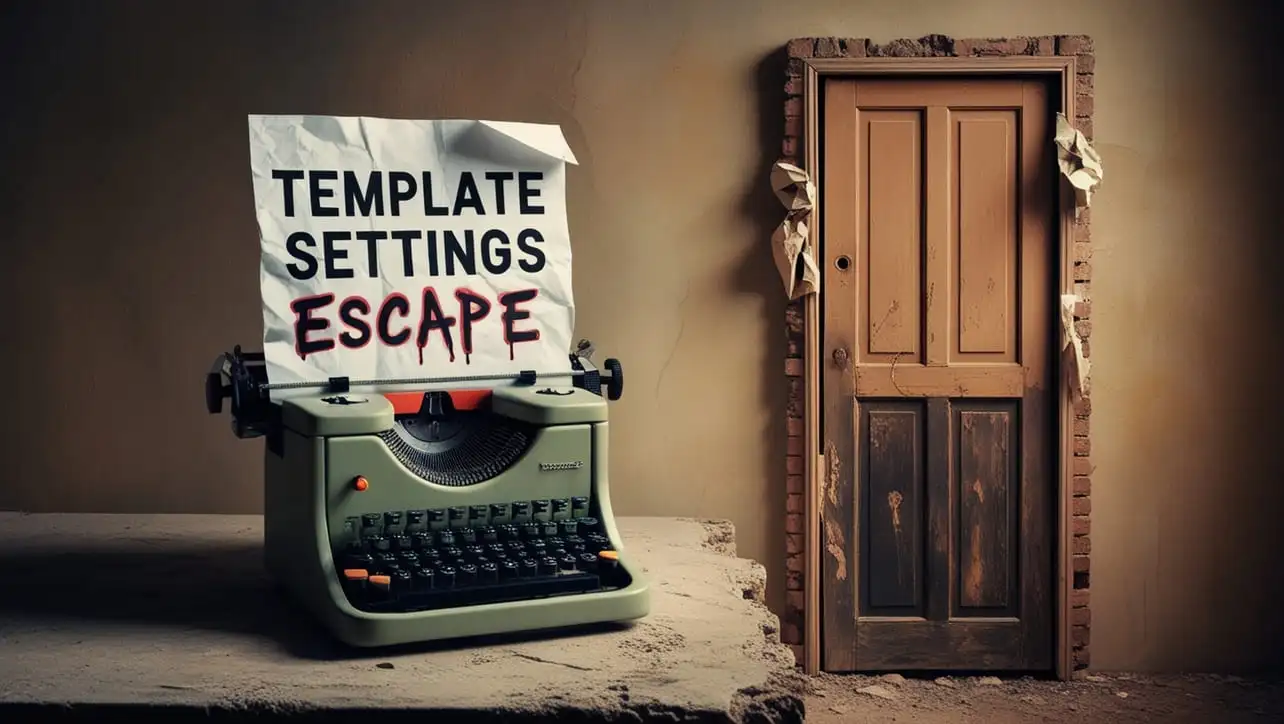
Lodash _.templateSettings.escape Property
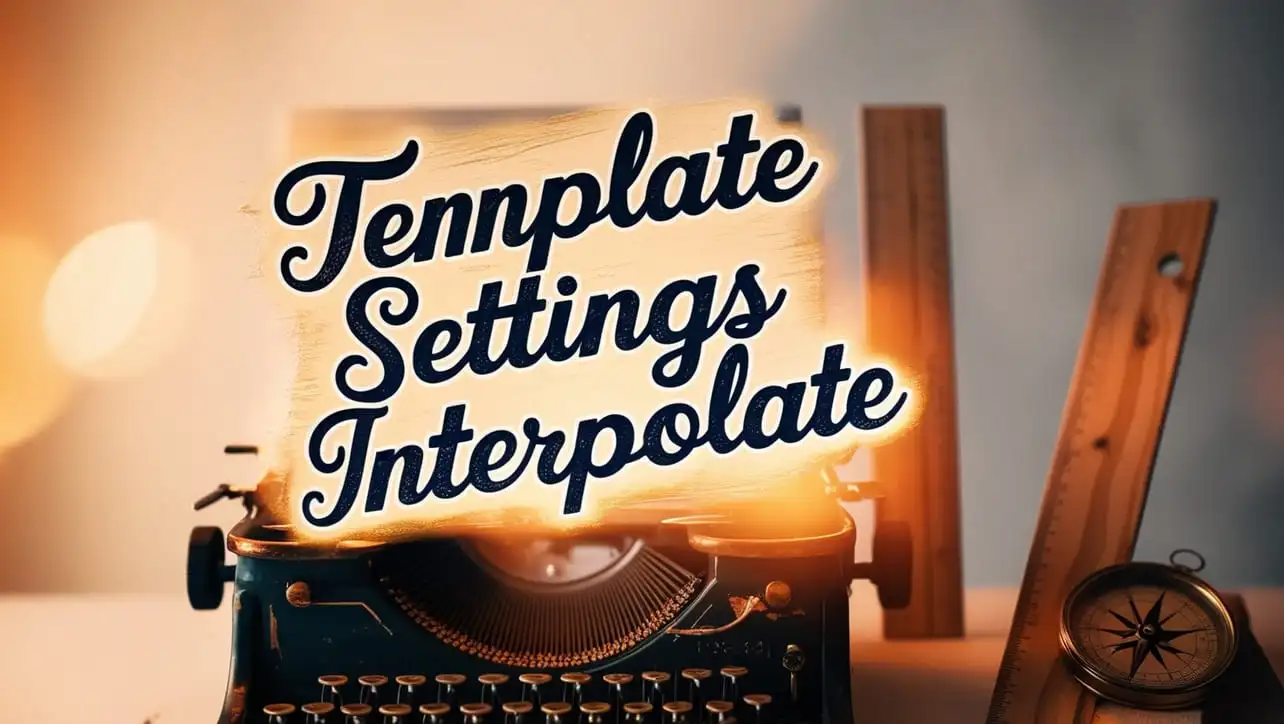
Lodash _.templateSettings.interpolate Property
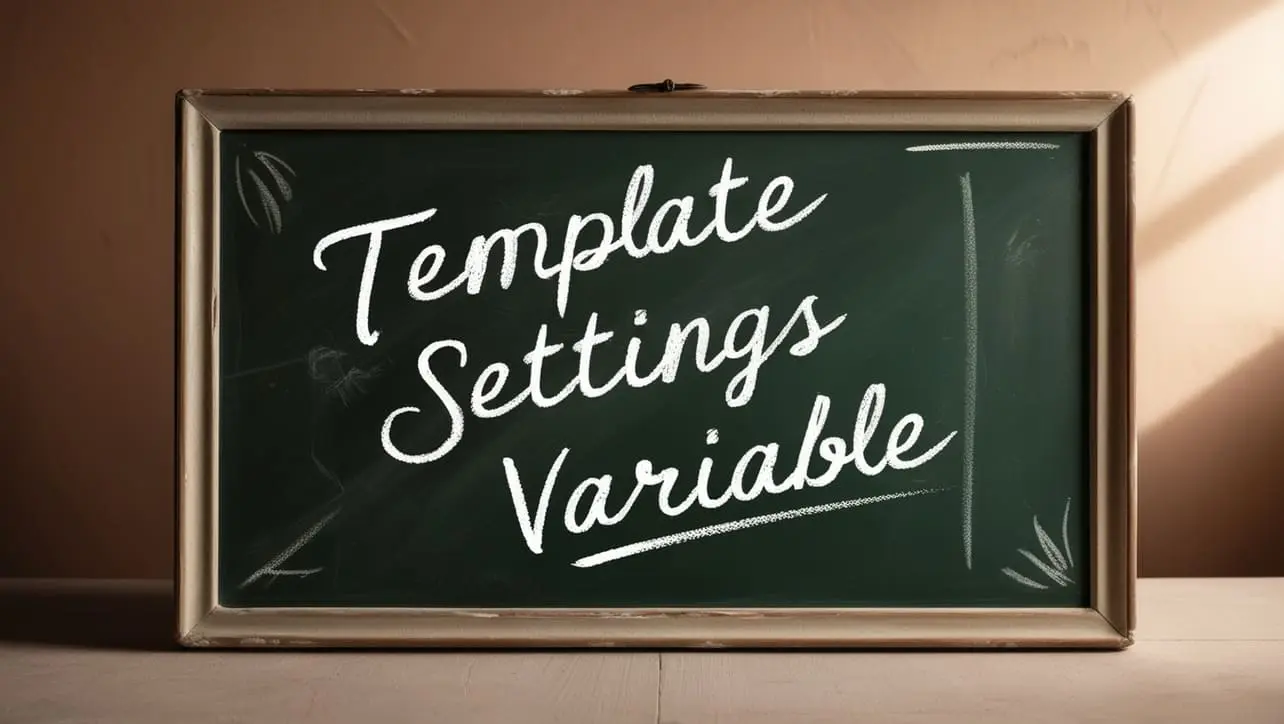
If you have any doubts regarding this article (Lodash _.union() Array Method), please comment here. I will help you immediately.