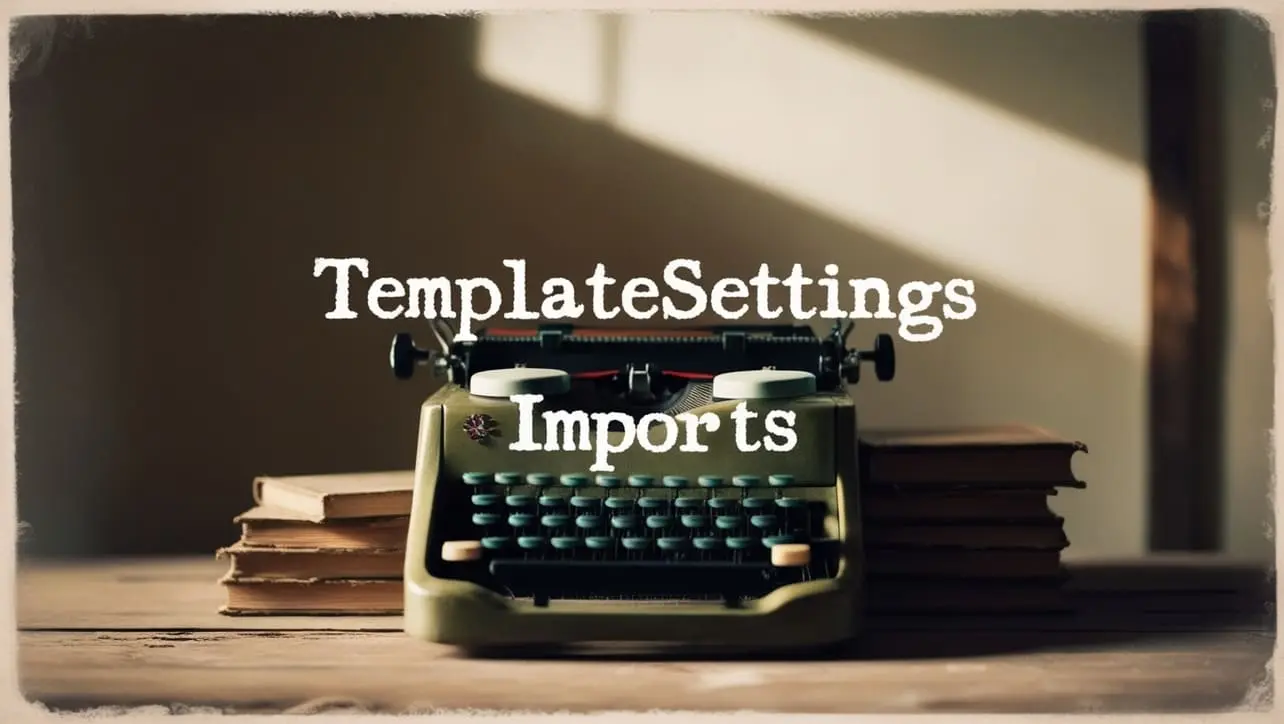
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.takeRightWhile() Array Method
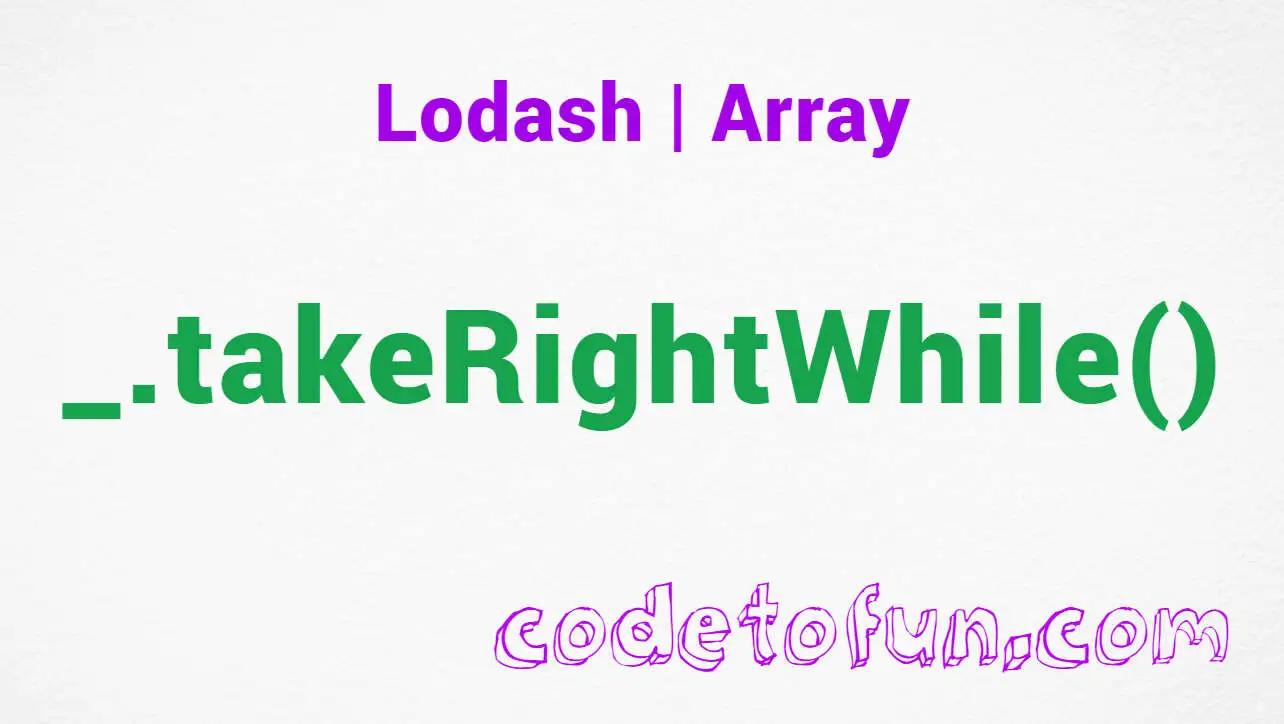
Photo Credit to CodeToFun
🙋 Introduction
Efficiently navigating and extracting data from arrays is a common task in JavaScript development. Lodash, a powerful utility library, offers a range of functions to streamline such operations. One notable method is _.takeRightWhile()
, designed to retrieve a contiguous portion of an array's tail based on a given predicate function. This method provides developers with a versatile tool for extracting relevant data efficiently.
🧠 Understanding _.takeRightWhile()
The _.takeRightWhile()
method in Lodash allows you to extract elements from the end of an array until the provided predicate function returns a falsy value. This facilitates the extraction of a subarray that meets specific criteria from the trailing portion of the original array.
💡 Syntax
_.takeRightWhile(array, [predicate=_.identity])
- array: The array to process.
- predicate (Optional): The function invoked per iteration.
📝 Example
Let's explore a practical example to understand the functionality of _.takeRightWhile()
:
const _ = require('lodash');
const users = [
{ id: 1, name: 'Alice', active: true },
{ id: 2, name: 'Bob', active: true },
{ id: 3, name: 'Charlie', active: false },
{ id: 4, name: 'David', active: false },
{ id: 5, name: 'Eve', active: true },
];
const activeUsers = _.takeRightWhile(users, user => user.active);
console.log(activeUsers);
// Output: [{ id: 5, name: 'Eve', active: true }]
In this example, the activeUsers array is derived by taking elements from the end of the users array until a user with active: false is encountered.
🏆 Best Practices
Understand Predicate Function:
Ensure a clear understanding of the predicate function used in
_.takeRightWhile()
. The predicate determines the condition for including elements in the result array.example.jsCopiedconst numbers = [5, 10, 15, 20, 25]; const result = _.takeRightWhile(numbers, n => n > 15); console.log(result); // Output: [20, 25]
Handle Complex Predicates:
Handle more complex predicates by customizing the predicate function to accommodate multiple conditions.
example.jsCopiedconst products = [ { id: 1, name: 'Laptop', price: 1200, inStock: true }, { id: 2, name: 'Smartphone', price: 800, inStock: false }, { id: 3, name: 'Tablet', price: 400, inStock: true }, ]; const expensiveAndInStock = _.takeRightWhile(products, p => p.price > 500 && p.inStock); console.log(expensiveAndInStock); // Output: [{ id: 1, name: 'Laptop', price: 1200, inStock: true }]
Combine with Other Methods:
Combine
_.takeRightWhile()
with other Lodash methods to create more advanced data extraction workflows.example.jsCopiedconst data = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const result = _.takeRightWhile(_.sortBy(data), n => n > 5); console.log(result); // Output: [6, 7, 8, 9]
📚 Use Cases
Filtering Recent Activities:
Use
_.takeRightWhile()
to filter recent activities based on a timestamp, ensuring that only the latest activities are included.example.jsCopiedconst activities = [ { id: 1, action: 'comment', timestamp: 1646304000000 }, { id: 2, action: 'like', timestamp: 1646390400000 }, { id: 3, action: 'share', timestamp: 1646476800000 }, { id: 4, action: 'comment', timestamp: 1646563200000 }, ]; const recentActivities = _.takeRightWhile(activities, activity => Date.now() - activity.timestamp < 24 * 60 * 60 * 1000); console.log(recentActivities);
Extracting Unfinished Tasks:
Utilize
_.takeRightWhile()
to extract the most recent unfinished tasks from a task list.example.jsCopiedconst tasks = [ { id: 1, description: 'Task 1', completed: true }, { id: 2, description: 'Task 2', completed: true }, { id: 3, description: 'Task 3', completed: false }, { id: 4, description: 'Task 4', completed: false }, ]; const unfinishedTasks = _.takeRightWhile(tasks, task => !task.completed); console.log(unfinishedTasks);
Tailoring Notifications:
Tailor notifications by using
_.takeRightWhile()
to extract the most recent relevant events.example.jsCopiedconst notifications = [ { id: 1, message: 'New message', type: 'message' }, { id: 2, message: 'Friend request', type: 'friend-request' }, { id: 3, message: 'Product update', type: 'update' }, ]; const recentMessages = _.takeRightWhile(notifications, notification => notification.type === 'message'); console.log(recentMessages);
🎉 Conclusion
The _.takeRightWhile()
method in Lodash empowers developers to efficiently extract elements from the end of an array based on a specified condition. Whether filtering recent activities, extracting unfinished tasks, or tailoring notifications, this method provides a versatile solution for data extraction in JavaScript.
Incorporate _.takeRightWhile()
into your array manipulation toolkit and elevate your JavaScript development workflow!
👨💻 Join our Community:
Author
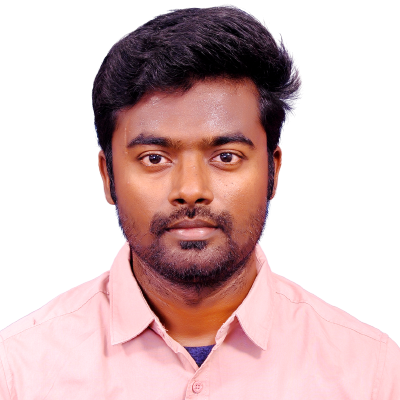
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
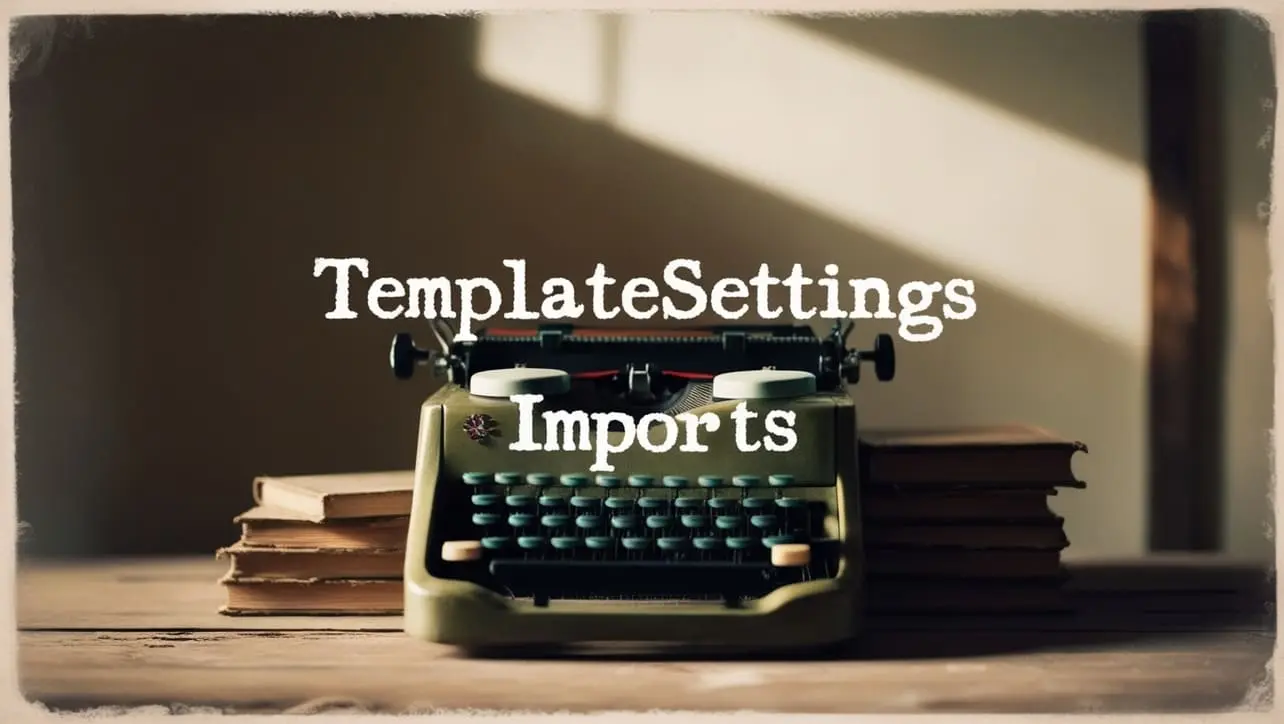
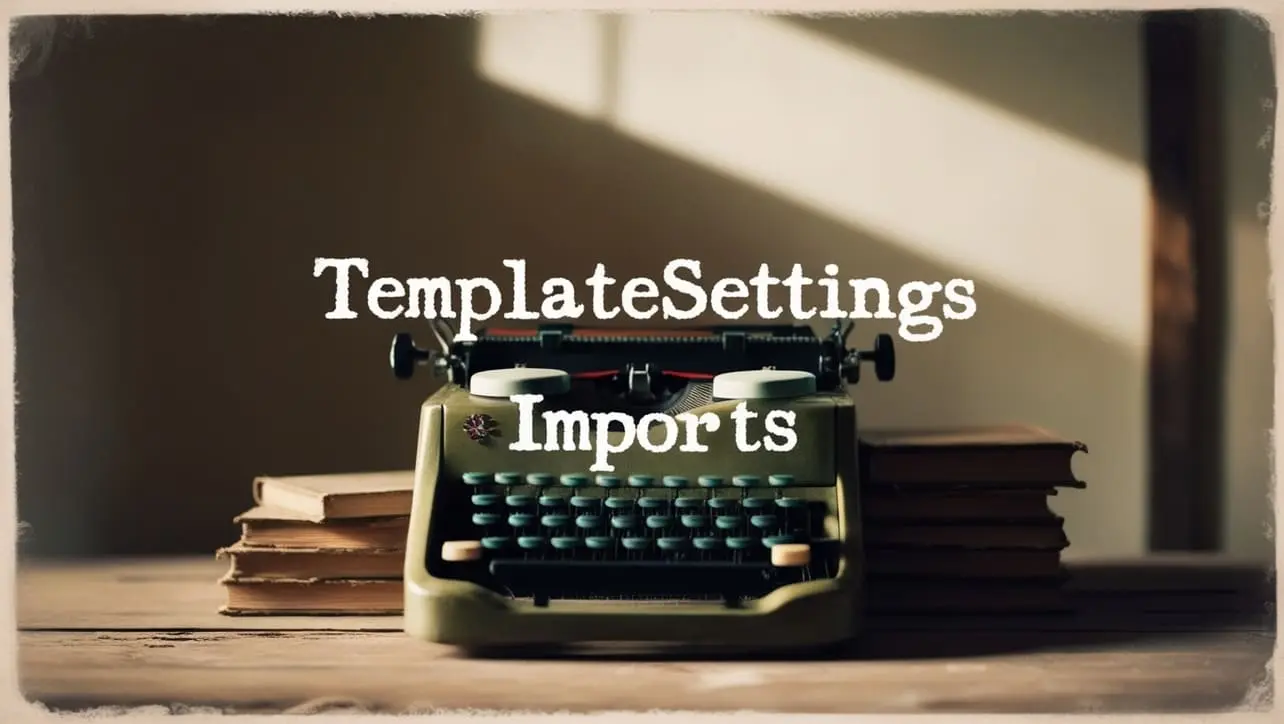
Lodash _.templateSettings.imports Property
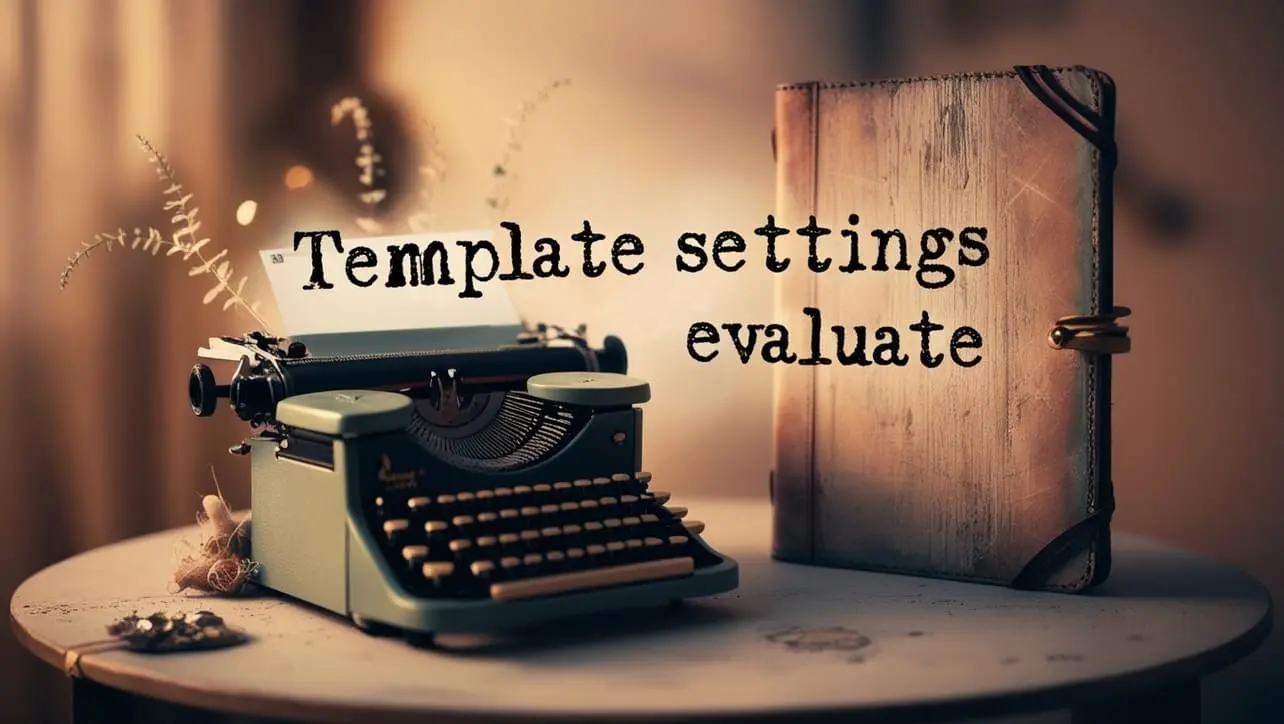
Lodash _.templateSettings.evaluate Property
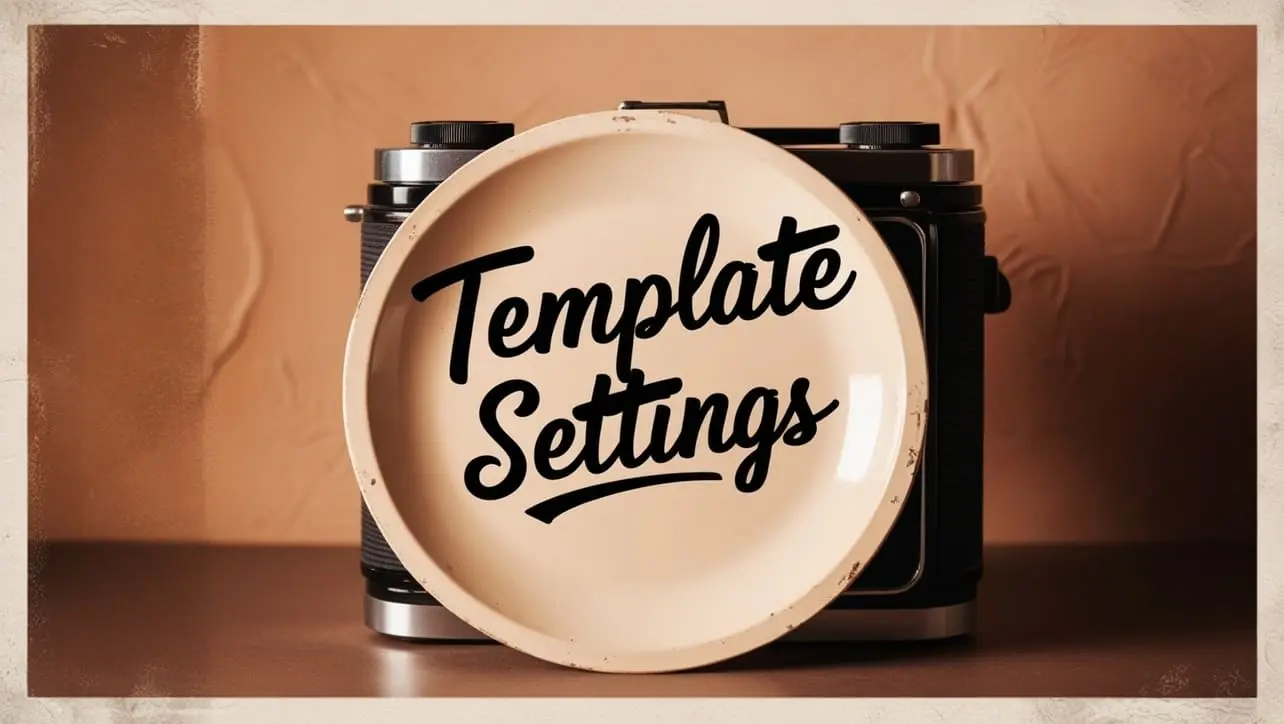
Lodash _.templateSettings Property
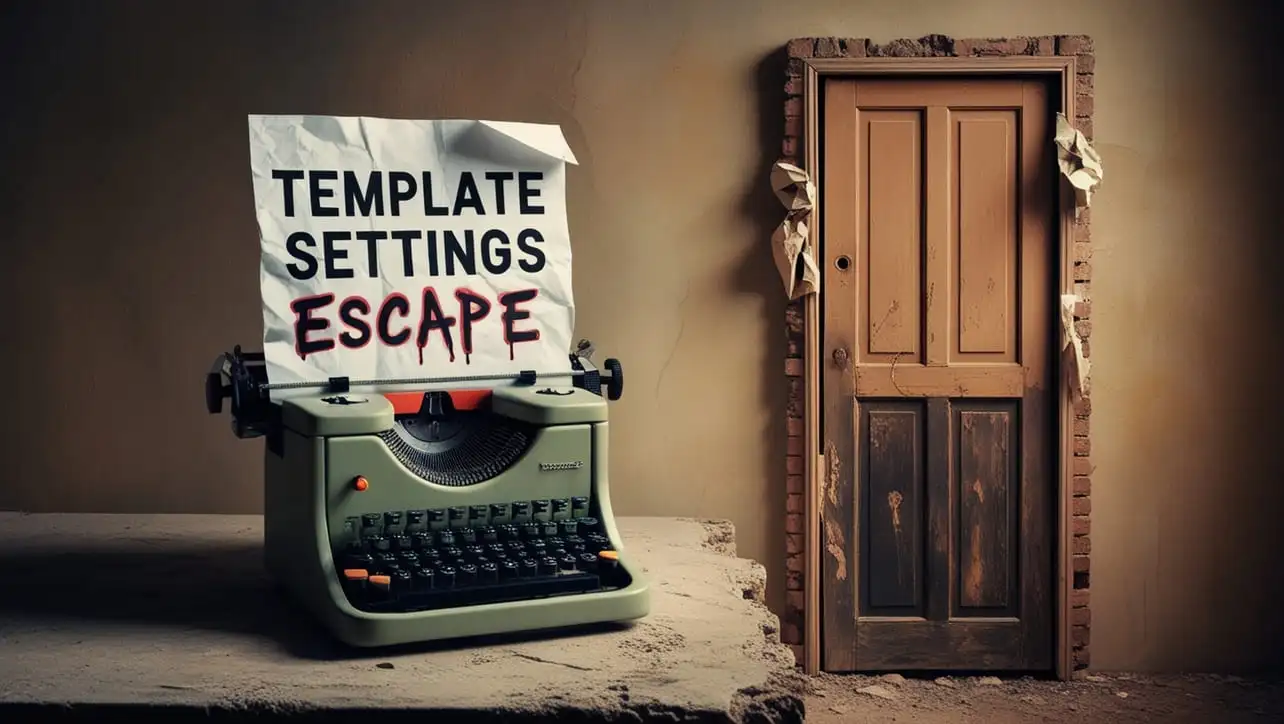
Lodash _.templateSettings.escape Property
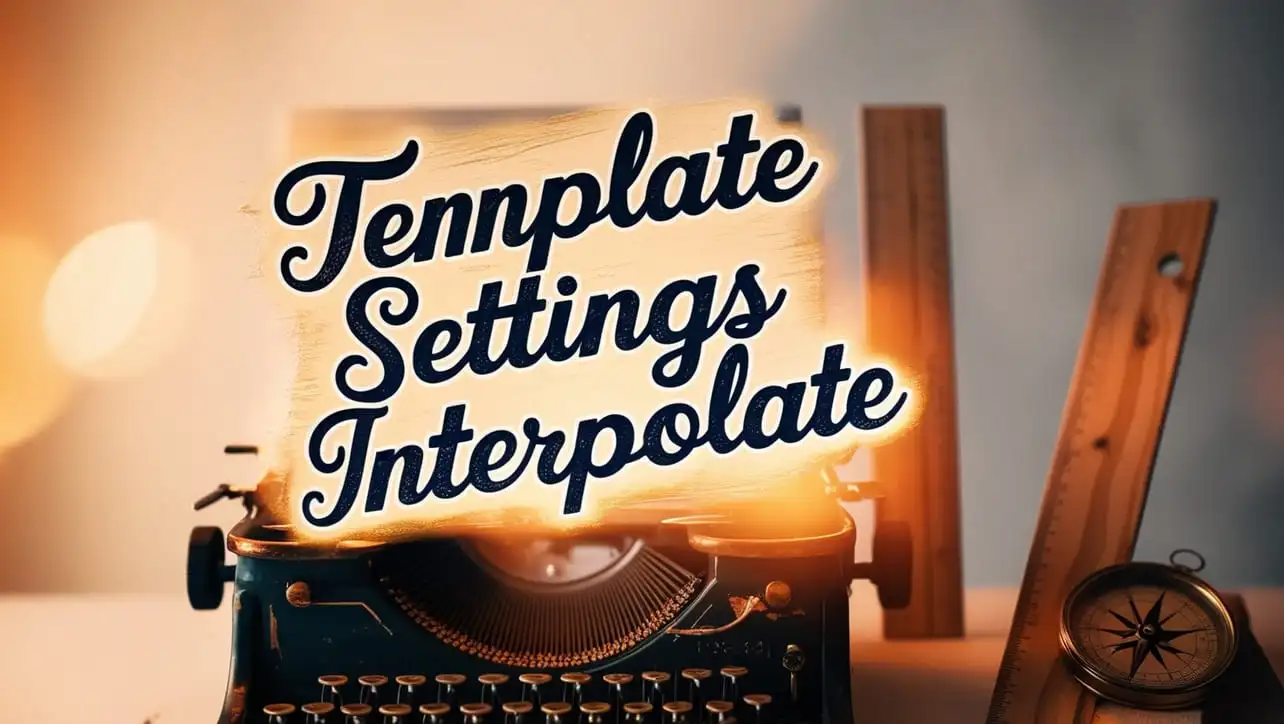
Lodash _.templateSettings.interpolate Property
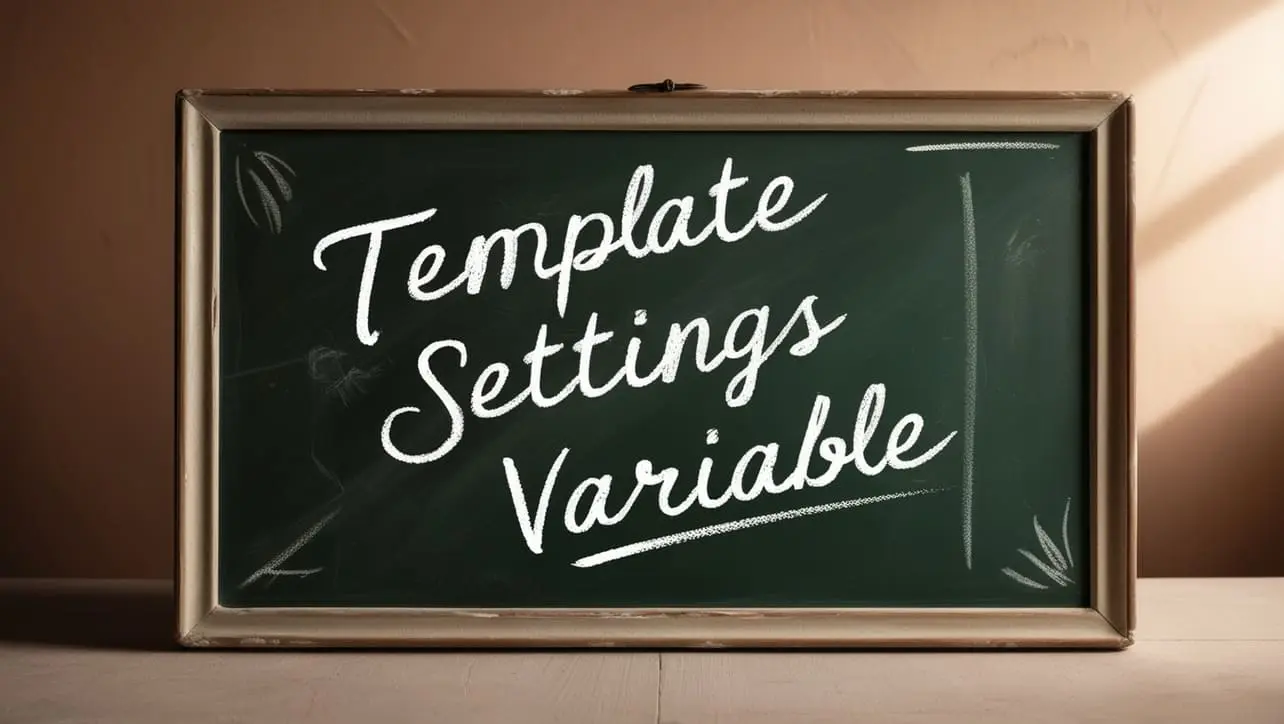
If you have any doubts regarding this article (Lodash _.takeRightWhile() Array Method), please comment here. I will help you immediately.