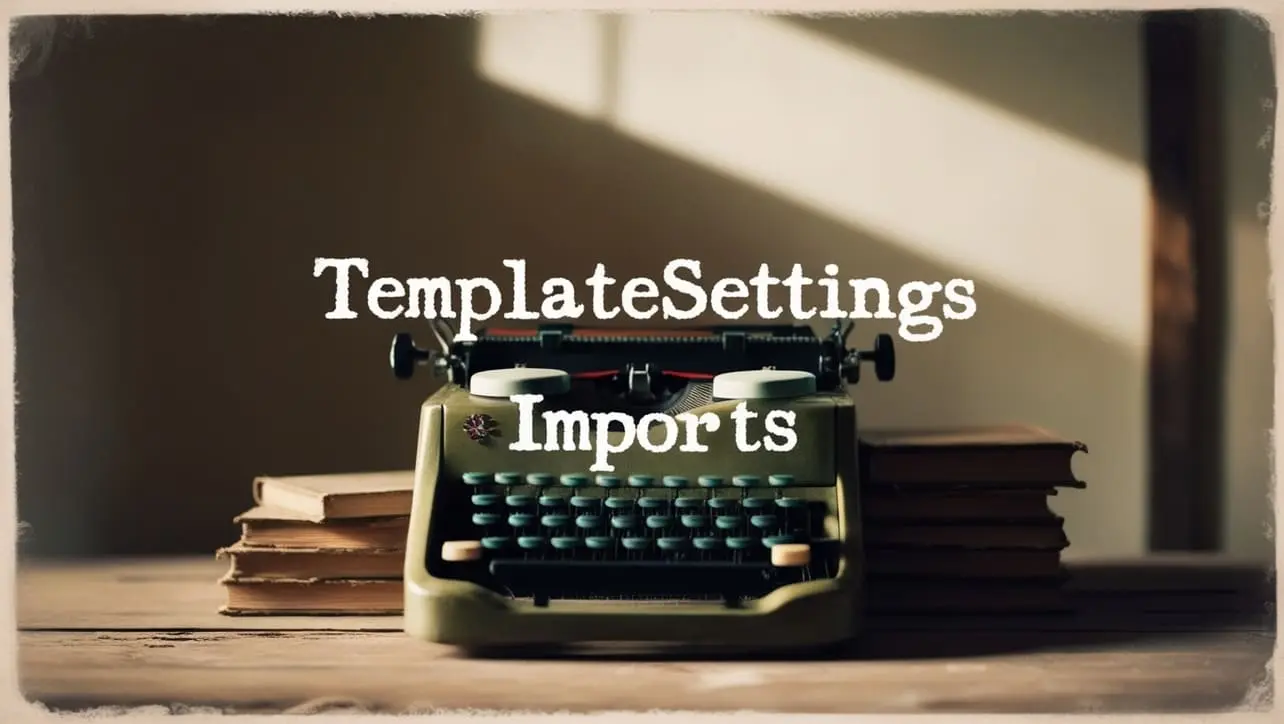
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.takeRight() Array Method
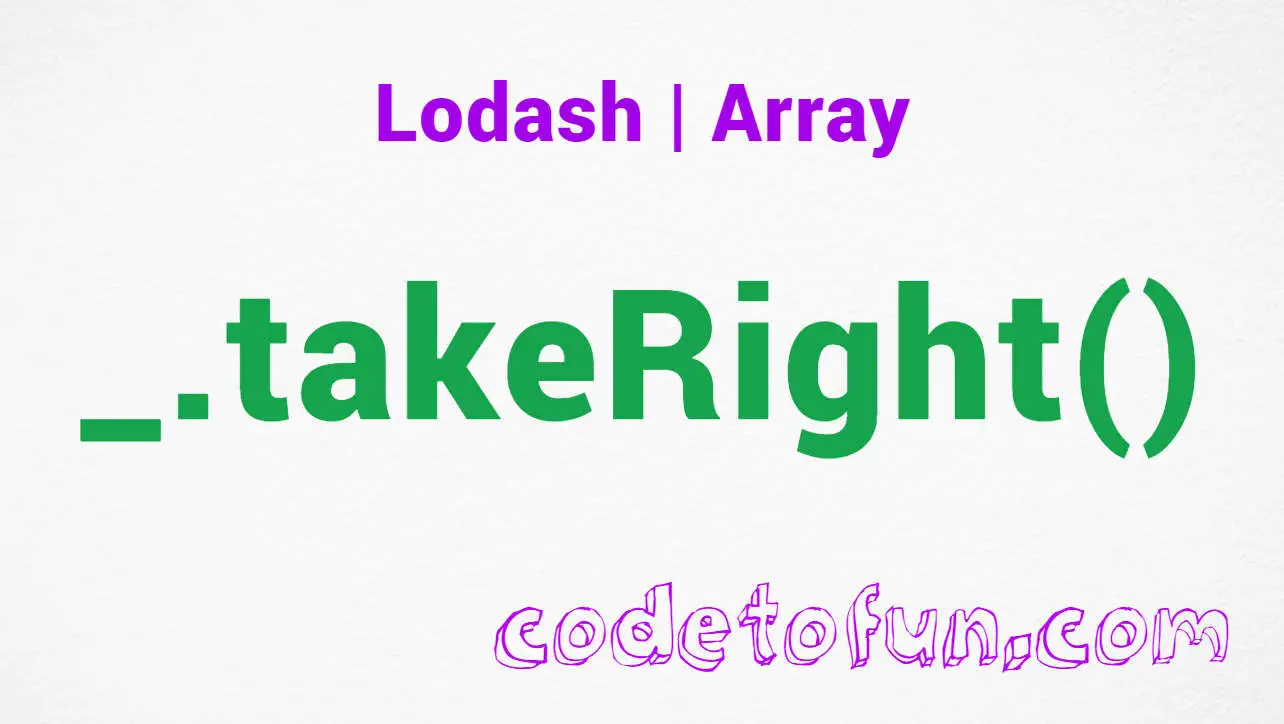
Photo Credit to CodeToFun
🙋 Introduction
When it comes to handling arrays in JavaScript, developers often encounter scenarios where extracting a specific number of elements from the end of an array is essential. Enter Lodash, a utility library that simplifies array manipulation. Within its arsenal of functions is the _.takeRight()
method, a powerful tool designed to retrieve the last elements of an array effortlessly.
In this guide, we'll explore the intricacies of _.takeRight()
and discover how it can enhance your array manipulation capabilities.
🧠 Understanding _.takeRight()
The _.takeRight()
method in Lodash is designed to create a slice of an array with n elements taken from the end. This allows developers to conveniently extract the last elements of an array, whether it's for displaying recent items, handling pagination, or other use cases where accessing the end of an array is crucial.
💡 Syntax
_.takeRight(array, [n=1])
- array: The array to query.
- n (Optional): The number of elements to take from the end of the array (default is 1).
📝 Example
Let's delve into a practical example to grasp the functionality of _.takeRight()
:
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5];
const lastTwoElements = _.takeRight(originalArray, 2);
console.log(lastTwoElements);
// Output: [4, 5]
In this example, the originalArray is processed by _.takeRight()
, resulting in a new array containing the last two elements.
🏆 Best Practices
Validating Inputs:
Before using
_.takeRight()
, ensure that the input array is valid. Additionally, validate the value of n to avoid unexpected behavior.example.jsCopiedconst inputArray = [1, 2, 3, 4, 5]; const numberOfElements = 3; if (!Array.isArray(inputArray) || inputArray.length === 0) { console.error('Invalid input array'); return; } const result = _.takeRight(inputArray, numberOfElements); console.log(result);
Edge Cases Handling:
Consider edge cases, such as when the specified number of elements (n) is greater than the length of the array. Implement appropriate error handling or default behaviors to address these scenarios.
example.jsCopiedconst arrayWithFewerElements = [1, 2]; const requestedNumberOfElements = 5; const resultWithDefault = _.takeRight(arrayWithFewerElements, requestedNumberOfElements); console.log(resultWithDefault); // Output: [1, 2] (since there are fewer elements than requested)
Use in Pagination:
For scenarios where paginating through data is required,
_.takeRight()
can be employed to efficiently retrieve the last page of results.example.jsCopiedconst dataForPagination = /* ...fetch data from API or elsewhere... */; const pageSize = 10; const lastPage = _.takeRight(dataForPagination, pageSize); console.log(lastPage);
📚 Use Cases
Displaying Recent Items:
_.takeRight()
is handy when you want to display the most recent items in a list, ensuring a user sees the latest updates or additions.example.jsCopiedconst recentItems = /* ...fetch recent items from a database or API... */; const numberOfItemsToShow = 5; const lastFiveRecentItems = _.takeRight(recentItems, numberOfItemsToShow); console.log(lastFiveRecentItems);
Handling Pagination:
In scenarios where you're paginating through a large dataset,
_.takeRight()
can simplify retrieving the last page of results.example.jsCopiedconst dataForPagination = /* ...fetch data from API or elsewhere... */; const pageSize = 10; const lastPage = _.takeRight(dataForPagination, pageSize); console.log(lastPage);
Handling User Input:
When dealing with user input or dynamic scenarios,
_.takeRight()
can be used to easily handle a specified number of items from the end.example.jsCopiedconst userInputArray = /* ...get user input as an array... */; const numberOfItemsToTake = 3; const result = _.takeRight(userInputArray, numberOfItemsToTake); console.log(result);
🎉 Conclusion
The _.takeRight()
method in Lodash is a versatile solution for extracting elements from the end of an array with ease. Whether you're dealing with recent items, implementing pagination, or handling dynamic user input, this method provides a clean and efficient way to retrieve the desired elements.
Explore the capabilities of _.takeRight()
and enhance your array manipulation toolkit in JavaScript!
👨💻 Join our Community:
Author
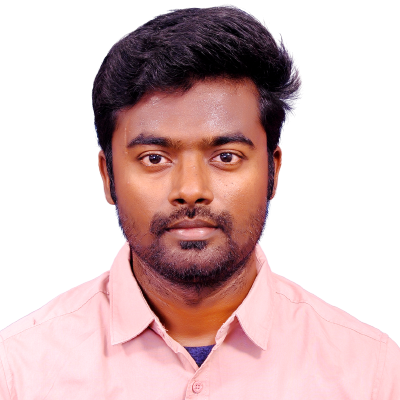
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
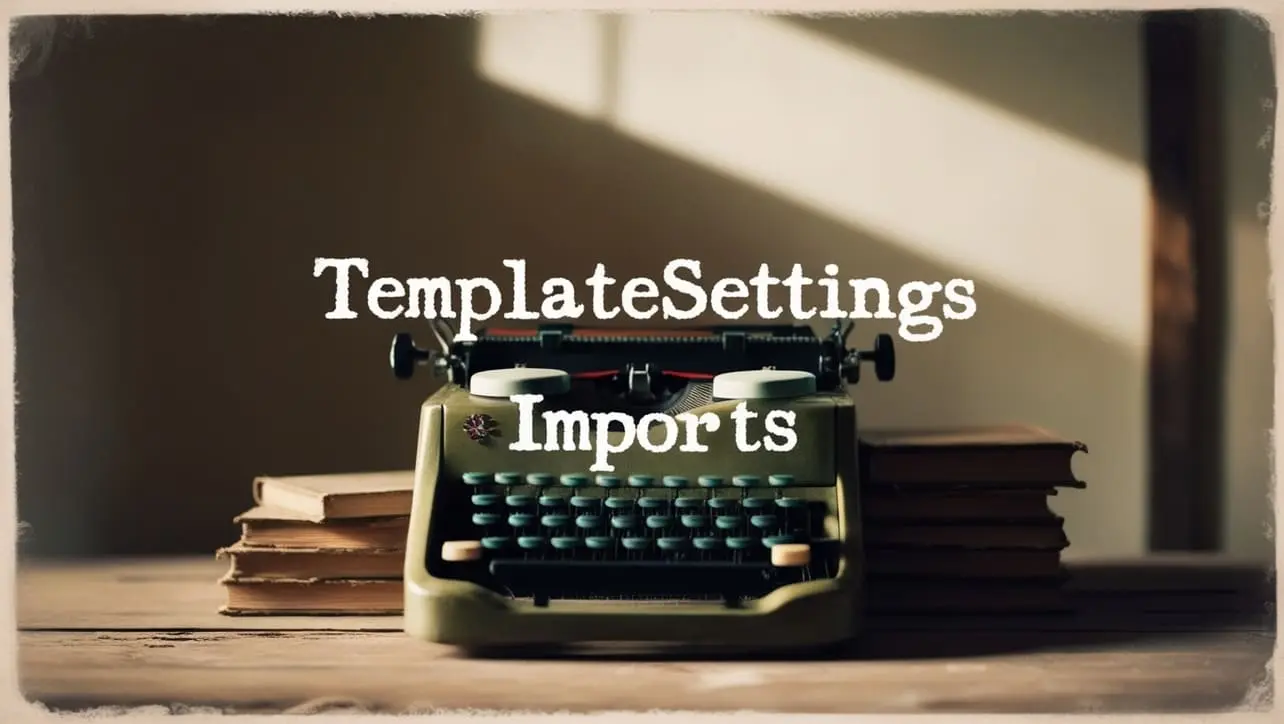
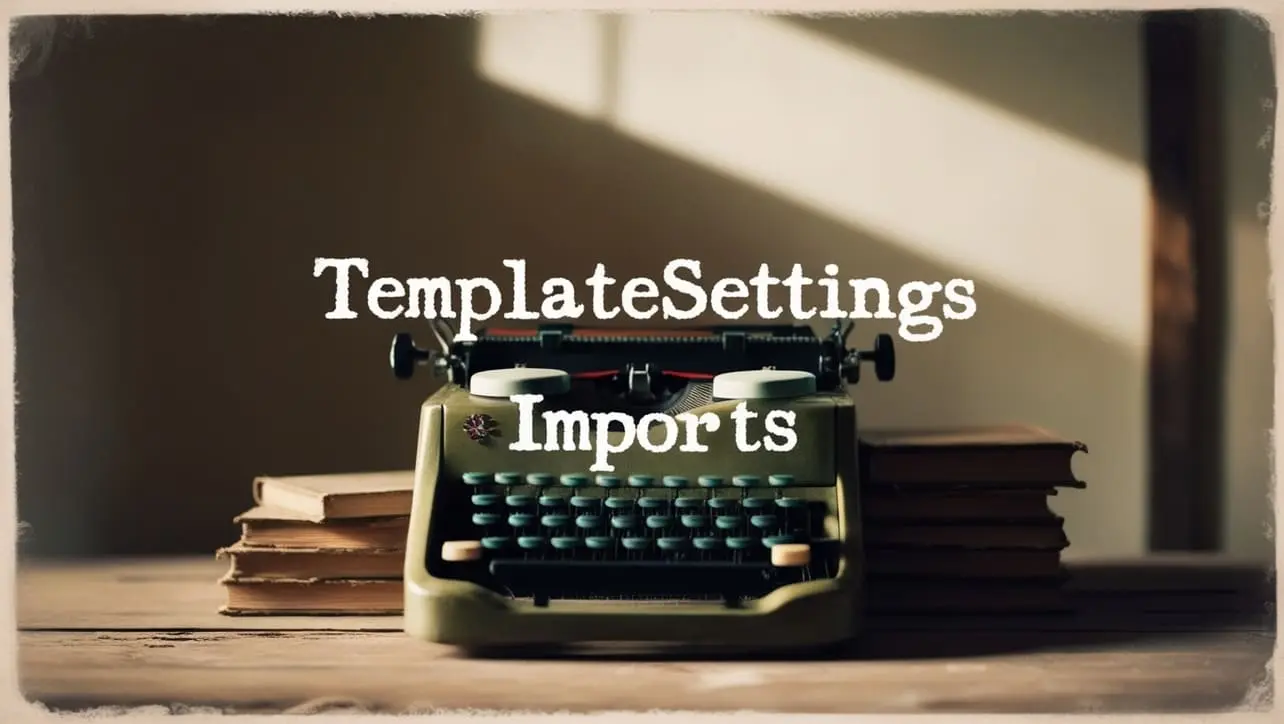
Lodash _.templateSettings.imports Property
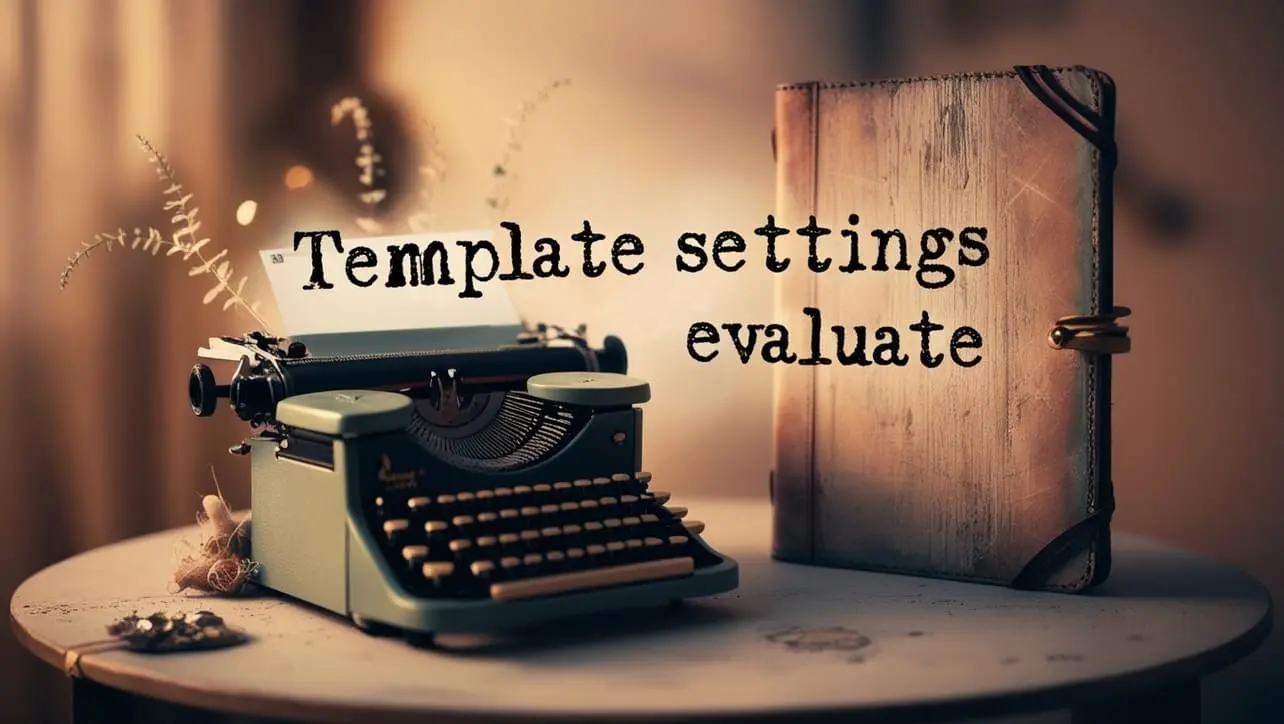
Lodash _.templateSettings.evaluate Property
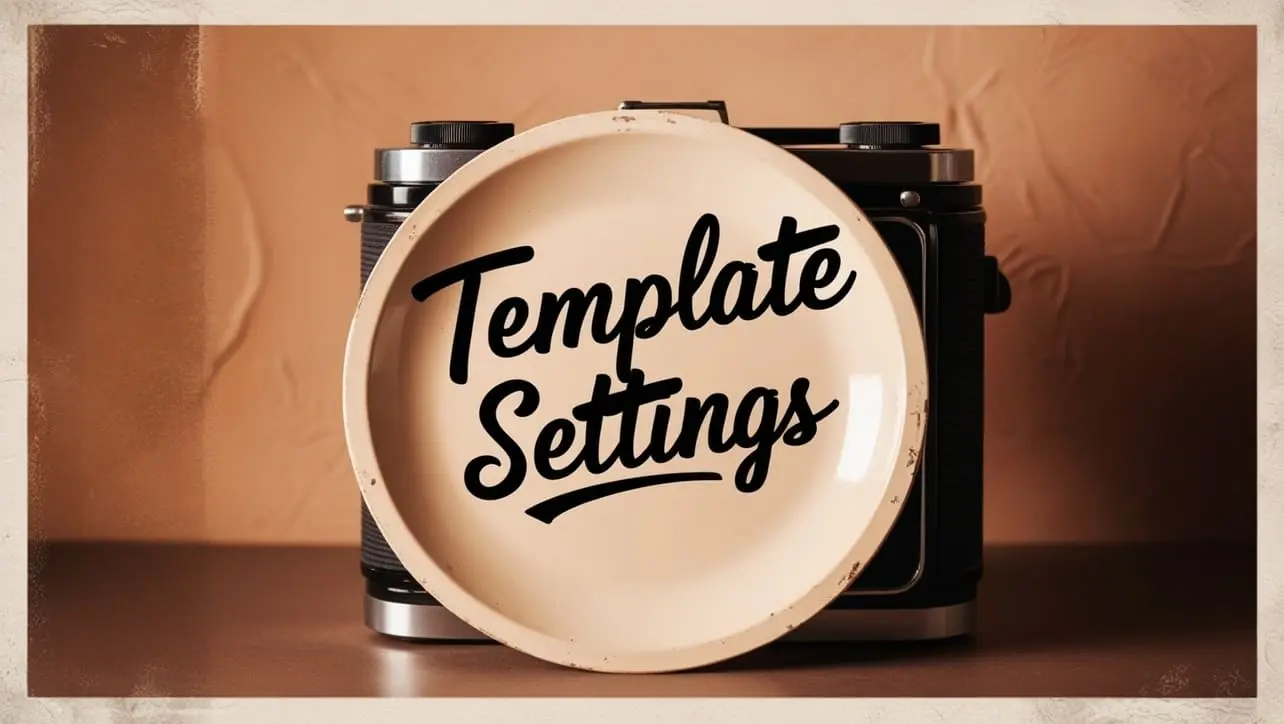
Lodash _.templateSettings Property
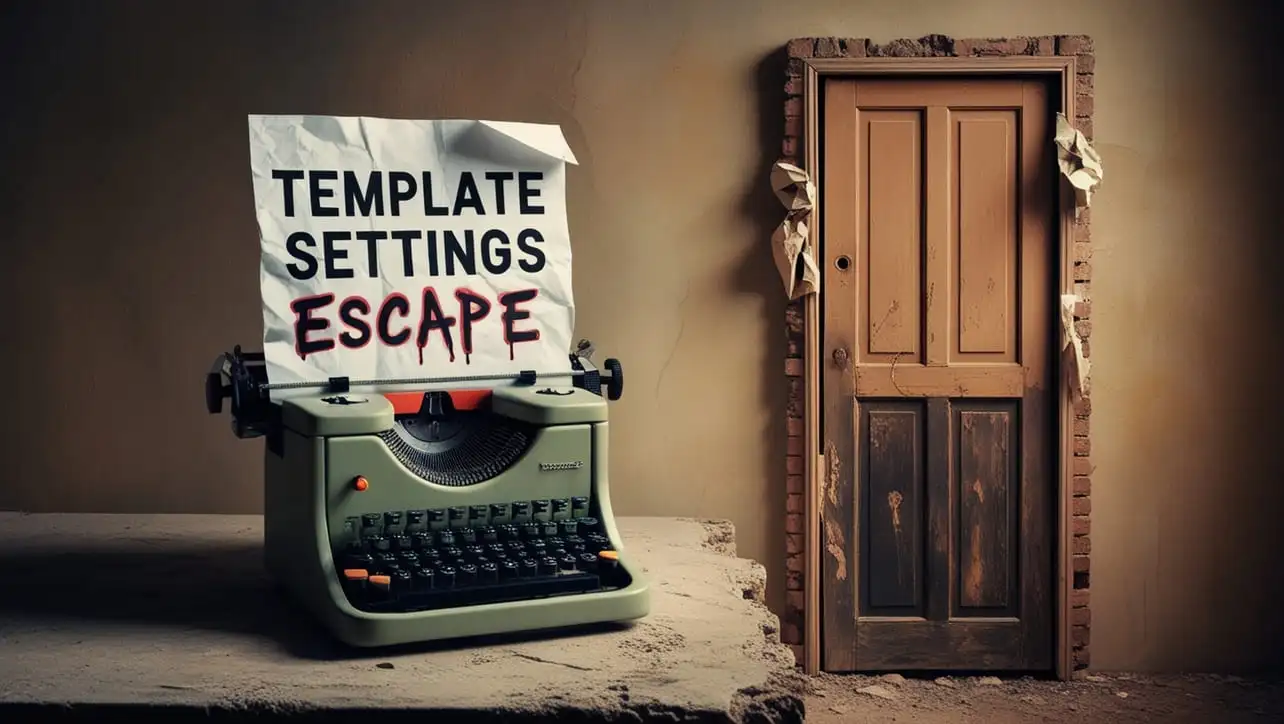
Lodash _.templateSettings.escape Property
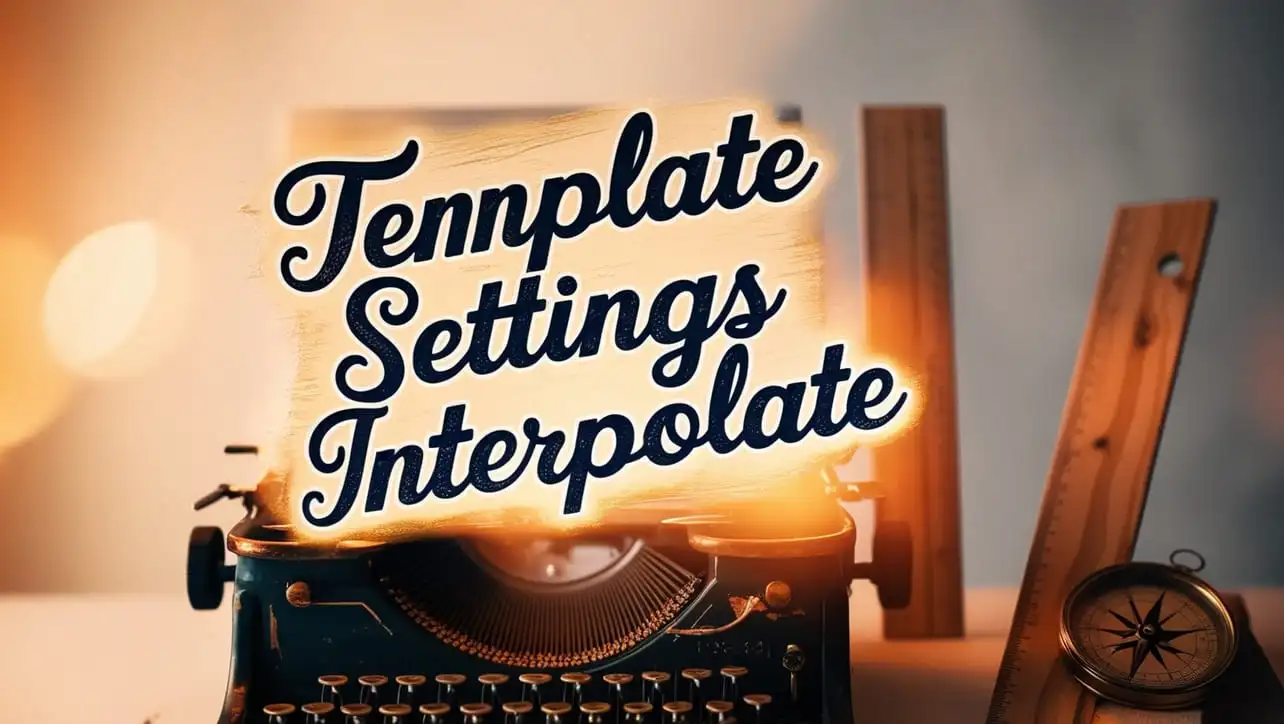
Lodash _.templateSettings.interpolate Property
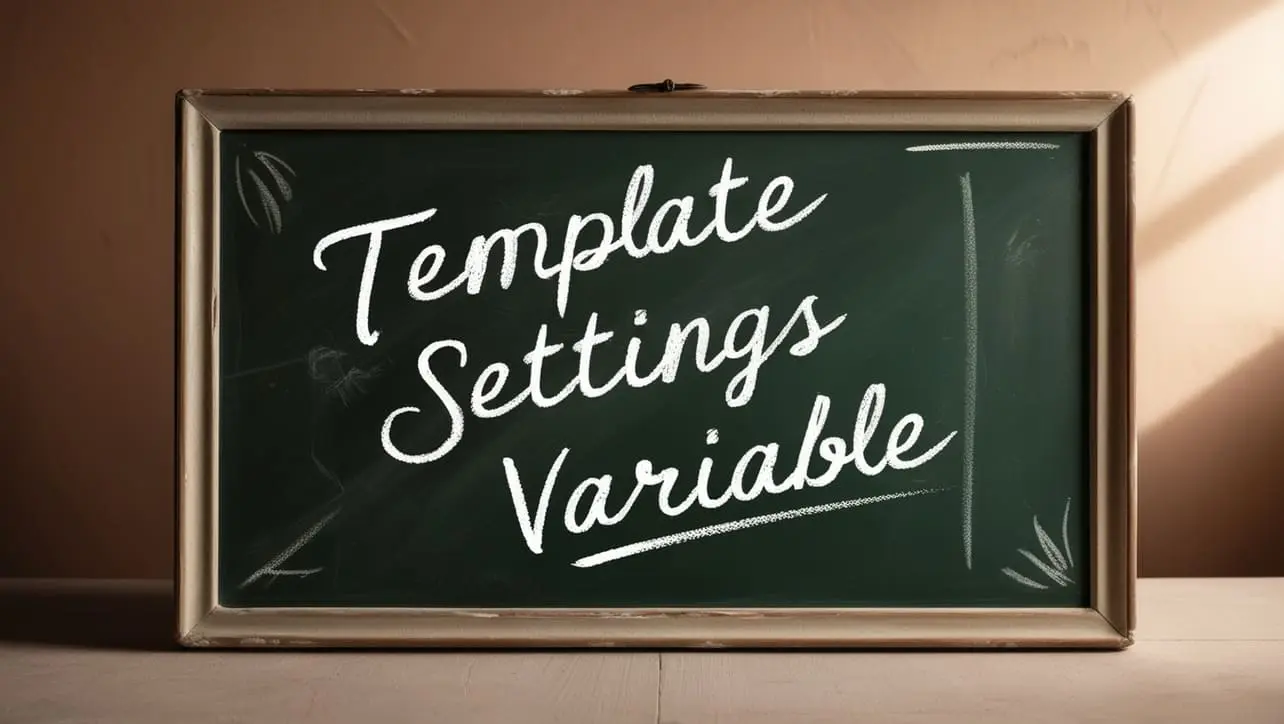
If you have any doubts regarding this article (Lodash _.takeRight() Array Method), please comment here. I will help you immediately.