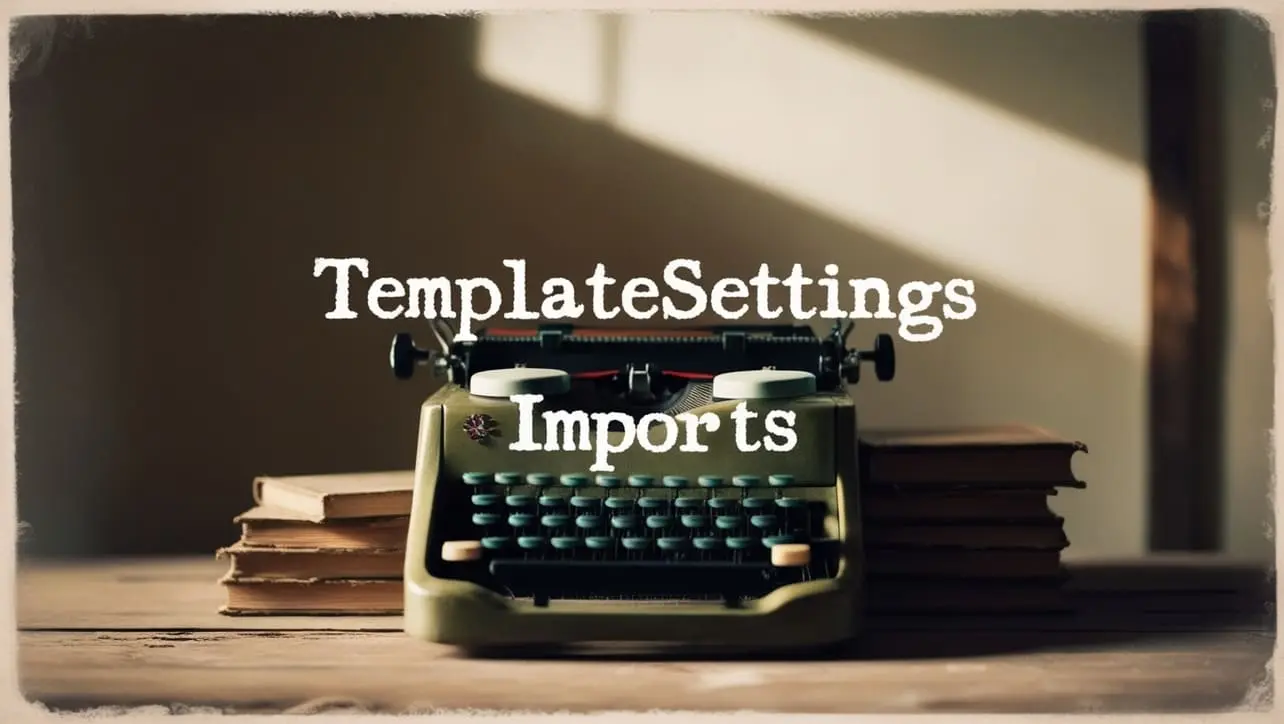
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.take() Array Method
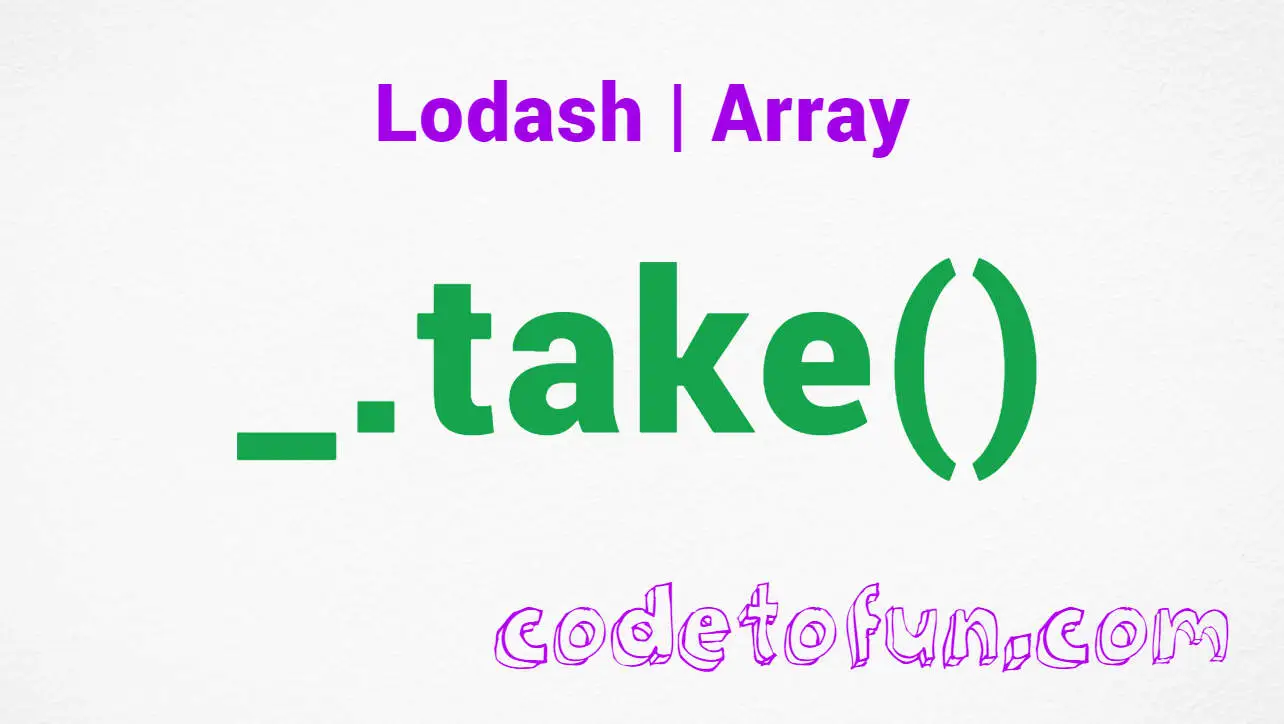
Photo Credit to CodeToFun
🙋 Introduction
Efficiently working with arrays is a fundamental aspect of JavaScript development. Lodash, a comprehensive utility library, provides an arsenal of functions to streamline array manipulation. Among these functions, the _.take()
method stands out as a versatile tool for extracting a specified number of elements from the beginning of an array.
This method is invaluable when dealing with paginated data, implementing previews, or simply selecting a subset of elements.
🧠 Understanding _.take()
The _.take()
method in Lodash allows you to create a new array containing the first N elements of an existing array, where N is the specified number. This method is particularly useful when you need to display or process a limited portion of an array, enhancing performance and user experience.
💡 Syntax
_.take(array, [n=1])
- array: The array to take elements from.
- n (Optional): The number of elements to take from the beginning of the array (default is 1).
📝 Example
Let's dive into a practical example to understand the functionality of _.take()
:
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const takenElements = _.take(originalArray, 3);
console.log(takenElements);
// Output: [1, 2, 3]
In this example, the originalArray is processed by _.take()
, resulting in a new array containing the first 3 elements.
🏆 Best Practices
Specify the Number of Elements:
Always specify the number of elements you want to take using the n parameter. This ensures clarity in your code and avoids unexpected behavior.
example.jsCopiedconst dataToDisplay = /* ...fetch data from API or elsewhere... */; const numberOfElementsToDisplay = 5; const displayedData = _.take(dataToDisplay, numberOfElementsToDisplay); console.log(displayedData);
Handle Edge Cases:
Consider scenarios where the array might be empty or when you need to take more elements than the array contains. Implement proper error handling or default behaviors to address these edge cases.
example.jsCopiedconst emptyArray = []; const moreThanArrayLength = 10; const result1 = _.take(emptyArray); // Returns: [] const result2 = _.take(originalArray, moreThanArrayLength); // Returns: [1, 2, 3, 4, 5, 6, 7, 8, 9] console.log(result1); console.log(result2);
Combine with Other Methods:
Leverage the versatility of Lodash by combining
_.take()
with other array methods to create powerful workflows.example.jsCopiedconst combinedResult = _.take(_.sortBy(originalArray), 2); console.log(combinedResult); // Output: [1, 2]
📚 Use Cases
Pagination:
When implementing paginated displays,
_.take()
can be used to extract a specific number of elements for each page, providing a seamless navigation experience.example.jsCopiedconst allData = /* ...fetch all data from API or elsewhere... */; const pageSize = 10; const currentPage = 2; const paginatedData = _.take(allData, pageSize * currentPage); console.log(paginatedData);
Preview Functionality:
In scenarios where you want to provide a preview of content, such as in a blog or news feed,
_.take()
can help you extract a limited number of elements for display.example.jsCopiedconst articleContent = /* ...fetch article content from API or elsewhere... */; const previewLength = 3; const articlePreview = _.take(articleContent, previewLength); console.log(articlePreview);
Selecting Subset for Processing:
When dealing with large datasets and needing to process only a portion of the data,
_.take()
can be combined with other methods to efficiently handle the required subset.example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; const subsetForProcessing = _.take(_.filter(largeDataset, item => item.isActive), 5); console.log(subsetForProcessing);
🎉 Conclusion
The _.take()
method in Lodash is a valuable asset for JavaScript developers working with arrays. Its simplicity and flexibility make it an excellent choice for scenarios where you need to extract a specific number of elements from the beginning of an array. By incorporating this method into your code, you can enhance both the efficiency and readability of your projects.
Explore the world of Lodash and unlock the potential of array manipulation with _.take()
!
👨💻 Join our Community:
Author
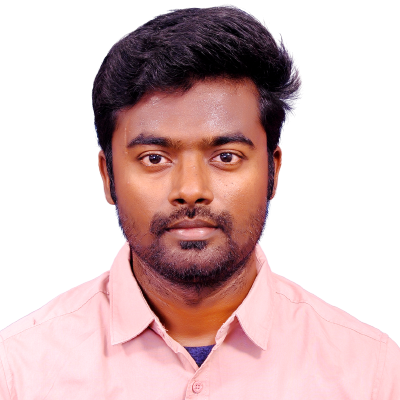
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
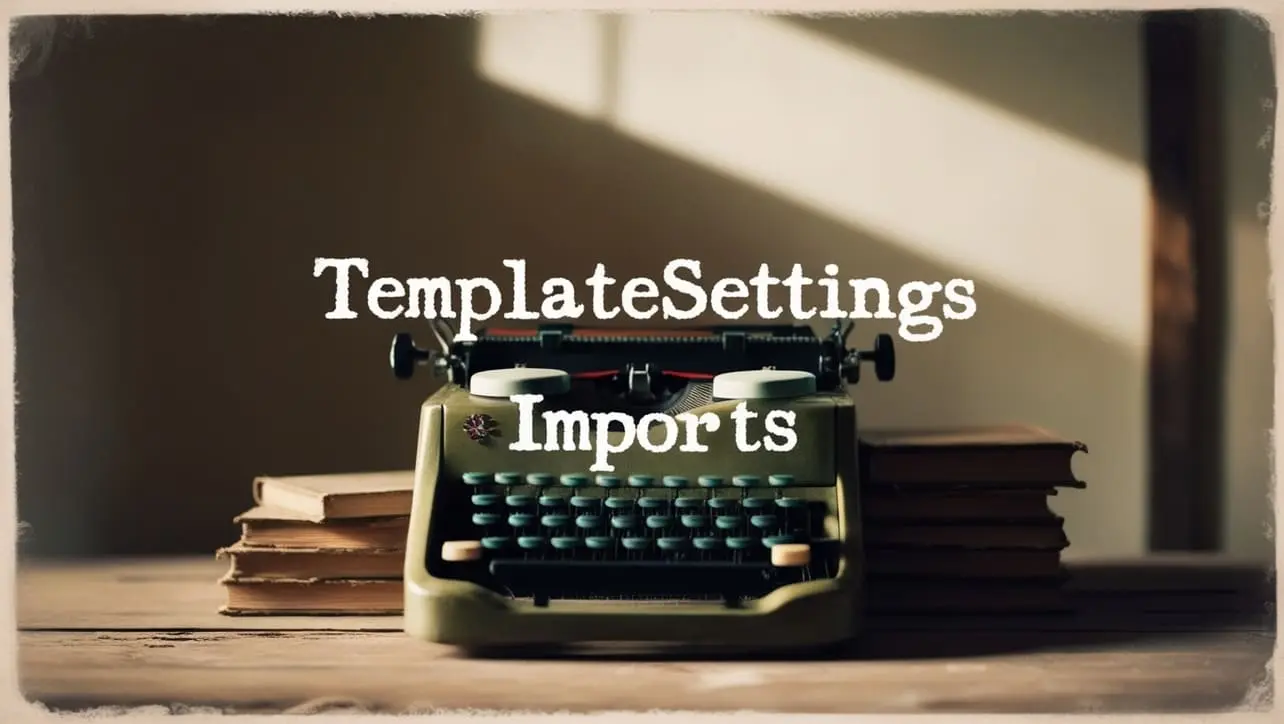
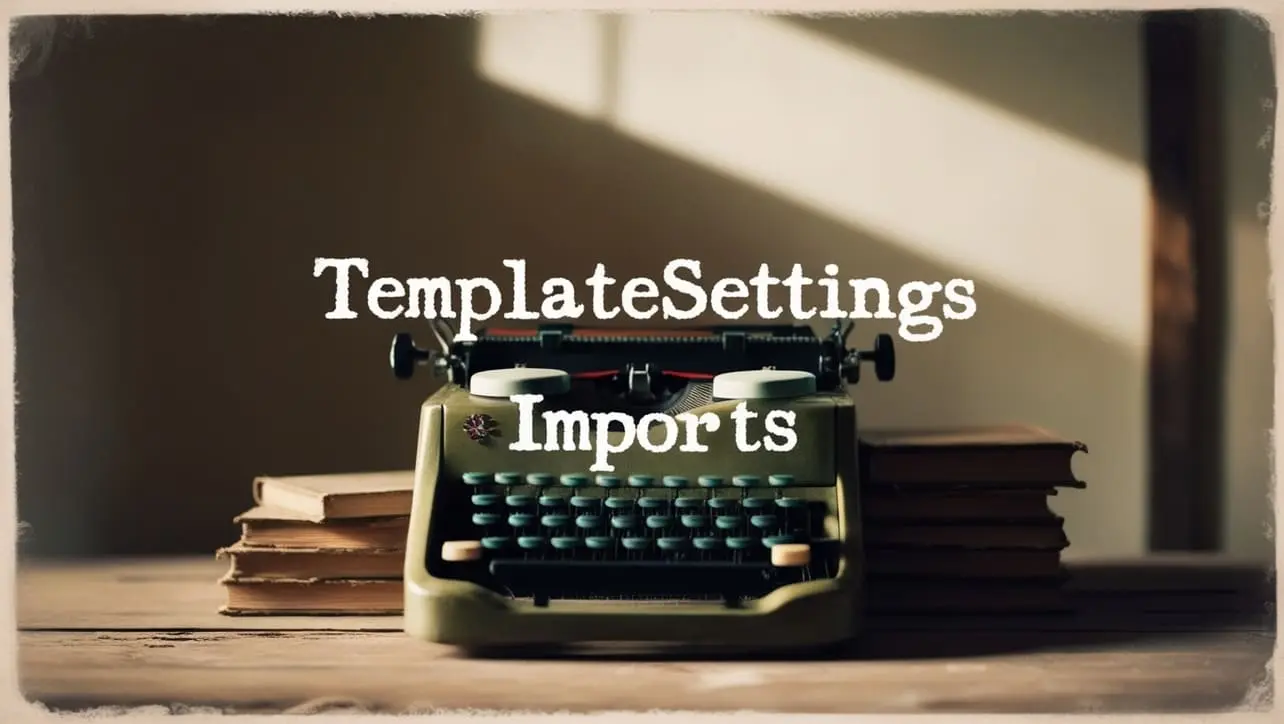
Lodash _.templateSettings.imports Property
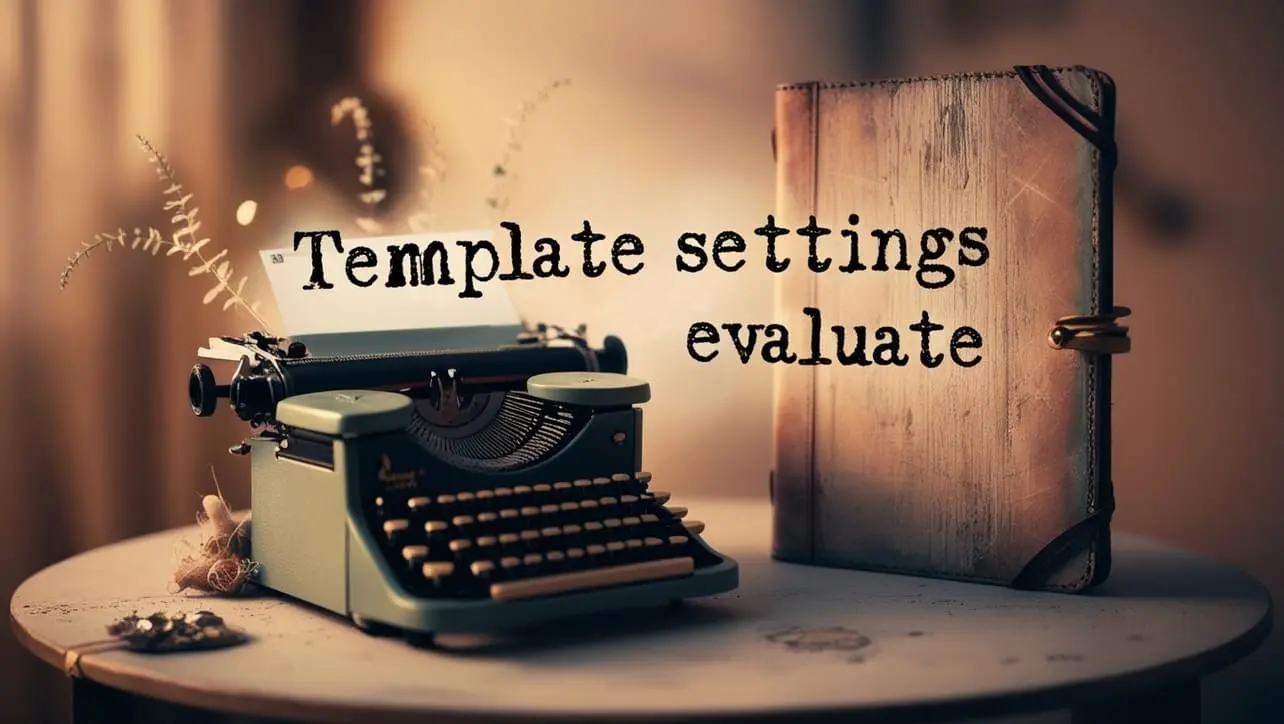
Lodash _.templateSettings.evaluate Property
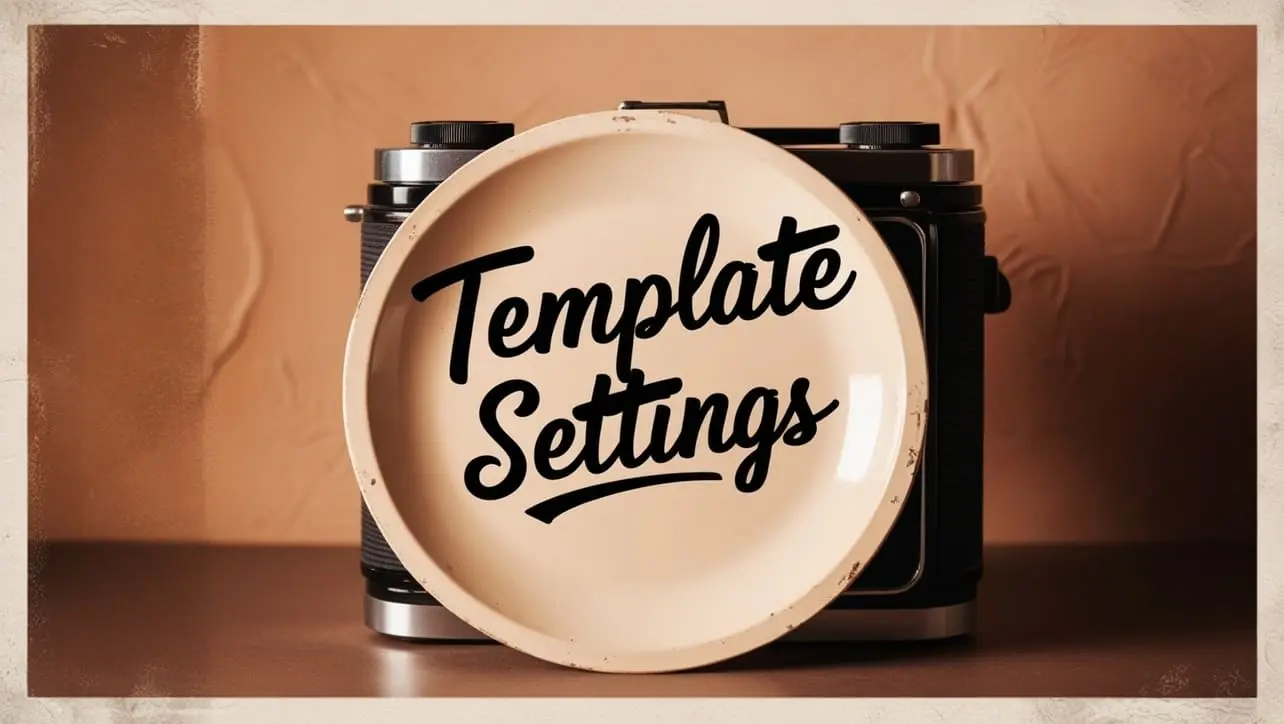
Lodash _.templateSettings Property
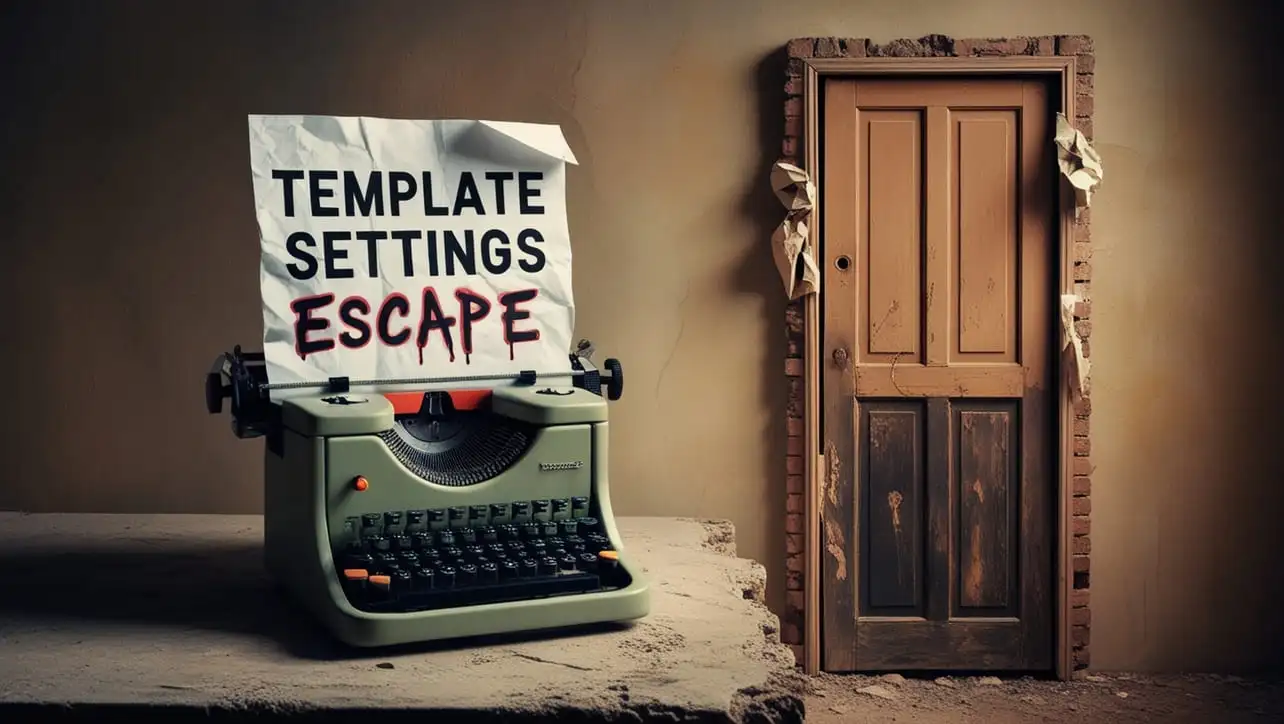
Lodash _.templateSettings.escape Property
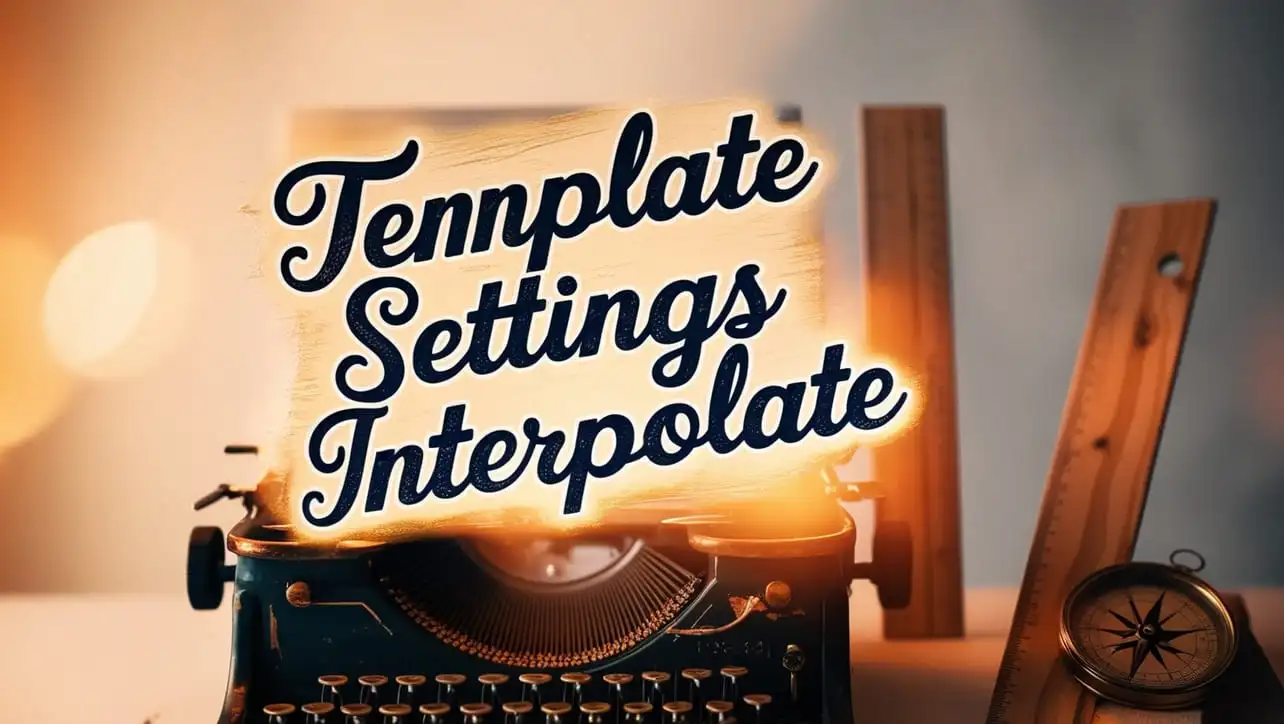
Lodash _.templateSettings.interpolate Property
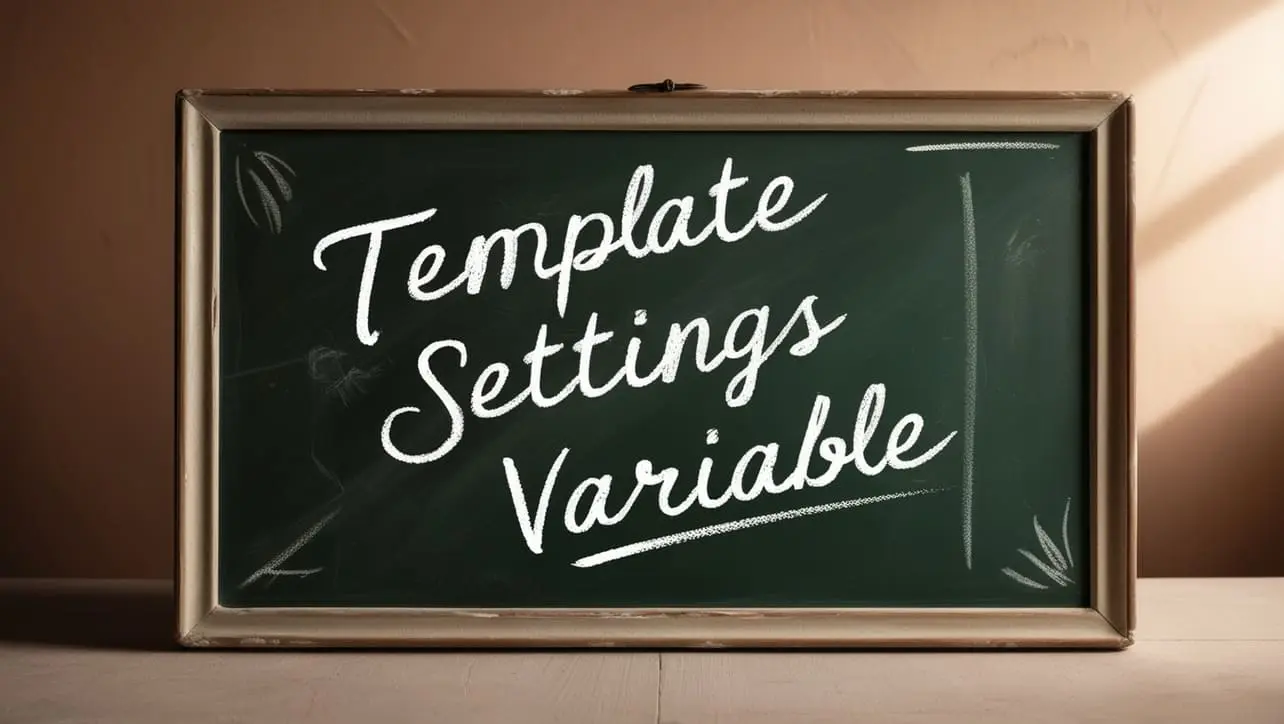
If you have any doubts regarding this article (Lodash _.take() Array Method), please comment here. I will help you immediately.