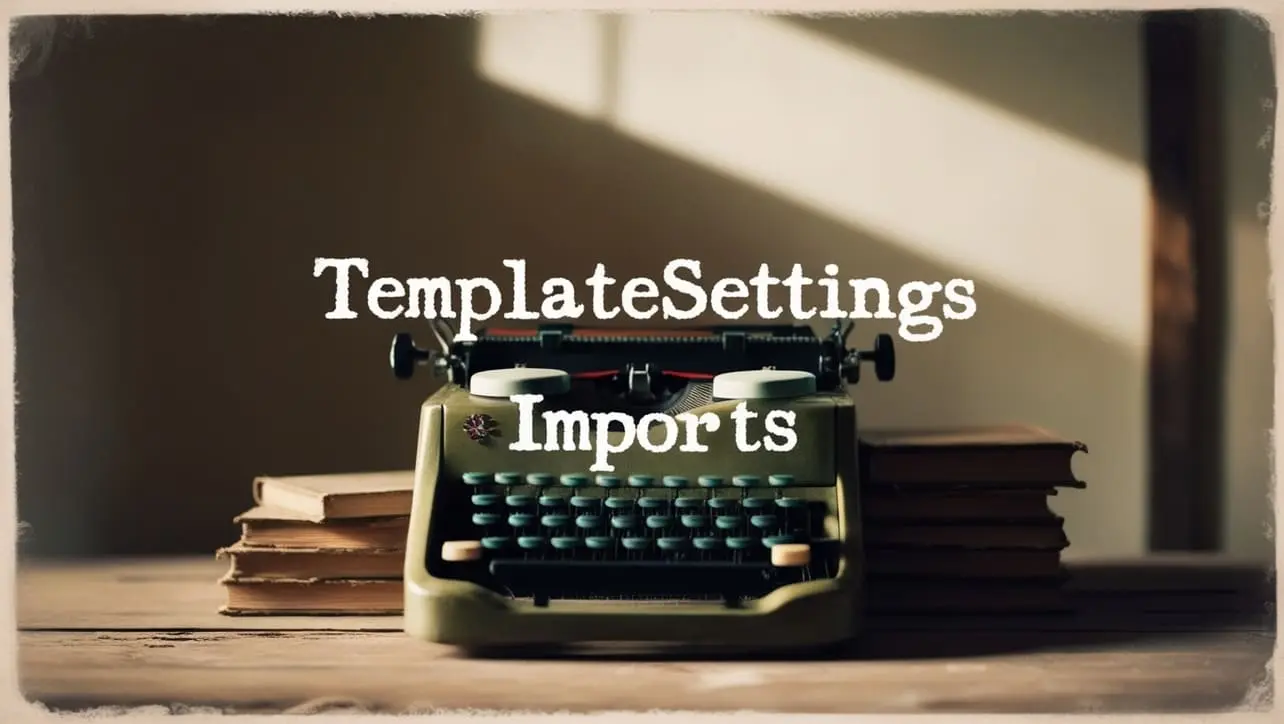
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedLastIndexBy() Array Method
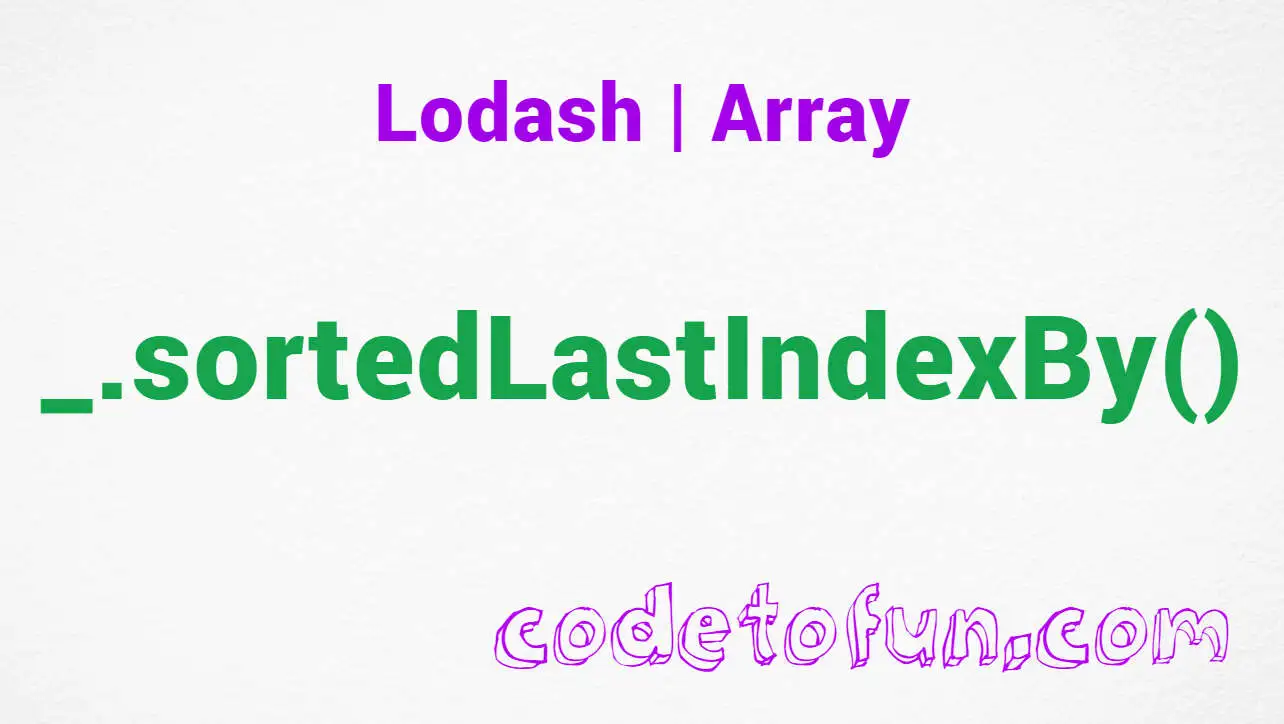
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, efficient array manipulation is often key to optimizing algorithms and improving overall performance. The Lodash library comes to the rescue with its rich set of utility functions, among which the _.sortedLastIndexBy()
method stands out.
This method empowers developers to find the highest index at which an element should be inserted into a sorted array while considering a specific criterion. It's a valuable tool for anyone dealing with sorted datasets or seeking to enhance the efficiency of their code.
🧠 Understanding _.sortedLastIndexBy()
The _.sortedLastIndexBy()
method in Lodash provides a way to find the highest index at which an element should be inserted into a sorted array based on the result of a provided iteratee function. This can be particularly useful when working with sorted data and wanting to maintain the order.
💡 Syntax
_.sortedLastIndexBy(array, value, [iteratee=_.identity])
- array: The sorted array to inspect.
- value: The value to evaluate.
- iteratee: The iteratee invoked per element (default is _.identity).
📝 Example
Let's dive into a practical example to illustrate the power of _.sortedLastIndexBy()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sortedArray = [{ 'x': 4 }, { 'x': 5 }, { 'x': 6 }];
const valueToInsert = { 'x': 5 };
const lastIndex = _.sortedLastIndexBy(sortedArray, valueToInsert, 'x');
console.log(lastIndex);
// Output: 3
In this example, the sortedArray contains objects with a key 'x'. The _.sortedLastIndexBy()
method is used to find the highest index at which the valueToInsert should be placed while considering the 'x' property.
🏆 Best Practices
Understand the Sorted Array:
Ensure that your array is sorted before using
_.sortedLastIndexBy()
. The method assumes a sorted array, and incorrect input might lead to unexpected results.example.jsCopiedconst unsortedArray = [3, 1, 4, 1, 5, 9, 2]; const valueToInsert = 6; // Incorrect usage with an unsorted array const incorrectIndex = _.sortedLastIndexBy(unsortedArray, valueToInsert); console.log(incorrectIndex); // Output: Unpredictable result due to unsorted array
Choose Appropriate Iteratee:
Select the iteratee function carefully based on the structure of your objects within the array. It should extract the property or value used for comparison.
example.jsCopiedconst arrayOfObjects = [{ 'name': 'Alice' }, { 'name': 'Bob' }, { 'name': 'Charlie' }]; const objectToInsert = { 'name': 'David' }; // Incorrect usage with an incorrect iteratee const incorrectIndex = _.sortedLastIndexBy(arrayOfObjects, objectToInsert, 'age'); console.log(incorrectIndex); // Output: Unpredictable result due to incorrect iteratee
Handle Edge Cases:
Consider edge cases, such as an empty array or an array with only one element. Implement appropriate error handling or default behaviors to address these scenarios.
example.jsCopiedconst emptyArray = []; const valueToInsert = 42; // Handle empty array const emptyArrayIndex = _.sortedLastIndexBy(emptyArray, valueToInsert); console.log(emptyArrayIndex); // Output: 0 (default insertion point for an empty array)
📚 Use Cases
Insertion in Sorted Lists:
When maintaining a sorted list of data,
_.sortedLastIndexBy()
is handy for determining the correct position to insert a new element while preserving the order.example.jsCopiedconst sortedListOfDates = ['2022-01-01', '2022-02-01', '2022-03-01']; const dateToInsert = '2022-02-15'; const insertionIndex = _.sortedLastIndexBy(sortedListOfDates, dateToInsert, Date.parse); console.log(insertionIndex);
Object Property Sorting:
In scenarios where objects within an array have a specific property used for sorting,
_.sortedLastIndexBy()
simplifies finding the correct insertion point.example.jsCopiedconst arrayOfObjects = [{ 'name': 'Alice' }, { 'name': 'Bob' }, { 'name': 'Charlie' }]; const objectToInsert = { 'name': 'David' }; const insertionIndexByName = _.sortedLastIndexBy(arrayOfObjects, objectToInsert, 'name'); console.log(insertionIndexByName);
🎉 Conclusion
The _.sortedLastIndexBy()
method in Lodash is a powerful tool for efficiently handling sorted arrays in JavaScript. Its ability to find the highest index for insertion based on a specific criterion makes it invaluable for maintaining order and optimizing code. By incorporating this method into your projects, you can streamline your array manipulation tasks and improve overall performance.
Explore the capabilities of Lodash and unlock the potential of array manipulation with _.sortedLastIndexBy()
!
👨💻 Join our Community:
Author
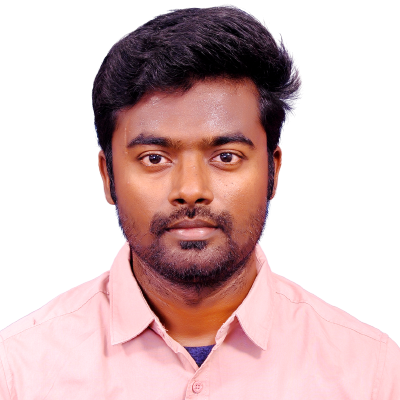
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
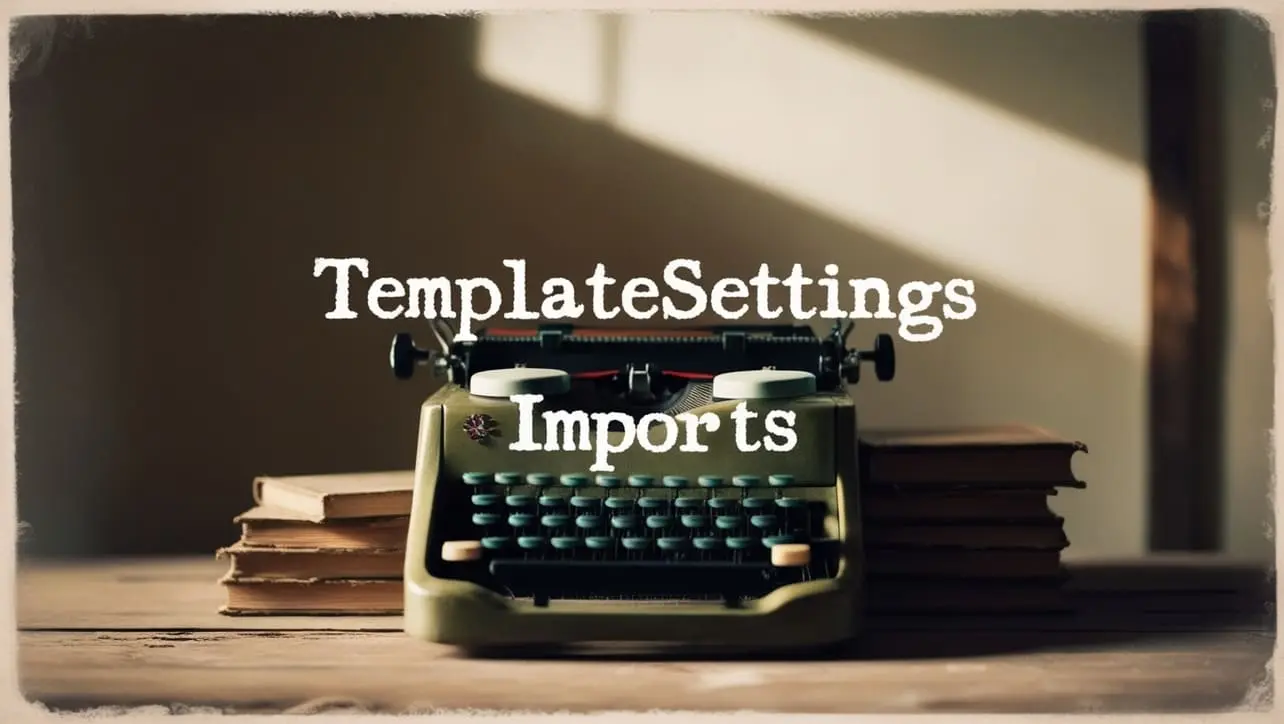
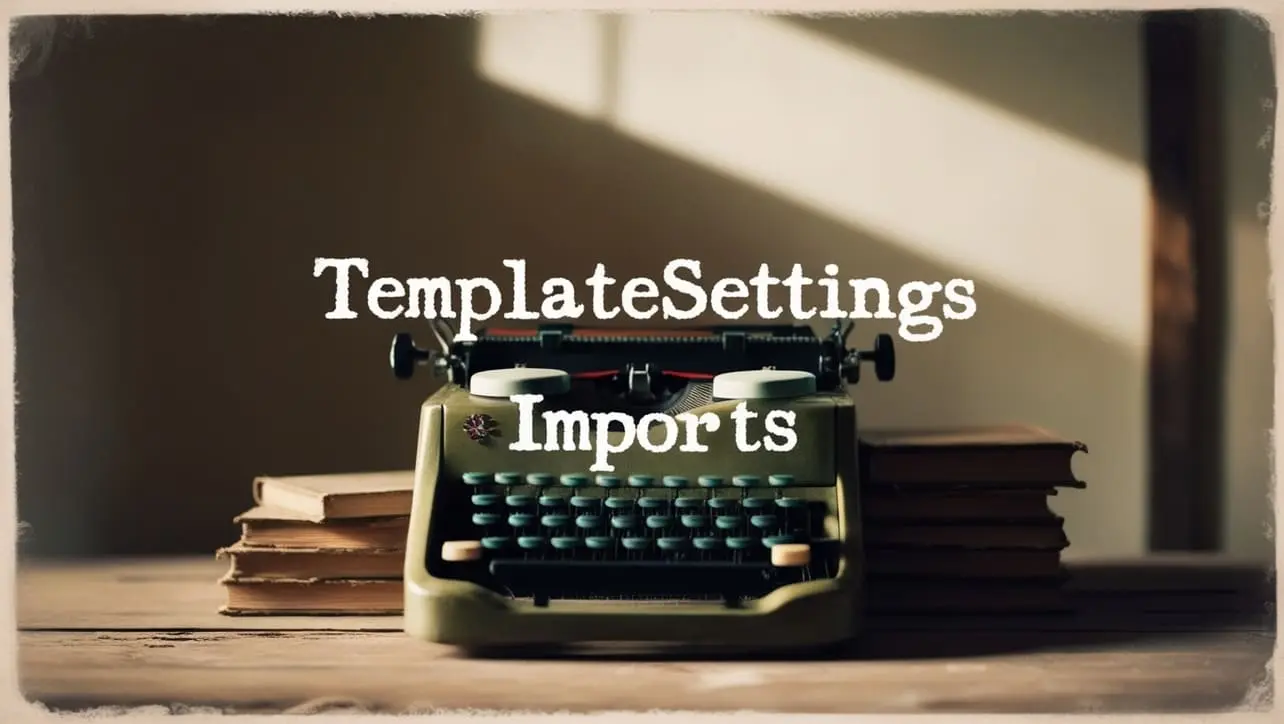
Lodash _.templateSettings.imports Property
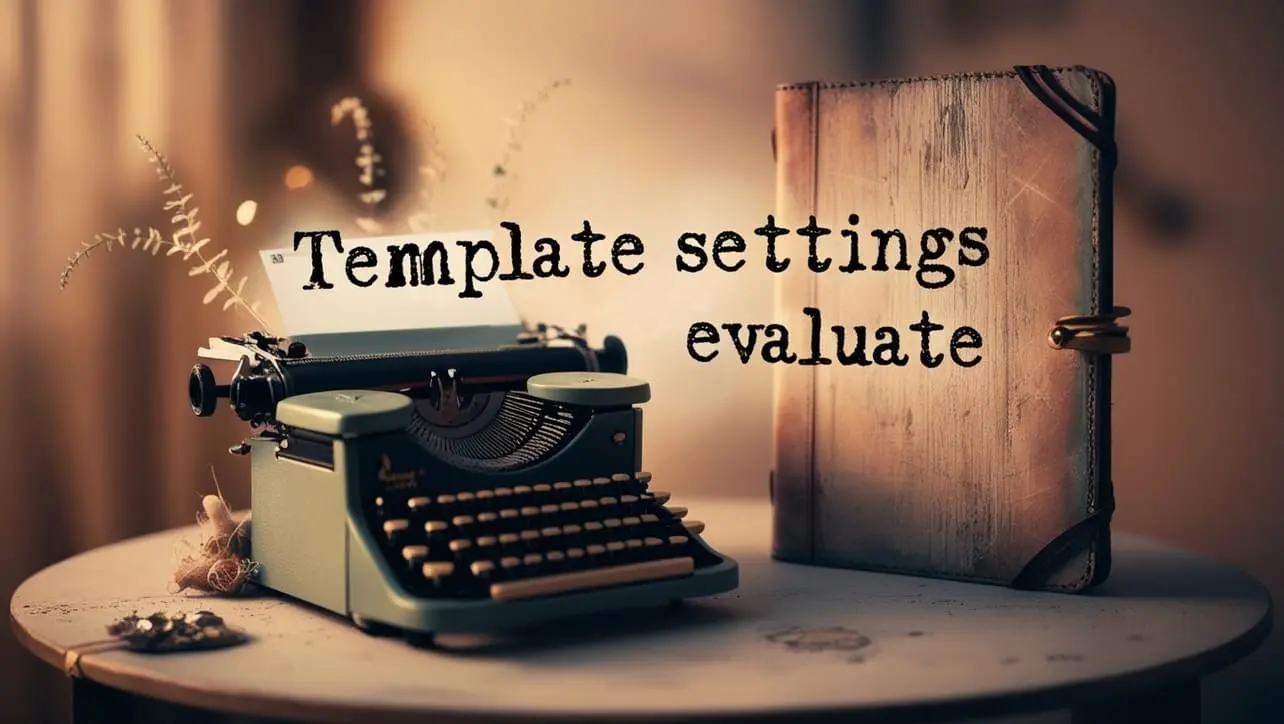
Lodash _.templateSettings.evaluate Property
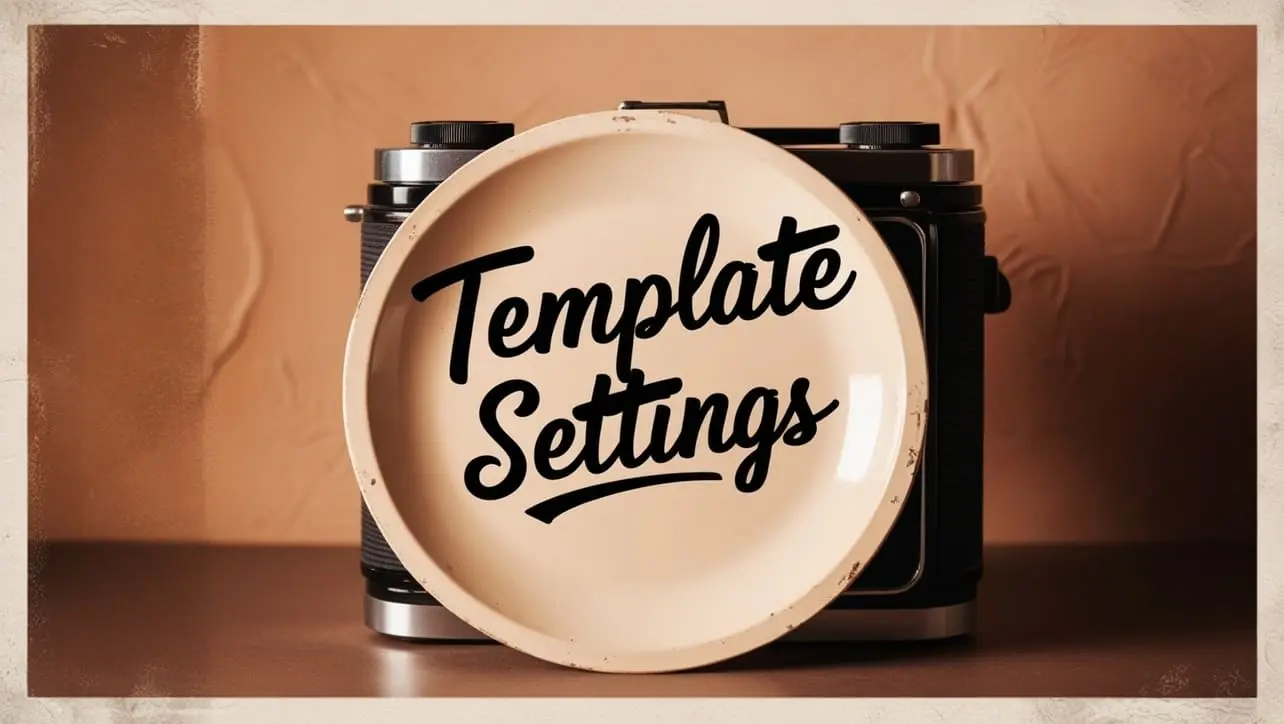
Lodash _.templateSettings Property
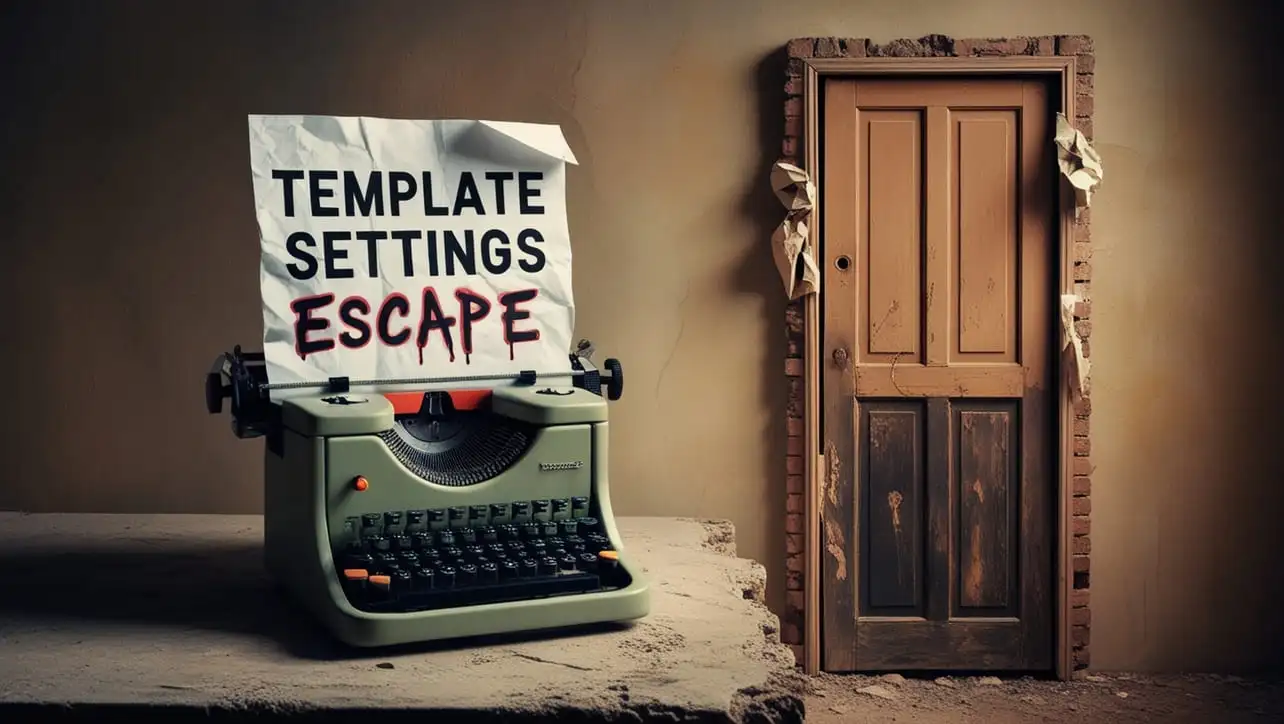
Lodash _.templateSettings.escape Property
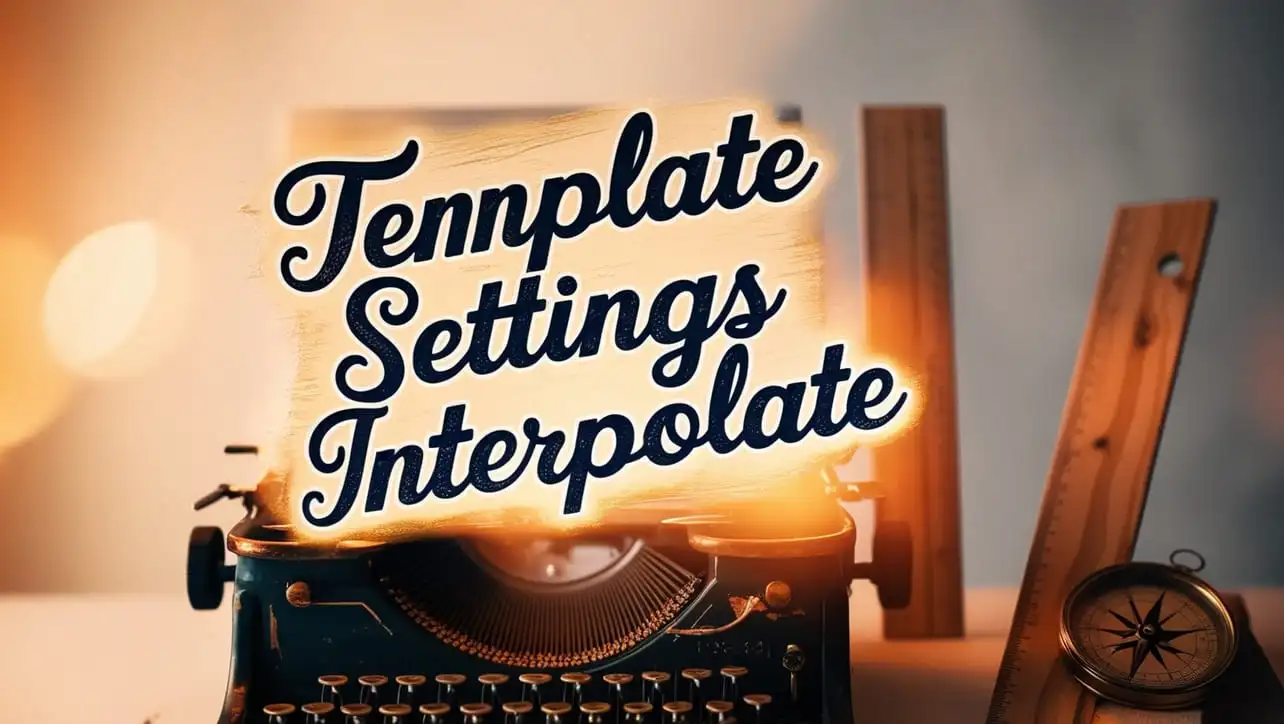
Lodash _.templateSettings.interpolate Property
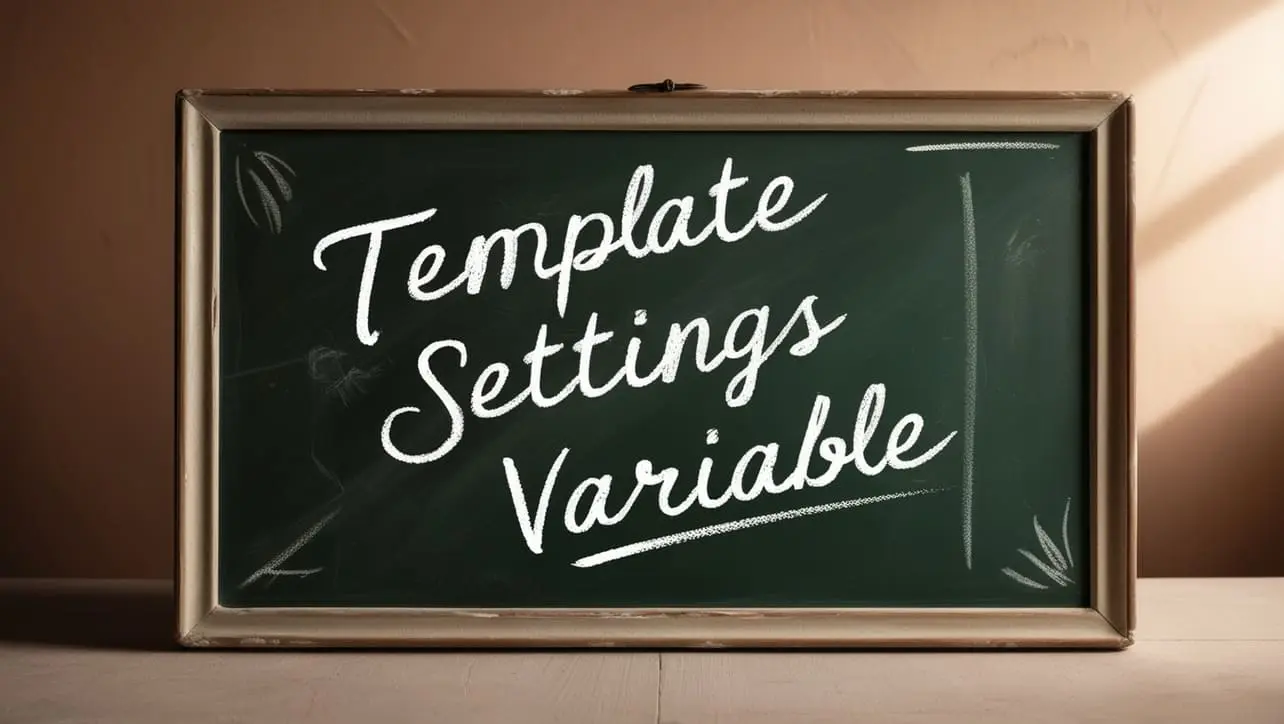
If you have any doubts regarding this article (Lodash _.sortedLastIndexBy() Array Method), please comment here. I will help you immediately.