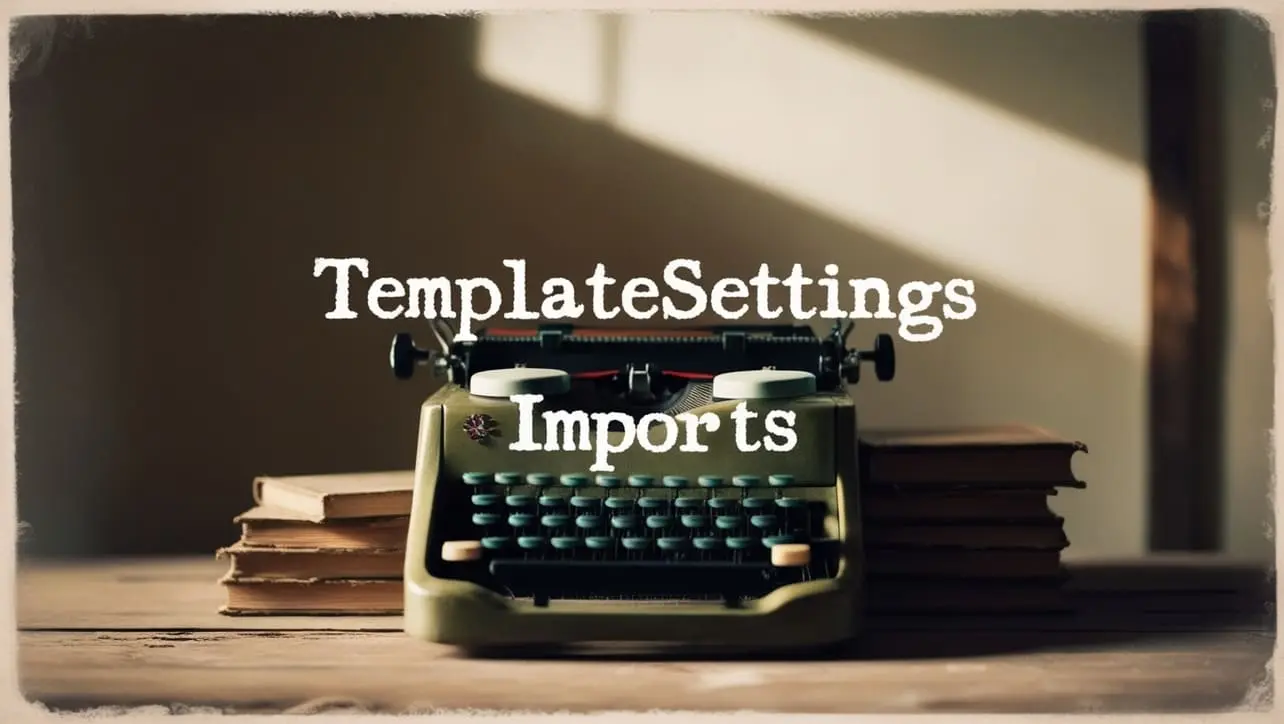
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedIndexBy() Array Method
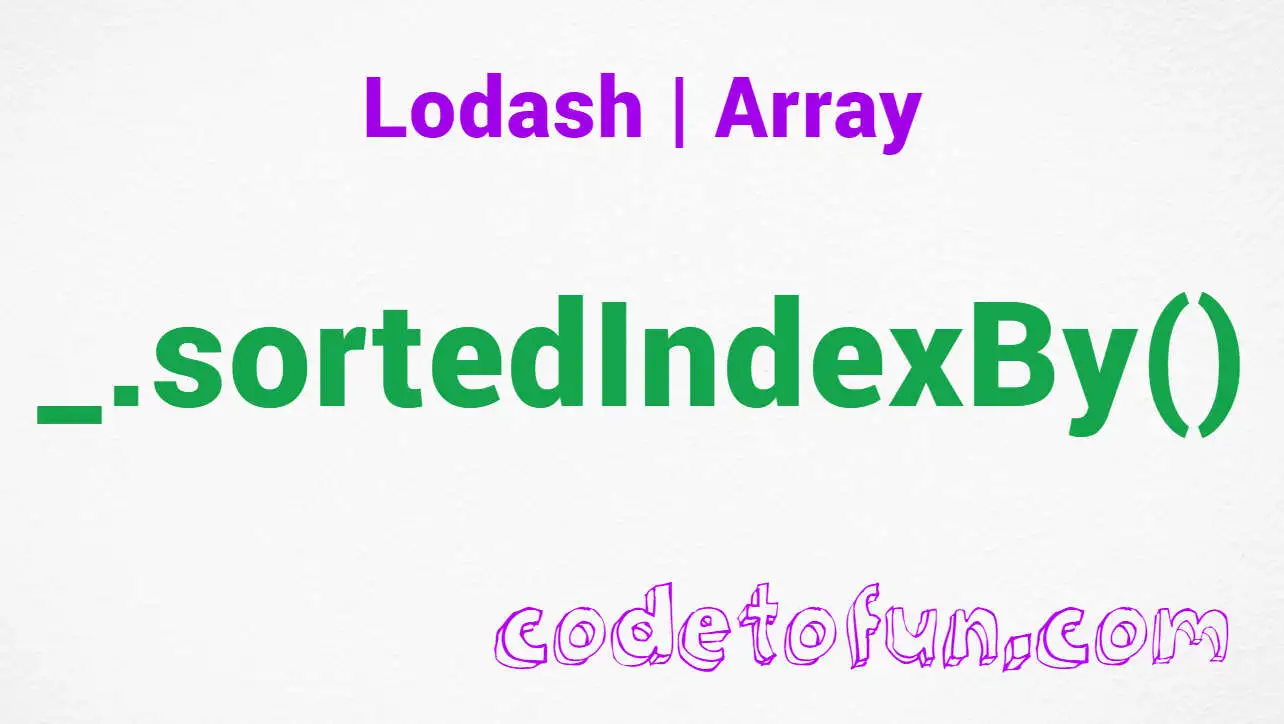
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, efficient array manipulation is a common requirement.
The Lodash library comes to the rescue with a rich set of utility functions, and one such powerful tool is the _.sortedIndexBy()
method. This method provides a convenient way to find the index at which an element should be inserted into a sorted array, based on a specified iteratee function.
🧠 Understanding _.sortedIndexBy()
The _.sortedIndexBy()
method in Lodash is designed for situations where you have a sorted array and want to determine the position where a new element should be inserted while maintaining the array's order.
💡 Syntax
_.sortedIndexBy(array, value, [iteratee=_.identity])
- array: The sorted array to inspect.
- value: The value to evaluate.
- iteratee: The iteratee invoked for each element. The result of this function will be used for the comparison.
📝 Example
Let's delve into a practical example to grasp the functionality of _.sortedIndexBy()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sortedArray = [{ x: 10 }, { x: 20 }, { x: 30 }];
const newValue = { x: 25 };
const insertionIndex = _.sortedIndexBy(sortedArray, newValue, 'x');
console.log(insertionIndex);
// Output: 2
In this example, newValue is an object with a property x, and the sortedArray is an array of objects sorted based on their x property. The _.sortedIndexBy()
method is used to find the index at which newValue should be inserted to maintain the sorted order.
🏆 Best Practices
Understand the Sorted Array:
Ensure that the input array is sorted before using
_.sortedIndexBy()
. If the array is not sorted, the result may be unpredictable.example.jsCopiedconst unsortedArray = [3, 1, 4, 1, 5, 9, 2, 6, 5]; // Sorting the array const sortedArray = _.sortBy(unsortedArray); const insertionIndex = _.sortedIndexBy(sortedArray, 7); console.log(insertionIndex); // Output: 7
Choose an Appropriate Iteratee:
Select an iteratee function that correctly extracts the comparison value from each element. This is crucial for accurate positioning within the sorted array.
example.jsCopiedconst arrayOfObjects = [{ date: '2022-01-01' }, { date: '2022-02-01' }, { date: '2022-03-01' }]; const newObject = { date: '2022-02-15' }; const insertionIndex = _.sortedIndexBy(arrayOfObjects, newObject, obj => new Date(obj.date)); console.log(insertionIndex); // Output: 2
📚 Use Cases
Maintaining Sorted Lists:
Use
_.sortedIndexBy()
when working with lists that need to be kept in a sorted order, such as maintaining an alphabetical or numerical ordering.example.jsCopiedconst alphabeticalList = ['apple', 'banana', 'orange', 'strawberry']; const newFruit = 'kiwi'; const insertionIndex = _.sortedIndexBy(alphabeticalList, newFruit); console.log(insertionIndex); // Output: 2
Efficient Searching in Sorted Data:
In scenarios where you have a large dataset sorted based on a specific property,
_.sortedIndexBy()
helps efficiently find the position for new elements.example.jsCopiedconst sortedData = /* ...fetch data from API or elsewhere... */; const newData = /* ...create or receive new data... */; const insertionIndex = _.sortedIndexBy(sortedData, newData, 'timestamp'); console.log(insertionIndex);
🎉 Conclusion
The _.sortedIndexBy()
method in Lodash is a valuable asset for JavaScript developers dealing with sorted arrays. Whether you are maintaining ordered lists or efficiently searching in large datasets, this method offers a clean and reliable solution.
Unlock the potential of array manipulation with _.sortedIndexBy()
and explore the myriad possibilities it opens up for your JavaScript projects!
👨💻 Join our Community:
Author
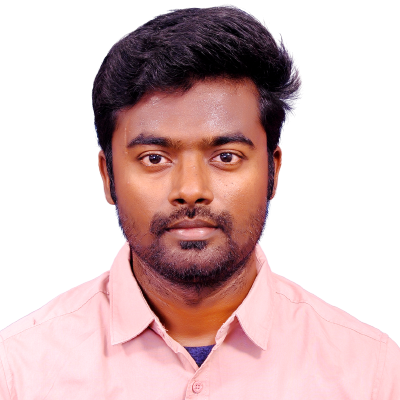
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
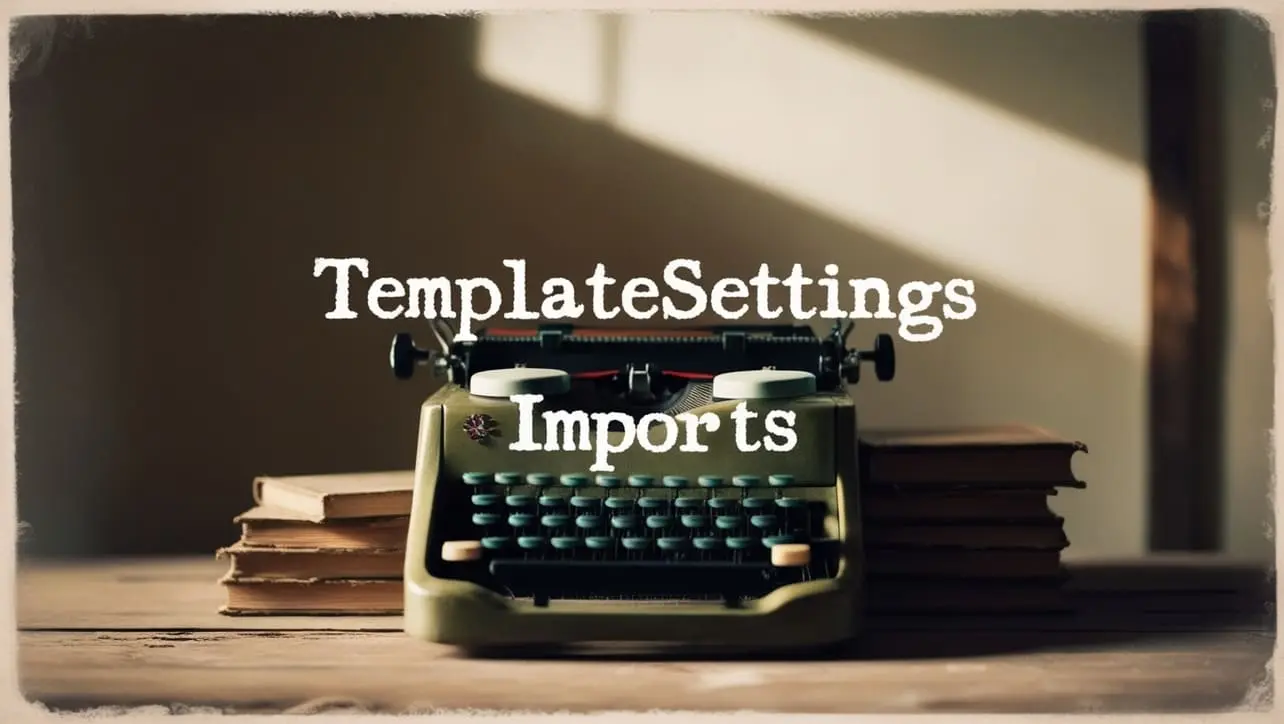
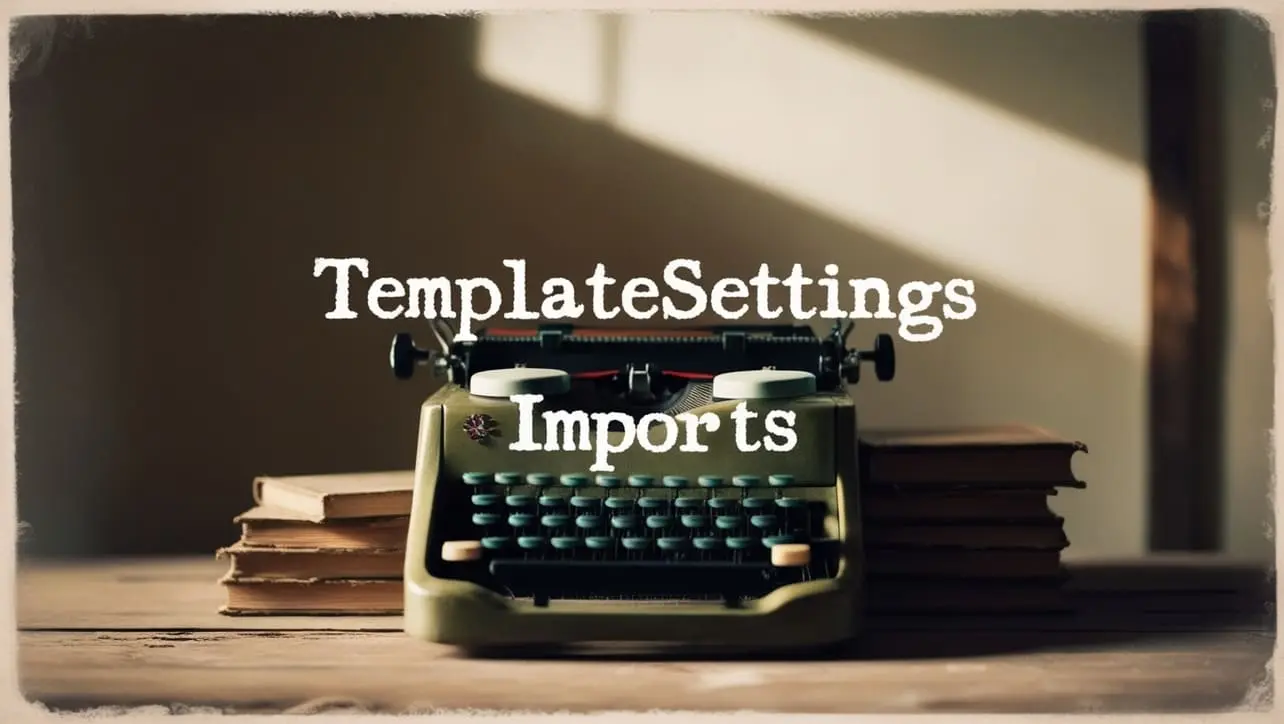
Lodash _.templateSettings.imports Property
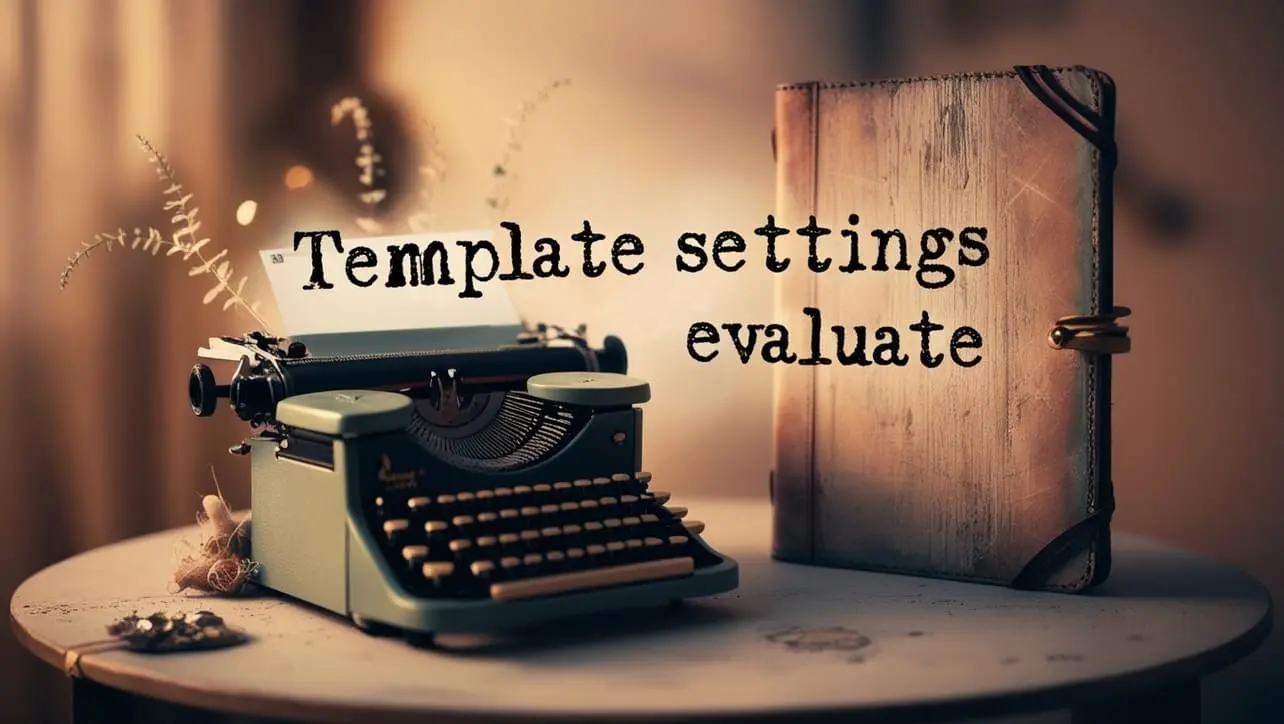
Lodash _.templateSettings.evaluate Property
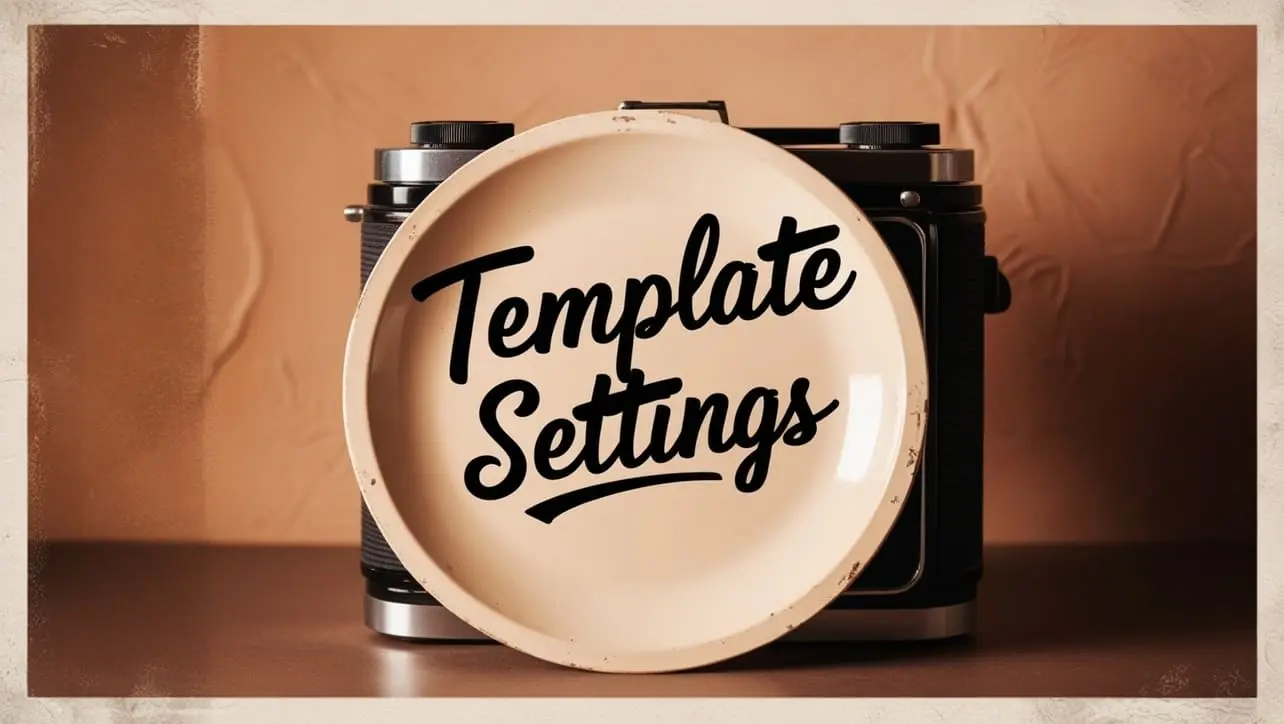
Lodash _.templateSettings Property
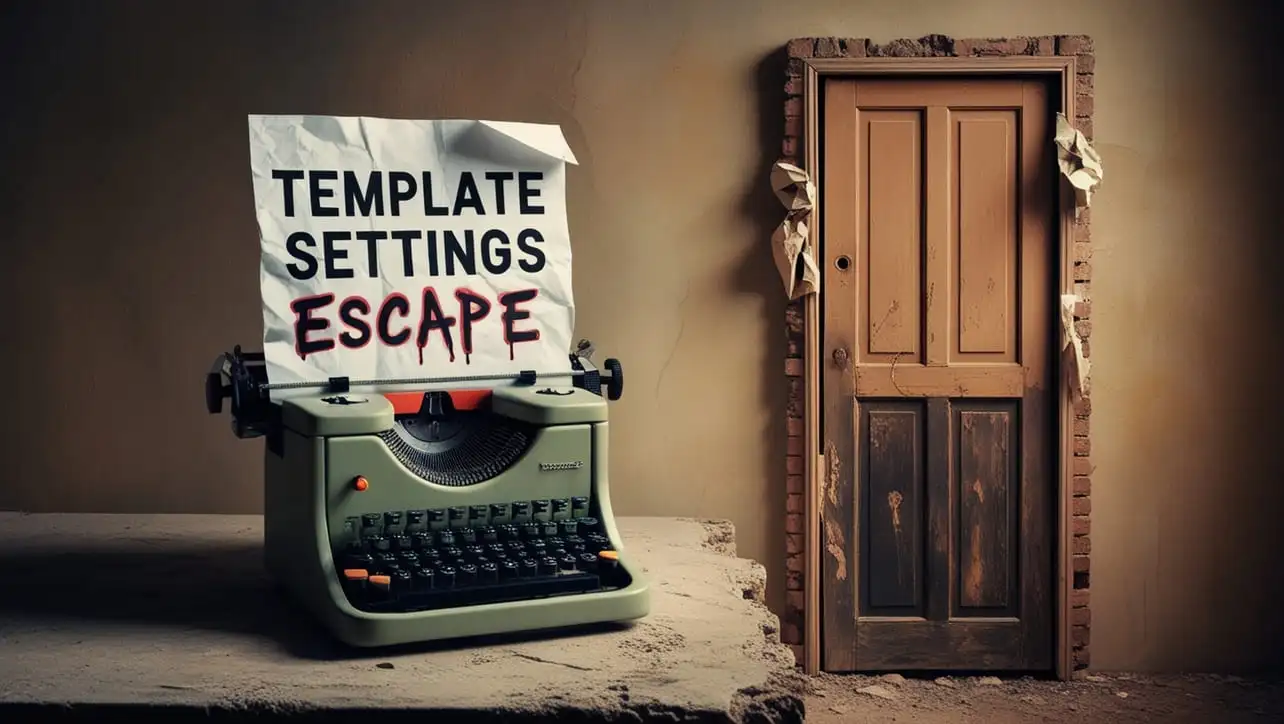
Lodash _.templateSettings.escape Property
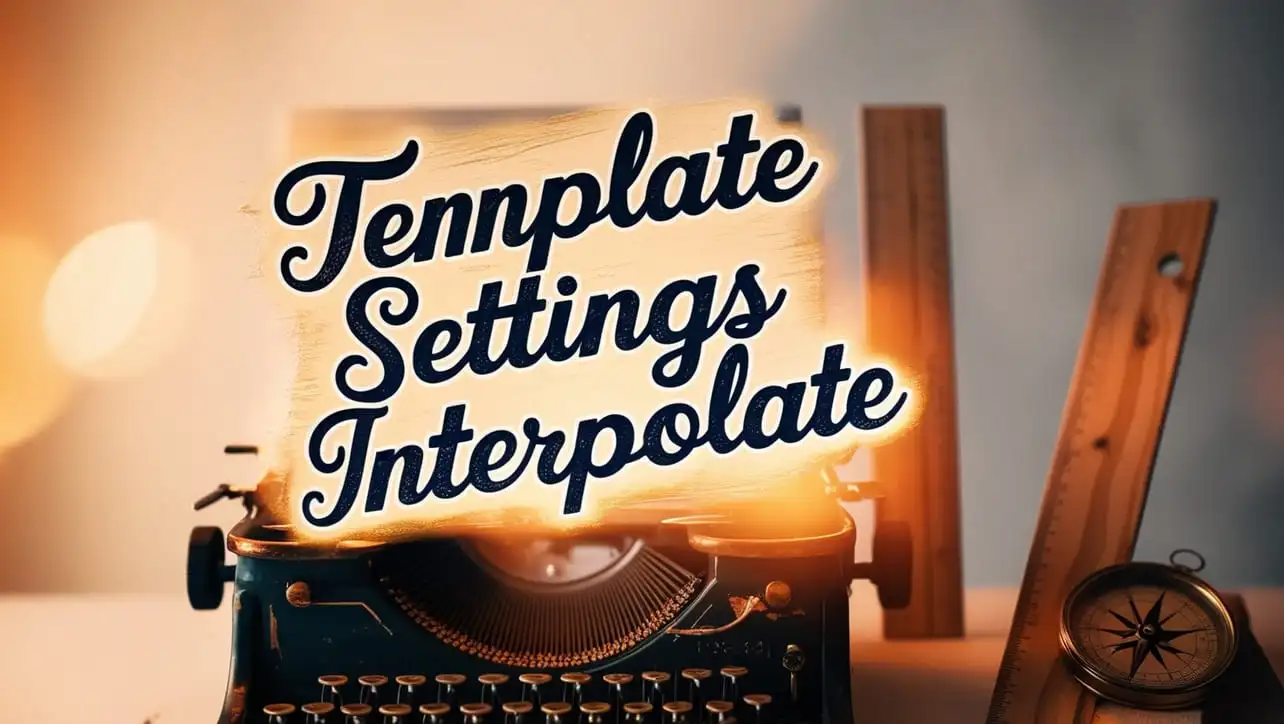
Lodash _.templateSettings.interpolate Property
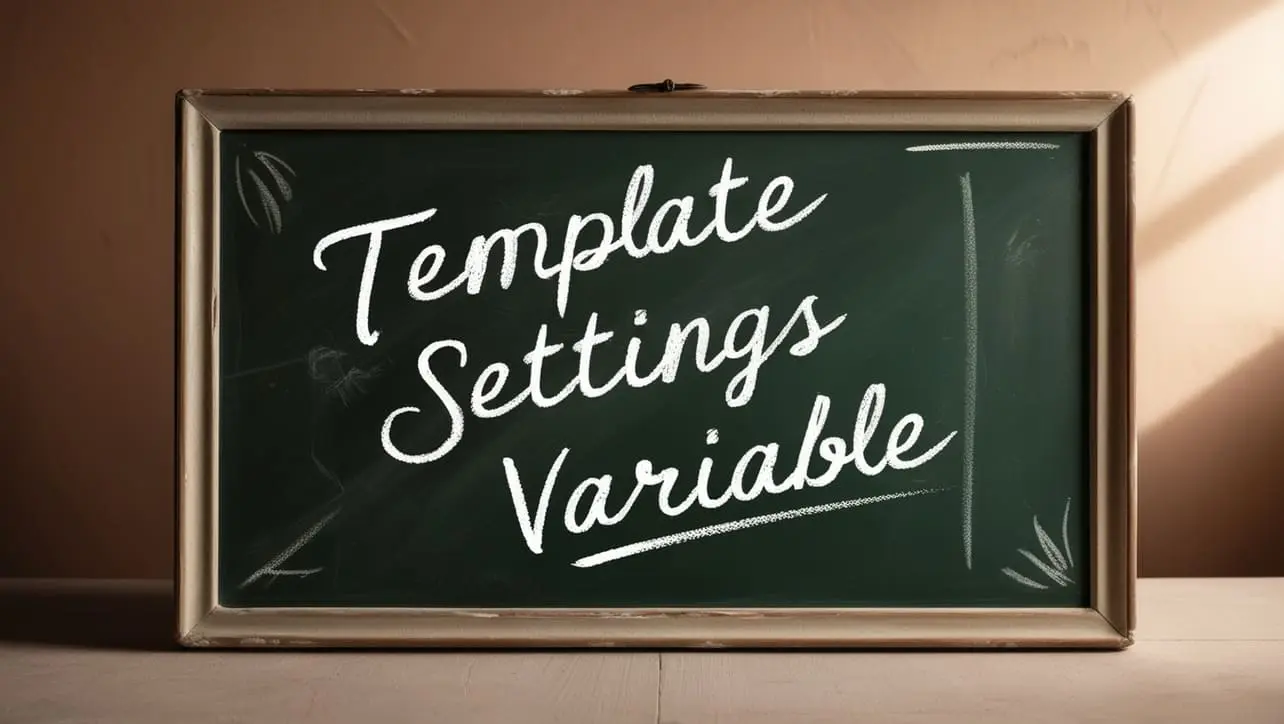
If you have any doubts regarding this article (Lodash _.sortedIndexBy() Array Method), please comment here. I will help you immediately.