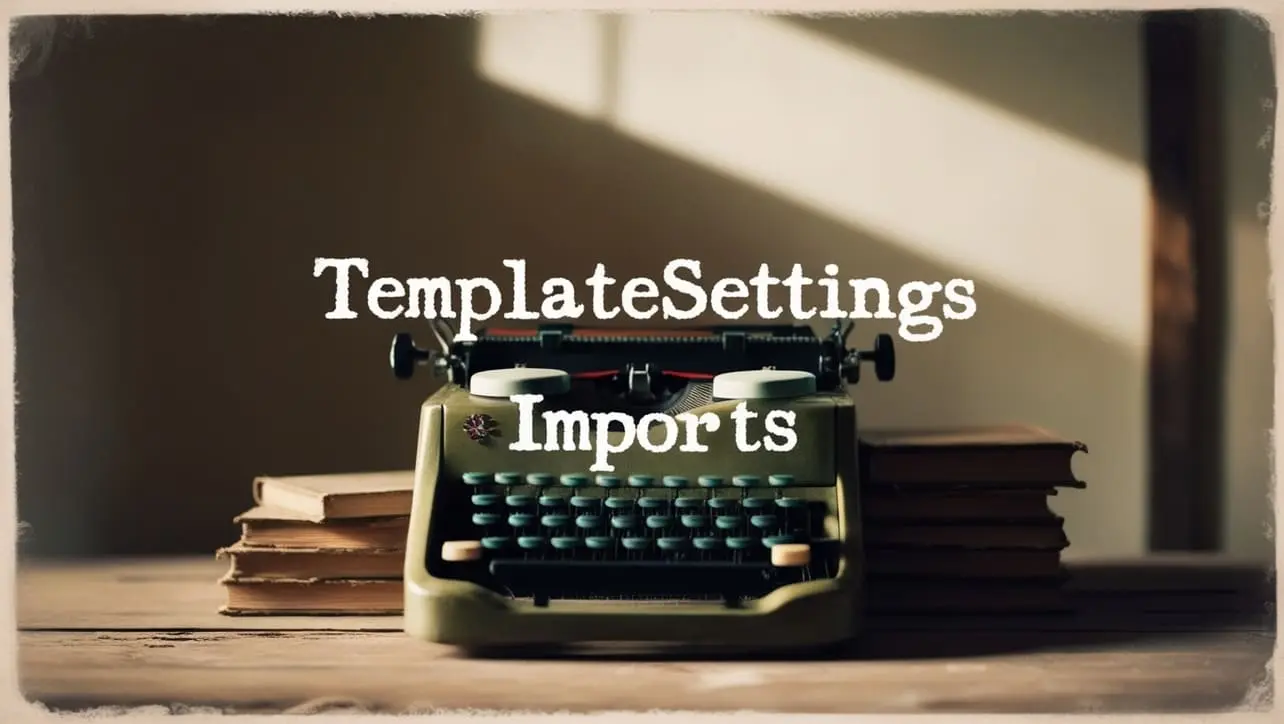
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.pullAt() Array Method
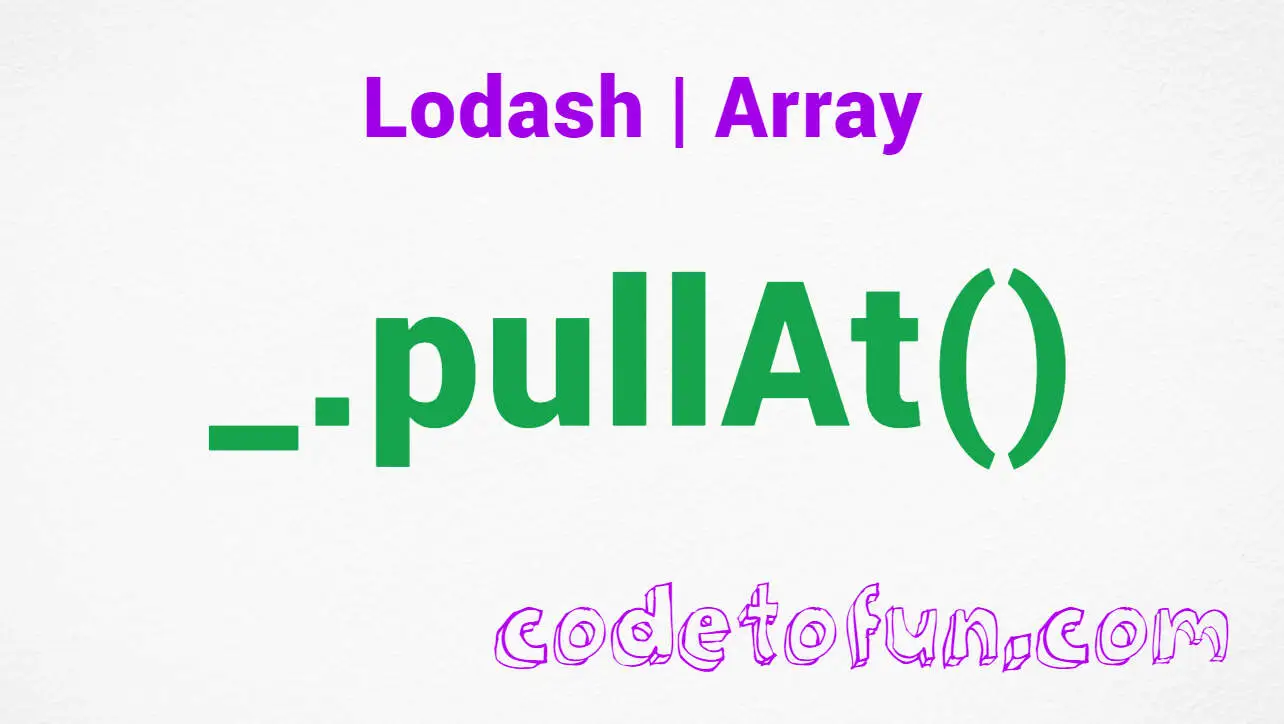
Photo Credit to CodeToFun
🙋 Introduction
Array manipulation is a common task in JavaScript development, and the Lodash library provides a robust set of tools to simplify this process.
One such powerful method is _.pullAt()
, designed to remove elements from an array at specified indices. This method offers flexibility and efficiency, making it a valuable asset in scenarios where precise control over element removal is crucial.
🧠 Understanding _.pullAt()
The _.pullAt()
method in Lodash allows you to remove elements from an array based on the provided indices. This is particularly useful when you need to extract specific elements or perform targeted deletions within an array.
💡 Syntax
_.pullAt(array, [indexes])
- array: The array to modify.
- indexes: An array of indices indicating the elements to be removed.
📝 Example
Let's dive into a practical example to illustrate the power of _.pullAt()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = ['apple', 'banana', 'cherry', 'date'];
const removedElements = _.pullAt(originalArray, [1, 3]);
console.log(originalArray);
// Output: ['apple', 'cherry']
console.log(removedElements);
// Output: ['banana', 'date']
In this example, the elements at indices 1 and 3 are removed from the originalArray, and the removed elements are stored in the removedElements array.
🏆 Best Practices
Validate Indices:
Before using
_.pullAt()
, ensure that the provided indices are valid and within the bounds of the array. Invalid indices can lead to unexpected behavior or errors.example.jsCopiedconst invalidIndices = [2, 5, 8]; const validIndices = invalidIndices.filter(index => index >= 0 && index < originalArray.length); const resultArray = _.pullAt(originalArray, validIndices); console.log(resultArray);
Immutable Approach:
If you want to keep the original array intact and create a new array with the removed elements, consider using the spread operator or other array manipulation methods.
example.jsCopiedconst originalArray = ['apple', 'banana', 'cherry', 'date']; const indexesToRemove = [1, 3]; const newArray = [...originalArray]; const removedElements = indexesToRemove.map(index => newArray.splice(index, 1)[0]); console.log(newArray); // Output: ['apple', 'cherry'] console.log(removedElements); // Output: ['banana', 'date']
Efficient Batch Removal:
For removing multiple elements at once,
_.pullAt()
excels in terms of readability and simplicity. However, for large arrays, consider using other methods like Array.prototype.filter() for better performance.example.jsCopiedconst indexesToRemove = [1, 3]; const newArray = originalArray.filter((_, index) => !indexesToRemove.includes(index)); console.log(newArray); // Output: ['apple', 'cherry']
📚 Use Cases
Specific Element Removal:
When you need to remove specific elements from an array,
_.pullAt()
provides a concise and efficient solution.example.jsCopiedconst fruits = ['apple', 'banana', 'cherry', 'date']; const indicesToRemove = [1, 3]; const modifiedFruits = _.pullAt(fruits, indicesToRemove); console.log(modifiedFruits); // Output: ['apple', 'cherry']
Controlled Deletion:
In scenarios where you want to precisely control which elements to delete,
_.pullAt()
empowers you to specify the indices for deletion.example.jsCopiedconst data = /* ...retrieve data from an external source... */; const indicesToDelete = /* ...determine indices based on criteria... */; _.pullAt(data, indicesToDelete); console.log(data); // Output: Modified array with specified elements removed
Undo Operations:
In situations where users can undo their actions, keeping track of removed elements with
_.pullAt()
allows you to easily revert changes.example.jsCopiedconst initialData = /* ...initialize data... */; const modifiedData = [...initialData]; const removedElements = _.pullAt(modifiedData, /* ...indices... */); // ...perform actions... // User decides to undo changes modifiedData.push(...removedElements); console.log(modifiedData); // Output: Reverted array with elements restored
🎉 Conclusion
The _.pullAt()
method in Lodash is a versatile tool for array manipulation, providing a straightforward way to remove elements at specified indices. Whether you need to precisely control deletions or perform targeted extractions, _.pullAt()
can significantly simplify your code.
Explore the capabilities of Lodash and leverage _.pullAt()
to enhance the efficiency and clarity of your array manipulation tasks!
👨💻 Join our Community:
Author
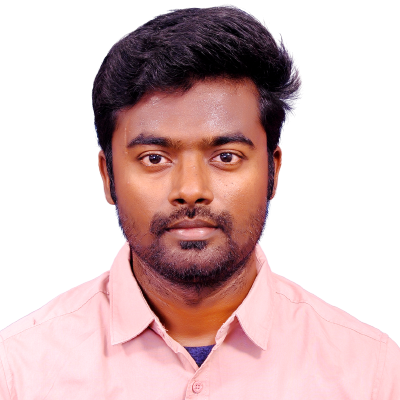
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
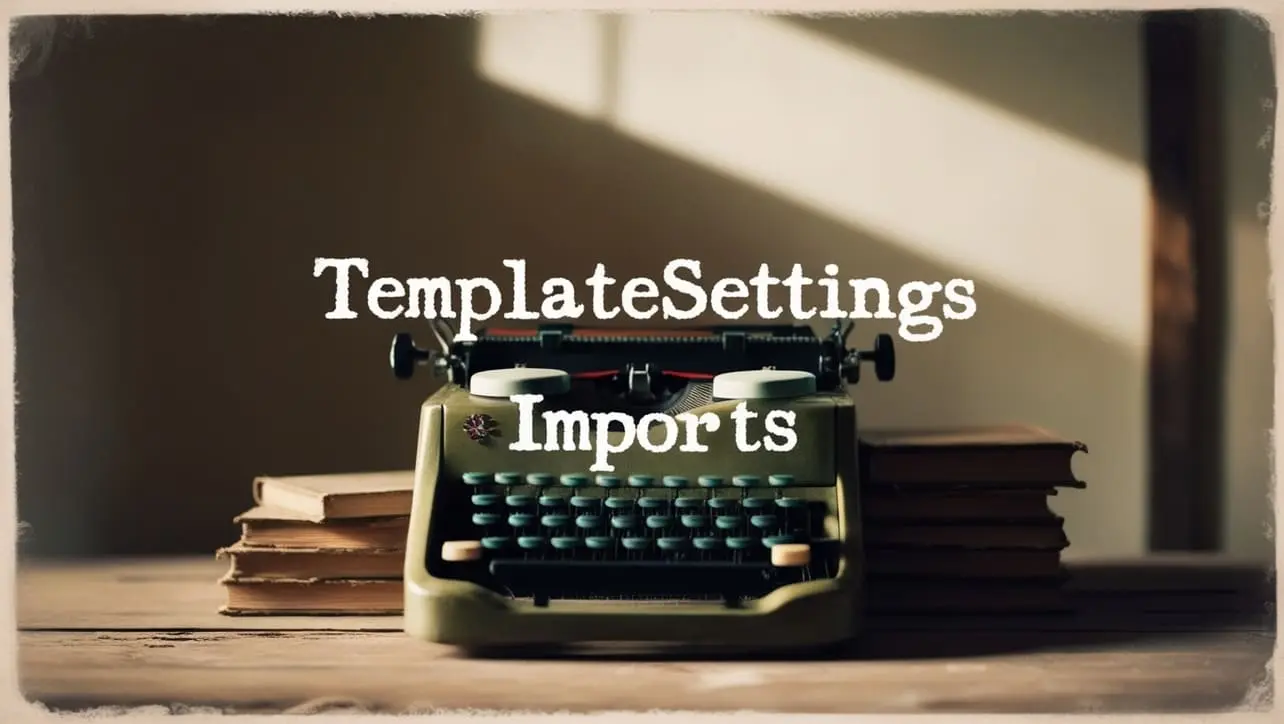
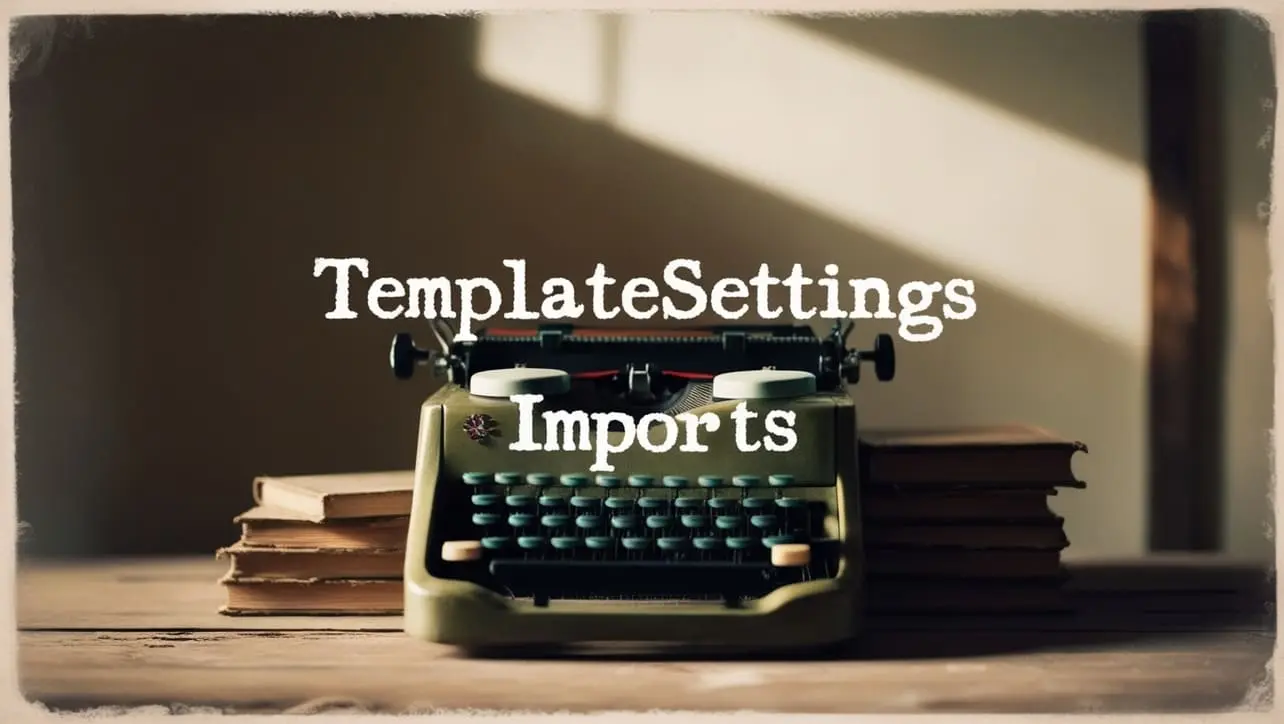
Lodash _.templateSettings.imports Property
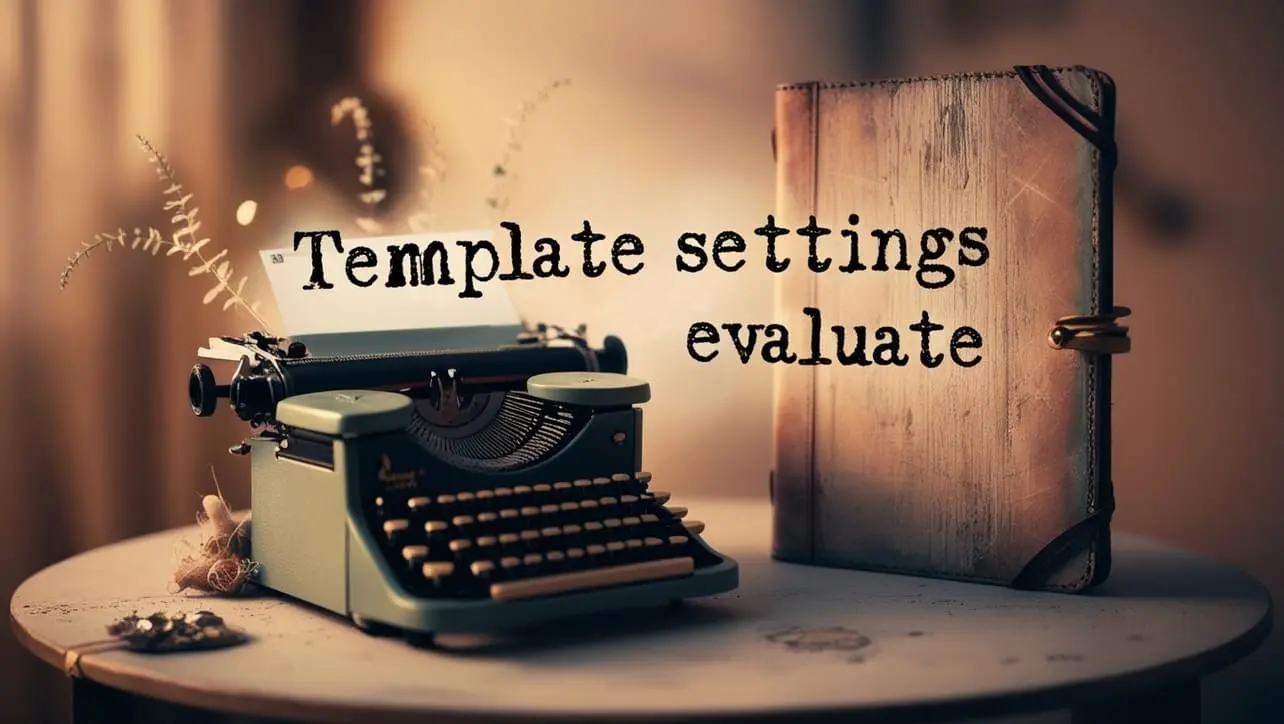
Lodash _.templateSettings.evaluate Property
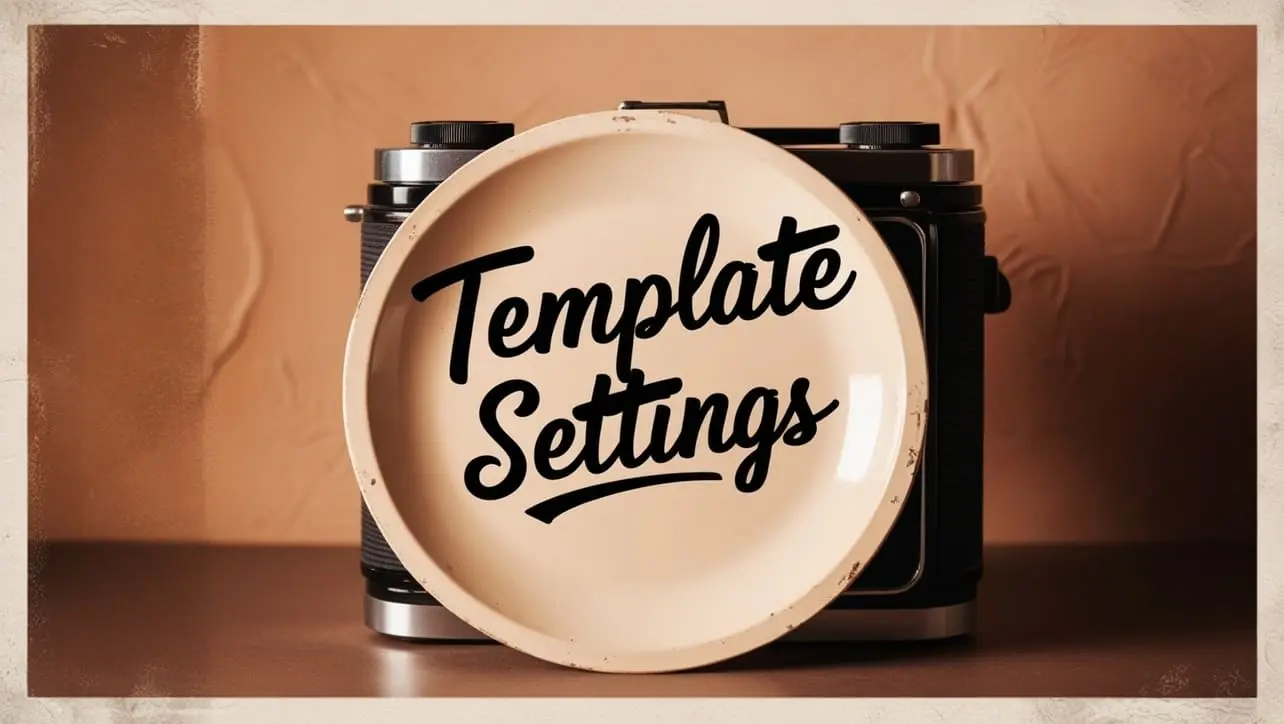
Lodash _.templateSettings Property
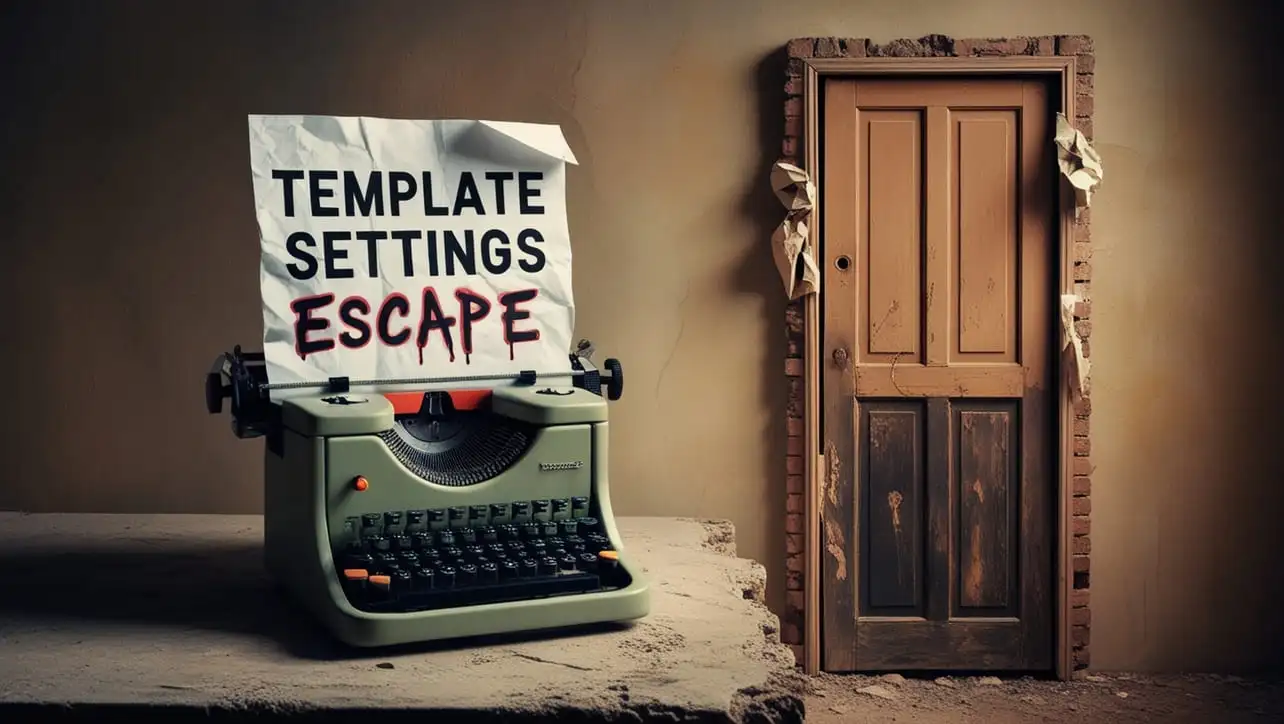
Lodash _.templateSettings.escape Property
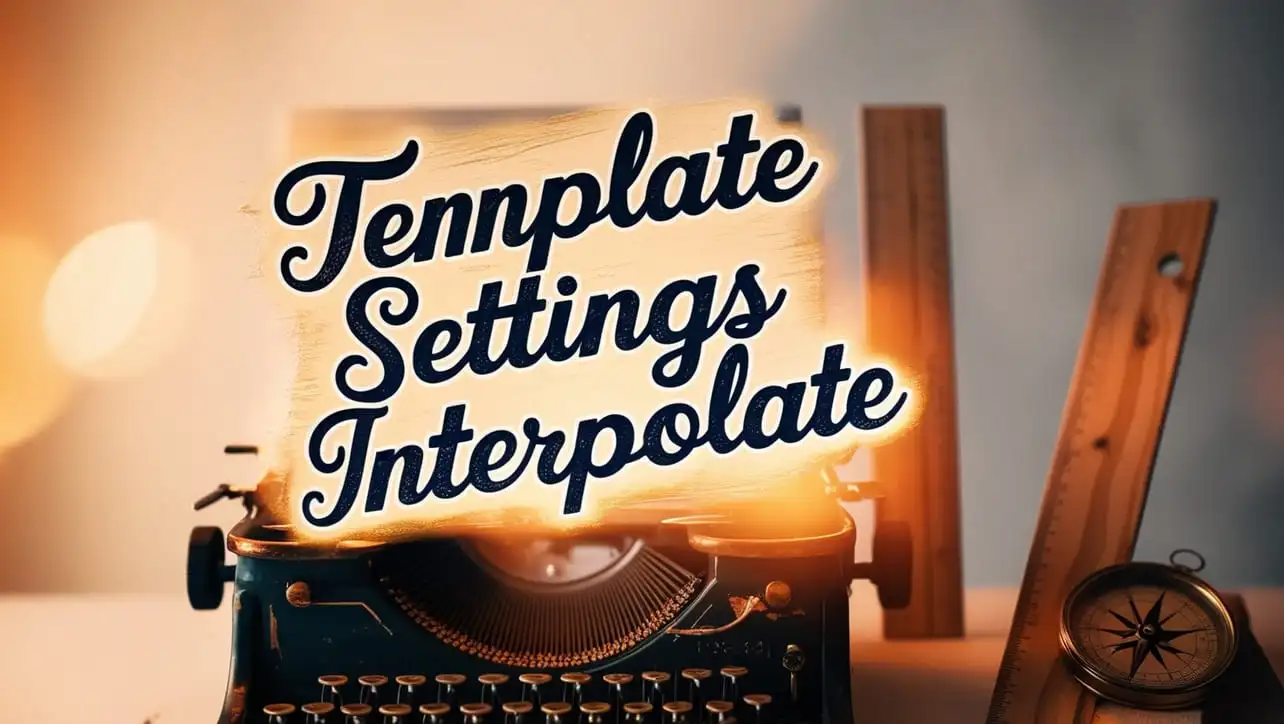
Lodash _.templateSettings.interpolate Property
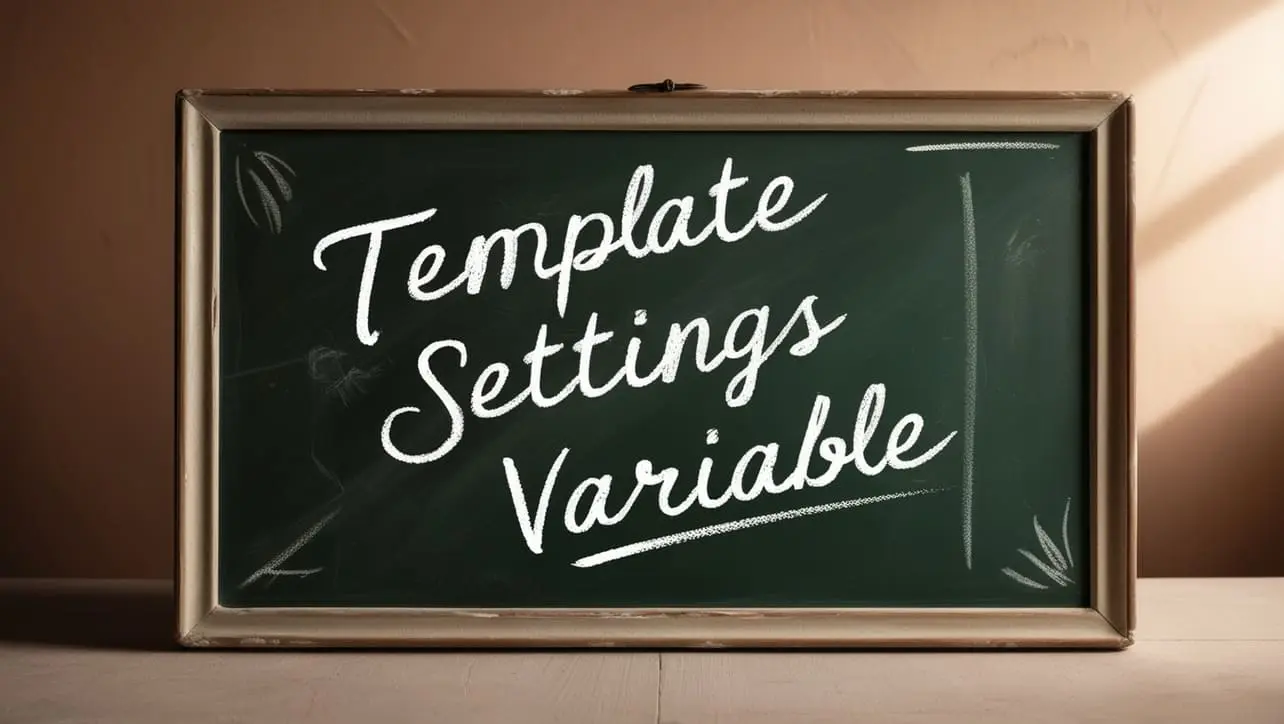
If you have any doubts regarding this article (Lodash _.pullAt() Array Method), please comment here. I will help you immediately.