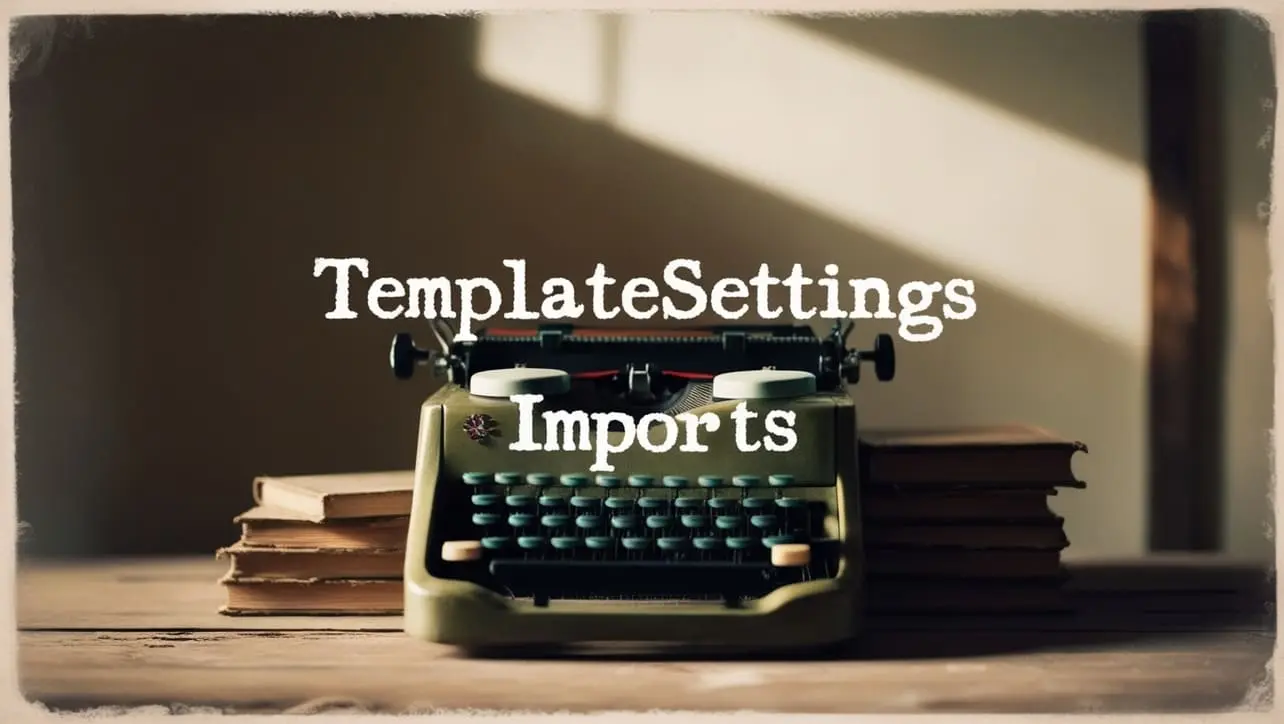
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.pull() Array Method
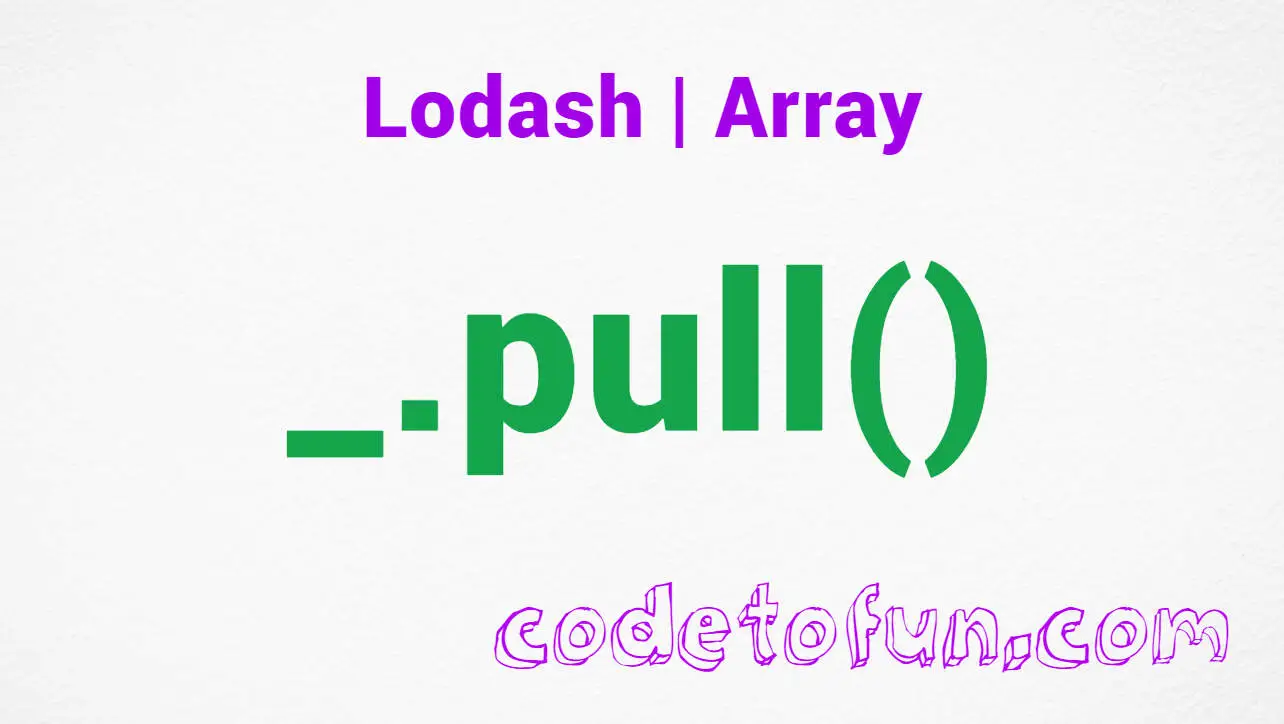
Photo Credit to CodeToFun
🙋 Introduction
When it comes to manipulating arrays in JavaScript, the Lodash library offers a wide array of utility functions, and one such powerful method is _.pull()
.
This method provides a straightforward way to remove specified values from an array, streamlining code and enhancing readability.
🧠 Understanding _.pull()
The _.pull()
method in Lodash is designed to remove all occurrences of specified values from an array. This can be particularly useful when you need to filter unwanted elements, creating a more concise and focused array.
💡 Syntax
_.pull(array, ...values)
- array: The array from which values will be removed.
- values: The values to remove from the array.
📝 Example
Let's dive into a practical example to illustrate the power of _.pull()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 2, 5, 6, 2, 7];
_.pull(originalArray, 2);
console.log(originalArray);
// Output: [1, 3, 4, 5, 6, 7]
In this example, all occurrences of the value 2 are removed from the originalArray, resulting in a new array without those elements.
🏆 Best Practices
Understand Mutability:
_.pull()
modifies the original array in place. Be aware of this mutability, especially if you need to preserve the original array.understand-mutability.jsCopiedconst originalArray = [1, 2, 3, 4, 2, 5, 6, 2, 7]; const newArray = _.pull([...originalArray], 2); console.log(originalArray); // Output: [1, 2, 3, 4, 2, 5, 6, 2, 7] console.log(newArray); // Output: [1, 3, 4, 5, 6, 7]
Multiple Values:
_.pull()
allows you to remove multiple values in a single call, providing a convenient way to clean up arrays.multiple-values.jsCopiedconst arrayWithDuplicates = [1, 2, 3, 4, 2, 5, 6, 2, 7]; _.pull(arrayWithDuplicates, 2, 4, 6); console.log(arrayWithDuplicates); // Output: [1, 3, 5, 7]
Use with Filtering:
Combine
_.pull()
with other array filtering methods to create more complex and targeted transformations.use-with-filtering.jsCopiedconst originalArray = [1, 2, 3, 4, 2, 5, 6, 2, 7]; const valuesToRemove = [2, 4, 6]; const newArray = originalArray.filter(value => !valuesToRemove.includes(value)); console.log(newArray); // Output: [1, 3, 5, 7]
📚 Use Cases
Data Cleaning:
_.pull()
is excellent for data cleaning, removing unwanted values or duplicates from arrays.data-cleaning.jsCopiedconst dataToClean = [10, 20, 30, null, 40, undefined, 50, null]; _.pull(dataToClean, null, undefined); console.log(dataToClean); // Output: [10, 20, 30, 40, 50]
Filtering User Input:
When dealing with user input or external data,
_.pull()
can help filter out specific values to maintain data integrity.filtering-user-input.jsCopiedconst userInput = ['apple', 'banana', 'apple', 'orange', 'grape']; _.pull(userInput, 'apple'); console.log(userInput); // Output: ['banana', 'orange', 'grape']
Managing Exclusions:
In scenarios where you have a list of values to exclude,
_.pull()
can efficiently remove them from an array.managing-exclusions.jsCopiedconst originalList = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const excludedValues = [2, 4, 6, 8]; _.pull(originalList, ...excludedValues); console.log(originalList); // Output: [1, 3, 5, 7, 9]
🎉 Conclusion
The _.pull()
method in Lodash is a versatile tool for array manipulation, providing a clean and efficient way to remove specified values. Whether you're cleaning up data, filtering user input, or managing exclusions, _.pull()
can simplify your code and enhance the clarity of your array transformations.
Explore the capabilities of Lodash and leverage the power of _.pull()
in your JavaScript projects!
👨💻 Join our Community:
Author
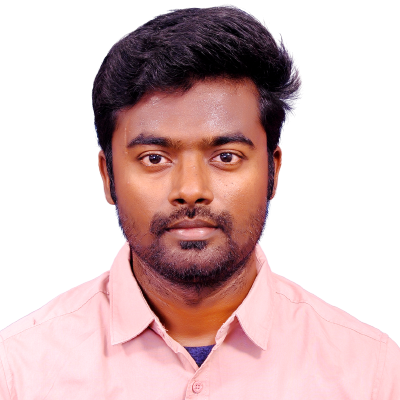
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
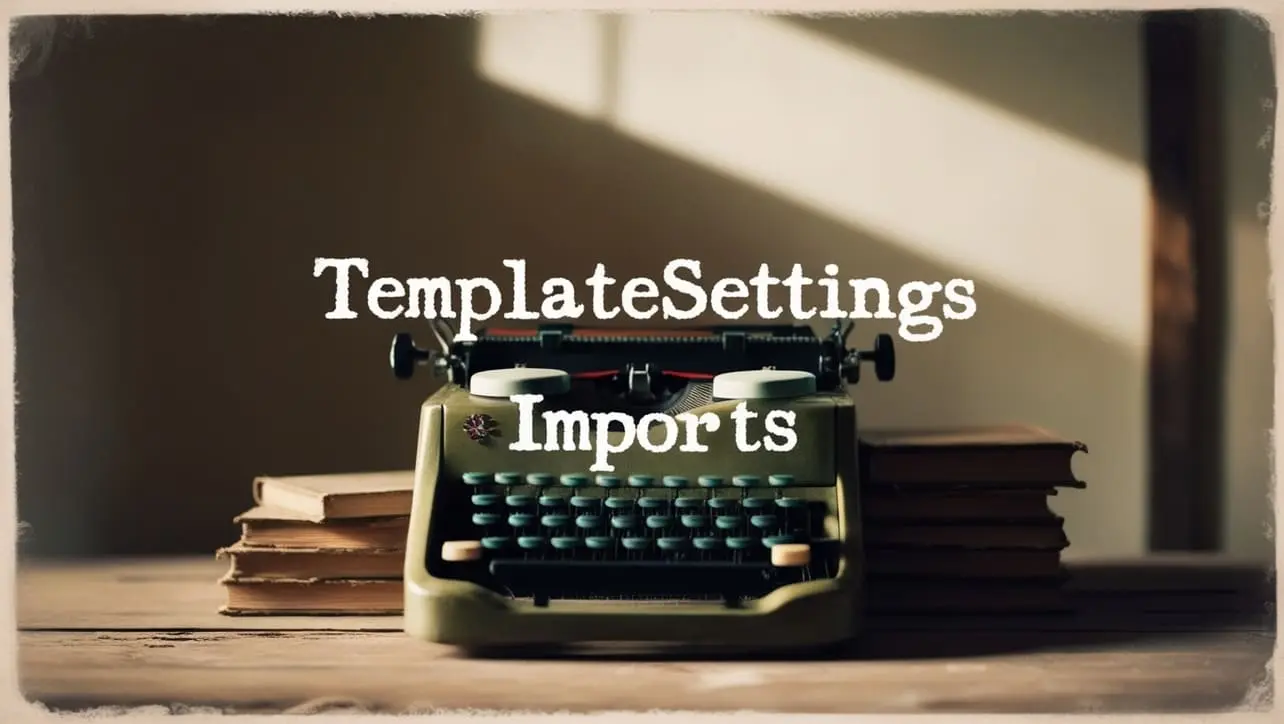
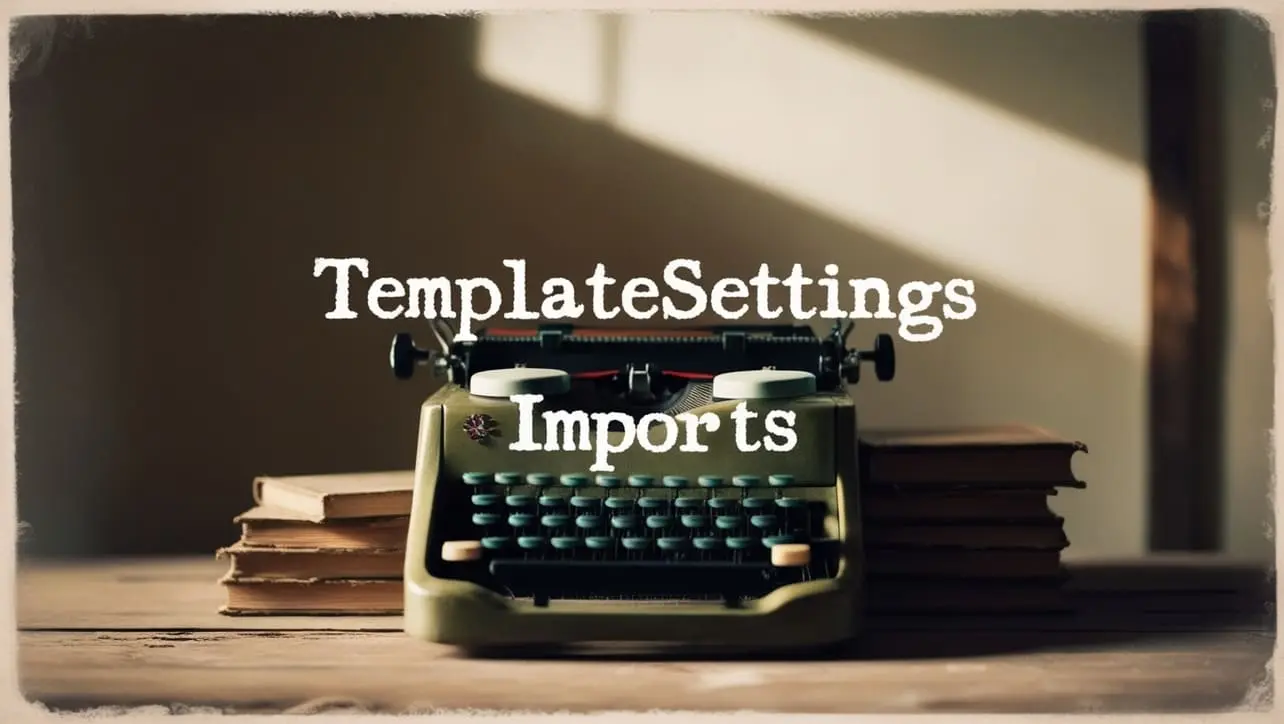
Lodash _.templateSettings.imports Property
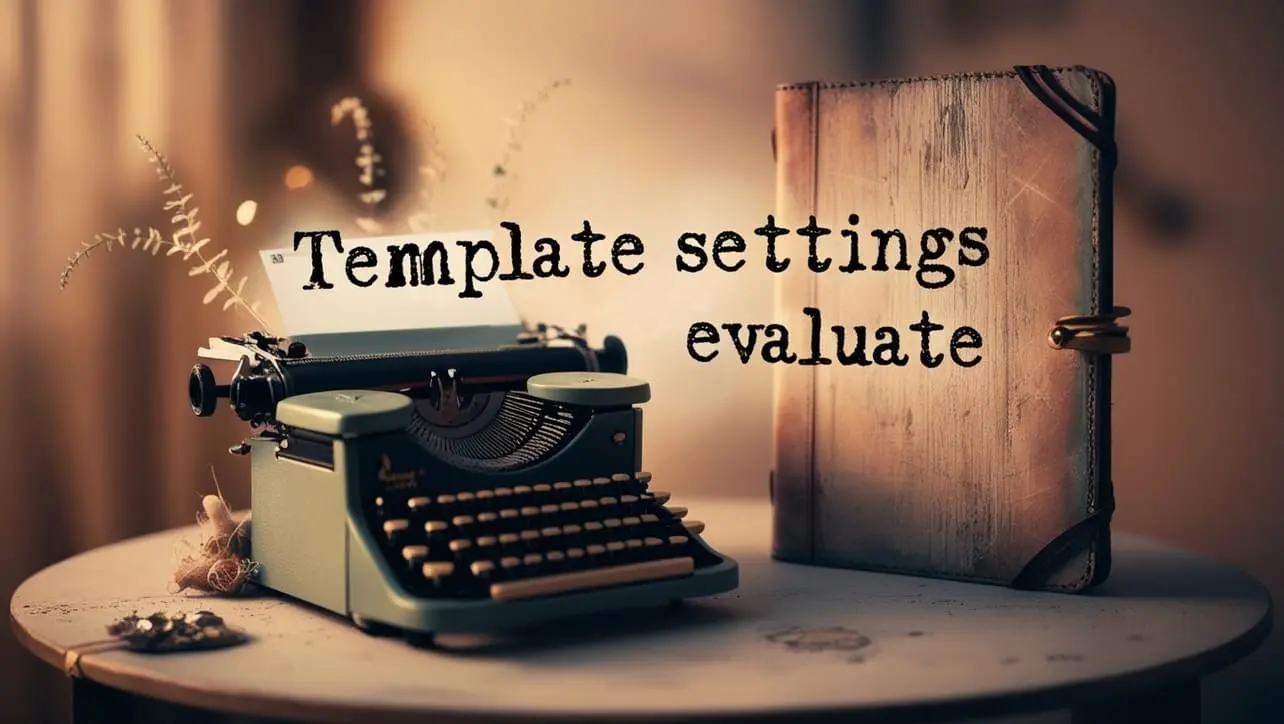
Lodash _.templateSettings.evaluate Property
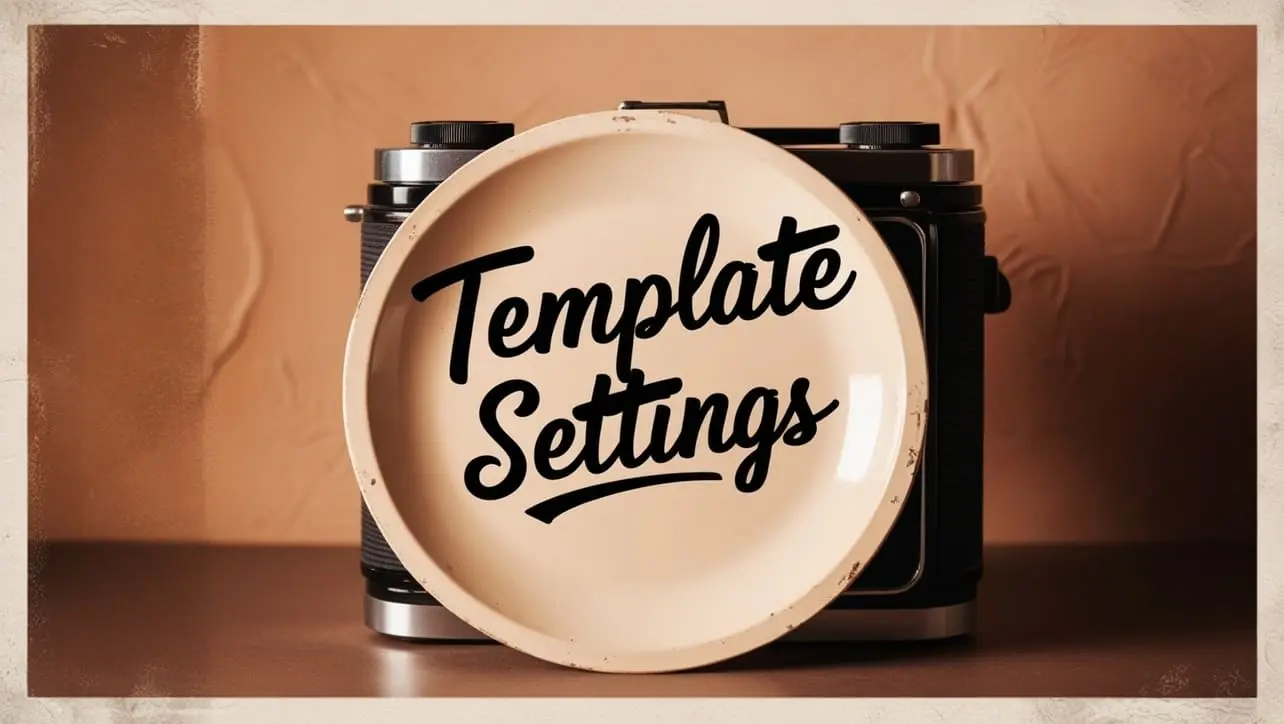
Lodash _.templateSettings Property
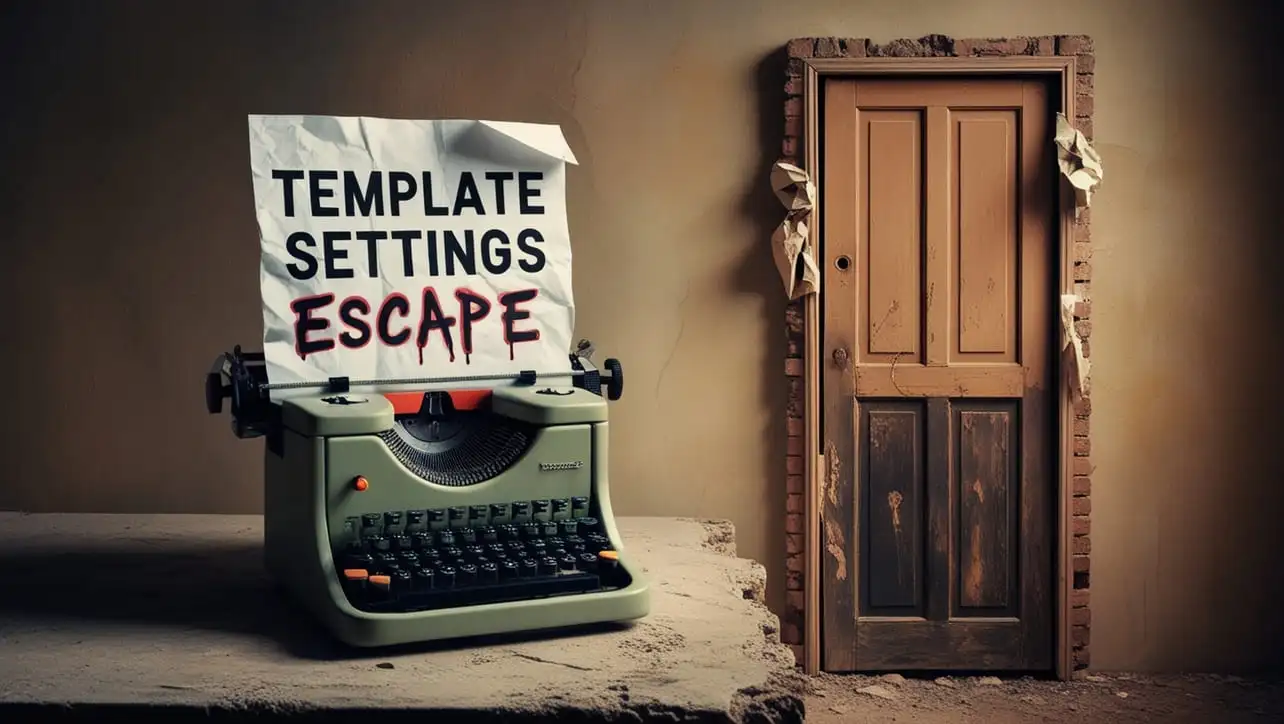
Lodash _.templateSettings.escape Property
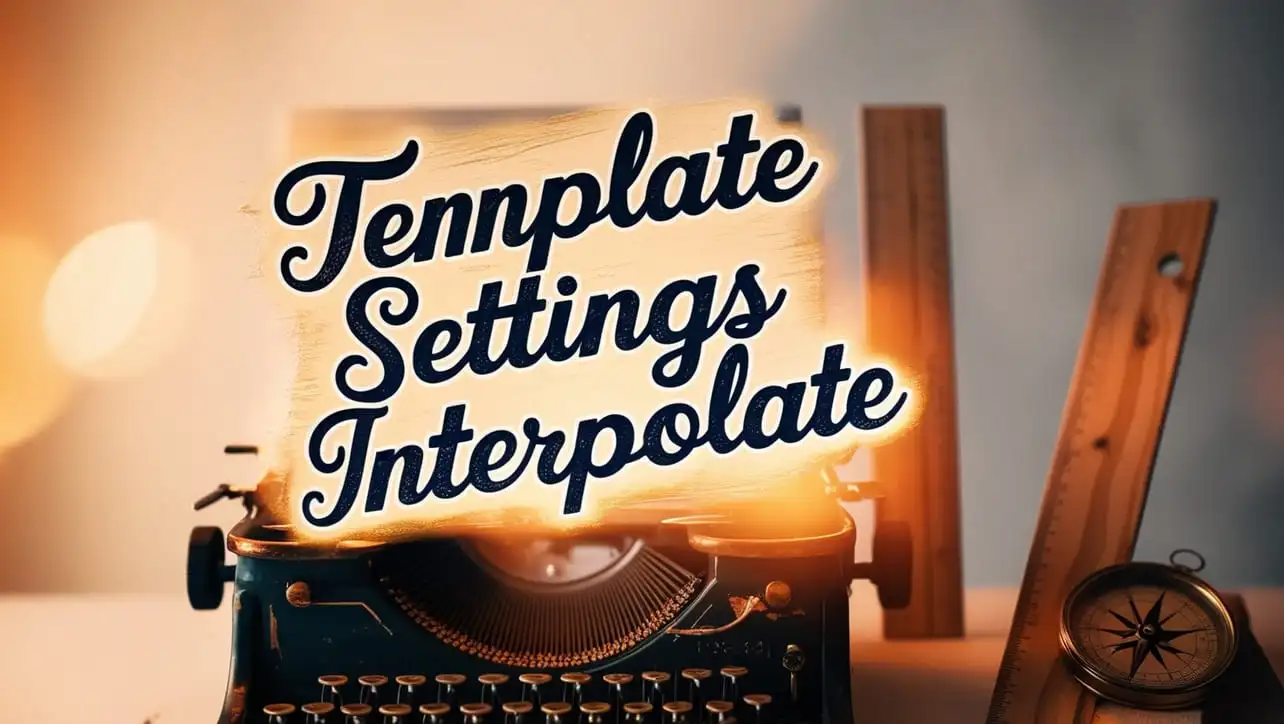
Lodash _.templateSettings.interpolate Property
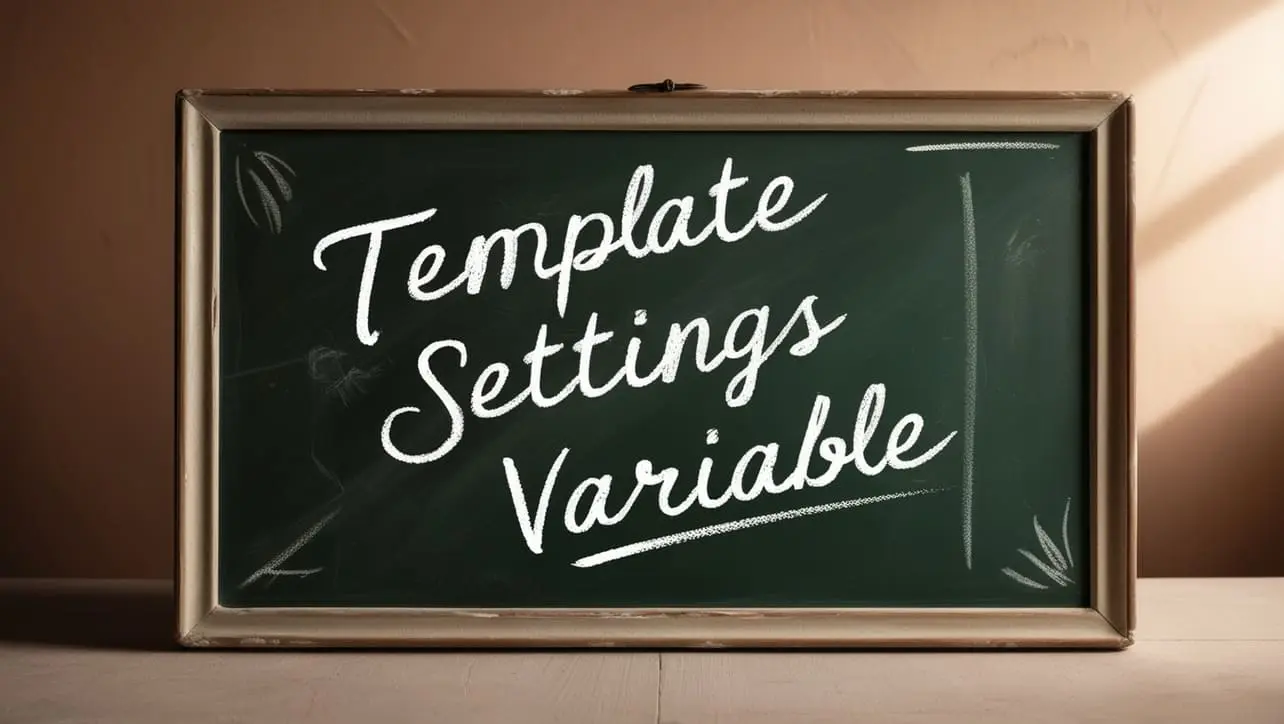
If you have any doubts regarding this article (Lodash _.pull() Array Method), please comment here. I will help you immediately.