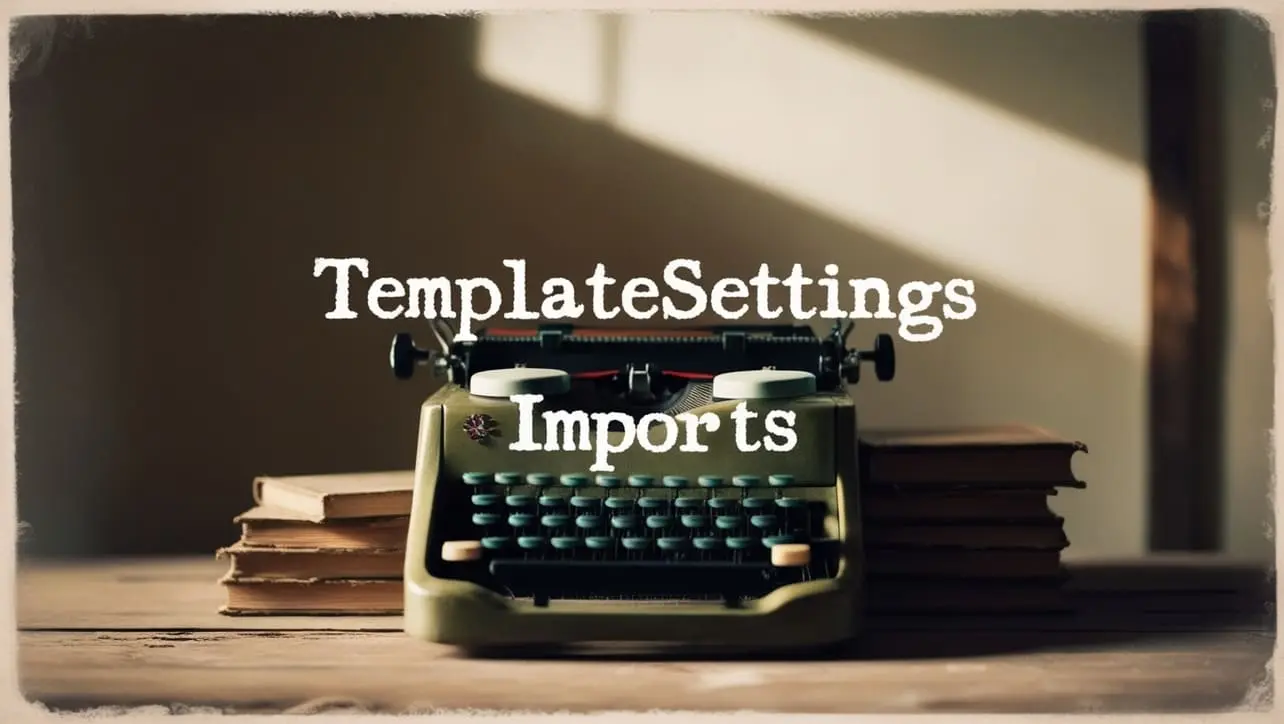
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.lastIndexOf() Array Method
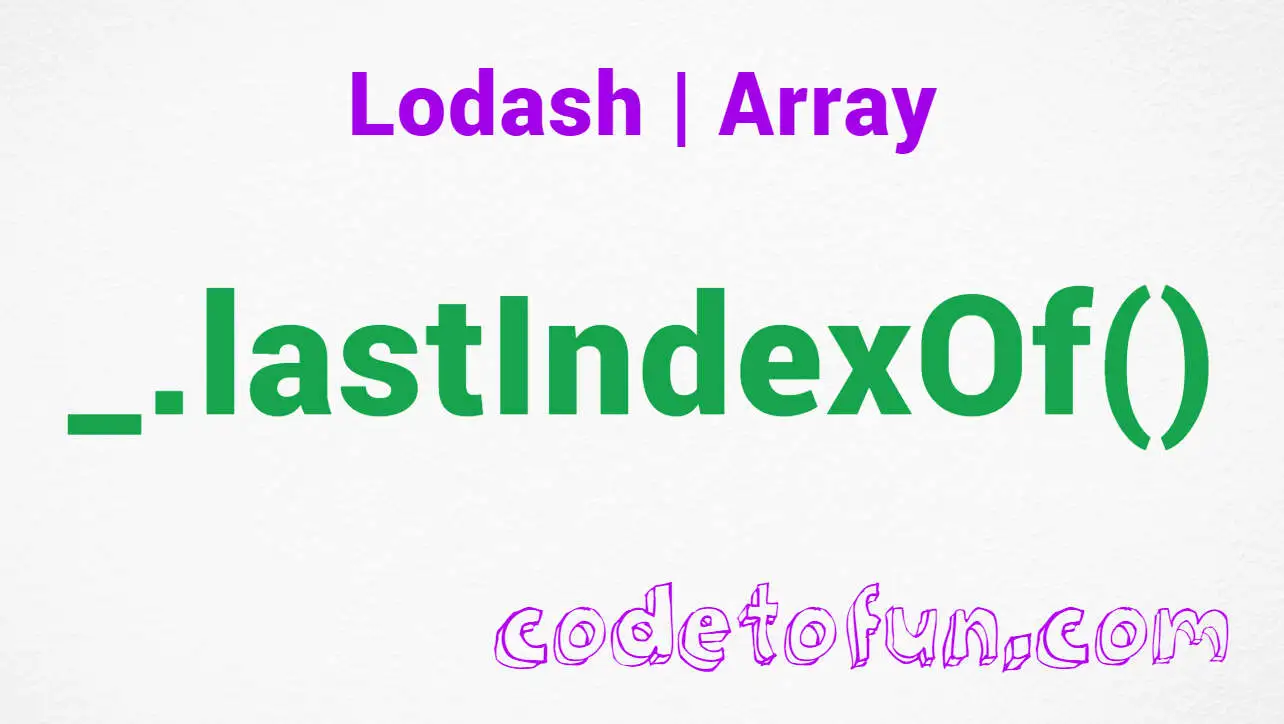
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, effective array manipulation is fundamental, and the Lodash library offers a myriad of utility functions to streamline these tasks. Among these, the _.lastIndexOf()
method stands out, providing a concise solution for finding the last occurrence of an element in an array.
This method proves invaluable when working with arrays, allowing developers to efficiently locate and manage elements.
🧠 Understanding _.lastIndexOf()
The _.lastIndexOf()
method in Lodash is designed to find the index of the last occurrence of a specified value within an array. This can be especially useful when dealing with ordered datasets, facilitating operations such as removing the last occurrence of an element or identifying the position of the latest entry.
💡 Syntax
_.lastIndexOf(array, value, [fromIndex=array.length-1])
- array: The array to search.
- value: The value to search for.
- fromIndex: The index to start the search from (default is array.length-1).
📝 Example
Let's delve into a practical example to illustrate the application of _.lastIndexOf()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const dataArray = [1, 2, 3, 4, 2, 5, 6, 2, 7];
const lastIndex = _.lastIndexOf(dataArray, 2);
console.log(lastIndex);
// Output: 7
In this example, the dataArray contains multiple occurrences of the value 2, and _.lastIndexOf()
identifies the index of the last occurrence.
🏆 Best Practices
Handle Nonexistent Values:
When using
_.lastIndexOf()
, account for situations where the specified value is not present in the array. The method returns -1 in such cases, and it's essential to handle this condition in your code.handle-nonexistent-values.jsCopiedconst nonExistentValue = 8; const indexOfNonExistent = _.lastIndexOf(dataArray, nonExistentValue); if (indexOfNonExistent === -1) { console.log(`${nonExistentValue} does not exist in the array.`); } else { console.log(`Index of ${nonExistentValue}: ${indexOfNonExistent}`); }
Specify the Starting Index:
For efficiency or specific use cases, you can specify the starting index (fromIndex) to narrow down the search range.
specify-starting-index.jsCopiedconst startingIndex = 4; const lastIndexFromIndex = _.lastIndexOf(dataArray, 2, startingIndex); console.log(lastIndexFromIndex); // Output: 4
Leverage Negative Indices:
To count positions from the end of the array, use negative values for fromIndex. This is particularly helpful when searching for the last occurrences.
negative-indices.jsCopiedconst lastIndexFromEnd = _.lastIndexOf(dataArray, 2, -2); console.log(lastIndexFromEnd); // Output: 4
📚 Use Cases
Removing Last Occurrence:
Easily remove the last occurrence of a specific value from an array.
removing-last-occurrence.jsCopiedconst valueToRemove = 2; const indexToRemove = _.lastIndexOf(dataArray, valueToRemove); if (indexToRemove !== -1) { dataArray.splice(indexToRemove, 1); } console.log(dataArray); // Output: [1, 2, 3, 4, 2, 5, 6, 7]
Find the Last Entry:
Identify the position of the last entry in a dataset.
find-last-entry.jsCopiedconst lastEntry = dataArray[_.lastIndexOf(dataArray, dataArray[dataArray.length - 1])]; console.log(lastEntry); // Output: 7
🎉 Conclusion
The _.lastIndexOf()
method in Lodash is a valuable addition to a JavaScript developer's toolkit, providing a straightforward solution for locating the last occurrence of a value in an array. By incorporating this method into your code, you can enhance the efficiency and precision of your array manipulation tasks.
Discover the power of _.lastIndexOf()
in Lodash and elevate your array handling capabilities!
👨💻 Join our Community:
Author
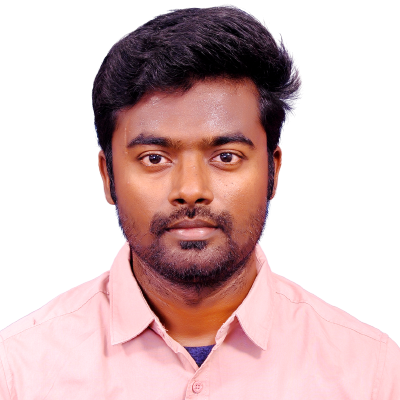
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
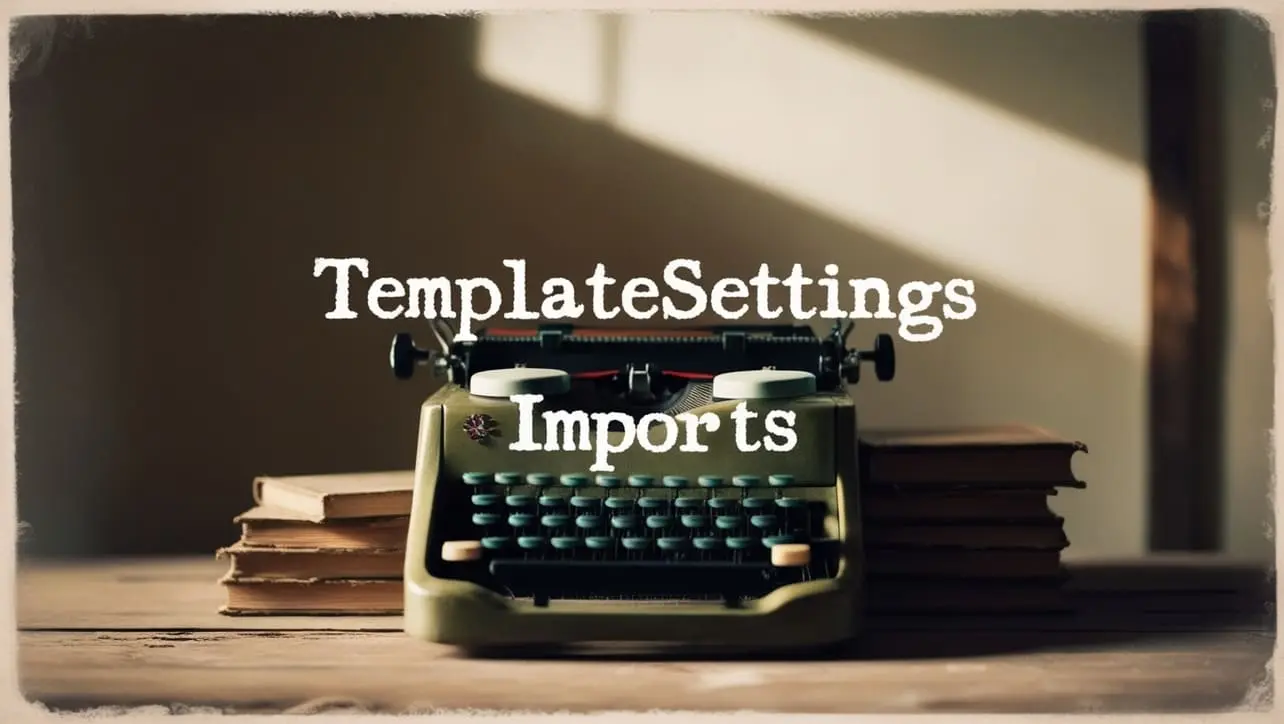
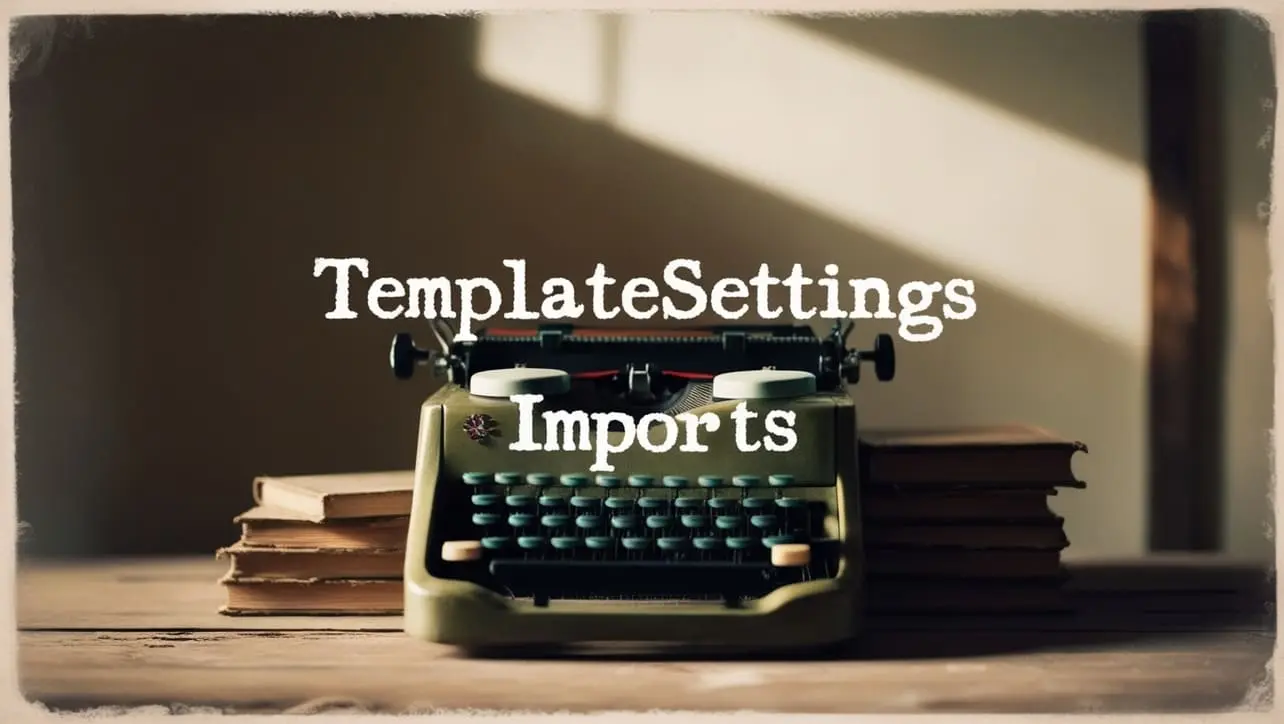
Lodash _.templateSettings.imports Property
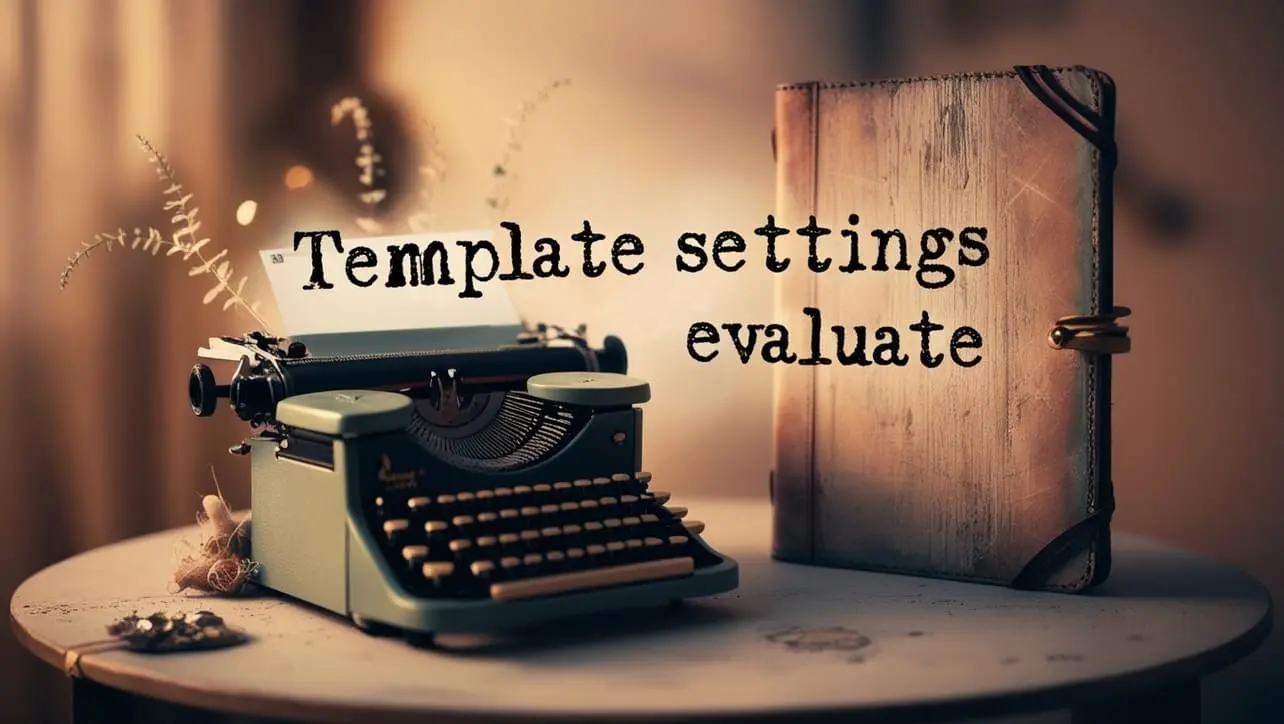
Lodash _.templateSettings.evaluate Property
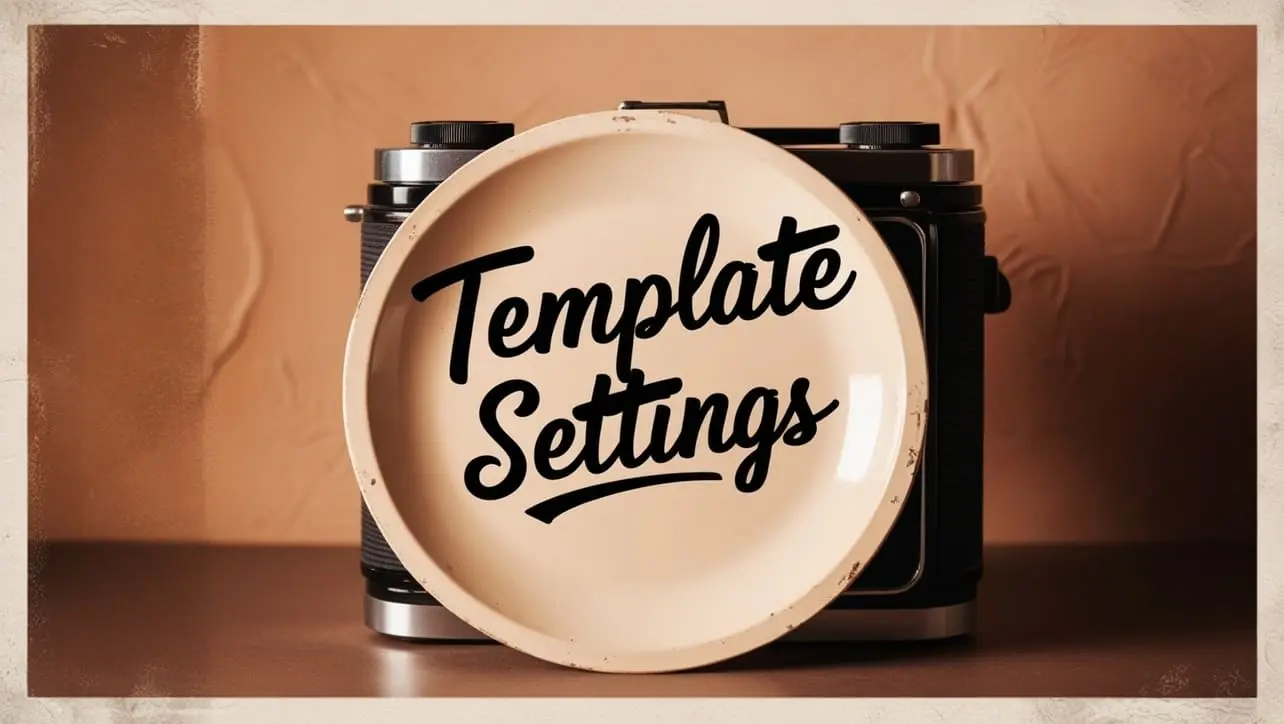
Lodash _.templateSettings Property
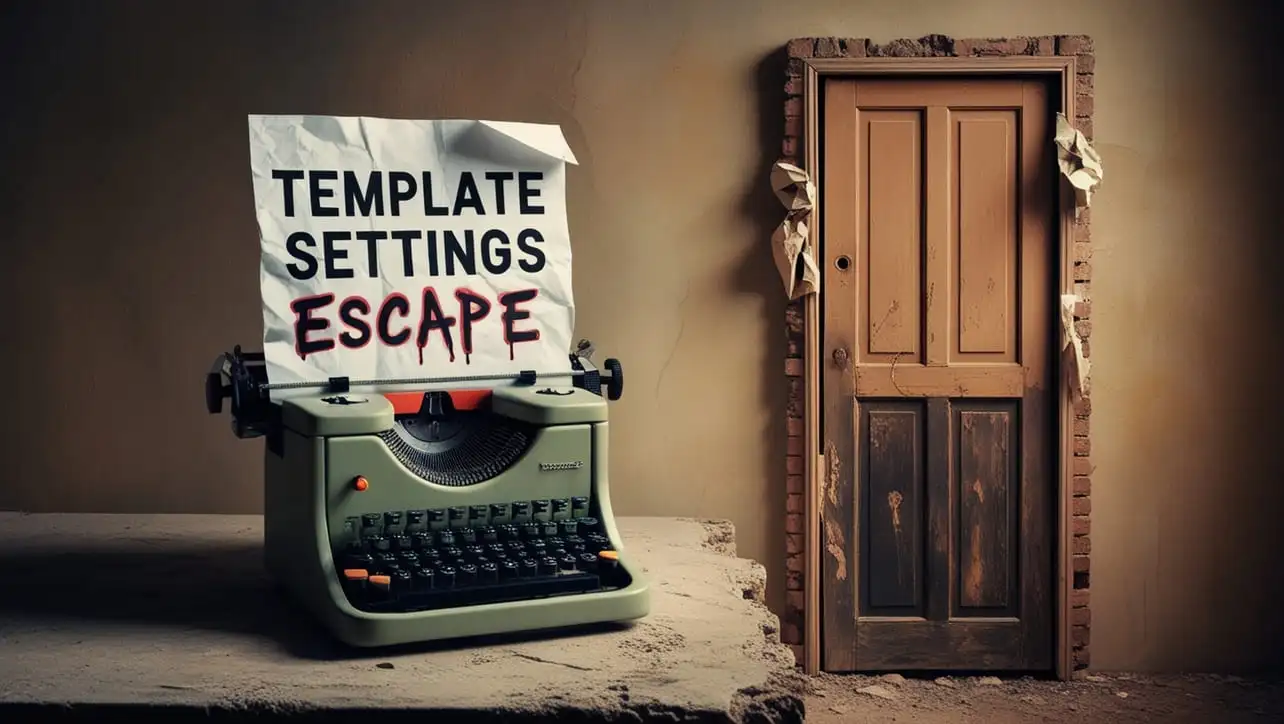
Lodash _.templateSettings.escape Property
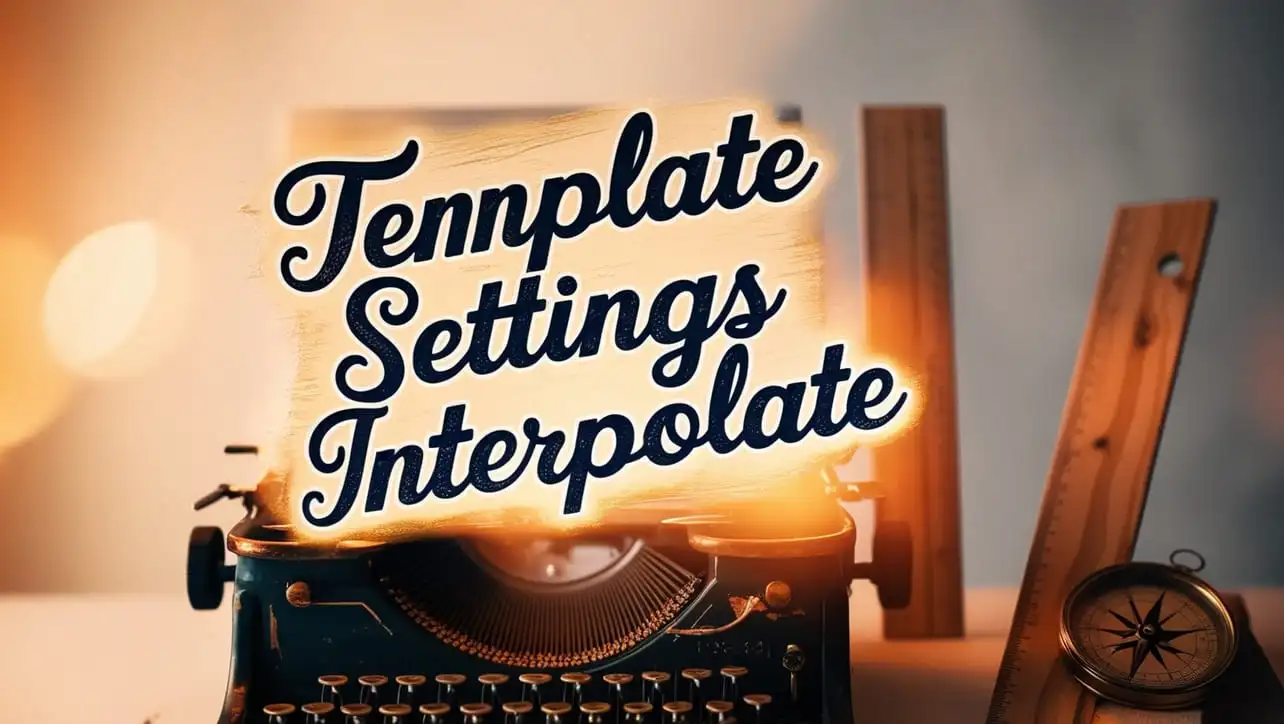
Lodash _.templateSettings.interpolate Property
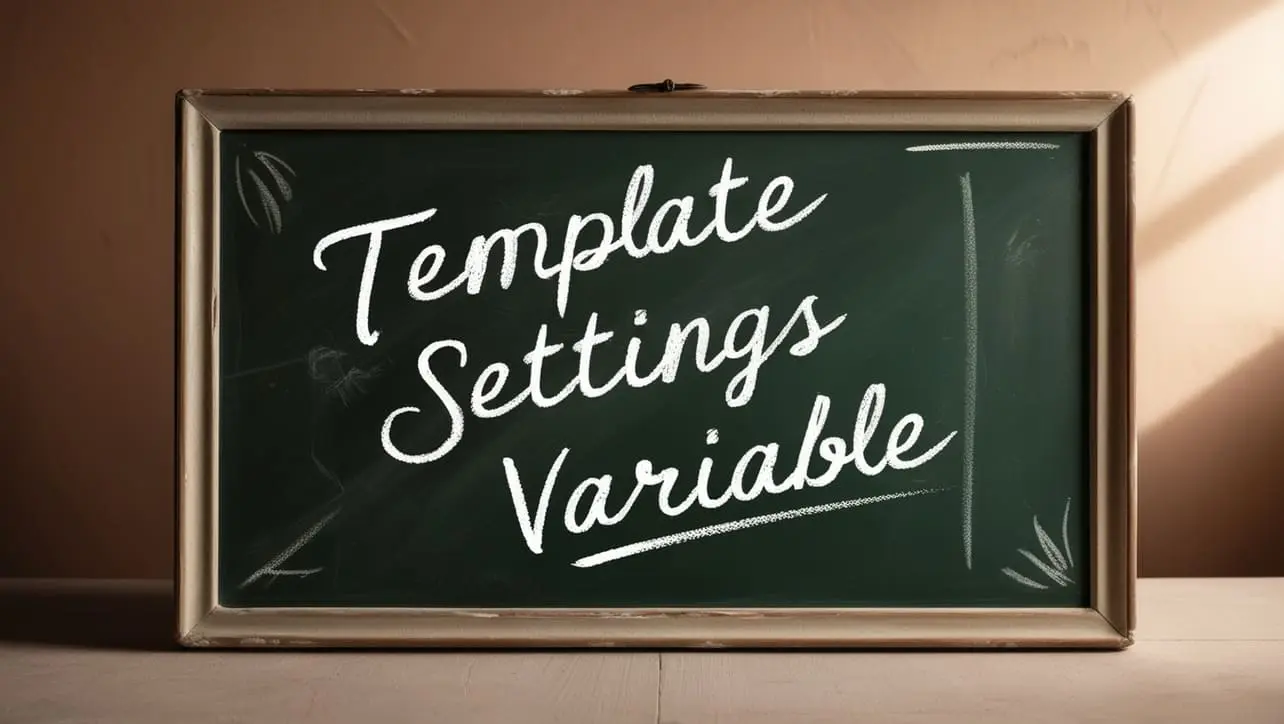
If you have any doubts regarding this article (Lodash _.lastIndexOf() Array Method), please comment here. I will help you immediately.