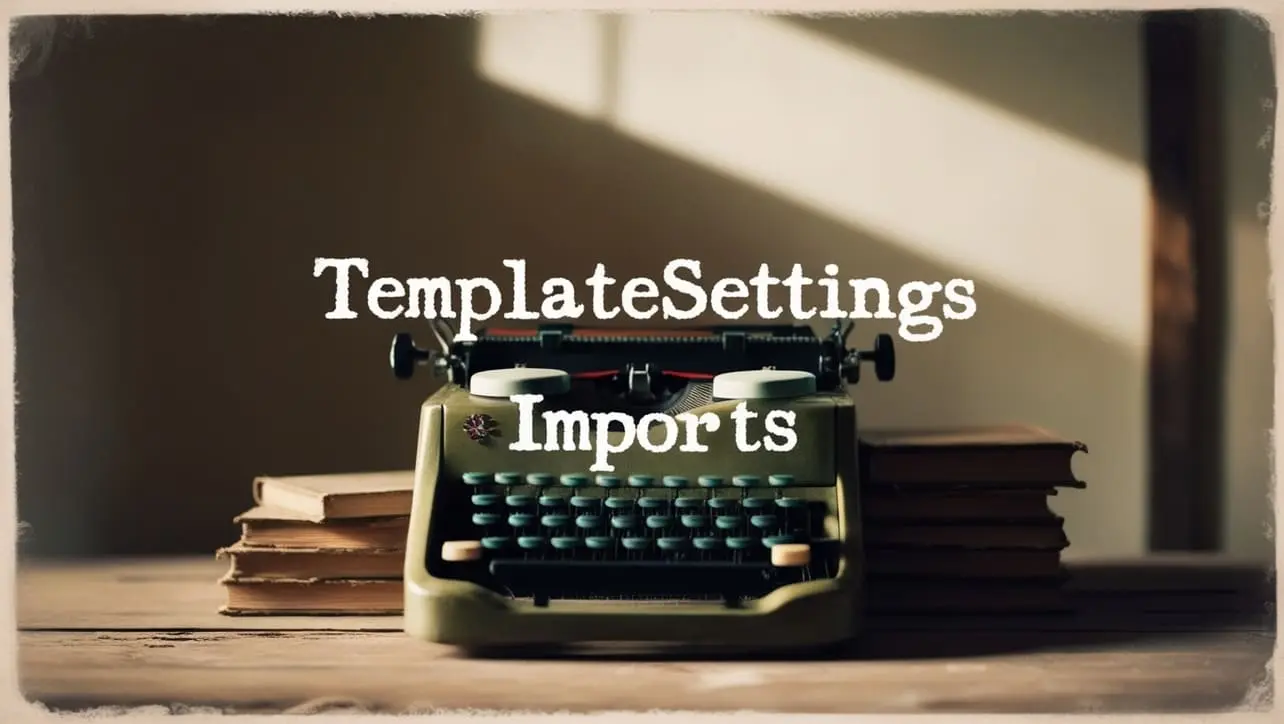
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.last() Array Method
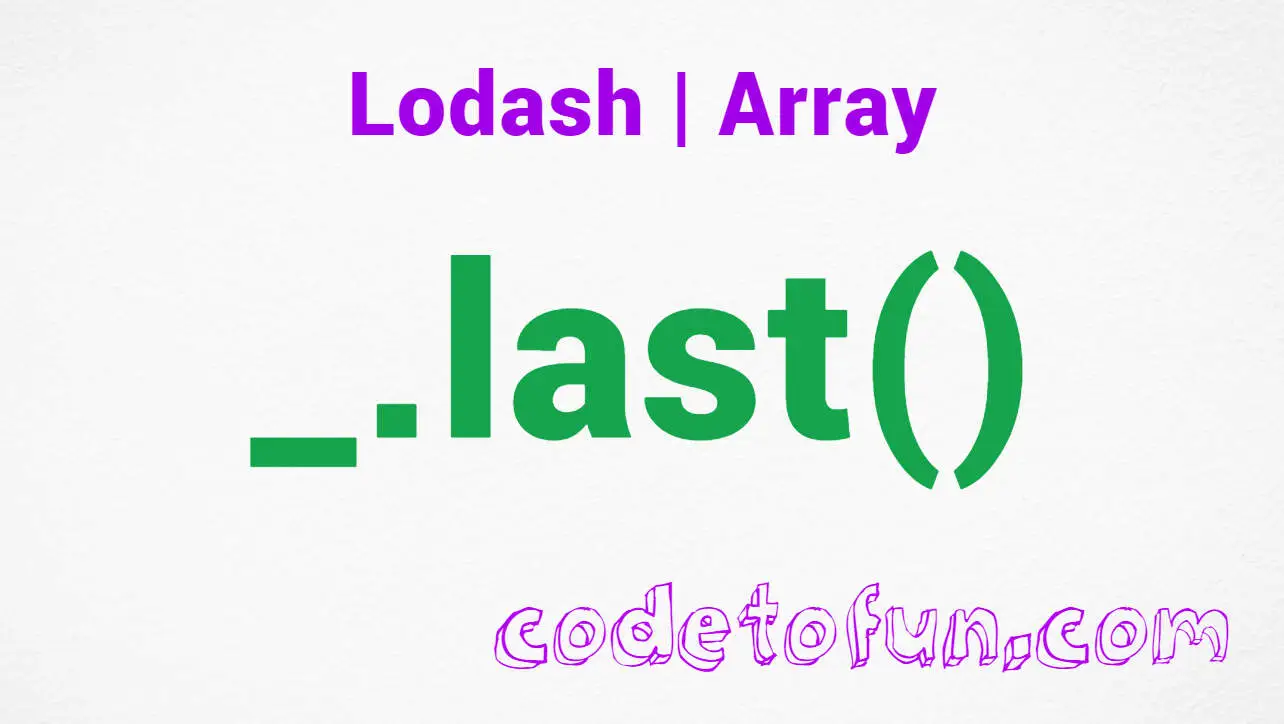
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, efficient array manipulation is a fundamental skill.
The Lodash library offers a variety of utility functions, and one such handy tool is the _.last()
method.
This method simplifies the process of retrieving the last element or elements from an array, proving invaluable for developers seeking concise and readable code.
🧠 Understanding _.last()
The _.last()
method in Lodash provides a straightforward way to access the last element or elements of an array.
💡 Syntax
_.last(array, [n=1])
- array: The array to query.
- n: The number of elements to retrieve from the end of the array (default is 1).
📝 Example
Let's delve into a practical example to illustrate the utility of _.last()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const exampleArray = [1, 2, 3, 4, 5];
const lastElement = _.last(exampleArray);
console.log(lastElement);
// Output: 5
In this example, the lastElement variable holds the value of the last element in the exampleArray.
🏆 Best Practices
Check Array Length:
Before using
_.last()
, ensure that the array has elements to avoid unexpected results.check-array-length.jsCopiedconst emptyArray = []; const result = _.last(emptyArray); console.log(result); // Output: undefined
Specify Number of Elements:
Explicitly specify the number of elements you want to retrieve using the optional n parameter.
number-elements.jsCopiedconst arrayWithMultipleElements = [1, 2, 3, 4, 5]; const multipleLastElements = _.last(arrayWithMultipleElements, 3); console.log(multipleLastElements); // Output: [3, 4, 5]
Handle Edge Cases:
Consider scenarios where the array might be shorter than the requested number of elements. Implement appropriate error handling or default behaviors.
handle-edge-cases.jsCopiedconst shortArray = [1, 2]; const result = _.last(shortArray, 3); console.log(result); // Output: [1, 2]
📚 Use Cases
Retrieving Last Element:
The primary use case of
_.last()
is to retrieve the last element from an array.retrieving-last-element.jsCopiedconst data = /* ...fetch data from API or elsewhere... */; const lastItem = _.last(data); console.log(lastItem);
Retrieving Last N Elements:
When you need to get the last N elements from an array, use the optional n parameter.
retrieving-last-n-elements.jsCopiedconst recentItems = _.last(data, 5); console.log(recentItems);
🎉 Conclusion
The _.last()
method in Lodash is a valuable asset for JavaScript developers dealing with arrays. Its simplicity and flexibility make it an excellent choice for scenarios where accessing the last element or elements of an array is a common task. By incorporating this method into your code, you can enhance the clarity and efficiency of your projects.
Discover the power of Lodash and simplify your array manipulation with _.last()
!
👨💻 Join our Community:
Author
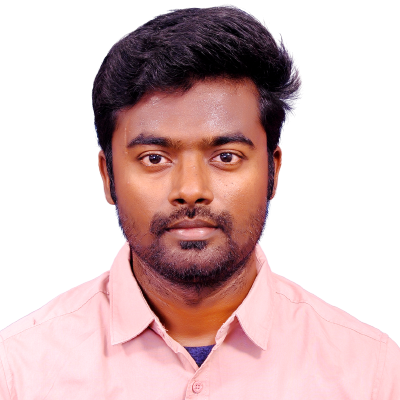
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
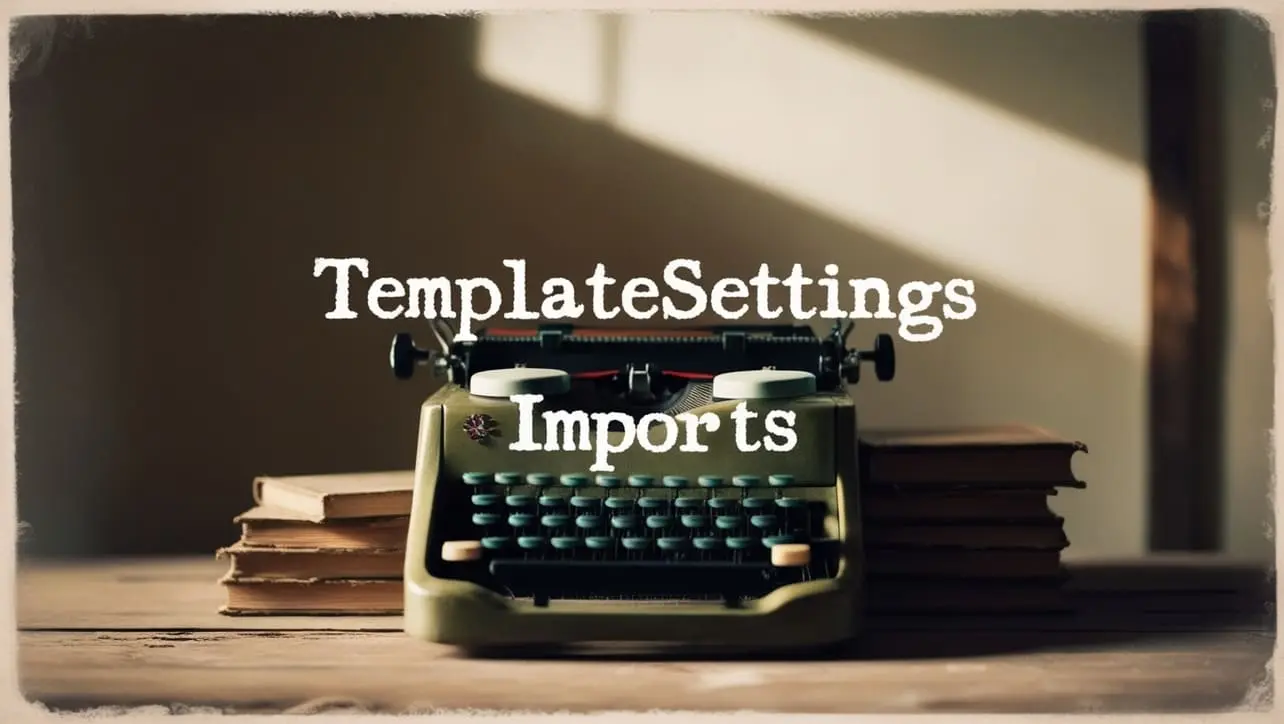
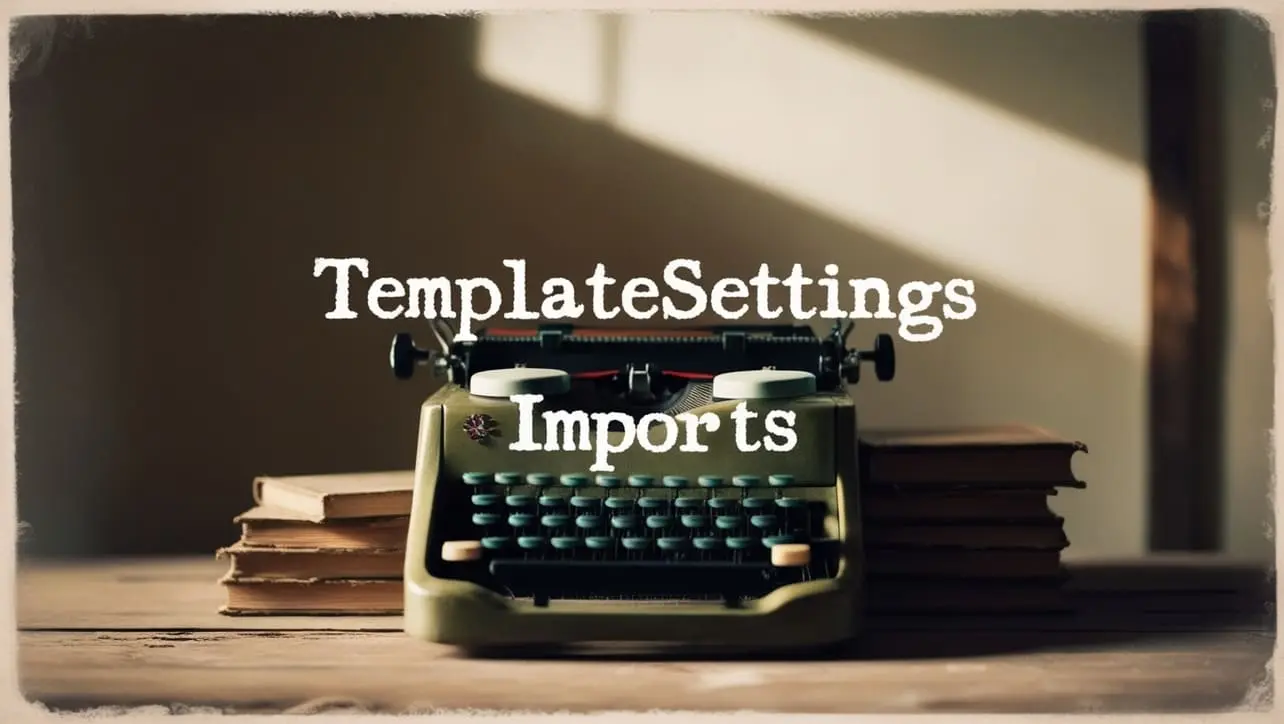
Lodash _.templateSettings.imports Property
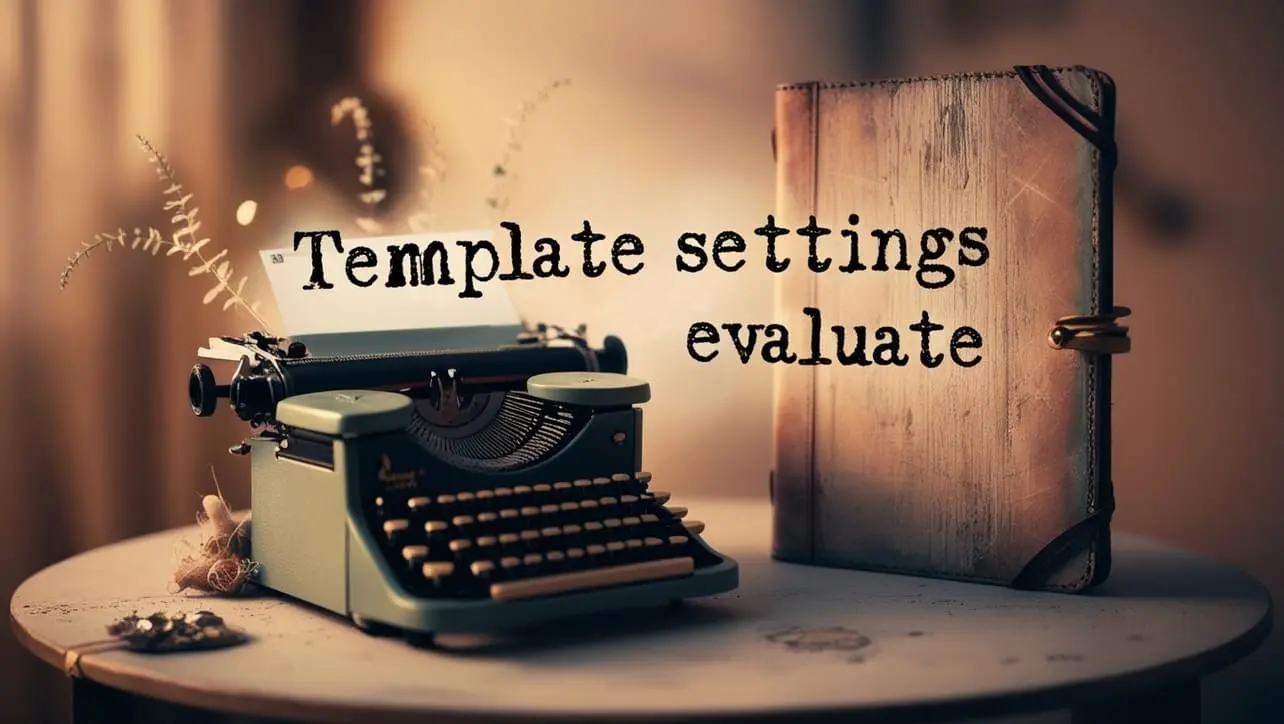
Lodash _.templateSettings.evaluate Property
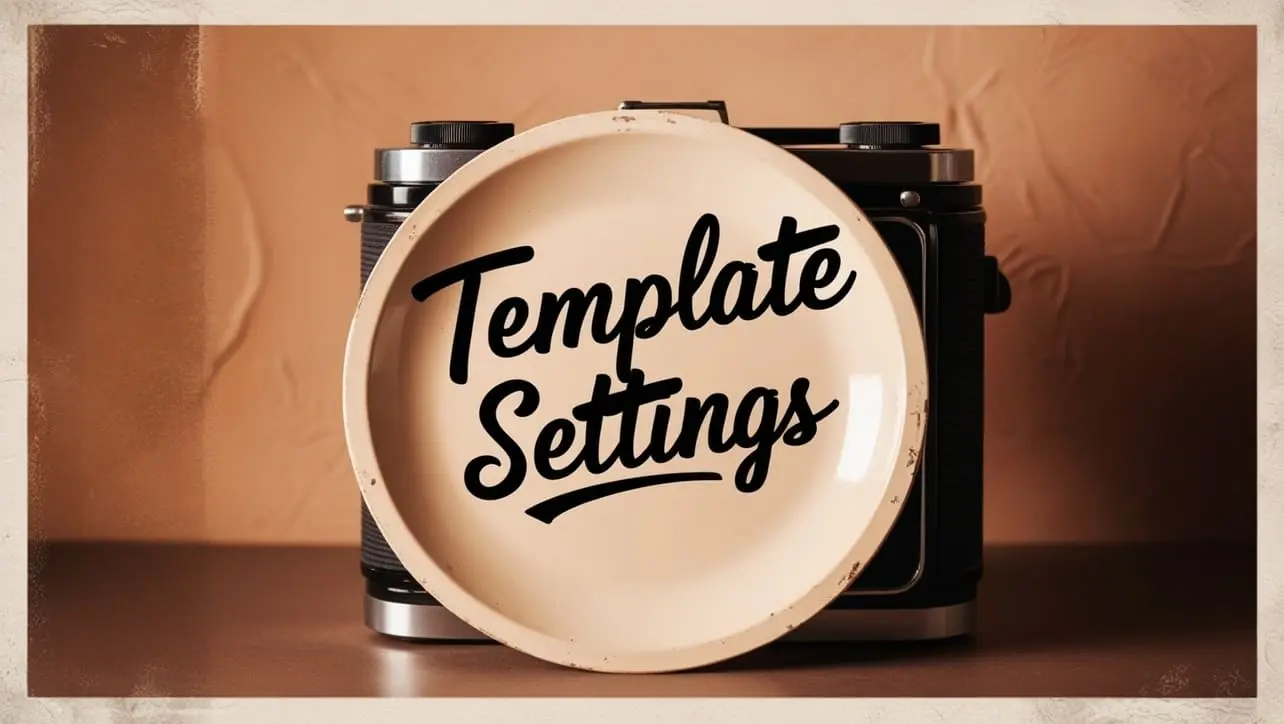
Lodash _.templateSettings Property
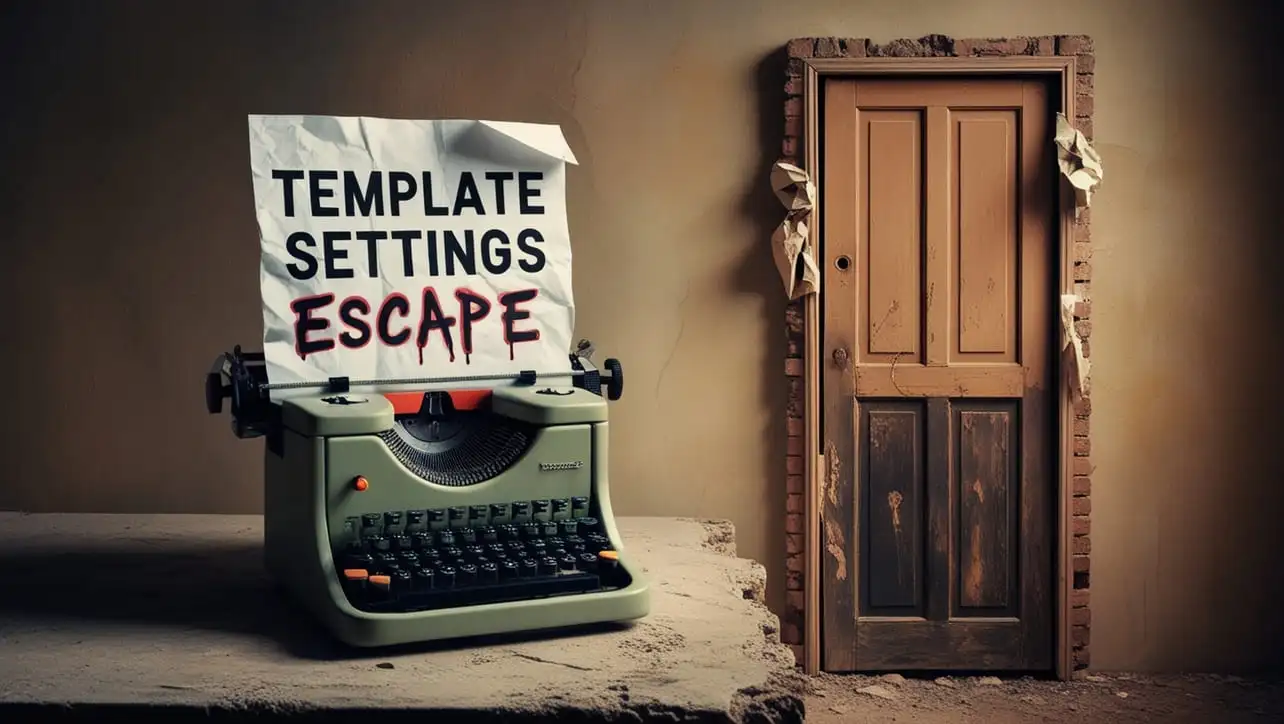
Lodash _.templateSettings.escape Property
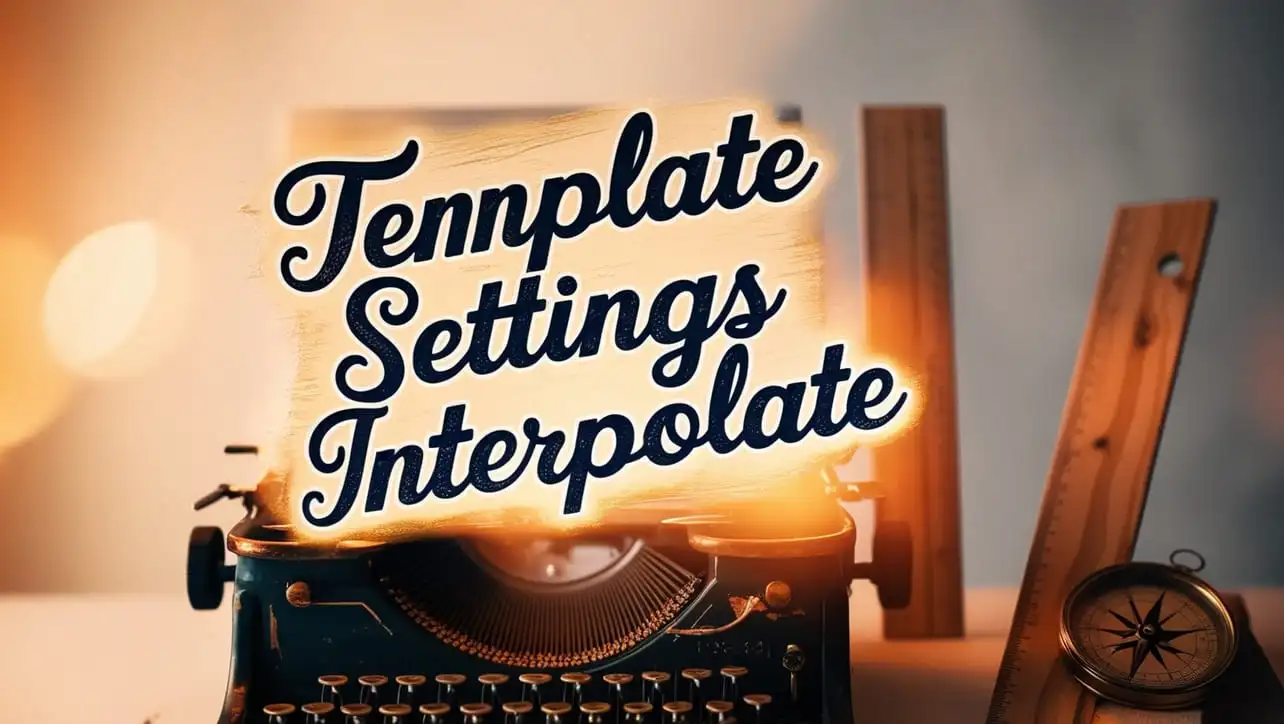
Lodash _.templateSettings.interpolate Property
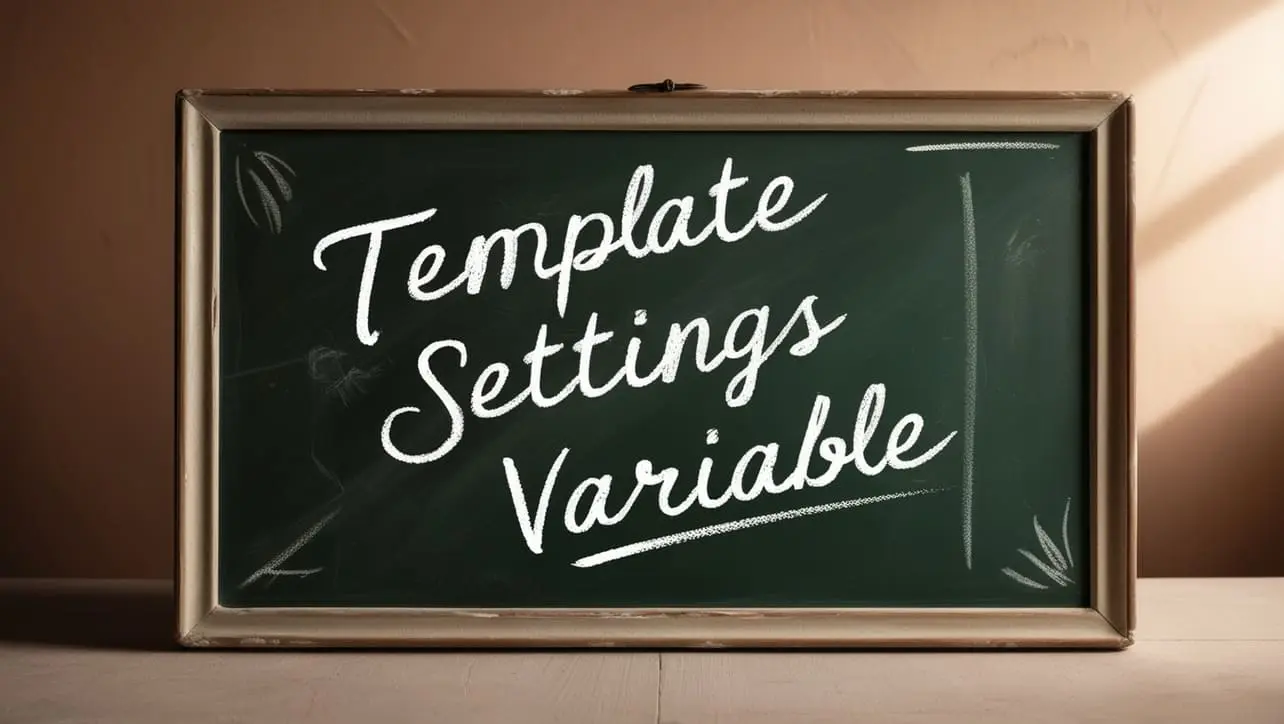
If you have any doubts regarding this article (Lodash _.last() Array Method), please comment here. I will help you immediately.