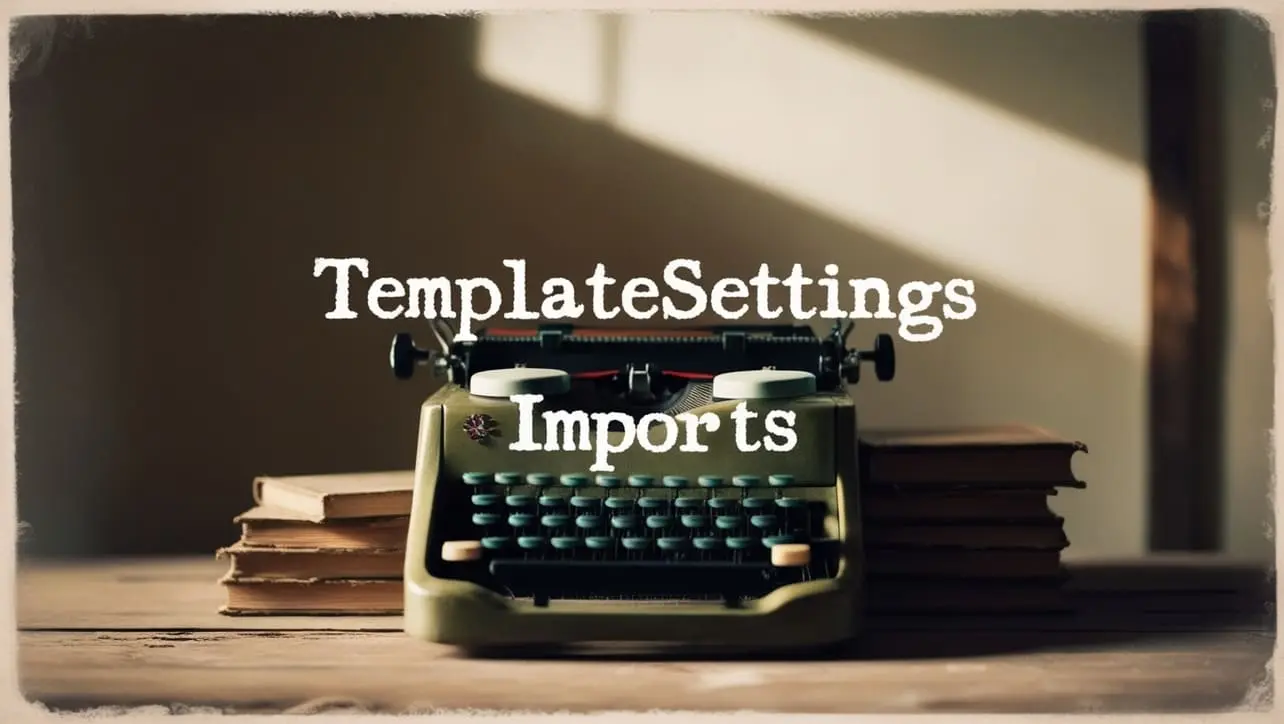
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.join() Array Method
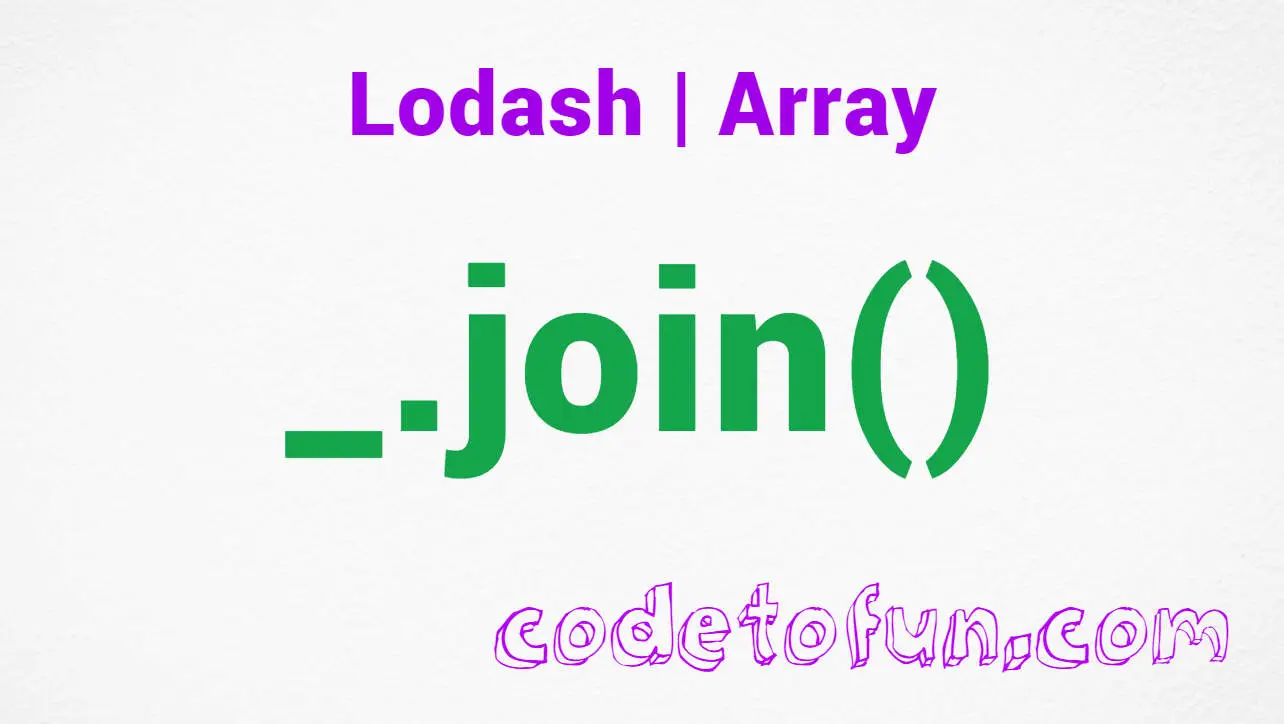
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays often require transformation into strings for various purposes, such as displaying information or constructing query parameters.
Lodash provides a convenient method, _.join()
, to achieve this seamlessly. This method allows you to join the elements of an array into a single string using a specified separator.
🧠 Understanding _.join()
The _.join()
method in Lodash simplifies the process of creating a string from the elements of an array. It takes an array and a separator as parameters, returning a string with the array elements joined together.
💡 Syntax
_.join(array, [separator=','])
- array: The array to process.
- separator: The string used to separate array elements in the resulting string. The default is ','.
📝 Example
Let's explore a practical example to illustrate the use of _.join()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const arrayToJoin = ['apple', 'orange', 'banana'];
const joinedString = _.join(arrayToJoin, ' | ');
console.log(joinedString);
// Output: 'apple | orange | banana'
In this example, the arrayToJoin elements are joined into a string using the specified separator (' | ').
🏆 Best Practices
Validate Inputs:
Before using
_.join()
, ensure that the input array is valid. Handle cases where the array may be empty or contain non-string elements.validate-inputs.jsCopiedconst invalidArray = [1, true, { key: 'value' }]; const separator = ','; if (!Array.isArray(invalidArray) || invalidArray.length === 0) { console.error('Invalid input array'); return; } const validatedJoinedString = _.join(invalidArray, separator); console.log(validatedJoinedString); // Output: '1,true,[object Object]'
Choose Appropriate Separator:
Select a separator that fits your use case. Consider using meaningful separators for better readability.
appropriate-separator.jsCopiedconst numericArray = [1, 2, 3]; const numericSeparator = '-'; const numericJoinedString = _.join(numericArray, numericSeparator); console.log(numericJoinedString); // Output: '1-2-3'
Escaping Special Characters:
If your array elements or separator contain special characters, ensure proper escaping to avoid unexpected results.
escaping-special-characters.jsCopiedconst specialCharsArray = ['Hello', 'World', 'with|separator']; const separatorWithSpecialChars = '|'; const escapedString = _.join(specialCharsArray.map(_.escape), separatorWithSpecialChars); console.log(escapedString); // Output: 'Hello|World|with\|separator'
📚 Use Cases
Displaying Lists:
_.join()
is handy for constructing human-readable lists from arrays, enhancing the presentation of data.displaying-lists.jsCopiedconst shoppingList = ['Milk', 'Bread', 'Eggs']; const formattedList = _.join(shoppingList, ', '); console.log(`Shopping List: ${formattedList}`); // Output: 'Shopping List: Milk, Bread, Eggs'
Query Parameters:
When constructing URLs or query parameters,
_.join()
aids in creating well-formed strings.query-parameters.jsCopiedconst queryParams = { page: 1, limit: 10, filter: 'recent' }; const queryString = _.join(Object.entries(queryParams).map(pair => pair.join('=')), '&'); console.log(`Query String: ${queryString}`); // Output: 'page=1&limit=10&filter=recent'
Custom Formatting:
For scenarios where custom formatting of array elements is required,
_.join()
can be combined with other Lodash methods.custom-formatting.jsCopiedconst prices = [10.5, 20.75, 5]; const formattedPrices = _.join(prices.map(price => `$${price.toFixed(2)}`), ' | '); console.log(formattedPrices); // Output: '$10.50 | $20.75 | $5.00'
🎉 Conclusion
The _.join()
method in Lodash provides a simple and effective way to transform arrays into strings. Whether you're constructing display elements, query parameters, or custom-formatted strings, _.join()
proves to be a valuable tool for JavaScript developers.
Explore the versatility of _.join()
and elevate the way you handle arrays in your JavaScript projects!
👨💻 Join our Community:
Author
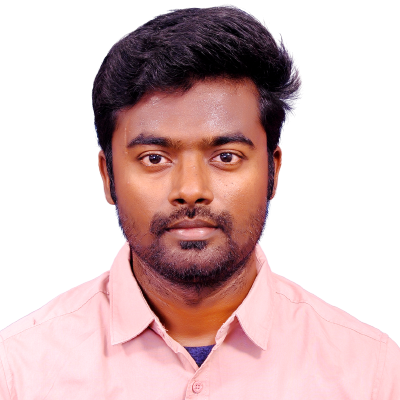
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
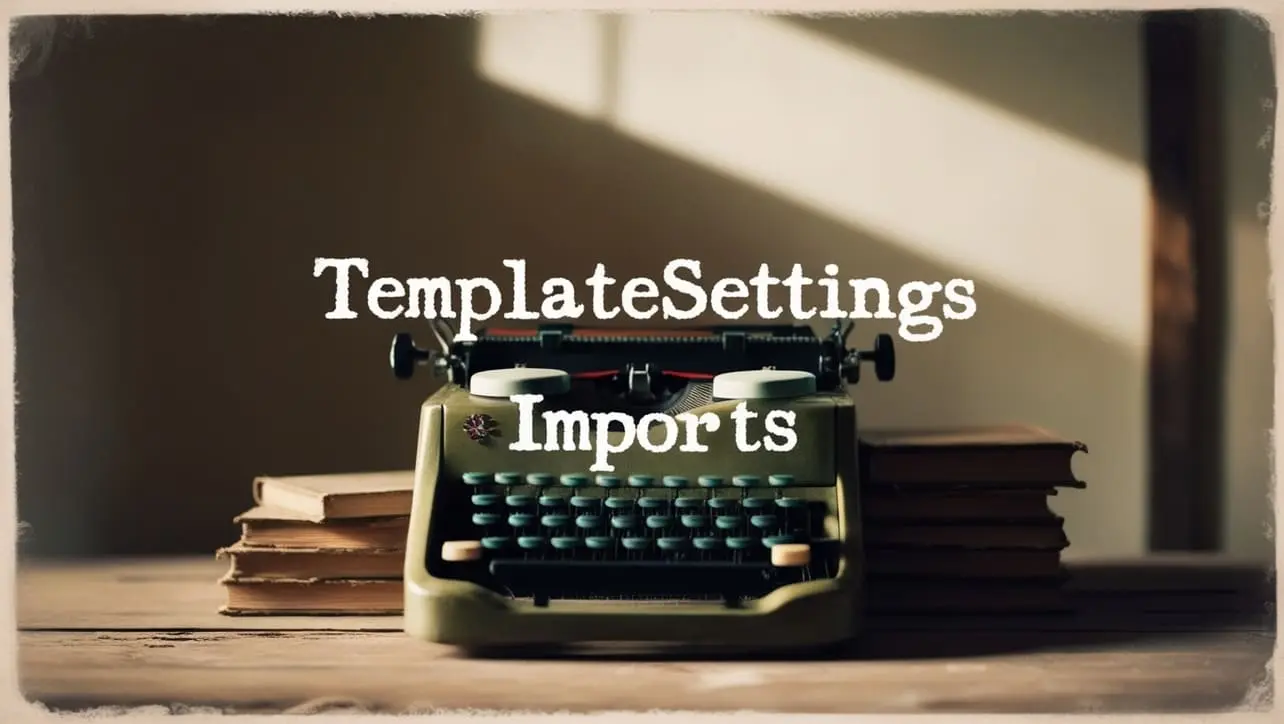
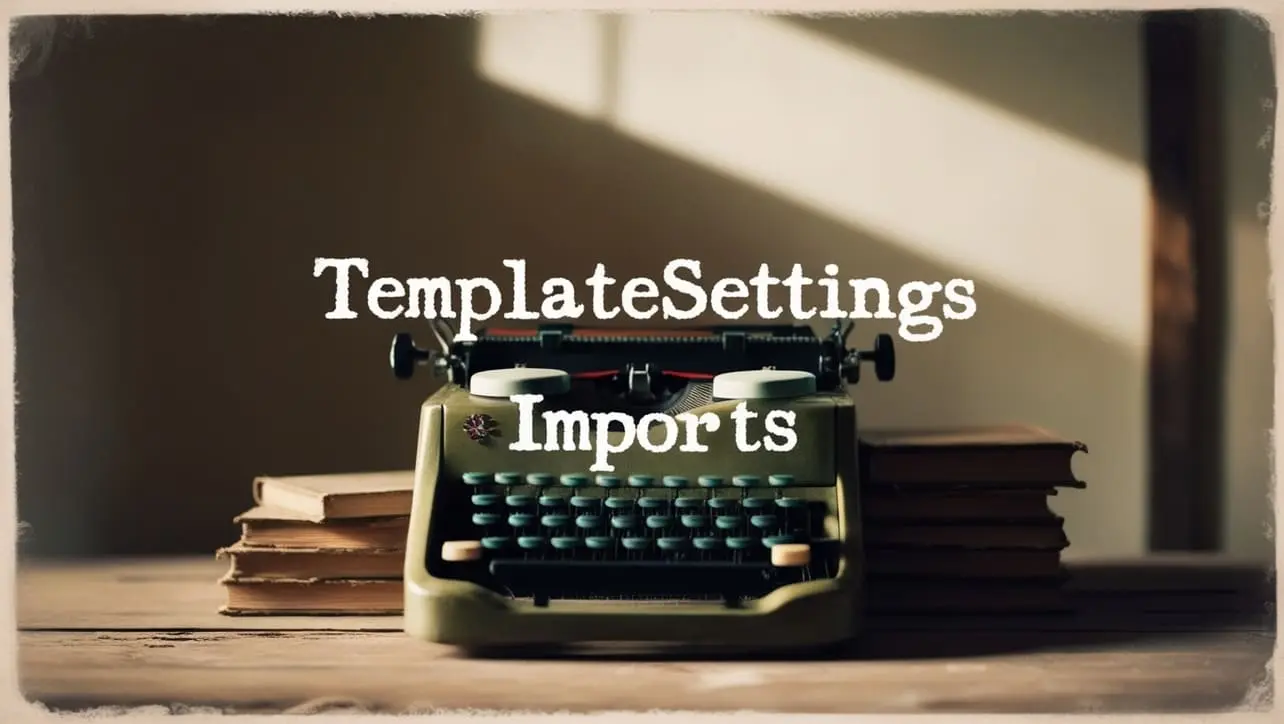
Lodash _.templateSettings.imports Property
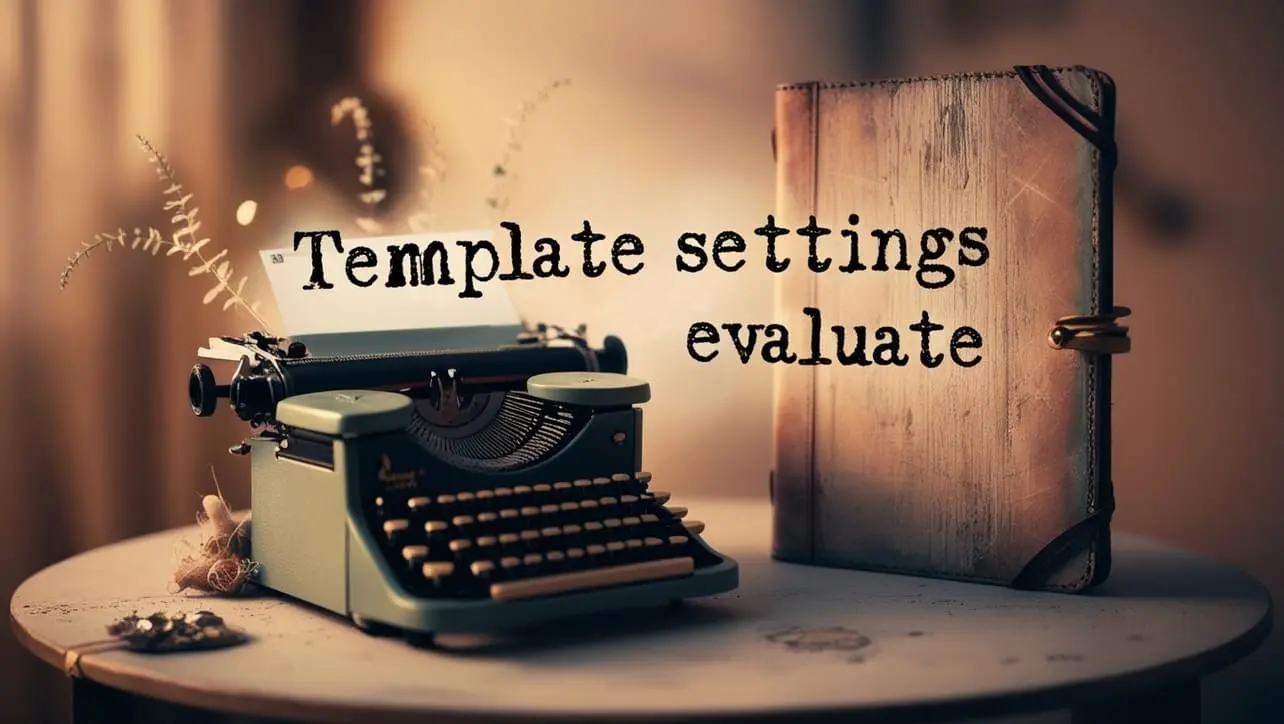
Lodash _.templateSettings.evaluate Property
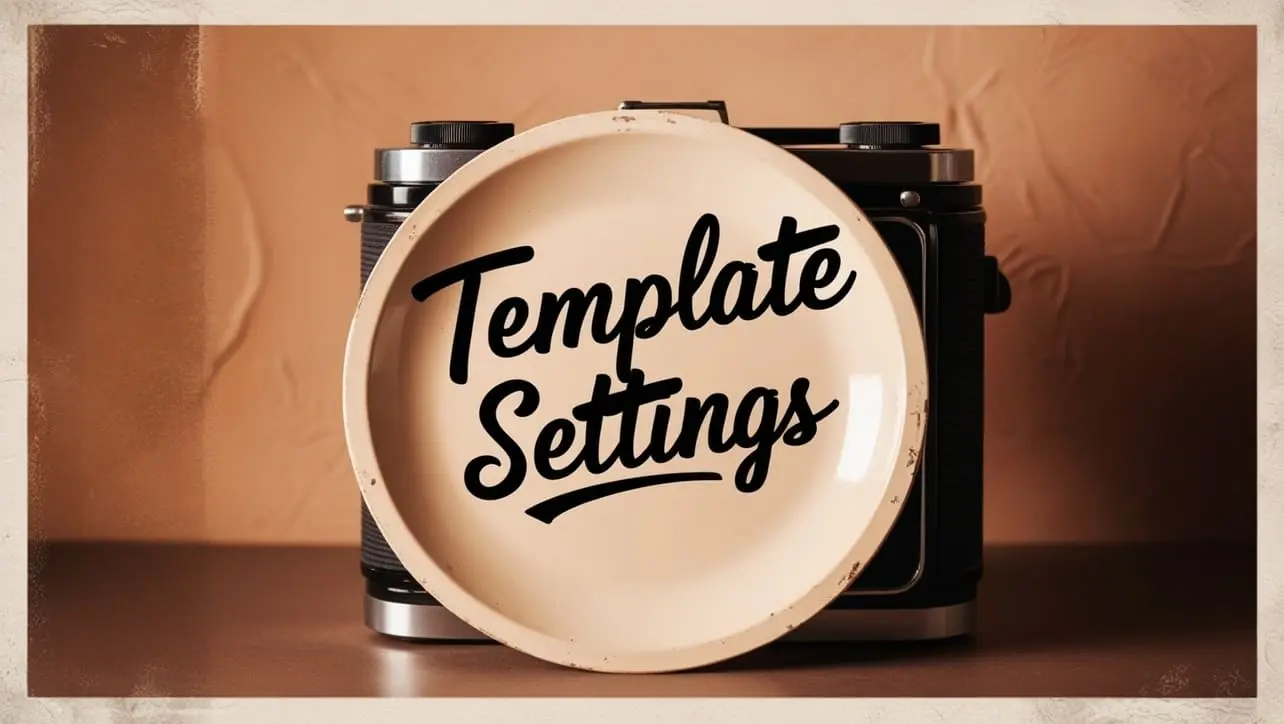
Lodash _.templateSettings Property
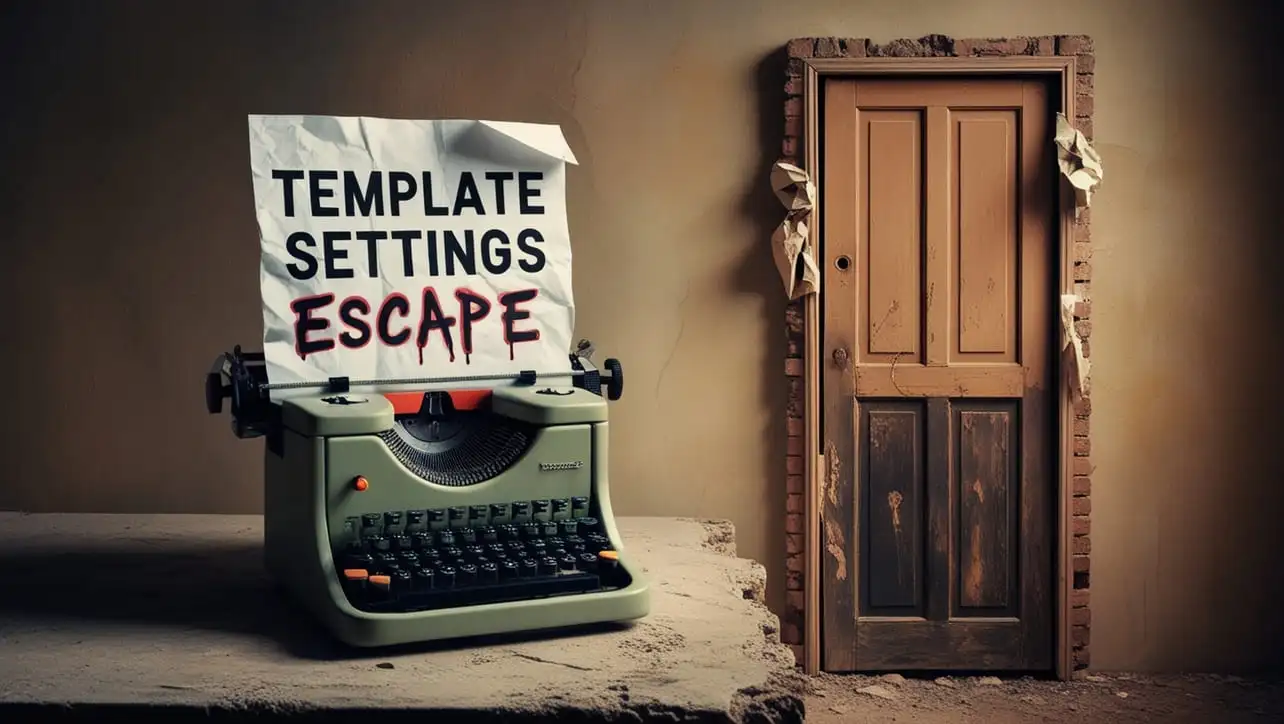
Lodash _.templateSettings.escape Property
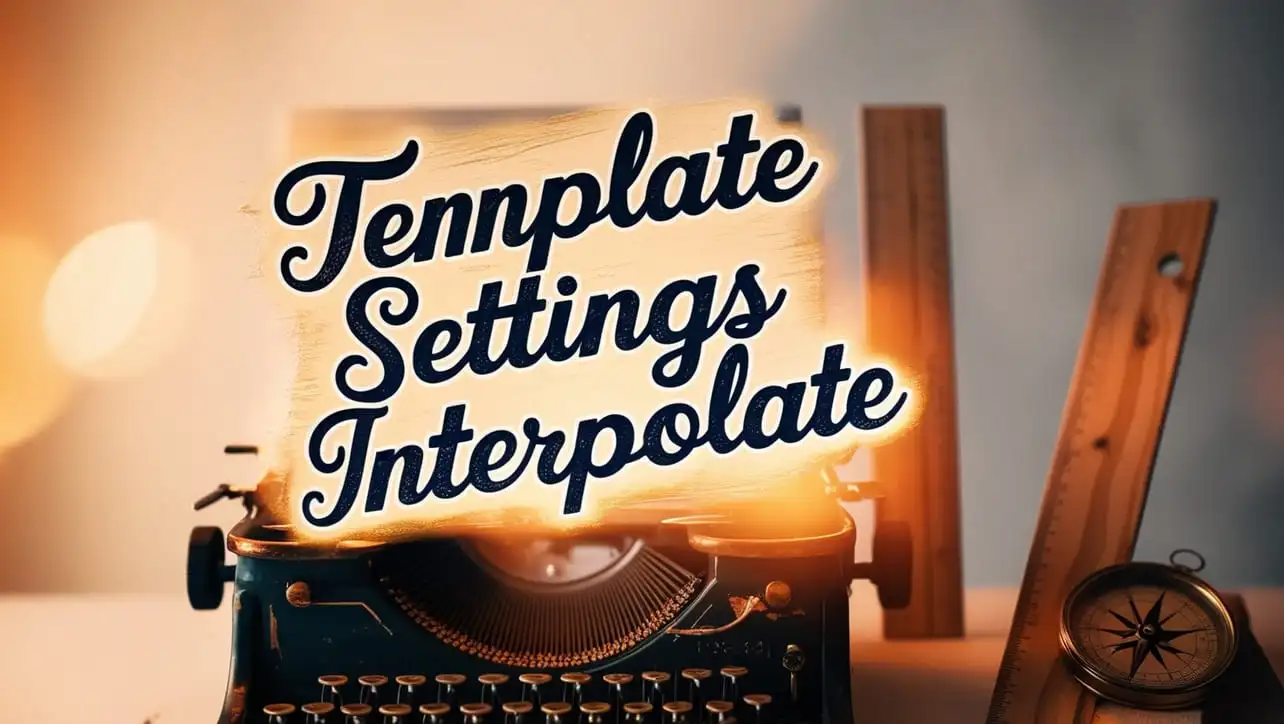
Lodash _.templateSettings.interpolate Property
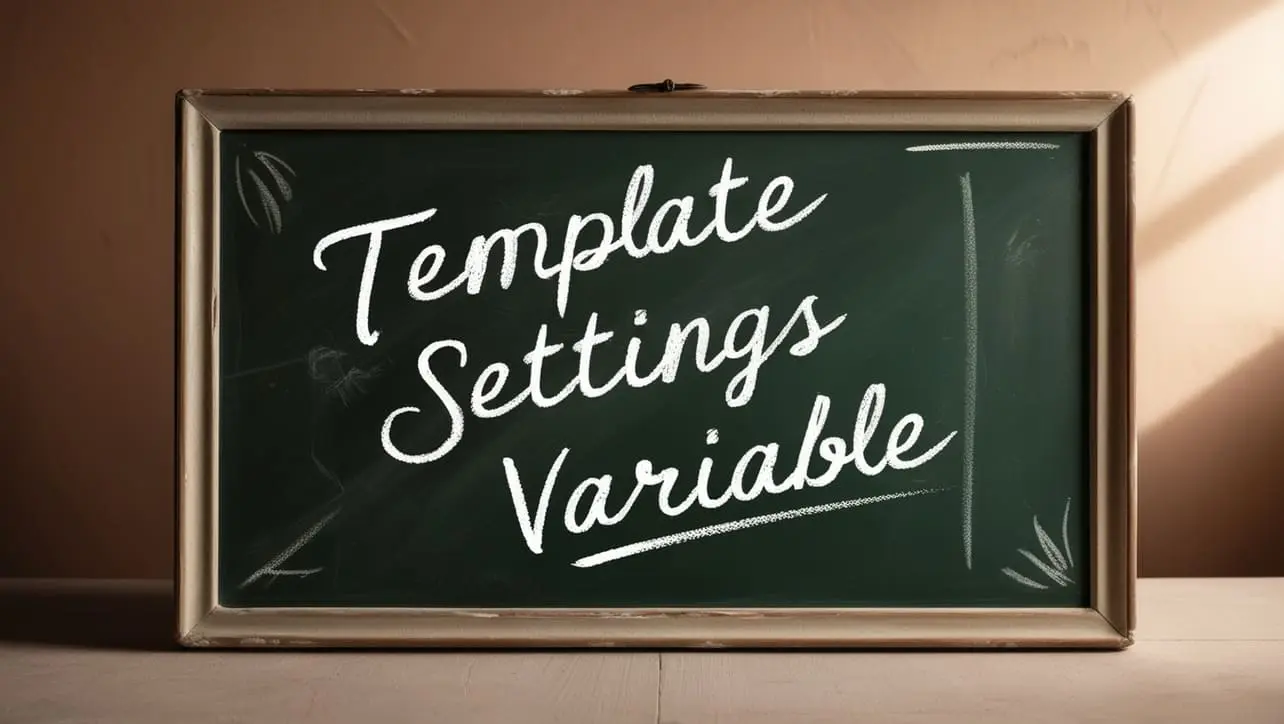
If you have any doubts regarding this article (Lodash _.join() Array Method), please comment here. I will help you immediately.