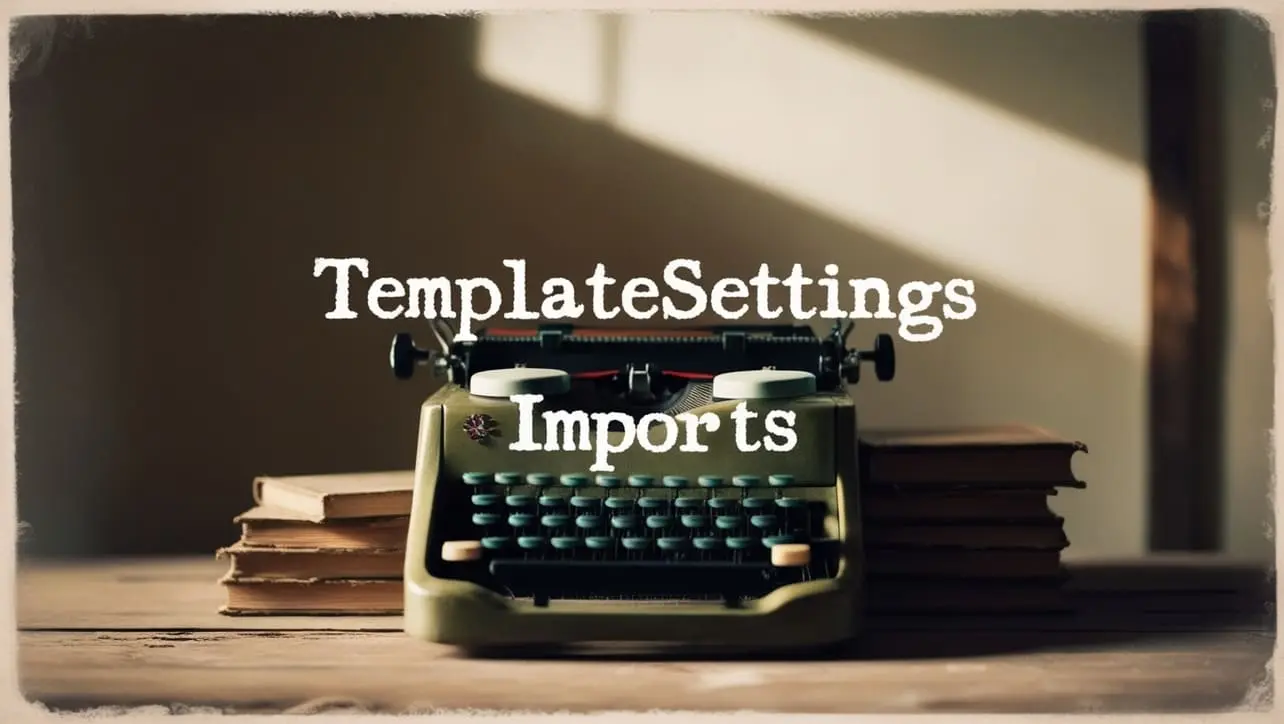
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.initial() Array Method
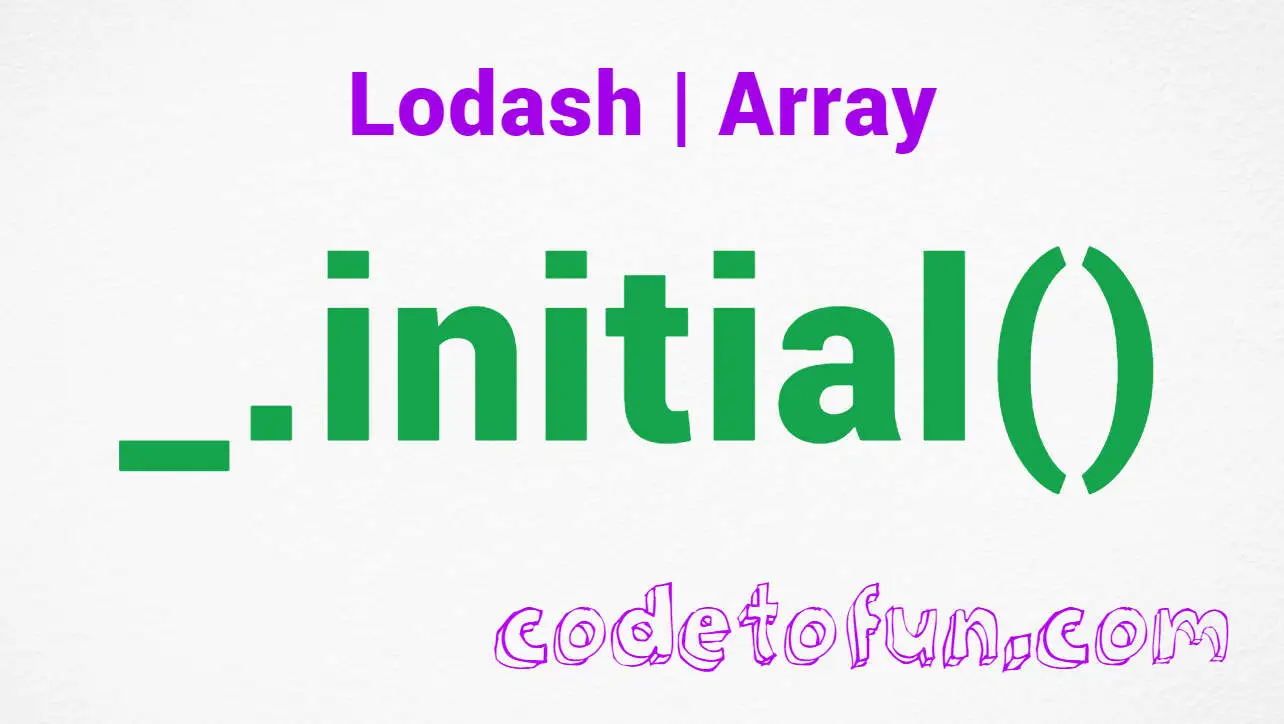
Photo Credit to CodeToFun
🙋 Introduction
When working with arrays in JavaScript, there are situations where you need to exclude the last element or elements from an array. This is where the _.initial()
method from the Lodash library comes to the rescue.
The _.initial()
method simplifies the process of obtaining all elements of an array except the last one, providing a clean and efficient solution for developers.
🧠 Understanding _.initial()
The _.initial()
method in Lodash allows you to retrieve all elements of an array except the last one. This can be particularly useful when dealing with arrays where the last element is not needed or should be excluded from further processing.
💡 Syntax
_.initial(array)
- array: The array from which to exclude the last element.
📝 Example
Let's dive into a practical example to illustrate the power of _.initial()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5];
const arrayWithInitial = _.initial(originalArray);
console.log(arrayWithInitial);
// Output: [1, 2, 3, 4]
In this example, the originalArray is processed using _.initial()
, resulting in a new array excluding the last element.
🏆 Best Practices
Validate Inputs:
Before using
_.initial()
, ensure that the input array is valid and contains elements. Avoid calling_.initial()
on an empty array to prevent unexpected behavior.validate-inputs.jsCopiedif (!Array.isArray(originalArray) || originalArray.length === 0) { console.error('Invalid input array'); return; } const arrayWithInitial = _.initial(originalArray); console.log(arrayWithInitial);
Handle Edge Cases:
Consider edge cases, such as an array with only one element. In such cases,
_.initial()
will return an empty array.handle-edge-cases.jsCopiedconst singleElementArray = [42]; const resultArray = _.initial(singleElementArray); // Returns: [] console.log(resultArray);
📚 Use Cases
Removing Last Element:
The primary use case for
_.initial()
is to exclude the last element from an array when it is not needed.removing-last-element.jsCopiedconst dataToProcess = /* ...retrieve data from a source... */; const processedData = _.initial(dataToProcess); console.log(processedData);
Building New Arrays:
When constructing new arrays based on existing ones,
_.initial()
can be employed to exclude the last element and create a modified array.building-new-arrays.jsCopiedconst originalData = [/* ...some data... */]; const modifiedData = _.initial(originalData).map(/* ...apply transformations... */); console.log(modifiedData);
🎉 Conclusion
The _.initial()
method in Lodash offers a convenient way to exclude the last element from an array, streamlining array manipulation in your JavaScript projects. By incorporating this method into your code, you can enhance the clarity and efficiency of your array-related operations.
Explore the capabilities of Lodash and unlock the potential of array manipulation with _.initial()
!
👨💻 Join our Community:
Author
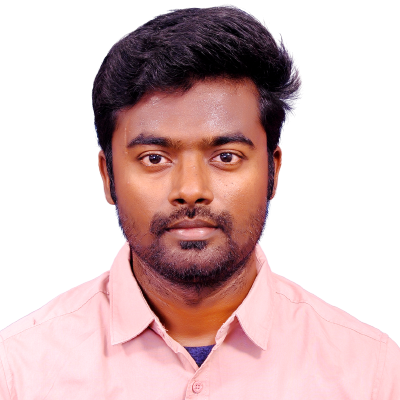
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
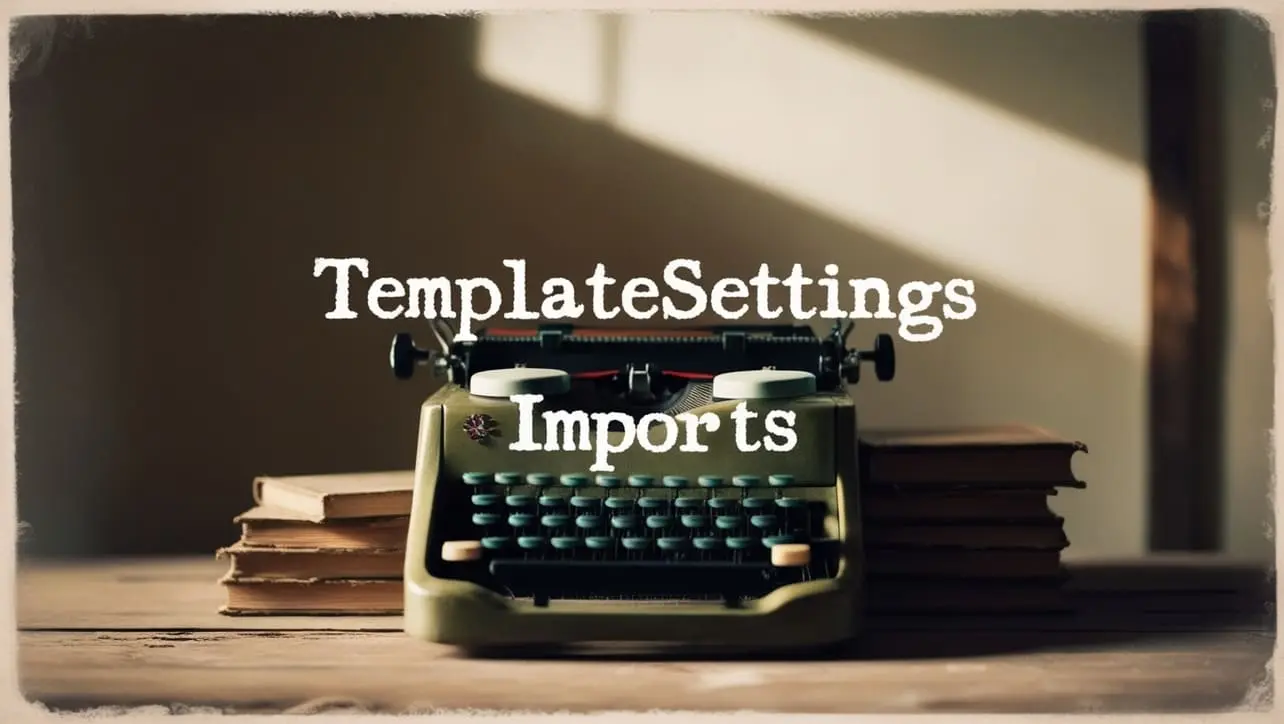
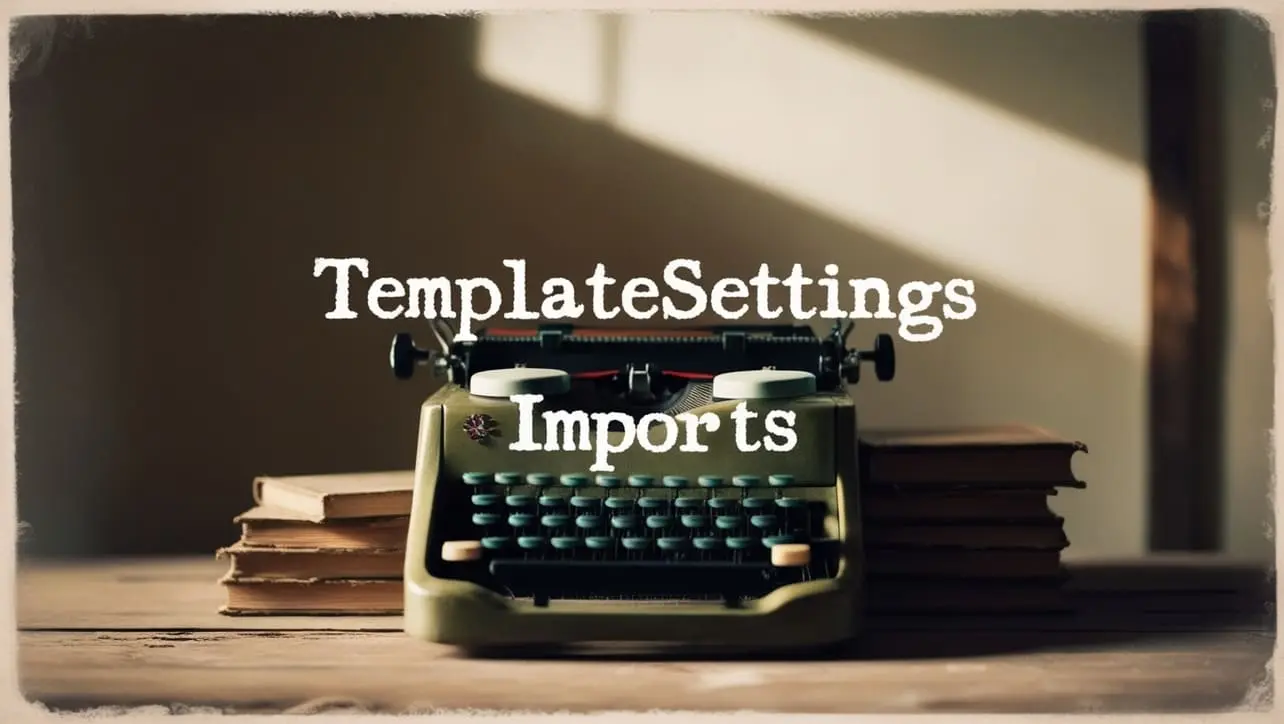
Lodash _.templateSettings.imports Property
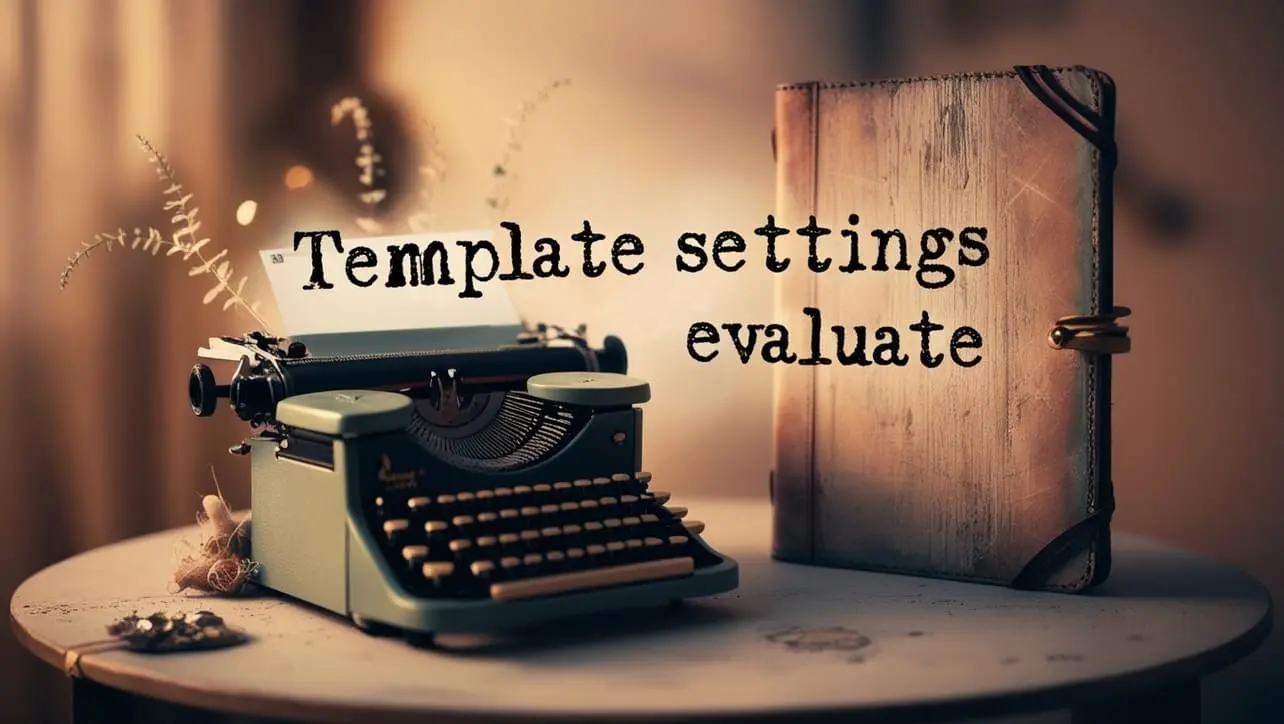
Lodash _.templateSettings.evaluate Property
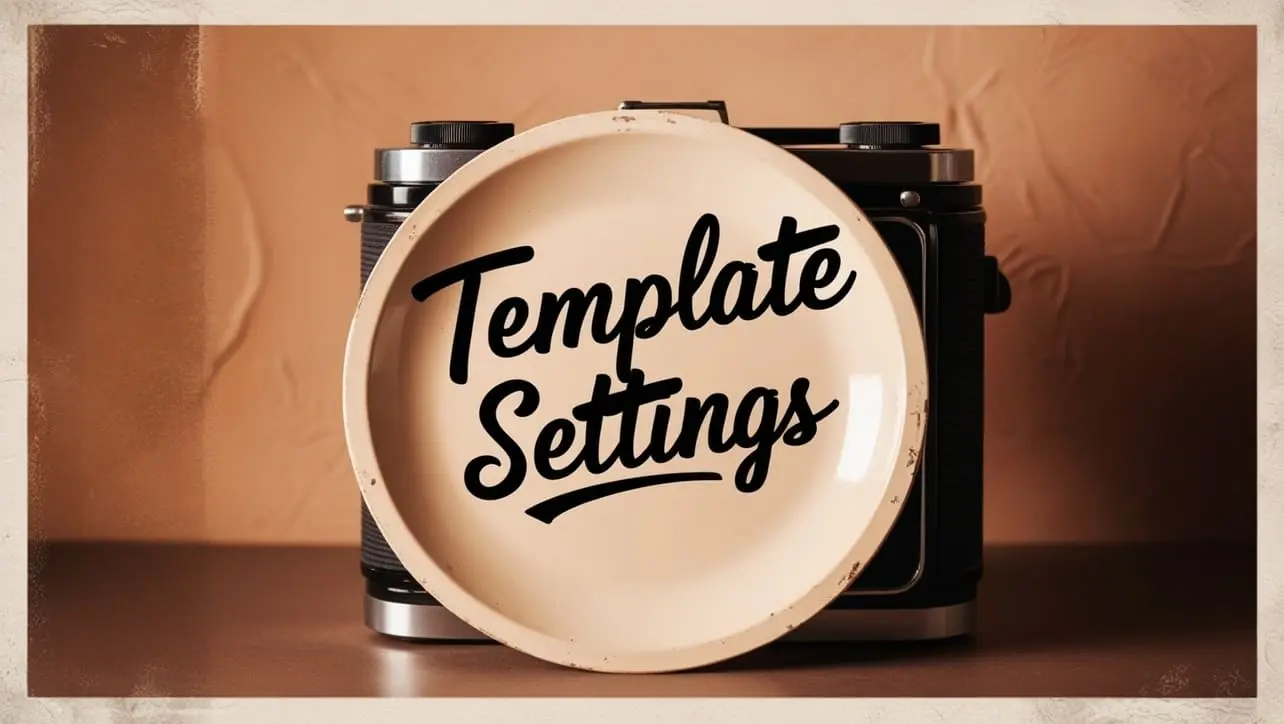
Lodash _.templateSettings Property
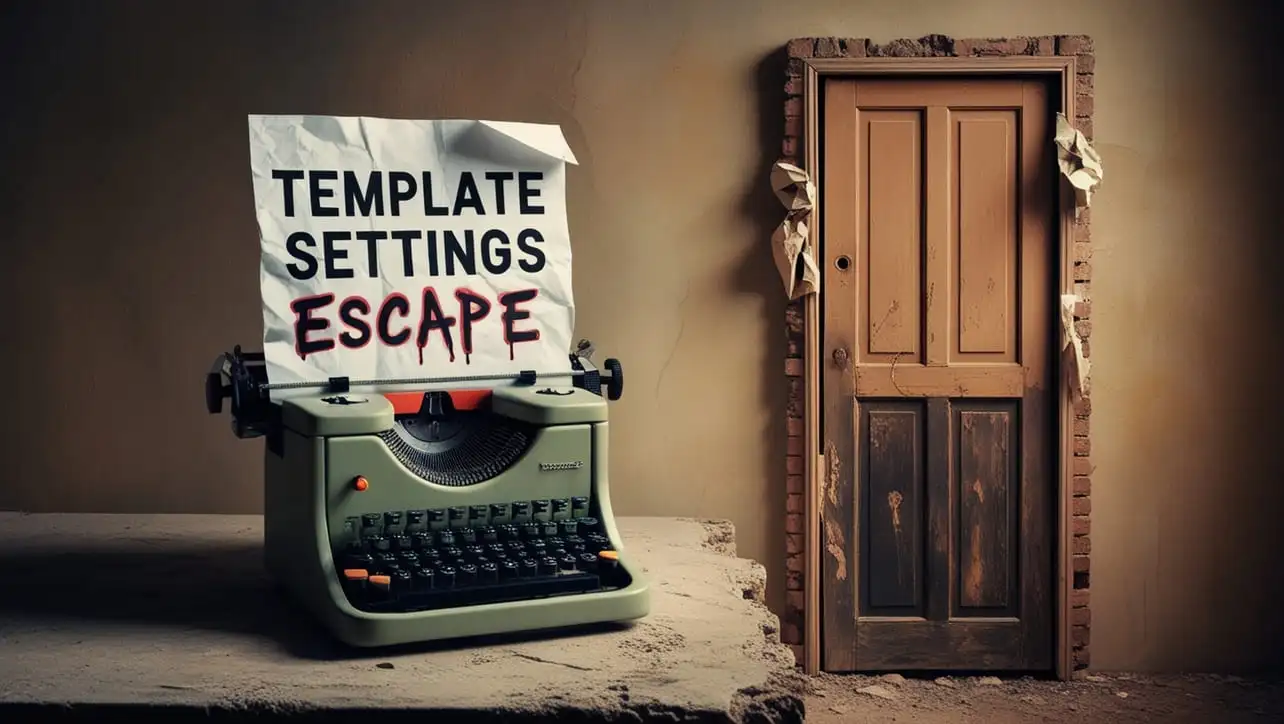
Lodash _.templateSettings.escape Property
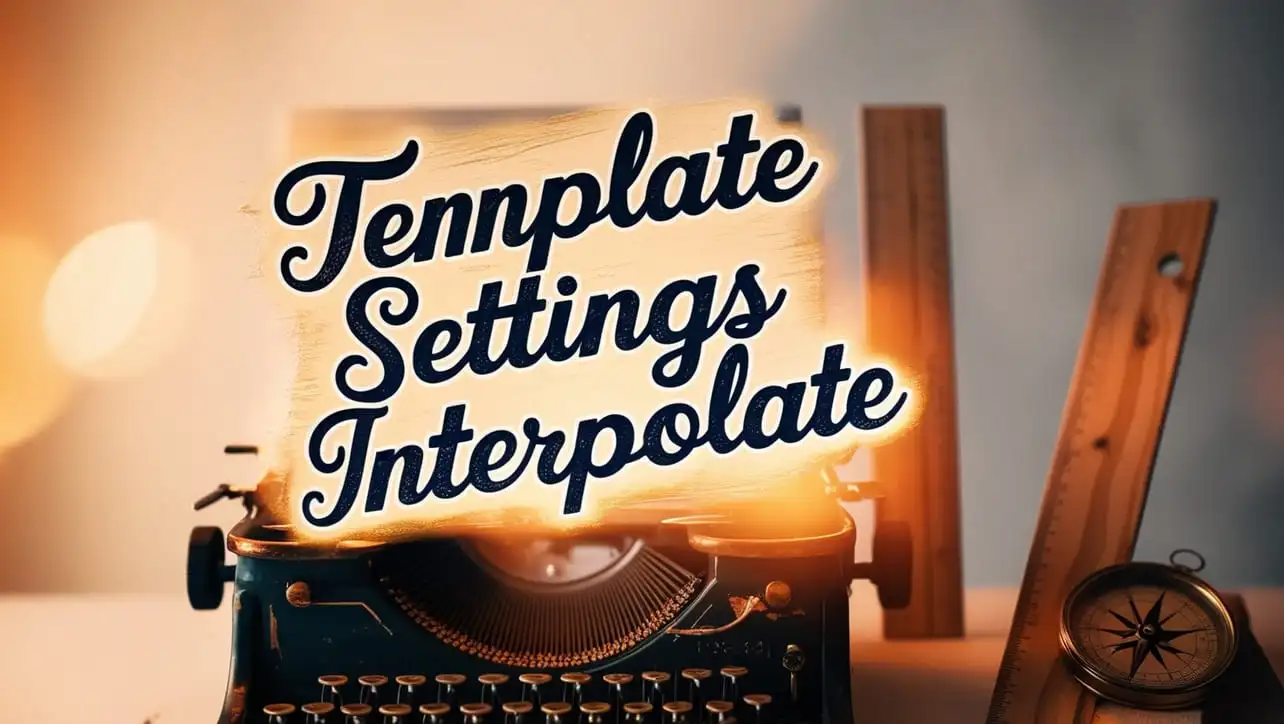
Lodash _.templateSettings.interpolate Property
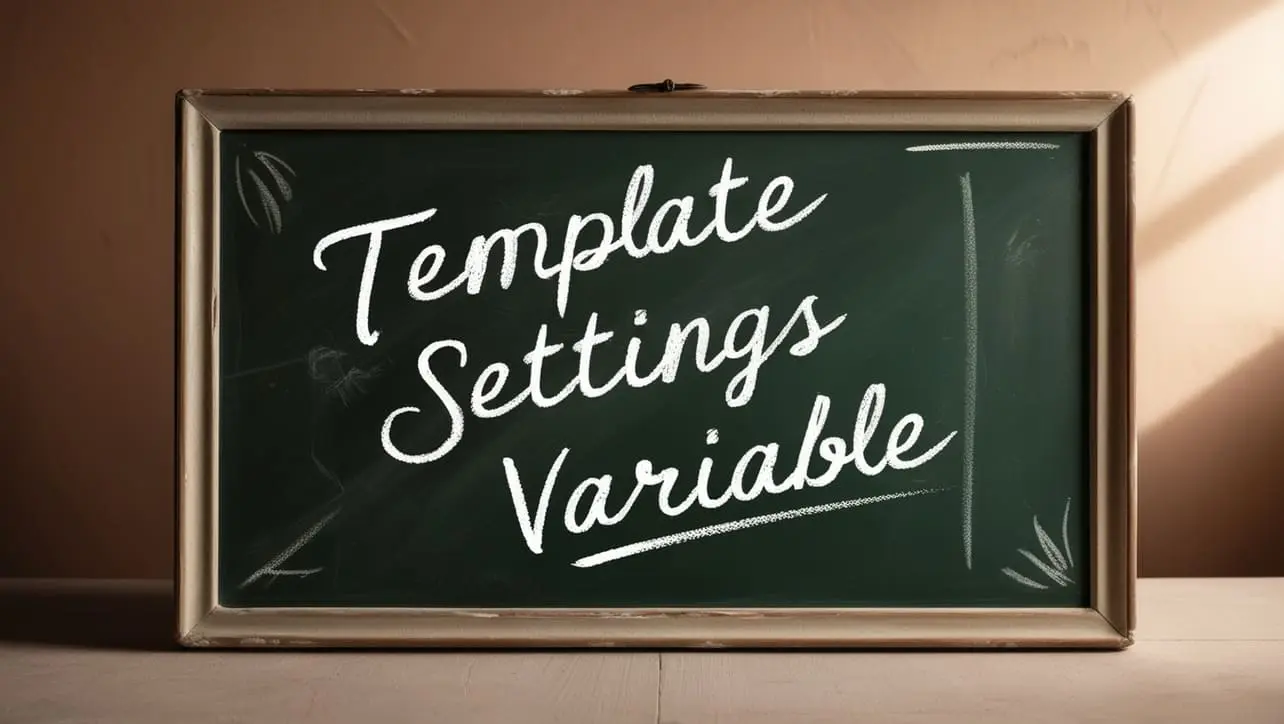
If you have any doubts regarding this article (Lodash _.initial() Array Method), please comment here. I will help you immediately.