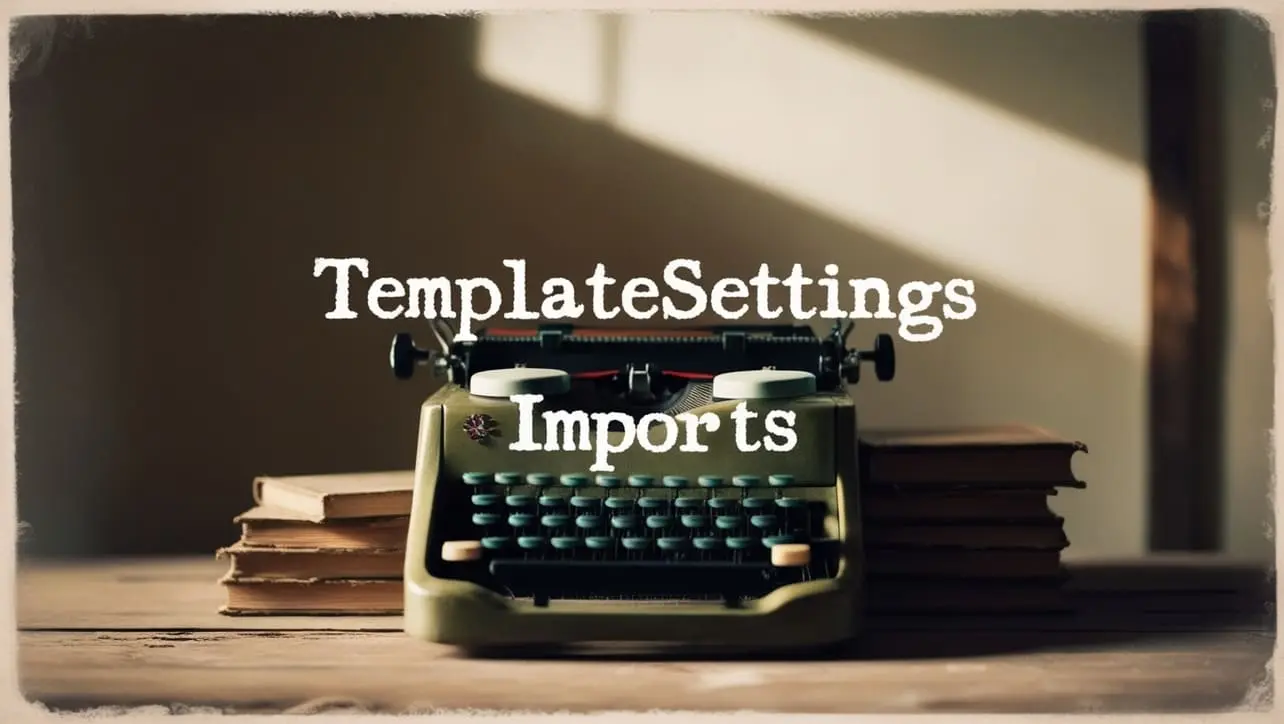
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.findIndex() Array Method
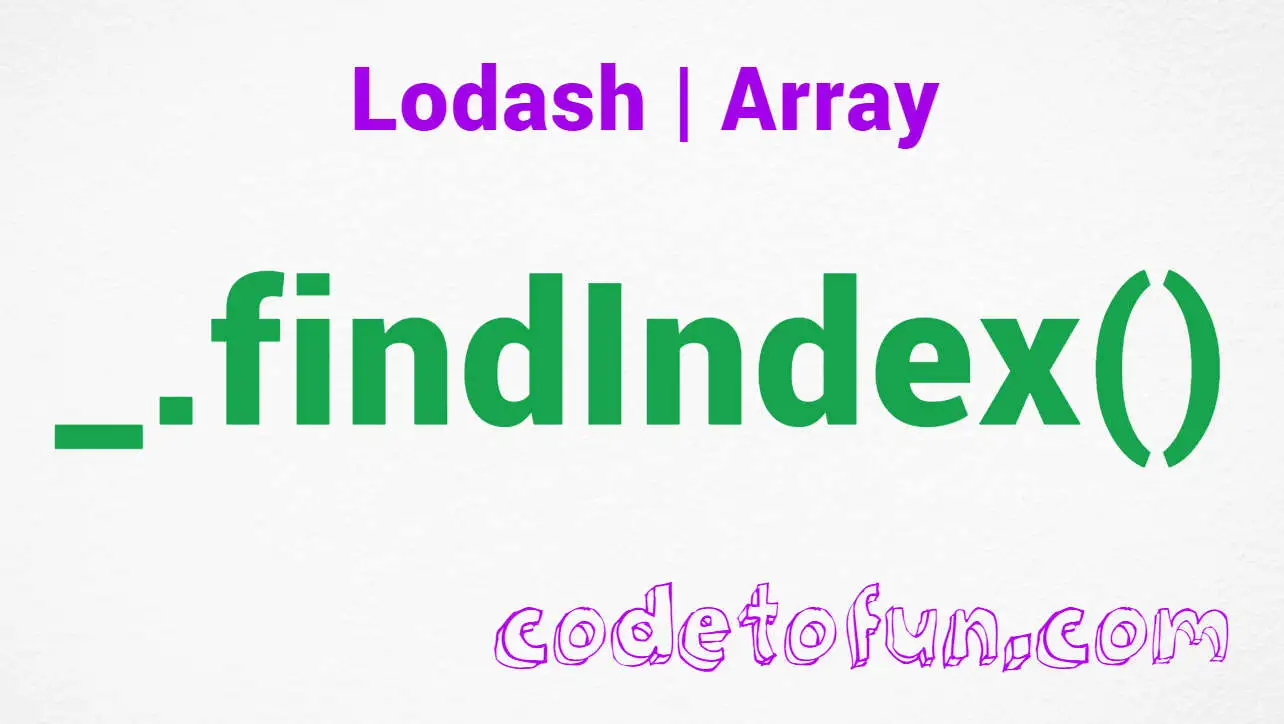
Photo Credit to CodeToFun
🙋 Introduction
Efficiently searching for elements within an array is a common task in JavaScript development. Lodash, a popular utility library, provides a powerful tool for this with the _.findIndex()
method. This method allows developers to locate the index of the first element in an array that satisfies a given condition.
Let's explore the details of this method and how it can enhance your array manipulation.
🧠 Understanding _.findIndex()
The _.findIndex()
method in Lodash is designed to find the index of the first element in an array that satisfies a provided testing function. This method is particularly useful when you need to identify the position of a specific element that meets certain criteria.
💡 Syntax
_.findIndex(array, [predicate=_.identity], [fromIndex=0])
- array: The array to search.
- predicate: The function invoked per iteration.
- fromIndex: The index to start the search (default is 0).
📝 Example
Let's delve into a practical example to illustrate the functionality of _.findIndex()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' },
{ id: 4, name: 'David' }
];
const index = _.findIndex(users, { name: 'Charlie' });
console.log(index);
// Output: 2
In this example, the _.findIndex()
method searches the users array for an object with the property name equal to Charlie, returning its index.
🏆 Best Practices
Validate Inputs:
Before using
_.findIndex()
, ensure that the input array is valid and contains elements. Additionally, verify the predicate function to avoid unexpected results.validate-inputs.jsCopiedif (!Array.isArray(users) || users.length === 0) { console.error('Invalid input array'); return; } const predicate = user => user.age > 18; // Example predicate function const index = _.findIndex(users, predicate); console.log(index);
Handle Edge Cases:
Consider edge cases, such as an empty array or a non-existent element. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst emptyArray = []; const nonExistentElement = { name: 'Eve' }; const emptyArrayIndex = _.findIndex(emptyArray, { age: 18 }); // Returns: -1 const nonExistentElementIndex = _.findIndex(users, nonExistentElement); // Returns: -1 console.log(emptyArrayIndex); console.log(nonExistentElementIndex);
Specify Starting Index:
For performance optimization or specific search requirements, utilize the fromIndex parameter to specify the starting index of the search.
specify-starting-index.jsCopiedconst index = _.findIndex(users, { name: 'Charlie' }, 2); // Start searching from index 2 console.log(index);
📚 Use Cases
User Authentication:
In user authentication systems,
_.findIndex()
can be used to check if a username exists in a list of registered users.user-authentication.jsCopiedconst registeredUsers = /* ...fetch users from database or elsewhere... */; const usernameToCheck = 'Alice'; const userIndex = _.findIndex(registeredUsers, { username: usernameToCheck }); if (userIndex !== -1) { console.log(`${usernameToCheck} exists in the database.`); } else { console.log(`${usernameToCheck} does not exist.`); }
Data Filtering:
When working with datasets,
_.findIndex()
can assist in filtering out specific data points based on given criteria.data-filtering.jsCopiedconst dataSet = /* ...fetch data from API or elsewhere... */; const thresholdValue = 100; const firstIndexAboveThreshold = _.findIndex(dataSet, value => value > thresholdValue); console.log(firstIndexAboveThreshold);
Conditional Rendering in UI:
In user interfaces, you can use
_.findIndex()
to conditionally render components based on the presence of specific data.conditional-rendering-in-ui.jsCopiedconst renderUserProfile = () => { const indexOfCurrentUser = _.findIndex(users, { id: getCurrentUserId() }); if (indexOfCurrentUser !== -1) { // Render user profile } else { // Display a message or alternative content } };
🎉 Conclusion
The _.findIndex()
method in Lodash is a versatile tool for efficiently searching arrays based on specified criteria. Whether you're working with simple arrays or complex data structures, this method can simplify the process of finding the index of the first matching element. By incorporating _.findIndex()
into your projects, you can enhance the readability and maintainability of your JavaScript code.
Explore the capabilities of Lodash and unlock the potential of array searching with _.findIndex()
!
👨💻 Join our Community:
Author
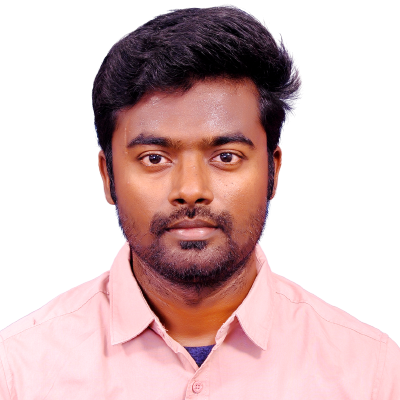
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
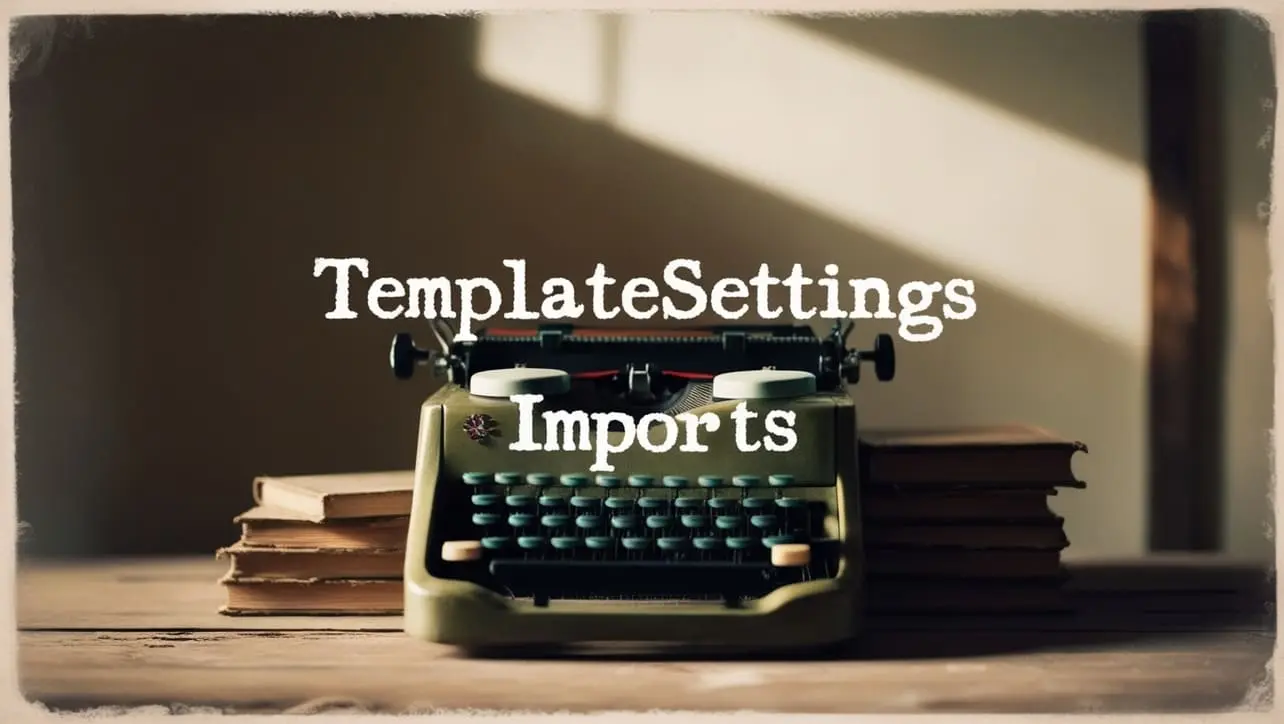
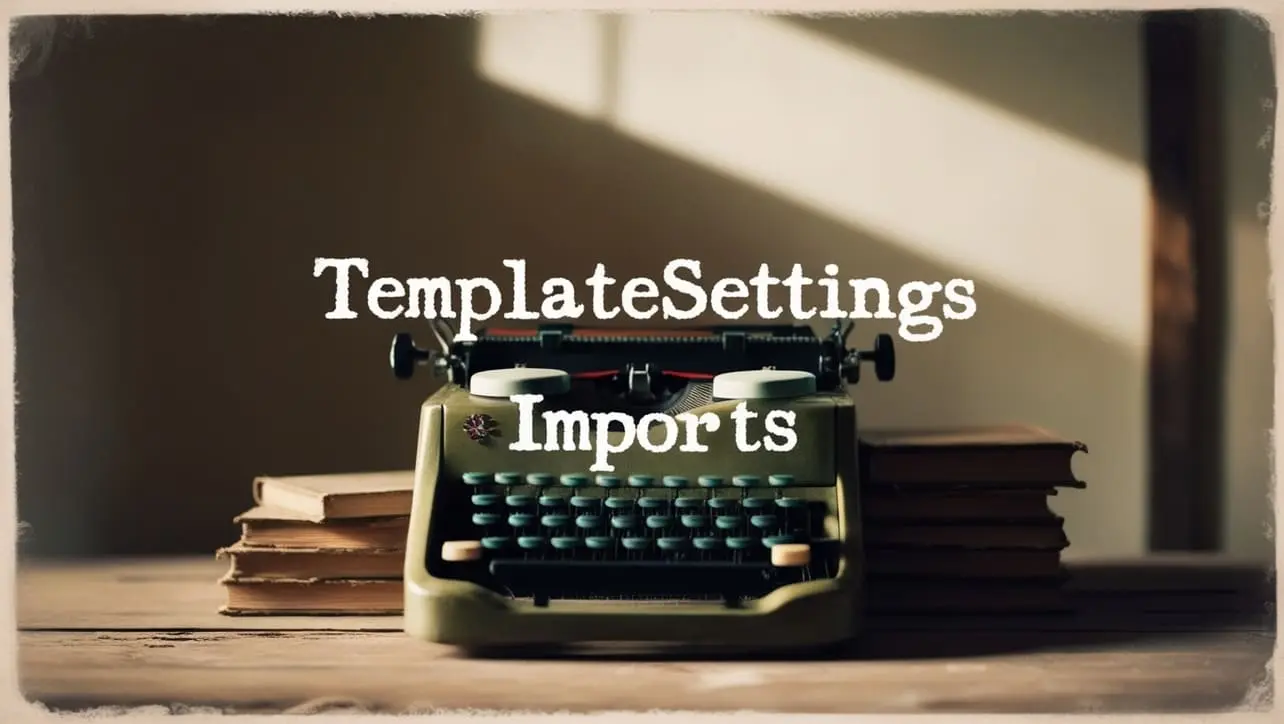
Lodash _.templateSettings.imports Property
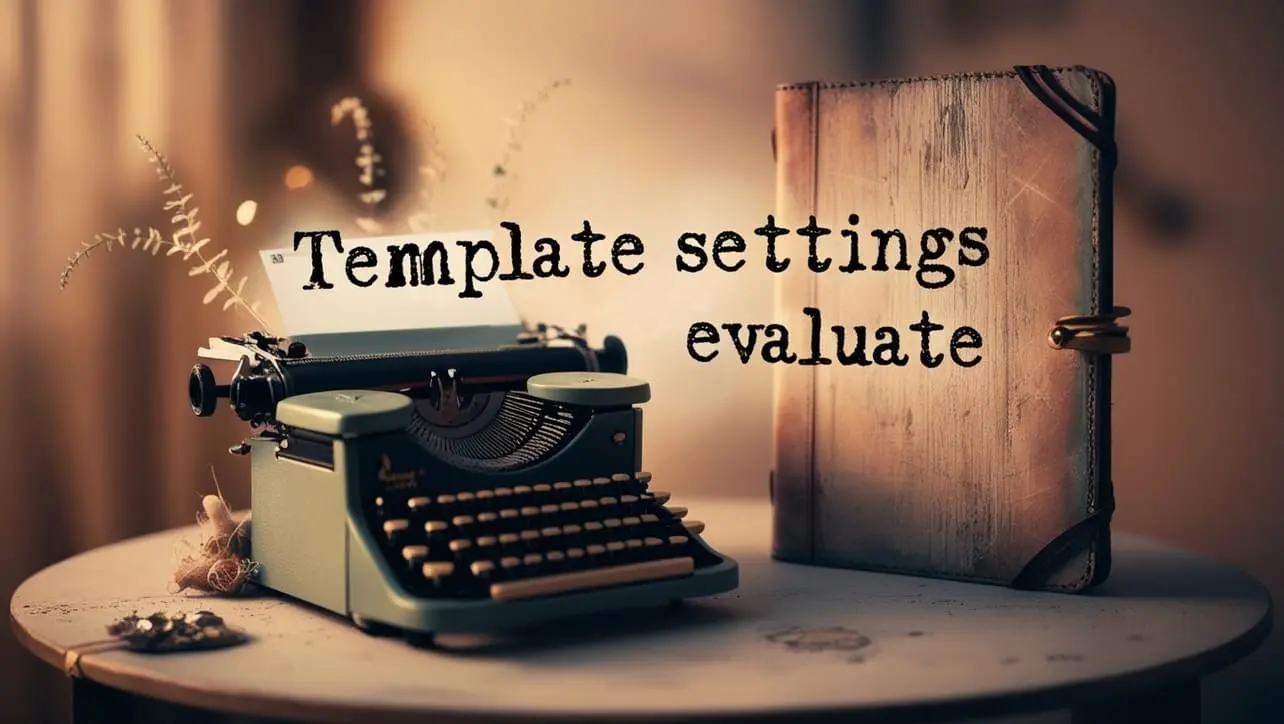
Lodash _.templateSettings.evaluate Property
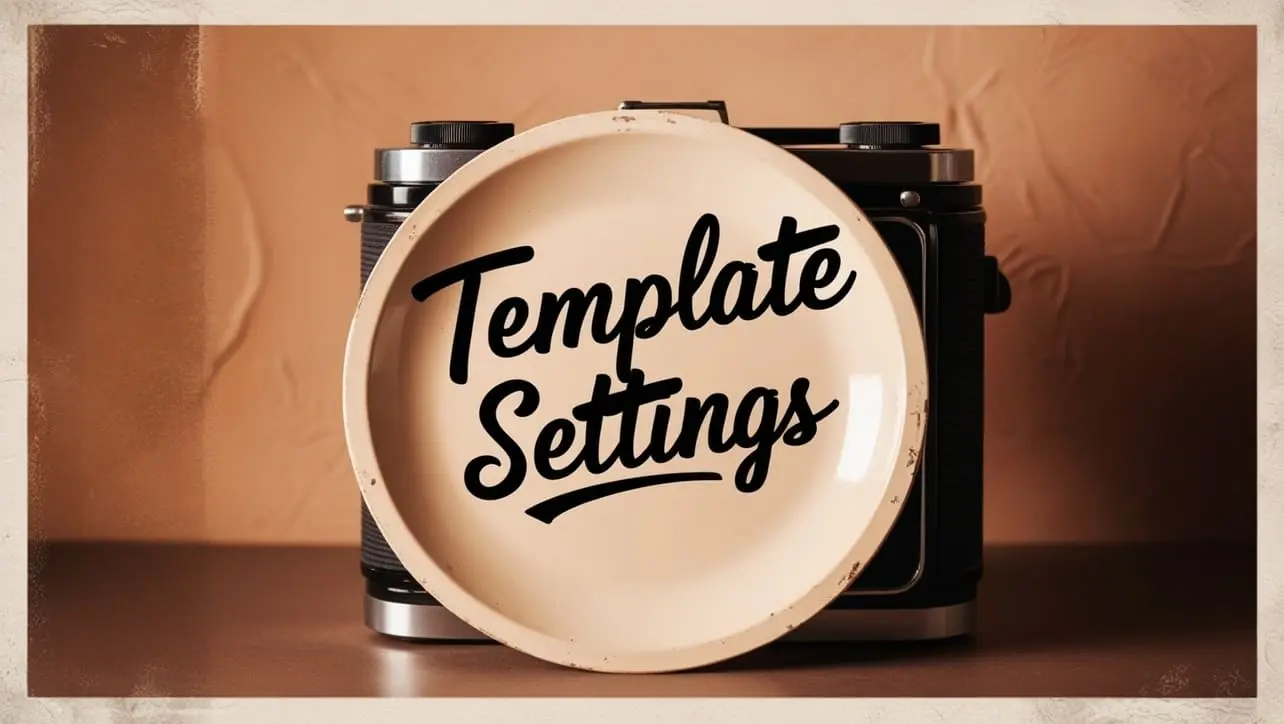
Lodash _.templateSettings Property
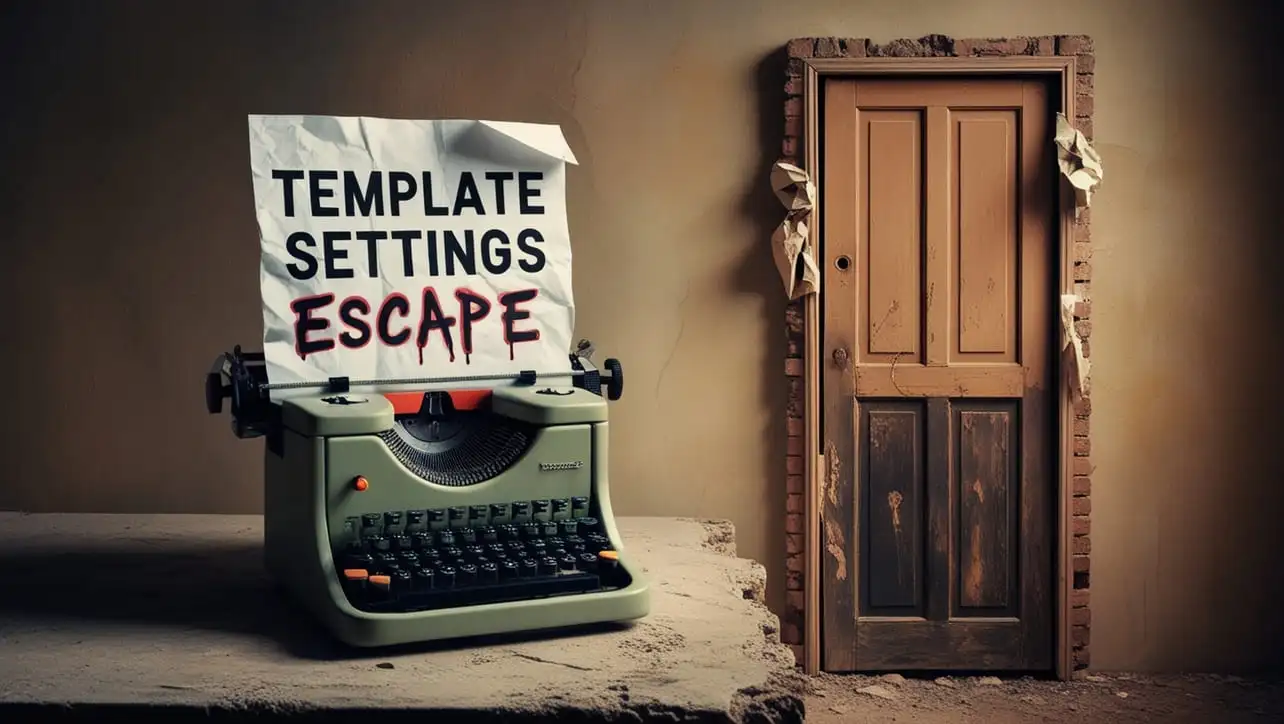
Lodash _.templateSettings.escape Property
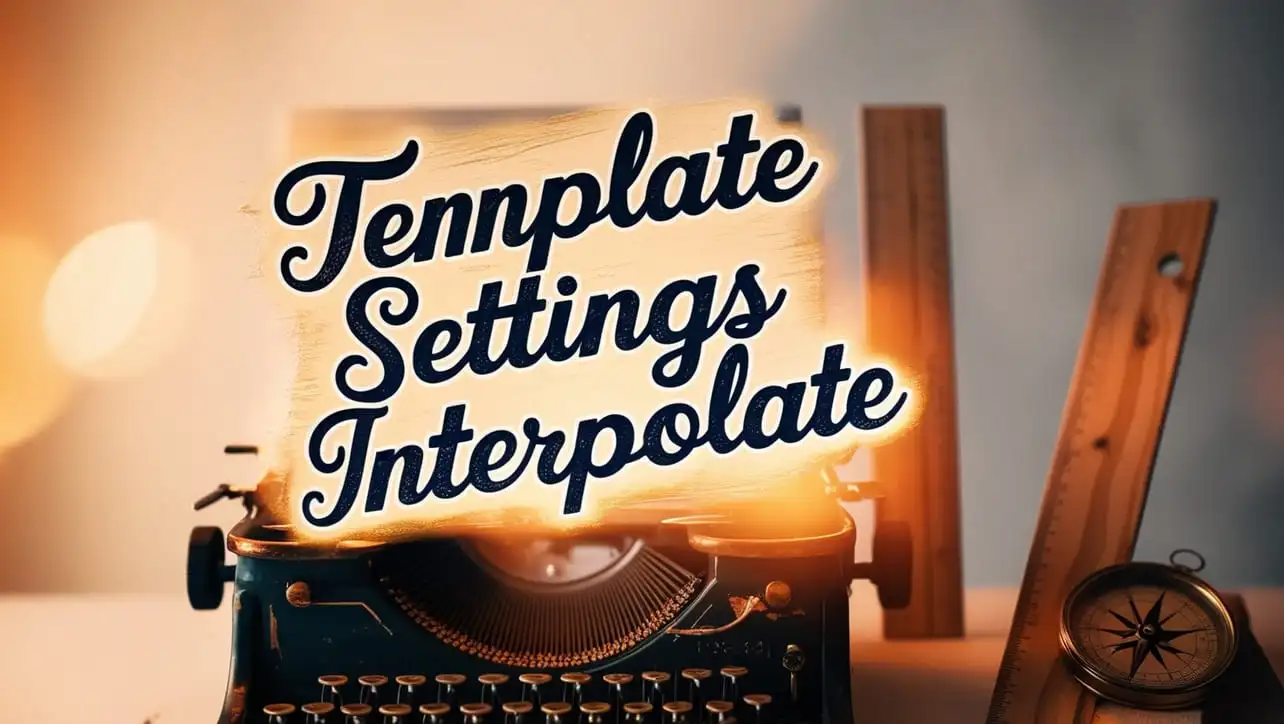
Lodash _.templateSettings.interpolate Property
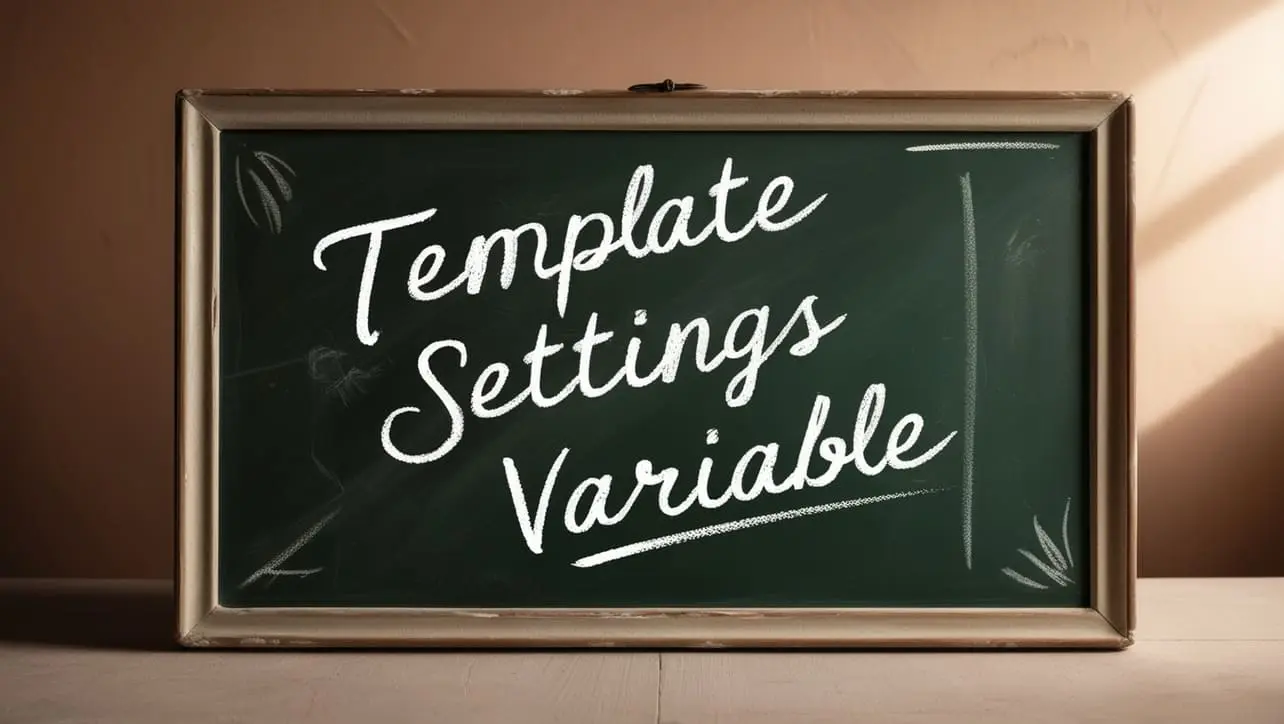
If you have any doubts regarding this article (Lodash _.findIndex() Array Method), please comment here. I will help you immediately.