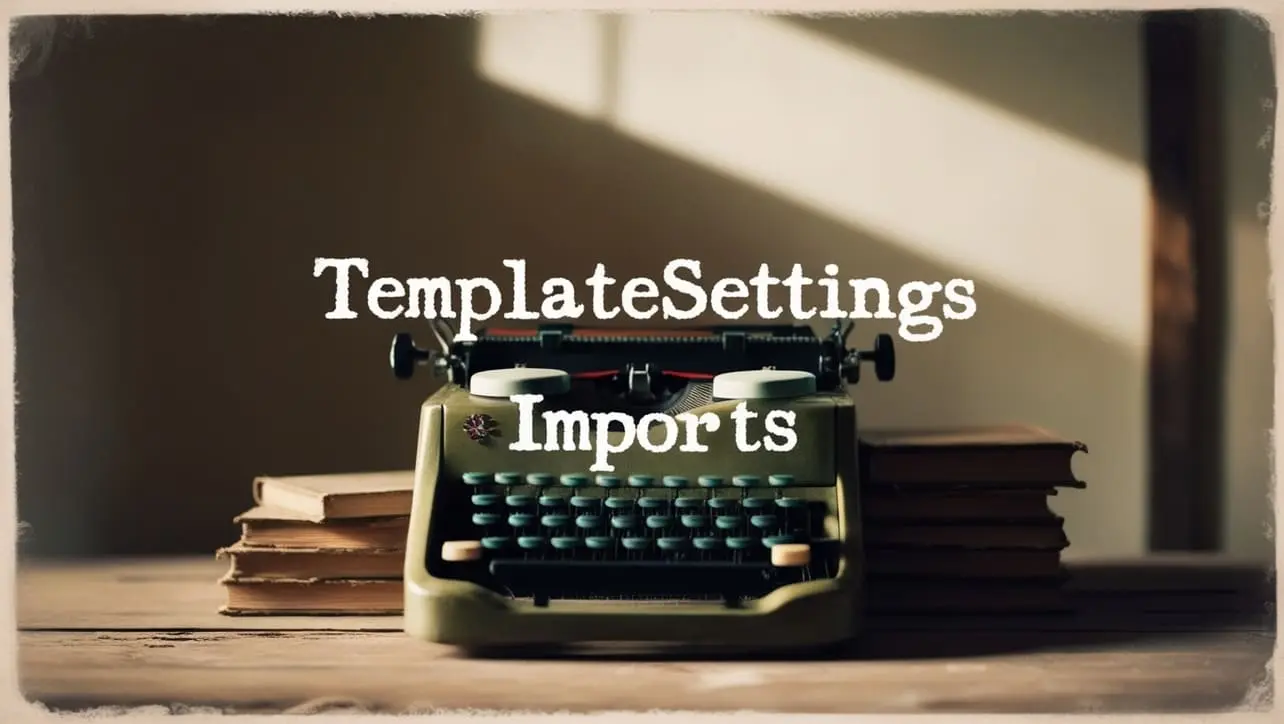
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.fill() Array Method
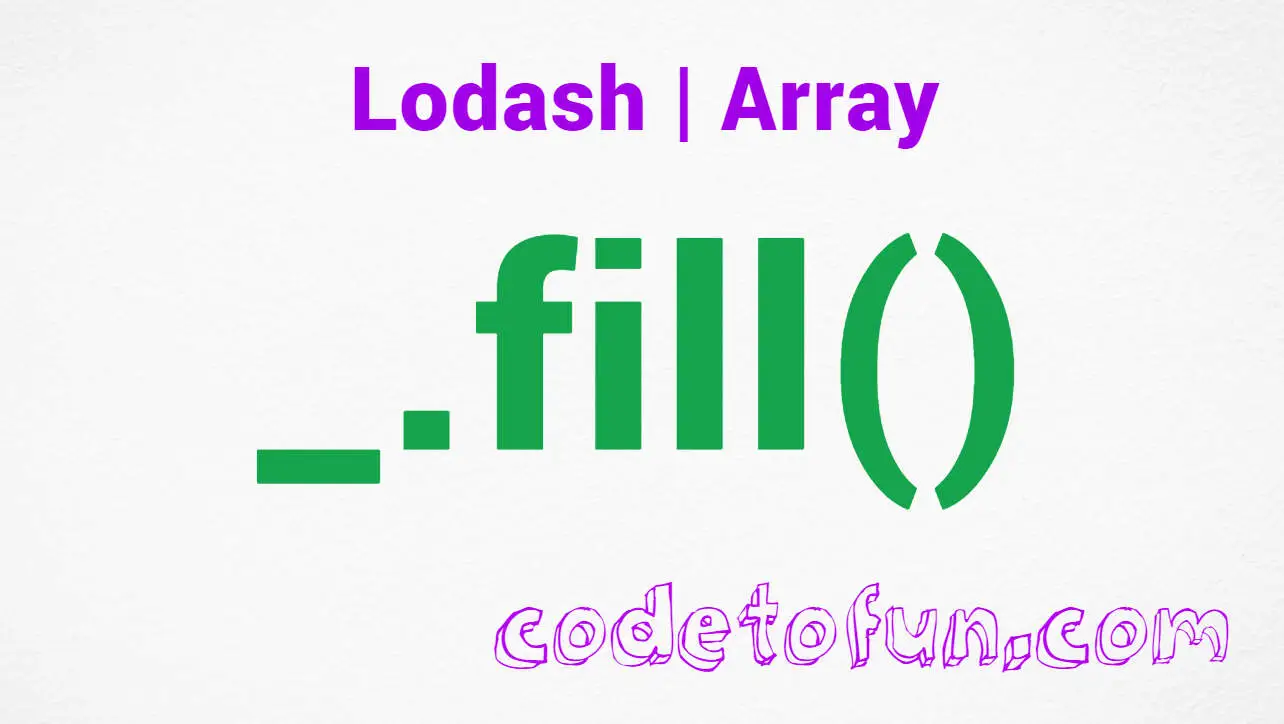
Photo Credit to CodeToFun
🙋 Introduction
Efficiently manipulating arrays is a fundamental aspect of JavaScript development. Lodash, a powerful utility library, provides a wide array of functions to simplify common programming tasks. One such versatile function is _.fill()
.
This method allows developers to populate specified portions of an array with a given value, offering flexibility and control over array data.
🧠 Understanding _.fill()
The _.fill()
method in Lodash is designed to fill a portion of an array with a specified value. This can be particularly useful when initializing arrays, updating specific ranges, or resetting values within a given range.
💡 Syntax
_.fill(array, value, [start=0], [end=array.length])
- array: The array to fill.
- value: The value to fill the array with.
- start: The index to start filling the array (default is 0).
- end: The index to stop filling the array (default is array.length).
📝 Example
Let's explore a simple example to illustrate the usage of _.fill()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const targetArray = [1, 2, 3, 4, 5];
const filledArray = _.fill(targetArray, '*', 1, 4);
console.log(filledArray);
// Output: [1, '*', '*', '*', 5]
In this example, the filledArray is created by replacing elements from index 1 to 3 (excluding index 4) with the specified value '*' in the targetArray.
🏆 Best Practices
Validate Inputs:
Before using
_.fill()
, ensure that the input array is valid and contains elements. Additionally, validate the start and end indices to avoid unexpected behavior.validate-inputs.jsCopiedif (!Array.isArray(targetArray) || targetArray.length === 0) { console.error('Invalid input array'); return; } const fillValue = '*'; const startIndex = 1; const endIndex = 4; const validatedFilledArray = _.fill(targetArray, fillValue, startIndex, endIndex); console.log(validatedFilledArray);
Handle Edge Cases:
Consider edge cases, such as an empty array or an end index greater than the array length. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst emptyArray = []; const invalidEndIndex = 10; const emptyArrayFilled = _.fill(emptyArray, fillValue); // Returns: [] const invalidEndIndexFilled = _.fill(targetArray, fillValue, startIndex, invalidEndIndex); // Returns: [1, '*', '*', '*', 5] console.log(emptyArrayFilled); console.log(invalidEndIndexFilled);
Customize Fill Values:
Experiment with different fill values based on your specific use case. Whether it's a string, number, or complex object,
_.fill()
provides flexibility in customizing array content.customize-fill-values.jsCopiedconst customObject = { prop: 'customValue' }; const customFilledArray = _.fill(Array(5), customObject); console.log(customFilledArray);
📚 Use Cases
Initialization:
_.fill()
is handy for initializing arrays with default values, creating a consistent starting point for further operations.initialization.jsCopiedconst initializedArray = _.fill(Array(5), 0); console.log(initializedArray); // Output: [0, 0, 0, 0, 0]
Resetting Values:
Reset specific values in an array without modifying the rest. This is useful for implementing undo functionalities or dynamic data updates.
resetting-values.jsCopiedconst dataToReset = [10, 20, 30, 40, 50]; const resetIndices = [1, 3]; const resetValue = 0; const resetData = _.fill(dataToReset, resetValue, ...resetIndices); console.log(resetData); // Output: [10, 0, 30, 0, 50]
Pattern Generation:
Create arrays with specific patterns or repetitions using
_.fill()
.pattern-generation.jsCopiedconst repeatingPattern = _.fill(Array(5), 'ABC'); console.log(repeatingPattern); // Output: ['ABC', 'ABC', 'ABC', 'ABC', 'ABC']
🎉 Conclusion
The _.fill()
method in Lodash empowers JavaScript developers to efficiently manipulate array data by providing a concise and flexible way to fill specific ranges with desired values. Whether initializing arrays, resetting values, or generating patterns, _.fill()
is a valuable tool in your programming toolkit.
Explore the capabilities of _.fill()
and elevate your array manipulation skills with Lodash!
👨💻 Join our Community:
Author
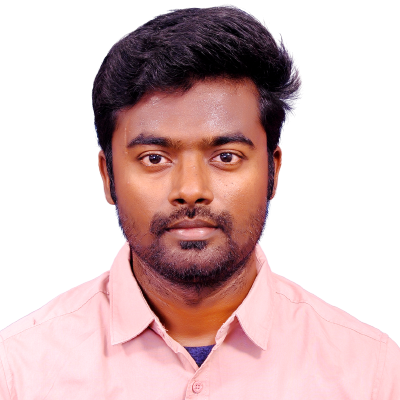
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
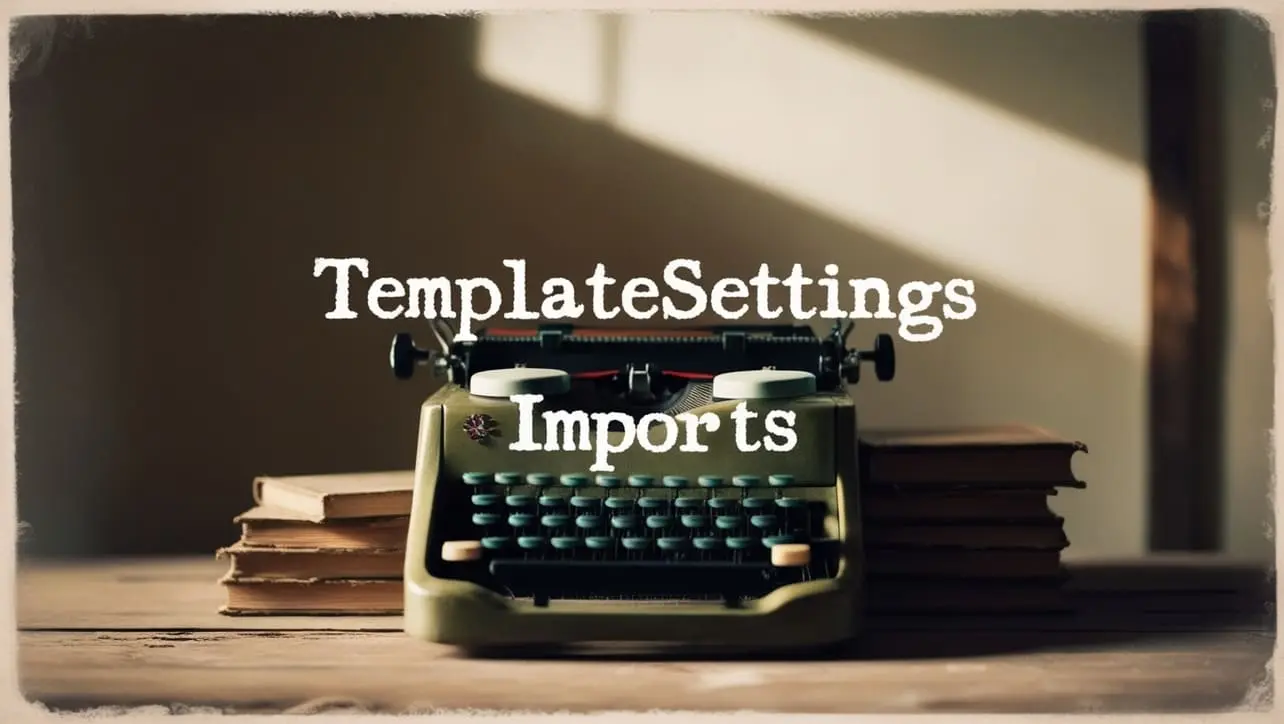
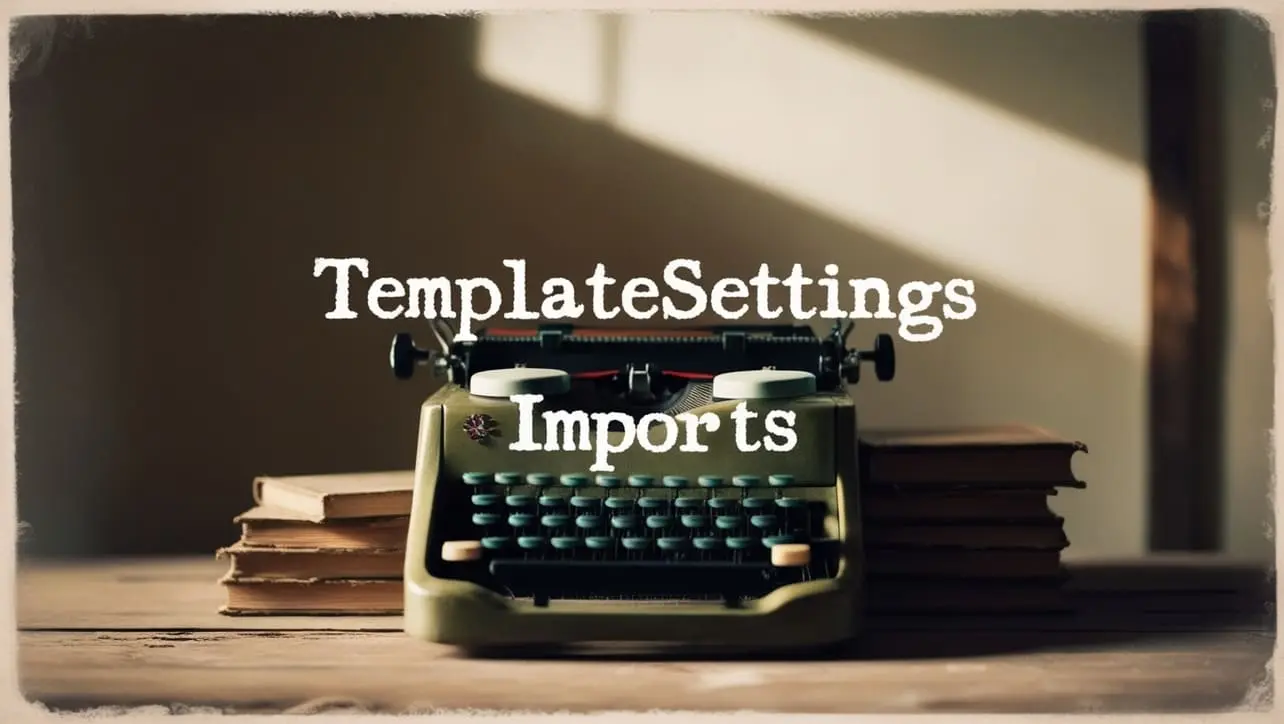
Lodash _.templateSettings.imports Property
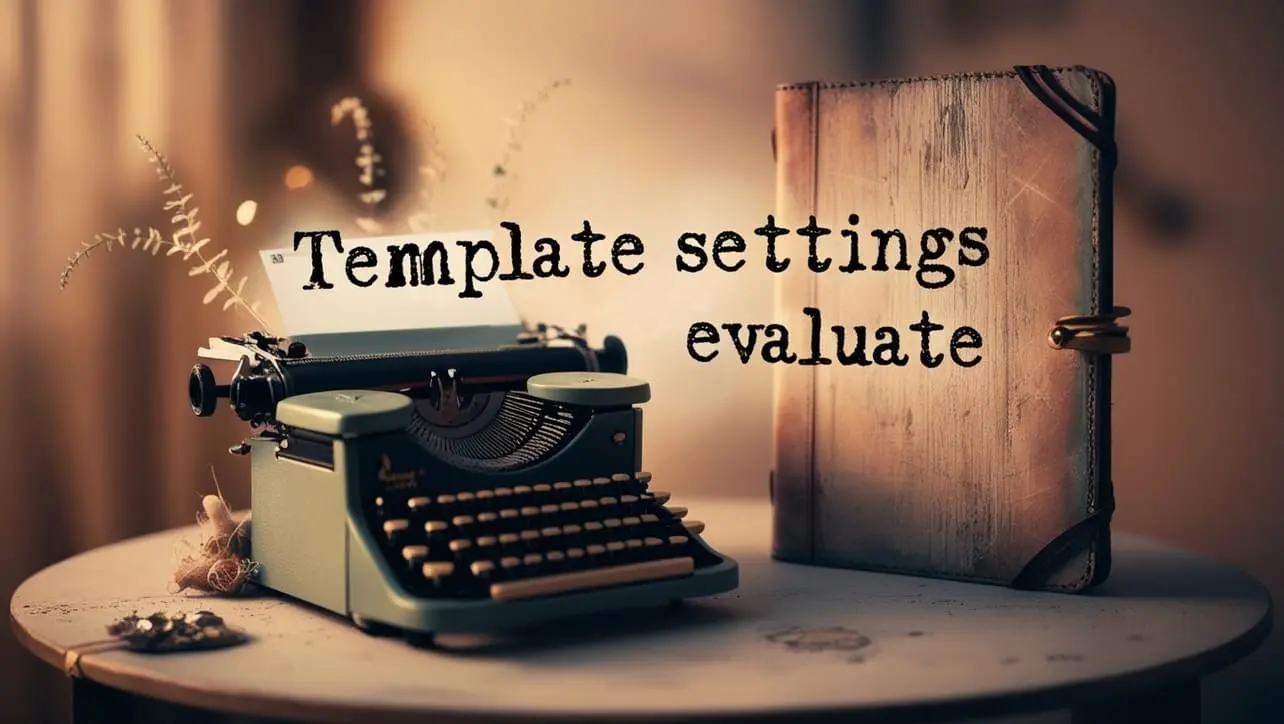
Lodash _.templateSettings.evaluate Property
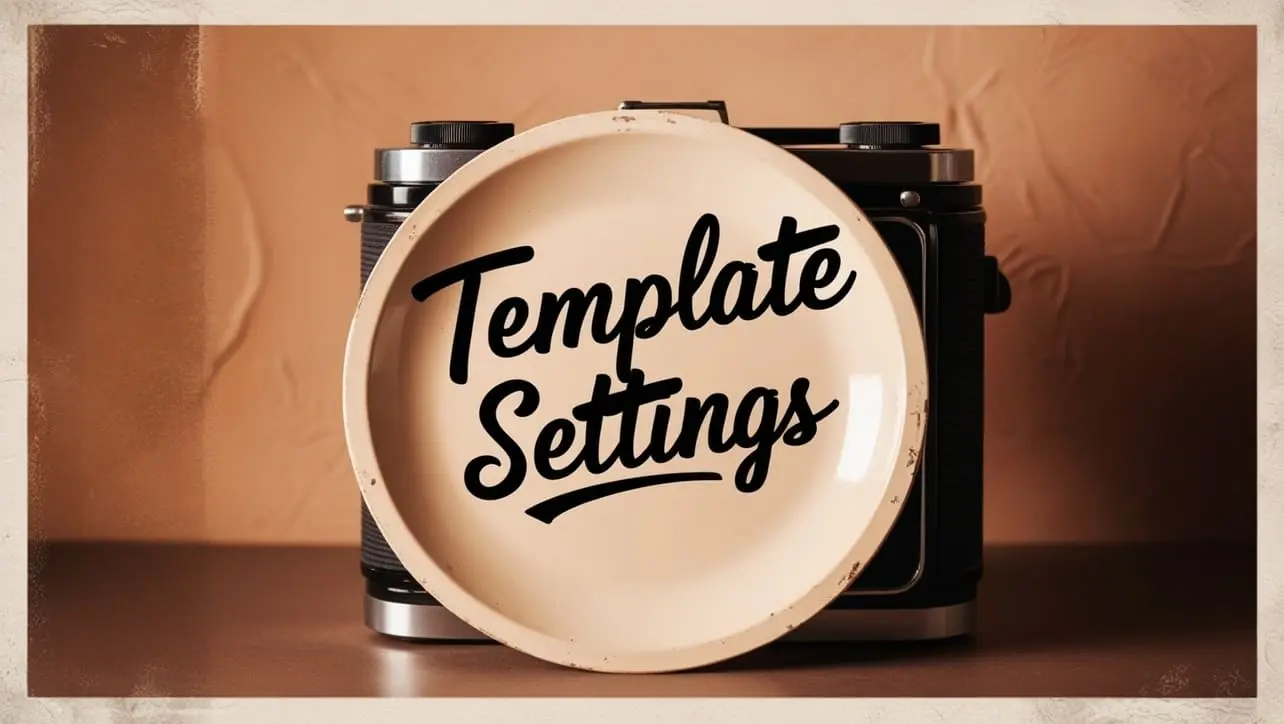
Lodash _.templateSettings Property
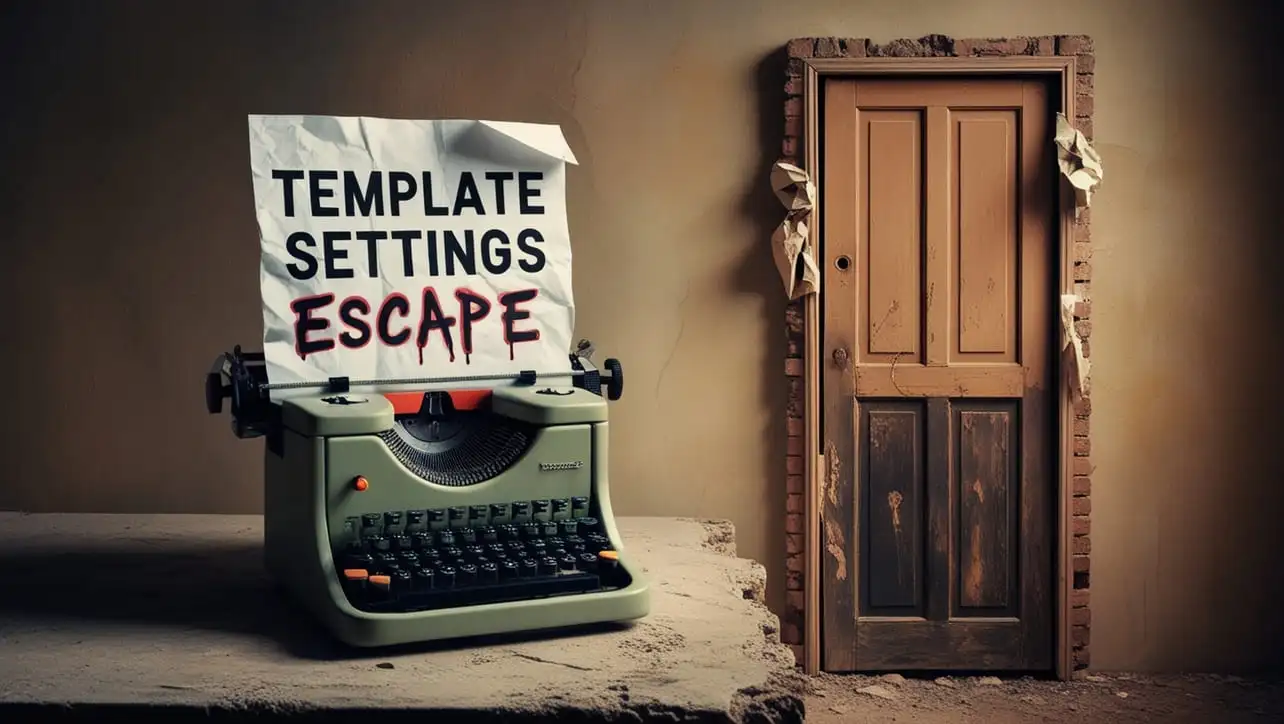
Lodash _.templateSettings.escape Property
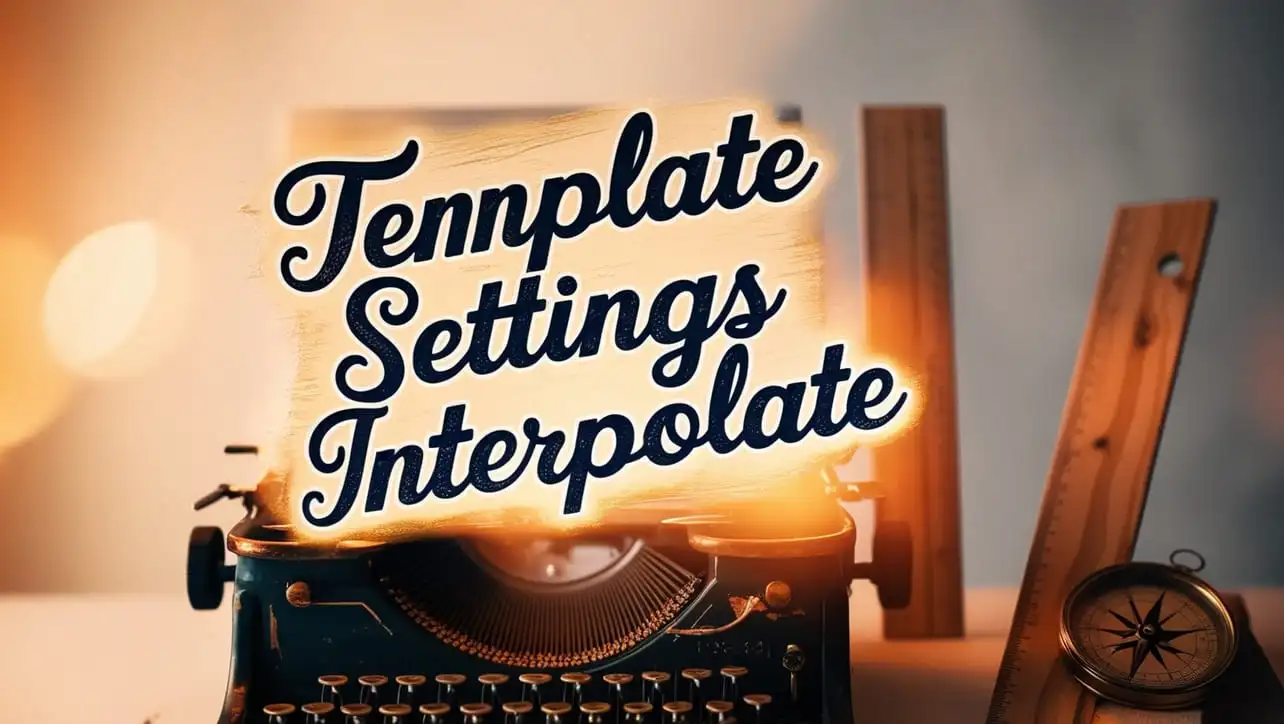
Lodash _.templateSettings.interpolate Property
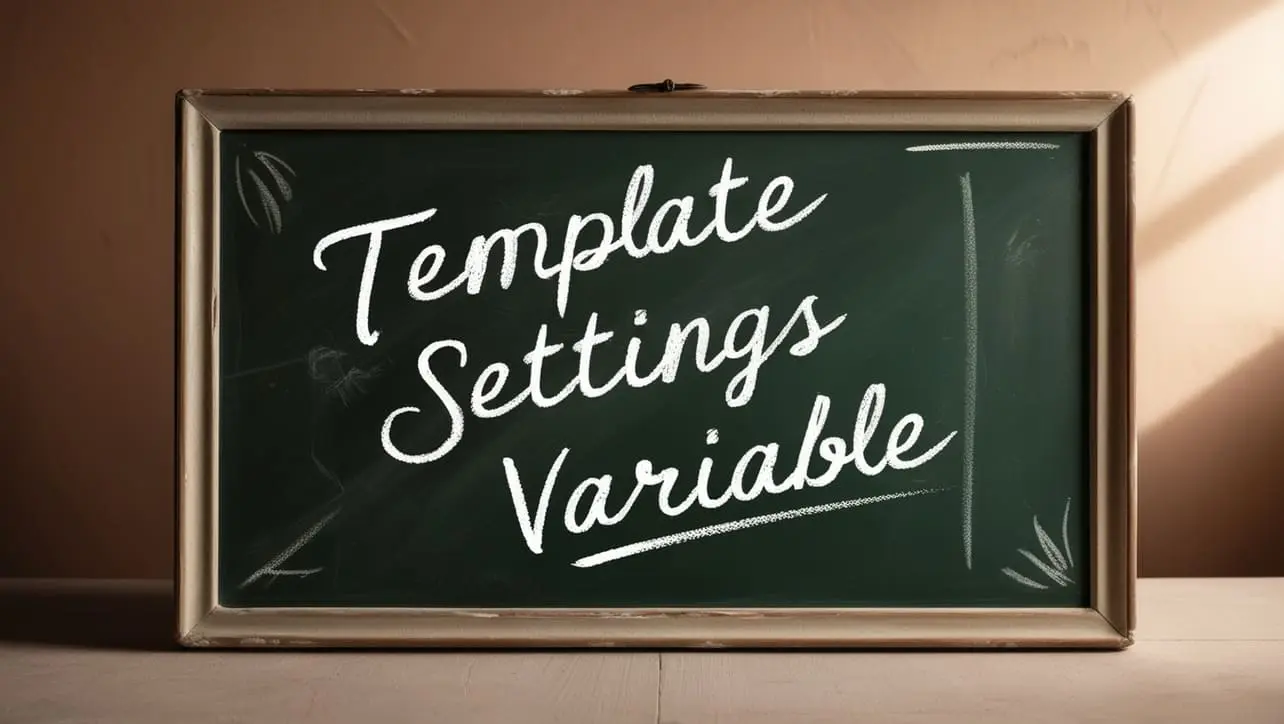
If you have any doubts regarding this article (Lodash _.fill() Array Method), please comment here. I will help you immediately.