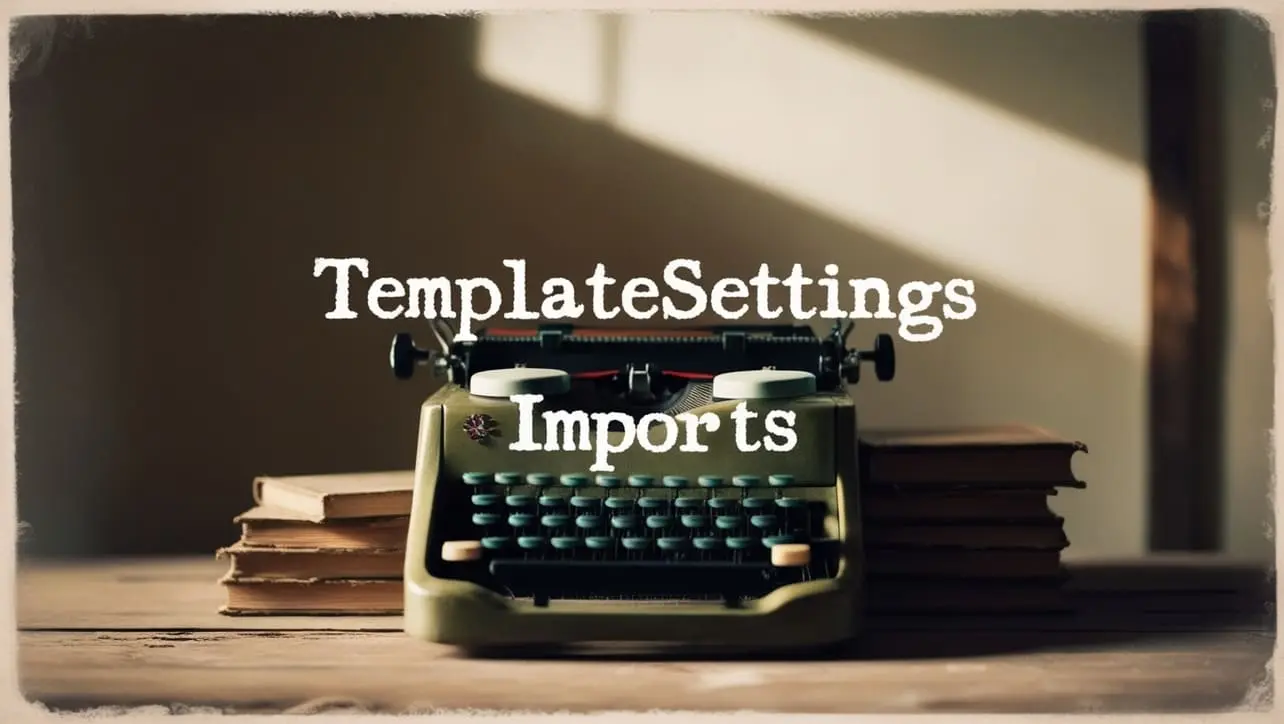
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.dropWhile() Array Method
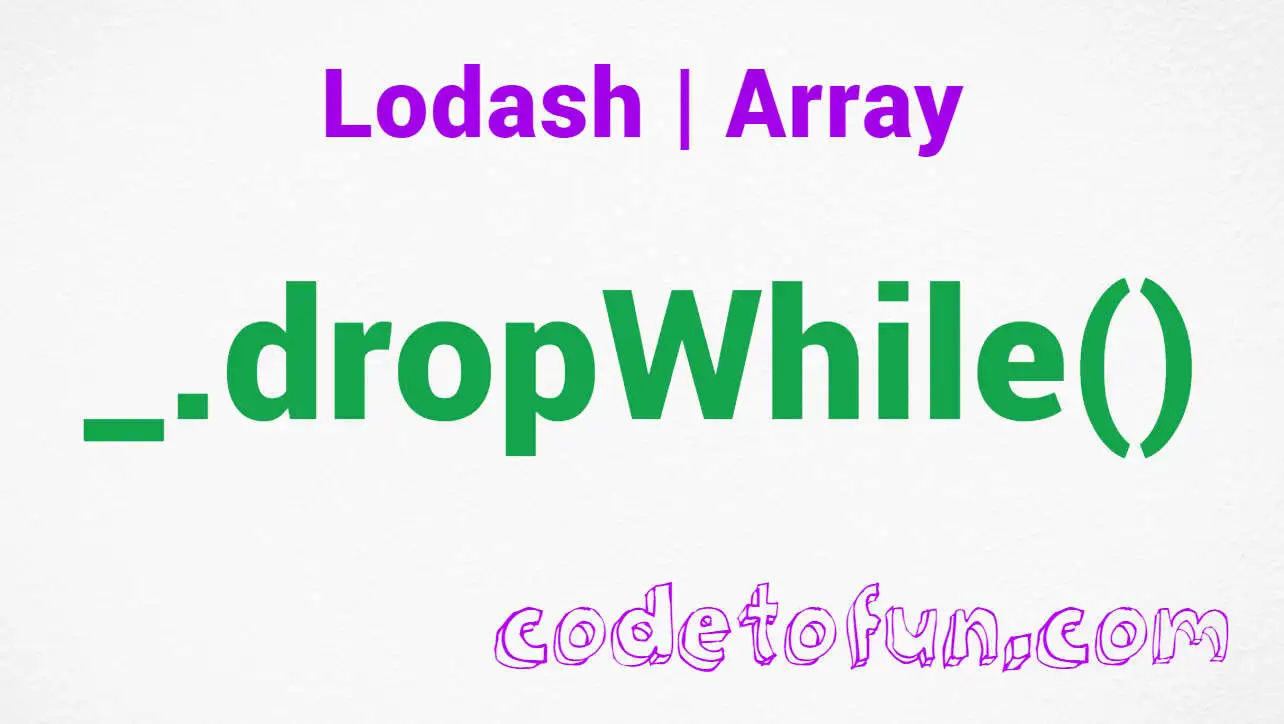
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, array manipulation is a common task, and the Lodash library provides a wealth of utility functions to simplify these operations. One such versatile method is _.dropWhile()
, which allows developers to selectively exclude elements from the beginning of an array based on a specified condition.
This method proves valuable in scenarios where data filtering is essential to achieve desired outcomes.
🧠 Understanding _.dropWhile()
The _.dropWhile()
method in Lodash removes elements from the beginning of an array until the provided predicate function returns false. This enables developers to skip unwanted elements and start processing the array from a specific point.
💡 Syntax
_.dropWhile(array, [predicate=_.identity])
- array: The array to process.
- predicate: The function invoked per iteration, returning true to continue dropping elements (default is _.identity).
📝 Example
Let's delve into a practical example to grasp the functionality of _.dropWhile()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const result = _.dropWhile(numbers, (num) => num < 5);
console.log(result);
// Output: [5, 6, 7, 8, 9]
In this example, elements from the beginning of the numbers array are dropped until the predicate function (num) => num < 5 returns false.
🏆 Best Practices
Define Clear Predicates:
When using
_.dropWhile()
, ensure that the predicate function clearly defines the condition for dropping elements. Ambiguous or complex predicates may lead to unexpected results.define-clear-predicates.jsCopiedconst users = [ { name: 'Alice', age: 25 }, { name: 'Bob', age: 30 }, { name: 'Charlie', age: 22 }, ]; // Ambiguous predicate: drops until age is not undefined const ambiguousResult = _.dropWhile(users, (user) => user.age); console.log(ambiguousResult); // Returns: [{ name: 'Bob', age: 30 }, { name: 'Charlie', age: 22 }] // Clear predicate: drops until age is less than 30 const clearResult = _.dropWhile(users, (user) => user.age < 30); console.log(clearResult); // Returns: [{ name: 'Bob', age: 30 }, { name: 'Charlie', age: 22 }]
Handle Edge Cases:
Consider edge cases, such as an empty array or a predicate that always evaluates to true. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst emptyArray = []; const alwaysTruePredicate = () => true; const emptyResult = _.dropWhile(emptyArray, alwaysTruePredicate); // Returns: [] const alwaysTrueResult = _.dropWhile(numbers, alwaysTruePredicate); // Returns: [] console.log(emptyResult); console.log(alwaysTrueResult);
Combine with Other Lodash Methods:
Explore combining
_.dropWhile()
with other Lodash methods to create powerful data transformation pipelines. This can lead to more concise and expressive code.other-methods.jsCopiedconst data = /* ...fetch data from API or elsewhere... */; const transformedData = _ .chain(data) .dropWhile((item) => item.status === 'pending') .map((item) => item.name.toUpperCase()) .value(); console.log(transformedData);
📚 Use Cases
Skipping Initial Irrelevant Data:
_.dropWhile()
is beneficial when dealing with datasets where initial elements are irrelevant or do not meet specific criteria.skip-data.jsCopiedconst logEntries = /* ...fetch log data from server... */; const relevantEntries = _.dropWhile(logEntries, (entry) => entry.level !== 'error'); console.log(relevantEntries);
Filtering Sorted Arrays:
When working with sorted arrays,
_.dropWhile()
can efficiently filter out elements that no longer satisfy certain conditions.filtering-sorted-arrays.jsCopiedconst sortedNumbers = [1, 3, 5, 7, 9, 8, 6, 4, 2]; const filteredNumbers = _.dropWhile(sortedNumbers, (num, index, array) => num < array[index - 1]); console.log(filteredNumbers);
Dynamic Data Processing:
In scenarios where the condition for dropping elements is dynamic,
_.dropWhile()
allows for flexible data processing.dynamic-data-processing.jsCopiedconst dynamicCondition = /* ...calculate dynamic condition... */; const dynamicResult = _.dropWhile(data, (item) => /* ...check against dynamic condition... */); console.log(dynamicResult);
🎉 Conclusion
The _.dropWhile()
method in Lodash is a powerful tool for selectively excluding elements from the beginning of an array based on a specified condition.
By leveraging this method, developers can streamline data processing, skip irrelevant information, and create more efficient workflows. Incorporate _.dropWhile()
into your JavaScript projects to enhance the flexibility and effectiveness of your array manipulation tasks.
Explore the capabilities of Lodash, and unlock the potential of array manipulation with _.dropWhile()
!
👨💻 Join our Community:
Author
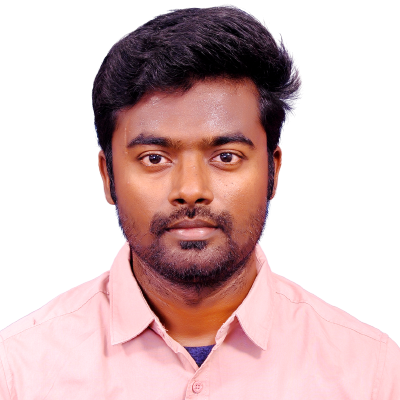
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
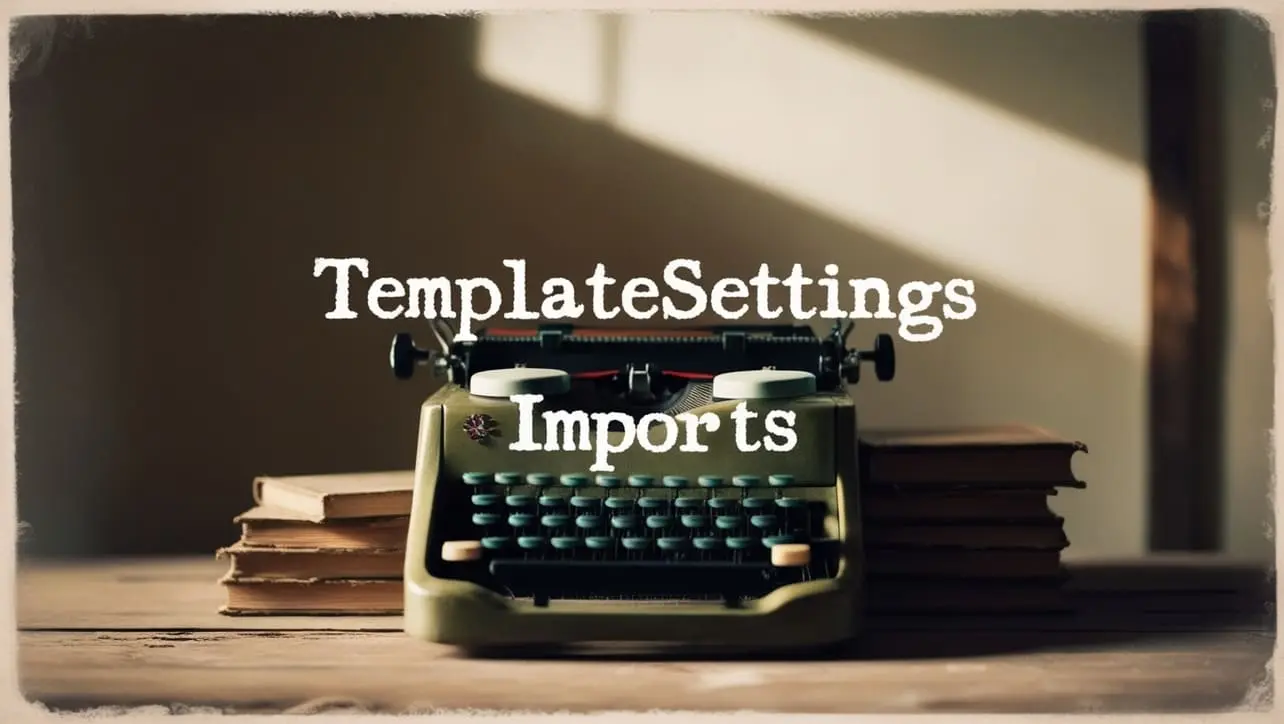
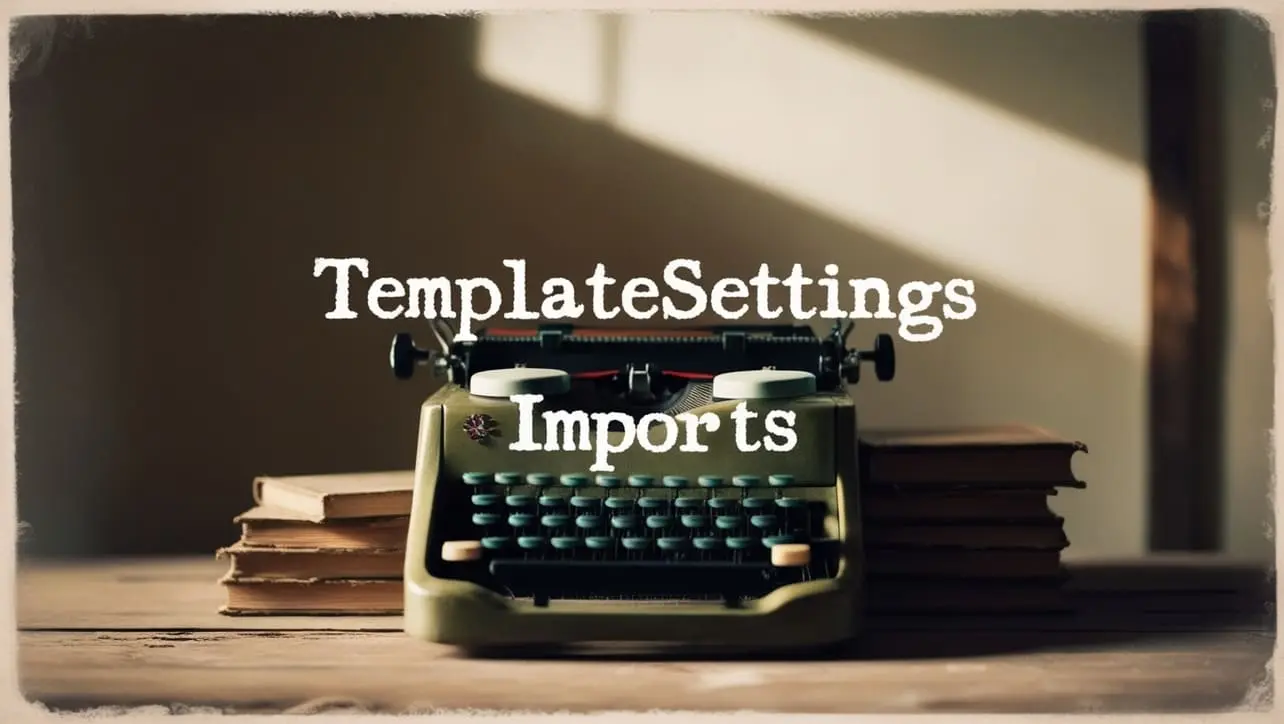
Lodash _.templateSettings.imports Property
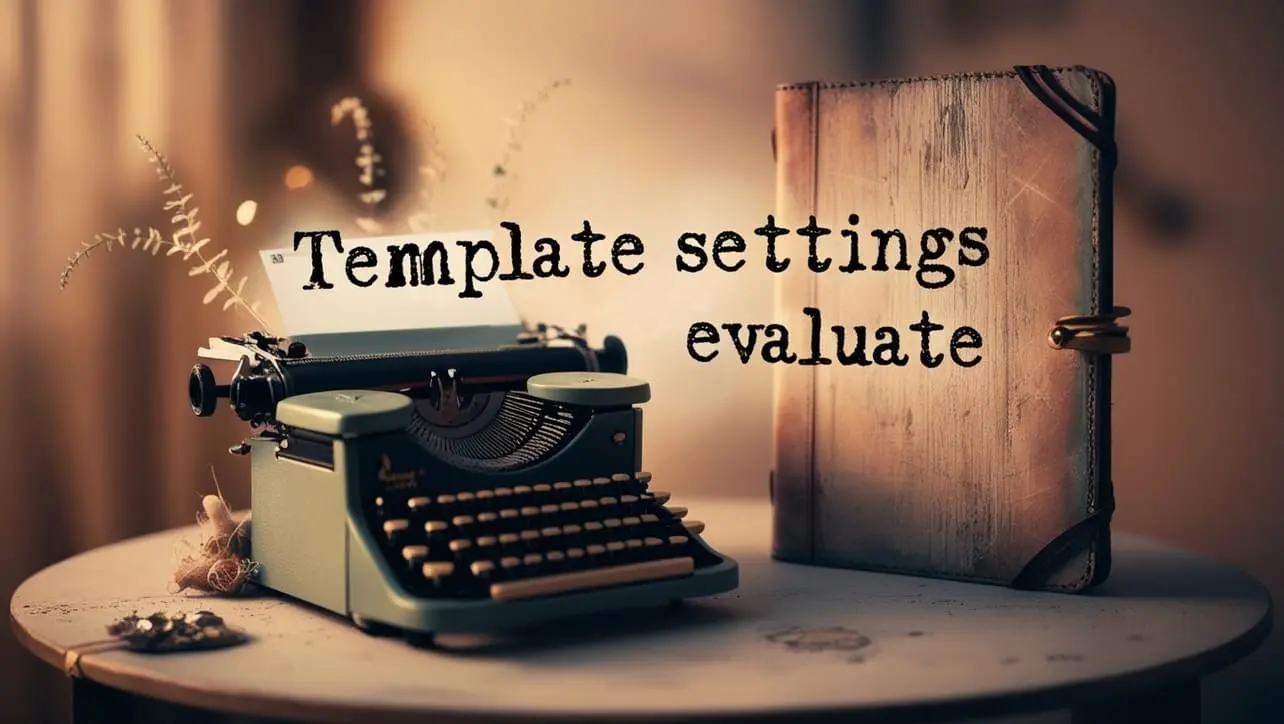
Lodash _.templateSettings.evaluate Property
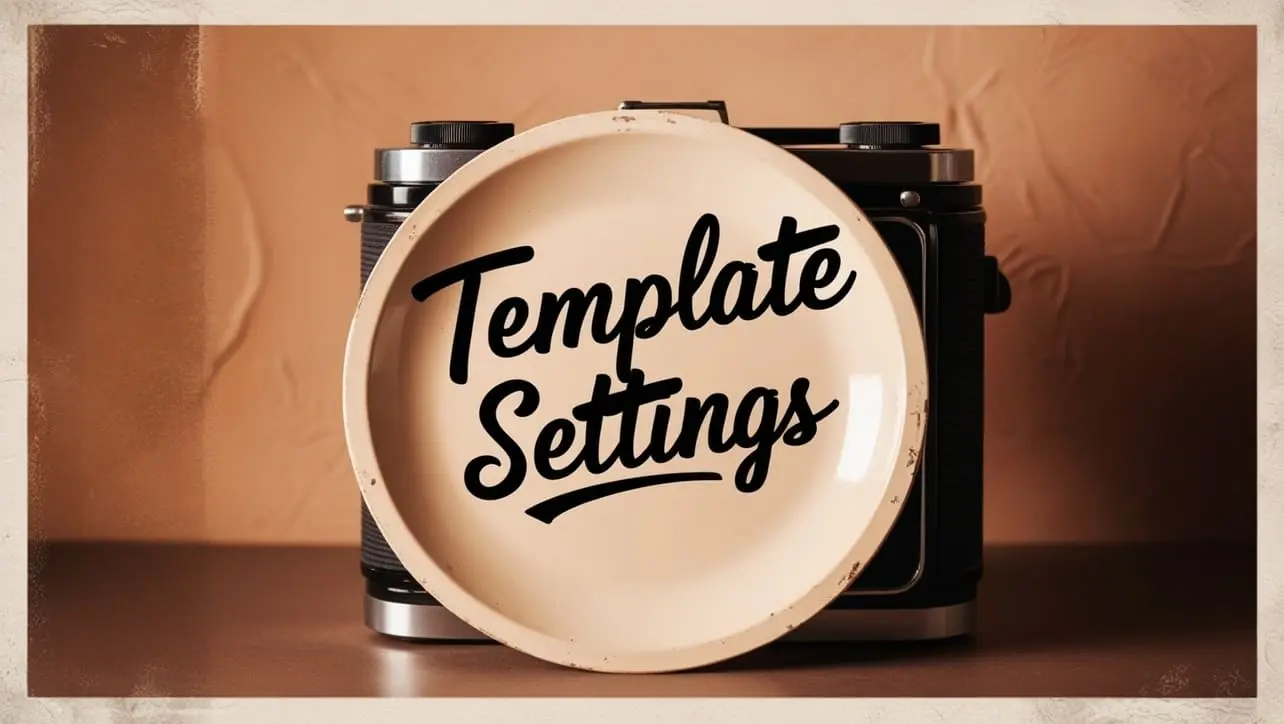
Lodash _.templateSettings Property
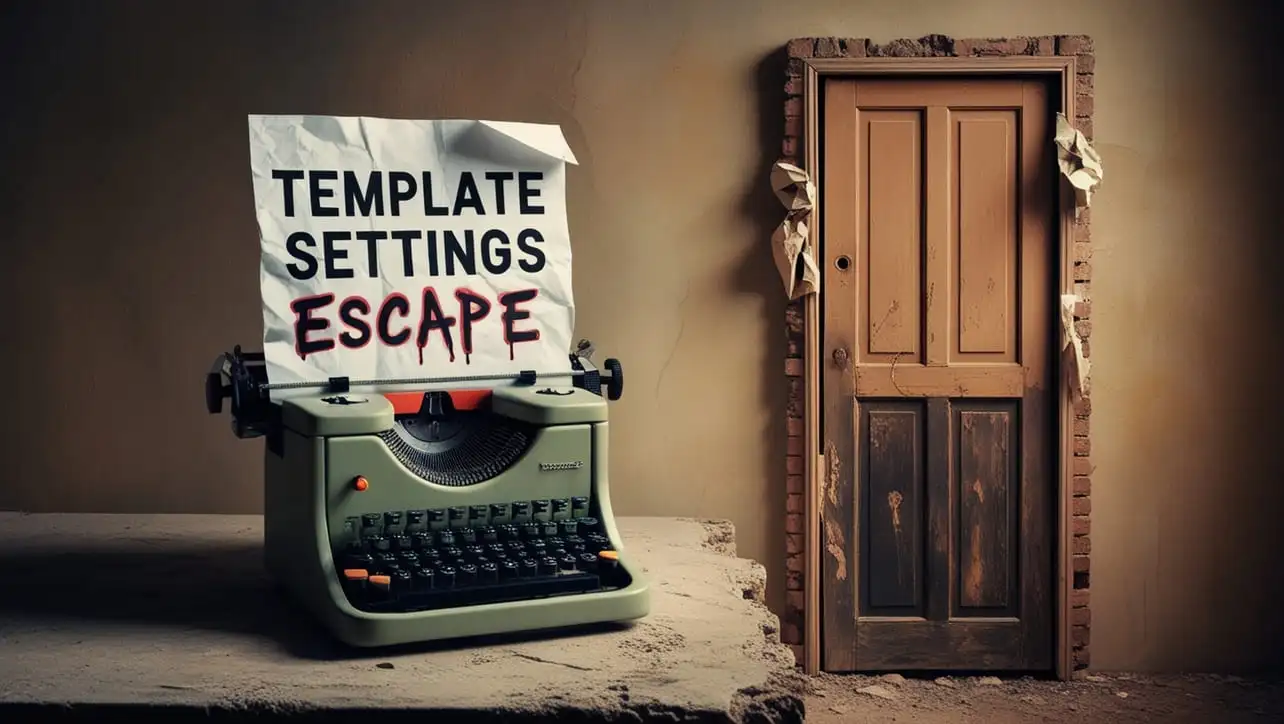
Lodash _.templateSettings.escape Property
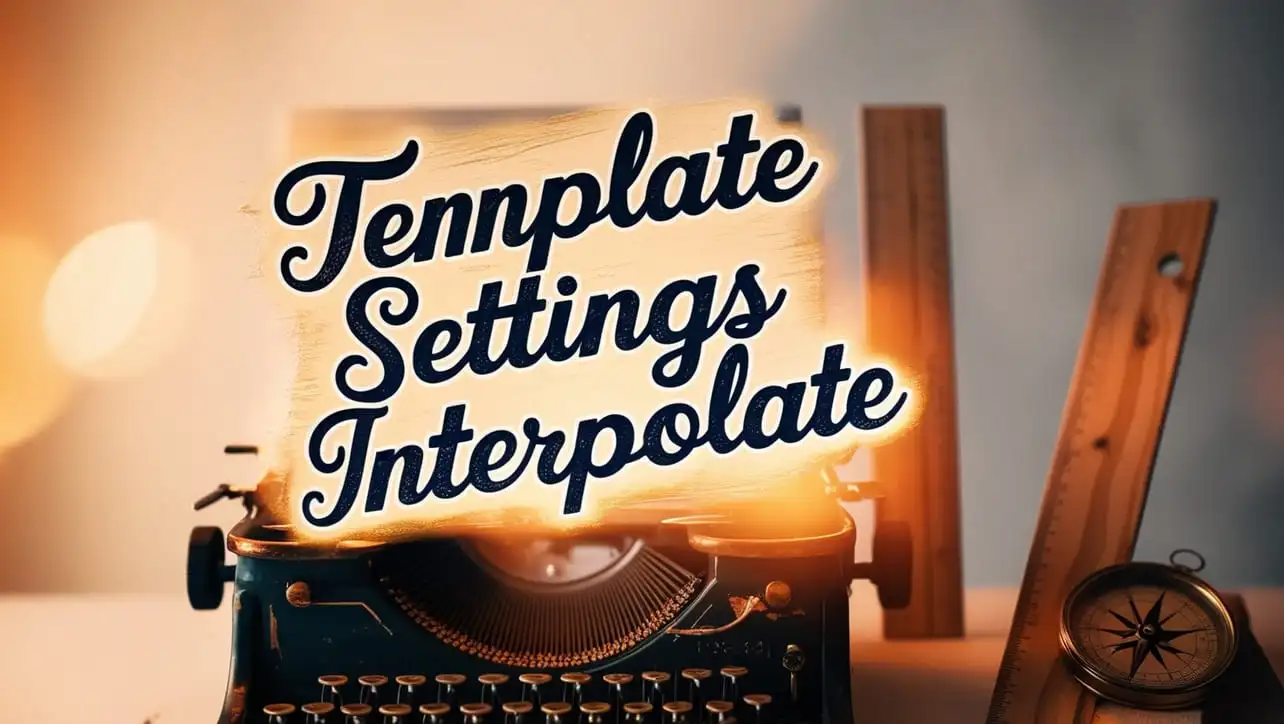
Lodash _.templateSettings.interpolate Property
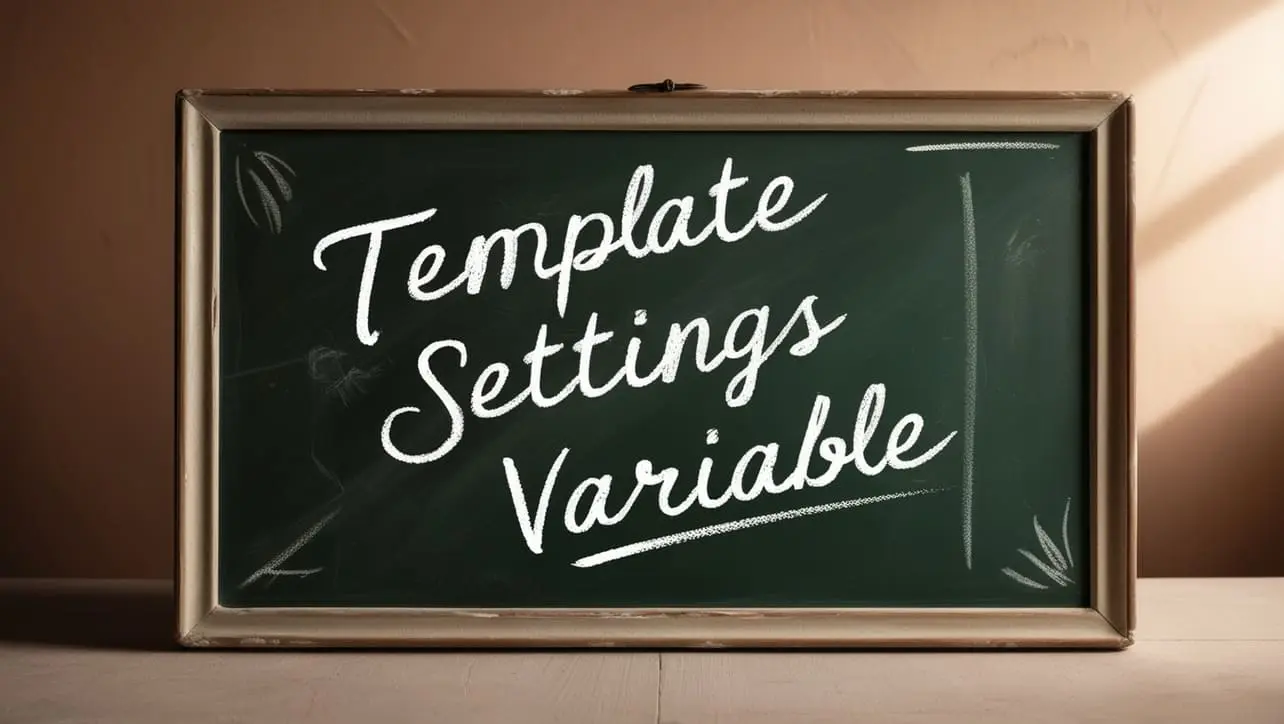
If you have any doubts regarding this article (Lodash _.dropWhile() Array Method), please comment here. I will help you immediately.