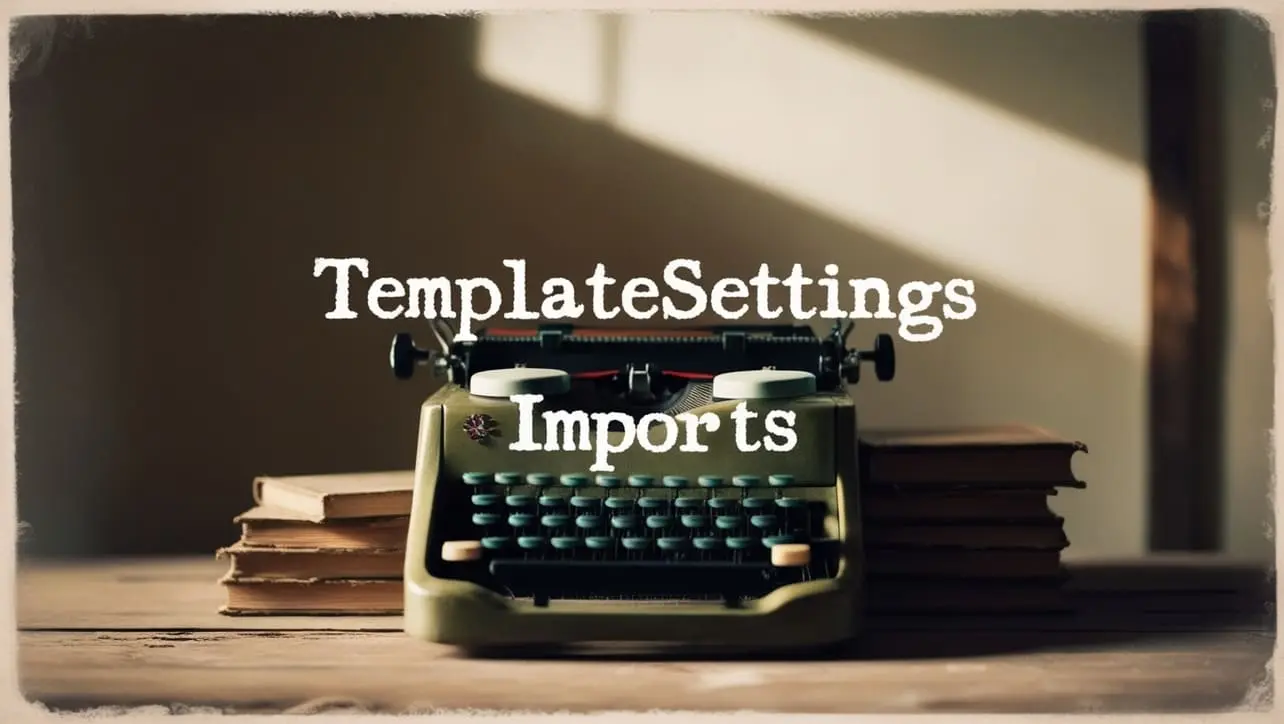
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.dropRightWhile() Array Method
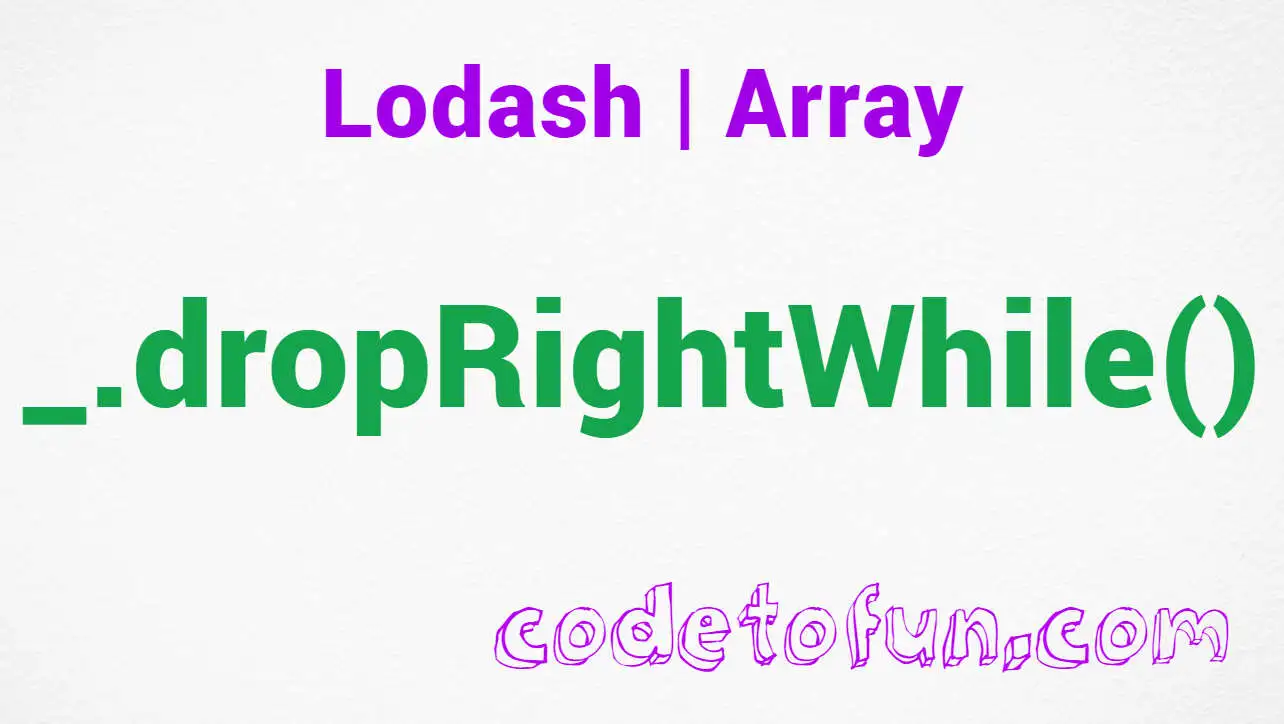
Photo Credit to CodeToFun
🙋 Introduction
When it comes to array manipulation in JavaScript, Lodash stands out with its rich set of utility functions. Among these, the _.dropRightWhile()
method provides a powerful way to trim elements from the end of an array based on a given condition.
In this guide, we'll delve into the details of the _.dropRightWhile()
method, exploring its syntax, usage, and best practices.
🧠 Understanding _.dropRightWhile()
The _.dropRightWhile()
method in Lodash is designed to remove elements from the end of an array until a provided predicate function returns false. This can be particularly useful when dealing with datasets where you want to exclude trailing elements based on a specific criterion.
💡 Syntax
_.dropRightWhile(array, [predicate=_.identity])
- array: The array to modify.
- predicate: The function invoked per iteration (default is _.identity).
📝 Example
Let's illustrate the usage of _.dropRightWhile()
with a practical example:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const data = [
{ id: 1, value: 10 },
{ id: 2, value: 20 },
{ id: 3, value: 30 },
{ id: 4, value: 15 },
{ id: 5, value: 5 },
];
const filteredData = _.dropRightWhile(data, (item) => item.value > 10);
console.log(filteredData);
// Output: [{ id: 1, value: 10 }, { id: 2, value: 20 }, { id: 3, value: 30 }]
In this example, the filteredData array is created by removing elements from the end of the data array where the value property is greater than 10.
🏆 Best Practices
Define a Clear Predicate Function:
Ensure that the predicate function used in
_.dropRightWhile()
clearly defines the condition for dropping elements. This promotes code readability and avoids unexpected behavior.clear-predicate-function.jsCopiedconst clearPredicate = (item) => item.status === 'active'; const filteredArray = _.dropRightWhile(data, clearPredicate); console.log(filteredArray);
Handle Edge Cases:
Consider potential edge cases, such as an empty array or a predicate function that never returns false. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst emptyArray = []; const noDropPredicate = (item) => item.value > 100; const result1 = _.dropRightWhile(emptyArray, noDropPredicate); // Returns: [] const result2 = _.dropRightWhile(data, noDropPredicate); // Returns: [] console.log(result1); console.log(result2);
Explore Alternative Solutions:
While
_.dropRightWhile()
is powerful, explore other Lodash methods or native JavaScript alternatives based on the specific requirements of your task.explore-alternative-solutions.jsCopied// Using native JavaScript array methods const nativeFilteredData = data.slice(0, data.findIndex(item => item.value <= 10)); console.log(nativeFilteredData);
📚 Use Cases
Removing Unwanted Trailers:
_.dropRightWhile()
is excellent for scenarios where you want to remove elements from the end of an array based on a specific condition. This is particularly useful for trimming data trailers that don't meet certain criteria.removing-unwanted-trailers.jsCopiedconst userActivity = /* ...fetch user activity data... */; const filteredActivity = _.dropRightWhile(userActivity, (activity) => activity.date < cutoffDate); console.log(filteredActivity);
Handling Time Series Data:
When working with time series data, you may want to drop trailing data points that fall below a certain threshold.
_.dropRightWhile()
simplifies this task.handling-time-series-data.jsCopiedconst timeSeriesData = /* ...fetch time series data... */; const filteredSeries = _.dropRightWhile(timeSeriesData, (point) => point.value < threshold); console.log(filteredSeries);
Efficient Pagination:
In scenarios where you implement pagination and want to exclude unnecessary pages at the end,
_.dropRightWhile()
can be a valuable ally.efficient-pagination.jsCopiedconst paginatedData = /* ...fetch paginated data... */; const validatedPages = _.dropRightWhile(paginatedData, (page) => page.isEmpty); console.log(validatedPages);
🎉 Conclusion
The _.dropRightWhile()
method in Lodash empowers JavaScript developers to efficiently manipulate arrays, selectively dropping elements from the end based on specified conditions. By integrating this method into your codebase, you can enhance the precision and effectiveness of your array manipulations.
Explore the capabilities of Lodash and leverage _.dropRightWhile()
to streamline your array processing tasks!
👨💻 Join our Community:
Author
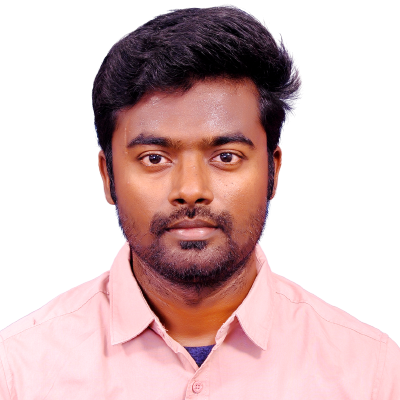
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
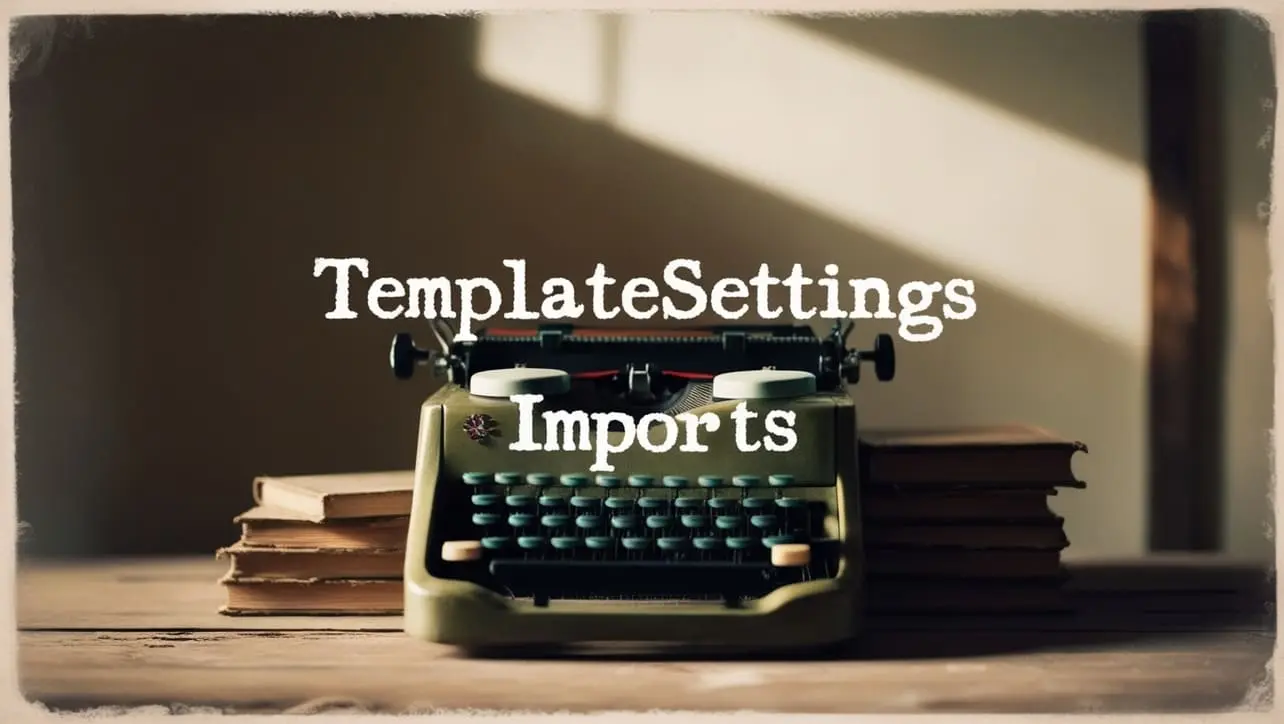
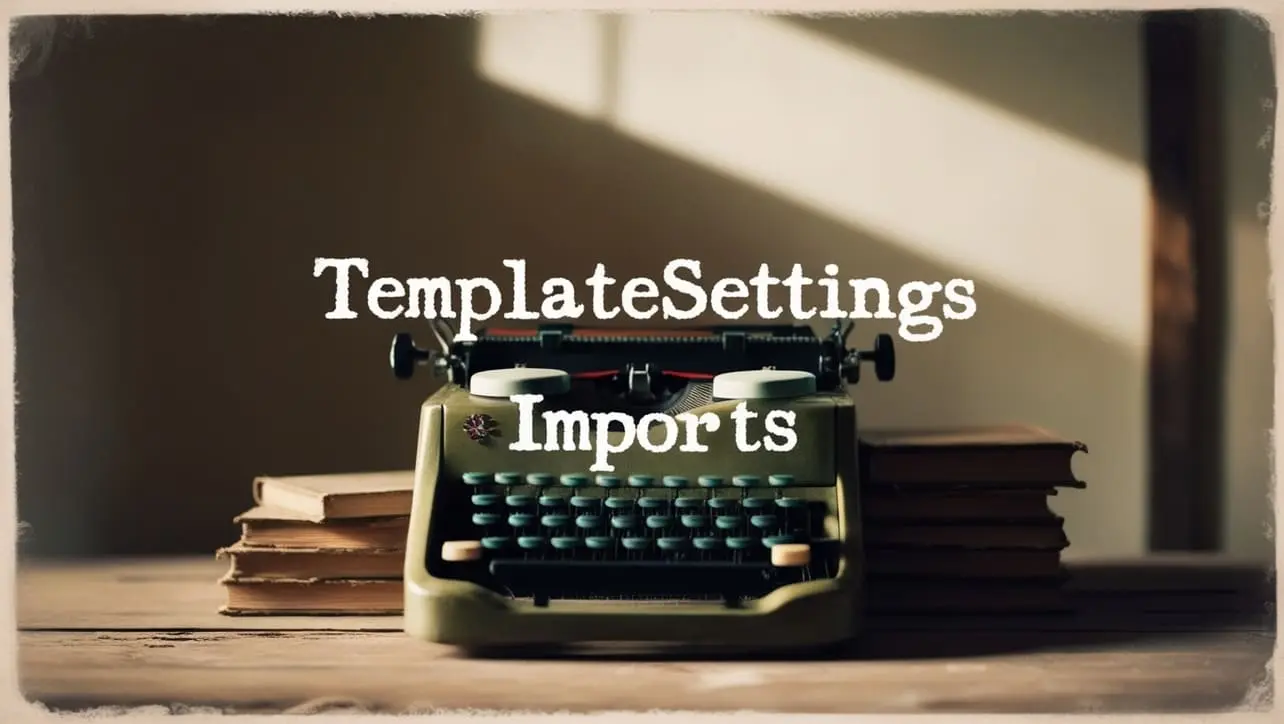
Lodash _.templateSettings.imports Property
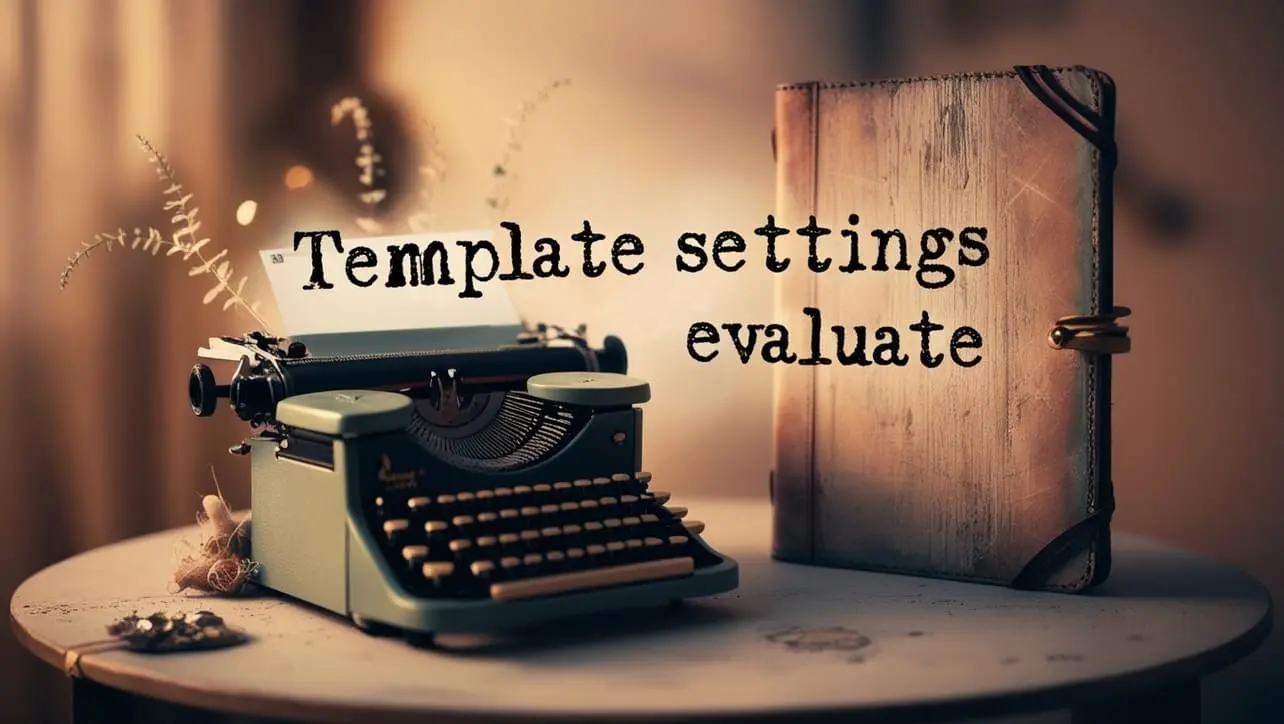
Lodash _.templateSettings.evaluate Property
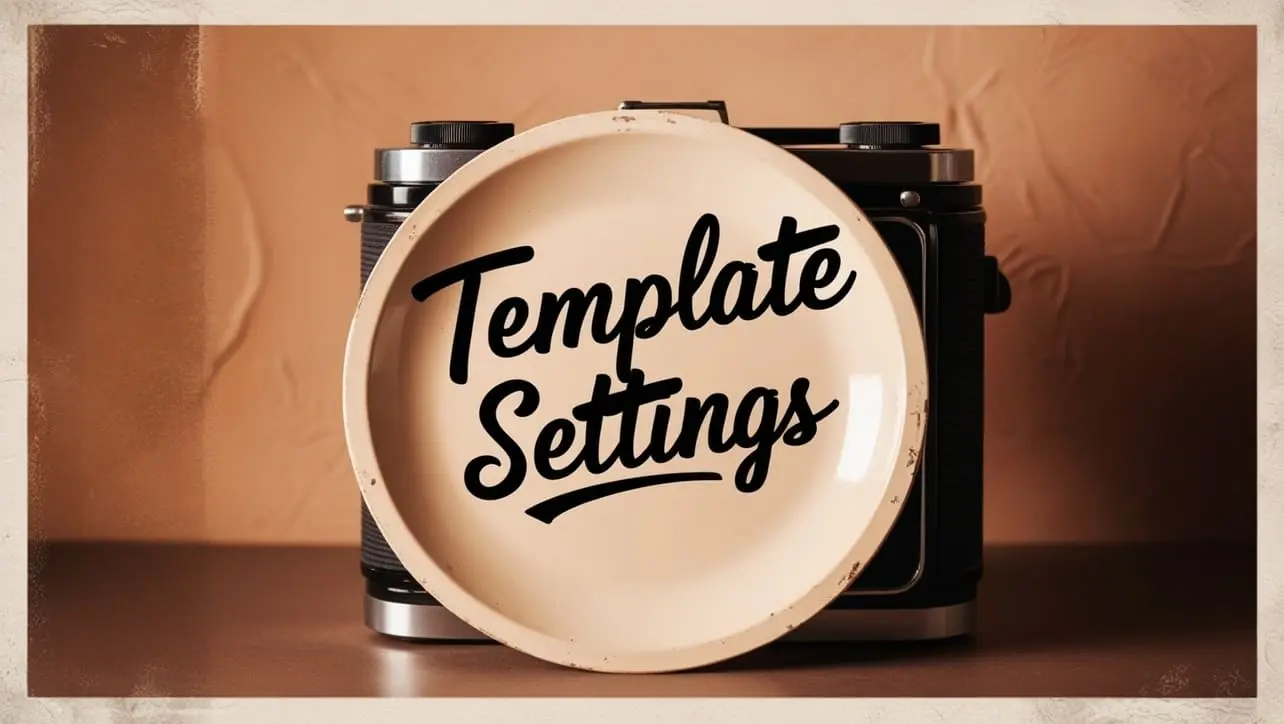
Lodash _.templateSettings Property
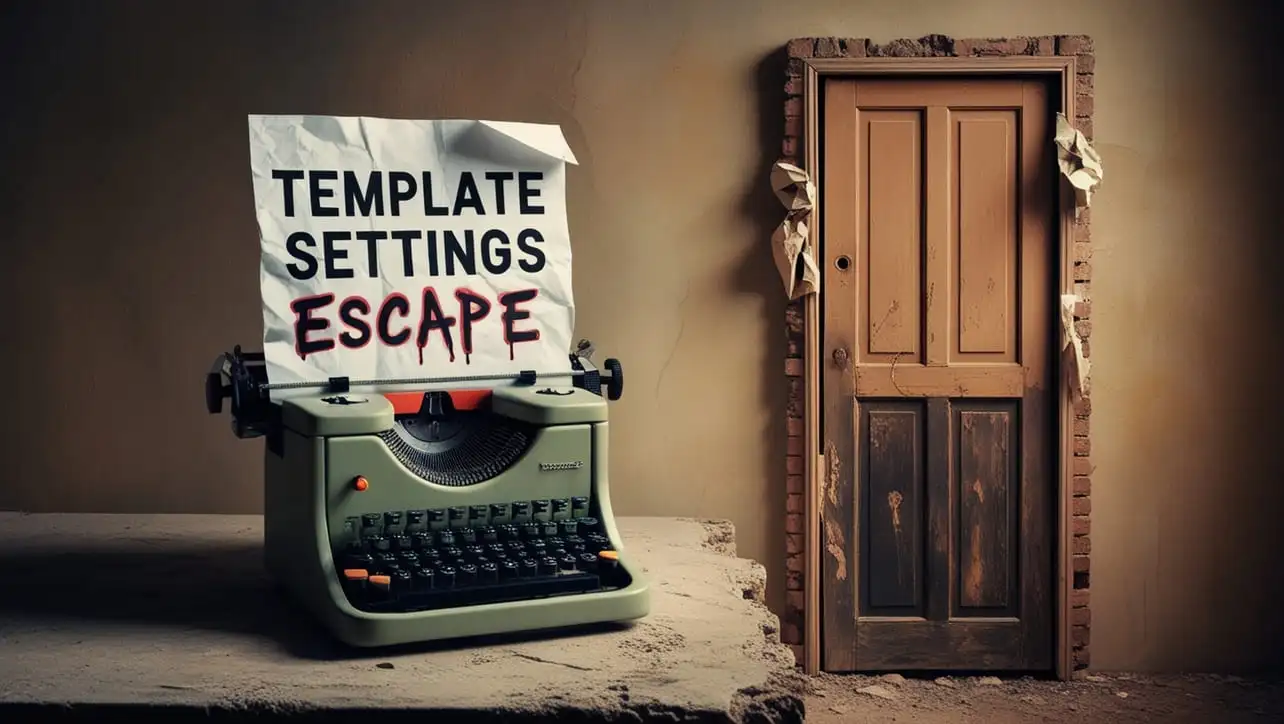
Lodash _.templateSettings.escape Property
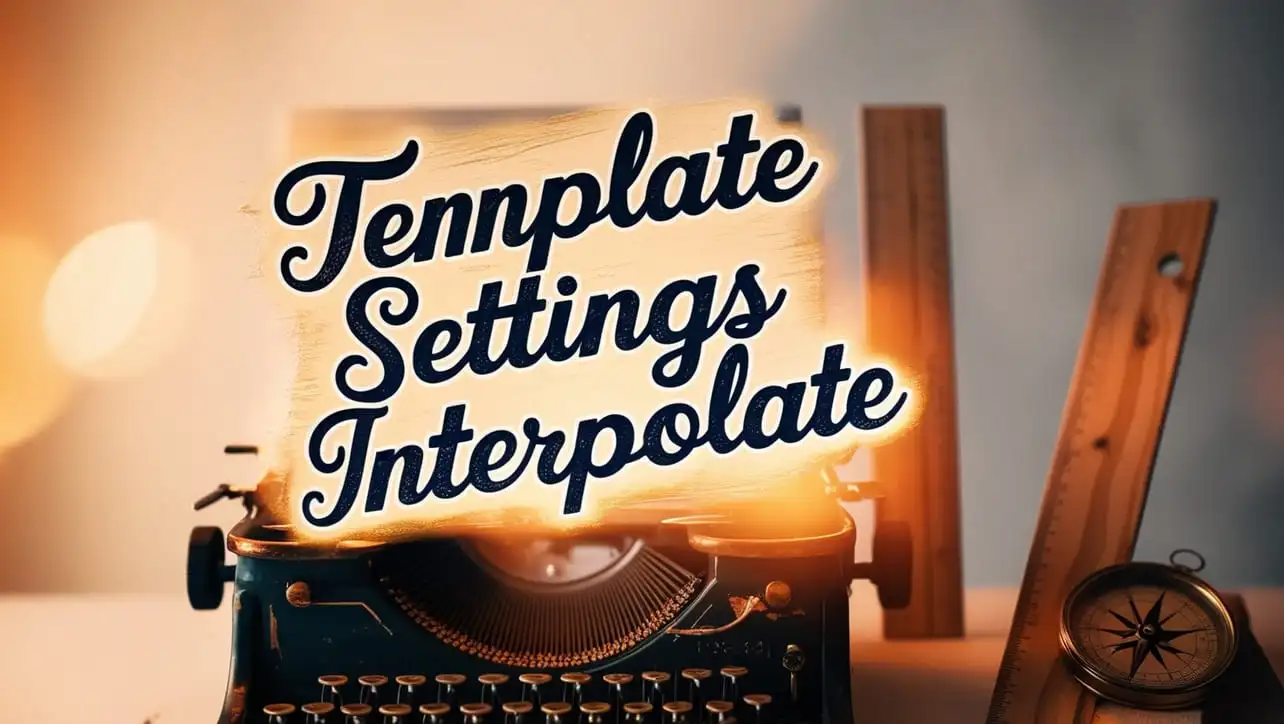
Lodash _.templateSettings.interpolate Property
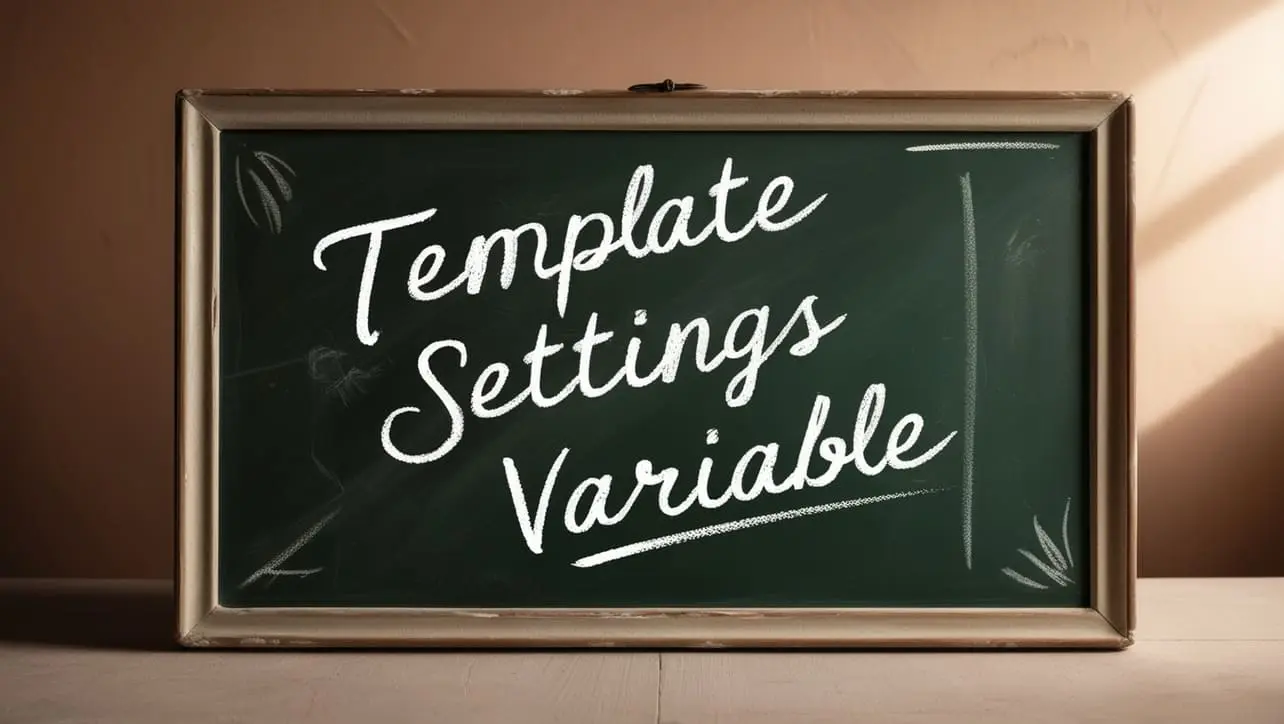
If you have any doubts regarding this article (Lodash _.dropRightWhile() Array Method), please comment here. I will help you immediately.